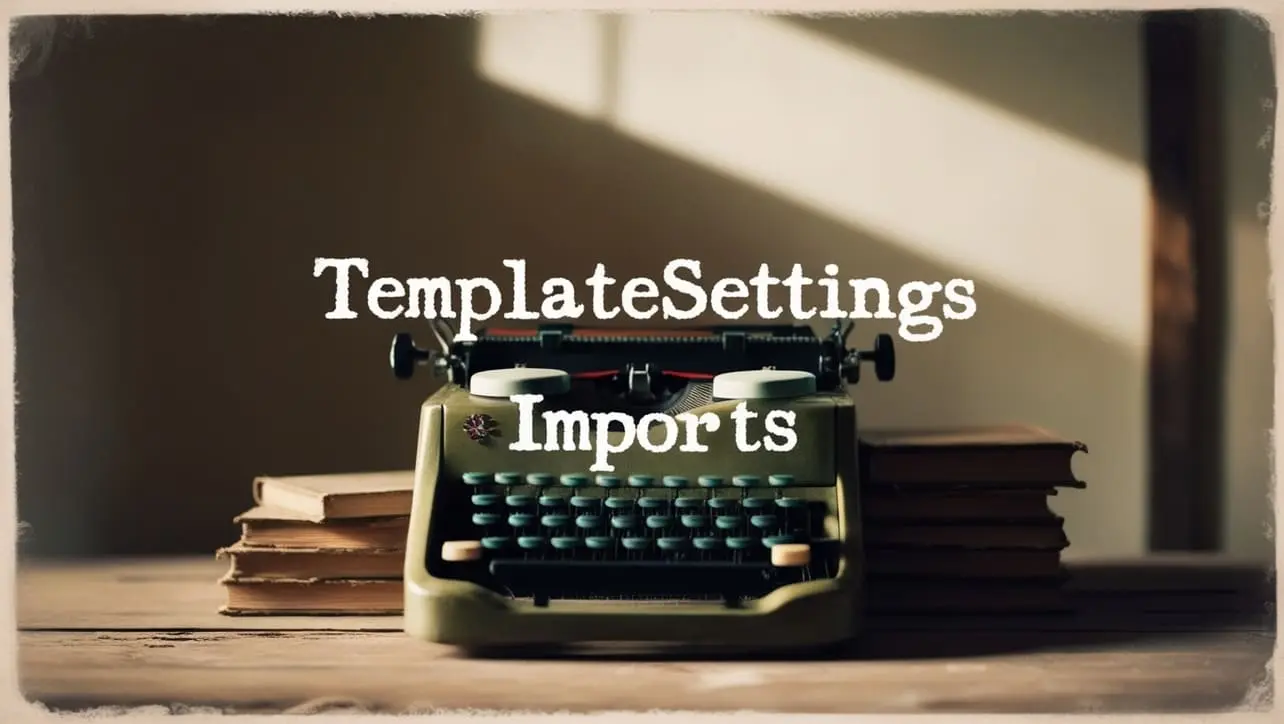
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.sortedIndex() Array Method
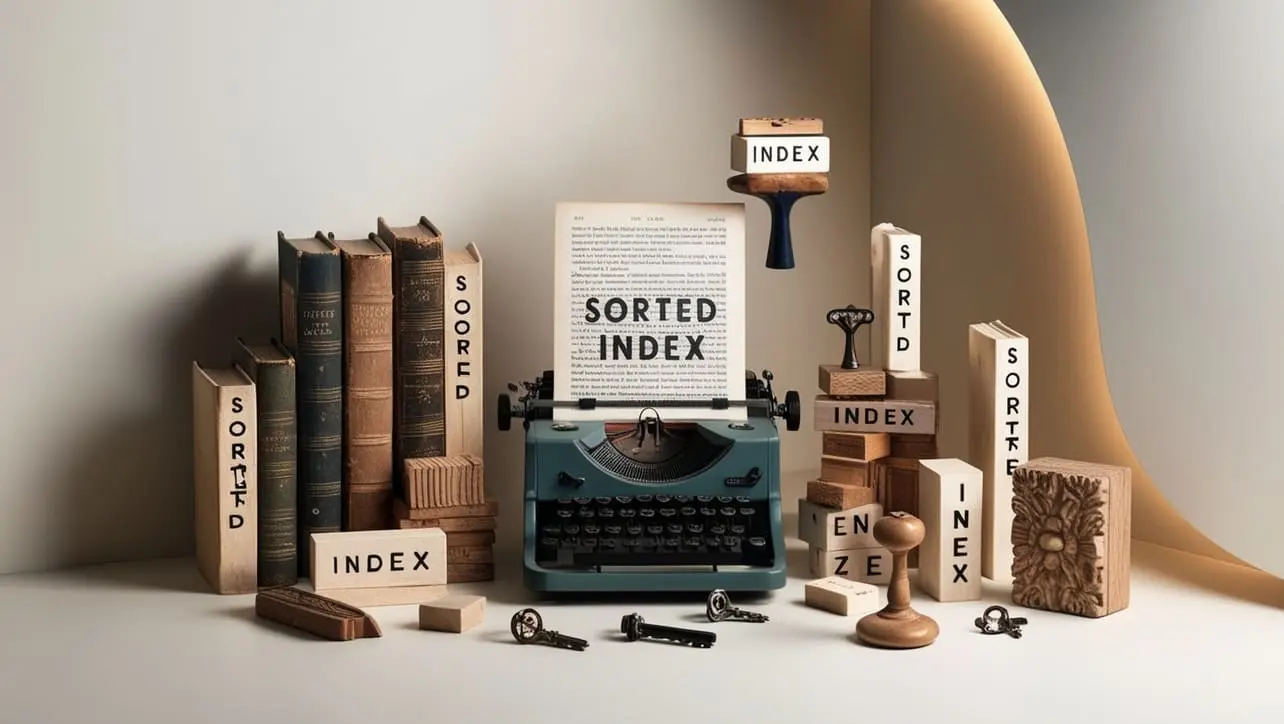
Photo Credit to CodeToFun
🙋 Introduction
When it comes to working with arrays in JavaScript, efficient searching and insertion are crucial aspects of programming.
Lodash provides a powerful tool in the form of the _.sortedIndex()
method. This method simplifies the process of finding the correct index to maintain the sorted order of an array, making it an invaluable asset for developers working with ordered datasets.
🧠 Understanding _.sortedIndex()
The _.sortedIndex()
method in Lodash is designed to find the index at which a value should be inserted into a sorted array to maintain its order. This is particularly useful for optimizing search and insertion operations, ensuring efficient data manipulation.
💡 Syntax
_.sortedIndex(array, value)
- array: The sorted array to search.
- value: The value to find the correct index for.
📝 Example
Let's explore a practical example to grasp the functionality of _.sortedIndex()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const sortedArray = [10, 20, 30, 40, 50];
const valueToInsert = 35;
const insertIndex = _.sortedIndex(sortedArray, valueToInsert);
console.log(insertIndex);
// Output: 3
In this example, valueToInsert is 35, and insertIndex is determined as 3, indicating the index at which 35 should be inserted to maintain the sorted order of sortedArray.
🏆 Best Practices
Validating Input:
Before using
_.sortedIndex()
, ensure that the input array is sorted. Unexpected behavior may occur if the array is not in ascending order.example.jsCopiedconst unsortedArray = [50, 30, 10, 40, 20]; if (!_.isEqual(unsortedArray, _.sortBy(unsortedArray))) { console.error('Input array must be sorted.'); return; } const insertIndexUnsorted = _.sortedIndex(unsortedArray, valueToInsert); console.log(insertIndexUnsorted);
Handling Duplicates:
Be aware that
_.sortedIndex()
may return the index of an existing value, especially when dealing with duplicate values in the array. Decide how duplicates should be handled based on your specific use case.example.jsCopiedconst sortedArrayWithDuplicates = [10, 20, 30, 30, 40, 50]; const valueToInsertDuplicate = 30; const insertIndexDuplicate = _.sortedIndex(sortedArrayWithDuplicates, valueToInsertDuplicate); console.log(insertIndexDuplicate); // Output: 2 (index of the first occurrence of 30)
Utilizing Binary Search:
Understand that
_.sortedIndex()
employs a binary search algorithm for efficiency. Leverage this for large datasets where binary search significantly outperforms linear search.example.jsCopiedconst largeSortedArray = /* ... fetch or generate a large sorted array ... */; const valueToInsertLargeArray = /* ... your value ... */; const insertIndexLargeArray = _.sortedIndex(largeSortedArray, valueToInsertLargeArray); console.log(insertIndexLargeArray);
📚 Use Cases
Maintaining Sorted Order:
The primary use case of
_.sortedIndex()
is to maintain the sorted order of an array while efficiently determining the correct index for inserting a new value.example.jsCopiedconst newSortedArray = _.sortBy([...sortedArray, valueToInsert]); console.log(newSortedArray);
Optimizing Search Operations:
Use
_.sortedIndex()
to optimize search operations in a sorted array, reducing the time complexity compared to linear search algorithms.example.jsCopiedconst searchValue = /* ... value to search for ... */; const searchIndex = _.sortedIndex(sortedArray, searchValue); console.log(searchIndex);
🎉 Conclusion
The _.sortedIndex()
method in Lodash is a powerful ally for developers dealing with sorted arrays. Its efficiency in finding the correct insertion index and its utilization of a binary search algorithm make it an excellent choice for scenarios where maintaining order and optimizing search operations are crucial.
Explore the world of Lodash and leverage the capabilities of _.sortedIndex()
to enhance the performance and efficiency of your array manipulation tasks!
👨💻 Join our Community:
Author
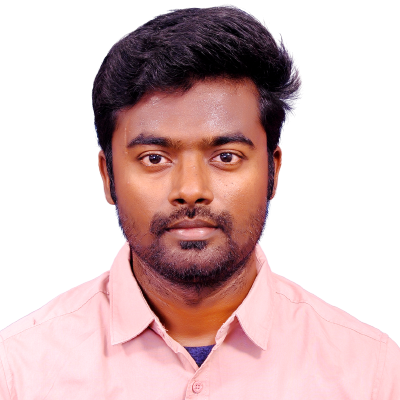
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
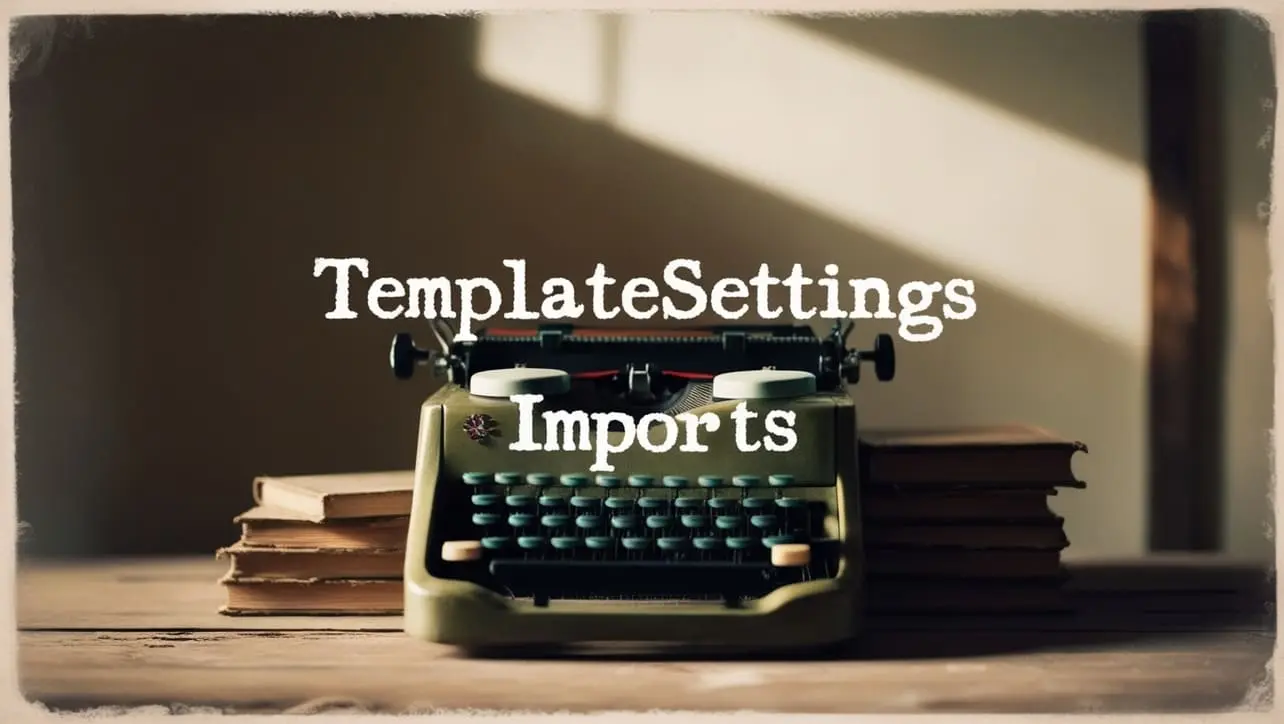
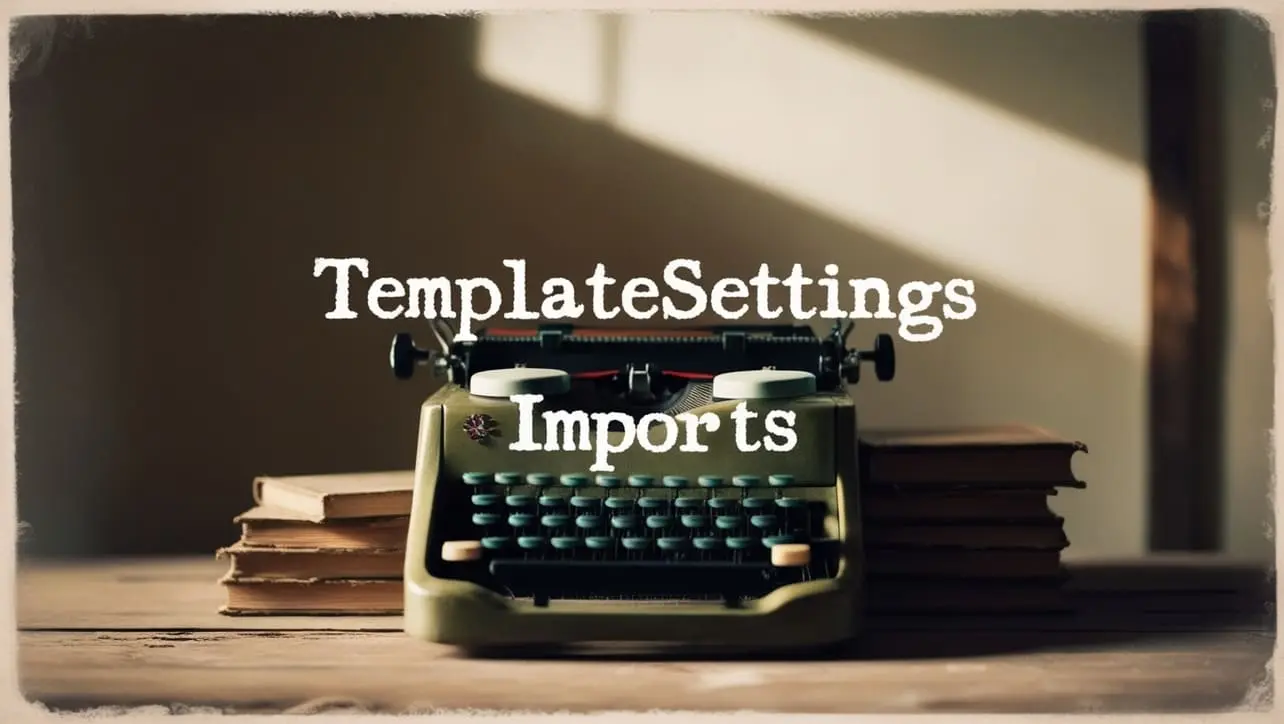
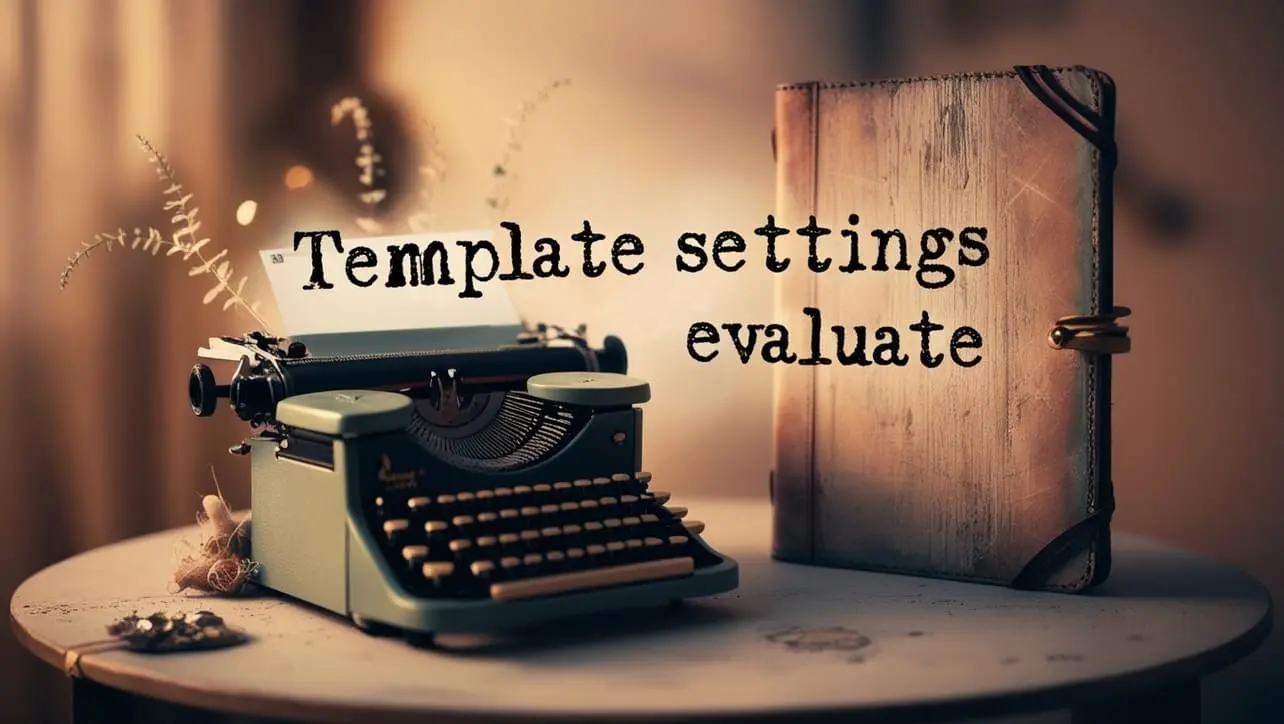
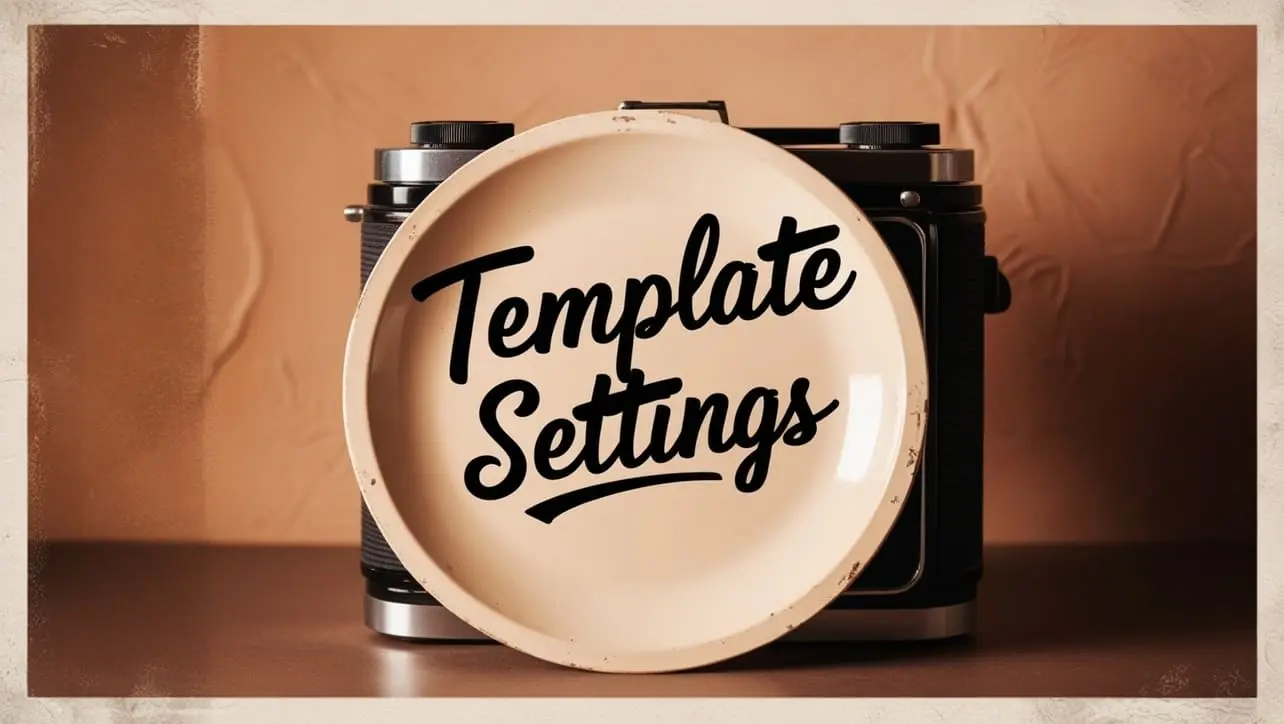
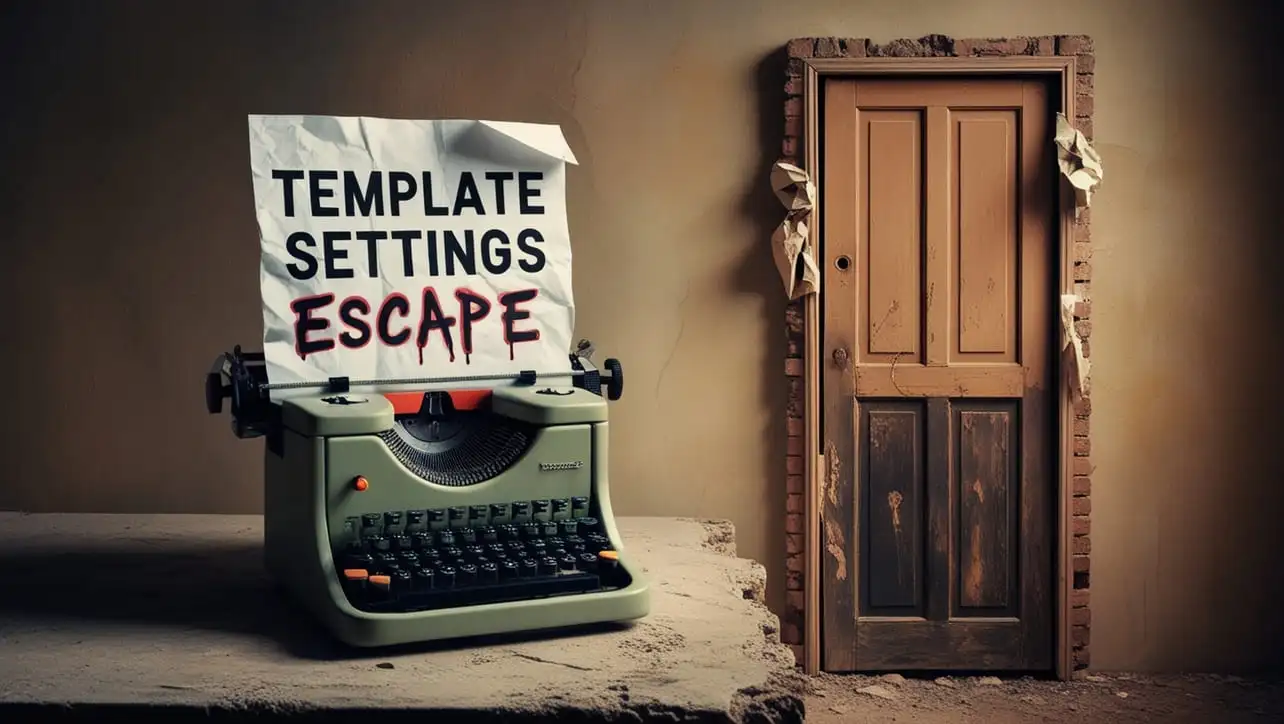
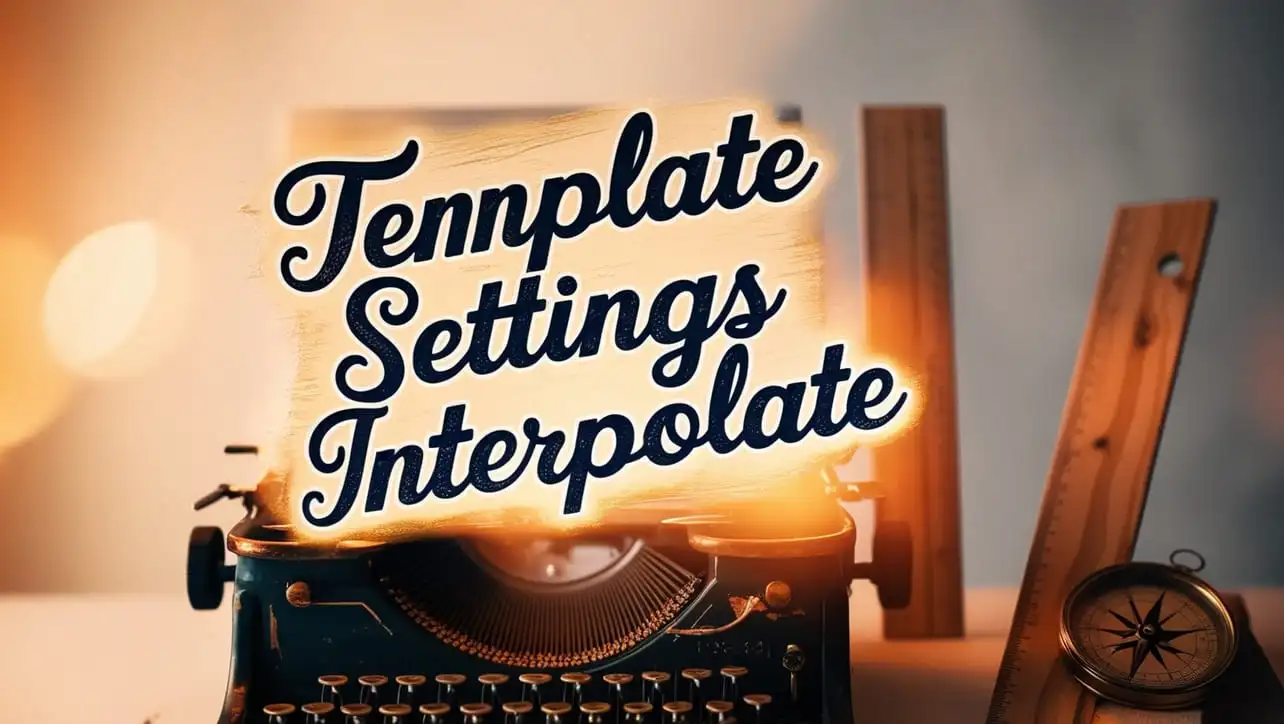
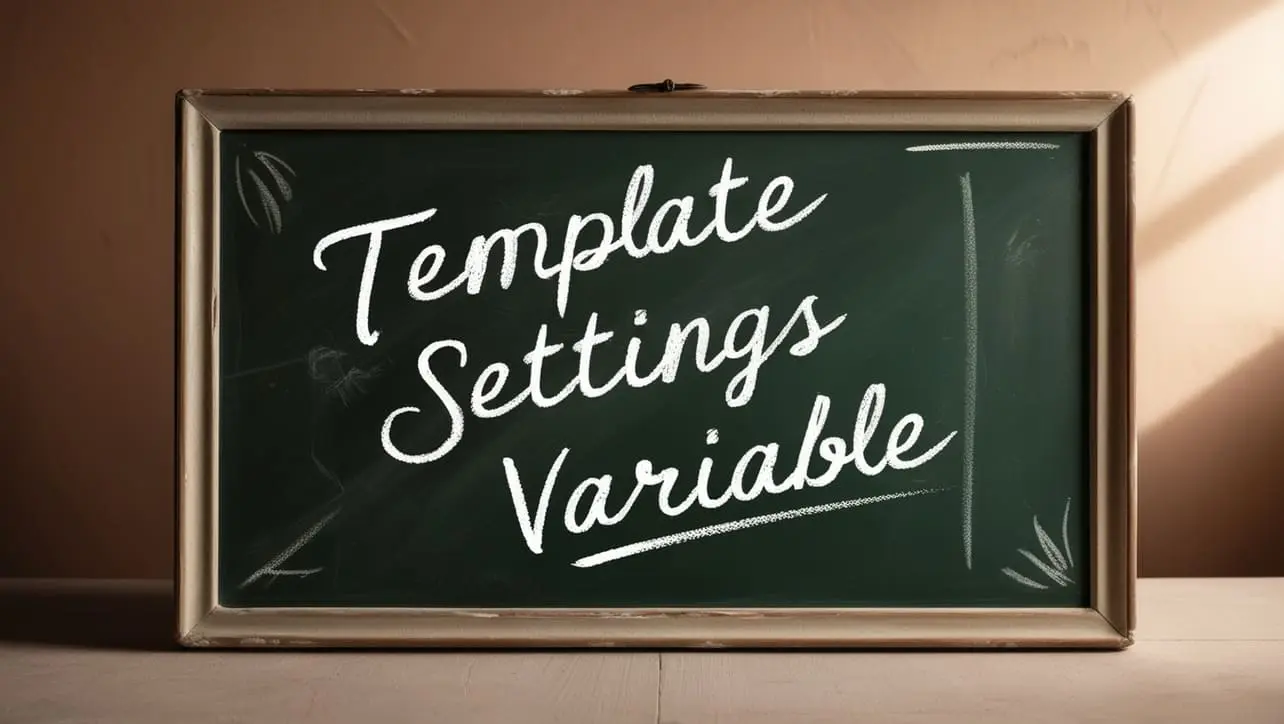
If you have any doubts regarding this article (Lodash _.sortedIndex() Array Method), please comment here. I will help you immediately.