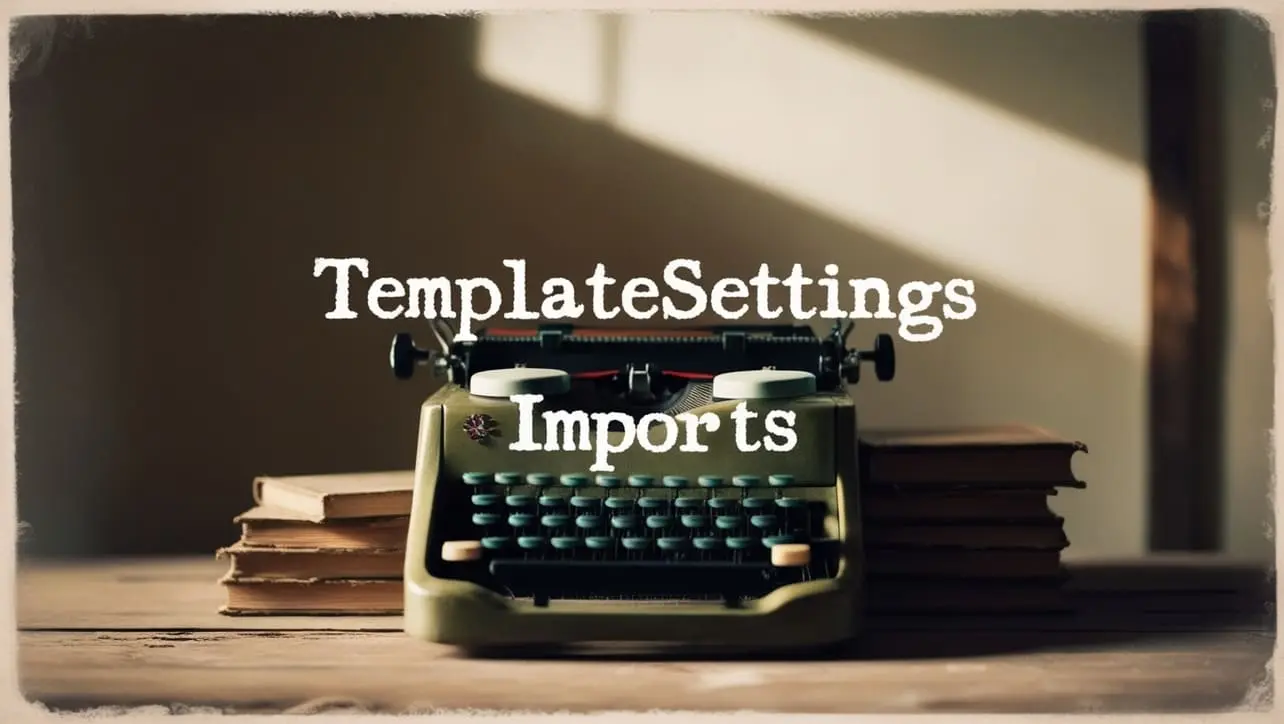
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.pullAllBy() Array Method
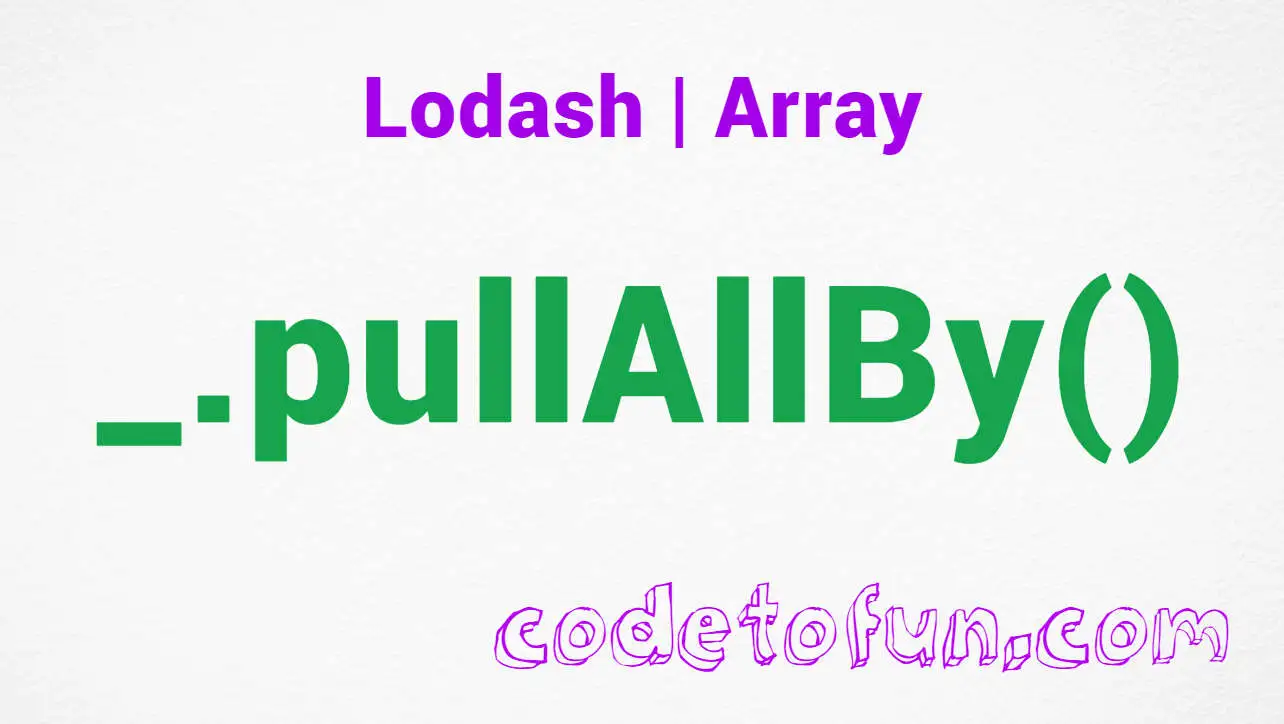
Photo Credit to CodeToFun
🙋 Introduction
Efficiently manipulating arrays is a common task in JavaScript development, and Lodash provides a rich set of utility functions to streamline these operations.
One such powerful method is _.pullAllBy()
. This method allows developers to remove elements from an array based on a specific property, providing a concise and effective solution for array manipulation.
🧠 Understanding _.pullAllBy()
The _.pullAllBy()
method in Lodash is designed to remove elements from an array by comparing a specified property of each element. This can be particularly useful when dealing with arrays of objects and wanting to exclude elements based on a specific property value.
💡 Syntax
_.pullAllBy(array, values, [iteratee=_.identity])
- array: The array to modify.
- values: The values to remove.
- iteratee: The iteratee invoked per element (default is _.identity).
📝 Example
Let's explore a practical example to illustrate how _.pullAllBy()
works:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' },
{ id: 3, name: 'Doe' }
];
const valuesToRemove = [
{ id: 1, name: 'John' },
{ id: 3, name: 'Doe' }
];
_.pullAllBy(originalArray, valuesToRemove, 'id');
console.log(originalArray);
// Output: [{ id: 2, name: 'Jane' }]
In this example, _.pullAllBy()
removes elements from originalArray where the 'id' property matches any of the 'id' properties in valuesToRemove.
🏆 Best Practices
Specify a Relevant Iteratee:
When using
_.pullAllBy()
, ensure that the iteratee function is specified appropriately. This function defines the property used for comparison. In the example above, 'id' is used as the iteratee.example.jsCopiedconst array = [{ id: 1 }, { id: 2 }, { id: 3 }]; const valuesToRemove = [{ id: 2 }]; _.pullAllBy(array, valuesToRemove, 'id'); console.log(array); // Output: [{ id: 1 }, { id: 3 }]
Understand Property Matching:
Be aware that
_.pullAllBy()
removes elements based on property matching. Ensure that the properties being compared have the same type and value to achieve the desired result.example.jsCopiedconst array = [{ id: 1 }, { id: '1' }, { id: 2 }]; const valuesToRemove = [{ id: '1' }]; _.pullAllBy(array, valuesToRemove, 'id'); console.log(array); // Output: [{ id: 1 }, { id: 2 }]
Validate Input Arrays:
Before applying
_.pullAllBy()
, validate that both the source array and the values to be removed are valid arrays. Handle edge cases where the input arrays may be empty or undefined.example.jsCopiedconst array = [{ id: 1 }, { id: 2 }, { id: 3 }]; const valuesToRemove = 'not an array'; if (Array.isArray(array) && Array.isArray(valuesToRemove)) { _.pullAllBy(array, valuesToRemove, 'id'); console.log(array); } else { console.error('Invalid input arrays'); }
📚 Use Cases
Filtering Unique Elements:
Use
_.pullAllBy()
to filter out unique elements from an array of objects based on a specific property.example.jsCopiedconst arrayWithDuplicates = /* ...an array with duplicate objects... */; const uniqueProperty = 'id'; _.pullAllBy(arrayWithDuplicates, arrayWithDuplicates, uniqueProperty); console.log(arrayWithDuplicates);
Simplifying Array Differences:
When comparing two arrays of objects and wanting to find the difference based on a specific property,
_.pullAllBy()
can be a concise solution.example.jsCopiedconst originalData = /* ...an array of objects... */; const newData = /* ...an updated array of objects... */; const propertyToCompare = 'id'; _.pullAllBy(originalData, newData, propertyToCompare); console.log(originalData);
🎉 Conclusion
The _.pullAllBy()
method in Lodash is a valuable asset for developers dealing with arrays of objects. It simplifies the process of removing elements based on a specified property, offering a clean and efficient solution. By incorporating this method into your code, you can enhance the manageability and clarity of array manipulation tasks.
Explore the capabilities of _.pullAllBy()
and optimize your array operations with Lodash!
👨💻 Join our Community:
Author
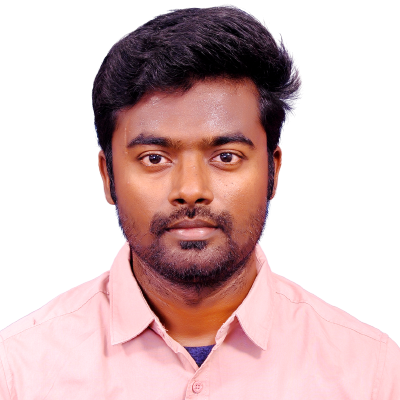
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
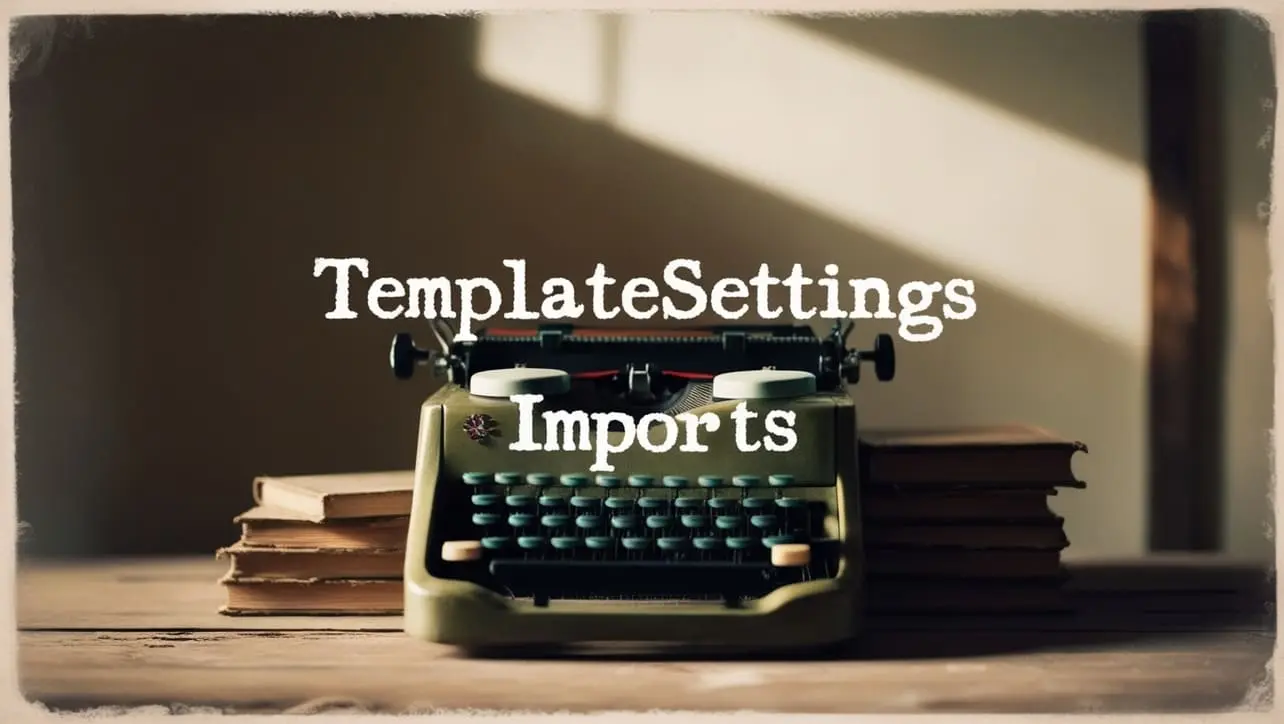
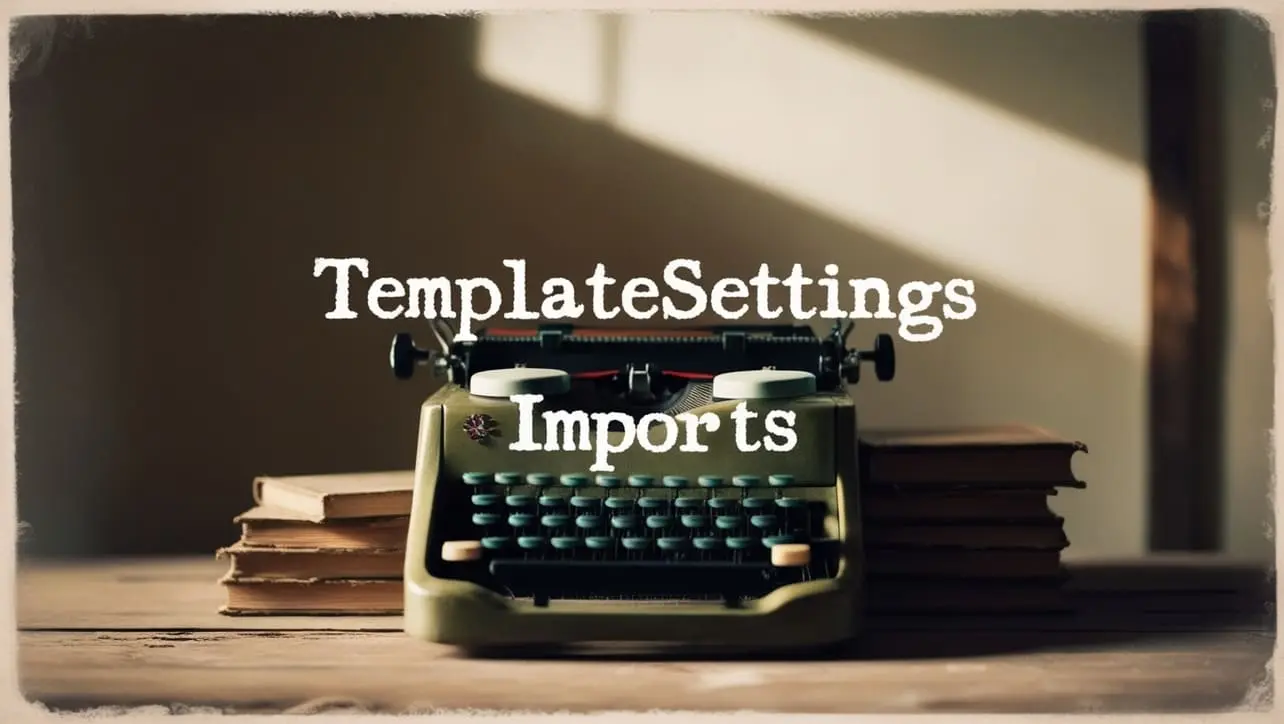
Lodash _.templateSettings.imports Property
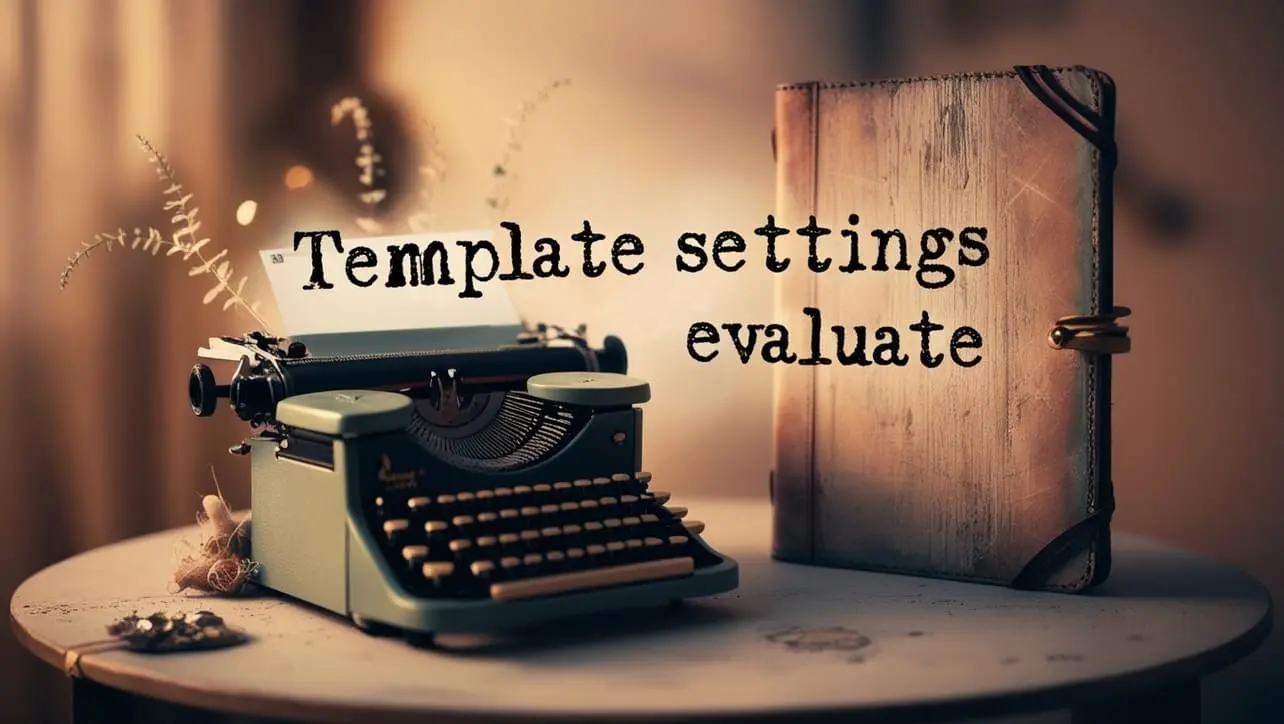
Lodash _.templateSettings.evaluate Property
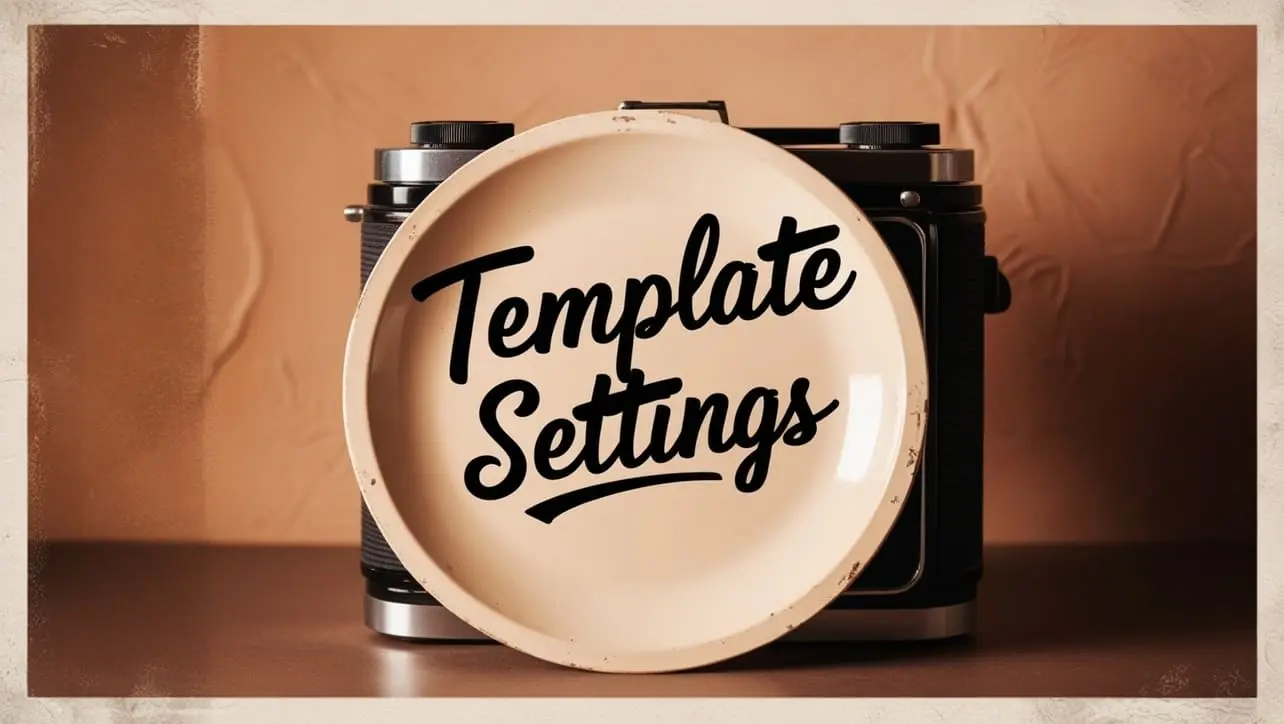
Lodash _.templateSettings Property
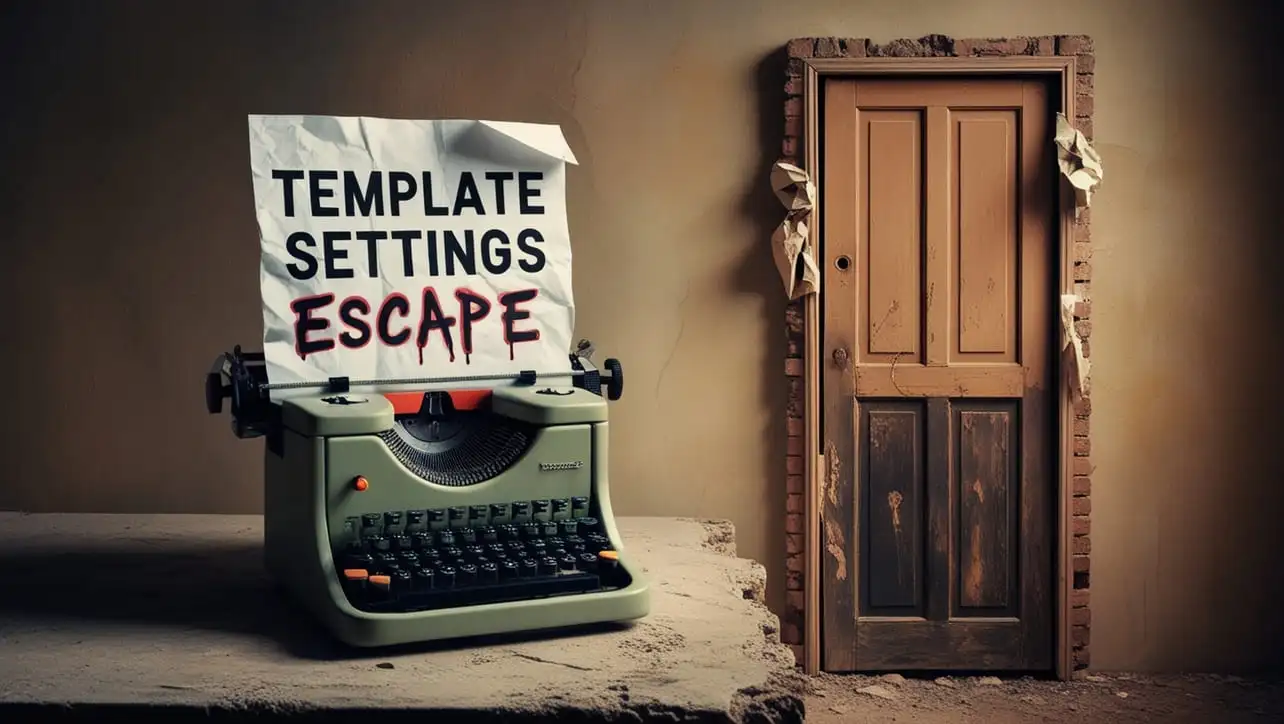
Lodash _.templateSettings.escape Property
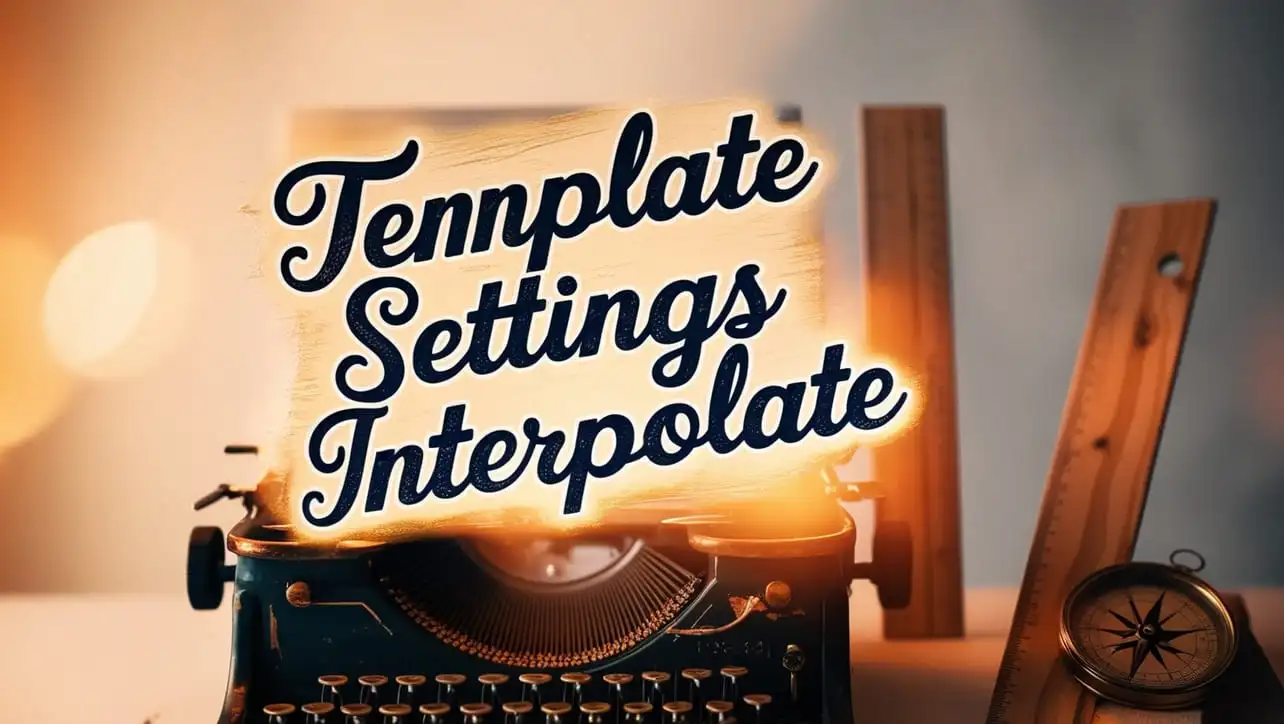
Lodash _.templateSettings.interpolate Property
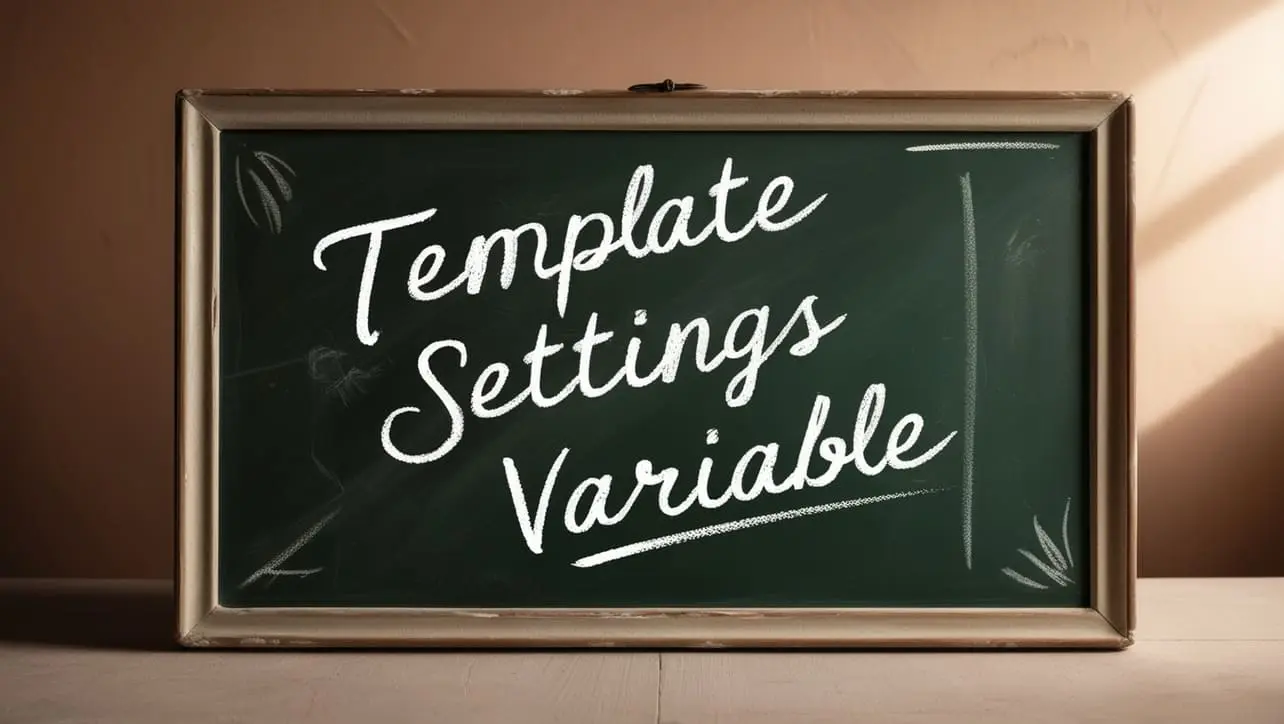
If you have any doubts regarding this article (Lodash _.pullAllBy() Array Method), please comment here. I will help you immediately.