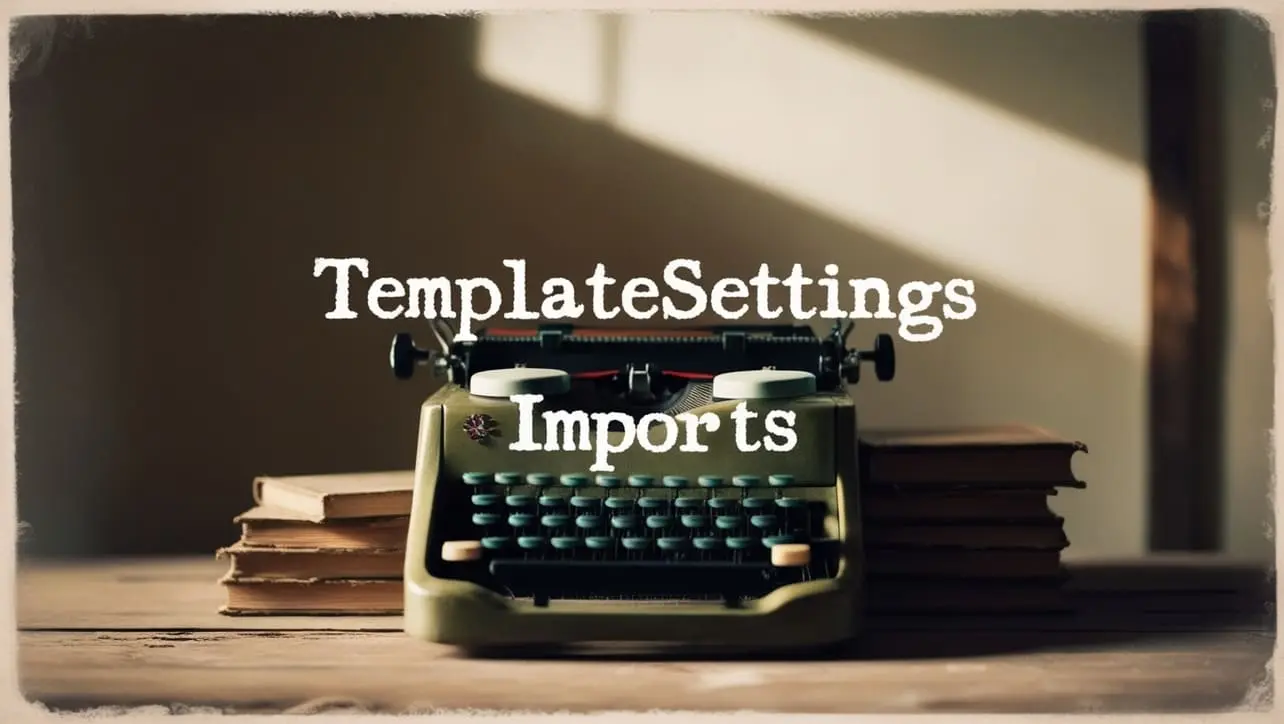
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.zipWith() Array Method
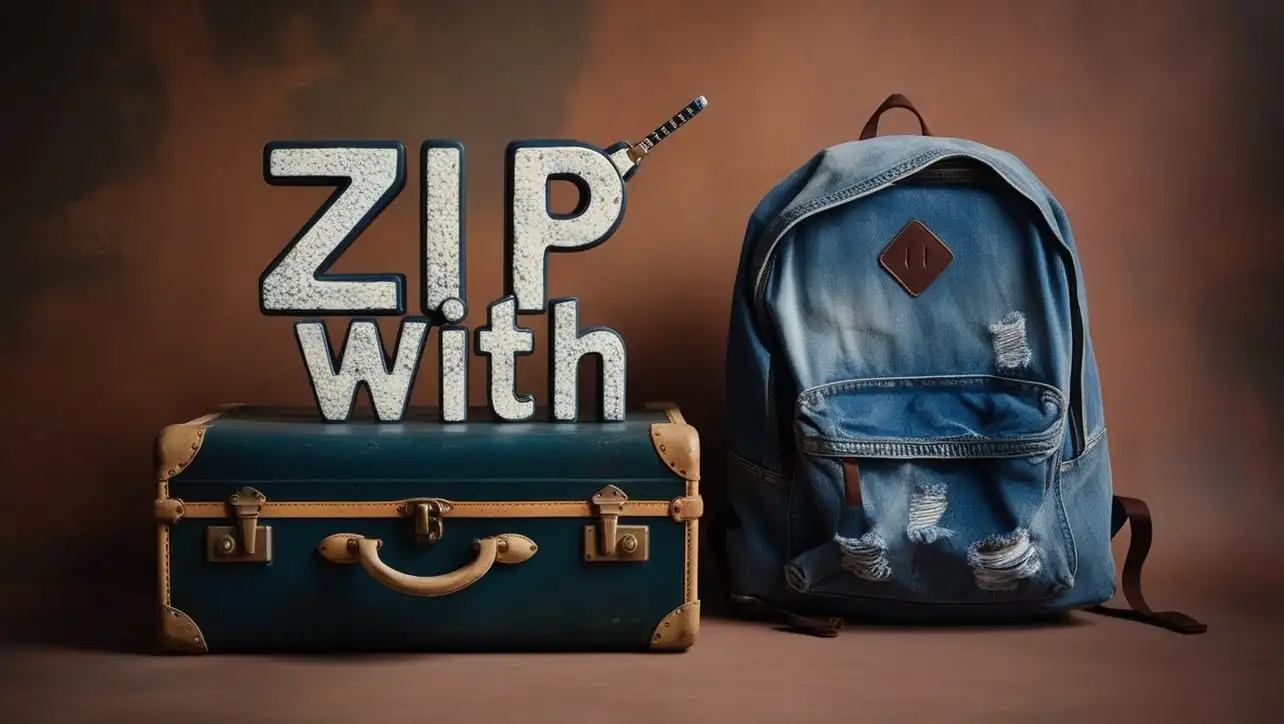
Photo Credit to CodeToFun
🙋 Introduction
In the ever-evolving landscape of JavaScript development, efficient manipulation of arrays is a common necessity. Lodash, a versatile utility library, provides developers with an arsenal of functions, and among them is the _.zipWith()
method.
This method is a powerful tool for merging multiple arrays and applying a custom function to the corresponding elements. It offers a flexible way to handle array combinations and transformations, adding a valuable layer to array manipulation capabilities.
🧠 Understanding _.zipWith()
The _.zipWith()
method in Lodash takes multiple arrays and a custom function as arguments. It then combines the arrays element-wise, applying the provided function to each set of corresponding elements. This allows developers to create new arrays with customized transformations.
💡 Syntax
_.zipWith(array1, array2, ..., iteratee)
- array1, array2, ...: Arrays to zip together.
- iteratee: The function invoked per iteration.
📝 Example
Let's explore a practical example to illustrate the functionality of _.zipWith()
:
const _ = require('lodash');
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const resultArray = _.zipWith(array1, array2, (a, b) => a + b);
console.log(resultArray);
// Output: [5, 7, 9]
In this example, array1 and array2 are zipped together, and the provided function (a, b) => a + b is applied to each corresponding pair of elements, resulting in a new array [5, 7, 9].
🏆 Best Practices
Understanding Zip Behavior:
Be aware of how
_.zipWith()
behaves when dealing with arrays of different lengths. The resulting array will have a length equal to the shortest input array. Ensure this behavior aligns with your expectations and requirements.example.jsCopiedconst array1 = [1, 2, 3]; const array2 = [4, 5, 6, 7]; const resultArray = _.zipWith(array1, array2, (a, b) => a + b); console.log(resultArray); // Output: [5, 7, 9]
Custom Transformation Function:
Leverage the power of custom transformation functions to manipulate zipped elements according to your specific requirements. This provides flexibility in creating diverse result arrays.
example.jsCopiedconst prices = [10, 20, 30]; const quantities = [2, 3, 4]; const totalPriceArray = _.zipWith(prices, quantities, (price, quantity) => price * quantity); console.log(totalPriceArray); // Output: [20, 60, 120]
Zip More Than Two Arrays:
_.zipWith()
allows you to zip together more than two arrays, providing a concise way to combine data from multiple sources.example.jsCopiedconst array1 = [1, 2, 3]; const array2 = ['a', 'b', 'c']; const array3 = [true, false, true]; const zippedArray = _.zipWith(array1, array2, array3, (a, b, c) => `${a}-${b}-${c}`); console.log(zippedArray); // Output: ['1-a-true', '2-b-false', '3-c-true']
📚 Use Cases
Combining Data from Different Sources:
_.zipWith()
is ideal for combining data from different arrays, providing a straightforward way to create new datasets.example.jsCopiedconst temperatures = [25, 30, 22]; const cities = ['New York', 'Paris', 'Tokyo']; const weatherReport = _.zipWith(temperatures, cities, (temp, city) => `${city}: ${temp}°C`); console.log(weatherReport); // Output: ['New York: 25°C', 'Paris: 30°C', 'Tokyo: 22°C']
Calculations with Multiple Arrays:
Performing calculations with corresponding elements from multiple arrays is a common use case for
_.zipWith()
, enabling the creation of result arrays based on custom formulas.example.jsCopiedconst array1 = [1, 2, 3]; const array2 = [4, 5, 6]; const squaredSumArray = _.zipWith(array1, array2, (a, b) => (a + b) ** 2); console.log(squaredSumArray); // Output: [25, 49, 81]
Dynamic Data Transformations:
Use
_.zipWith()
to dynamically transform data based on custom functions, allowing for adaptable and versatile array manipulation.example.jsCopiedconst dataPoints = [10, 20, 30]; const modifiers = [(x) => x * 2, (x) => x + 5, (x) => x ** 2]; const transformedData = _.zipWith(dataPoints, ...modifiers, (...args) => args.reduce((acc, curr) => curr(acc))); console.log(transformedData); // Output: [20, 25, 900]
🎉 Conclusion
The _.zipWith()
method in Lodash stands as a valuable asset for JavaScript developers seeking to merge arrays and apply custom transformations simultaneously. Whether you're combining data from different sources, performing calculations, or dynamically transforming arrays, _.zipWith()
provides a flexible and efficient solution.
Explore the possibilities of array manipulation with _.zipWith()
and enhance your JavaScript development toolkit!
👨💻 Join our Community:
Author
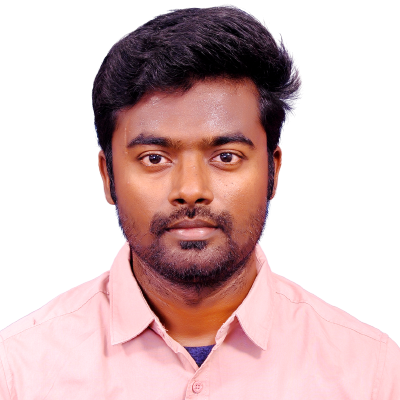
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
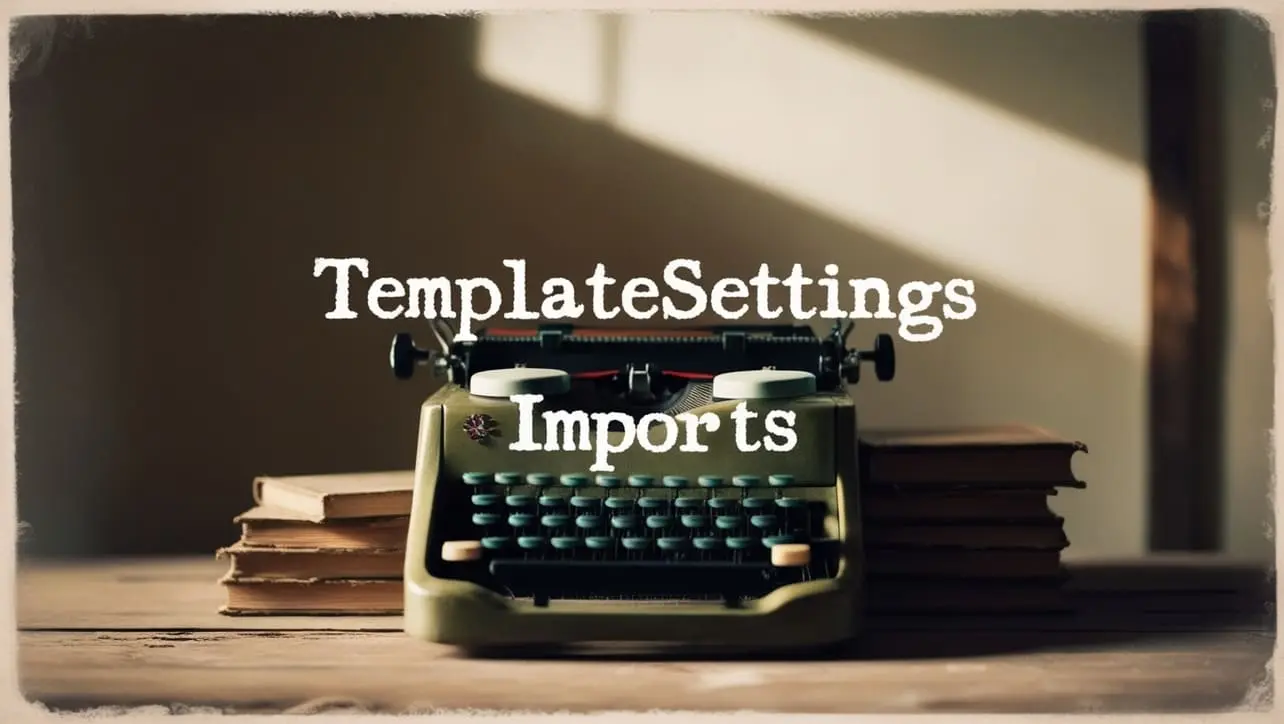
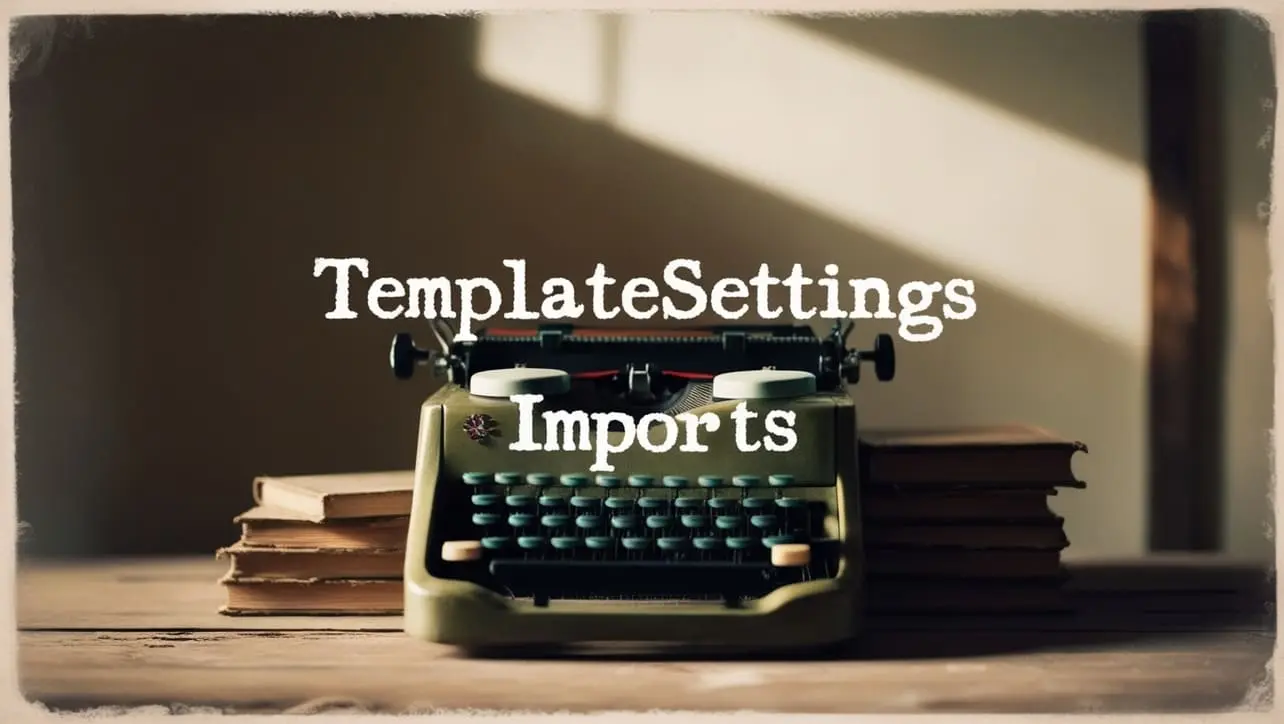
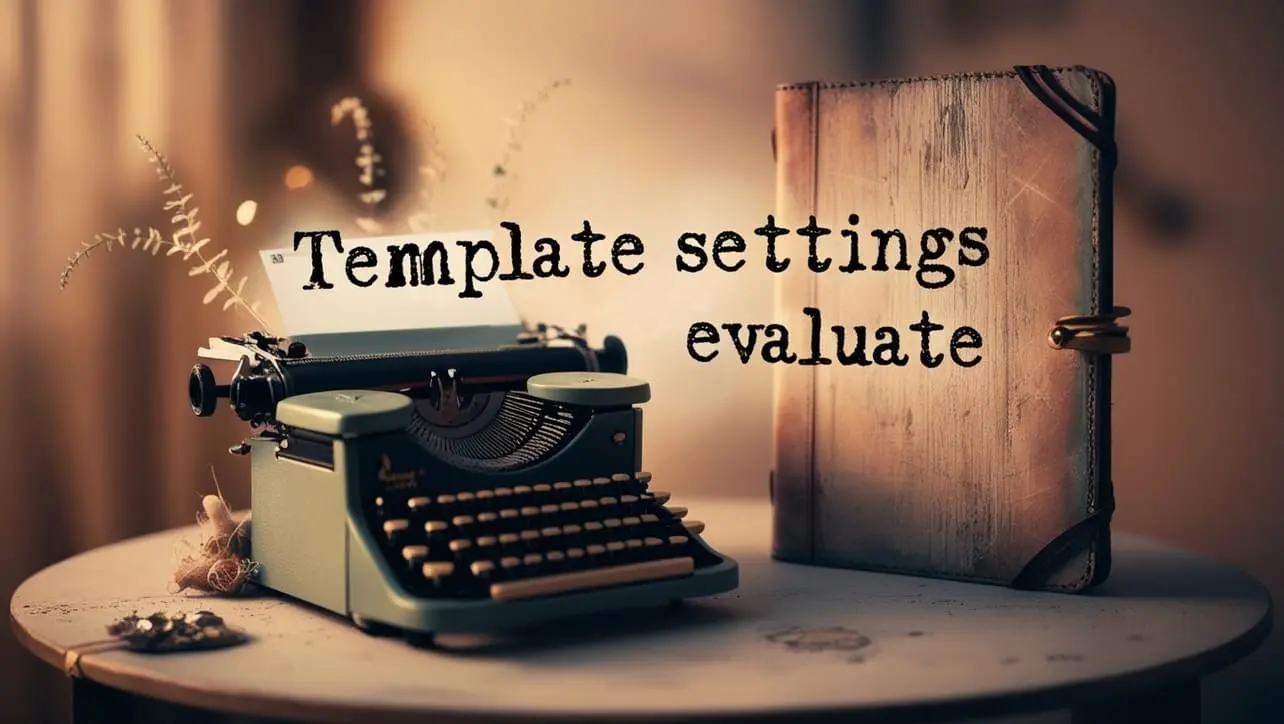
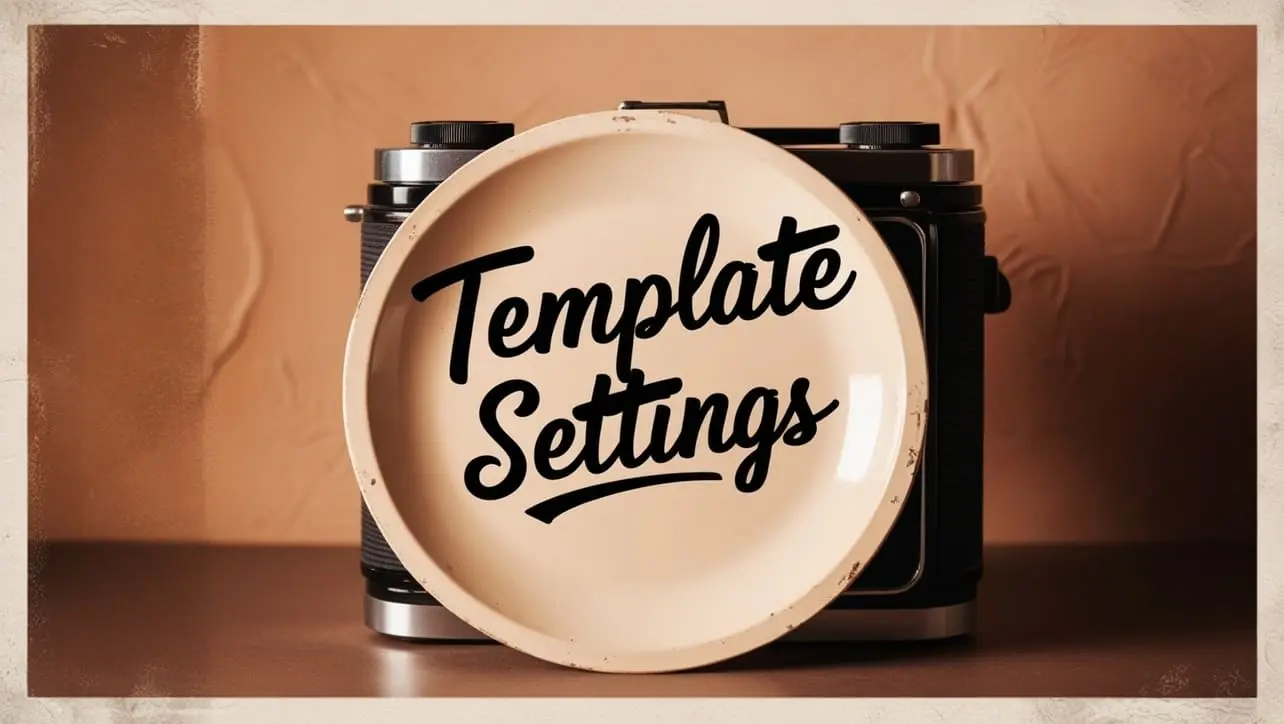
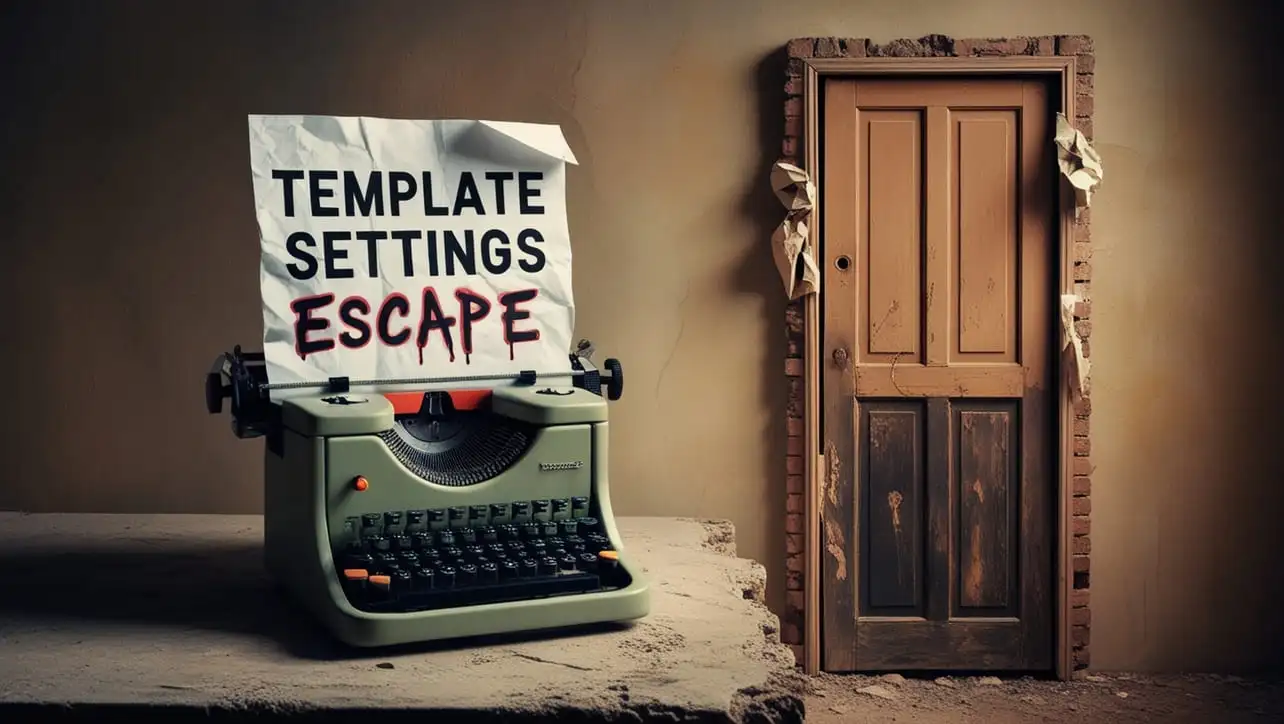
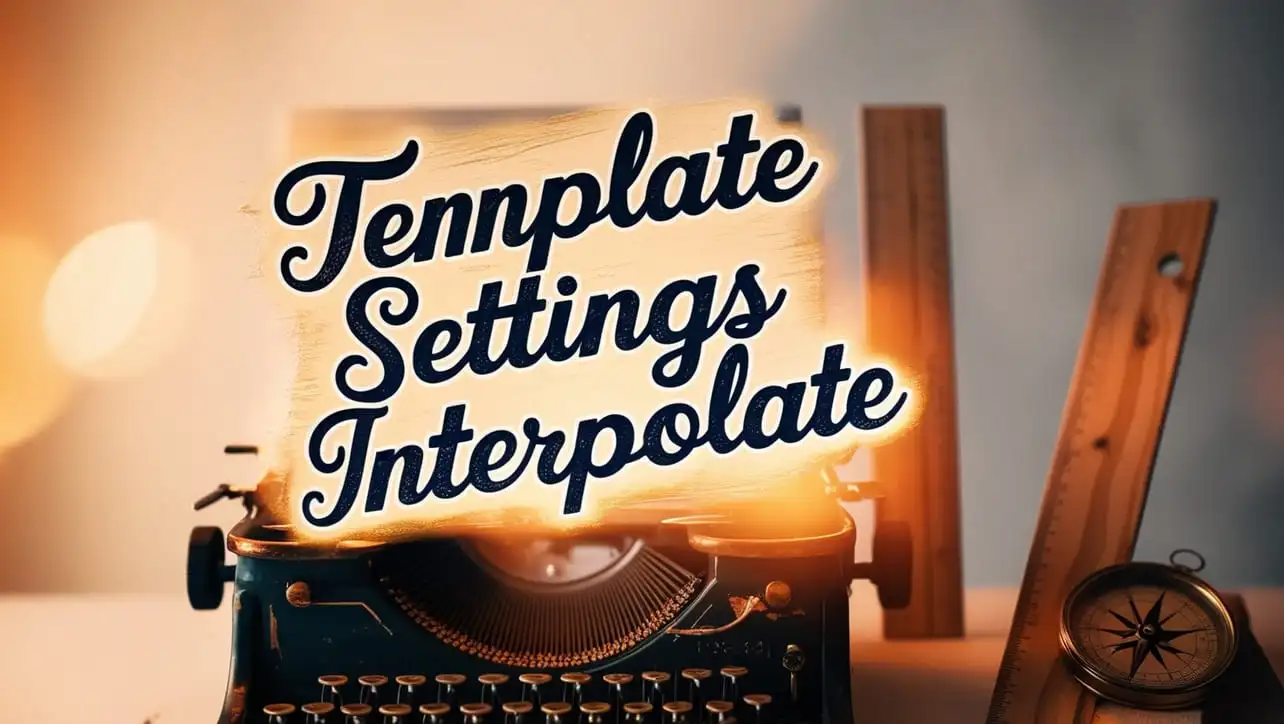
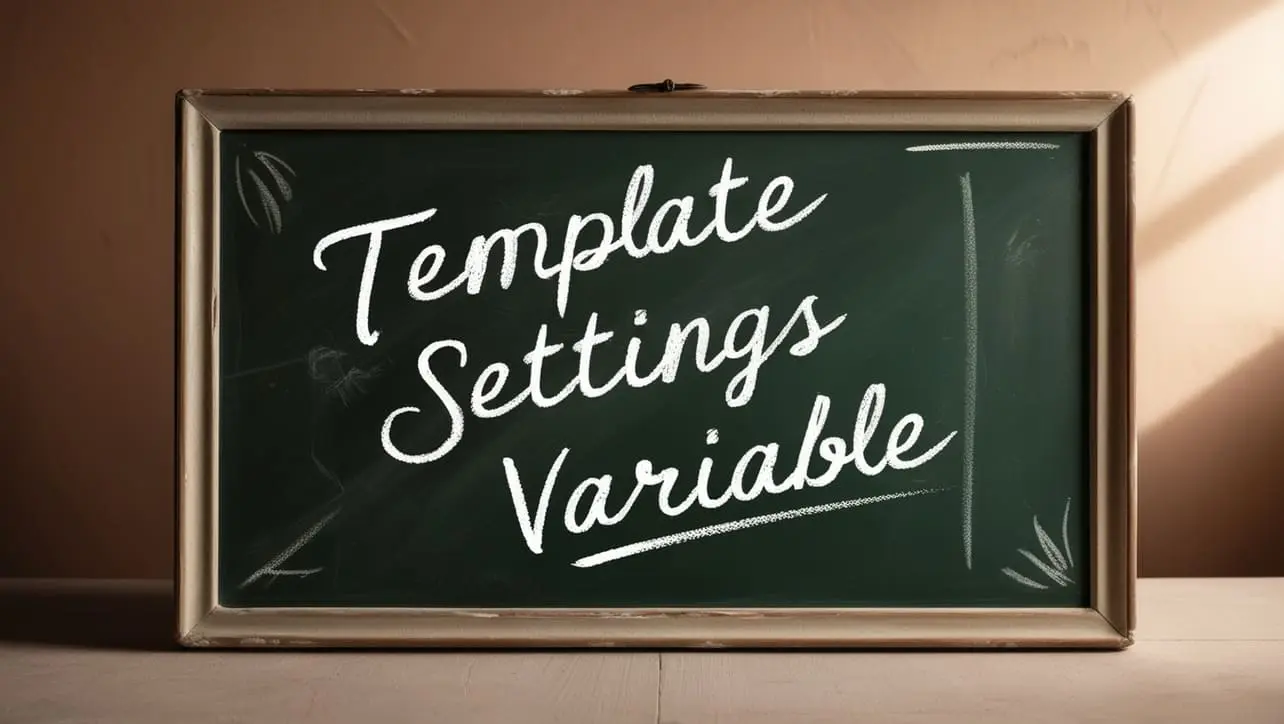
If you have any doubts regarding this article (Lodash _.zipWith() Array Method), please comment here. I will help you immediately.