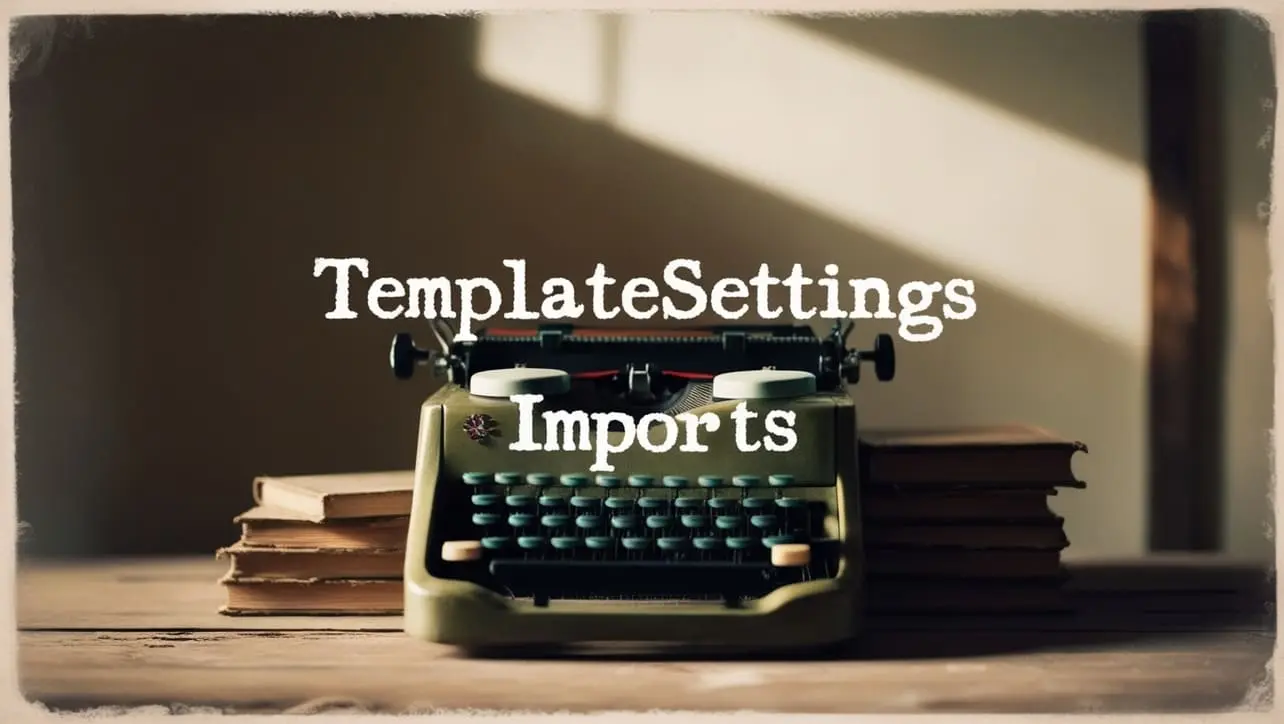
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.pullAllWith() Array Method
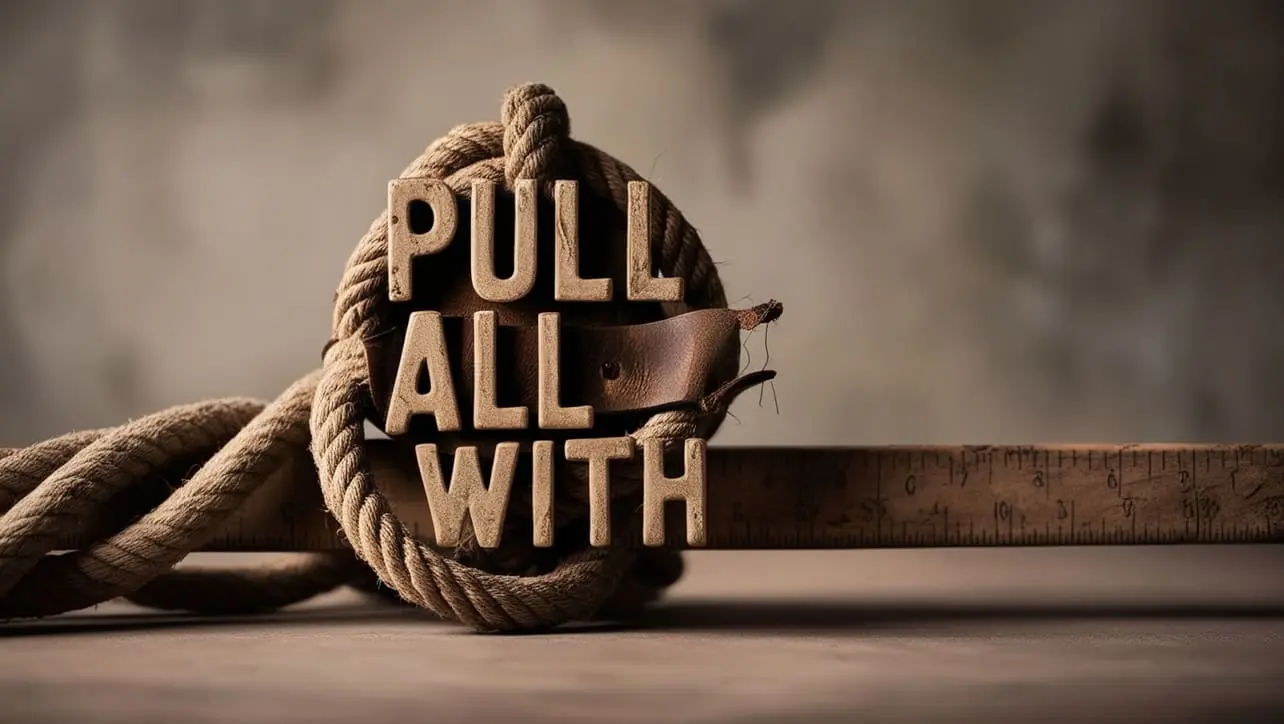
Photo Credit to CodeToFun
🙋 Introduction
Efficiently modifying arrays is a common requirement in JavaScript programming, and the Lodash library provides a variety of powerful tools to accomplish this.
The _.pullAllWith()
method is one such utility that simplifies the process of removing elements from an array based on a custom comparator function. This method proves invaluable when you need fine-grained control over element removal.
🧠 Understanding _.pullAllWith()
The _.pullAllWith()
method in Lodash is designed to remove all elements from an array that match a specified value using a custom comparator function. This enables developers to tailor the removal criteria to their specific needs, offering flexibility and precision.
💡 Syntax
_.pullAllWith(array, values, [comparator])
- array: The array from which elements should be removed.
- values: An array of values to be removed.
- comparator: A function that compares values for equality (default is _.isEqual).
📝 Example
Let's explore a practical example to illustrate the application of _.pullAllWith()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' },
{ id: 4, name: 'David' }
];
const valuesToRemove = [
{ id: 2, name: 'Bob' },
{ id: 4, name: 'David' }
];
const newArray = _.pullAllWith(originalArray, valuesToRemove, _.isEqual);
console.log(newArray);
// Output: [{ id: 1, name: 'Alice' }, { id: 3, name: 'Charlie' }]
In this example, elements from valuesToRemove are removed from the originalArray based on the custom comparator function (_.isEqual in this case).
🏆 Best Practices
Customize the Comparator:
Take advantage of the comparator parameter to tailor the comparison logic according to your specific requirements. This allows for flexible and precise element removal.
example.jsCopiedconst customComparator = (obj1, obj2) => obj1.id === obj2.id; const newArrayCustomComparator = _.pullAllWith(originalArray, valuesToRemove, customComparator); console.log(newArrayCustomComparator); // Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' }]
Avoid Mutating Original Array:
If preserving the original array is important, consider creating a new array with the desired modifications instead of modifying the original array in place.
example.jsCopiedconst newArrayWithoutModifyingOriginal = _.pullAllWith([...originalArray], valuesToRemove, _.isEqual); console.log(newArrayWithoutModifyingOriginal); // Output: [{ id: 1, name: 'Alice' }, { id: 3, name: 'Charlie' }]
📚 Use Cases
Data Filtering:
_.pullAllWith()
is useful in scenarios where you need to filter out specific elements from an array based on complex criteria.example.jsCopiedconst data = /* ...fetch data from API or elsewhere... */; const filterCriteria = /* ...define filter criteria... */; const filteredData = _.pullAllWith(data, filterCriteria, _.isEqual); console.log(filteredData);
Removing Duplicates:
When dealing with arrays containing objects and aiming to remove duplicate elements,
_.pullAllWith()
combined with a custom comparator can be a powerful solution.example.jsCopiedconst arrayWithDuplicates = /* ...an array with duplicate objects... */; const uniqueArray = _.uniqWith(arrayWithDuplicates, _.isEqual); console.log(uniqueArray);
🎉 Conclusion
The _.pullAllWith()
method in Lodash provides a convenient way to remove elements from an array based on a custom comparator function. This flexibility makes it a valuable tool in situations where fine-grained control over element removal is required.
Explore the capabilities of _.pullAllWith()
and leverage its features to efficiently modify arrays in your JavaScript projects. Enhance the precision of your array manipulations with Lodash!
👨💻 Join our Community:
Author
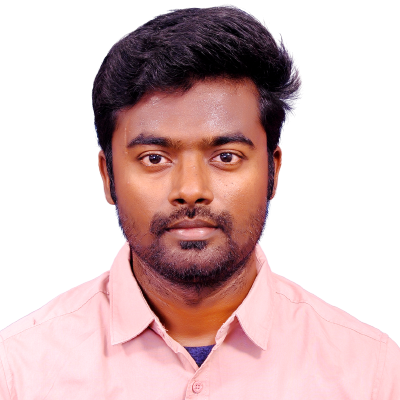
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
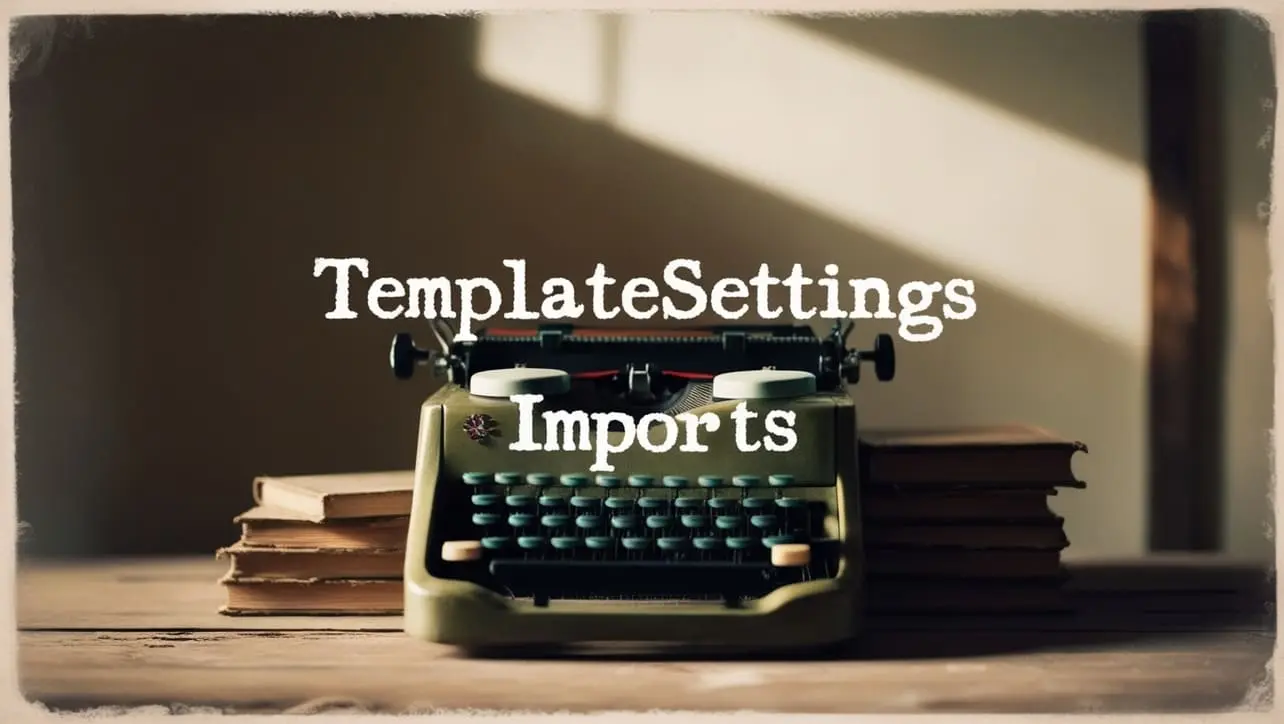
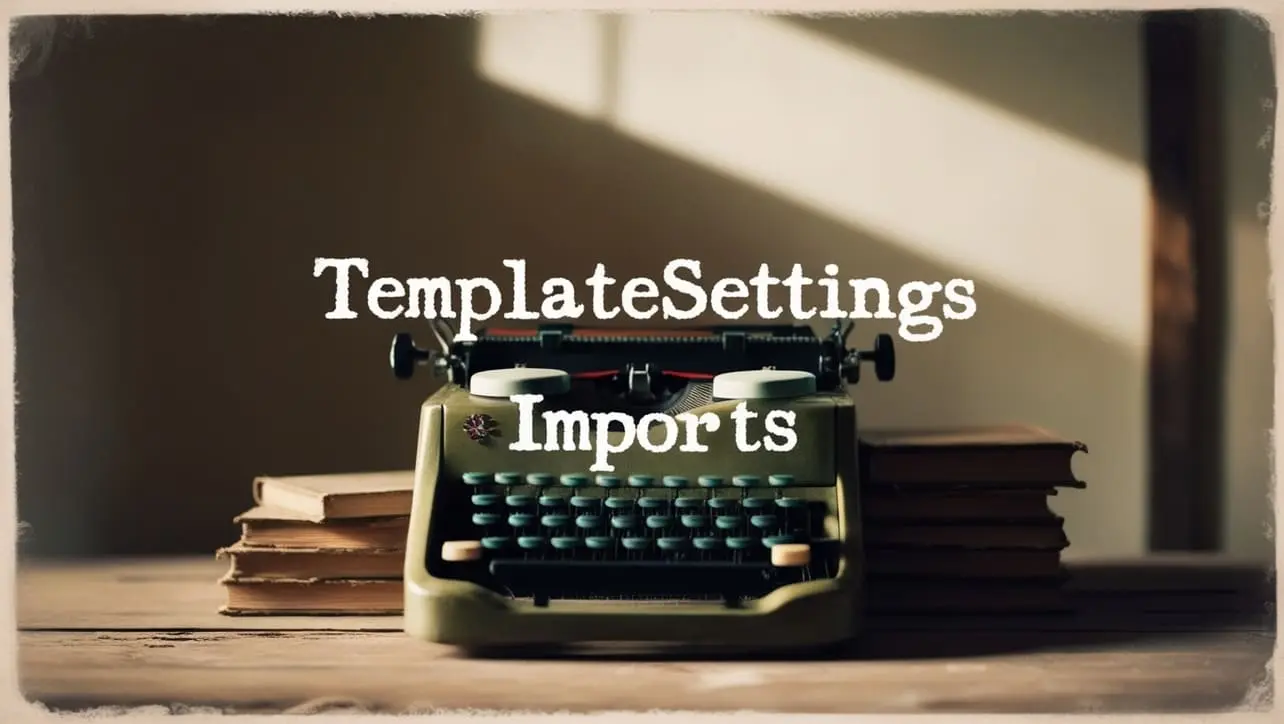
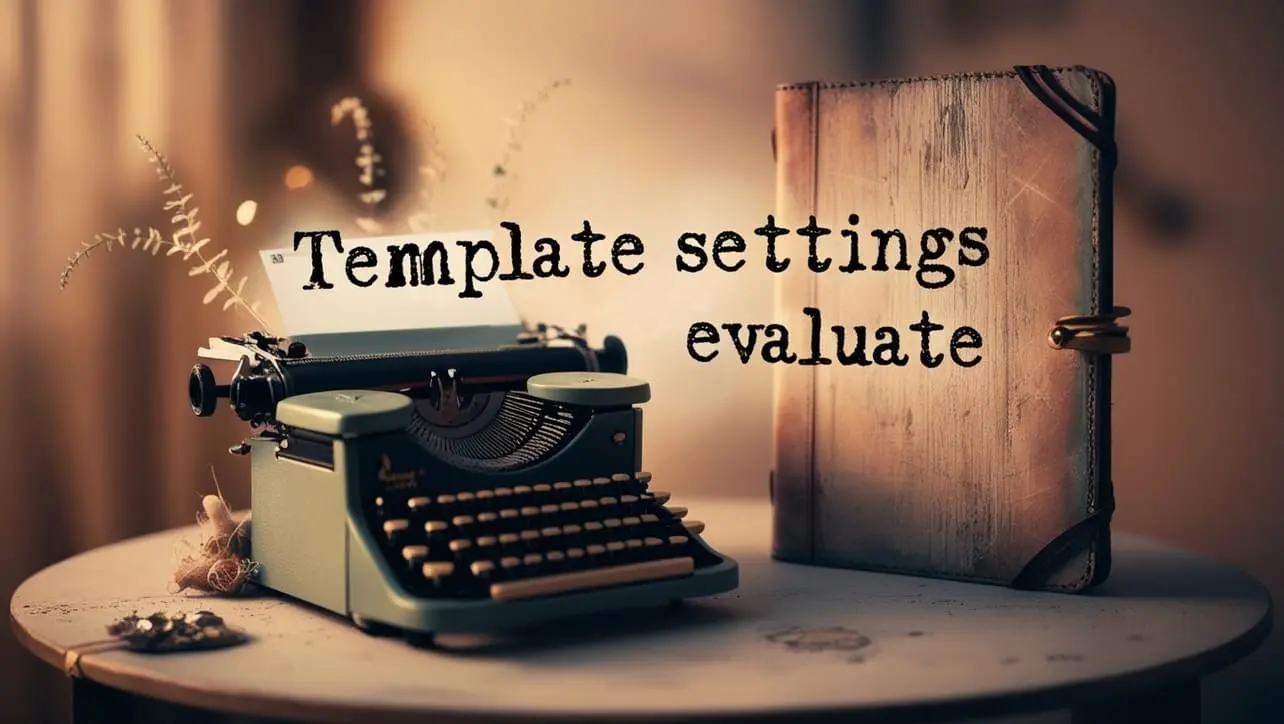
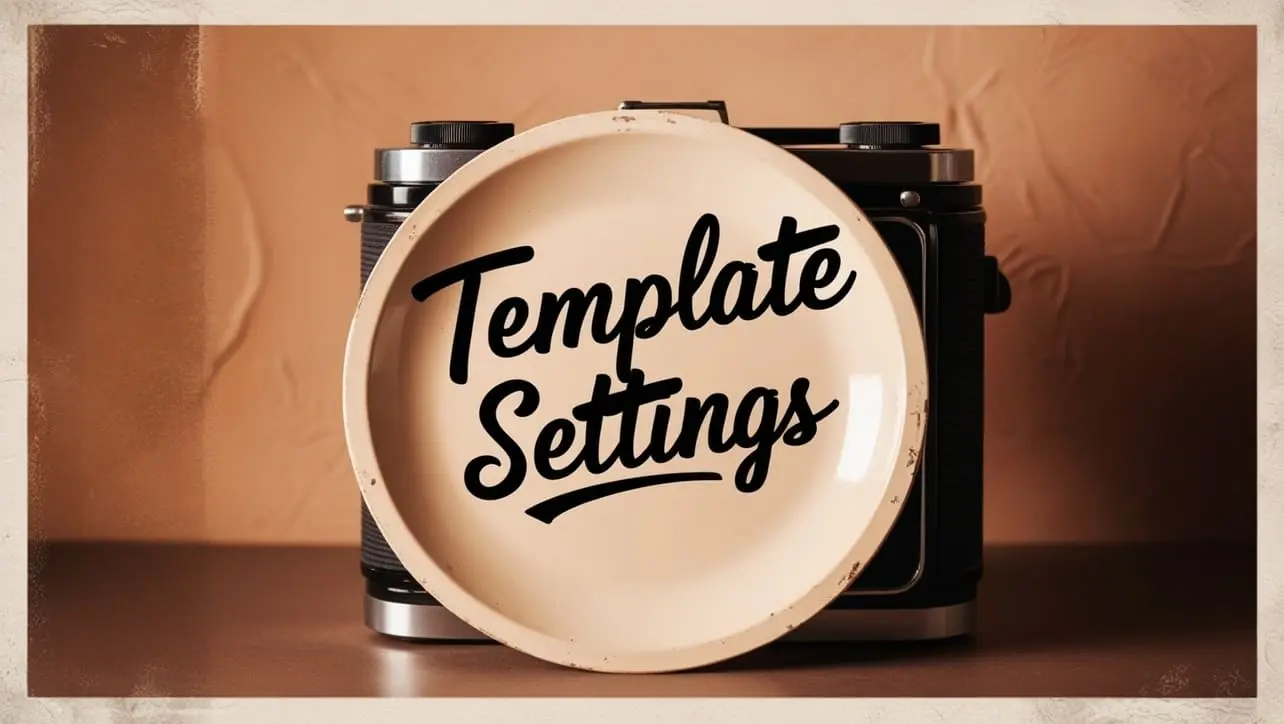
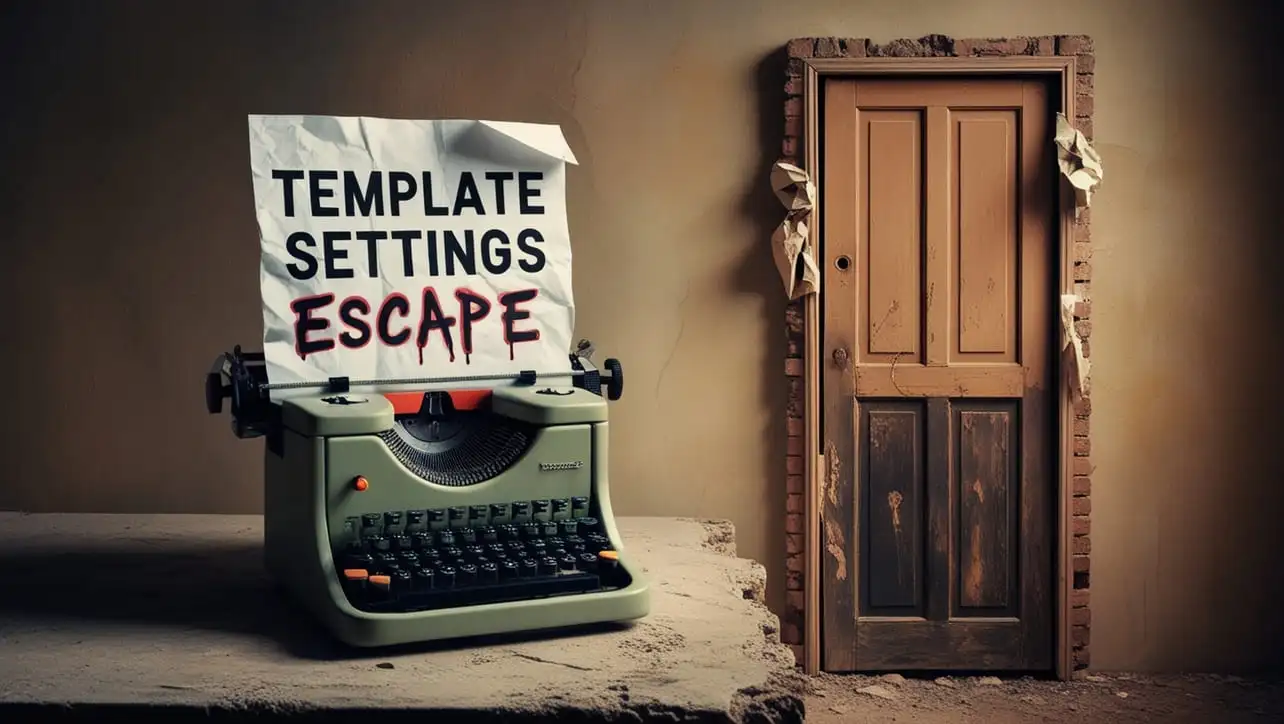
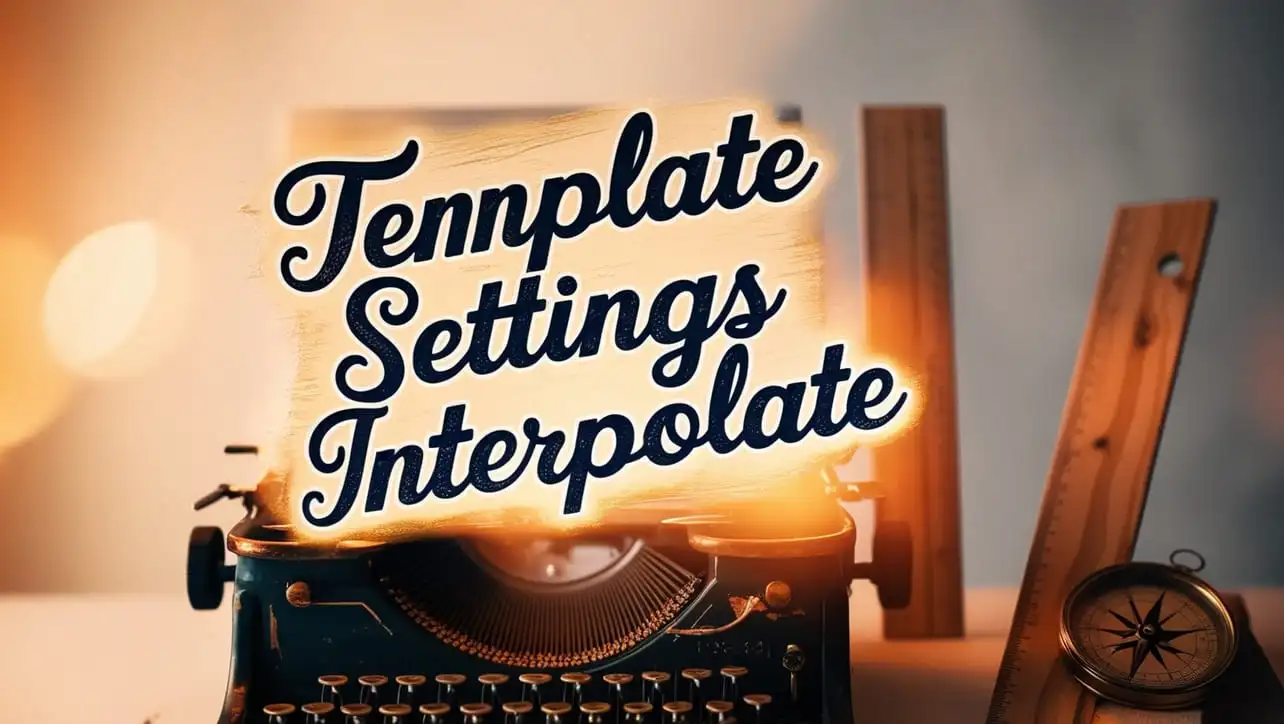
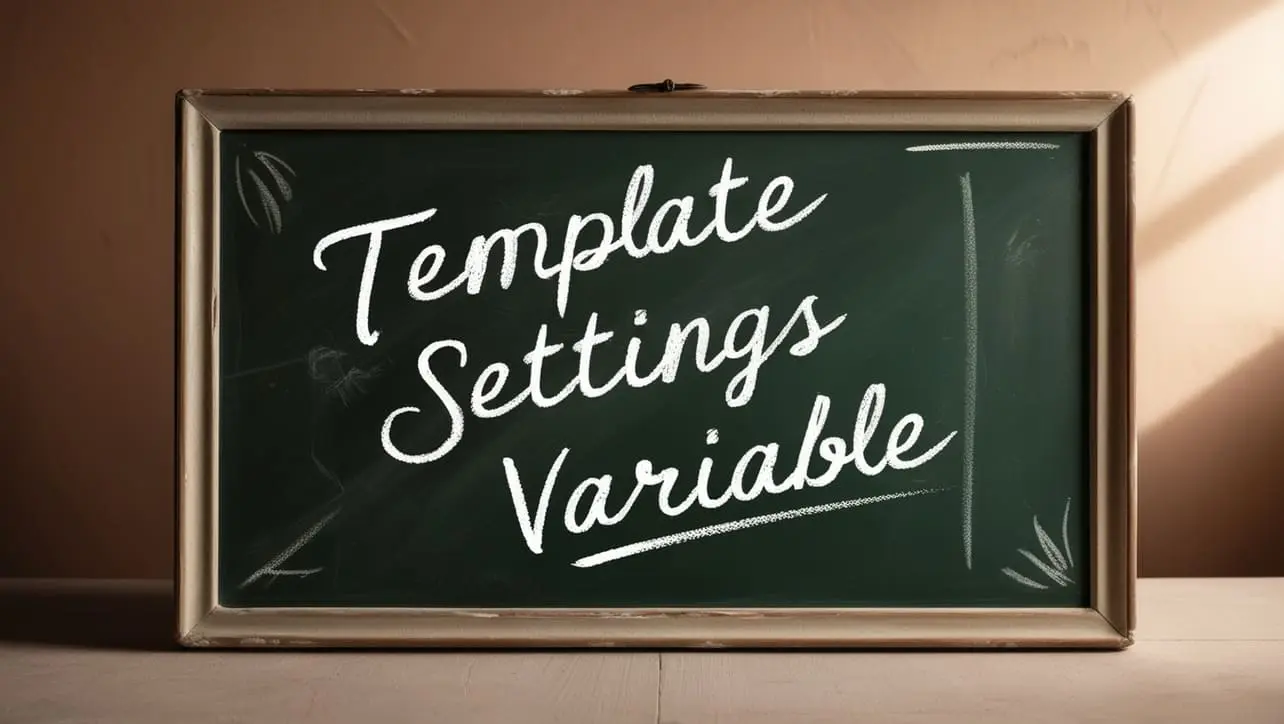
If you have any doubts regarding this article (Lodash _.pullAllWith() Array Method), please comment here. I will help you immediately.