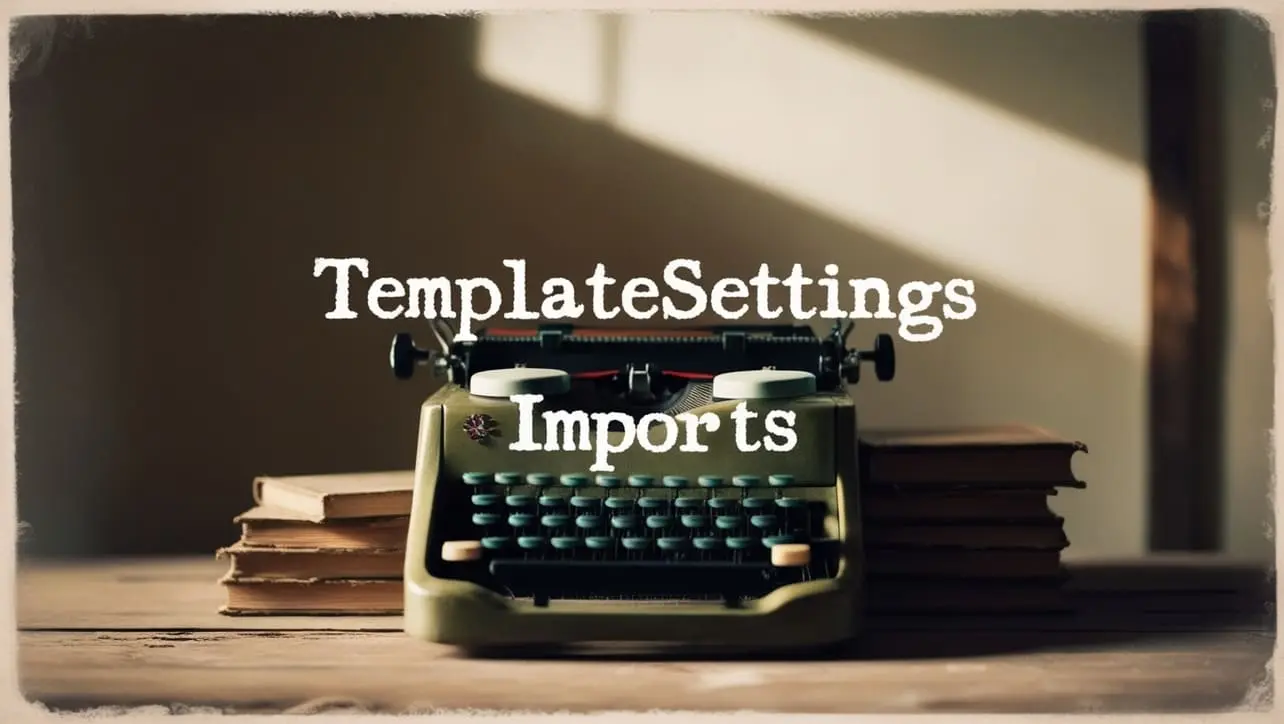
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.differenceBy() Array Method
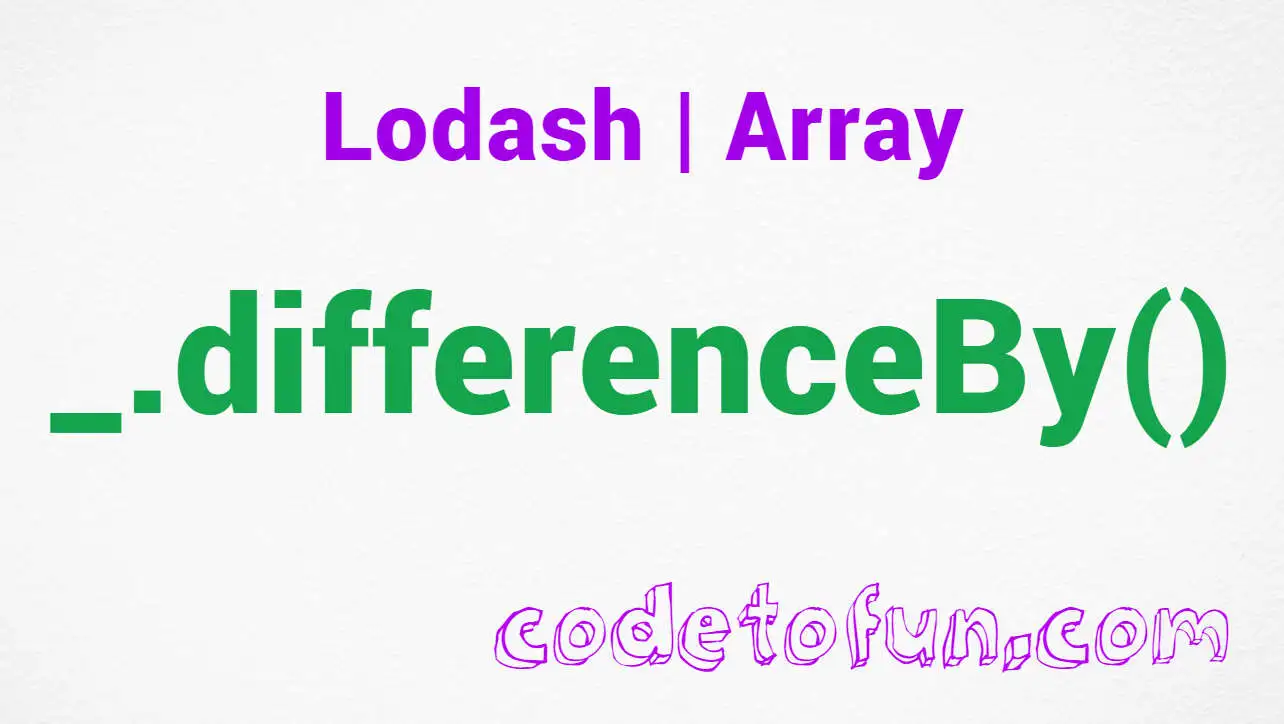
Photo Credit to CodeToFun
🙋 Introduction
Arrays in JavaScript often require sophisticated operations to compare and find differences. Lodash, a powerful utility library, provides the _.differenceBy()
method, which allows developers to find the difference between two arrays based on a specific property or criterion.
This method is particularly valuable when dealing with arrays of objects where a specific property defines uniqueness.
🧠 Understanding _.differenceBy()
The _.differenceBy()
method in Lodash compares two arrays and returns the difference based on a provided property or criteria. This enables developers to perform nuanced array comparisons, enhancing code flexibility and efficiency.
💡 Syntax
_.differenceBy(array, values, [iteratee=_.identity])
- array: The array to inspect.
- values: The values to exclude.
- iteratee: The iteratee invoked per element (default is _.identity).
📝 Example
Let's delve into a practical example to illustrate the usage of _.differenceBy()
:
const _ = require('lodash');
const array1 = [{ 'x': 1 }, { 'x': 2 }, { 'x': 3 }];
const array2 = [{ 'x': 2 }];
const differenceArray = _.differenceBy(array1, array2, 'x');
console.log(differenceArray);
// Output: [{ 'x': 1 }, { 'x': 3 }]
In this example, _.differenceBy()
is used to find the elements in array1 that are not present in array2 based on the 'x' property.
🏆 Best Practices
Validate Inputs:
Before using
_.differenceBy()
, ensure that both input arrays are valid and contain elements. Additionally, verify that the iteratee function produces meaningful results.validate-inputs.jsCopiedif (!Array.isArray(array1) || !Array.isArray(array2) || array1.length === 0 || array2.length === 0) { console.error('Invalid input arrays'); return; } const validatedDifferenceArray = _.differenceBy(array1, array2, 'x'); console.log(validatedDifferenceArray);
Handle Edge Cases:
Consider edge cases, such as empty arrays or arrays with complex objects where the iteratee may return unexpected results. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst emptyArray1 = []; const emptyArray2 = []; const complexObjectsArray = [{ 'x': { 'y': 1 } }, { 'x': { 'y': 2 } }]; const edgeCaseDifference1 = _.differenceBy(emptyArray1, emptyArray2, 'x'); // Returns: [] const edgeCaseDifference2 = _.differenceBy(complexObjectsArray, [], 'x'); // Returns: [{ 'x': { 'y': 1 } }, { 'x': { 'y': 2 } }] console.log(edgeCaseDifference1); console.log(edgeCaseDifference2);
Customize Iteratee Function:
Experiment with different iteratee functions based on your specific use case. Customizing the iteratee function allows you to compare elements based on specific criteria.
customize-iteratee-function.jsCopiedconst customIteratee = obj => obj.x + 1; const customDifferenceArray = _.differenceBy(array1, array2, customIteratee); console.log(customDifferenceArray);
📚 Use Cases
Removing Duplicates:
_.differenceBy()
can be employed to remove duplicate elements from an array based on a specific property.removing-duplicates.jsCopiedconst arrayWithDuplicates = [{ 'x': 1 }, { 'x': 2 }, { 'x': 1 }]; const uniqueArray = _.differenceBy(arrayWithDuplicates, [], 'x'); console.log(uniqueArray); // Output: [{ 'x': 1 }, { 'x': 2 }]
Filtering Based on Criteria:
Filtering arrays based on specific criteria becomes seamless with
_.differenceBy()
. Identify and extract elements that do not meet certain conditions.filtering-based-on-criteria.jsCopiedconst products = [{ 'id': 1, 'price': 20 }, { 'id': 2, 'price': 30 }, { 'id': 3, 'price': 20 }]; const discountedProducts = [{ 'id': 1, 'price': 20 }]; const nonDiscountedProducts = _.differenceBy(products, discountedProducts, 'id'); console.log(nonDiscountedProducts); // Output: [{ 'id': 2, 'price': 30 }, { 'id': 3, 'price': 20 }]
Data Synchronization:
In scenarios where you have two arrays representing different versions of data,
_.differenceBy()
aids in synchronizing and identifying changes.data-synchronization.jsCopiedconst oldData = [{ 'id': 1, 'value': 'A' }, { 'id': 2, 'value': 'B' }]; const newData = [{ 'id': 2, 'value': 'C' }, { 'id': 3, 'value': 'D' }]; const changedData = _.differenceBy(newData, oldData, 'id'); console.log(changedData); // Output: [{ 'id': 3, 'value': 'D' }]
🎉 Conclusion
The _.differenceBy()
method in Lodash is a valuable asset for developers working with arrays of complex objects. Its ability to find differences based on specific properties or criteria simplifies array manipulation and enhances code efficiency. By incorporating this method into your projects, you can streamline tasks involving array comparison and focus on building robust JavaScript applications.
Unlock the power of array manipulation with Lodash and the _.differenceBy()
method!
👨💻 Join our Community:
Author
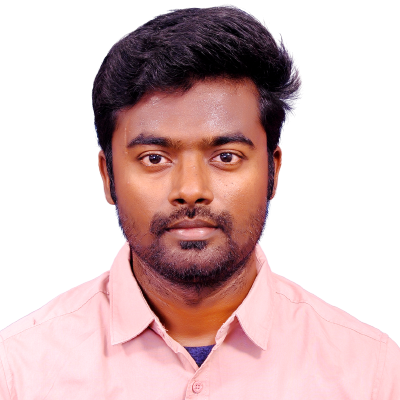
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
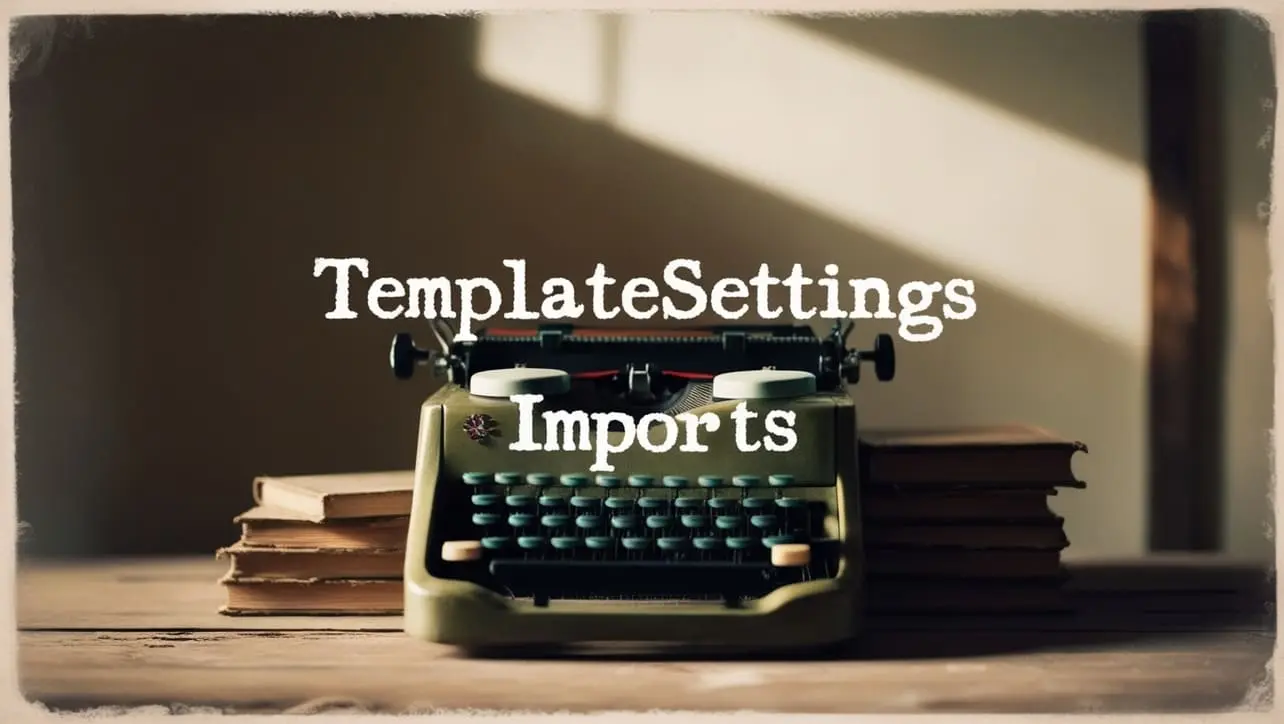
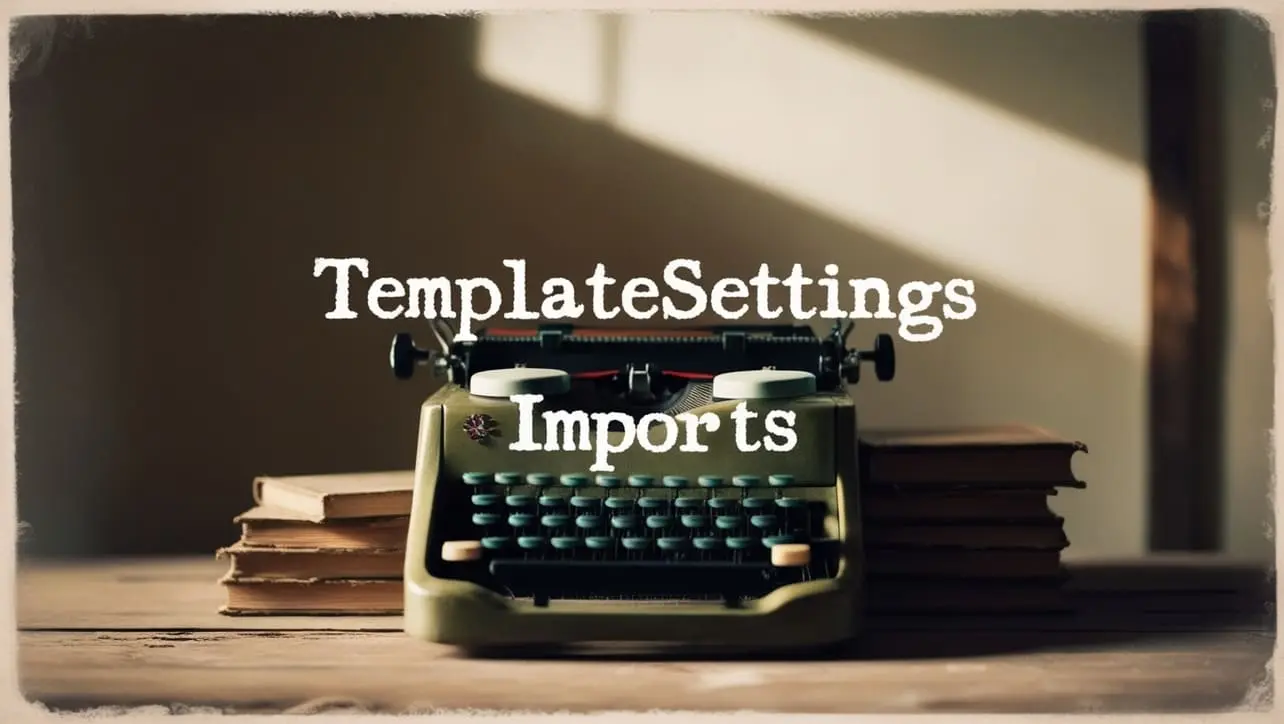
Lodash _.templateSettings.imports Property
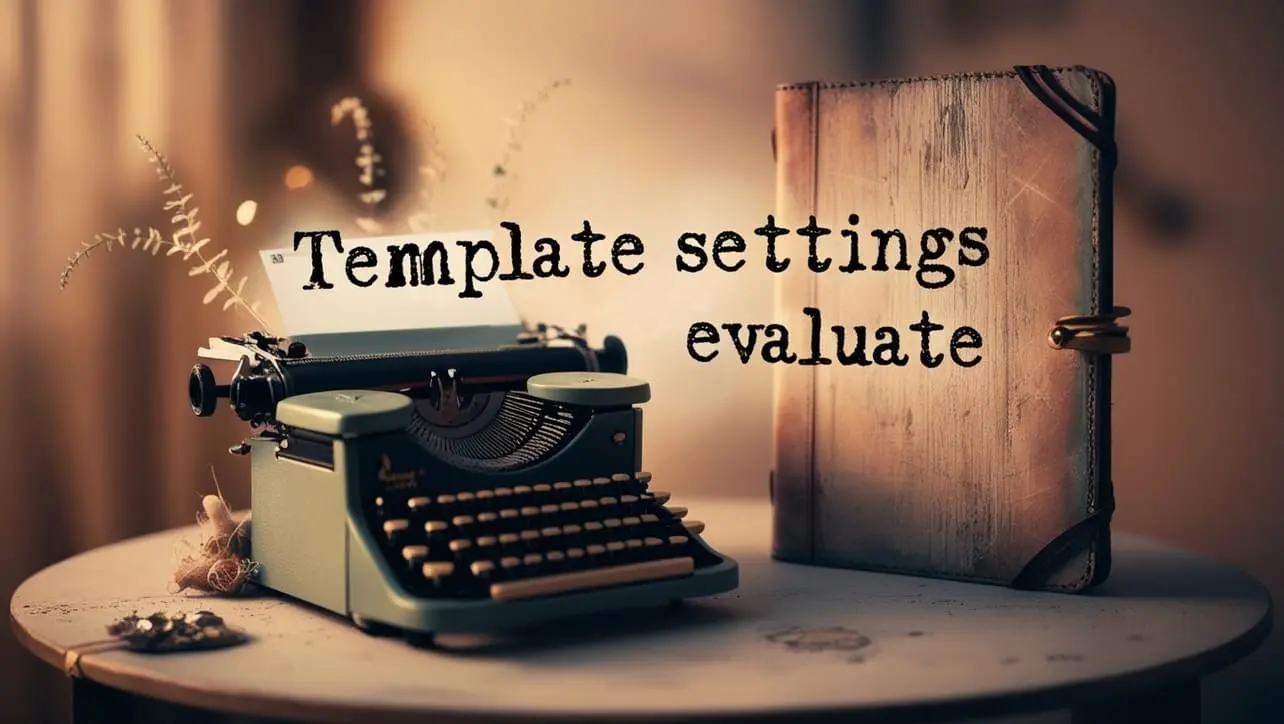
Lodash _.templateSettings.evaluate Property
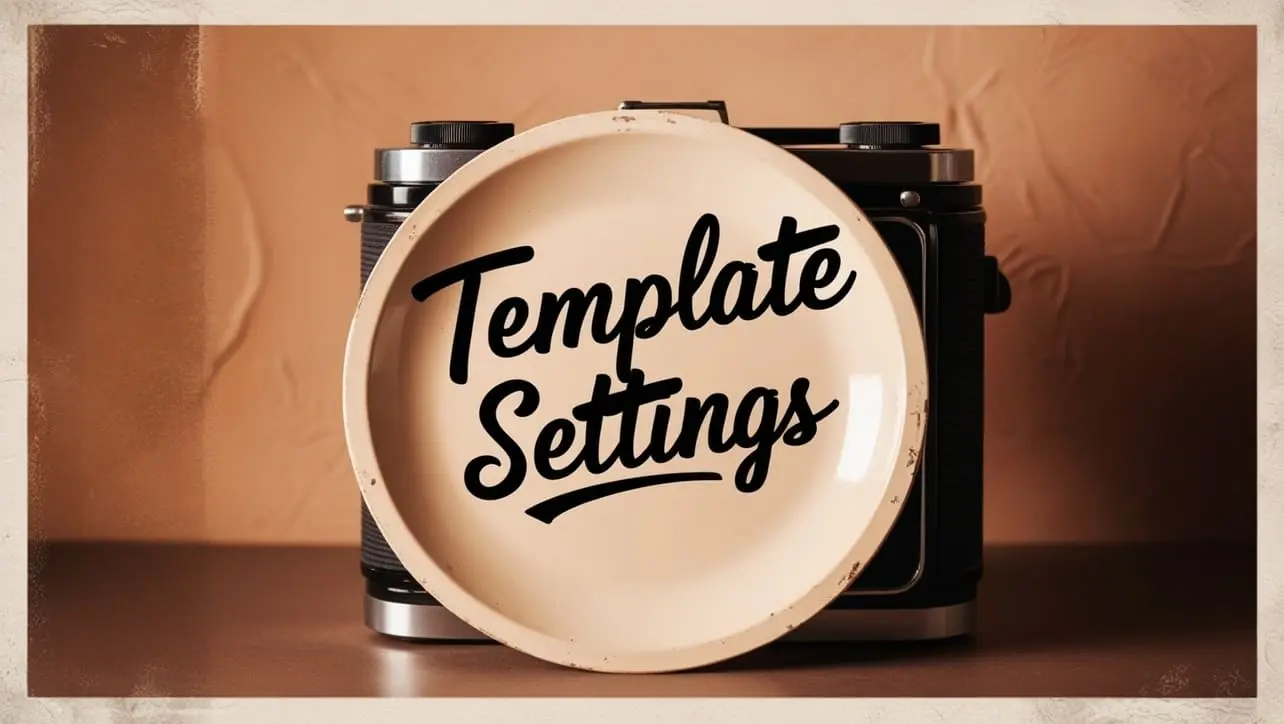
Lodash _.templateSettings Property
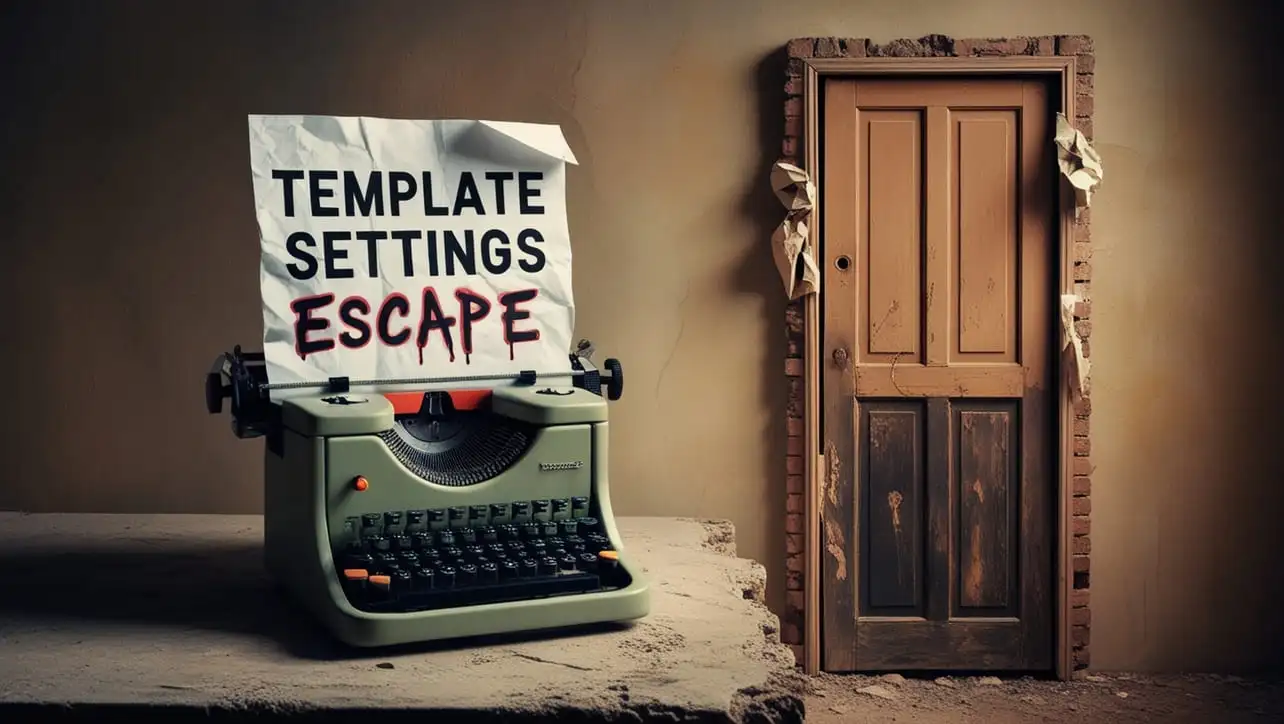
Lodash _.templateSettings.escape Property
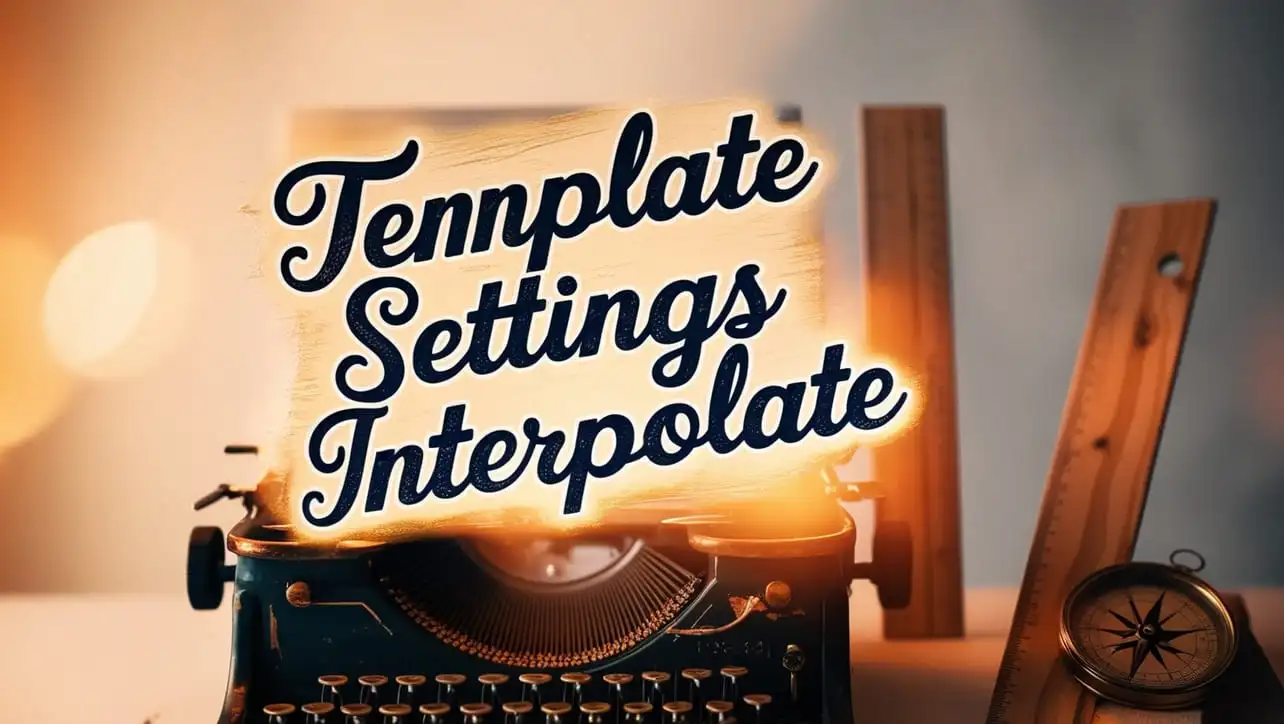
Lodash _.templateSettings.interpolate Property
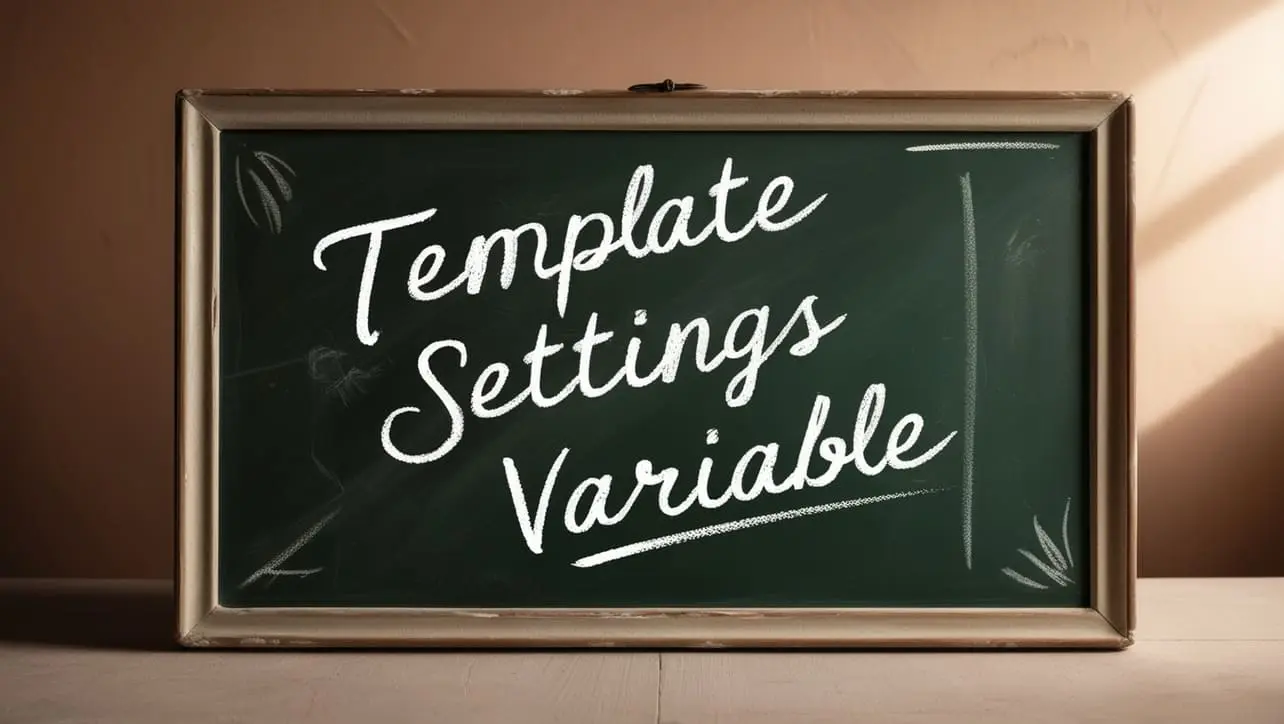
If you have any doubts regarding this article (Lodash _.differenceBy() Array Method), please comment here. I will help you immediately.