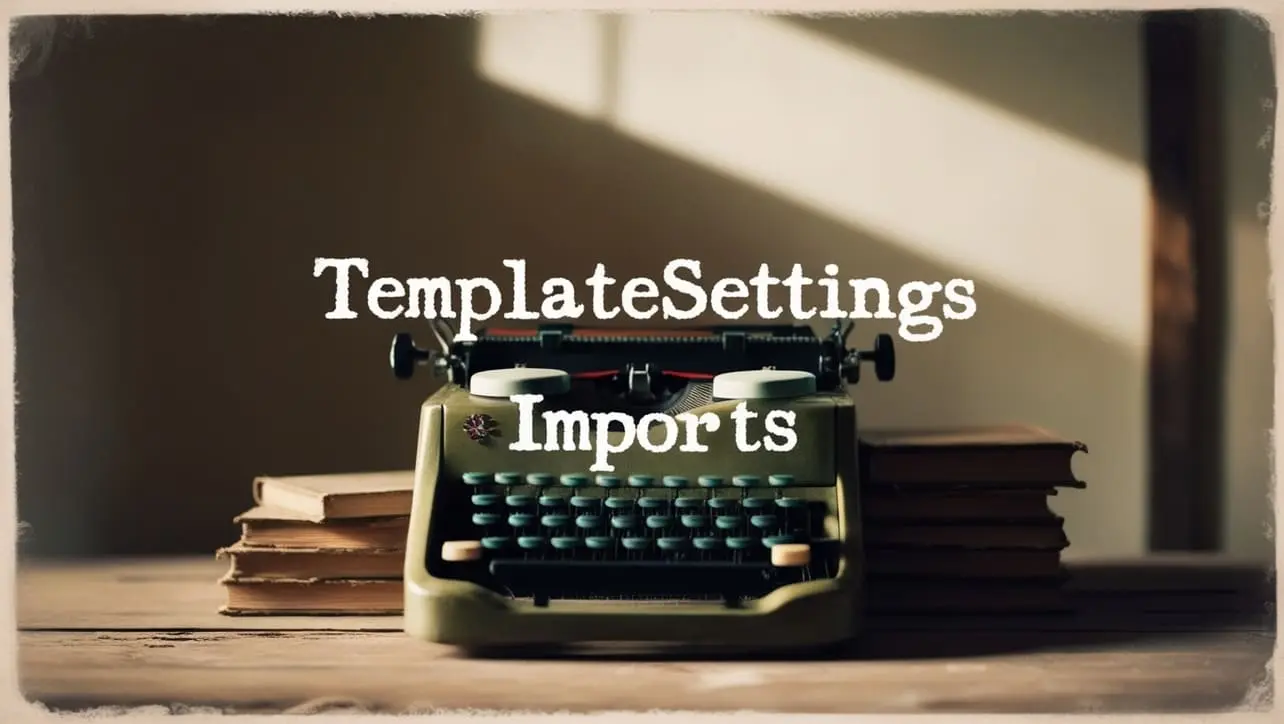
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedUniqBy() Array Method
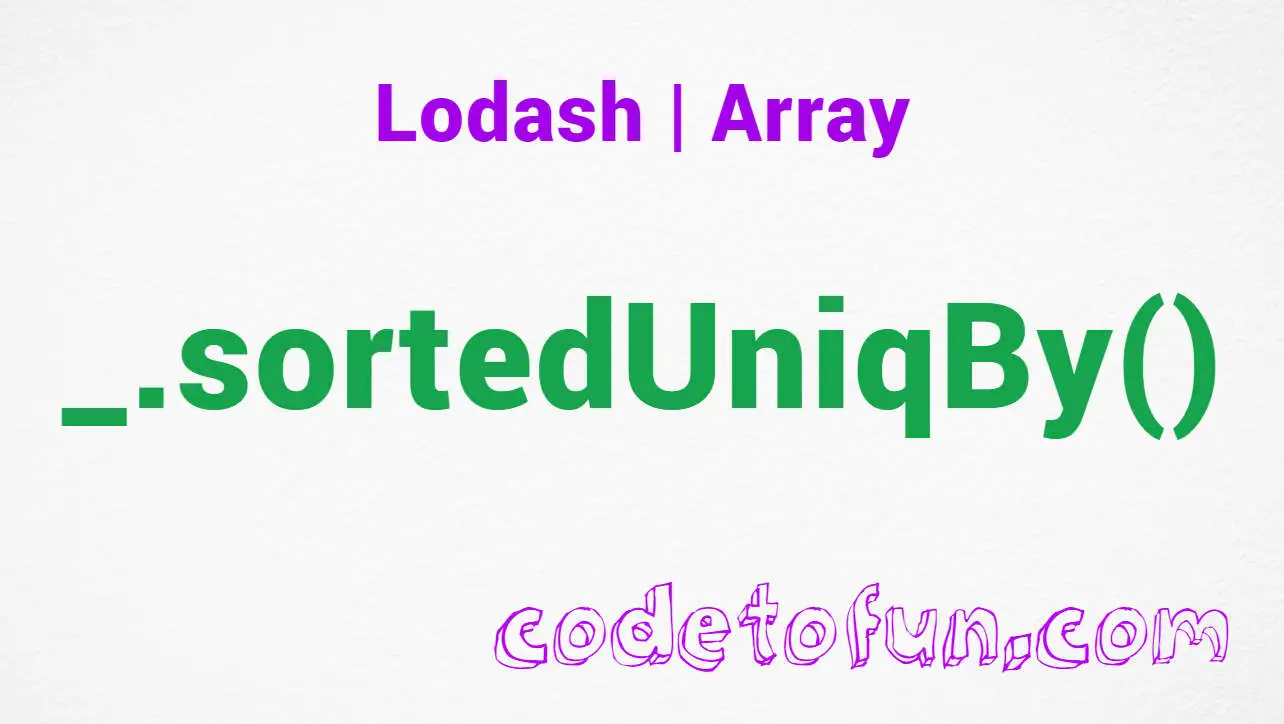
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficiently managing arrays is paramount. Enter Lodash, a robust utility library that offers a myriad of functions to simplify array manipulation. Among these functions lies the _.sortedUniqBy()
method, a powerful tool for eliminating duplicate values from sorted arrays based on a specific criterion. This method enhances code readability and performance, making it indispensable for developers dealing with sorted data.
🧠 Understanding _.sortedUniqBy()
The _.sortedUniqBy()
method in Lodash is designed to remove duplicate elements from a sorted array while allowing customization based on a provided iteratee function. This enables developers to specify the criteria by which uniqueness is determined, offering flexibility and precision in data manipulation.
💡 Syntax
_.sortedUniqBy(array, [iteratee])
- array: The sorted array to process.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's delve into a practical example to grasp the functionality of _.sortedUniqBy()
:
const _ = require('lodash');
const sortedArray = [1, 2, 2, 3, 3, 3, 4, 5, 6];
const uniqueArray = _.sortedUniqBy(sortedArray);
console.log(uniqueArray);
// Output: [1, 2, 3, 4, 5, 6]
In this example, the sortedArray is processed by _.sortedUniqBy()
, resulting in a new array containing only unique elements in sorted order.
🏆 Best Practices
Understanding Sorted Arrays:
Ensure that the input array is sorted before applying
_.sortedUniqBy()
. This method is optimized for sorted arrays, and applying it to unsorted data may yield unexpected results.example.jsCopiedconst unsortedArray = [3, 1, 2, 2, 4, 3]; const sortedUniqueArray = _.sortedUniqBy(_.sortBy(unsortedArray)); console.log(sortedUniqueArray); // Output: [1, 2, 3, 4]
Define Custom Criteria:
Utilize the iteratee parameter to define custom criteria for uniqueness. This allows you to tailor the behavior of
_.sortedUniqBy()
according to your specific requirements.example.jsCopiedconst arrayOfObjects = [ { id: 1, value: 'apple' }, { id: 2, value: 'banana' }, { id: 3, value: 'apple' }, ]; const uniqueObjects = _.sortedUniqBy(arrayOfObjects, obj => obj.value); console.log(uniqueObjects); // Output: [{ id: 1, value: 'apple' }, { id: 2, value: 'banana' }]
Performance Considerations:
Keep in mind the performance implications when working with large datasets. The
_.sortedUniqBy()
method offers optimized performance for sorted arrays, making it ideal for handling large volumes of data efficiently.example.jsCopiedconst largeSortedArray = /* ...generate a large sorted array... */; console.time('sortedUniqBy'); const uniqueValues = _.sortedUniqBy(largeSortedArray); console.timeEnd('sortedUniqBy'); console.log(uniqueValues);
📚 Use Cases
Removing Duplicate Entries:
_.sortedUniqBy()
is particularly useful when dealing with sorted datasets containing duplicate entries. By removing duplicates, you can ensure data integrity and streamline subsequent operations.example.jsCopiedconst sortedDataWithDuplicates = [10, 20, 20, 30, 30, 40, 50]; const uniqueData = _.sortedUniqBy(sortedDataWithDuplicates); console.log(uniqueData); // Output: [10, 20, 30, 40, 50]
Data Normalization:
In scenarios where data normalization is necessary,
_.sortedUniqBy()
can be employed to condense redundant information, facilitating cleaner and more concise data representation.example.jsCopiedconst normalizedData = [ { id: 1, category: 'fruit' }, { id: 2, category: 'vegetable' }, { id: 3, category: 'fruit' }, ]; const uniqueCategories = _.sortedUniqBy(normalizedData, obj => obj.category); console.log(uniqueCategories); // Output: [{ id: 1, category: 'fruit' }, { id: 2, category: 'vegetable' }]
Performance Optimization:
By leveraging the optimized performance of
_.sortedUniqBy()
for sorted arrays, you can enhance the efficiency of your code, especially when dealing with large datasets or performance-sensitive applications.example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; const sortedUniqueData = _.sortedUniqBy(_.sortBy(largeDataset)); console.log(sortedUniqueData);
🎉 Conclusion
The _.sortedUniqBy()
method in Lodash offers a powerful solution for removing duplicate elements from sorted arrays with customizable uniqueness criteria. Whether you're working with sorted datasets or striving for optimal performance, this method provides a versatile tool for array manipulation in JavaScript.
Unlock the potential of sorted array manipulation with _.sortedUniqBy()
and elevate your JavaScript development experience!
👨💻 Join our Community:
Author
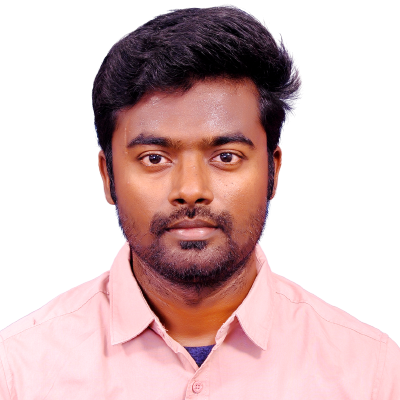
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
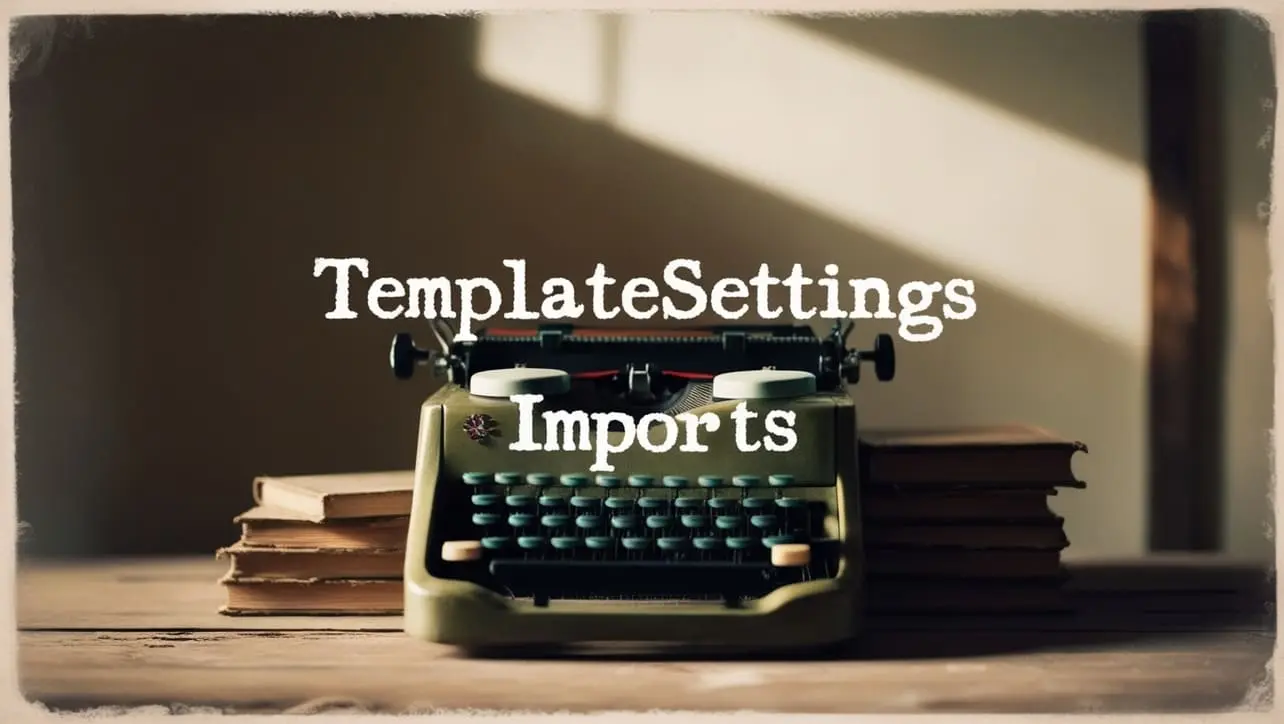
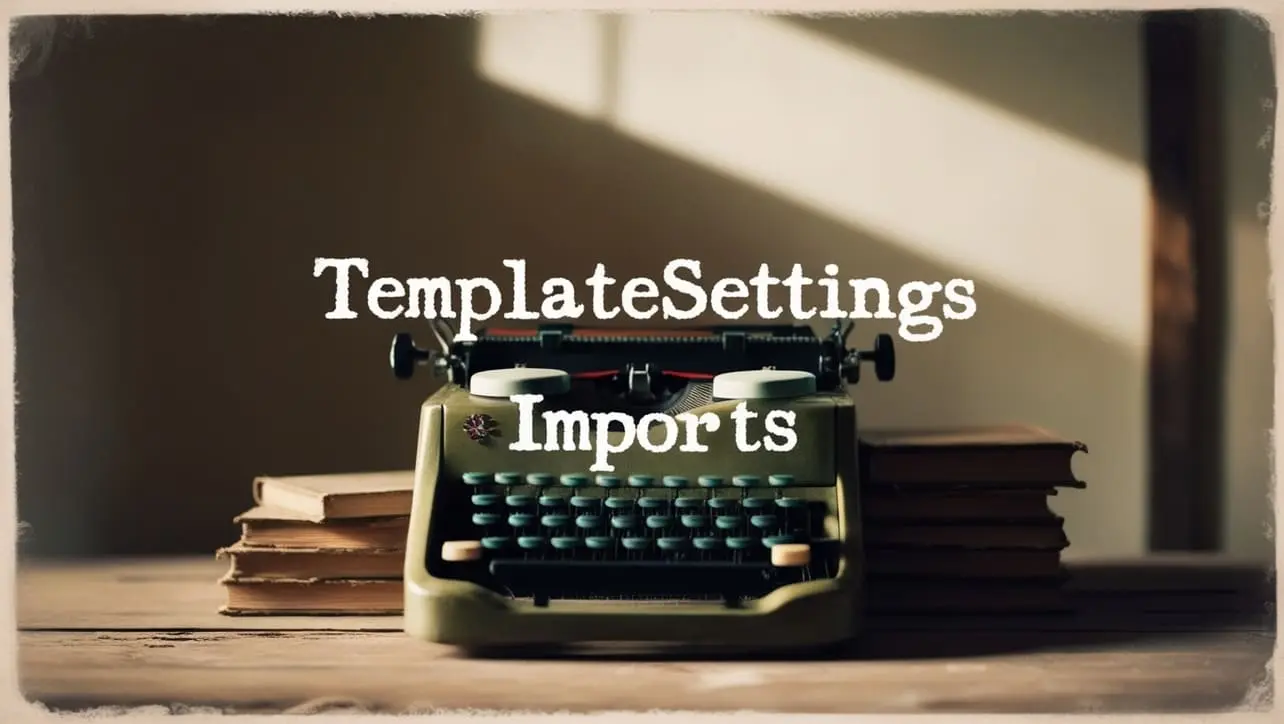
Lodash _.templateSettings.imports Property
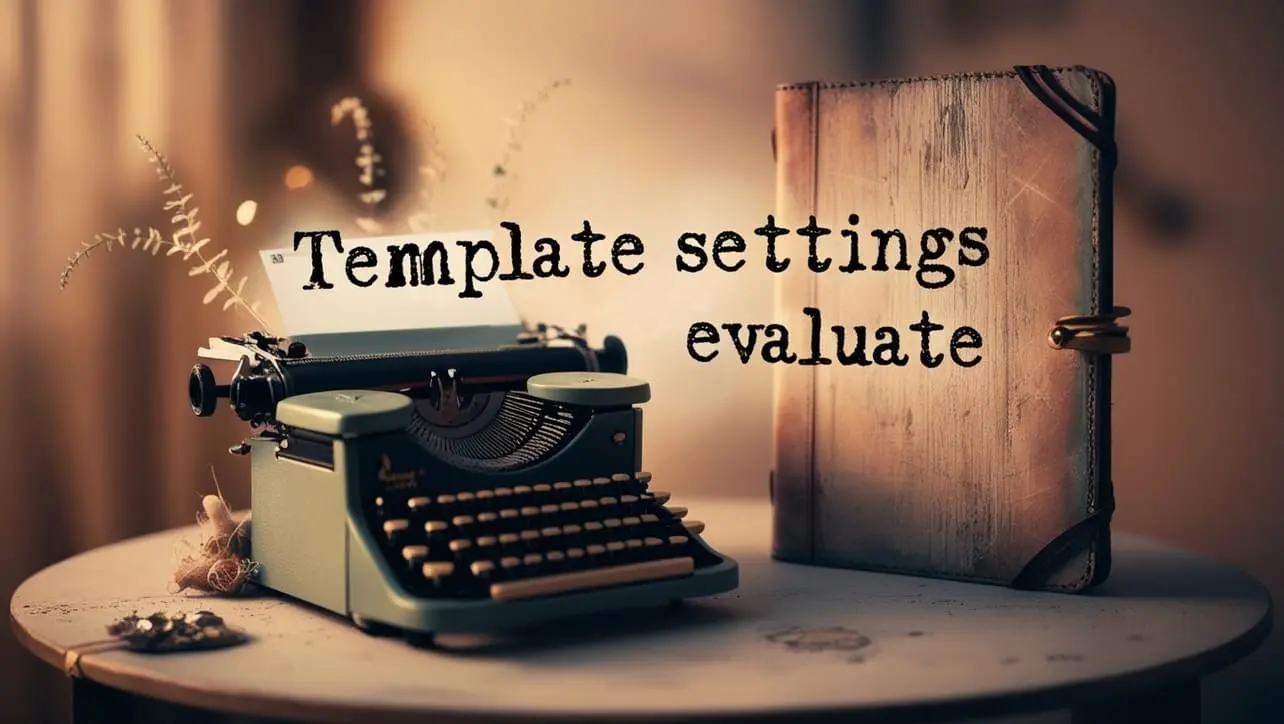
Lodash _.templateSettings.evaluate Property
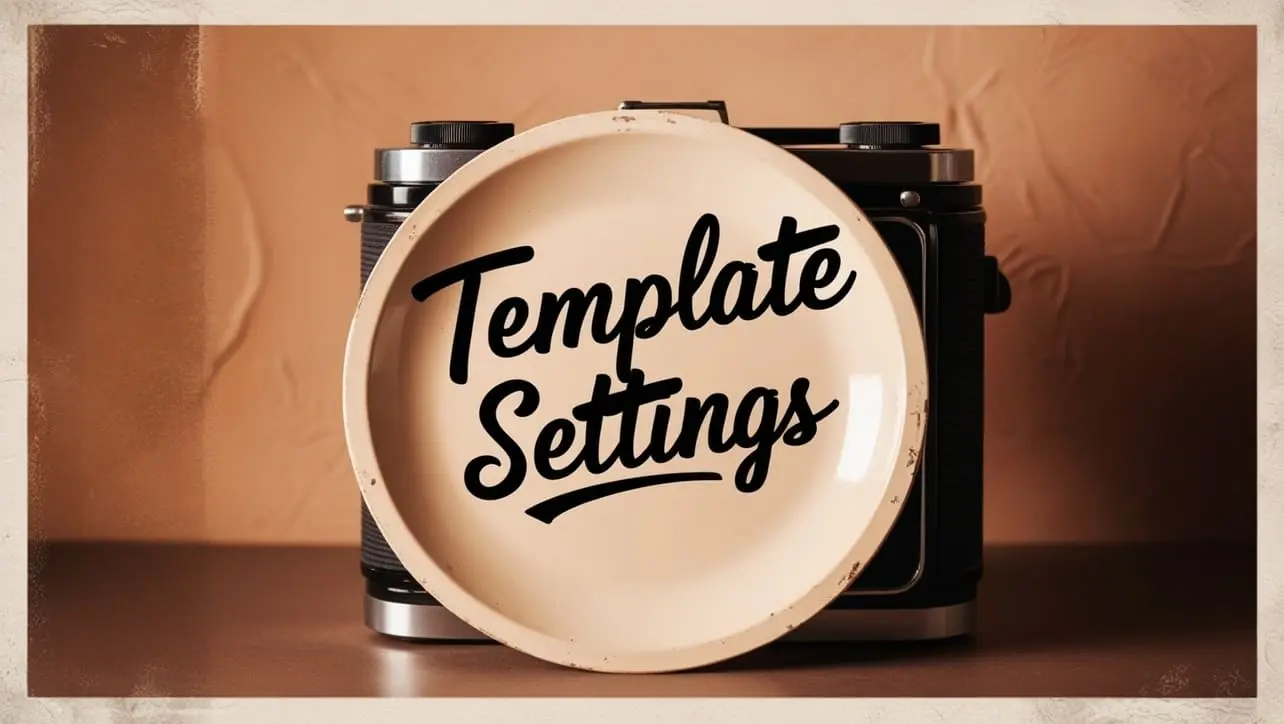
Lodash _.templateSettings Property
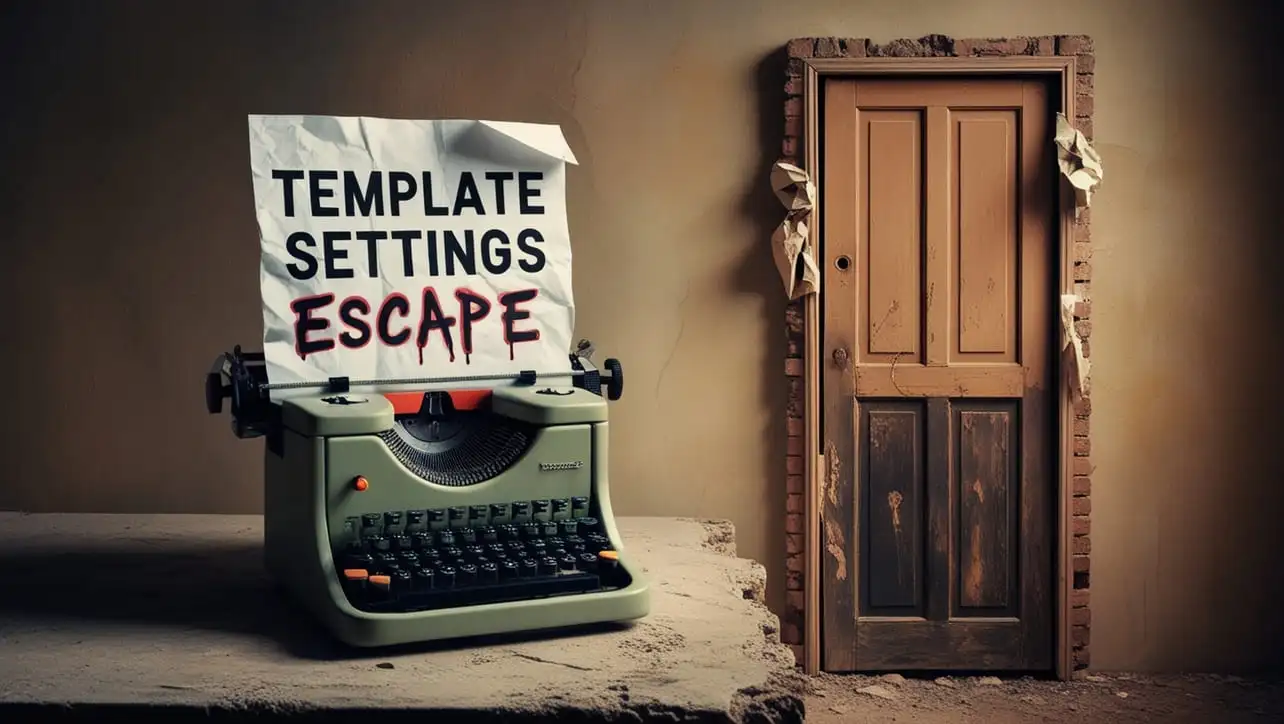
Lodash _.templateSettings.escape Property
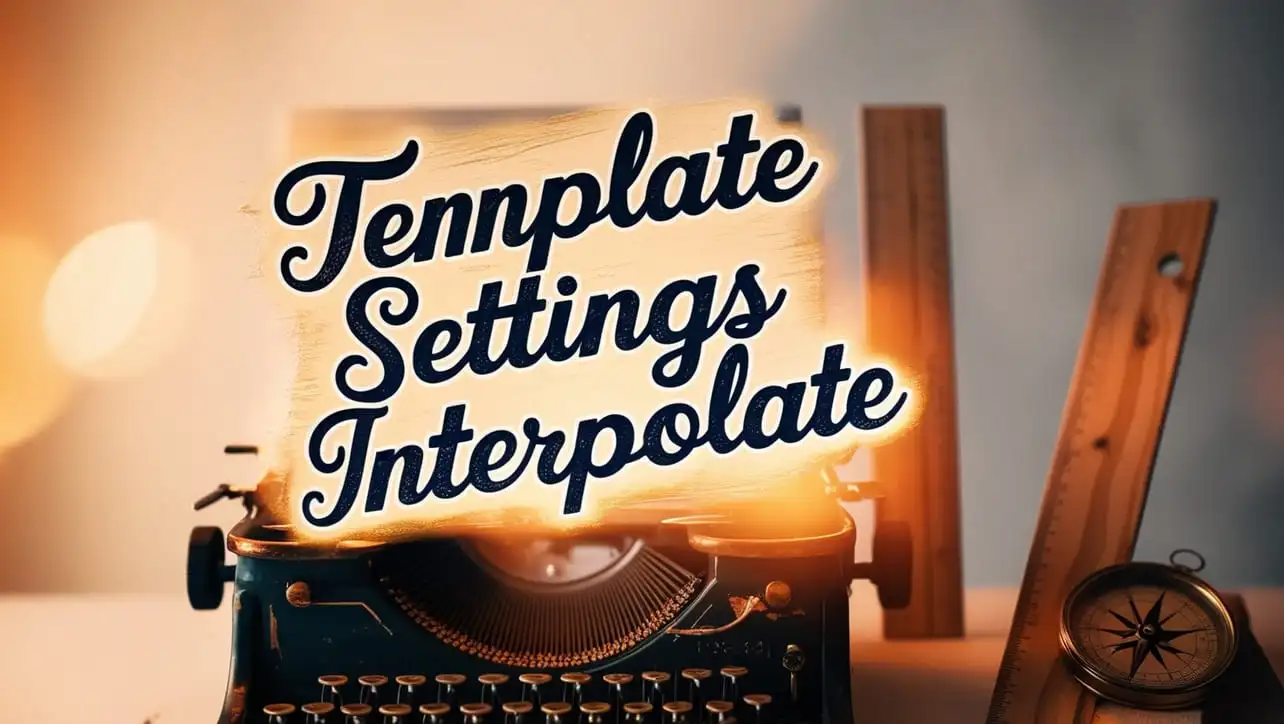
Lodash _.templateSettings.interpolate Property
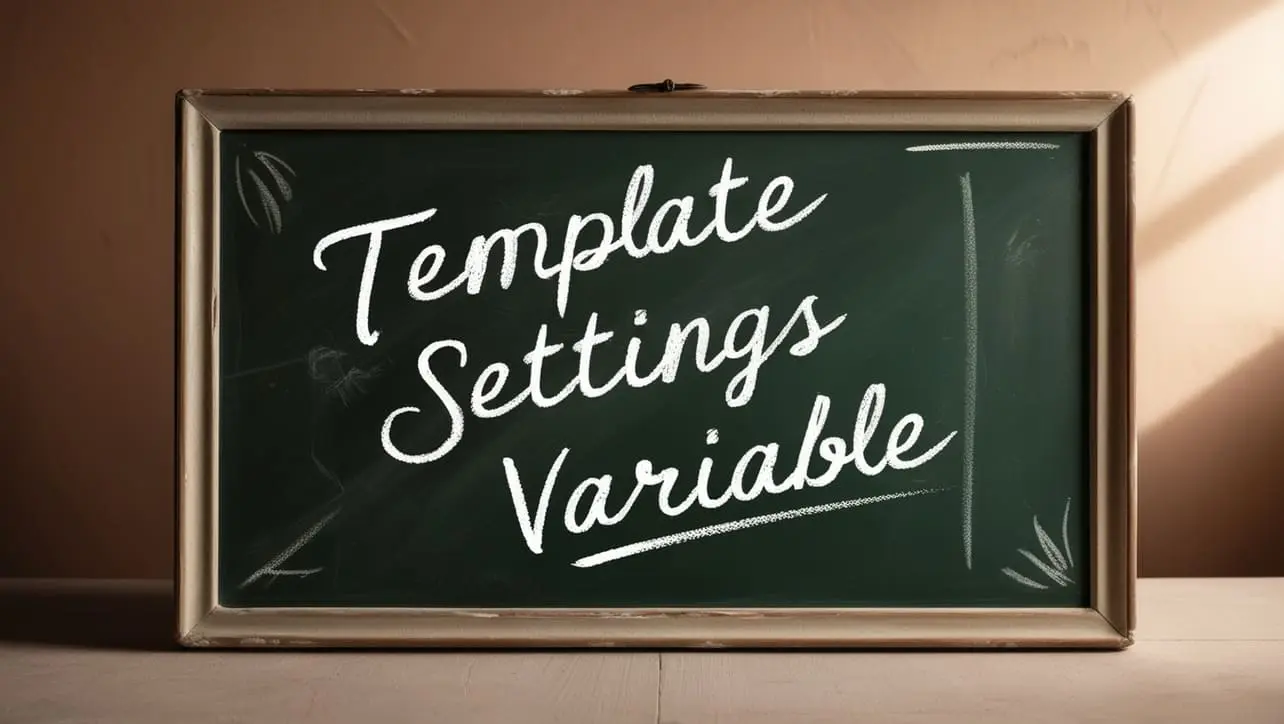
If you have any doubts regarding this article (Lodash _.sortedUniqBy() Array Method), please comment here. I will help you immediately.