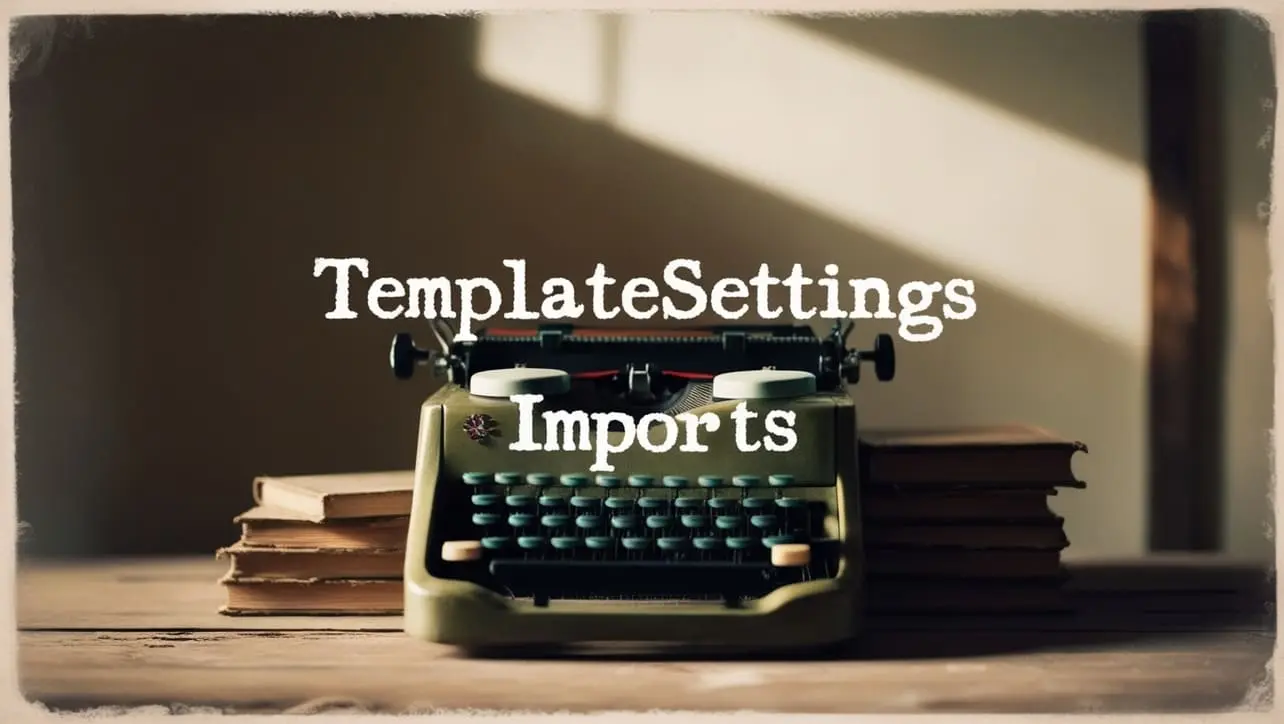
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.uniq() Array Method
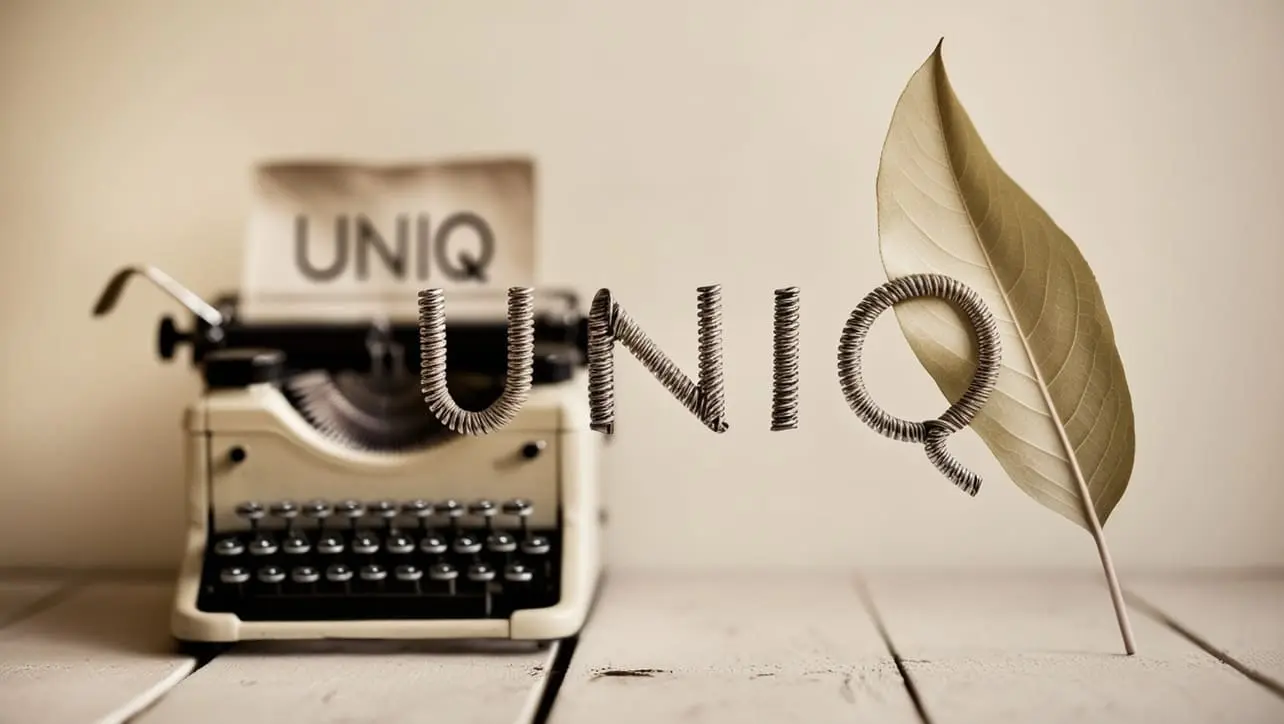
Photo Credit to CodeToFun
🙋 Introduction
Efficiently managing arrays is a fundamental aspect of JavaScript development. Lodash, a powerful utility library, offers a wealth of functions to simplify array manipulation, and among them is the _.uniq()
method.
This method is designed to create a unique copy of an array, removing duplicate values and providing developers with a versatile tool for enhancing data integrity and simplifying array processing.
🧠 Understanding _.uniq()
The _.uniq()
method in Lodash is a straightforward yet powerful tool for obtaining a unique version of an array. It eliminates duplicate values, leaving behind only distinct elements. This can be particularly useful when working with datasets where uniqueness is crucial.
💡 Syntax
_.uniq(array)
- array: The array to process.
📝 Example
Let's delve into a practical example to understand the functionality of _.uniq()
:
const _ = require('lodash');
const arrayWithDuplicates = [1, 2, 2, 3, 4, 4, 5, 6];
const uniqueArray = _.uniq(arrayWithDuplicates);
console.log(uniqueArray);
// Output: [1, 2, 3, 4, 5, 6]
In this example, the arrayWithDuplicates is processed by _.uniq()
, resulting in a new array containing only unique elements.
🏆 Best Practices
Understand Data Types:
Be aware of the data types within your array.
_.uniq()
performs a strict equality check, so elements with different types will be considered distinct. Consider pre-processing your data if you need more nuanced comparison.example.jsCopiedconst mixedTypeArray = [1, '1', 2, '2', 3, '3']; const uniqueMixedTypeArray = _.uniq(mixedTypeArray); console.log(uniqueMixedTypeArray); // Output: [1, '1', 2, '2', 3, '3']
Preserve Original Order:
By default,
_.uniq()
retains the first occurrence of each unique element and discards subsequent duplicates. If preserving the original order is essential, consider using the _.sortedUniq() method.example.jsCopiedconst arrayWithOrderDuplicates = [3, 1, 2, 1, 4, 3, 5, 2]; const uniqueArrayWithOrder = _.uniq(arrayWithOrderDuplicates); console.log(uniqueArrayWithOrder); // Output: [3, 1, 2, 4, 5]
Case Sensitivity:
For arrays containing string elements, note that
_.uniq()
is case-sensitive. If case-insensitive uniqueness is required, consider converting all strings to a consistent case before using the method.example.jsCopiedconst caseSensitiveArray = ['apple', 'Orange', 'Banana', 'orange', 'banana']; const uniqueCaseSensitiveArray = _.uniq(caseSensitiveArray); console.log(uniqueCaseSensitiveArray); // Output: ['apple', 'Orange', 'Banana', 'orange', 'banana']
📚 Use Cases
Simplifying Data Processing:
The primary use case for
_.uniq()
is simplifying data processing by removing duplicate values. This is particularly useful when dealing with user input or datasets prone to redundancies.example.jsCopiedconst userPreferences = ['dark', 'light', 'dark', 'sepia', 'light']; const uniquePreferences = _.uniq(userPreferences); console.log(uniquePreferences); // Output: ['dark', 'light', 'sepia']
Filtering Unique Values:
When you need to filter out unique values from a larger dataset,
_.uniq()
provides a concise solution.example.jsCopiedconst allColors = ['red', 'blue', 'green', 'red', 'yellow', 'blue']; const uniqueColors = _.uniq(allColors); console.log(uniqueColors); // Output: ['red', 'blue', 'green', 'yellow']
Improving Search Efficiency:
For arrays used in search operations or when checking the presence of specific values, using
_.uniq()
can optimize the process by eliminating unnecessary duplicates.example.jsCopiedconst searchKeywords = ['javascript', 'html', 'css', 'javascript', 'python']; const uniqueKeywords = _.uniq(searchKeywords); console.log(uniqueKeywords); // Output: ['javascript', 'html', 'css', 'python']
🎉 Conclusion
The _.uniq()
method in Lodash provides a convenient solution for obtaining unique arrays, simplifying data processing and enhancing array manipulation in JavaScript. Whether you need to streamline datasets, improve search efficiency, or filter out redundancies, _.uniq()
offers a versatile and efficient tool for array uniqueness.
Explore the capabilities of _.uniq()
and elevate your JavaScript development experience!
👨💻 Join our Community:
Author
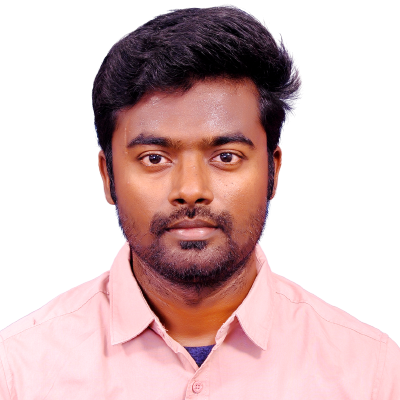
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
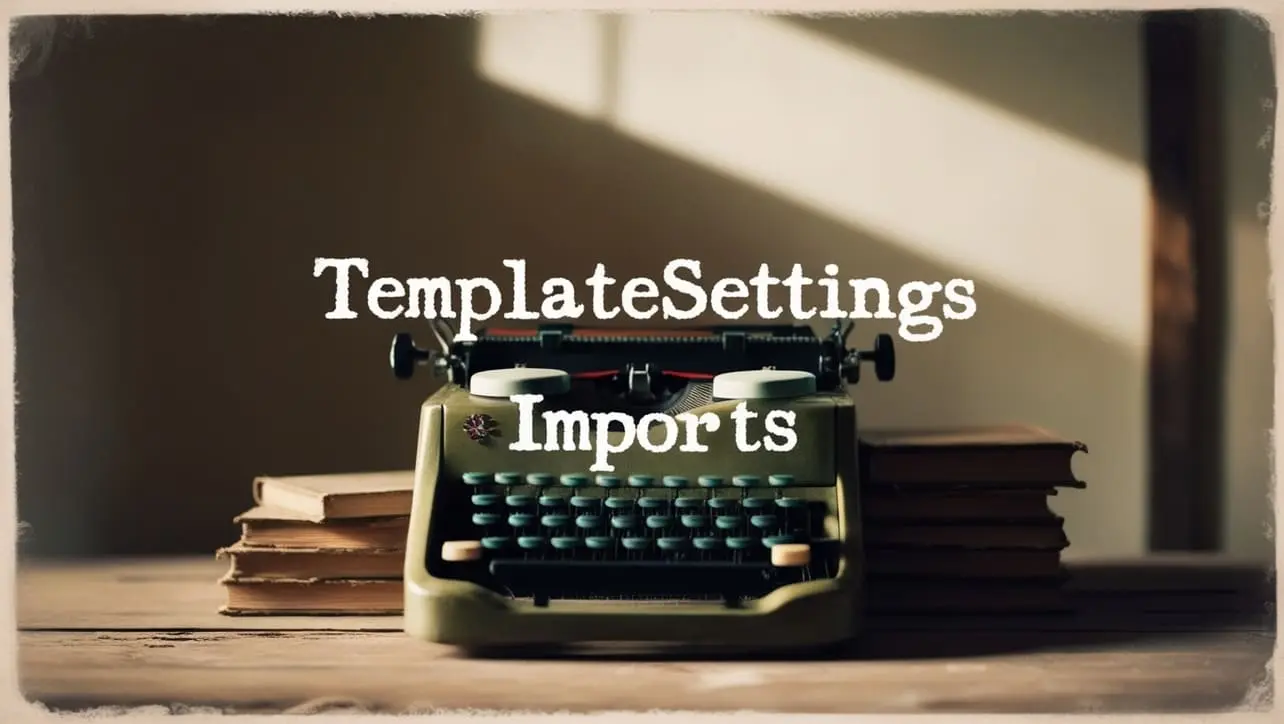
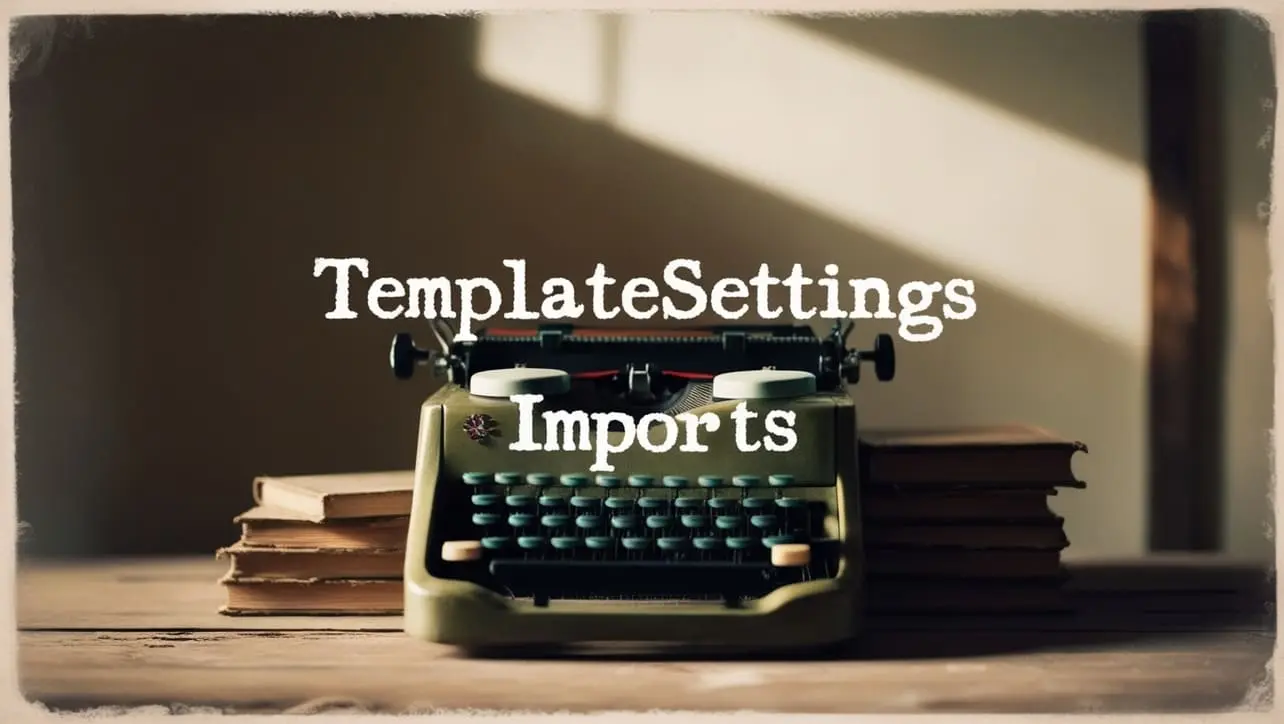
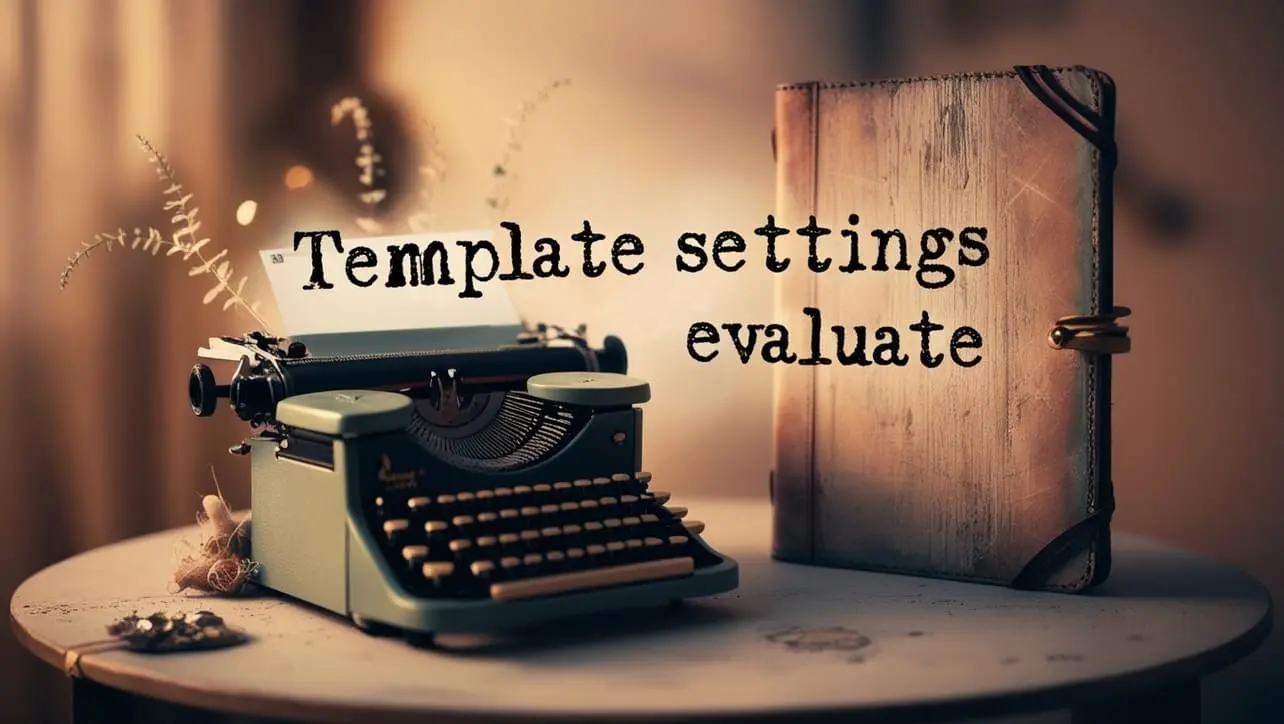
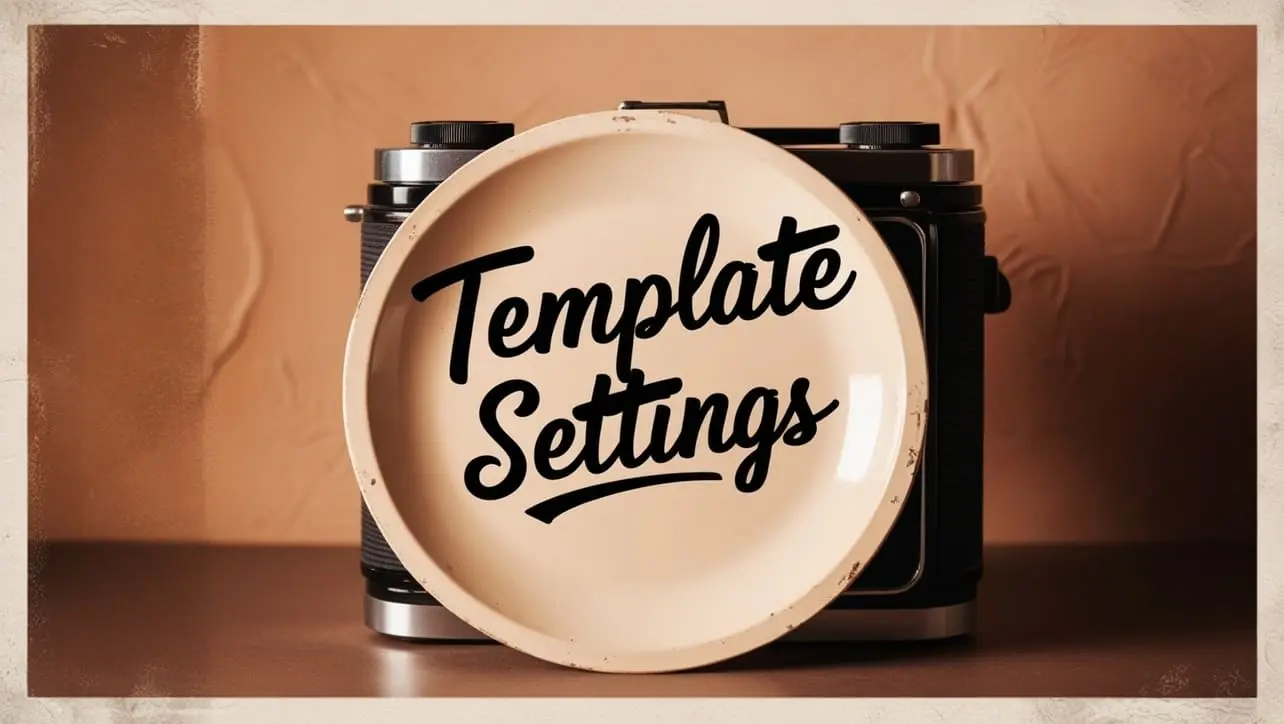
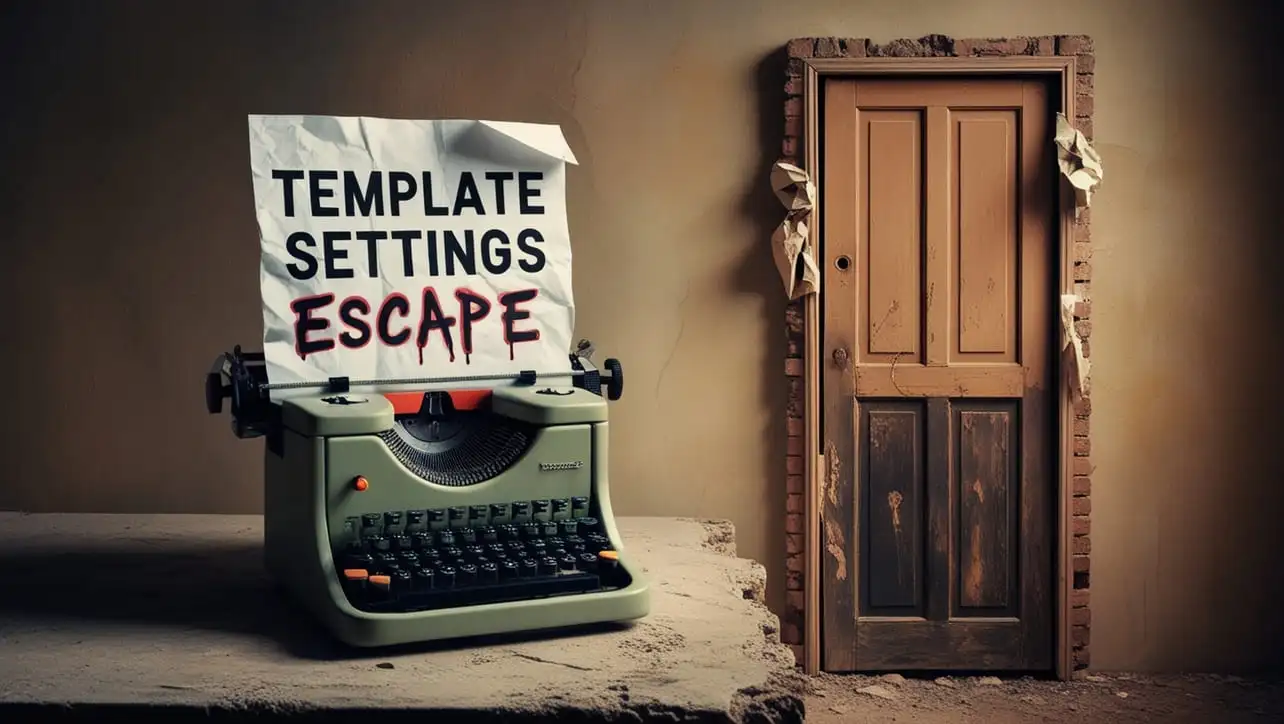
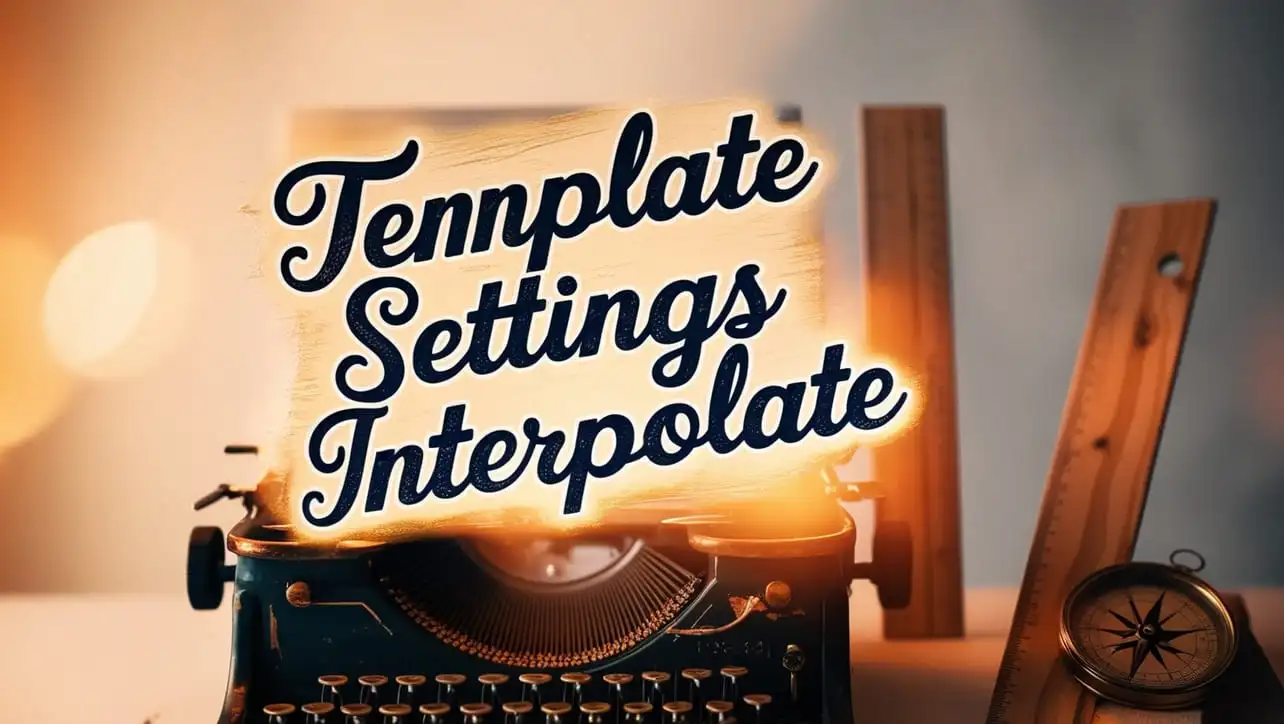
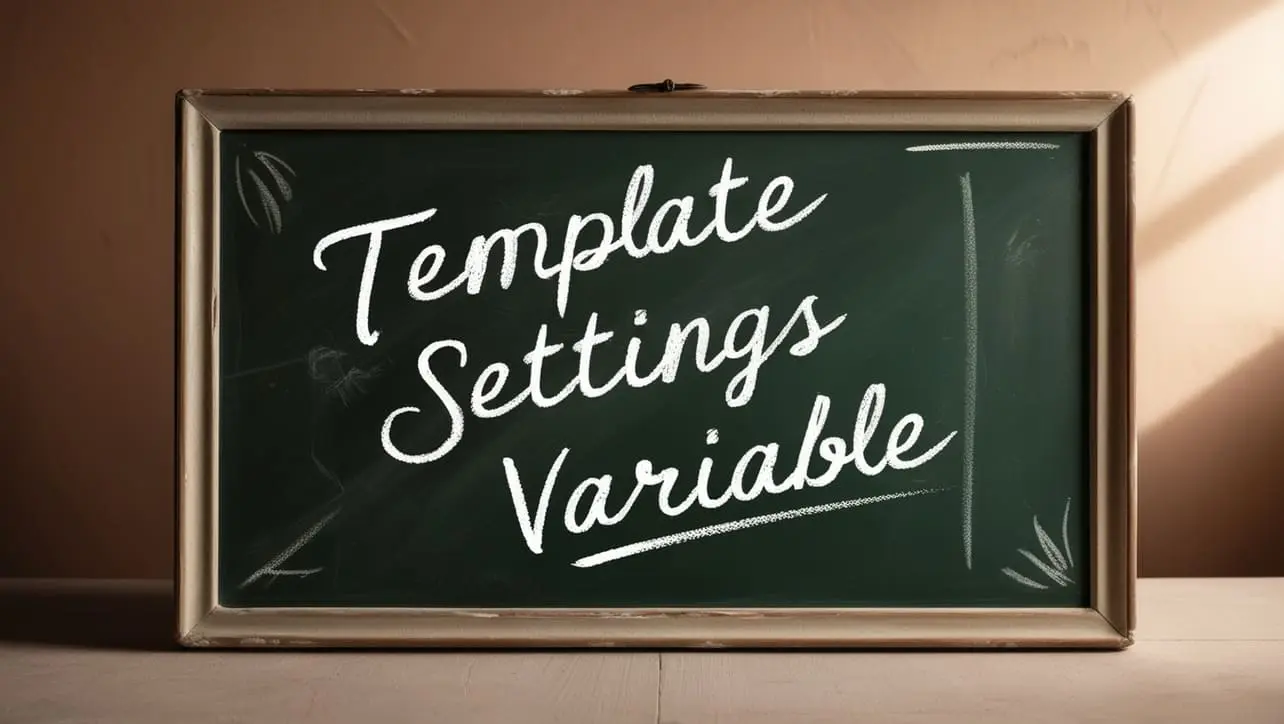
If you have any doubts regarding this article (Lodash _.uniq() Array Method), please comment here. I will help you immediately.