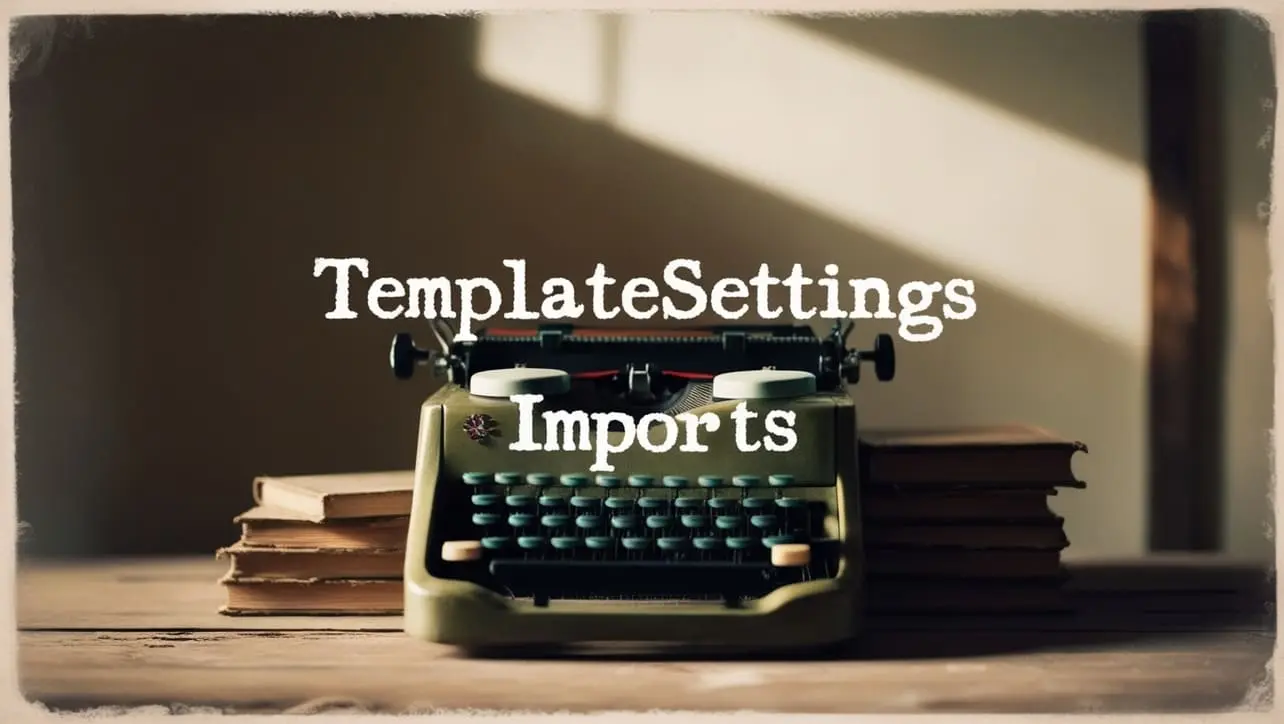
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.unzip() Array Method
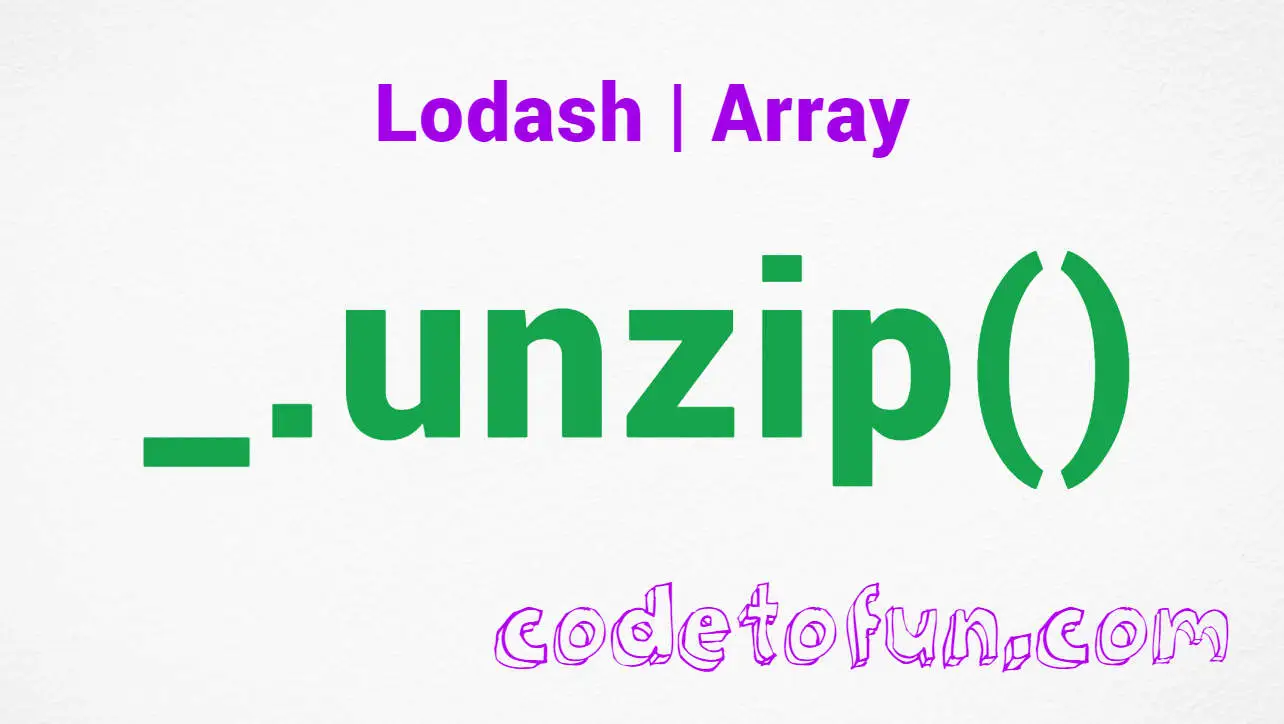
Photo Credit to CodeToFun
🙋 Introduction
Efficiently manipulating arrays is a common task in JavaScript development, and Lodash offers a range of utility functions to simplify these operations. Among them, the _.unzip()
method stands out, providing a convenient way to transpose arrays and restructure data.
This method proves invaluable when working with datasets that require reorganization or when handling parallel data representation.
🧠 Understanding _.unzip()
The _.unzip()
method in Lodash is designed to invert the effect of the _.zip() method. It takes an array of grouped elements (typically the result of a _.zip() operation) and transposes the data, returning a new array where the original groups become individual arrays. This operation is particularly useful in scenarios where data needs to be restructured for easier analysis or presentation.
💡 Syntax
_.unzip(array)
- array: The array to process.
📝 Example
Let's dive into a practical example to illustrate the functionality of _.unzip()
:
const _ = require('lodash');
const zippedData = [['John', 'Alice', 'Bob'], [25, 28, 22], ['Engineer', 'Designer', 'Developer']];
const unzippedData = _.unzip(zippedData);
console.log(unzippedData);
/*
Output:
[
['John', 25, 'Engineer'],
['Alice', 28, 'Designer'],
['Bob', 22, 'Developer']
]
*/
In this example, the zippedData array, created using _.zip(), is transposed back to its original structure using _.unzip()
.
🏆 Best Practices
Use with _.zip():
Pair
_.unzip()
with _.zip() for effective data transformation. These methods work seamlessly together, allowing you to structure and destructure data with ease.example.jsCopiedconst _ = require('lodash'); const originalData = [['A', 'B', 'C'], [1, 2, 3], ['x', 'y', 'z']]; // Zip the original data const zippedData = _.zip(...originalData); console.log(zippedData); /* Output: [ ['A', 1, 'x'], ['B', 2, 'y'], ['C', 3, 'z'] ] */ // Unzip the zipped data const unzippedData = _.unzip(zippedData); console.log(unzippedData); /* Output: [ ['A', 'B', 'C'], [1, 2, 3], ['x', 'y', 'z'] ] */
Handle Varying Array Lengths:
Be mindful of the lengths of the arrays within the input array.
_.unzip()
assumes equal lengths, so unexpected results may occur if the subarrays have different lengths.example.jsCopiedconst _ = require('lodash'); const unevenData = [['A', 'B', 'C'], [1, 2], ['x', 'y', 'z']]; const unzippedUnevenData = _.unzip(unevenData); console.log(unzippedUnevenData); /* Output: [ ['A', 1, 'x'], ['B', 2, 'y'], ['C', undefined, 'z'] // Note: undefined is added for the missing element ] */
Leverage in Data Transformation:
Use
_.unzip()
as part of a broader data transformation strategy. Combine it with other Lodash methods or native JavaScript functions to achieve the desired structure for your data.example.jsCopiedconst _ = require('lodash'); const originalData = [ { name: 'John', age: 25, role: 'Engineer' }, { name: 'Alice', age: 28, role: 'Designer' }, { name: 'Bob', age: 22, role: 'Developer' }, ]; // Extract values from objects const extractedData = originalData.map(({ name, age, role }) => [name, age, role]); console.log(extractedData); /* Output: [ ['John', 25, 'Engineer'], ['Alice', 28, 'Designer'], ['Bob', 22, 'Developer'] ] */ // Unzip the extracted data const unzippedData = _.unzip(extractedData); console.log(unzippedData); /* Output: [ ['John', 'Alice', 'Bob'], [25, 28, 22], ['Engineer', 'Designer', 'Developer'] ] */
📚 Use Cases
Data Restructuring:
_.unzip()
is ideal for restructuring data, especially when dealing with arrays of values that need to be transformed into a more meaningful format.example.jsCopiedconst _ = require('lodash'); const originalData = [['Name', 'John', 'Alice', 'Bob'], ['Age', 25, 28, 22]]; // Unzip the data for better readability const unzippedData = _.unzip(originalData); console.log(unzippedData); /* Output: [ ['Name', 'Age'], ['John', 25], ['Alice', 28], ['Bob', 22] ] */
Parallel Data Representation:
When working with parallel arrays,
_.unzip()
allows you to transform the data for more straightforward processing and analysis.example.jsCopiedconst _ = require('lodash'); const parallelData = [ ['John', 'Alice', 'Bob'], [25, 28, 22], ['Engineer', 'Designer', 'Developer'] ]; // Unzip the parallel data for individual analysis const individualData = _.unzip(parallelData); console.log(individualData); /* Output: [ ['John', 25, 'Engineer'], ['Alice', 28, 'Designer'], ['Bob', 22, 'Developer'] ] */
Dynamic Data Handling:
When dealing with dynamic data where the structure is not known beforehand,
_.unzip()
can be used to adapt to varying data shapes.example.jsCopiedconst _ = require('lodash'); const dynamicData = [ ['A', 1, 'x'], ['B', 2, 'y'], ['C', 3, 'z'] ]; // Unzip dynamically shaped data const unzippedDynamicData = _.unzip(dynamicData); console.log(unzippedDynamicData); /* Output: [ ['A', 'B', 'C'], [1, 2, 3], ['x', 'y', 'z'] ] */
🎉 Conclusion
The _.unzip()
method in Lodash provides a powerful means of transposing and restructuring arrays, offering flexibility in data manipulation. Whether you're reorganizing datasets, handling parallel data, or dynamically adapting to varying data shapes, _.unzip()
proves to be a versatile tool in your JavaScript development toolkit.
Explore the capabilities of _.unzip()
and elevate your array manipulation tasks with ease!
👨💻 Join our Community:
Author
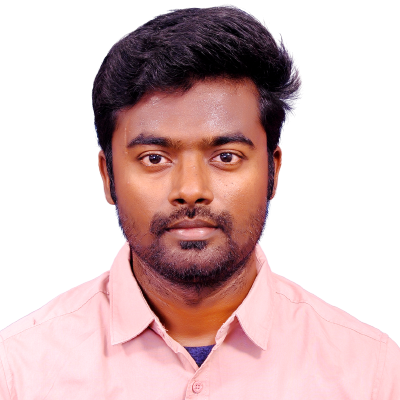
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
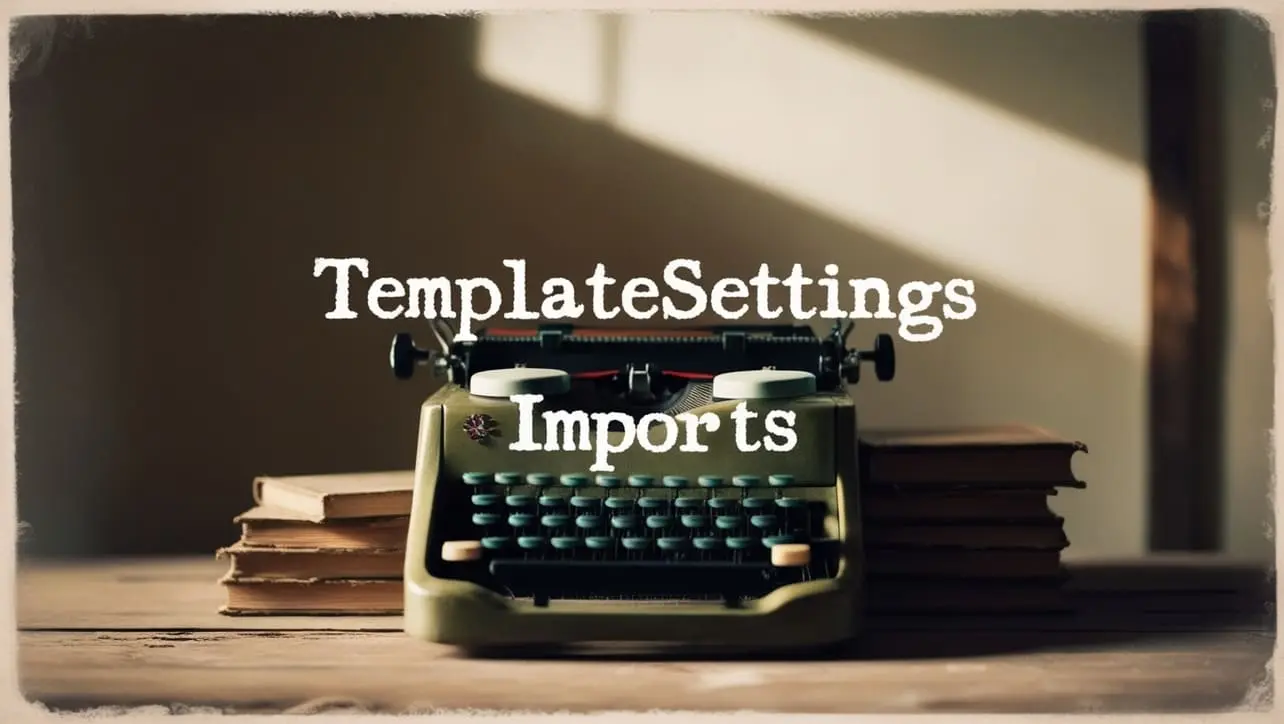
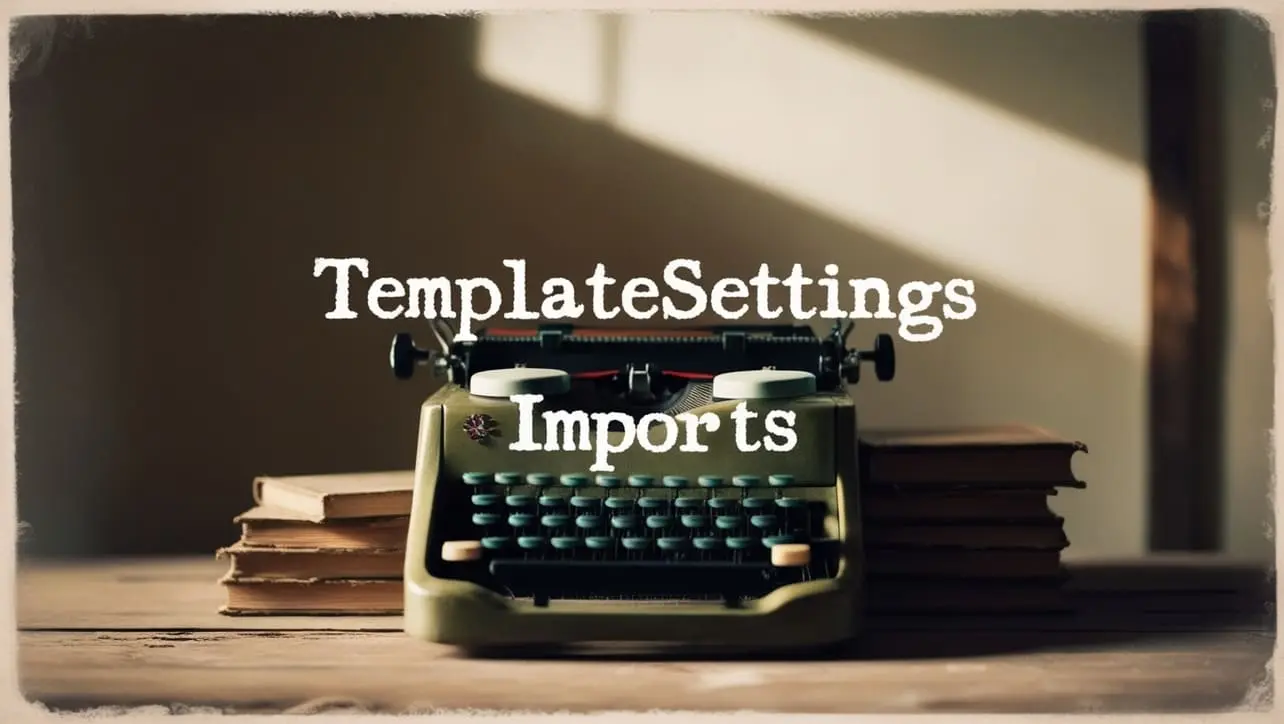
Lodash _.templateSettings.imports Property
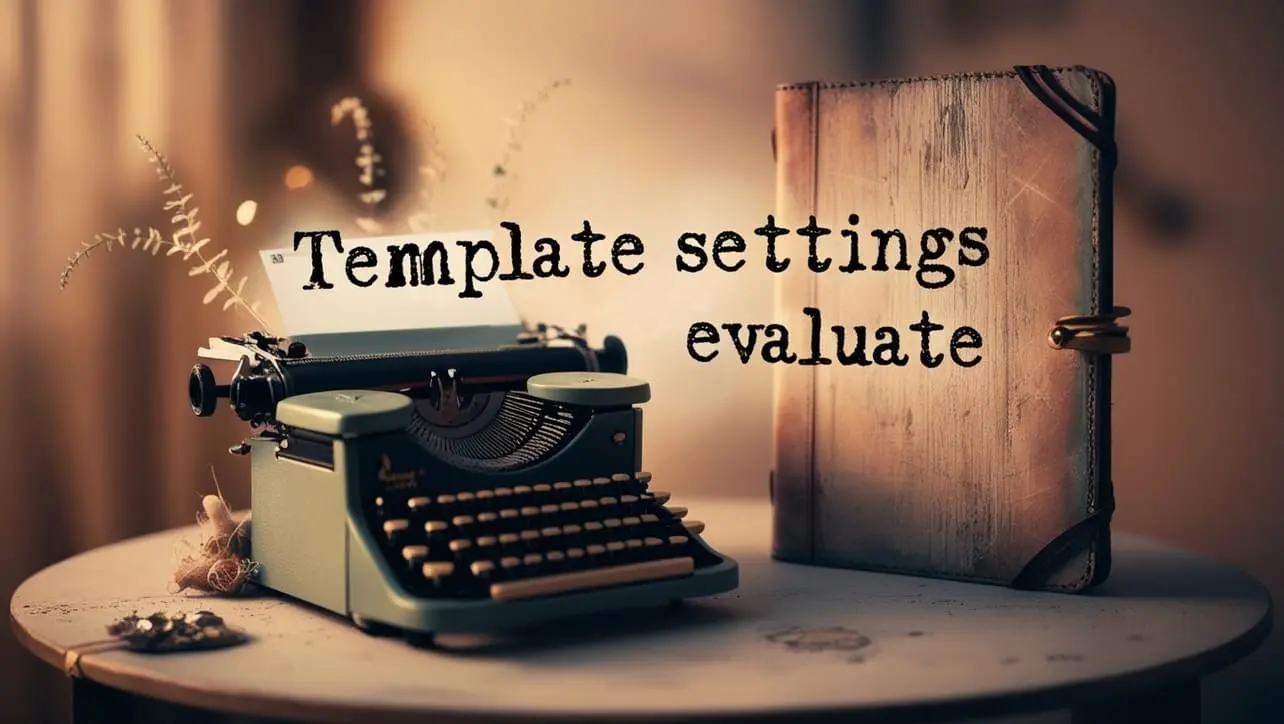
Lodash _.templateSettings.evaluate Property
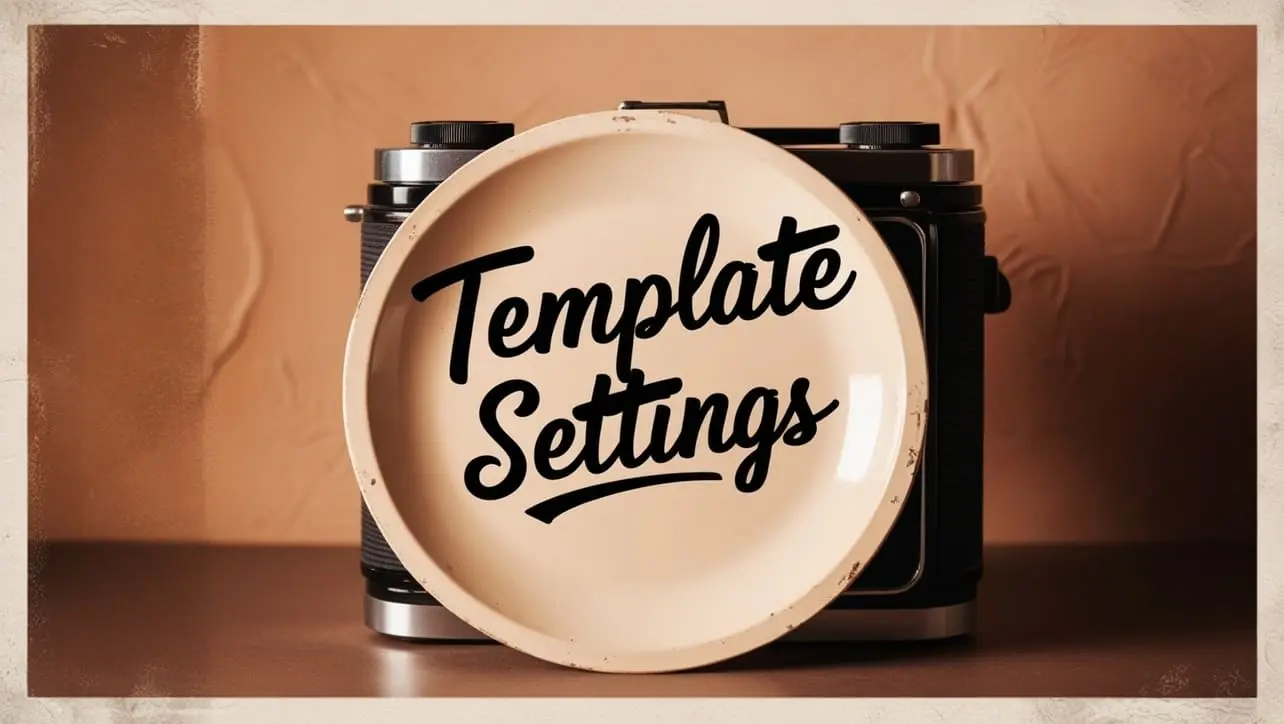
Lodash _.templateSettings Property
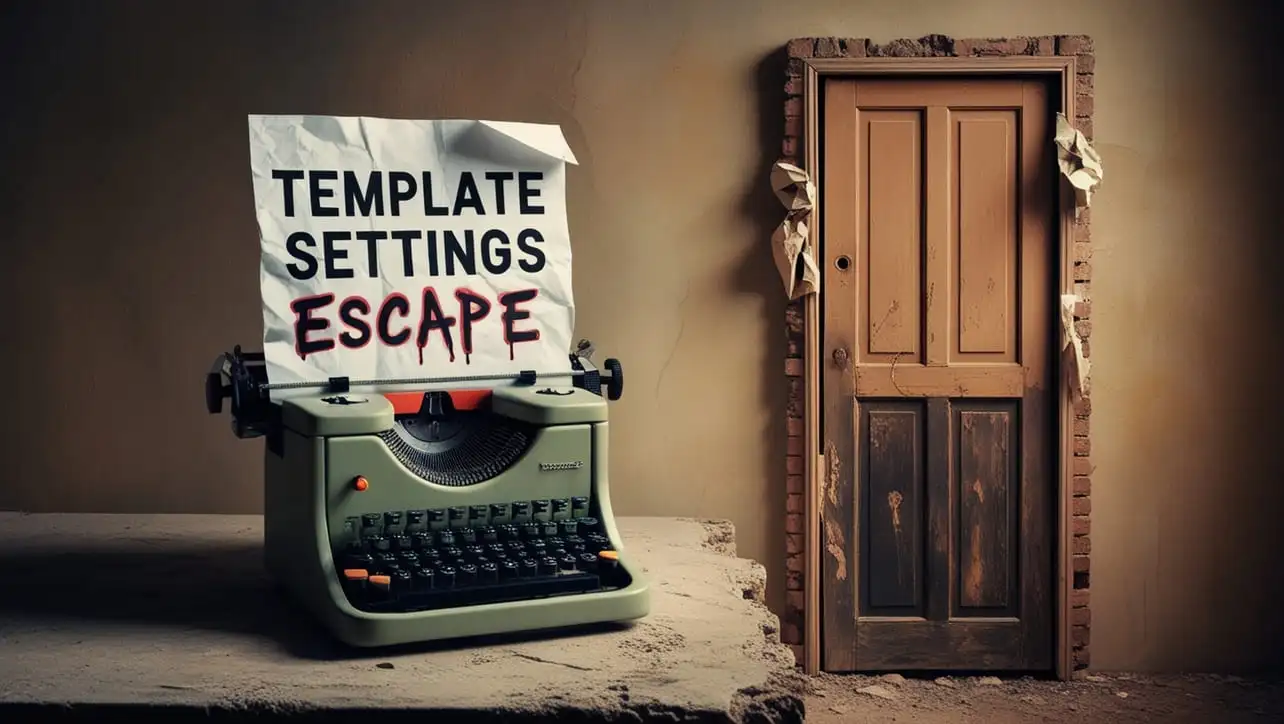
Lodash _.templateSettings.escape Property
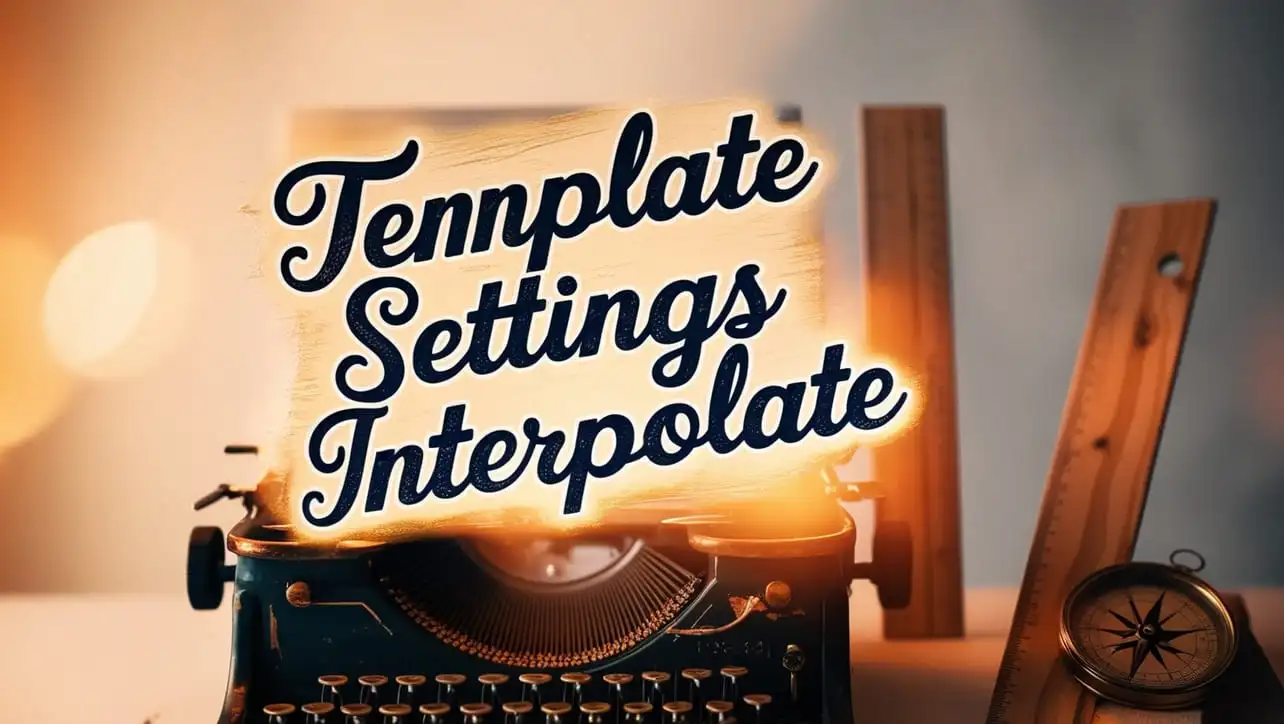
Lodash _.templateSettings.interpolate Property
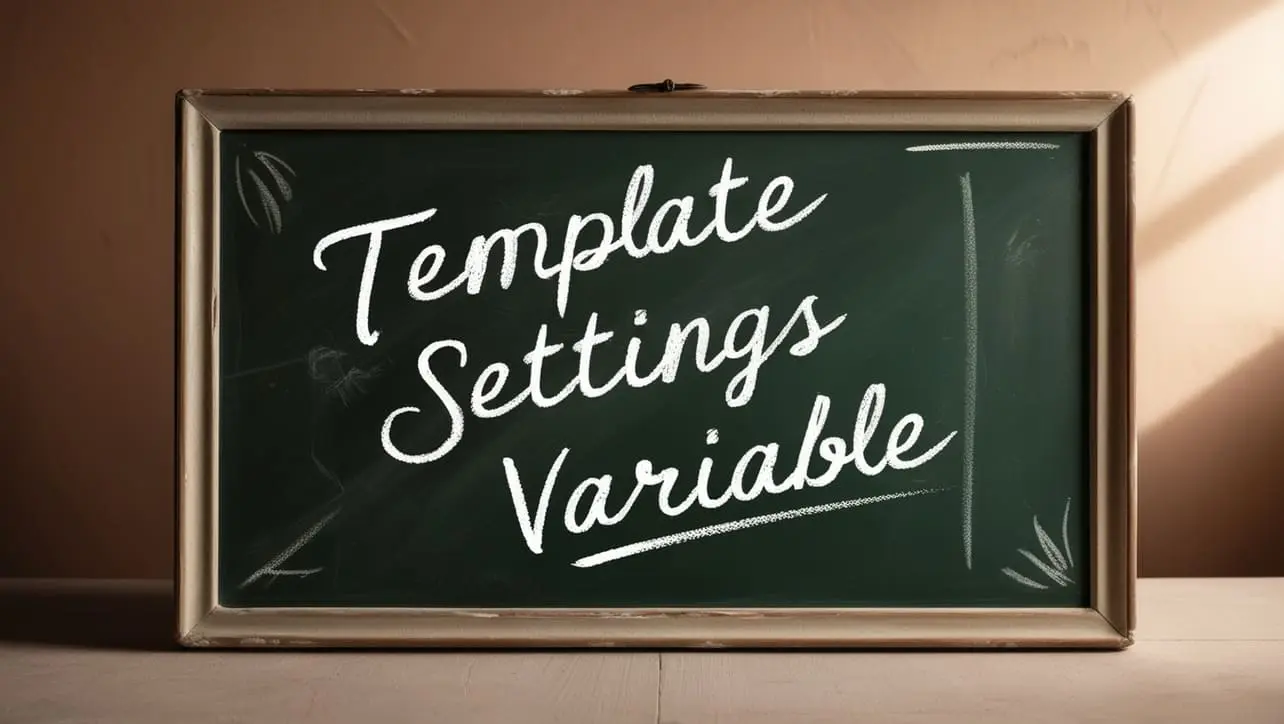
If you have any doubts regarding this article (Lodash _.unzip() Array Method), please comment here. I will help you immediately.