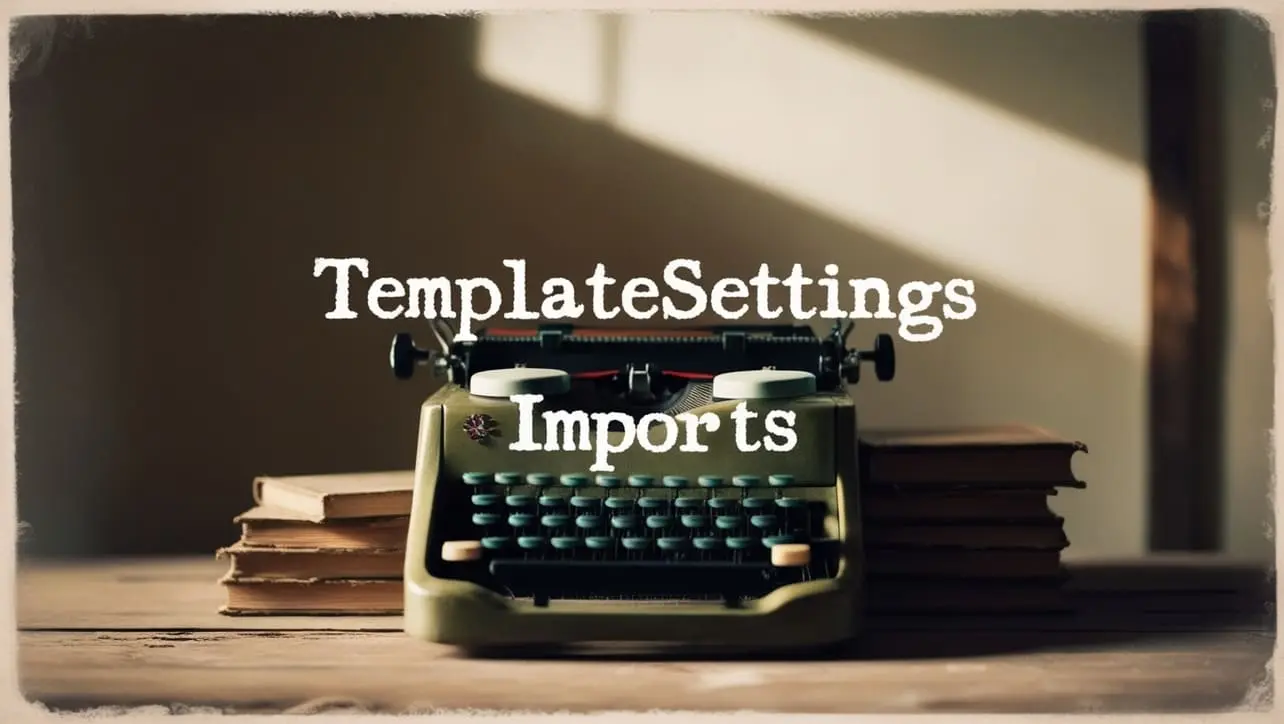
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.unzipWith() Array Method
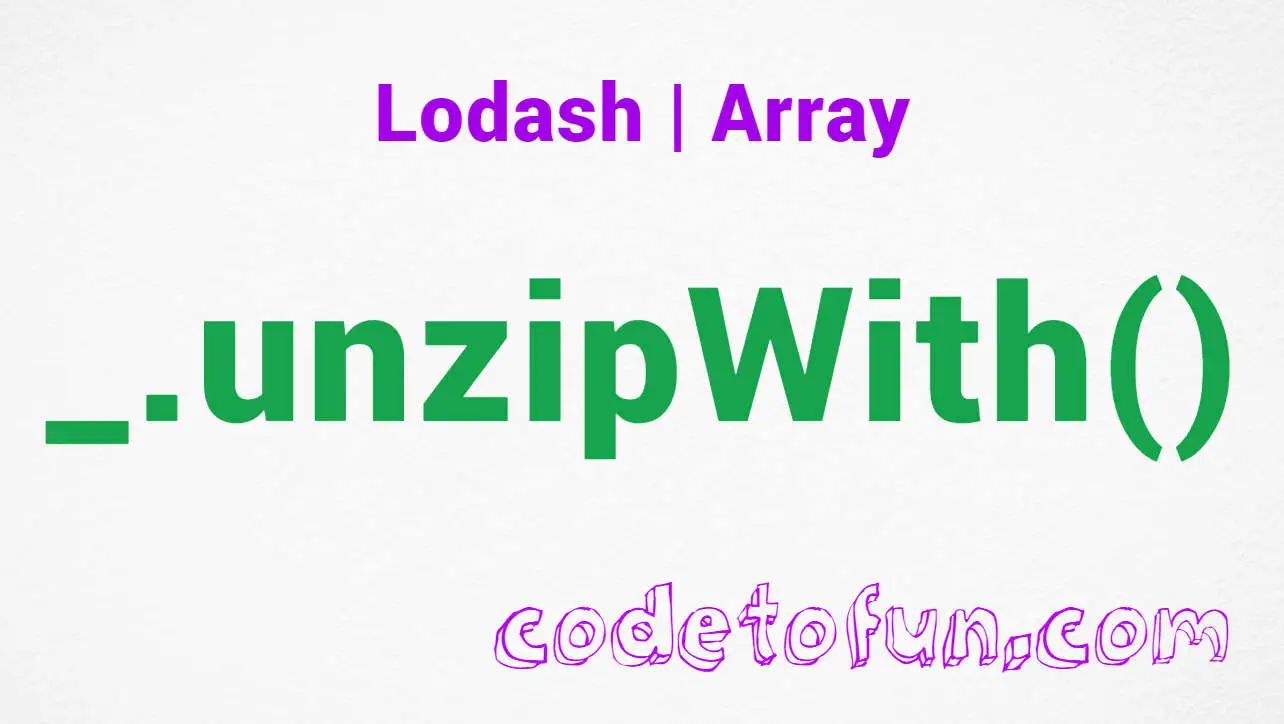
Photo Credit to CodeToFun
🙋 Introduction
Array manipulation in JavaScript often involves dealing with data in various formats. Lodash, a powerful utility library, provides developers with tools to streamline these tasks. Among its array manipulation arsenal is the _.unzipWith()
method, designed to transpose and combine arrays using a custom function.
This method offers flexibility and control, making it a valuable asset for developers working with diverse datasets.
🧠 Understanding _.unzipWith()
The _.unzipWith()
method in Lodash is designed to transform arrays by combining them based on a provided function. It works in tandem with _.zipWith(), allowing developers to unzip arrays back to their original structure using a custom iteratee function.
💡 Syntax
_.unzipWith(arrays, [iteratee])
- arrays: The array of arrays to process.
- iteratee: The function invoked per iteration.
📝 Example
Let's delve into a practical example to understand the functionality of _.unzipWith()
:
const _ = require('lodash');
const zippedArray = [[1, 4], [2, 5], [3, 6]];
const combinedArray = _.unzipWith(zippedArray, _.add);
console.log(combinedArray);
// Output: [6, 15]
In this example, the zippedArray is processed by _.unzipWith()
, combining the subarrays using the addition function (_.add), resulting in a new array.
🏆 Best Practices
Understanding Zipped Arrays:
Ensure that you are working with zipped arrays when using
_.unzipWith()
. Zipped arrays are typically obtained by using _.zipWith().example.jsCopiedconst array1 = [1, 2, 3]; const array2 = ['a', 'b', 'c']; const zippedArray = _.zipWith(array1, array2, _.concat); console.log(zippedArray); // Output: [[1, 'a'], [2, 'b'], [3, 'c']]
Custom Iteration Function:
Take advantage of the iteratee parameter to define a custom function that determines how the values should be combined during the unzipping process.
example.jsCopiedconst customIteratee = (value1, value2) => value1 * value2; const multipliedArray = _.unzipWith(zippedArray, customIteratee); console.log(multipliedArray); // Output: [1, 4, 9]
Handle Unequal Array Lengths:
Be aware that if the zipped arrays have different lengths, the resulting array will have a length equal to the shortest zipped array. Handle unequal array lengths according to your specific use case.
example.jsCopiedconst unevenZippedArray = [[1, 'a'], [2, 'b', '!'], [3, 'c']]; const combinedUnevenArray = _.unzipWith(unevenZippedArray, _.concat); console.log(combinedUnevenArray); // Output: [1, 'a', 2, 'b', 3, 'c']
📚 Use Cases
Data Transformation:
_.unzipWith()
is useful for transforming data, especially when dealing with arrays that represent different aspects of the same entities.example.jsCopiedconst dataArrays = [ [1, 'John', true], [2, 'Jane', false], [3, 'Doe', true], ]; const transformedData = _.unzipWith(dataArrays, (id, name, isActive) => ({ id, name, isActive })); console.log(transformedData); // Output: [{ id: 1, name: 'John', isActive: true }, { id: 2, name: 'Jane', isActive: false }, { id: 3, name: 'Doe', isActive: true }]
Custom Aggregation:
When you need to aggregate values from different arrays using a custom logic,
_.unzipWith()
allows you to specify the aggregation function.example.jsCopiedconst salesData = [[100, 150, 200], [120, 170, 210], [90, 130, 180]]; const totalSales = _.unzipWith(salesData, _.sum); console.log(totalSales); // Output: [310, 450, 590]
Dynamic Array Manipulation:
For scenarios where array structures are dynamic and need to be combined or transformed on-the-fly,
_.unzipWith()
offers a versatile solution.example.jsCopiedconst dynamicArrays = /* ...fetch arrays dynamically... */; const combinedDynamicArray = _.unzipWith(dynamicArrays, (value1, value2) => value1.concat(value2)); console.log(combinedDynamicArray);
🎉 Conclusion
The _.unzipWith()
method in Lodash provides a flexible and powerful mechanism for combining and transforming arrays based on a custom iteratee function. Whether you're dealing with zipped arrays, need to handle unequal lengths, or want to perform dynamic array manipulations, _.unzipWith()
empowers you to tailor your data manipulation according to your specific needs.
Explore the capabilities of _.unzipWith()
and enhance your array manipulation toolkit in JavaScript!
👨💻 Join our Community:
Author
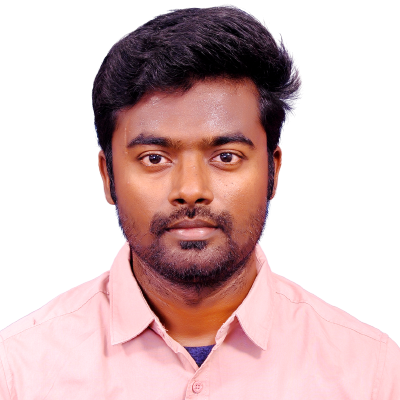
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
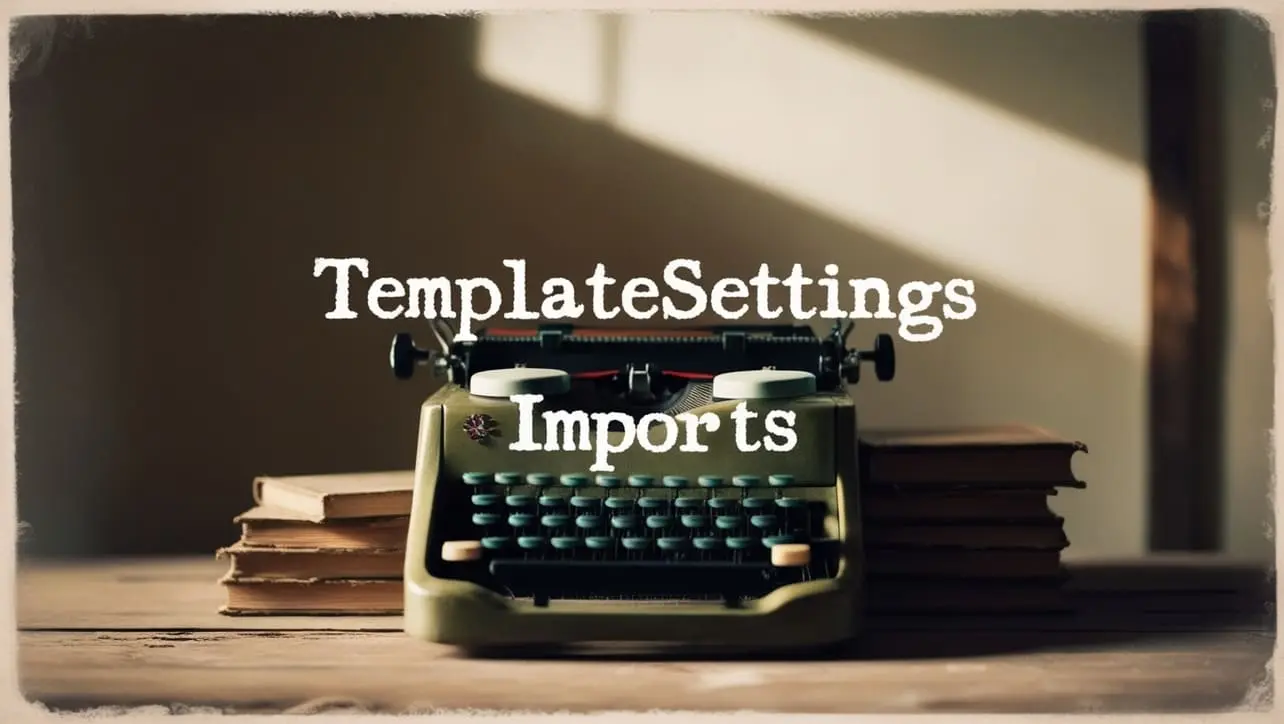
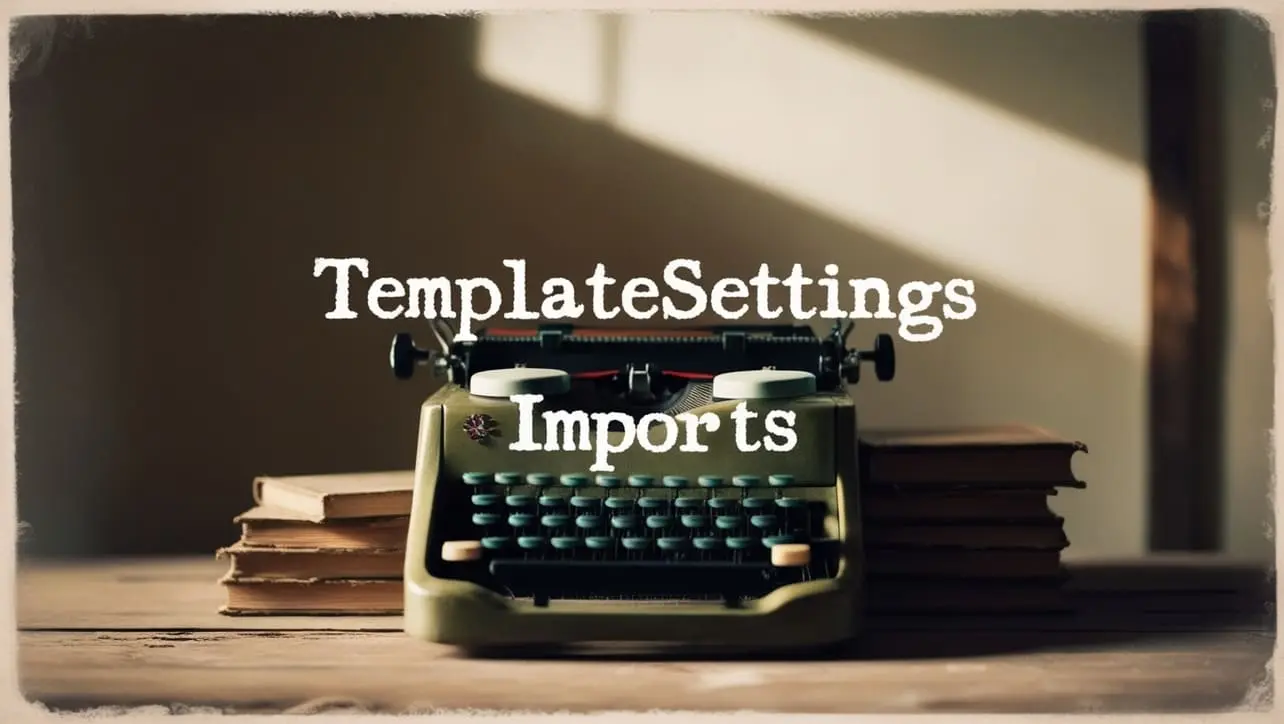
Lodash _.templateSettings.imports Property
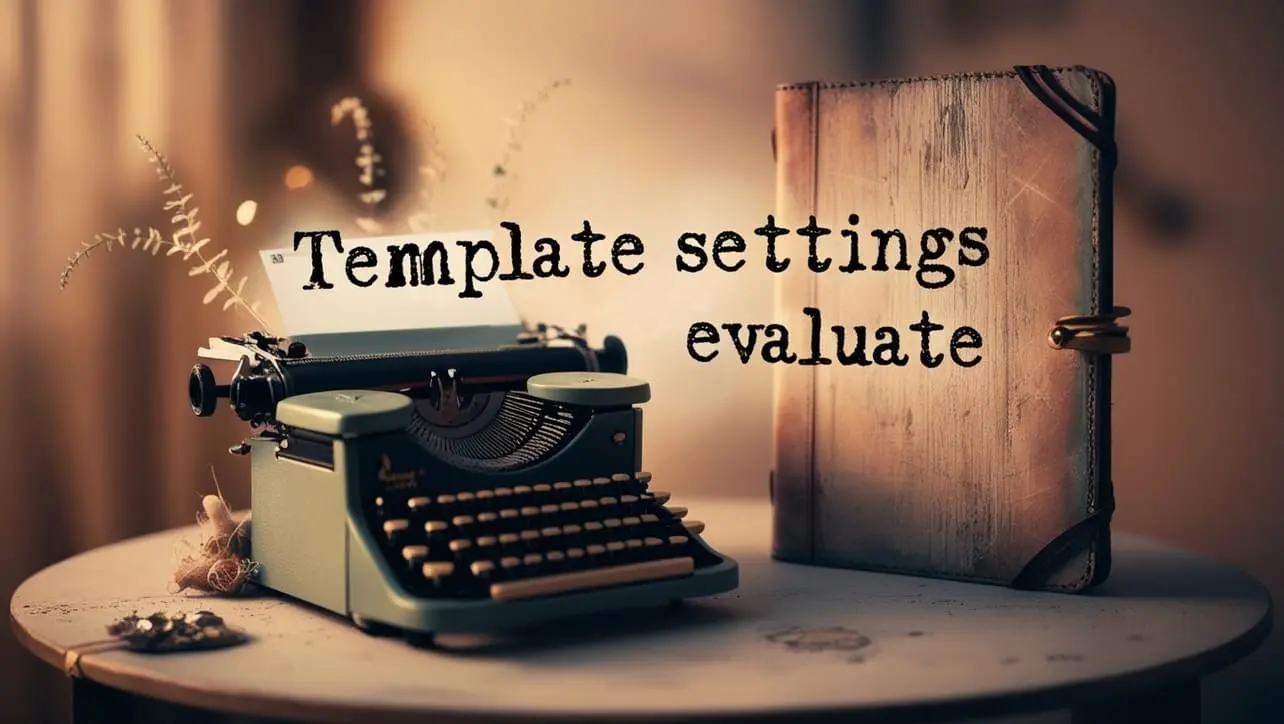
Lodash _.templateSettings.evaluate Property
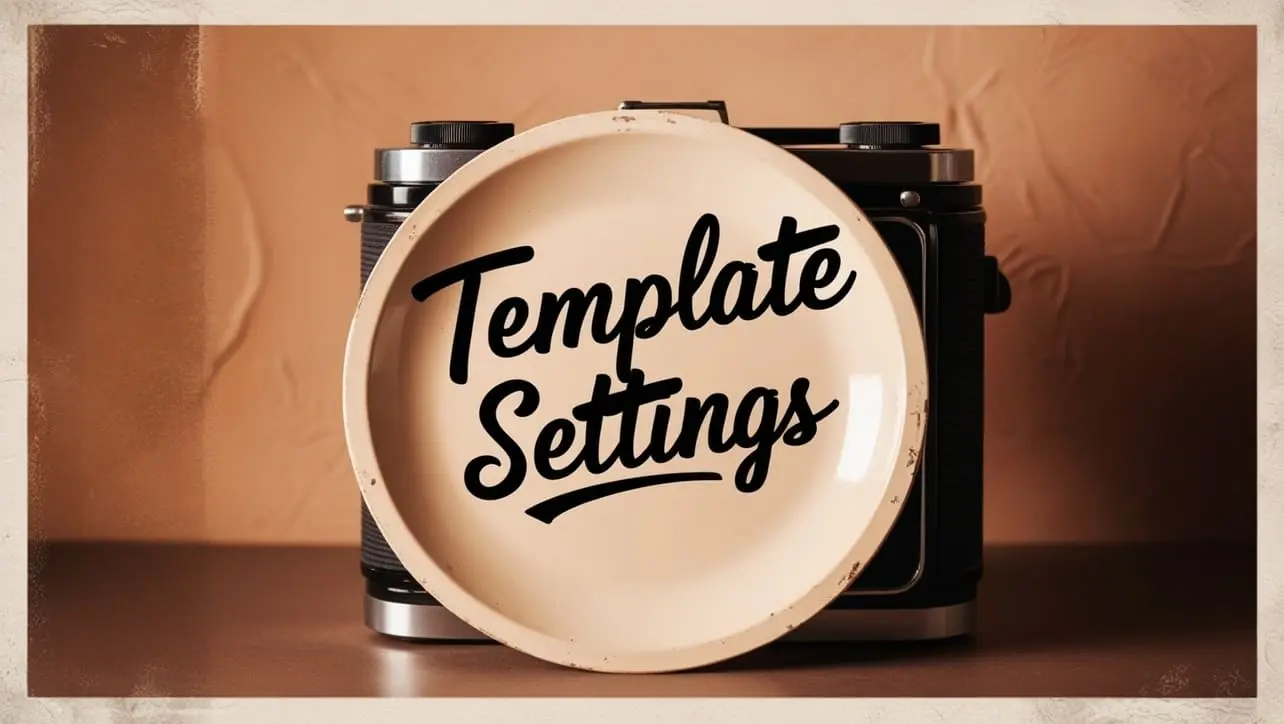
Lodash _.templateSettings Property
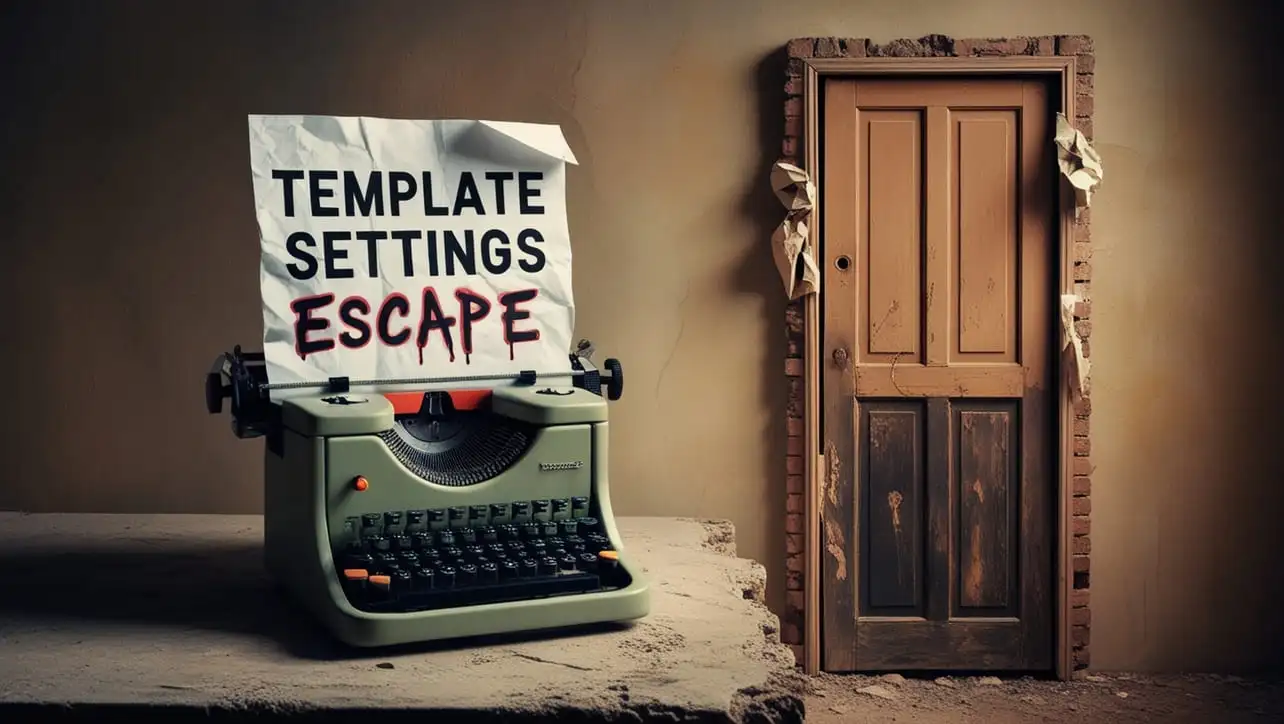
Lodash _.templateSettings.escape Property
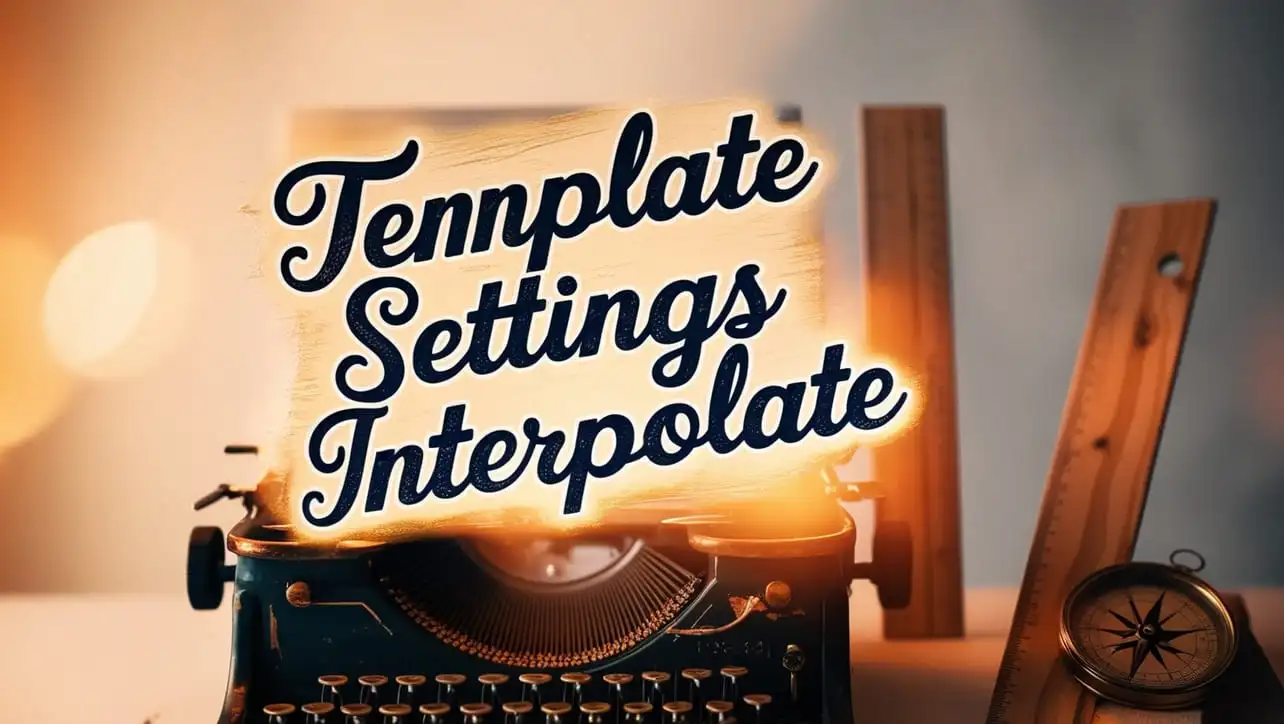
Lodash _.templateSettings.interpolate Property
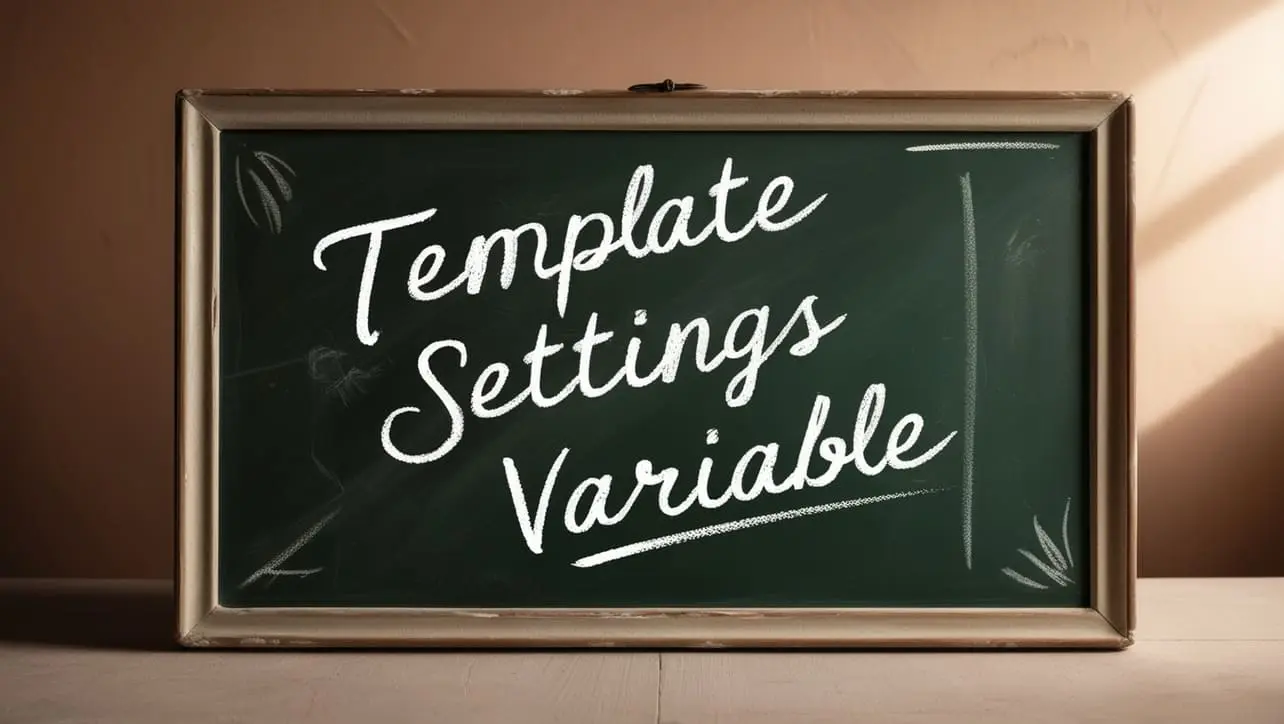
If you have any doubts regarding this article (Lodash _.unzipWith() Array Method), please comment here. I will help you immediately.