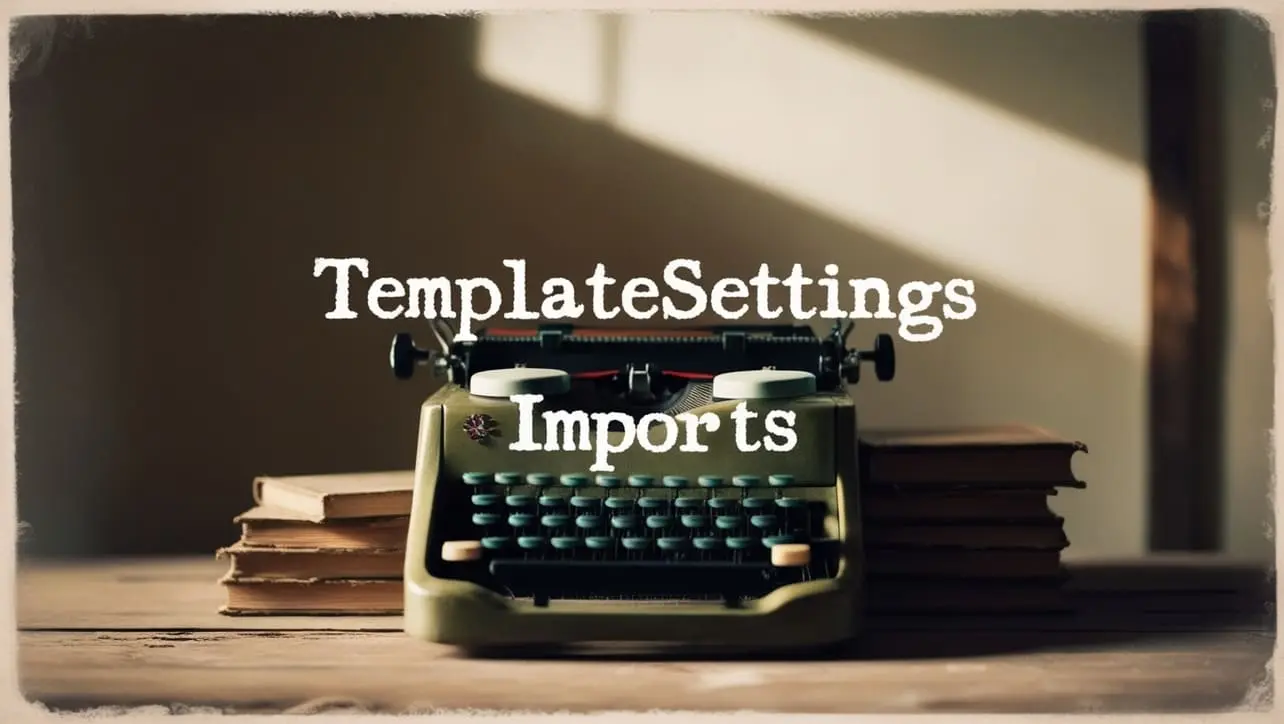
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.chunk() Array Method
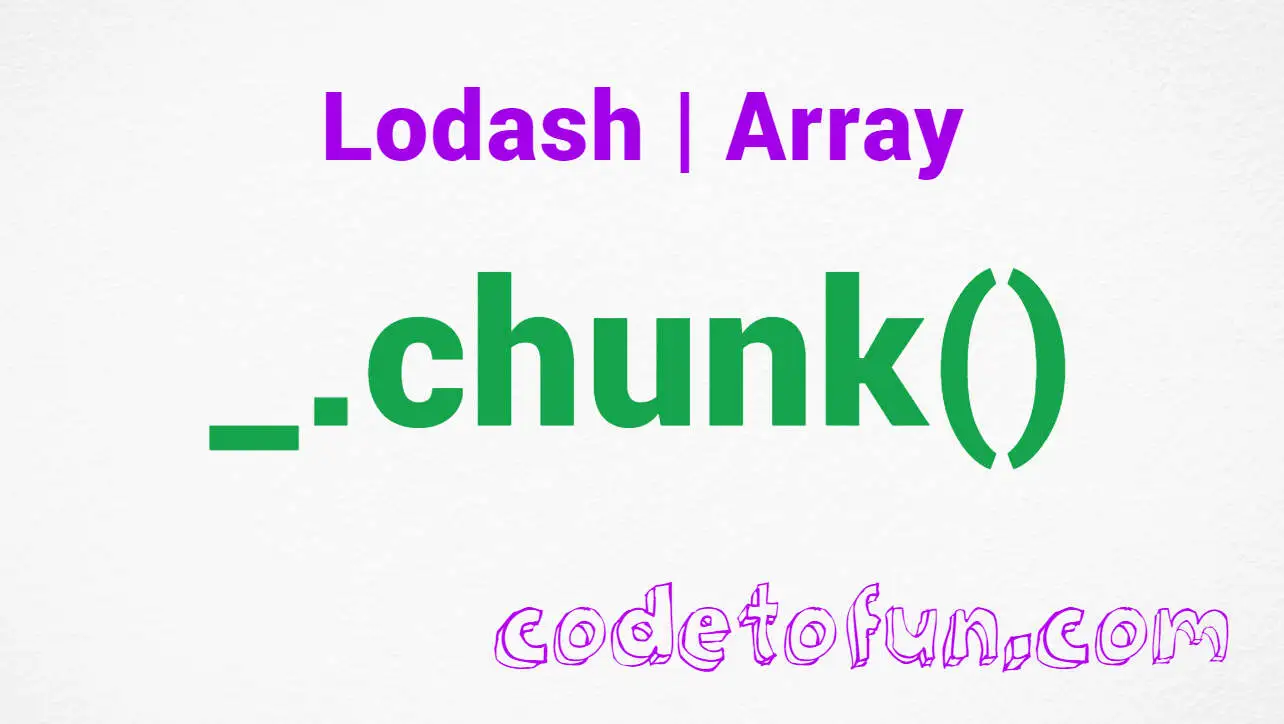
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript programming, efficient manipulation of arrays is crucial. The Lodash library offers a plethora of utility functions, and one such gem is the _.chunk()
method.
This method simplifies the process of breaking down an array into smaller chunks, making it a valuable tool for developers dealing with large datasets or wanting to enhance code readability.
🧠 Understanding _.chunk()
The _.chunk()
method in Lodash allows you to split an array into chunks of a specified size. This can be particularly useful when you need to process or display data in manageable portions, improving performance and user experience.
💡 Syntax
_.chunk(array, [size=1])
- array: The array to process.
- size: The size of each chunk (default is 1).
📝 Example
Let's dive into a practical example to illustrate the power of _.chunk()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const chunkedArray = _.chunk(originalArray, 3);
console.log(chunkedArray);
// Output: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
In this example, the originalArray is divided into chunks of size 3, creating a new array with subarrays.
🏆 Best Practices
Validate Inputs:
Before using
_.chunk()
, ensure that the input array is valid and contains elements. Additionally, validate the chunk size to avoid unexpected behavior.validate-inputs.jsCopiedif (!Array.isArray(originalArray) || originalArray.length === 0) { console.error('Invalid input array'); return; } const chunkSize = 3; // Set your desired chunk size if (chunkSize <= 0) { console.error('Invalid chunk size'); return; } const validatedChunkedArray = _.chunk(originalArray, chunkSize); console.log(validatedChunkedArray);
Handle Edge Cases:
Consider edge cases, such as an empty array or a chunk size larger than the array length. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst emptyArray = []; const largeChunkSize = 10; const emptyArrayChunks = _.chunk(emptyArray, 3); // Returns: [] const largeChunkSizeChunks = _.chunk(originalArray, largeChunkSize); // Returns: [[1, 2, 3, 4, 5, 6, 7, 8, 9]] console.log(emptyArrayChunks); console.log(largeChunkSizeChunks);
Optimal Chunk Size:
Experiment with different chunk sizes based on your specific use case. Finding the optimal chunk size can significantly impact performance.
optimal-chunk-size.jsCopiedconst experimentalChunkSize = 5; const experimentalChunks = _.chunk(originalArray, experimentalChunkSize); console.log(experimentalChunks);
📚 Use Cases
Pagination:
When dealing with paginated displays,
_.chunk()
can be employed to divide your data into pages, simplifying navigation and enhancing user experience.pagination.jsCopiedconst dataForPagination = /* ...fetch data from API or elsewhere... */; const pageSize = 10; const paginatedData = _.chunk(dataForPagination, pageSize); console.log(paginatedData);
Batch Processing:
For scenarios where you need to process data in batches, such as making API calls or database operations,
_.chunk()
can streamline the workflow.batch-processing.jsCopiedconst dataForBatchProcessing = /* ...fetch data from API or elsewhere... */; const batchSize = 50; const batchedData = _.chunk(dataForBatchProcessing, batchSize); console.log(batchedData);
Parallel Execution:
In parallel computing, breaking down a large dataset into chunks enables parallel execution of tasks, optimizing performance.
parallel-execution.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; const parallelTasks = []; _.chunk(largeDataset, 100).forEach(chunk => { parallelTasks.push(/* ...create tasks based on each chunk... */); }); // Execute tasks in parallel (use your preferred method or library for parallel execution) Promise.all(parallelTasks) .then(results => { console.log(results); }) .catch(error => { console.error(error); });
🎉 Conclusion
The _.chunk()
method in Lodash is a valuable tool for any JavaScript developer working with arrays. Its simplicity and versatility make it an excellent choice for tasks involving data segmentation and manipulation. By incorporating this method into your code, you can enhance both the efficiency and readability of your projects.
Explore the world of Lodash and unlock the potential of array manipulation with _.chunk()
!
👨💻 Join our Community:
Author
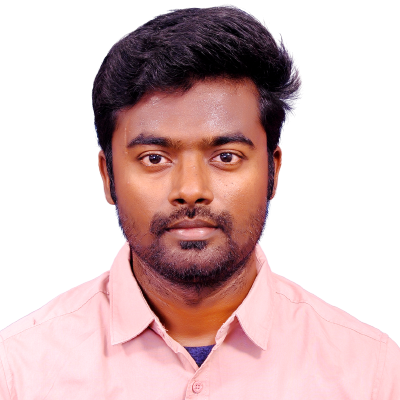
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
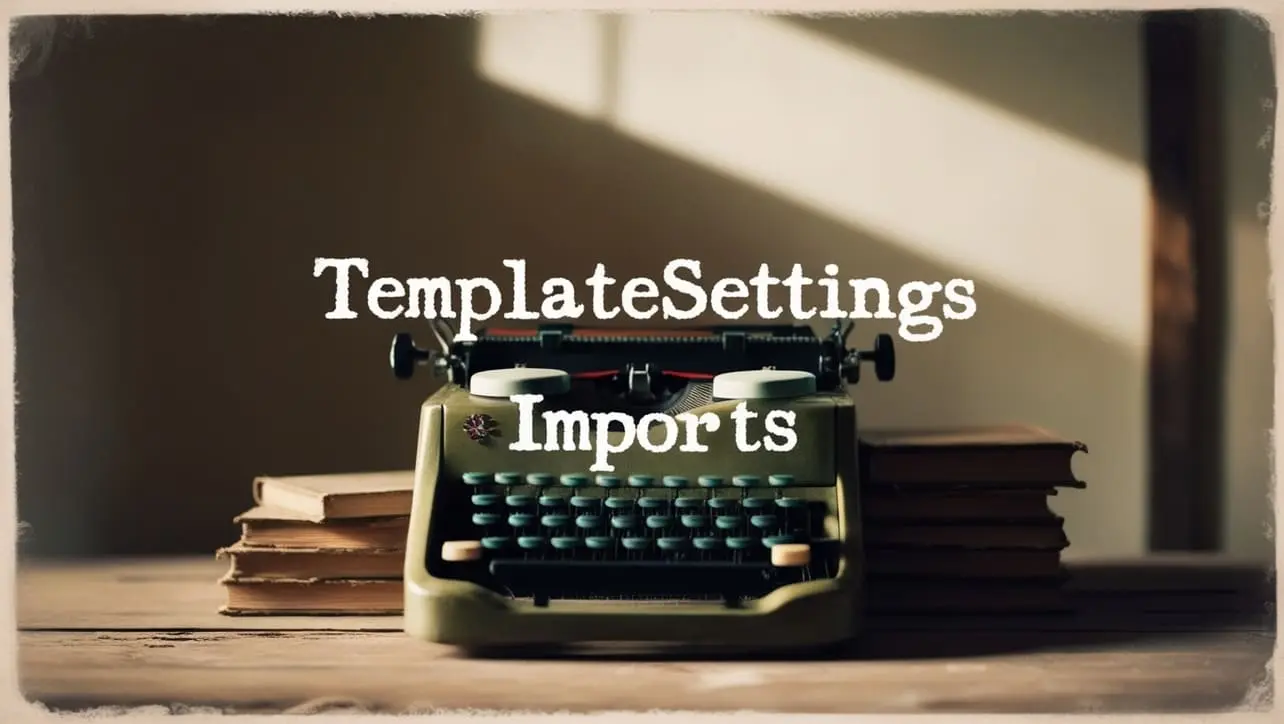
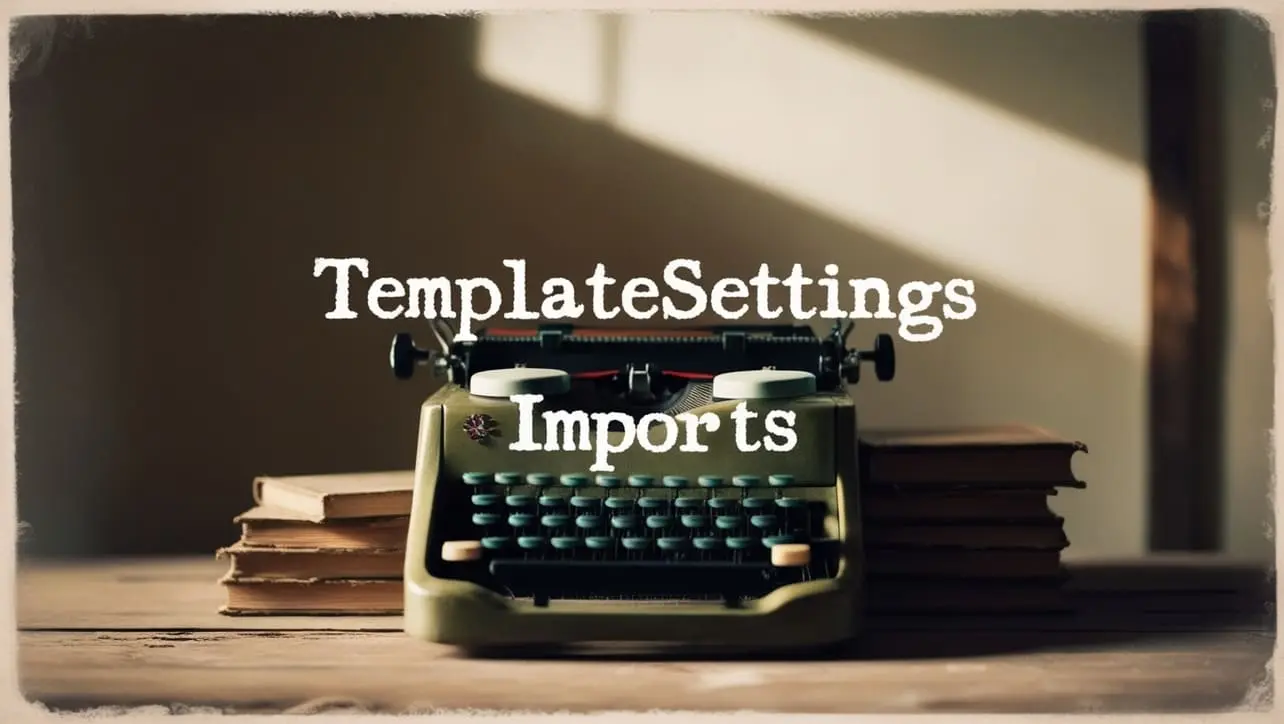
Lodash _.templateSettings.imports Property
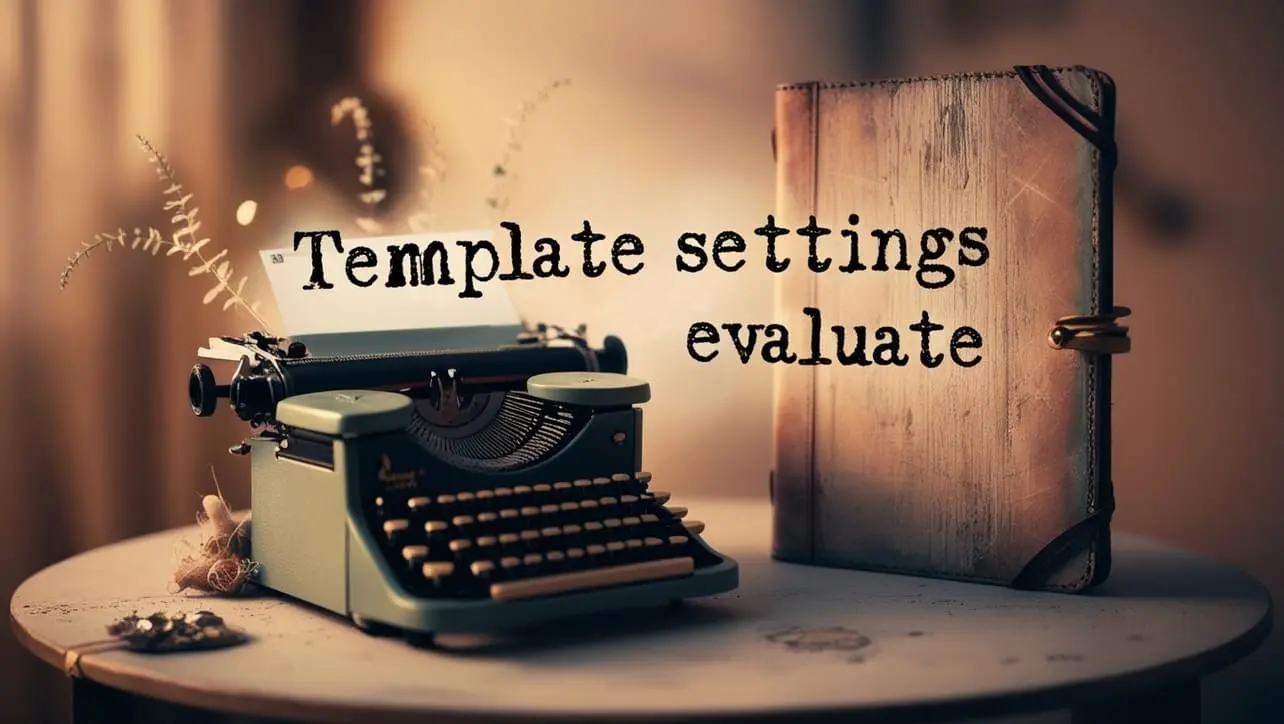
Lodash _.templateSettings.evaluate Property
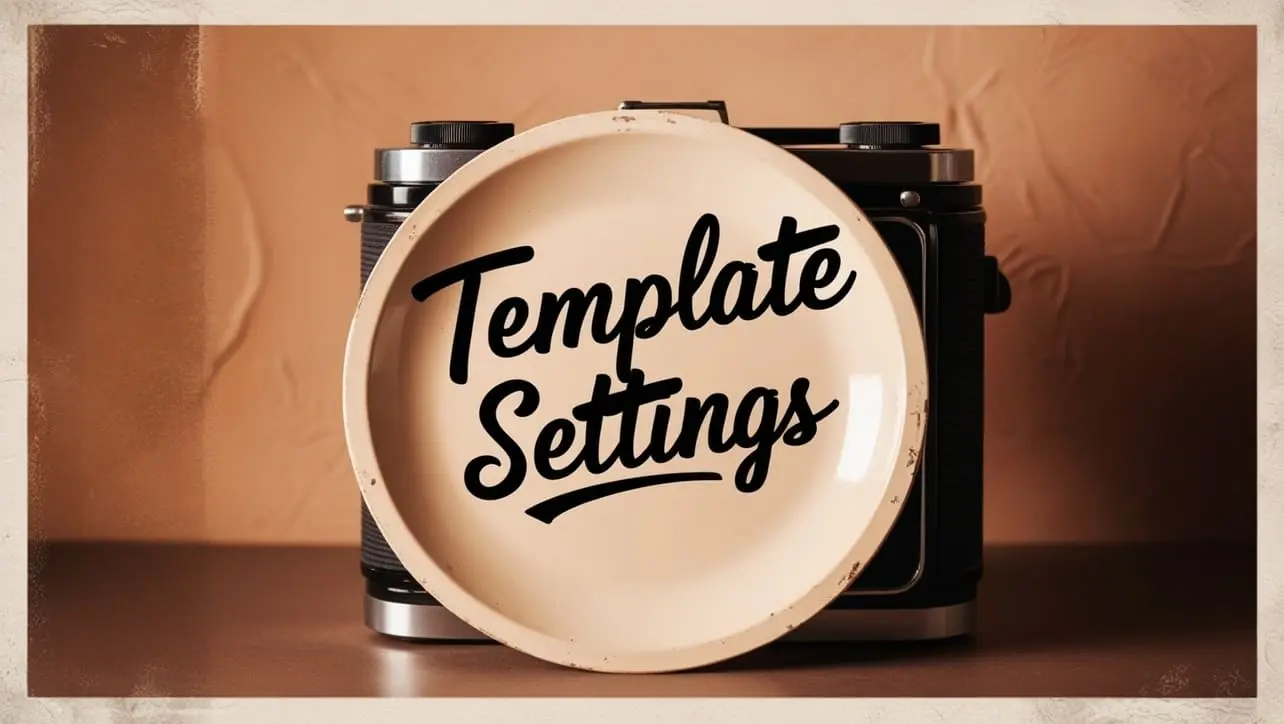
Lodash _.templateSettings Property
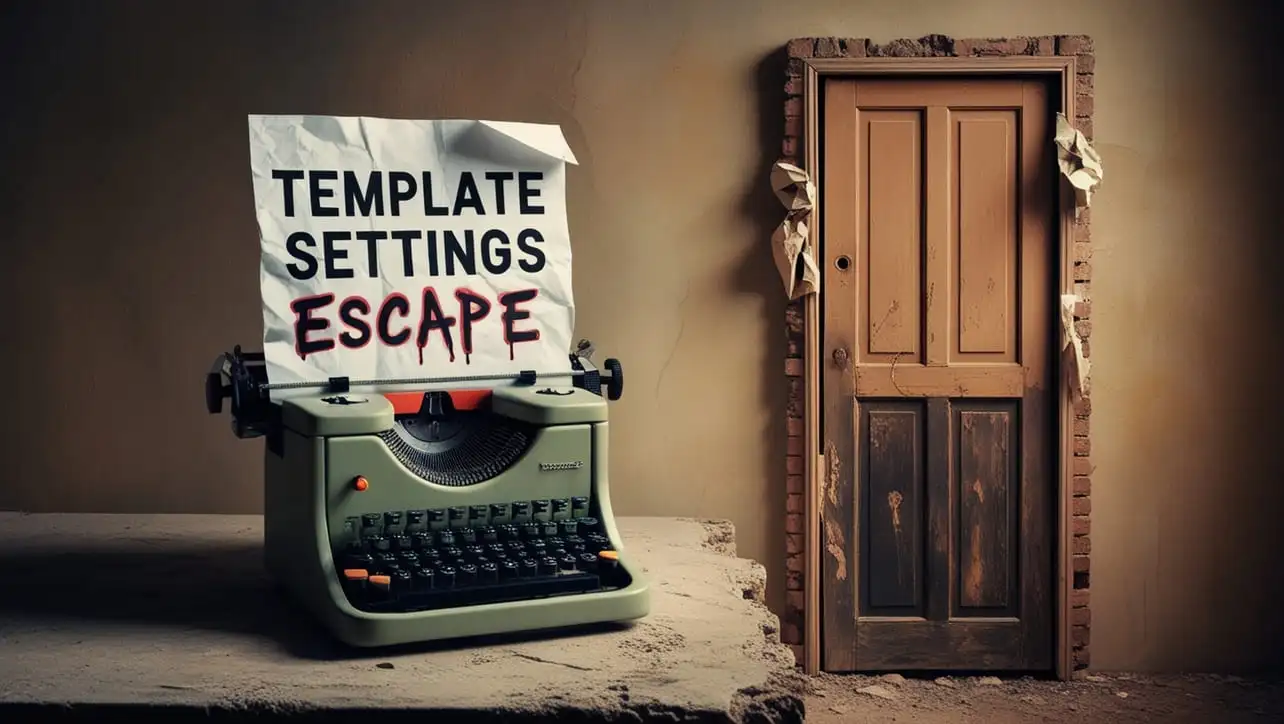
Lodash _.templateSettings.escape Property
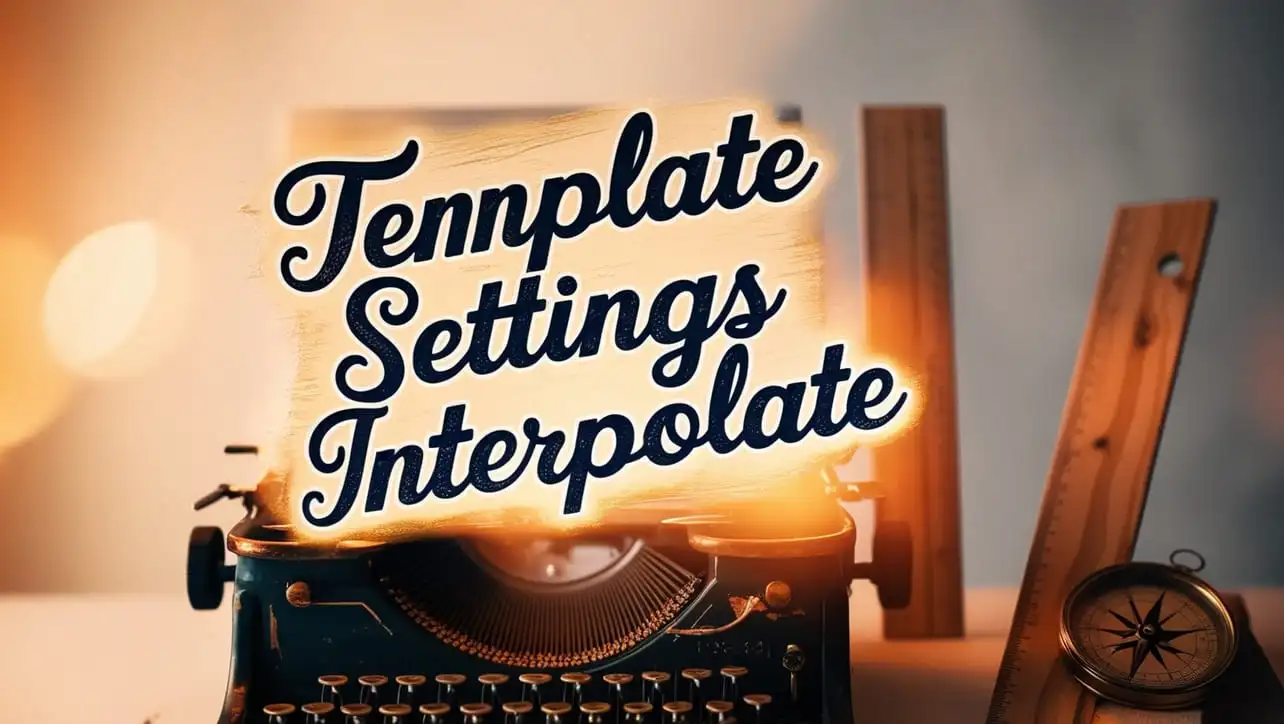
Lodash _.templateSettings.interpolate Property
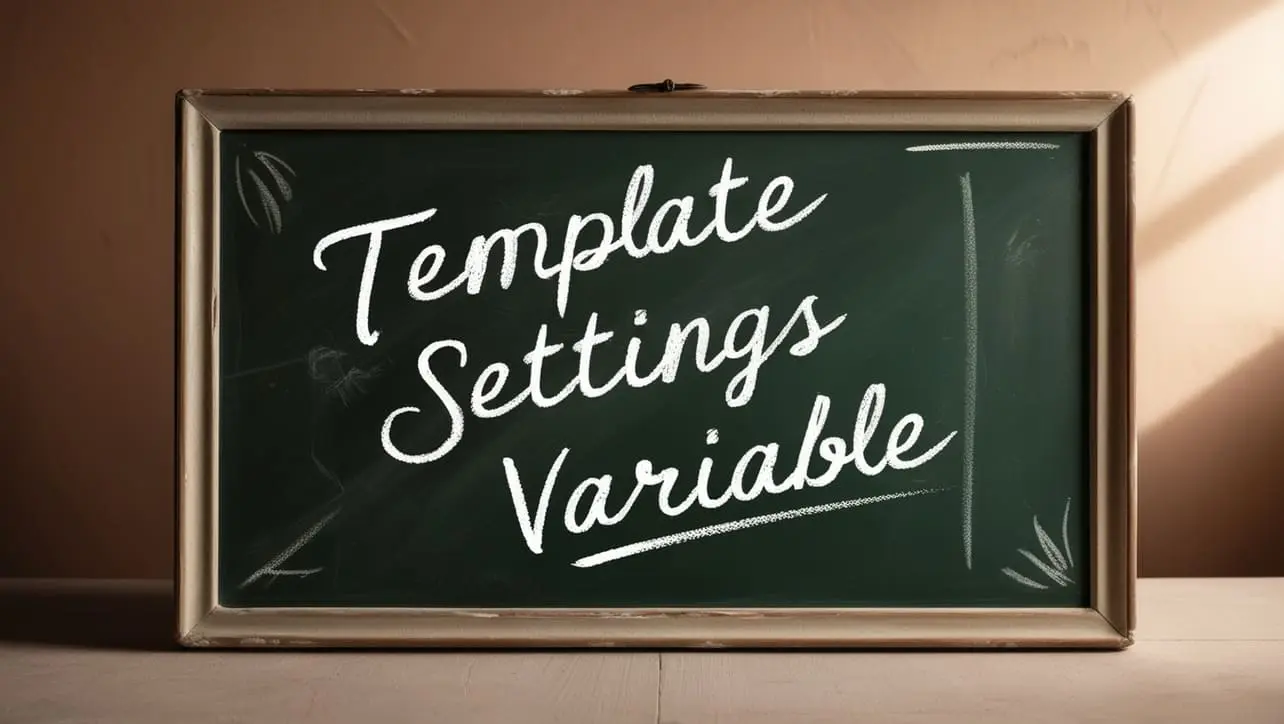
If you have any doubts regarding this article (Lodash _.chunk() Array Method), please comment here. I will help you immediately.