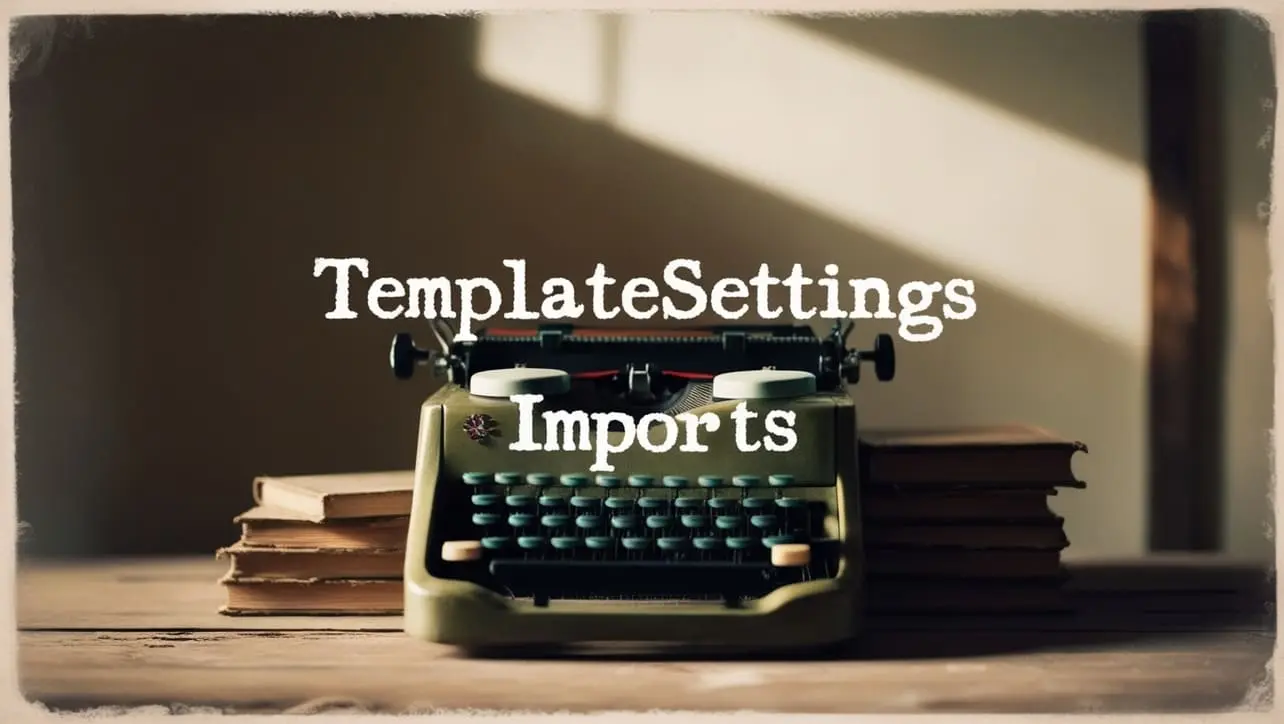
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.zip() Array Method
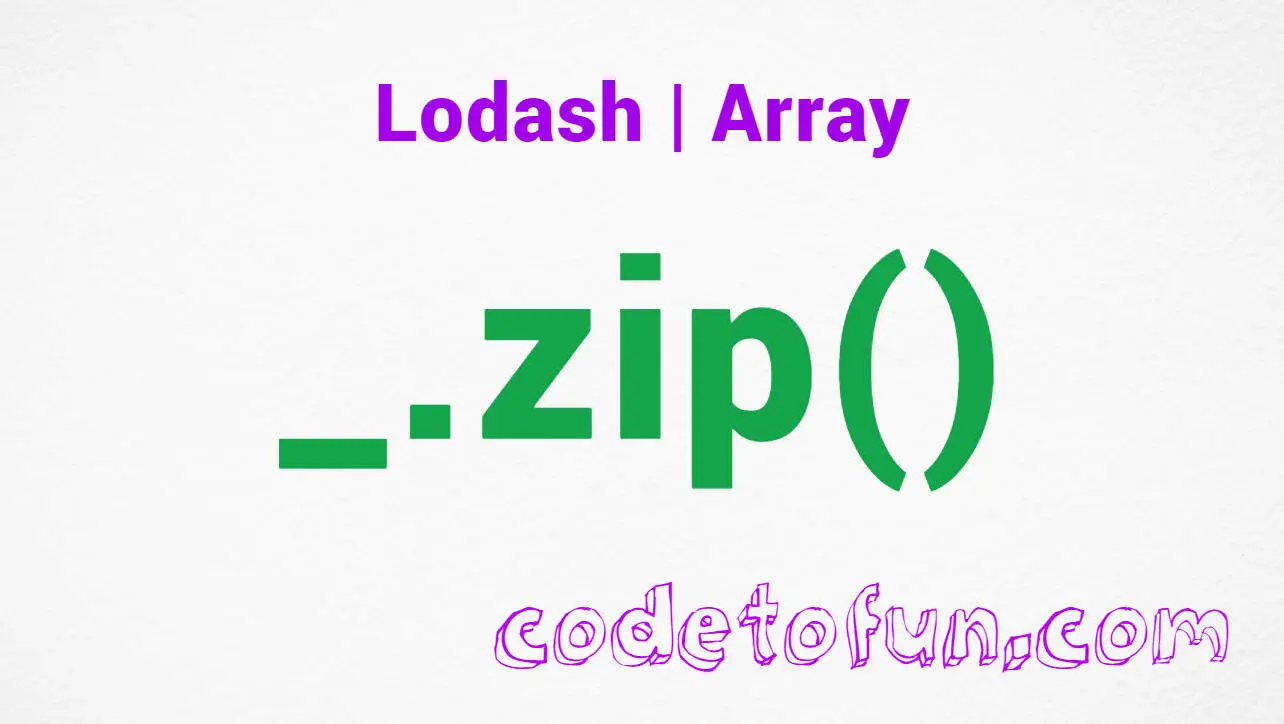
Photo Credit to CodeToFun
🙋 Introduction
Arrays often serve as fundamental data structures in JavaScript, and the ability to manipulate and combine them efficiently is crucial for developers. Enter Lodash, a versatile utility library that provides powerful functions for array operations. One such function is _.zip()
, a method that combines multiple arrays by grouping their elements into subarrays.
This method is particularly handy when dealing with data that needs to be organized or processed in a paired manner.
🧠 Understanding _.zip()
The _.zip()
method in Lodash takes multiple arrays and creates a new array by grouping the elements at the same index together. This results in an array of subarrays, each containing the elements from the input arrays at the corresponding position.
💡 Syntax
_.zip([arrays])
- arrays: The arrays to zip together.
📝 Example
Let's dive into a practical example to illustrate the functionality of _.zip()
:
const _ = require('lodash');
const array1 = [1, 2, 3];
const array2 = ['a', 'b', 'c'];
const array3 = [true, false, true];
const zippedArray = _.zip(array1, array2, array3);
console.log(zippedArray);
// Output: [[1, 'a', true], [2, 'b', false], [3, 'c', true]]
In this example, array1, array2, and array3 are zipped together to create a new array of subarrays.
🏆 Best Practices
Equalizing Array Lengths:
Ensure that the input arrays have the same length.
_.zip()
pairs elements at corresponding indices, and having arrays of different lengths may result in unexpected behavior.example.jsCopiedconst array4 = [10, 20, 30, 40]; const zippedUnequalArrays = _.zip(array1, array4); console.log(zippedUnequalArrays); // Output: [[1, 10], [2, 20], [3, 30], [undefined, 40]]
Handling Arrays of Objects:
When dealing with arrays of objects, consider using the _.map() function to extract specific properties before using
_.zip()
.example.jsCopiedconst arrayOfObjects1 = [{ name: 'Alice' }, { name: 'Bob' }, { name: 'Charlie' }]; const arrayOfObjects2 = [{ age: 25 }, { age: 30 }, { age: 22 }]; const names = _.map(arrayOfObjects1, 'name'); const ages = _.map(arrayOfObjects2, 'age'); const zippedObjects = _.zip(names, ages); console.log(zippedObjects); // Output: [['Alice', 25], ['Bob', 30], ['Charlie', 22]]
Unzipping Arrays:
To revert the zipping process, you can use _.unzip() to extract the original arrays.
example.jsCopiedconst unzippedArrays = _.unzip(zippedArray); console.log(unzippedArrays); // Output: [[1, 2, 3], ['a', 'b', 'c'], [true, false, true]]
📚 Use Cases
Data Pairing:
_.zip()
is useful when you need to pair data from multiple sources, such as combining arrays of names and ages.example.jsCopiedconst names = ['Alice', 'Bob', 'Charlie']; const ages = [25, 30, 22]; const pairedData = _.zip(names, ages); console.log(pairedData); // Output: [['Alice', 25], ['Bob', 30], ['Charlie', 22]]
Multi-array Operations:
Performing operations on multiple arrays simultaneously becomes more manageable with
_.zip()
.example.jsCopiedconst prices = [10, 20, 30]; const quantities = [2, 3, 1]; const totalCosts = _.map(_.zip(prices, quantities), ([price, quantity]) => price * quantity); console.log(totalCosts); // Output: [20, 60, 30]
Transposing Data:
When working with matrices or tabular data,
_.zip()
can transpose rows into columns.example.jsCopiedconst matrix = [ [1, 2, 3], [4, 5, 6], ]; const transposedMatrix = _.zip(...matrix); console.log(transposedMatrix); // Output: [[1, 4], [2, 5], [3, 6]]
🎉 Conclusion
The _.zip()
method in Lodash offers a convenient way to combine multiple arrays by grouping their elements into subarrays. Whether you're pairing data, transposing matrices, or performing multi-array operations, _.zip()
proves to be a valuable tool for array manipulation in JavaScript.
Explore the possibilities of array combination with _.zip()
and enhance your JavaScript development experience!
👨💻 Join our Community:
Author
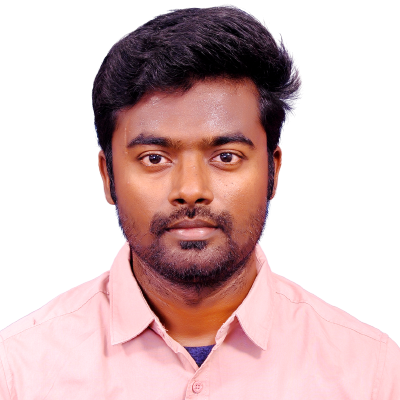
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
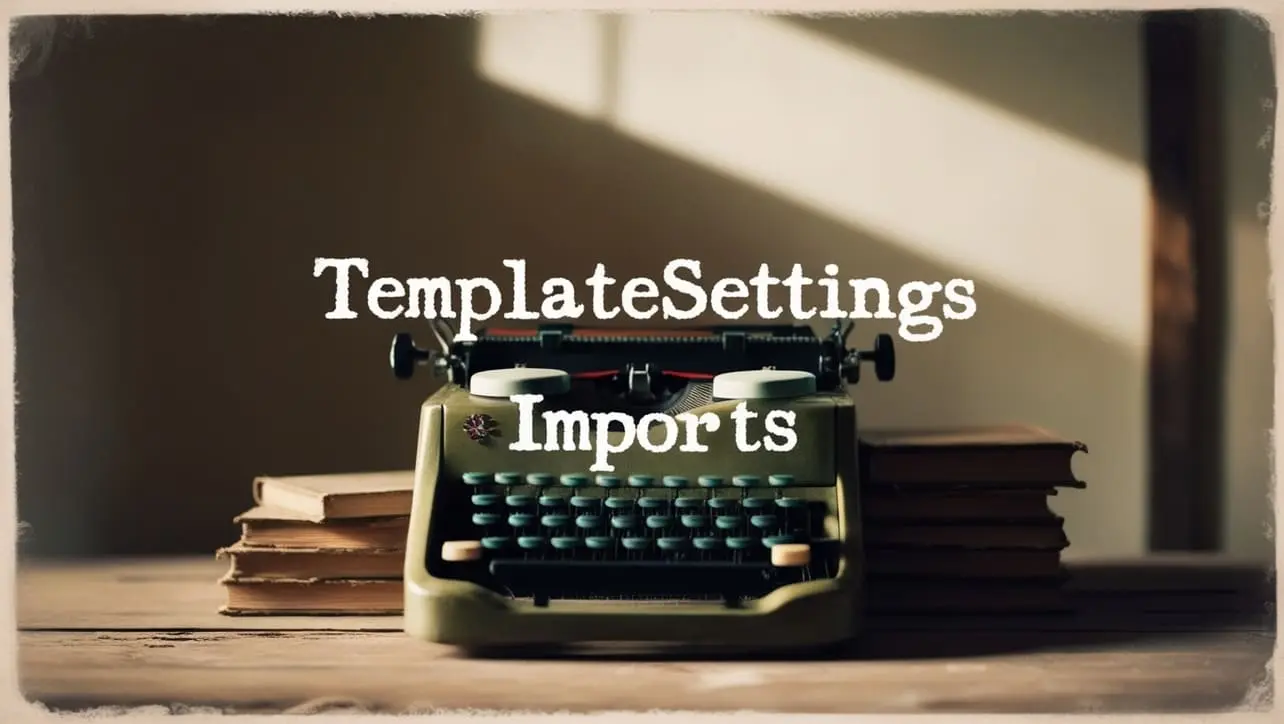
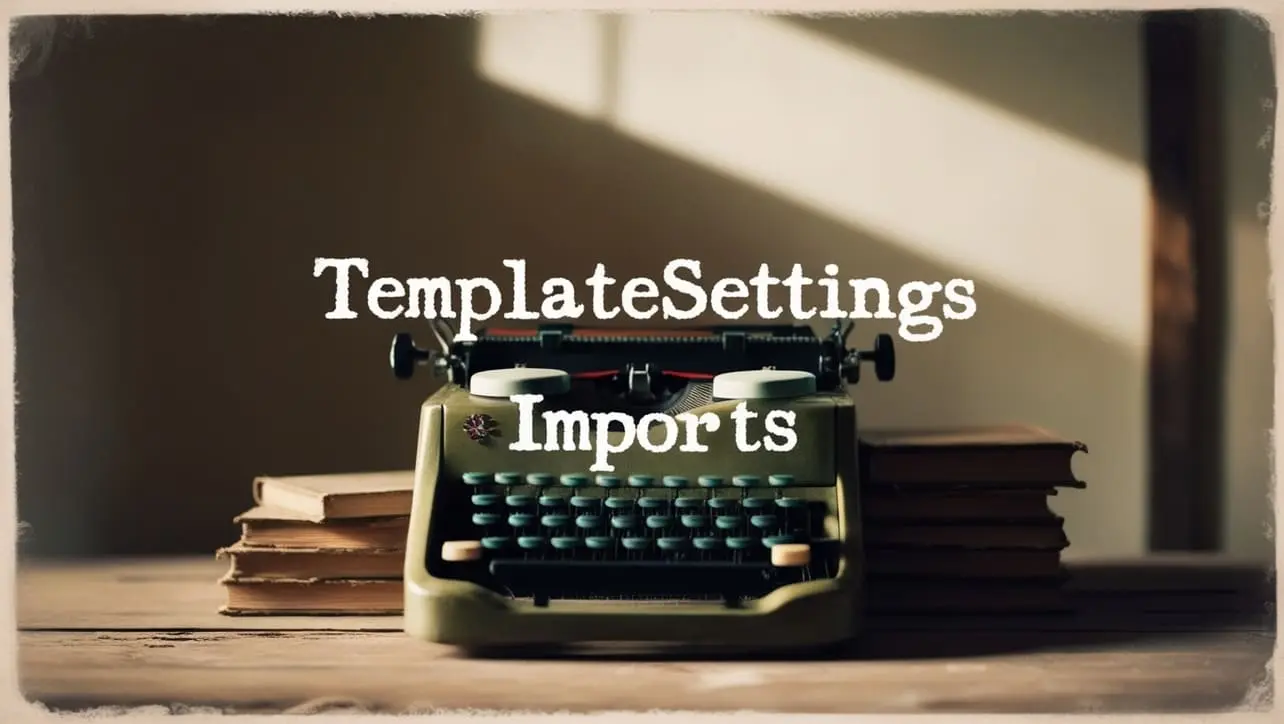
Lodash _.templateSettings.imports Property
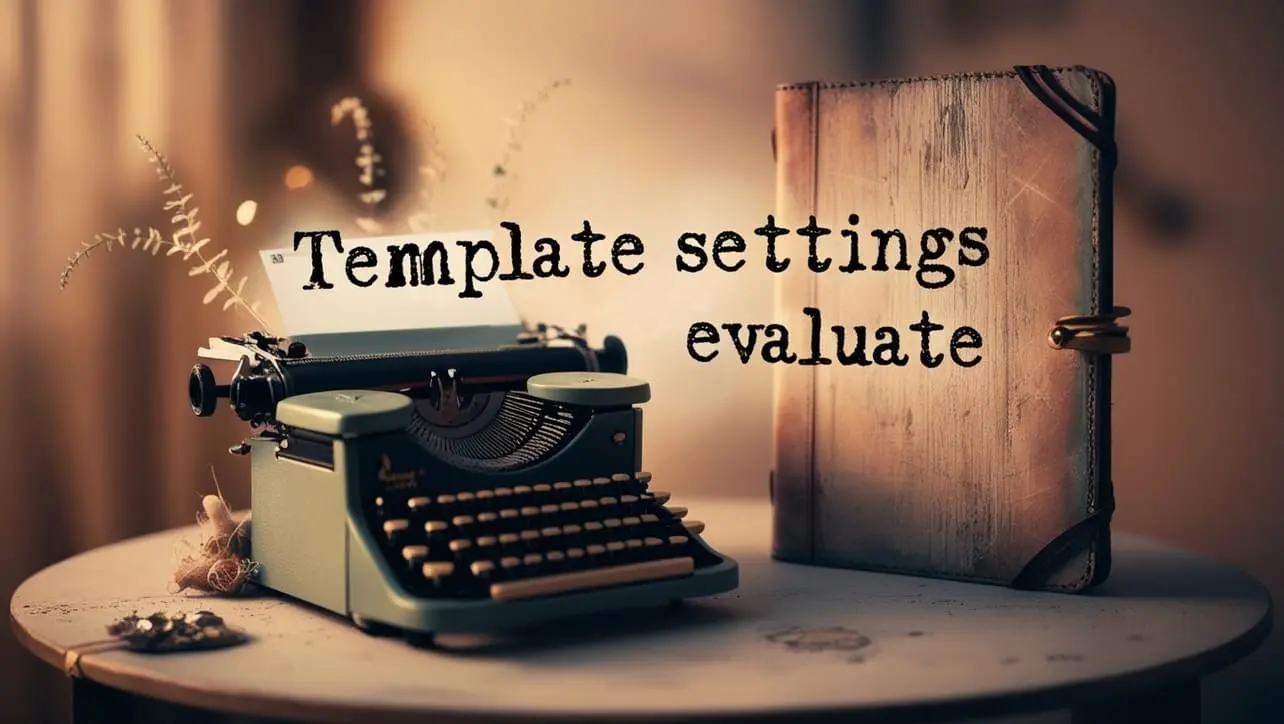
Lodash _.templateSettings.evaluate Property
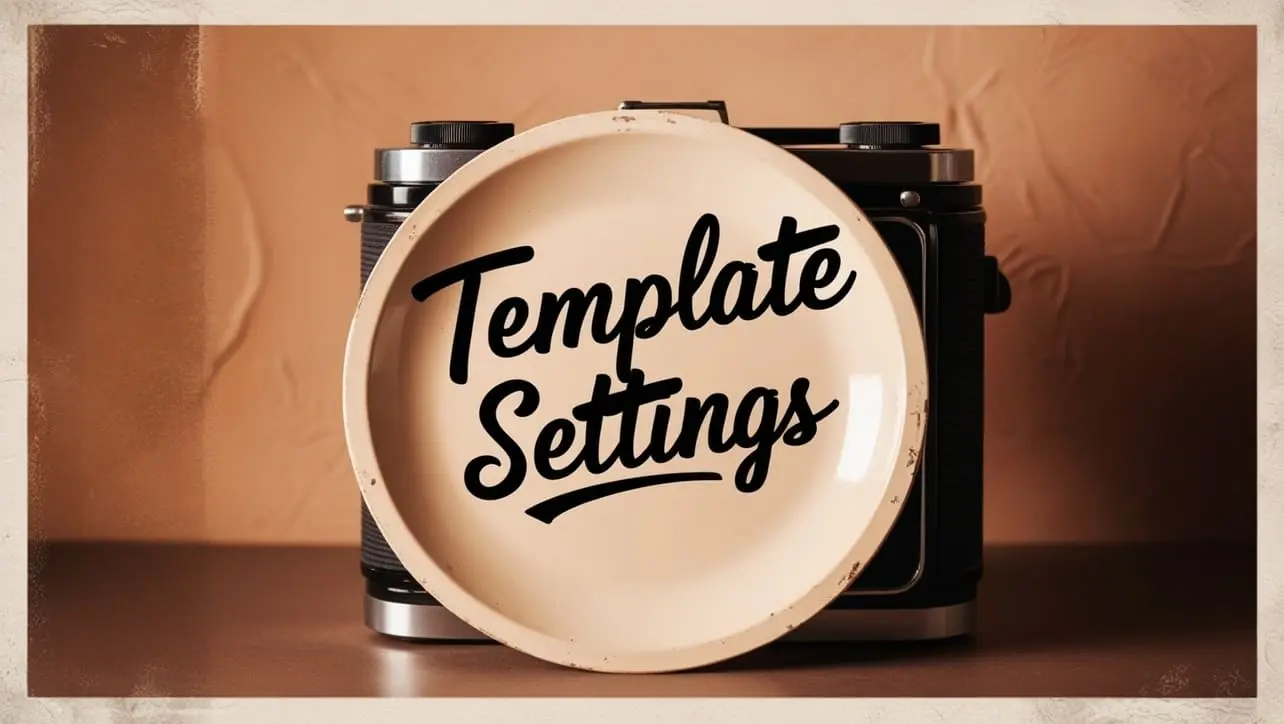
Lodash _.templateSettings Property
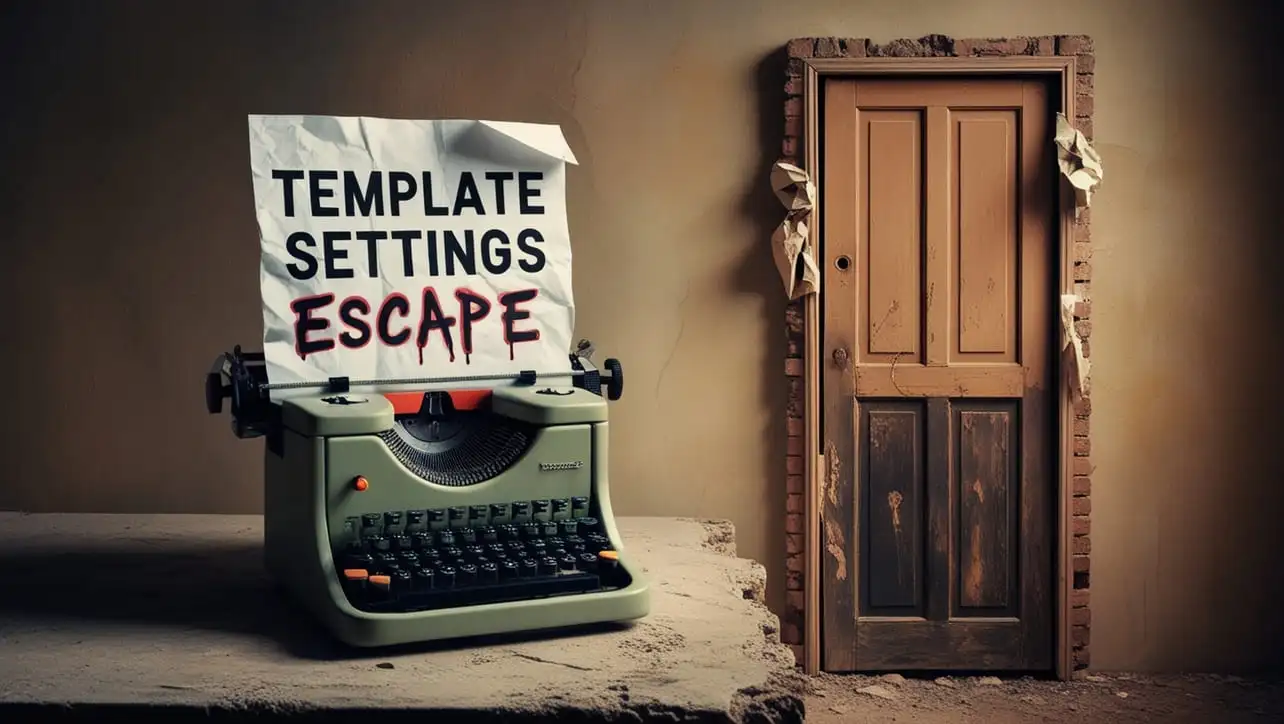
Lodash _.templateSettings.escape Property
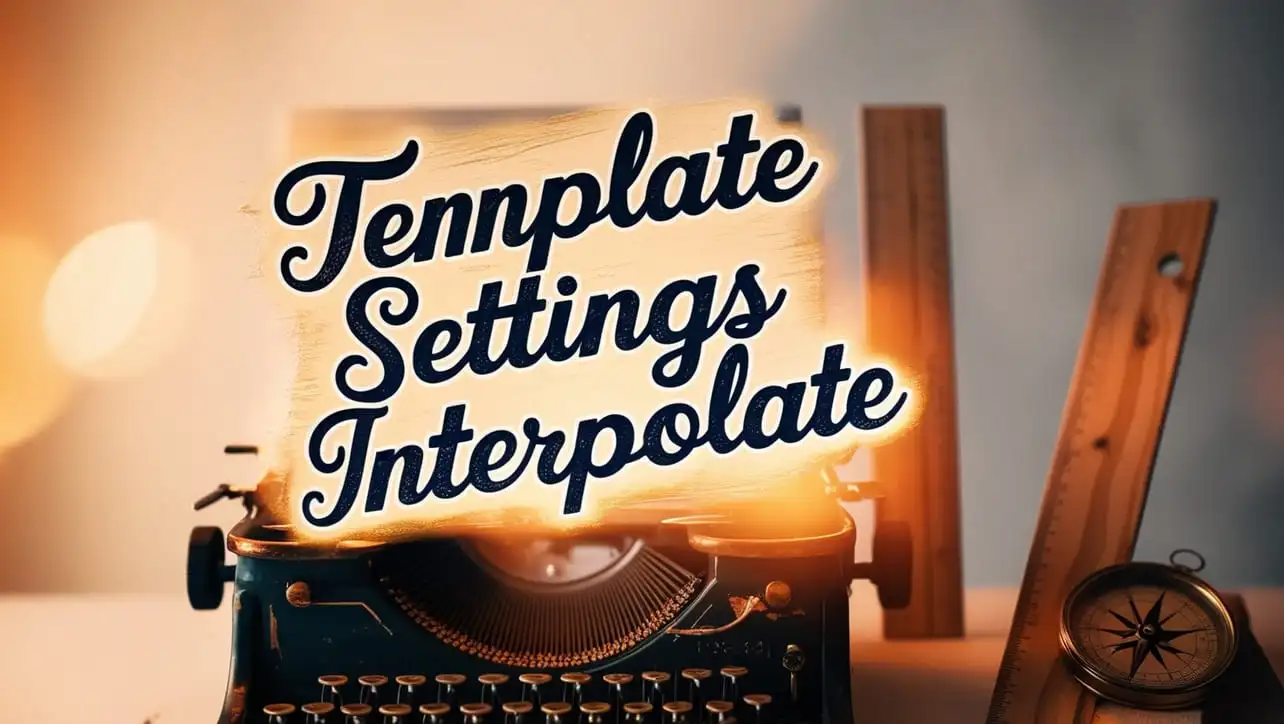
Lodash _.templateSettings.interpolate Property
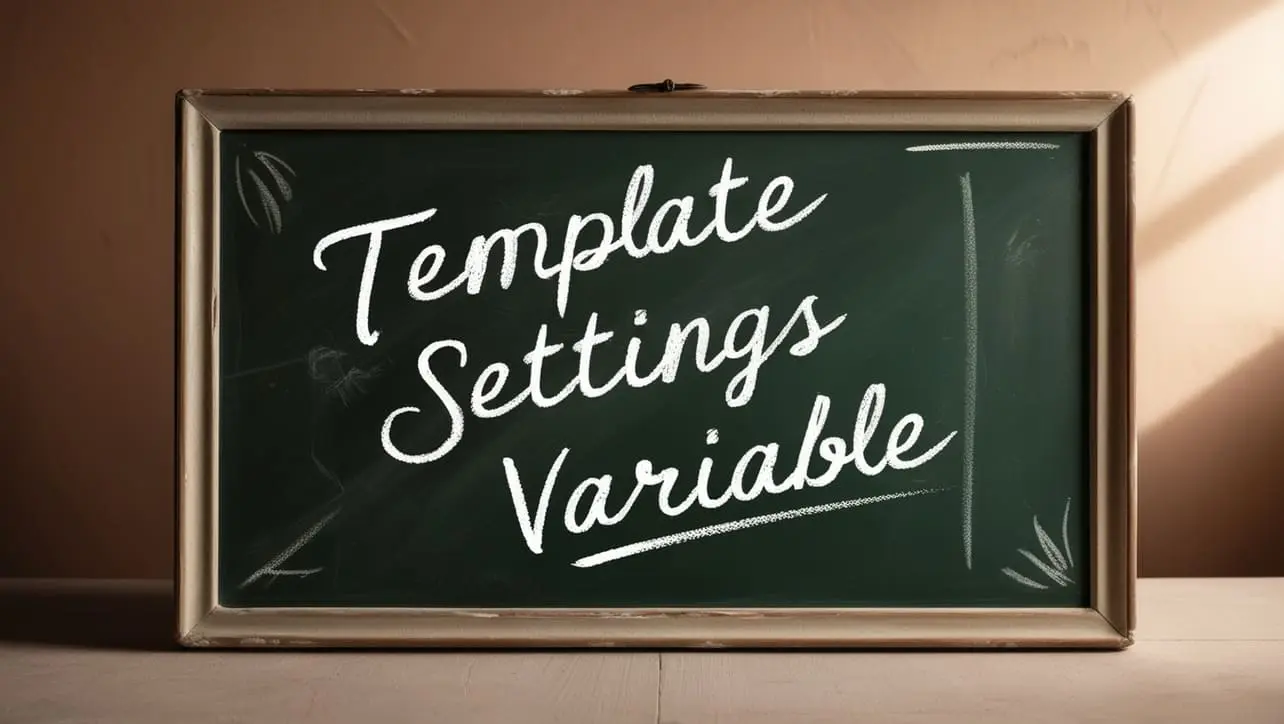
If you have any doubts regarding this article (Lodash _.zip() Array Method), please comment here. I will help you immediately.