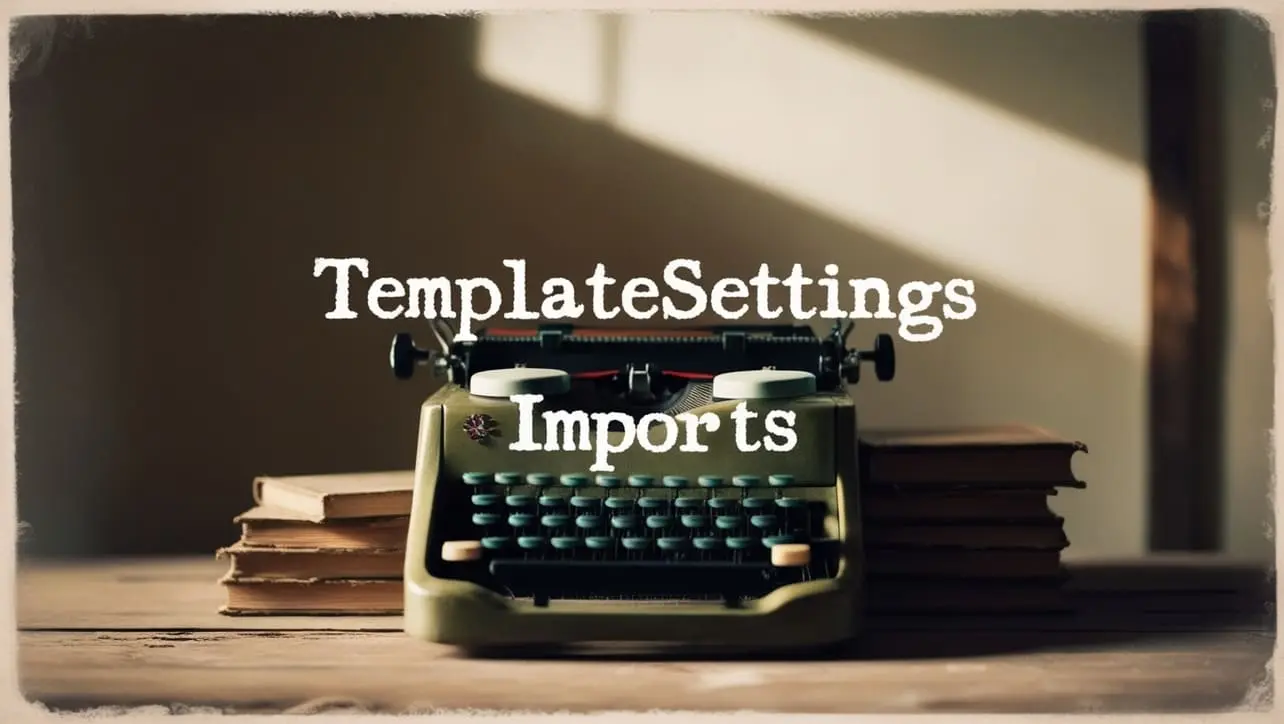
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.unionWith() Array Method
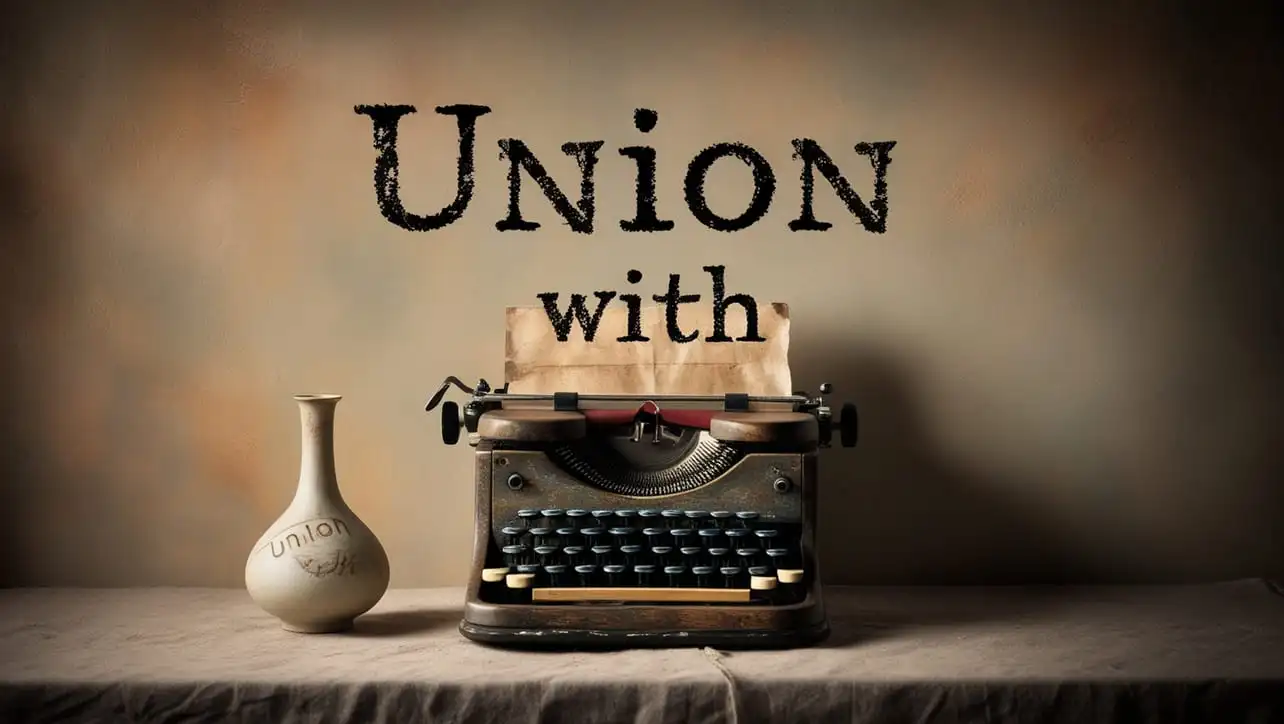
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, effective array manipulation is a fundamental skill. Lodash, a feature-rich utility library, provides developers with a multitude of tools for streamlined coding. One such tool is the _.unionWith()
method, designed to create a union of arrays using a custom comparator function.
This method proves invaluable when merging arrays with complex or custom objects, allowing for precise control over the merging process.
🧠 Understanding _.unionWith()
The _.unionWith()
method in Lodash serves the purpose of combining multiple arrays into a single array, using a custom comparator function to determine the uniqueness of elements. This enables developers to merge arrays in a way that goes beyond simple value comparisons, accommodating more intricate objects and structures.
💡 Syntax
_.unionWith([arrays], [comparator])
- arrays: The arrays to process.
- comparator: The function invoked per comparison.
📝 Example
Let's explore a practical example to illustrate the functionality of _.unionWith()
:
const _ = require('lodash');
const array1 = [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }];
const array2 = [{ id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }];
const array3 = [{ id: 4, name: 'David' }];
const mergedArray = _.unionWith(array1, array2, array3, _.isEqual);
console.log(mergedArray);
// Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }, { id: 4, name: 'David' }]
In this example, array1, array2, and array3 are merged into a new array, ensuring uniqueness based on the custom comparator function _.isEqual.
🏆 Best Practices
Understanding Comparator Function:
Comprehend the role of the comparator function in determining the uniqueness of elements. The comparator function is essential for customizing the comparison logic.
example.jsCopiedconst customComparator = (arrValue, otherValue) => arrValue.id === otherValue.id; const mergedArray = _.unionWith(array1, array2, array3, customComparator); console.log(mergedArray); // Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }, { id: 4, name: 'David' }]
Handling Complex Objects:
When dealing with arrays containing complex objects, ensure that the comparator function accounts for the specific properties that define uniqueness.
example.jsCopiedconst complexObjectComparator = (arrValue, otherValue) => arrValue.id === otherValue.id && arrValue.name === otherValue.name; const mergedArray = _.unionWith(array1, array2, array3, complexObjectComparator); console.log(mergedArray); // Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }, { id: 4, name: 'David' }]
Array Order Preservation:
Be aware that
_.unionWith()
preserves the order of elements from the original arrays. If maintaining the order is crucial, this method is a suitable choice.example.jsCopiedconst orderedArray1 = [1, 2, 3]; const orderedArray2 = [3, 4, 5]; const mergedOrderedArray = _.unionWith(orderedArray1, orderedArray2, _.isEqual); console.log(mergedOrderedArray); // Output: [1, 2, 3, 4, 5]
📚 Use Cases
Merging Arrays with Custom Objects:
_.unionWith()
shines when merging arrays containing custom objects, allowing developers to define the criteria for uniqueness.example.jsCopiedconst userArray1 = [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }]; const userArray2 = [{ id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }]; const userArray3 = [{ id: 4, name: 'David' }]; const mergedUserArray = _.unionWith(userArray1, userArray2, userArray3, _.isEqual); console.log(mergedUserArray); // Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }, { id: 4, name: 'David' }]
Handling Arrays with Overlapping Elements:
When dealing with arrays that share overlapping elements,
_.unionWith()
ensures that duplicates are eliminated based on the custom comparator.example.jsCopiedconst overlappingArray1 = [1, 2, 3]; const overlappingArray2 = [3, 4, 5]; const mergedUniqueArray = _.unionWith(overlappingArray1, overlappingArray2, _.isEqual); console.log(mergedUniqueArray); // Output: [1, 2, 3, 4, 5]
Preserving Array Order:
In scenarios where preserving the order of elements is crucial,
_.unionWith()
provides a solution by maintaining the order from the original arrays.example.jsCopiedconst orderedArrayA = ['apple', 'orange', 'banana']; const orderedArrayB = ['banana', 'kiwi', 'apple']; const mergedOrderedFruits = _.unionWith(orderedArrayA, orderedArrayB, _.isEqual); console.log(mergedOrderedFruits); // Output: ['apple', 'orange', 'banana', 'kiwi']
🎉 Conclusion
The _.unionWith()
method in Lodash offers a powerful mechanism for merging arrays while providing developers with the flexibility to define custom comparison logic. Whether dealing with complex objects, overlapping elements, or specific order preservation requirements, _.unionWith()
is a versatile tool for array manipulation in JavaScript.
Harness the capabilities of _.unionWith()
to enhance your array merging processes and elevate your JavaScript development experience!
👨💻 Join our Community:
Author
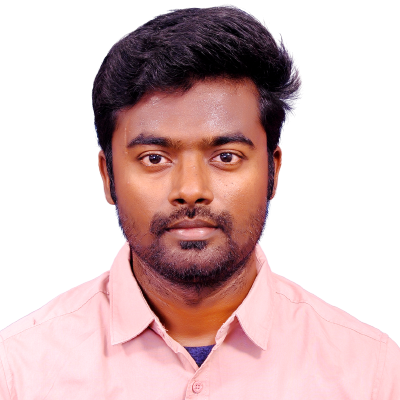
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
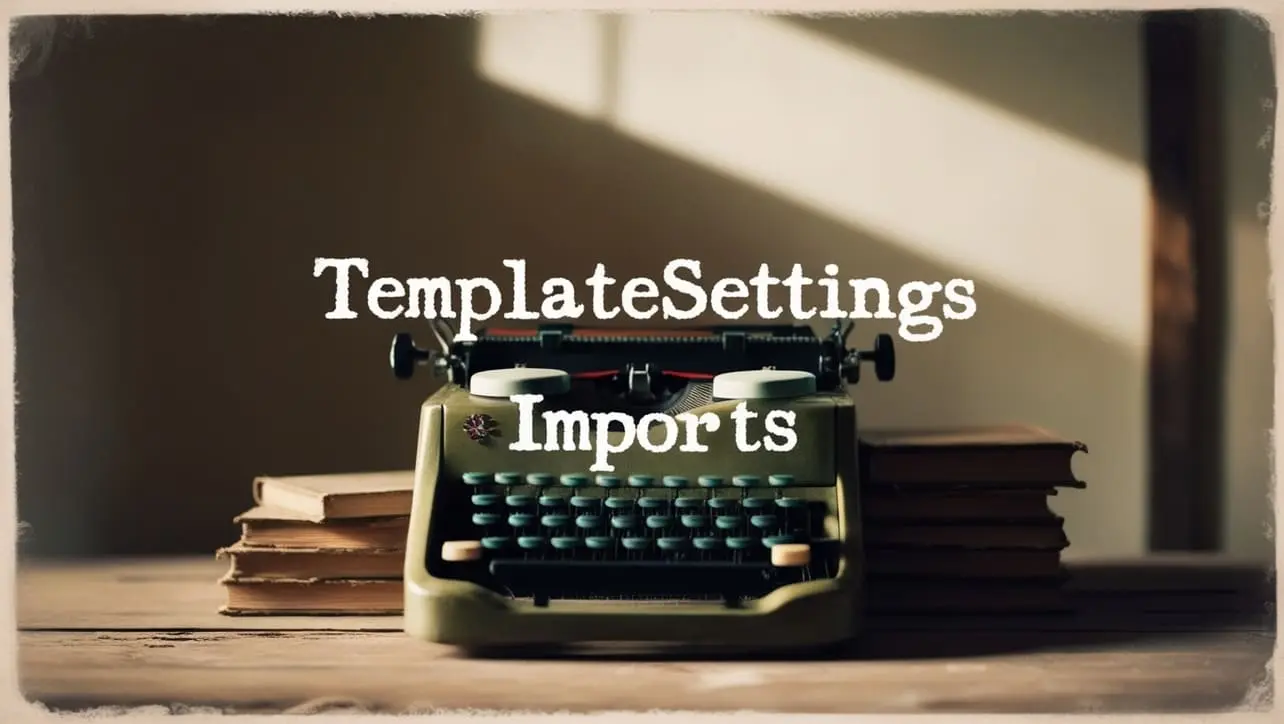
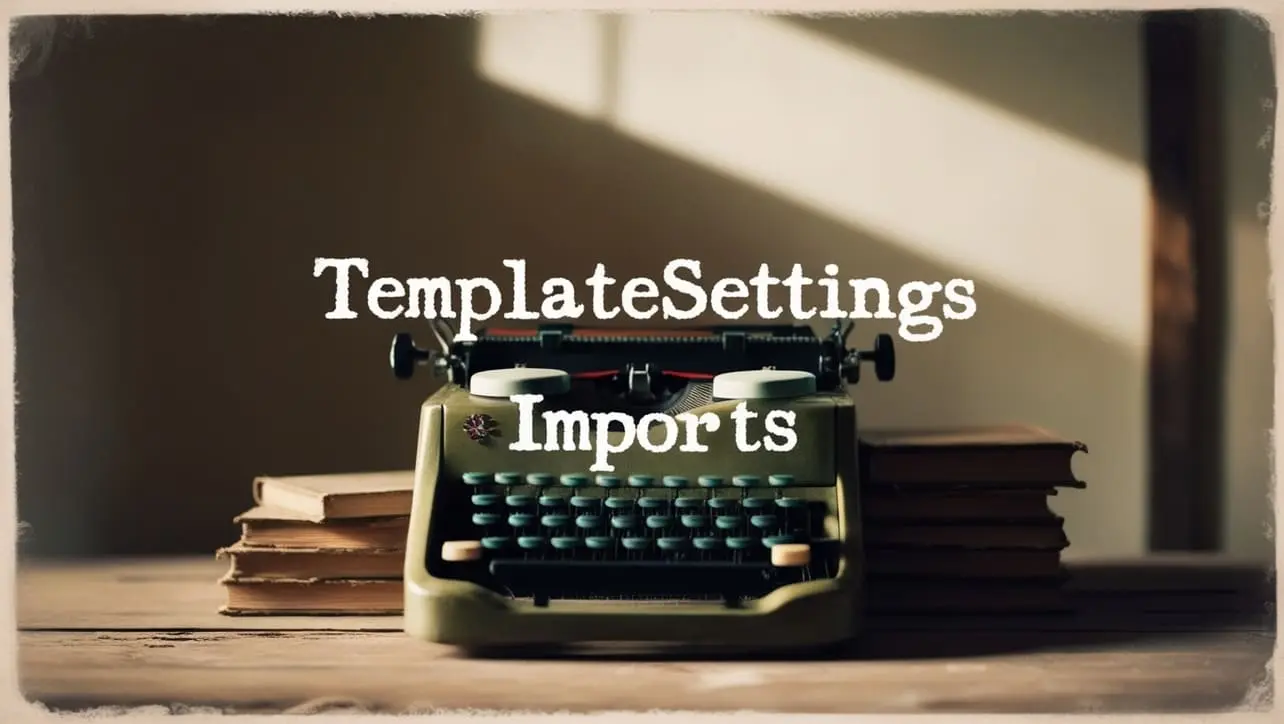
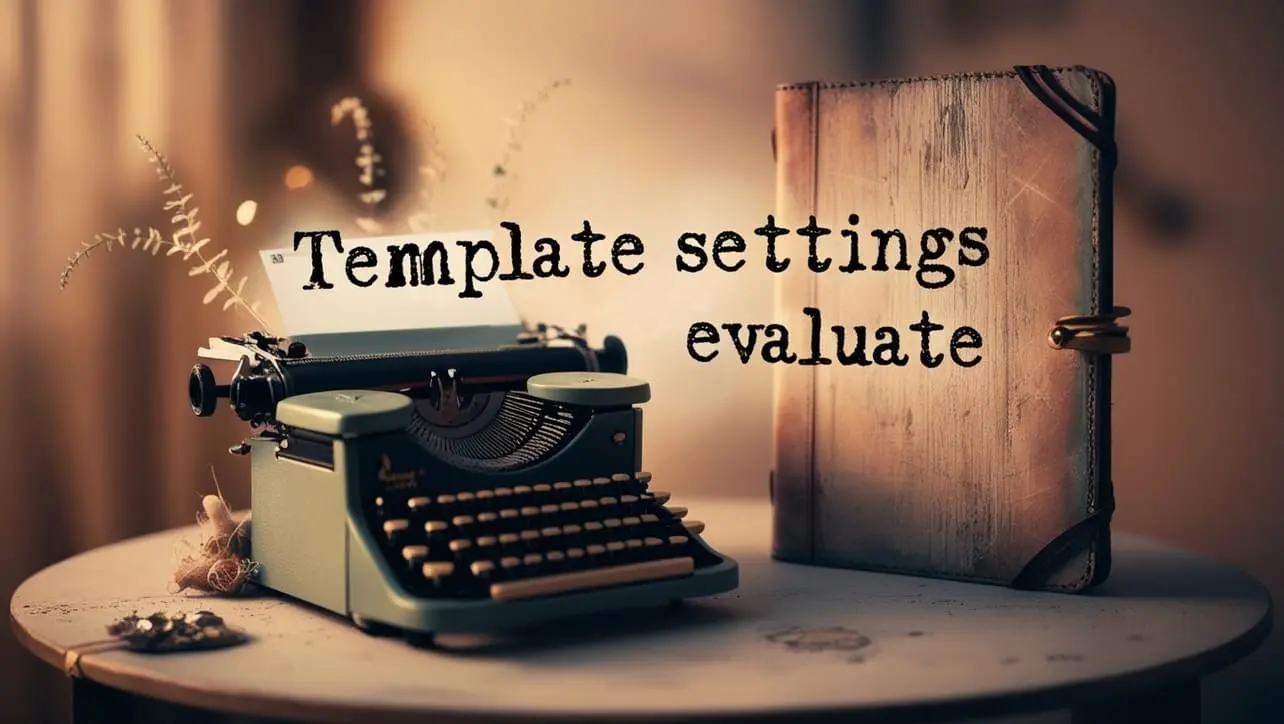
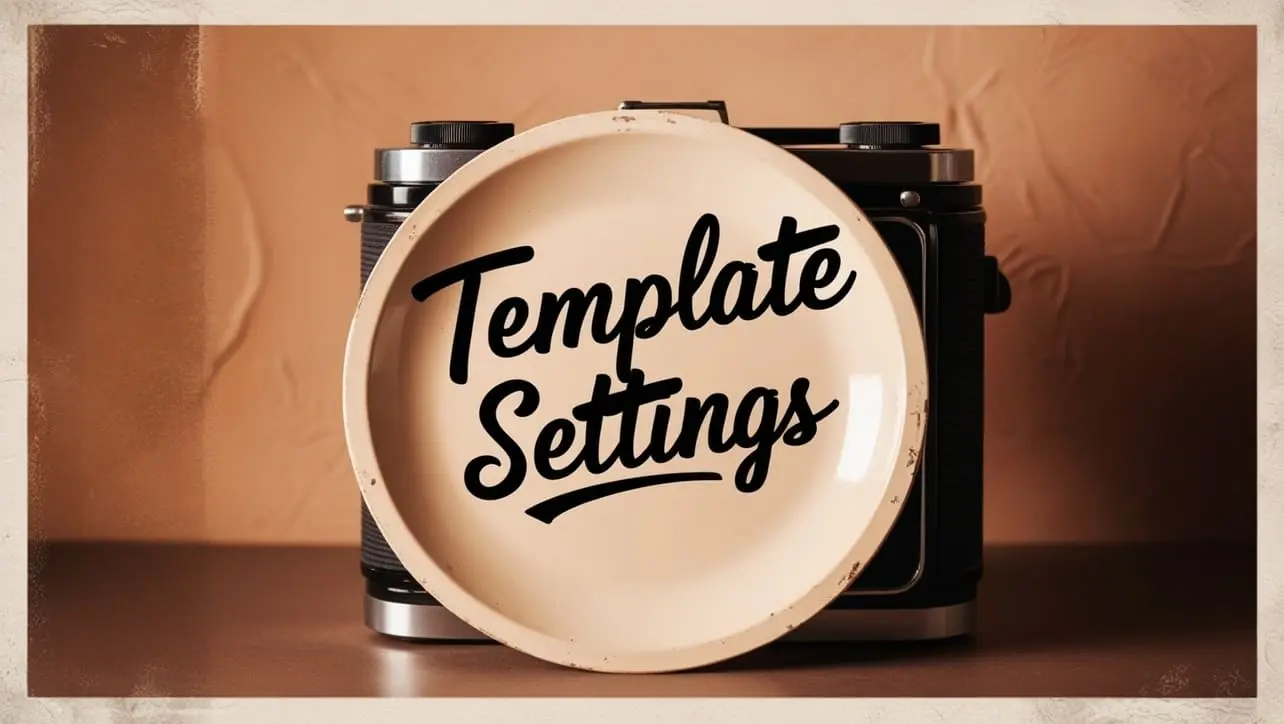
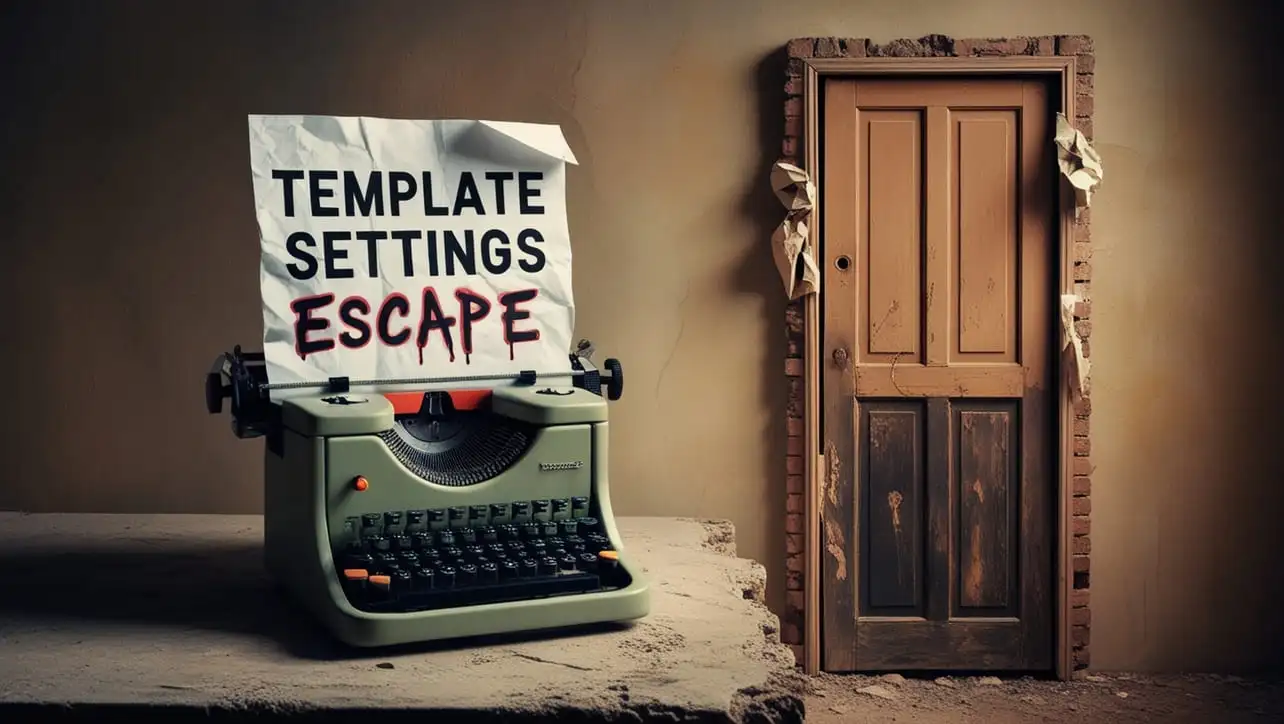
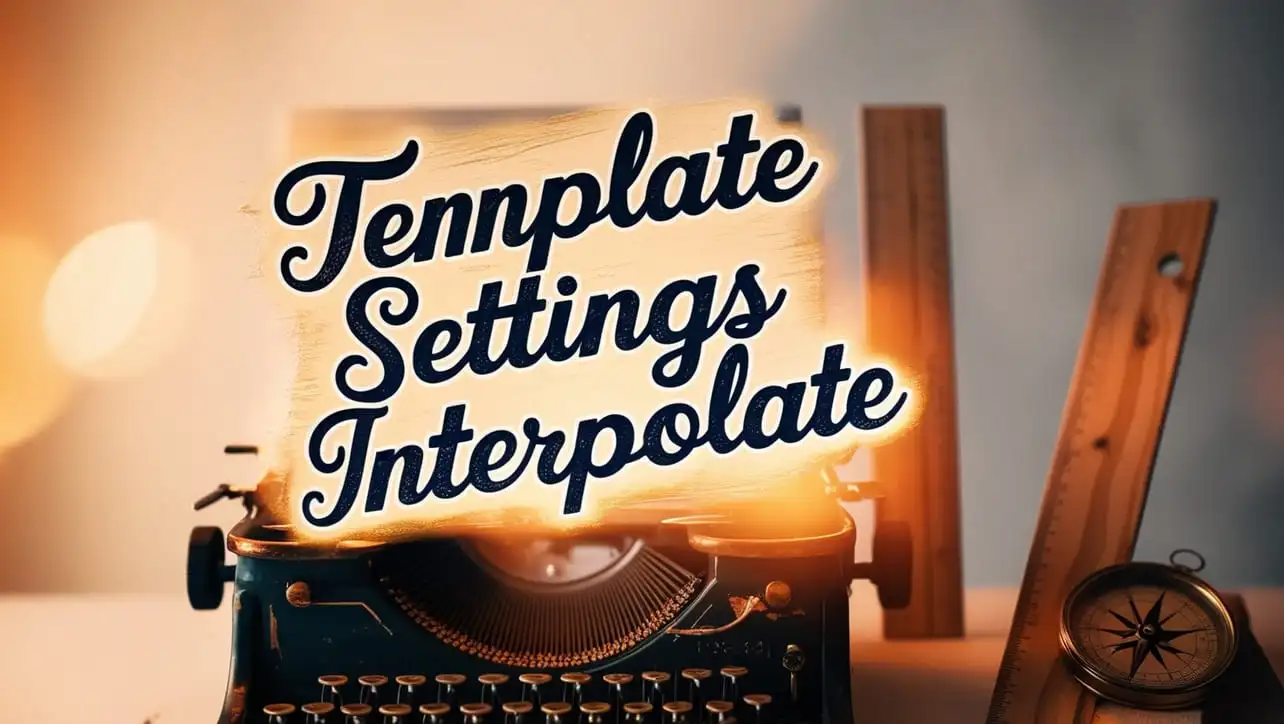
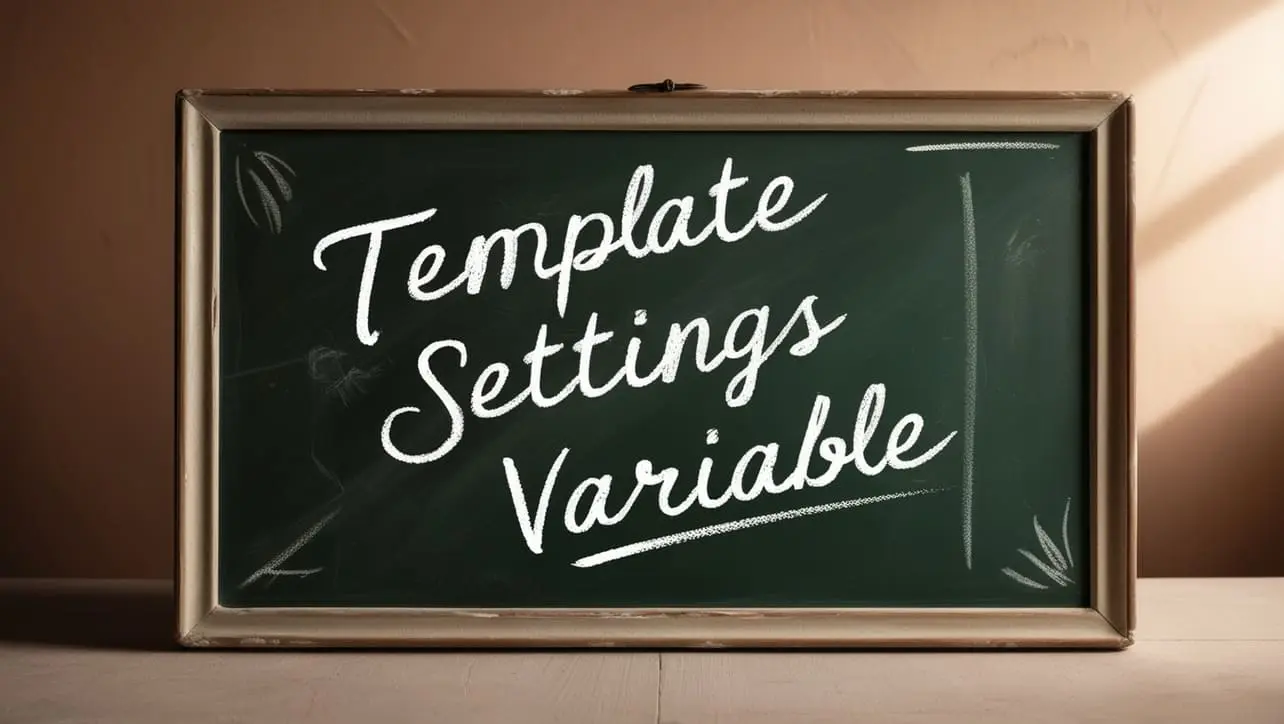
If you have any doubts regarding this article (Lodash _.unionWith() Array Method), please comment here. I will help you immediately.