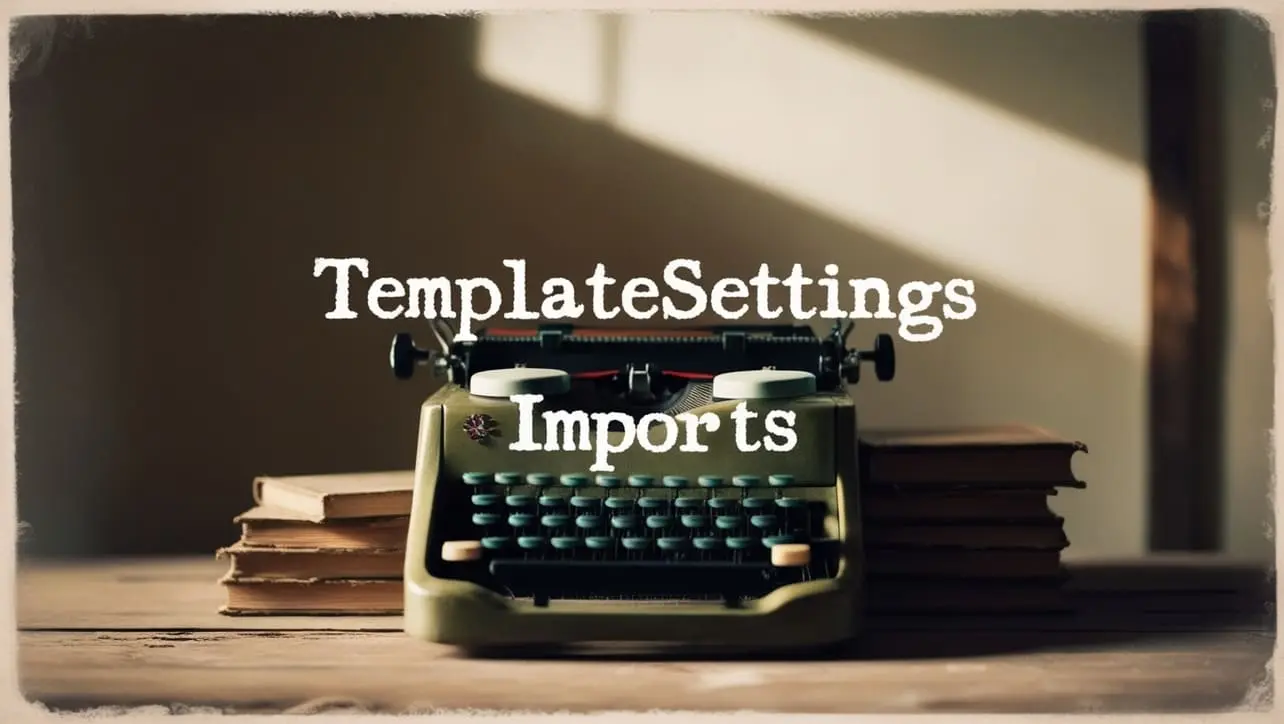
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.xorWith() Array Method
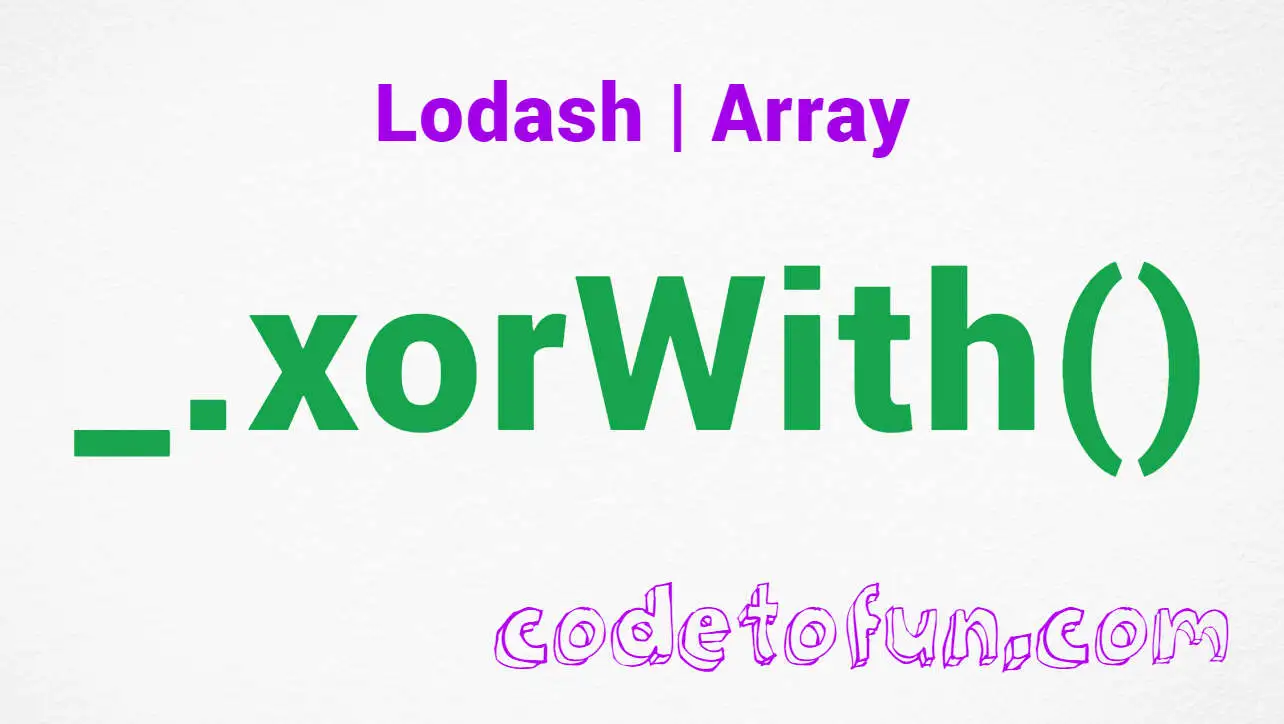
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, effective manipulation of arrays is a common requirement. Lodash, a comprehensive utility library, offers a myriad of functions, and one such gem is the _.xorWith()
method. This method provides a powerful tool for finding the symmetric difference between arrays, especially when dealing with complex comparison criteria.
Let's explore the intricacies of _.xorWith()
and how it can elevate your array manipulation capabilities.
🧠 Understanding _.xorWith()
The _.xorWith()
method in Lodash is designed to compute the symmetric difference between arrays based on a custom comparator function. It allows you to specify the criteria for equality between elements, providing a flexible approach to array comparison.
💡 Syntax
_.xorWith(...arrays, [comparator])
- ...arrays: The arrays to process.
- comparator (Optional): The function invoked per element.
📝 Example
Let's dive into a practical example to illustrate the power of _.xorWith()
:
const _ = require('lodash');
const array1 = [{ x: 1, y: 2 }, { x: 2, y: 3 }];
const array2 = [{ x: 1, y: 2 }, { x: 3, y: 4 }];
const symmetricDifference = _.xorWith(array1, array2, _.isEqual);
console.log(symmetricDifference);
// Output: [{ x: 2, y: 3 }, { x: 3, y: 4 }]
In this example, the symmetric difference between array1 and array2 is computed based on the custom comparator function _.isEqual.
🏆 Best Practices
Define a Custom Comparator:
Utilize the comparator parameter to define a custom function for comparing elements. This allows you to tailor the comparison logic to your specific requirements.
example.jsCopiedconst array1 = [1, 2, 3]; const array2 = [2, 3, 4]; const customComparator = (a, b) => Math.abs(a - b) === 1; const symmetricDifference = _.xorWith(array1, array2, customComparator); console.log(symmetricDifference); // Output: [1, 4]
Handle Complex Data Structures:
When working with complex data structures, such as objects or nested arrays, ensure that the comparator function accounts for the specific structure and comparison logic.
example.jsCopiedconst array1 = [{ id: 1 }, { id: 2 }]; const array2 = [{ id: 2 }, { id: 3 }]; const customComparator = (a, b) => a.id === b.id; const symmetricDifference = _.xorWith(array1, array2, customComparator); console.log(symmetricDifference); // Output: [{ id: 1 }, { id: 3 }]
Performance Considerations:
Be mindful of the performance implications, especially when dealing with large arrays. Custom comparators may introduce additional computation, so assess the impact on execution time.
example.jsCopiedconst largeArray1 = /* ... generate a large array ... */; const largeArray2 = /* ... generate another large array ... */; console.time('xorWith'); const result = _.xorWith(largeArray1, largeArray2, _.isEqual); console.timeEnd('xorWith'); console.log(result);
📚 Use Cases
Finding Unique Elements:
_.xorWith()
is particularly useful when you need to find unique elements that do not exist in both arrays. This is valuable for tasks such as identifying differences between datasets.example.jsCopiedconst array1 = [1, 2, 3]; const array2 = [2, 3, 4]; const symmetricDifference = _.xorWith(array1, array2, _.isEqual); console.log(symmetricDifference); // Output: [1, 4]
Handling Complex Comparisons:
When dealing with complex data structures or objects,
_.xorWith()
allows you to specify a custom comparator, enabling precise and tailored comparisons.example.jsCopiedconst array1 = [{ id: 1 }, { id: 2 }]; const array2 = [{ id: 2 }, { id: 3 }]; const customComparator = (a, b) => a.id === b.id; const symmetricDifference = _.xorWith(array1, array2, customComparator); console.log(symmetricDifference); // Output: [{ id: 1 }, { id: 3 }]
Symmetric Difference in Arrays:
Use
_.xorWith()
to find elements that exist in either of the arrays but not in both, providing a convenient way to handle the symmetric difference.example.jsCopiedconst array1 = ['apple', 'orange', 'banana']; const array2 = ['banana', 'grape', 'kiwi']; const symmetricDifference = _.xorWith(array1, array2, _.isEqual); console.log(symmetricDifference); // Output: ['apple', 'orange', 'grape', 'kiwi']
🎉 Conclusion
The _.xorWith()
method in Lodash empowers JavaScript developers to compute the symmetric difference between arrays with customizable comparison logic. Whether you're dealing with simple values or complex data structures, this method provides a versatile solution for array manipulation.
Explore the capabilities of _.xorWith()
and elevate your array manipulation skills in JavaScript!
👨💻 Join our Community:
Author
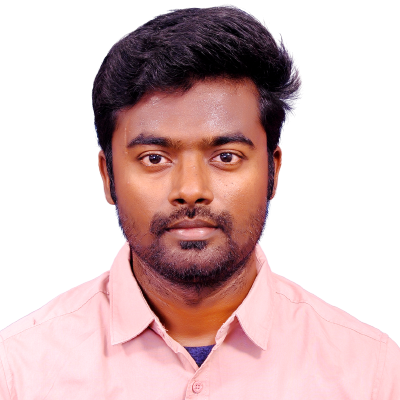
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
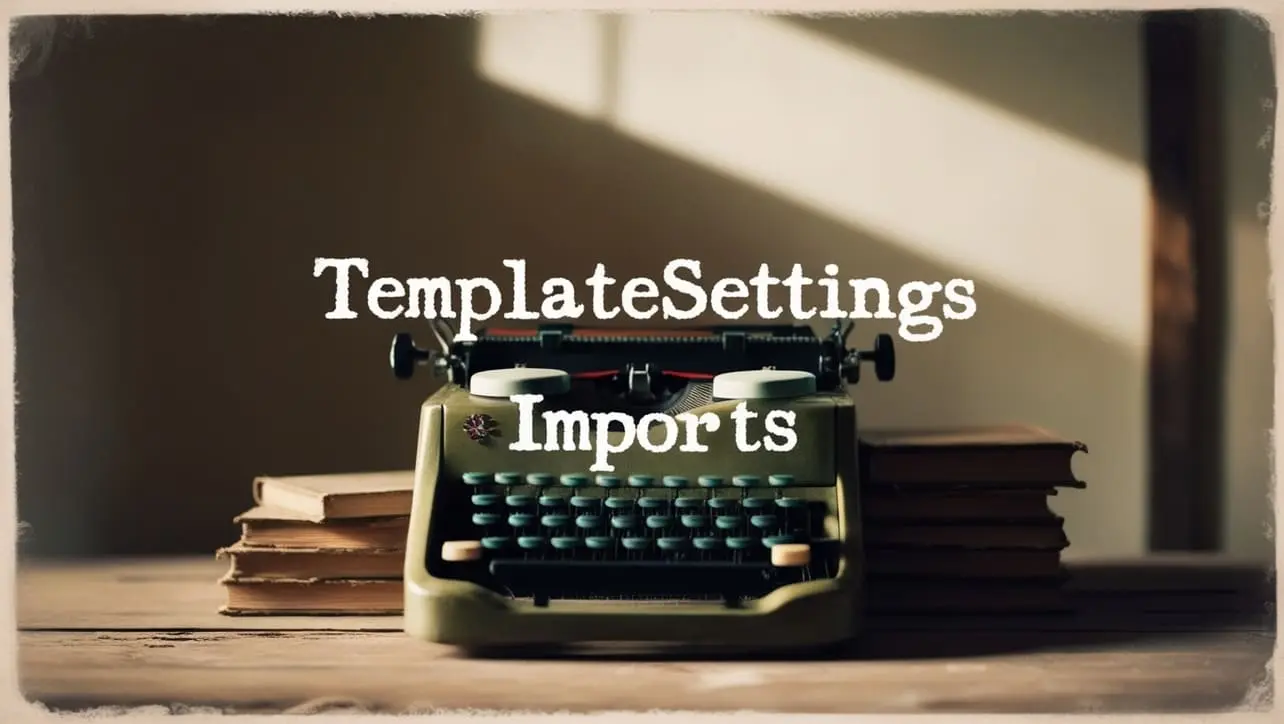
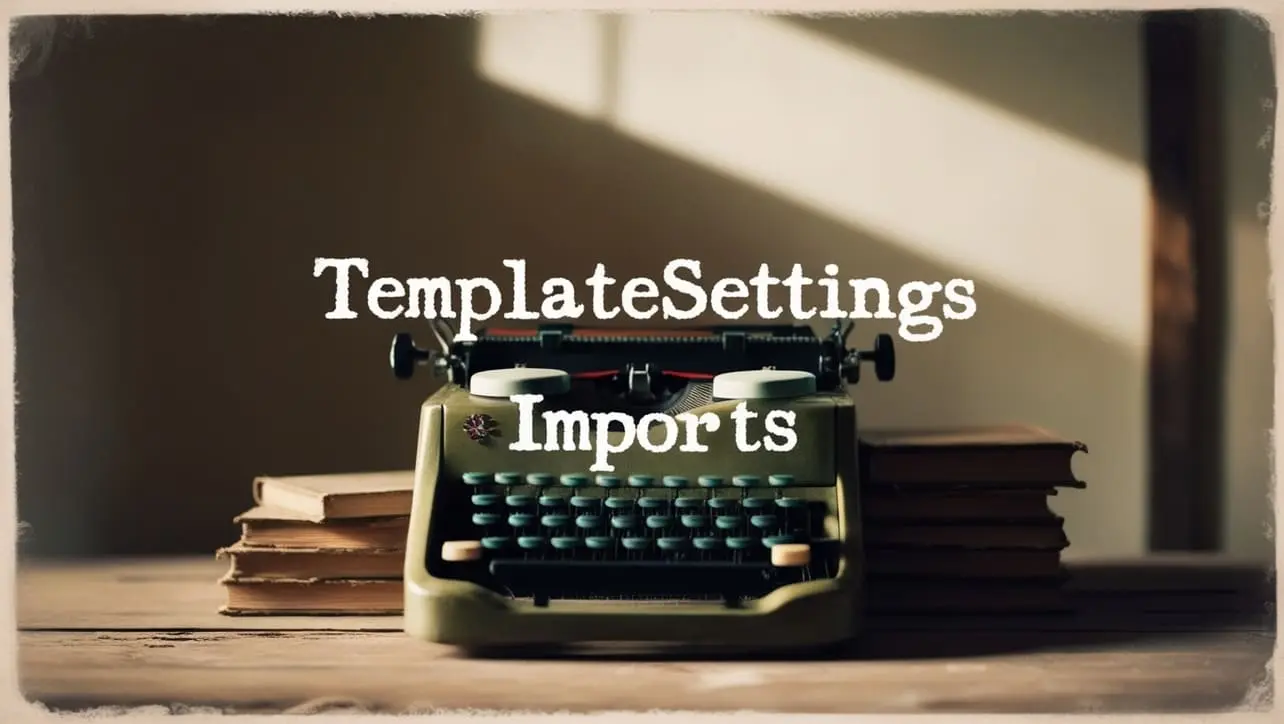
Lodash _.templateSettings.imports Property
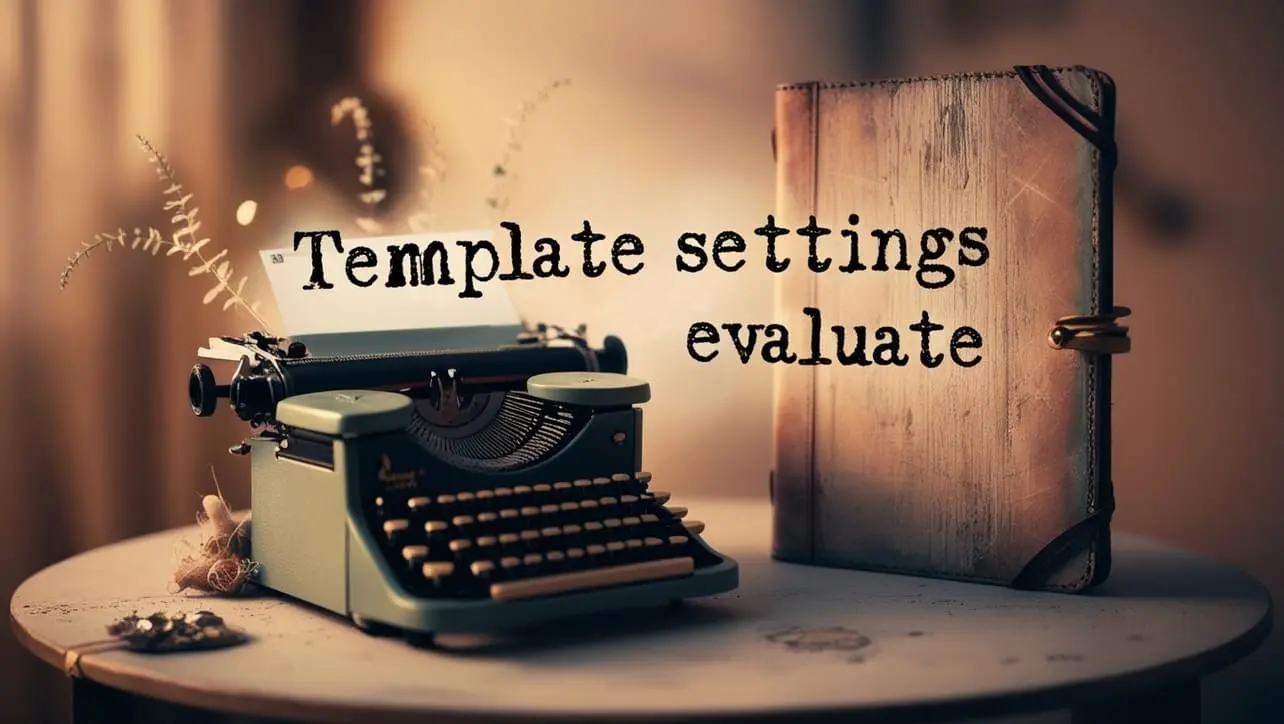
Lodash _.templateSettings.evaluate Property
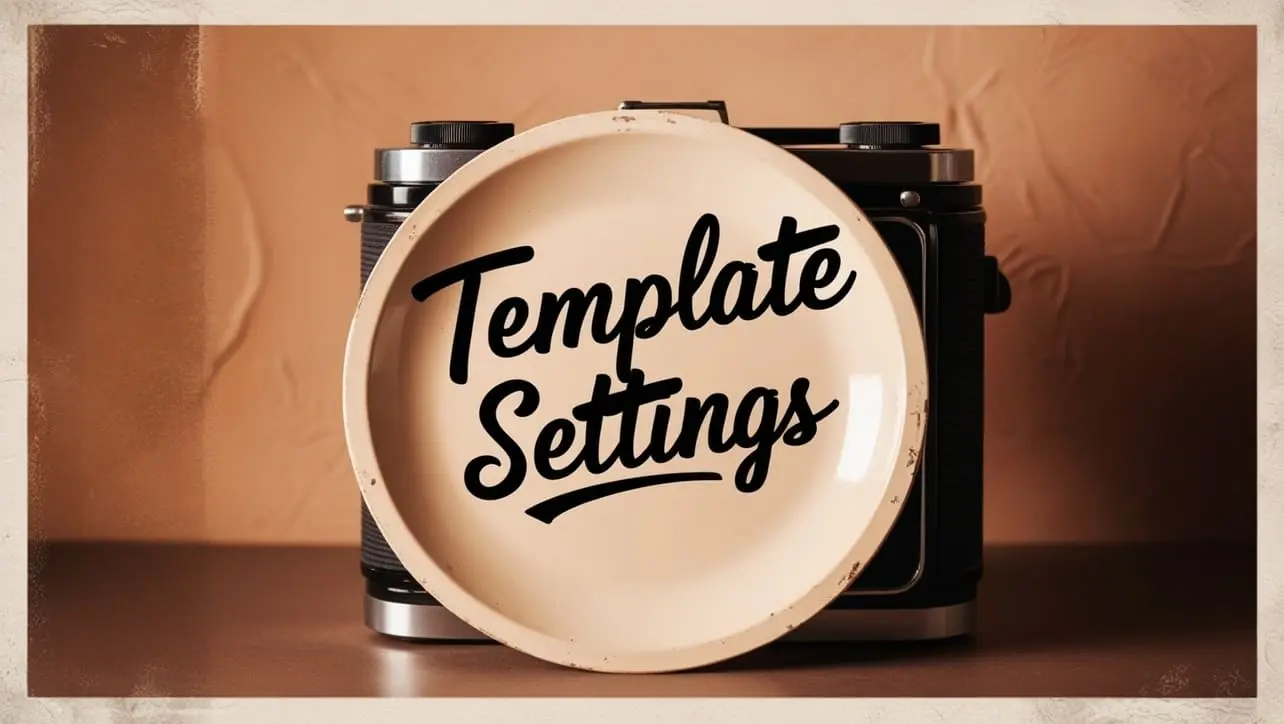
Lodash _.templateSettings Property
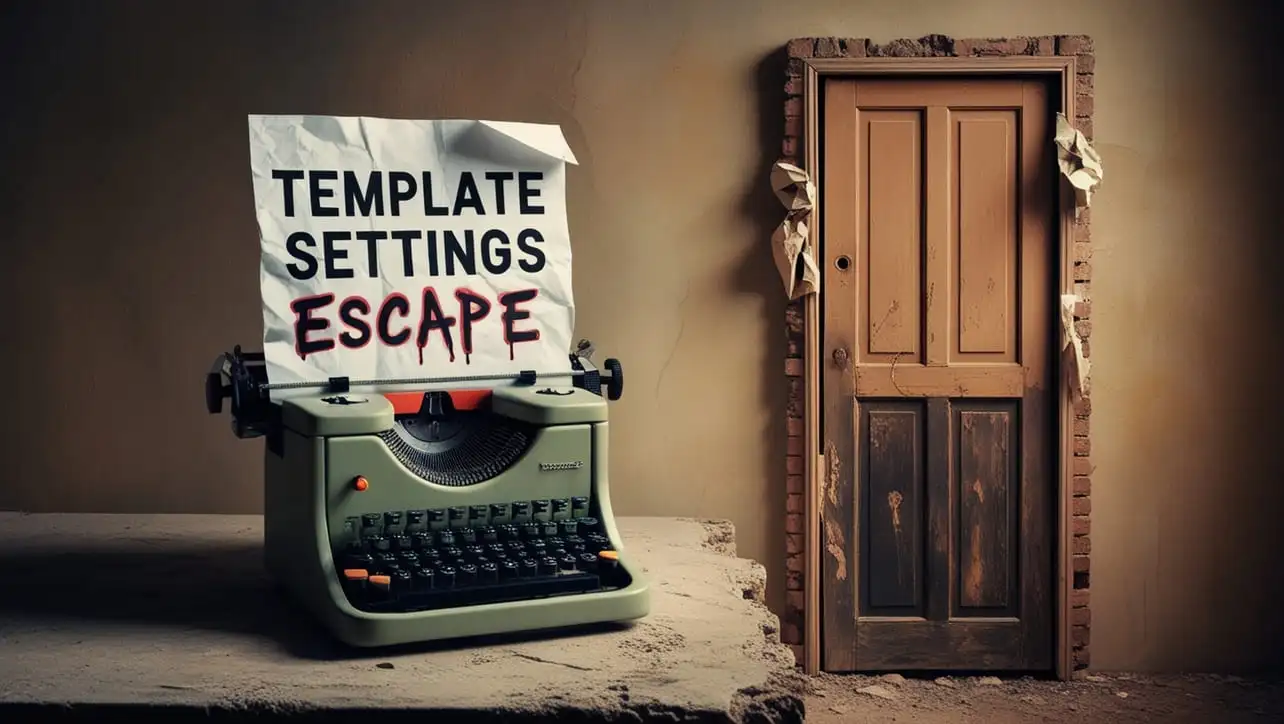
Lodash _.templateSettings.escape Property
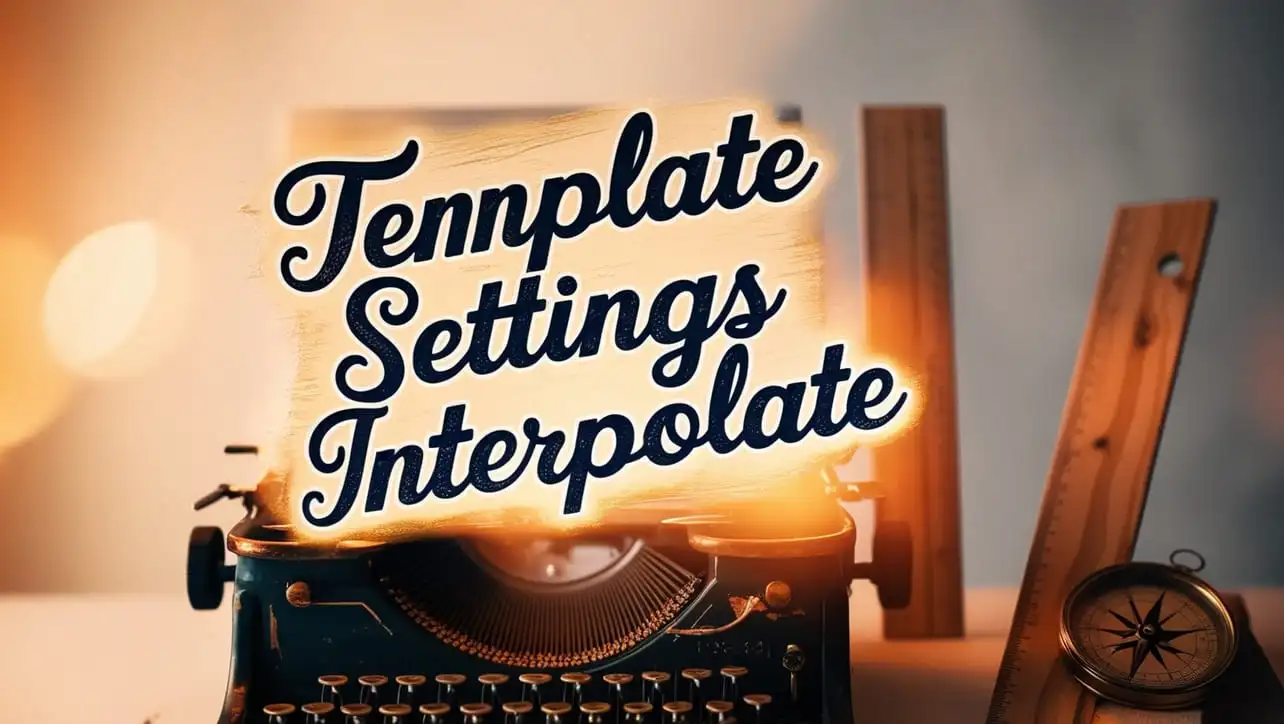
Lodash _.templateSettings.interpolate Property
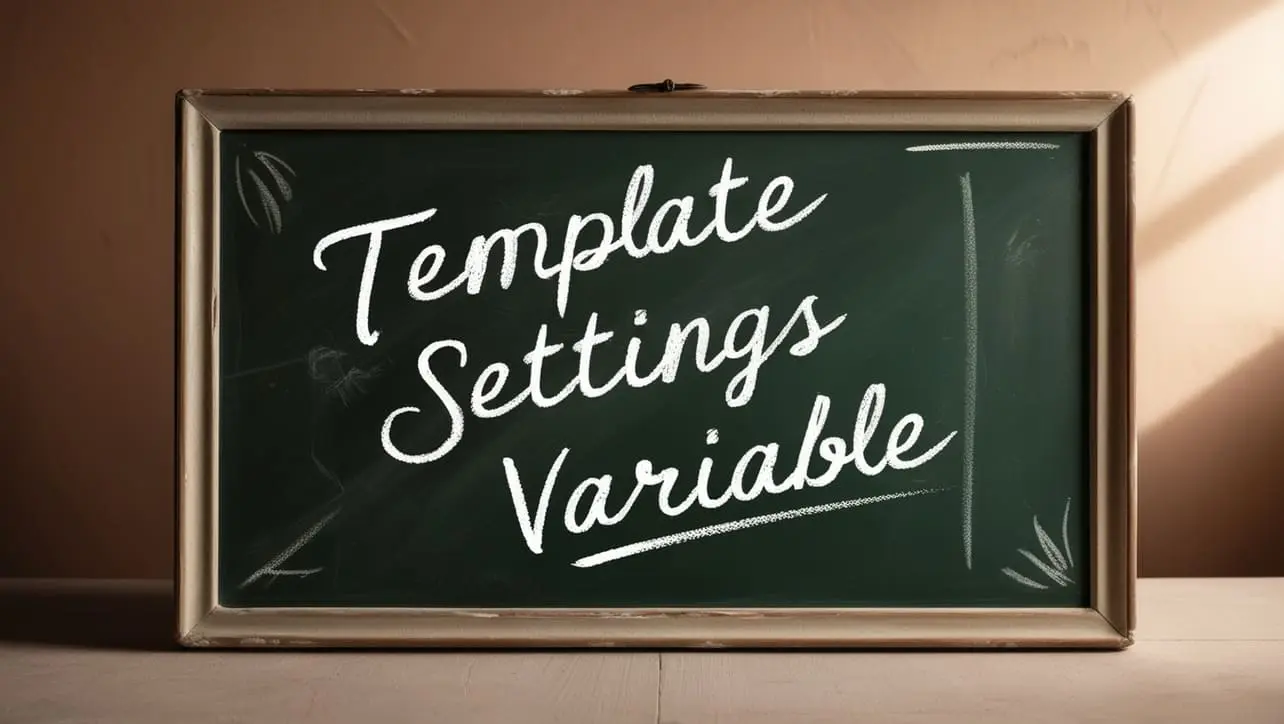
If you have any doubts regarding this article (Lodash _.xorWith() Array Method), please comment here. I will help you immediately.