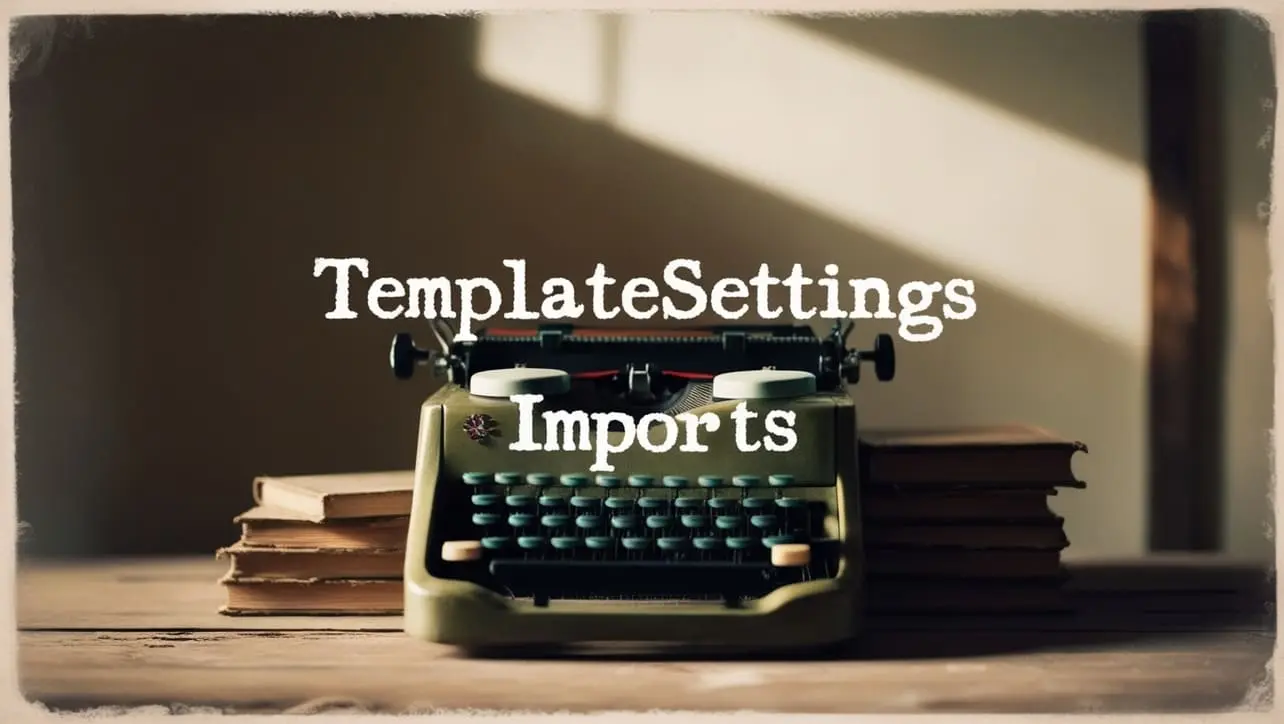
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.differenceWith() Array Method
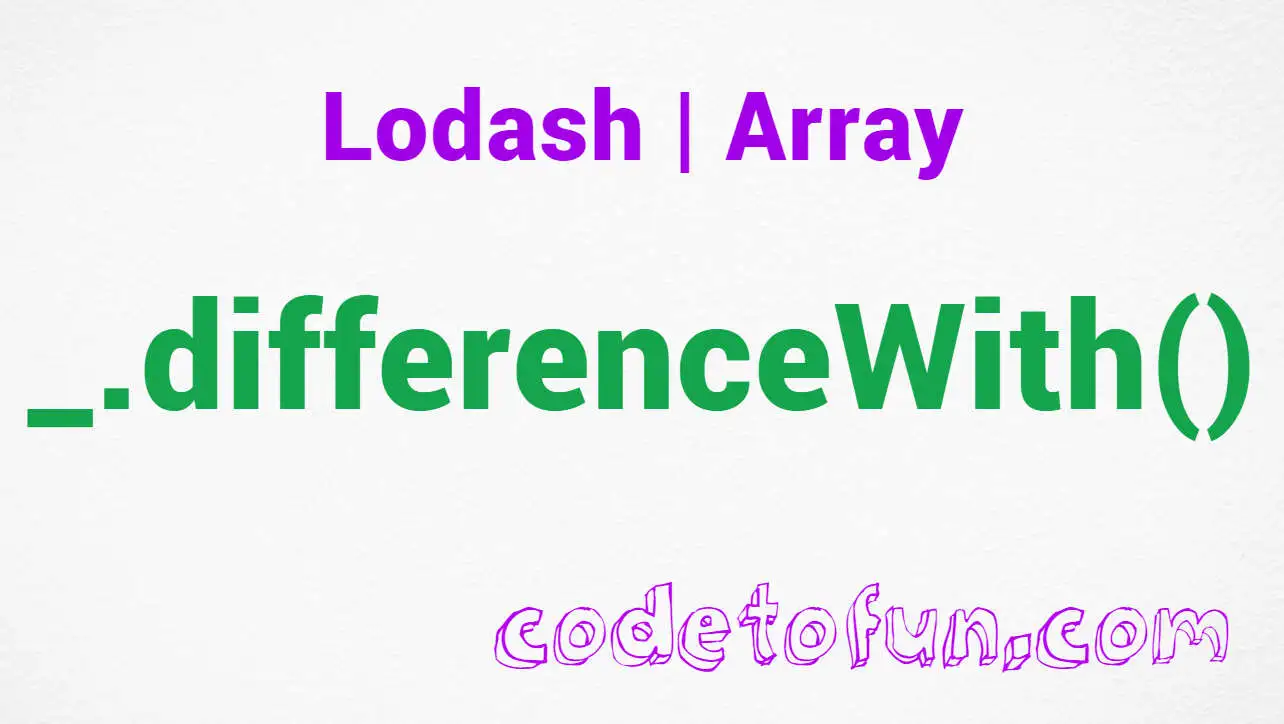
Photo Credit to CodeToFun
🙋 Introduction
When it comes to working with arrays in JavaScript, the Lodash library provides a rich set of utility functions, and one such powerful method is _.differenceWith()
.
This method allows developers to find the difference between two arrays based on a custom comparator function, providing a flexible and efficient solution for array comparison.
🧠 Understanding _.differenceWith()
The _.differenceWith()
method in Lodash is designed to find the difference between two arrays by using a custom comparator function to determine equality between elements. This method is particularly useful when you need to perform a specific comparison that goes beyond simple value matching.
💡 Syntax
_.differenceWith(array, values, [comparator])
- array: The array to inspect.
- values: The values to exclude.
- comparator (optional): The function to compare values.
📝 Example
Let's dive into an example to demonstrate the usage of _.differenceWith()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const array1 = [{ 'x': 1, 'y': 2 }, { 'x': 2, 'y': 1 }];
const array2 = [{ 'x': 1, 'y': 2 }];
const difference = _.differenceWith(array1, array2, _.isEqual);
console.log(difference);
// Output: [{ 'x': 2, 'y': 1 }]
In this example, the difference array contains elements from array1 that do not have a match in array2, based on the _.isEqual comparator function.
🏆 Best Practices
Understand Comparator Function:
Take time to understand the custom comparator function you provide. It should accurately reflect the logic for equality between elements. In the example above, _.isEqual is used for deep comparison.
Handle Edge Cases:
Consider edge cases, such as empty arrays or the absence of a comparator function. Implement appropriate checks and default behaviors to handle these scenarios gracefully.
handle-edge-cases.jsCopiedconst emptyArray = []; const result = _.differenceWith(emptyArray, array2, _.isEqual); // Returns: [] console.log(result);
Efficiency Considerations:
Be mindful of the efficiency of your comparator function, especially when working with large arrays. In some cases, optimizing the comparator function can significantly improve performance.
efficiency-considerations.jsCopiedconst optimizedComparator = (obj1, obj2) => { // ... optimized comparison logic ... }; const optimizedResult = _.differenceWith(array1, array2, optimizedComparator); console.log(optimizedResult);
📚 Use Cases
Filtering Unique Values:
Use
_.differenceWith()
to filter unique values from an array based on a custom comparison.filtering-unique-values.jsCopiedconst originalArray = /* ... fetch data ... */; const referenceArray = /* ... fetch reference data ... */; const uniqueValues = _.differenceWith(originalArray, referenceArray, _.isEqual); console.log(uniqueValues);
Custom Object Comparison:
When dealing with arrays of custom objects,
_.differenceWith()
enables you to customize the comparison logic.custom-object-comparison.jsCopiedconst productsInCart = /* ... fetch products in cart ... */; const productsToRemove = /* ... fetch products to remove ... */; const updatedCart = _.differenceWith(productsInCart, productsToRemove, (cartItem, itemToRemove) => { return cartItem.id === itemToRemove.id; }); console.log(updatedCart);
🎉 Conclusion
The _.differenceWith()
method in Lodash is a valuable tool for handling complex array comparison scenarios. Its flexibility allows developers to tailor the comparison logic to meet specific requirements, making it an essential addition to the array manipulation toolkit.
Explore the capabilities of _.differenceWith()
in Lodash and elevate your array comparison strategies to a new level of precision and customization!
👨💻 Join our Community:
Author
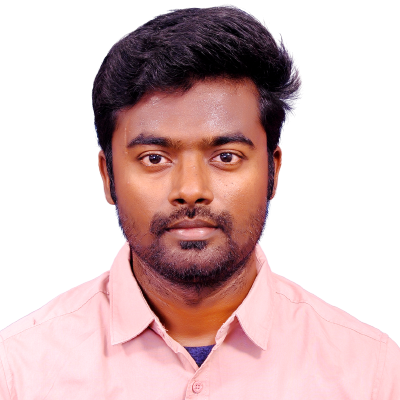
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
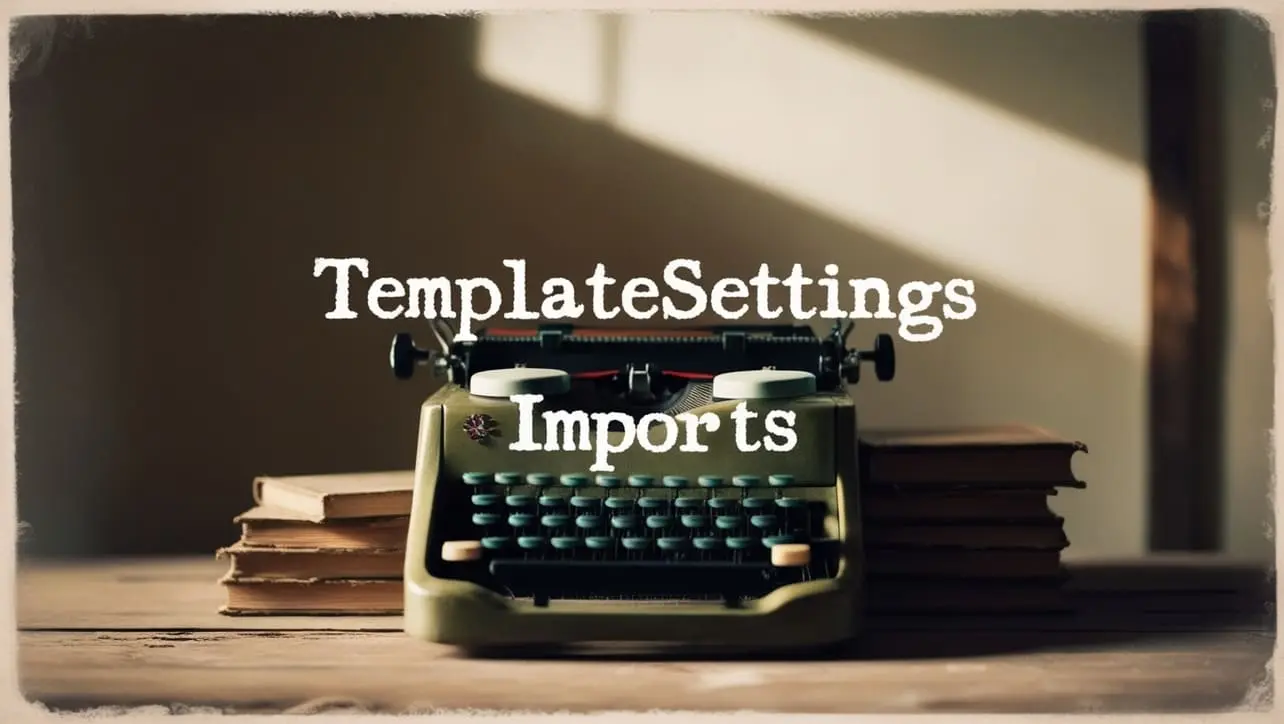
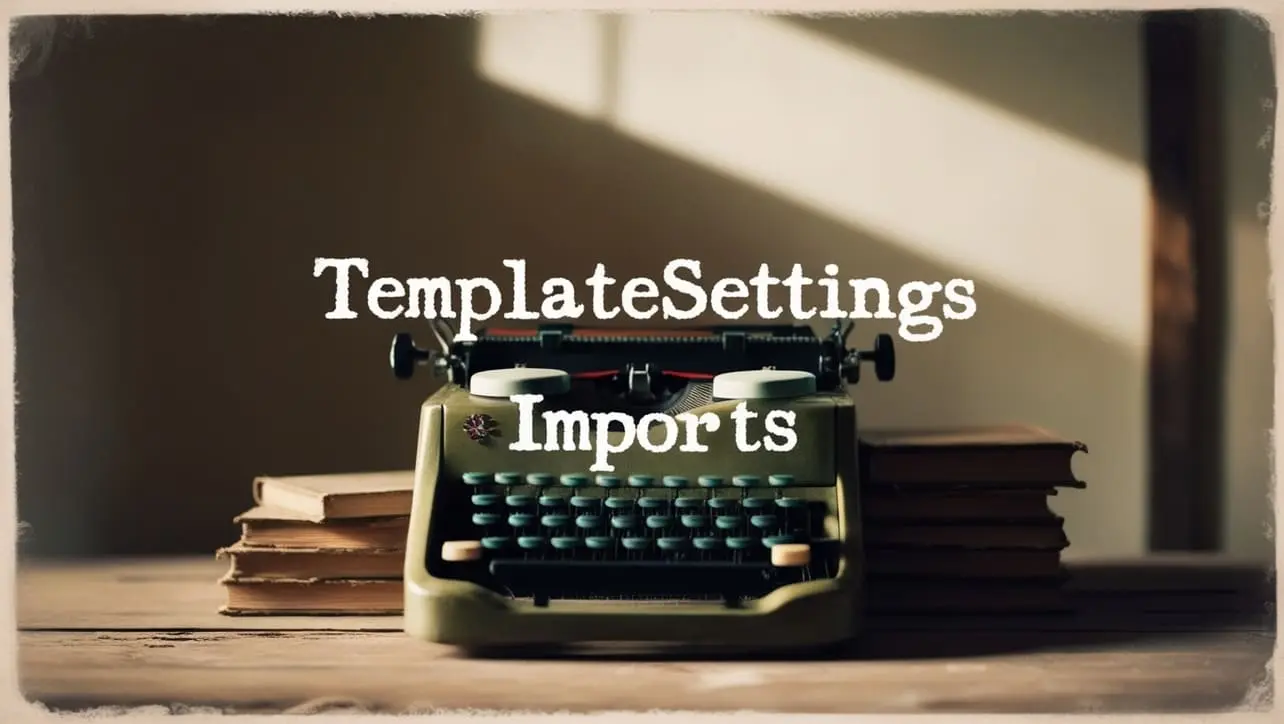
Lodash _.templateSettings.imports Property
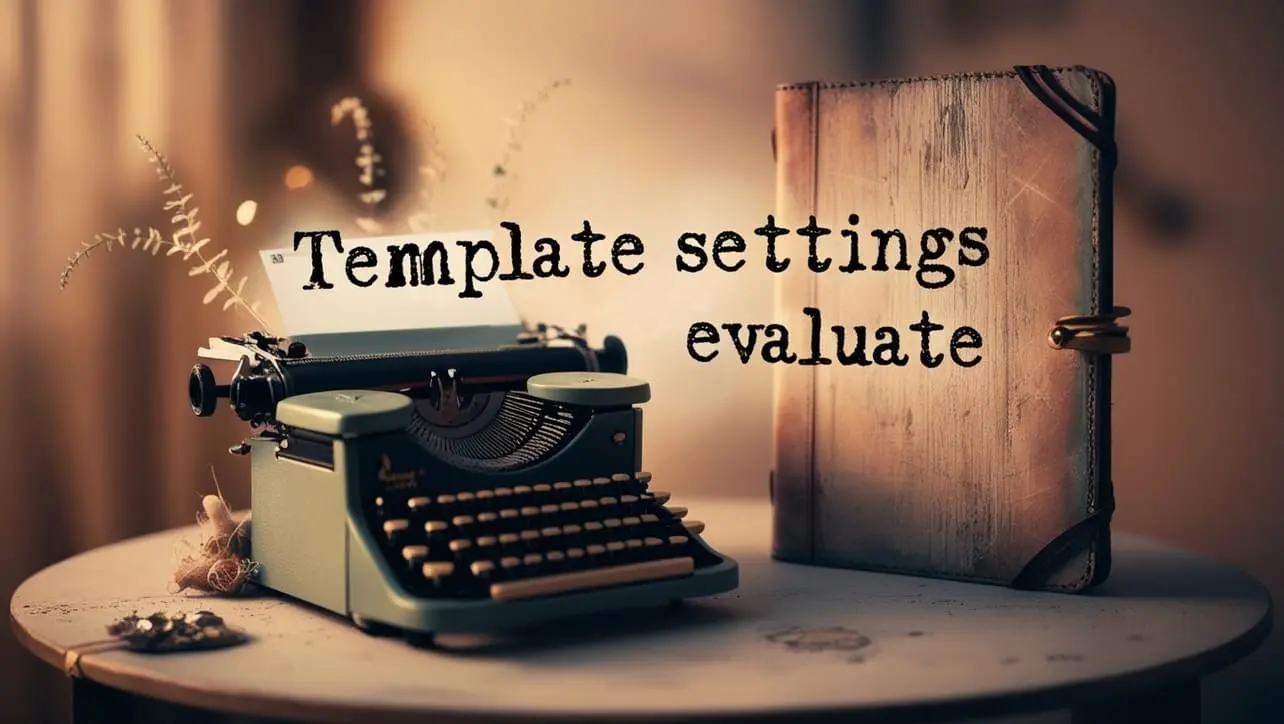
Lodash _.templateSettings.evaluate Property
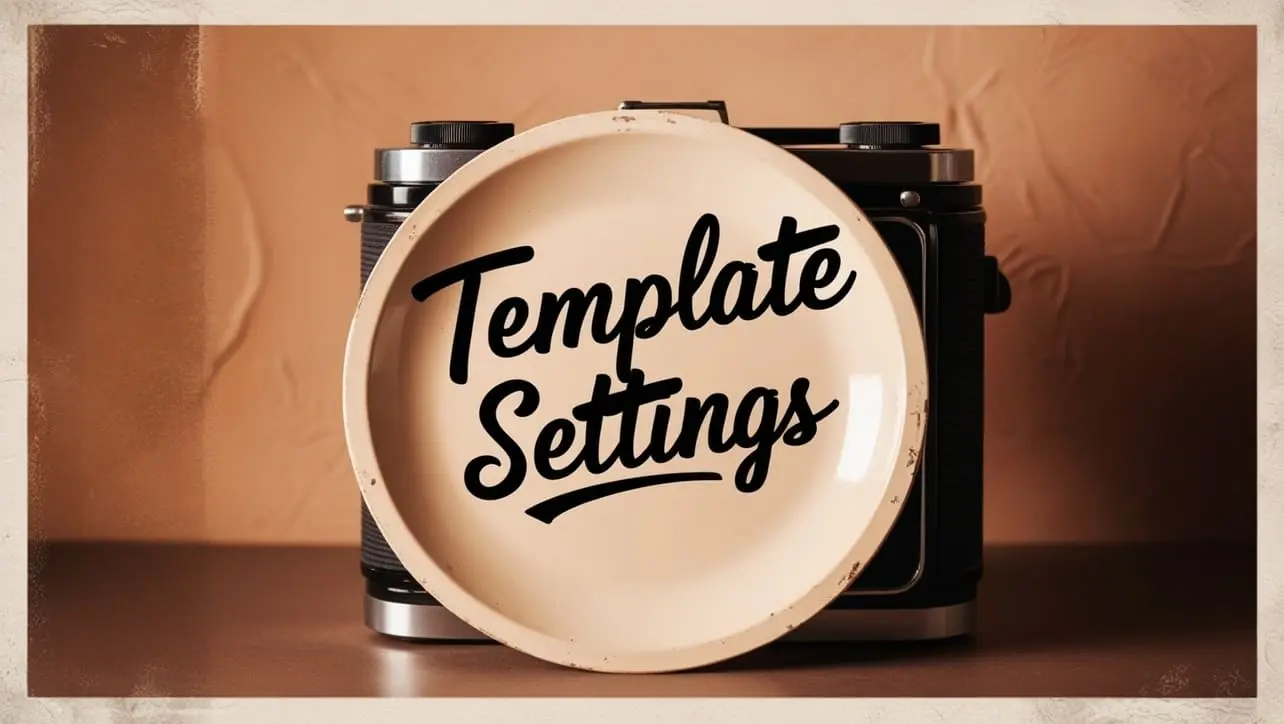
Lodash _.templateSettings Property
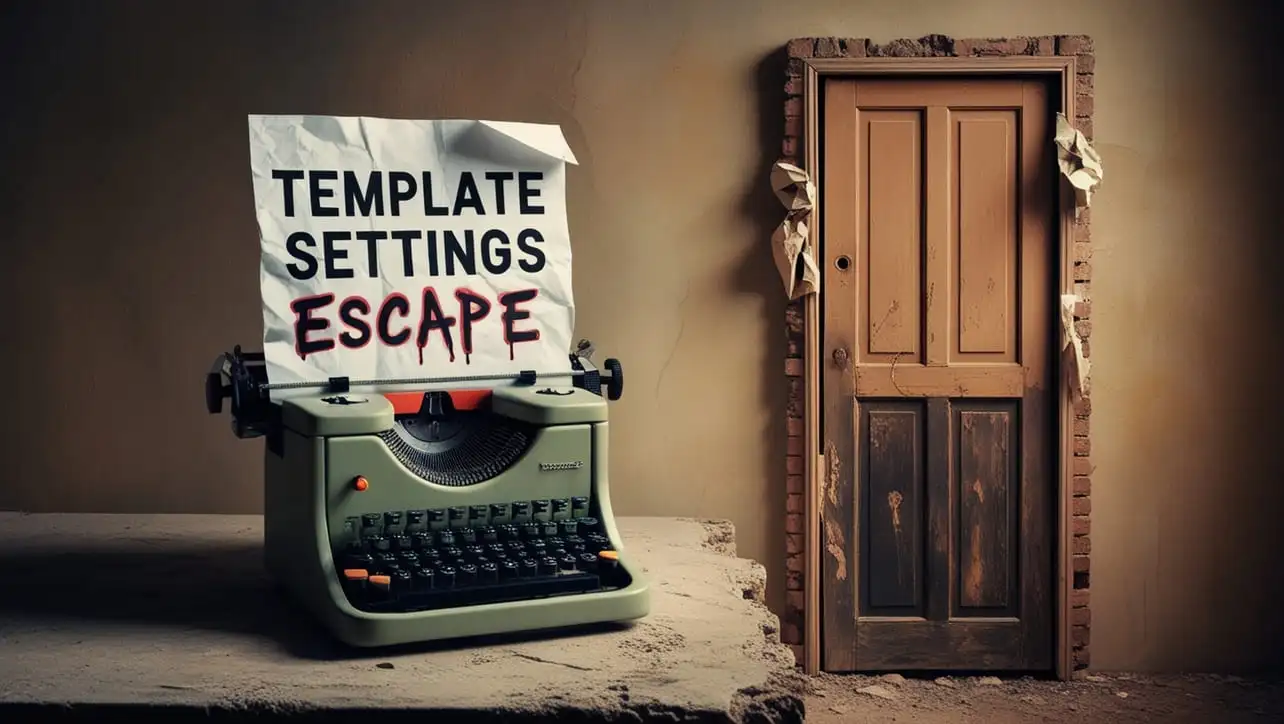
Lodash _.templateSettings.escape Property
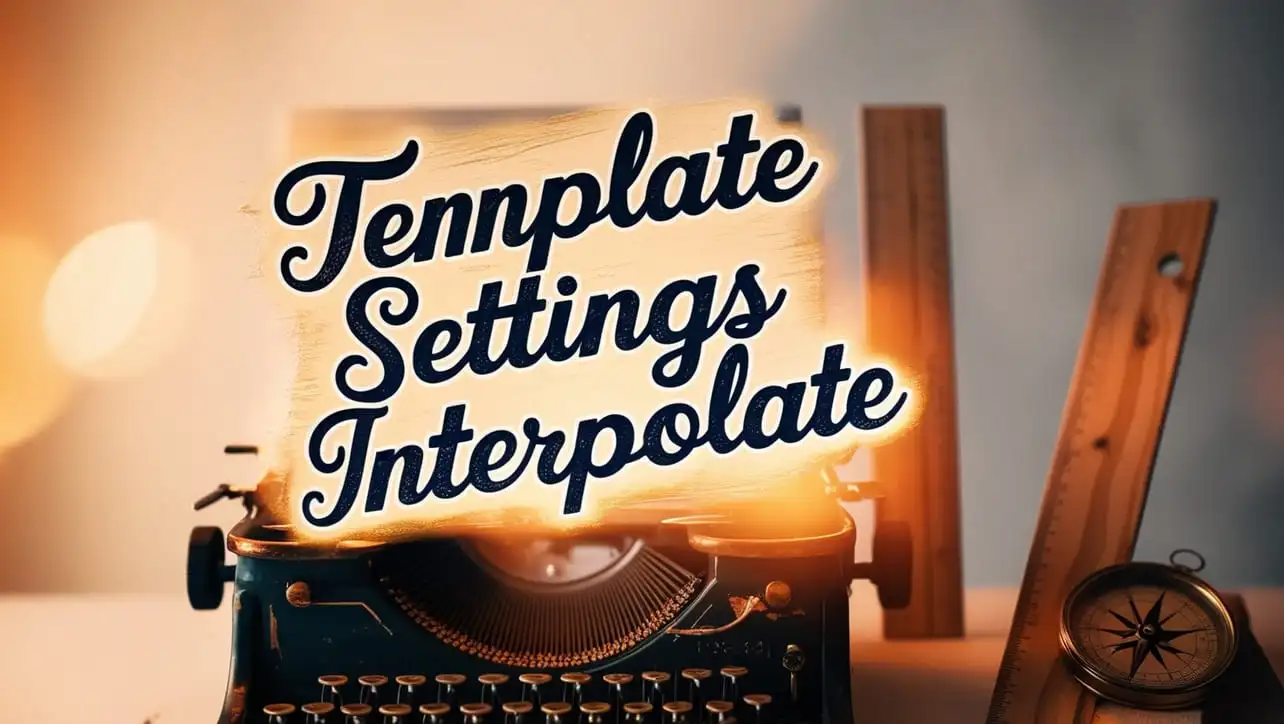
Lodash _.templateSettings.interpolate Property
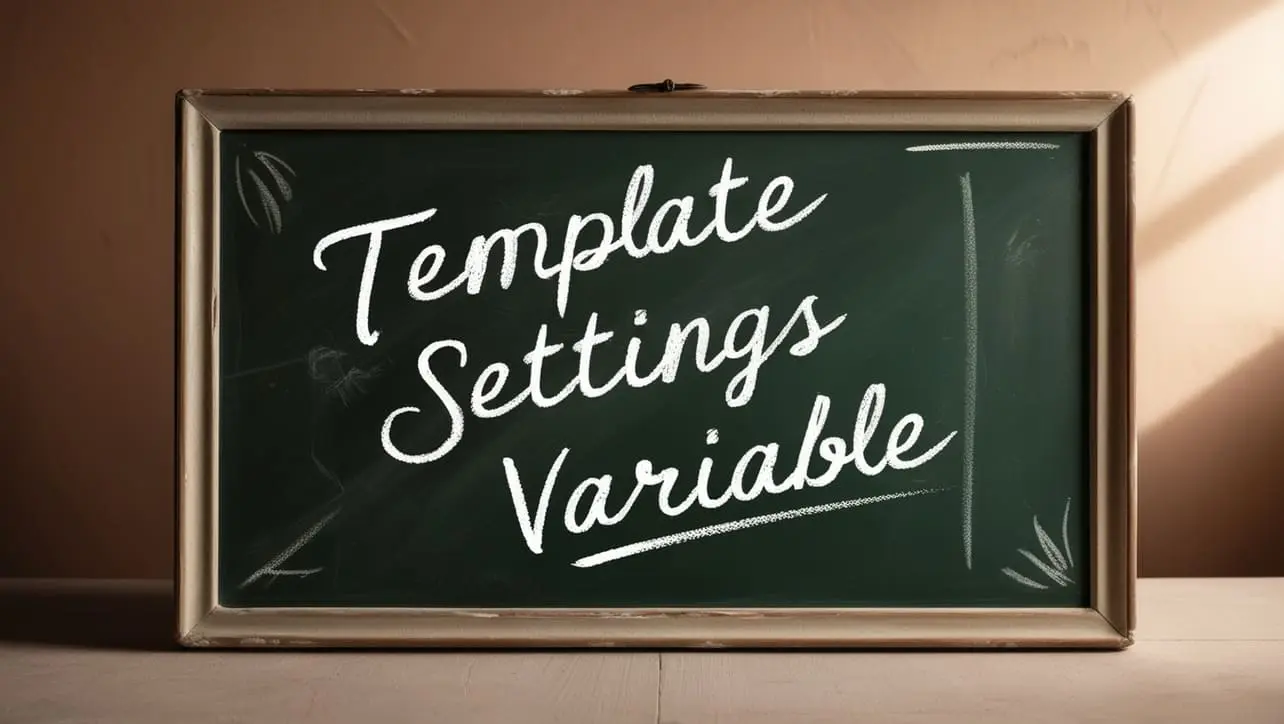
If you have any doubts regarding this article (Lodash _.differenceWith() Array Method), please comment here. I will help you immediately.