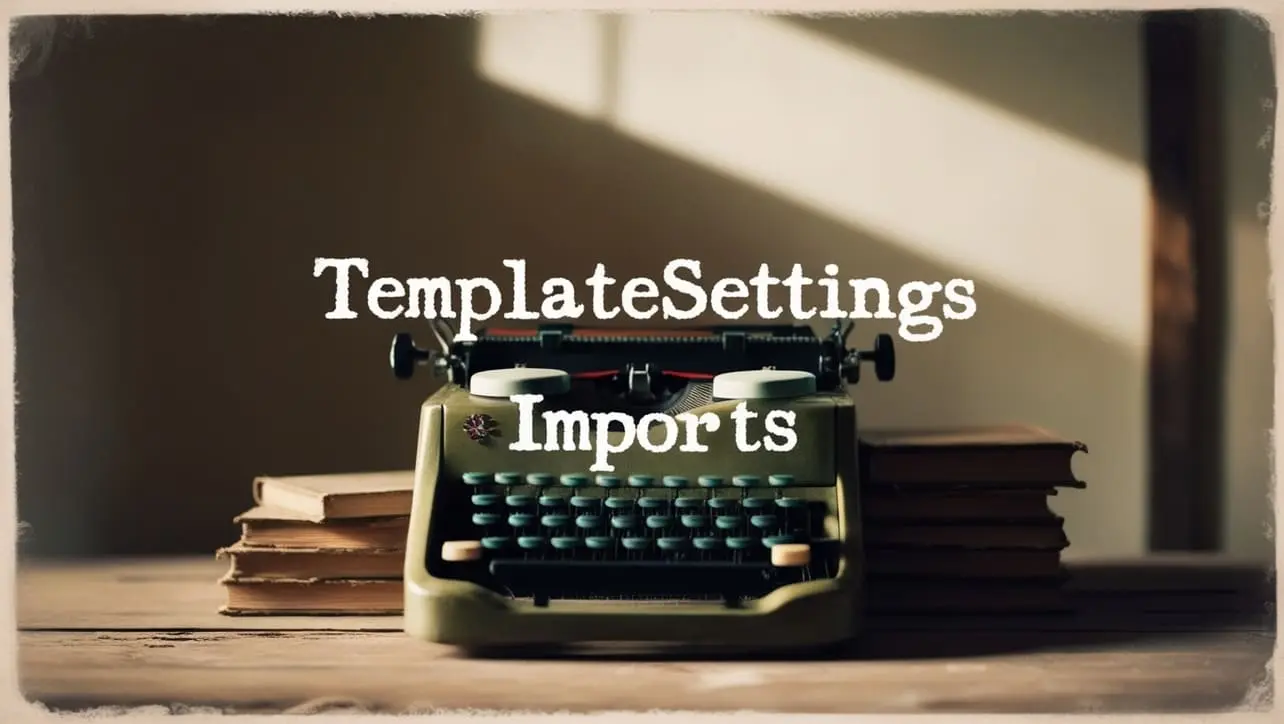
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.pullAll() Array Method
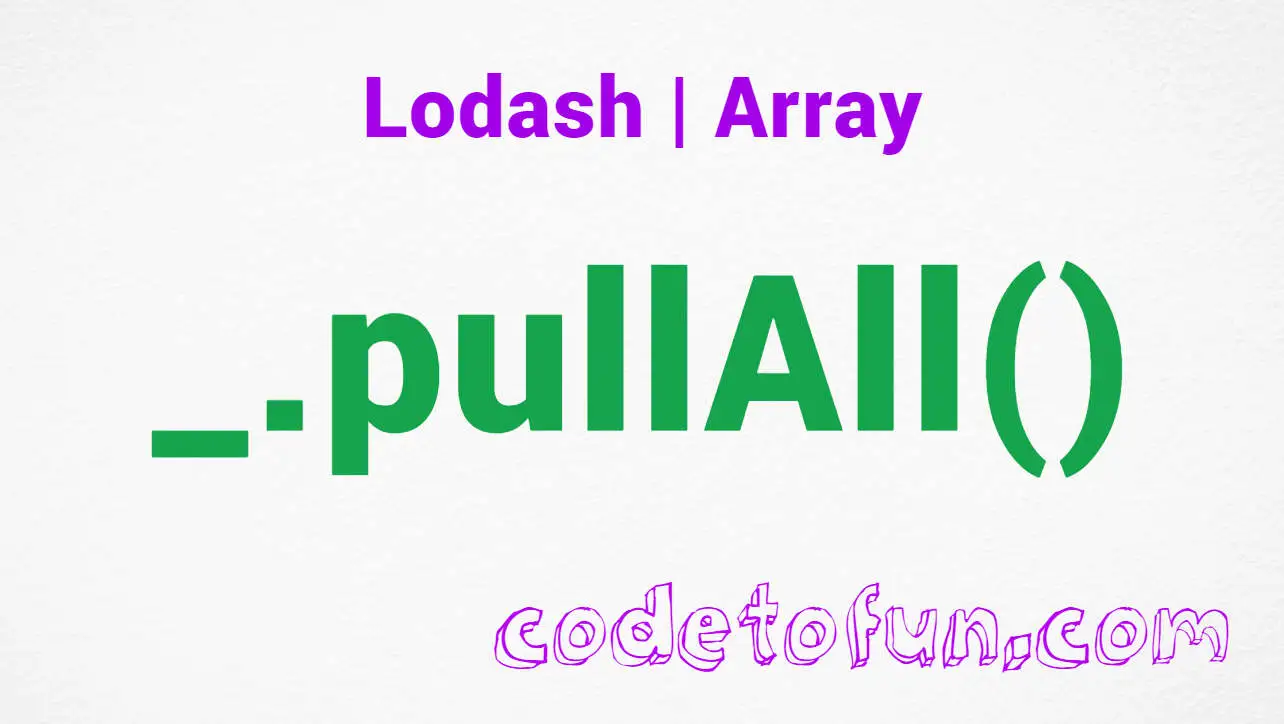
Photo Credit to CodeToFun
🙋 Introduction
Efficiently modifying arrays is a common task in JavaScript development, and the Lodash library provides powerful utility functions to streamline such operations.
One such function is _.pullAll()
, which simplifies the process of removing specified values from an array. This method is valuable for developers seeking an elegant and performant solution for array manipulation.
🧠 Understanding _.pullAll()
The _.pullAll()
method in Lodash is designed to remove all occurrences of specified values from an array. This can be particularly useful when you need to clean up or filter an array by excluding specific elements.
💡 Syntax
_.pullAll(array, values)
- array: The array to modify.
- values: The values to remove.
📝 Example
Let's explore a practical example to illustrate the usage of _.pullAll()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5, 3, 6, 7, 8, 9];
const valuesToRemove = [3, 5, 7];
_.pullAll(originalArray, valuesToRemove);
console.log(originalArray);
// Output: [1, 2, 4, 6, 8, 9]
In this example, the values [3, 5, 7] are removed from the originalArray, resulting in a modified array.
🏆 Best Practices
Clone Arrays Before Modification:
To avoid unintended side effects, consider cloning the array before applying
_.pullAll()
. This ensures that the original array remains unchanged.example.jsCopiedconst originalArray = [/* ... */]; const valuesToRemove = [/* ... */]; const clonedArray = [...originalArray]; _.pullAll(clonedArray, valuesToRemove); console.log(originalArray); // Unmodified console.log(clonedArray); // Modified
Validate Inputs:
Ensure that both the input array and the array of values to remove are valid before applying
_.pullAll()
.example.jsCopiedif (!Array.isArray(originalArray) || !Array.isArray(valuesToRemove)) { console.error('Invalid input: Both array and values must be arrays.'); return; } _.pullAll(originalArray, valuesToRemove); console.log(originalArray);
📚 Use Cases
Data Filtering:
_.pullAll()
is handy for filtering out specific values from an array, leaving only the desired elements.example.jsCopiedconst dataToFilter = [/* ...some data... */; const valuesToExclude = [/* ...values to exclude... */]; _.pullAll(dataToFilter, valuesToExclude); console.log(dataToFilter);
Cleanup Operations:
When working with datasets, you might need to perform cleanup operations by removing unwanted values.
example.jsCopiedconst messyData = [/* ...some data... */; const valuesToRemove = [/* ...values to remove... */]; _.pullAll(messyData, valuesToRemove); console.log(messyData);
🎉 Conclusion
The _.pullAll()
method in Lodash provides a clean and efficient way to remove specified values from an array. Whether you're filtering data or performing cleanup operations, this method proves to be a valuable addition to your JavaScript toolkit. By understanding its usage and best practices, you can leverage _.pullAll()
to enhance the clarity and efficiency of your array manipulation tasks.
Explore the versatility of Lodash and discover how _.pullAll()
can elevate your array manipulation capabilities!
👨💻 Join our Community:
Author
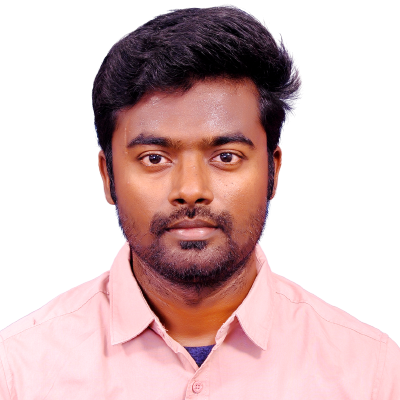
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
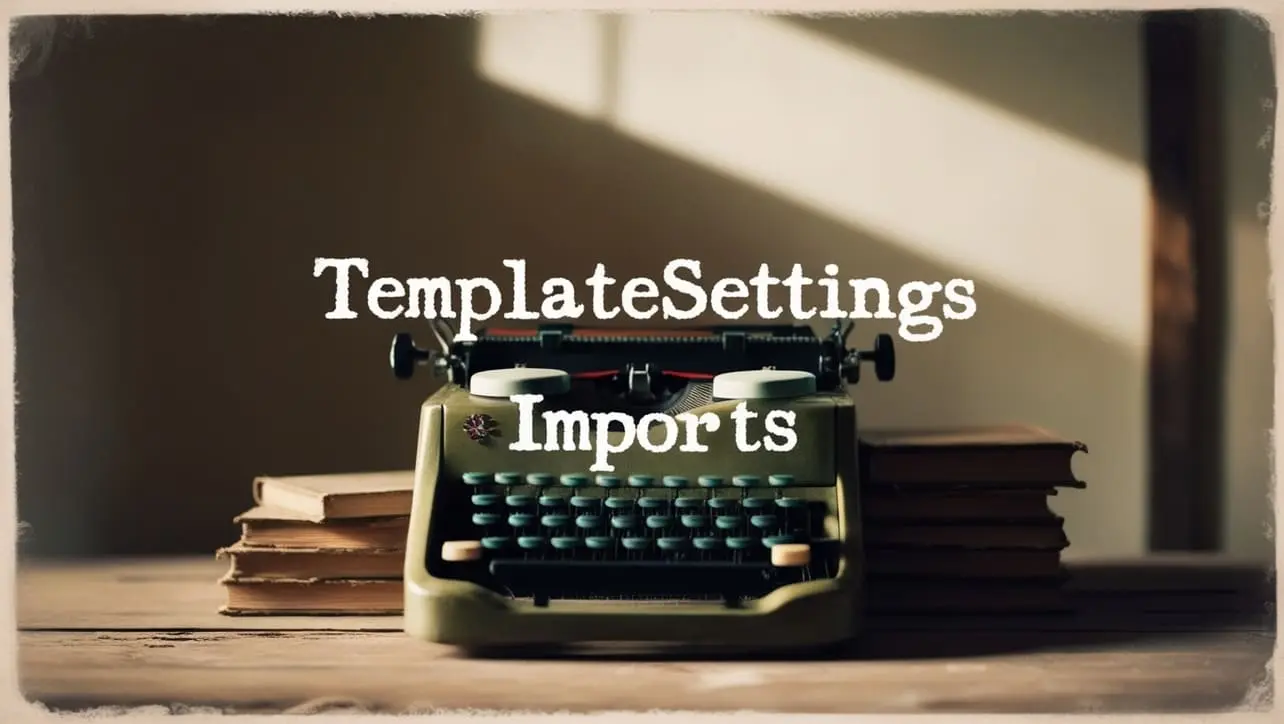
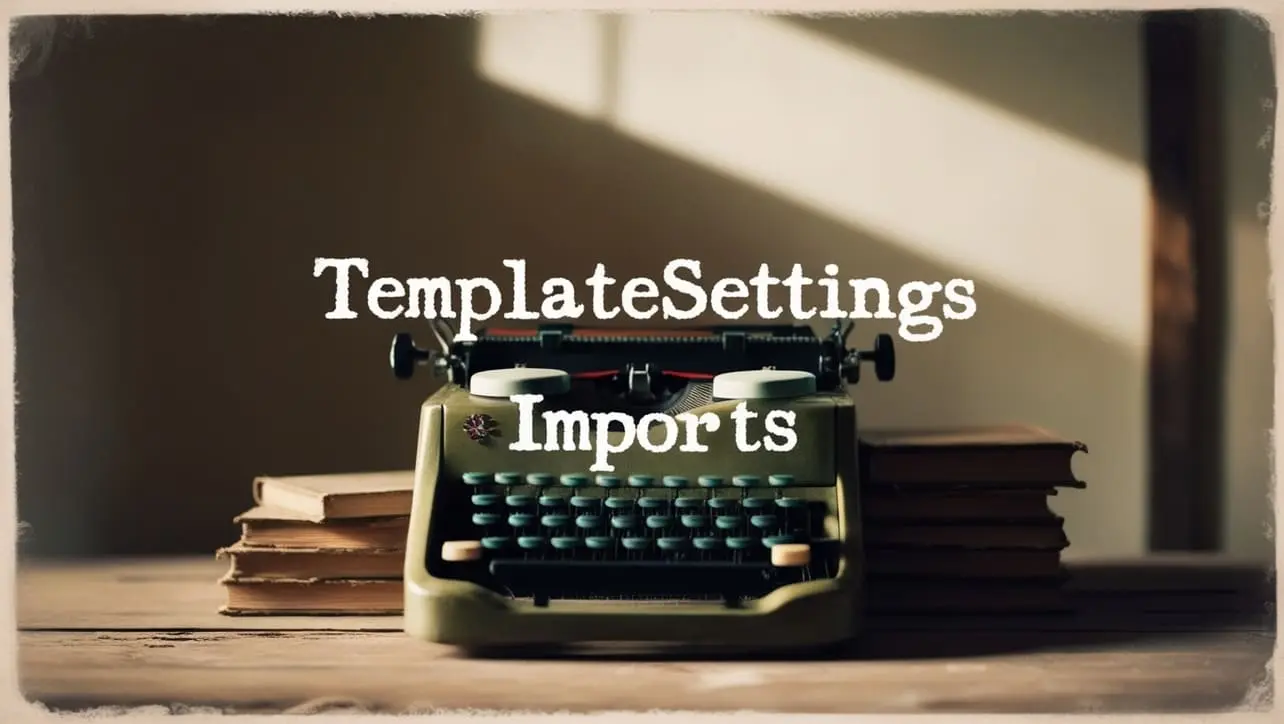
Lodash _.templateSettings.imports Property
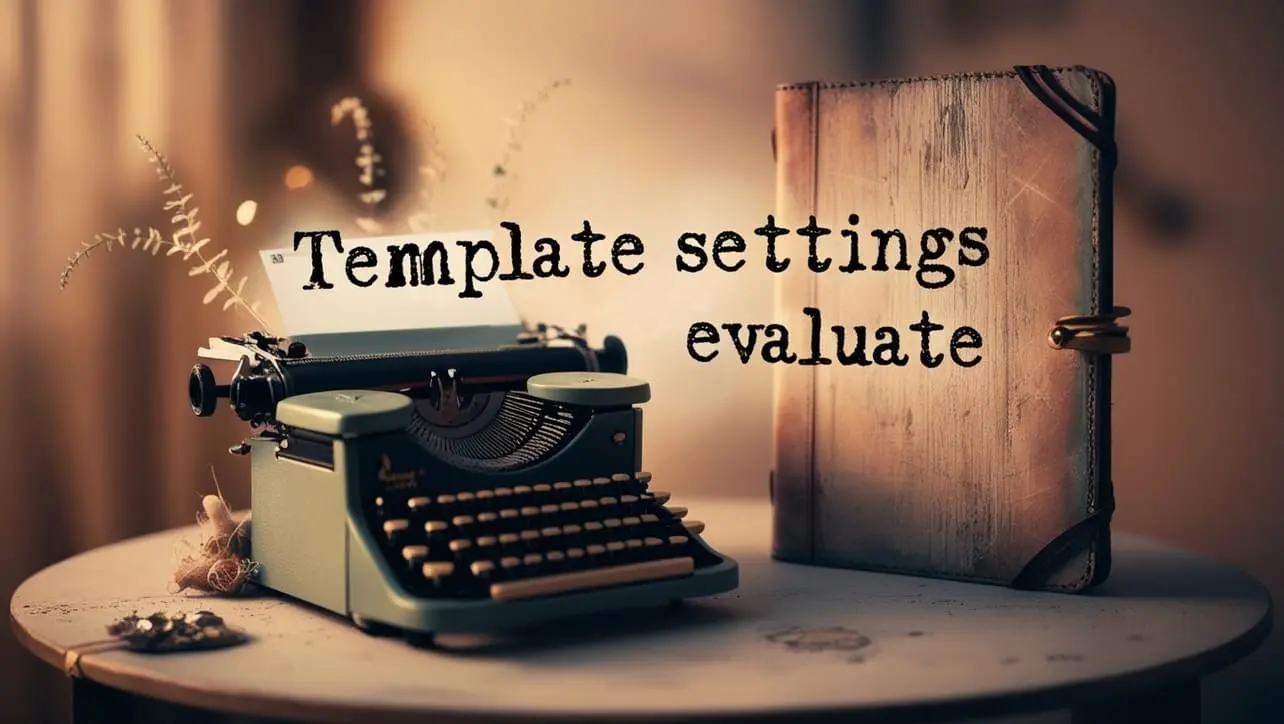
Lodash _.templateSettings.evaluate Property
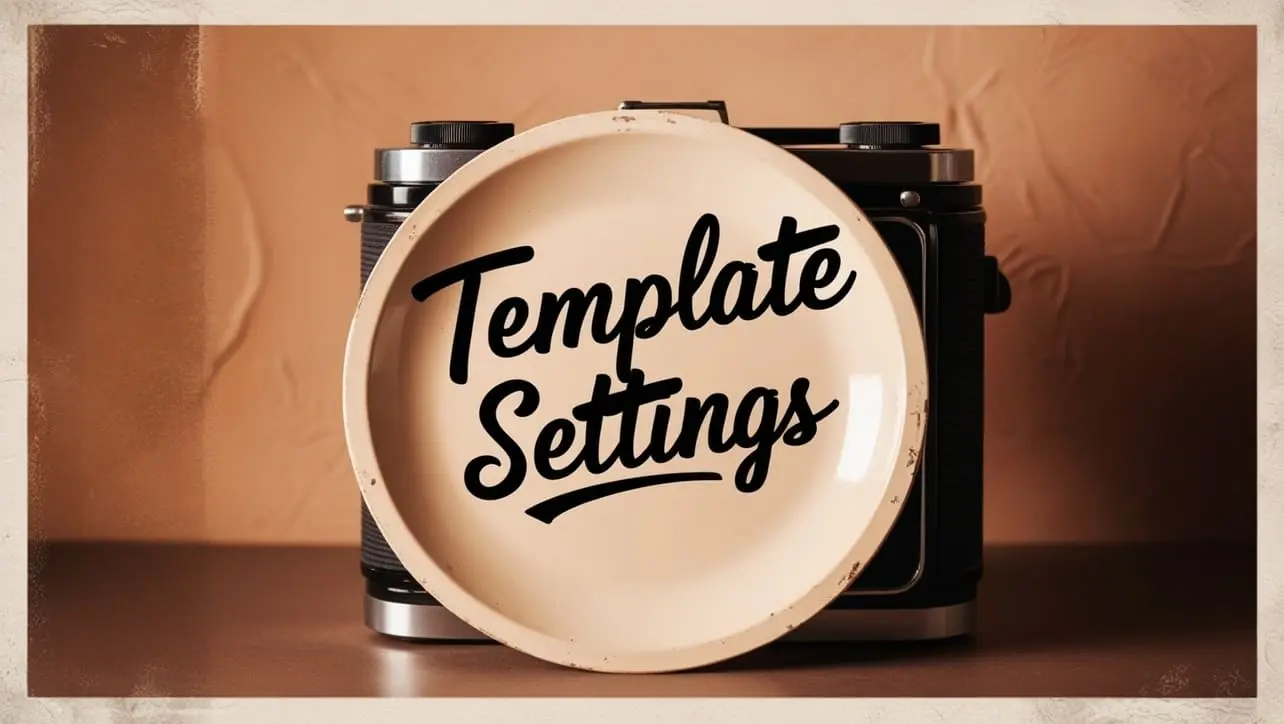
Lodash _.templateSettings Property
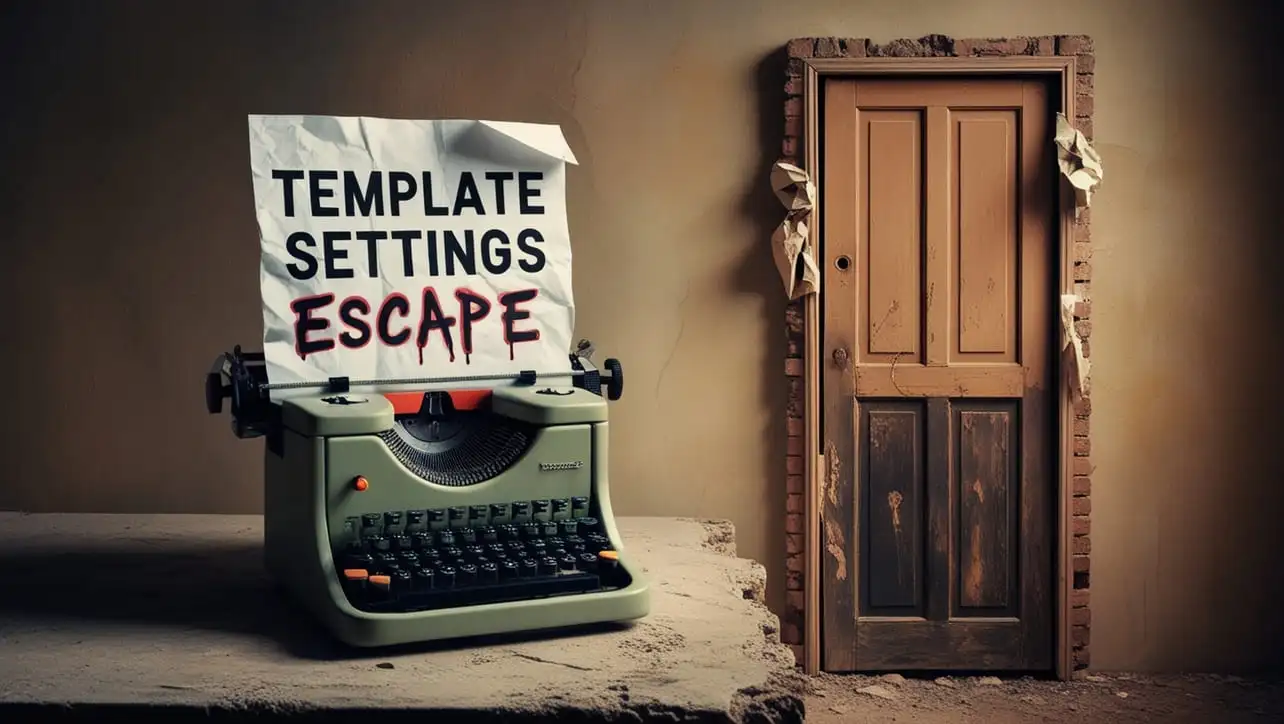
Lodash _.templateSettings.escape Property
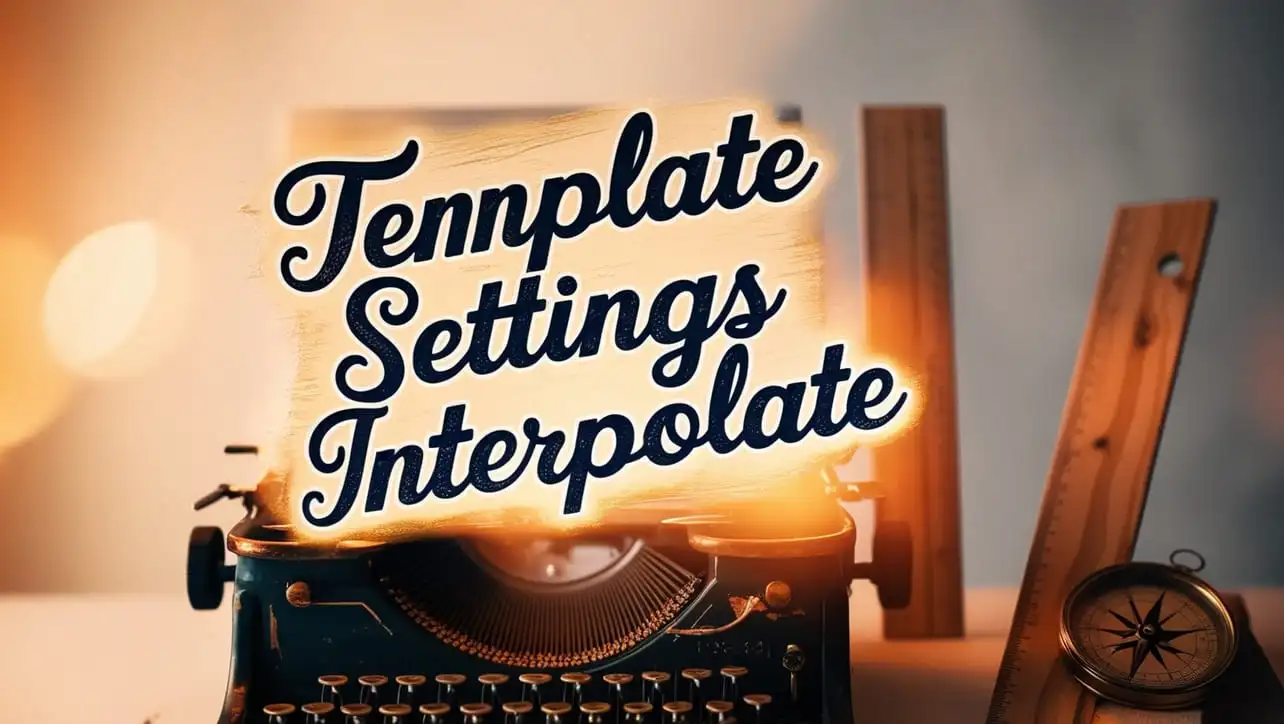
Lodash _.templateSettings.interpolate Property
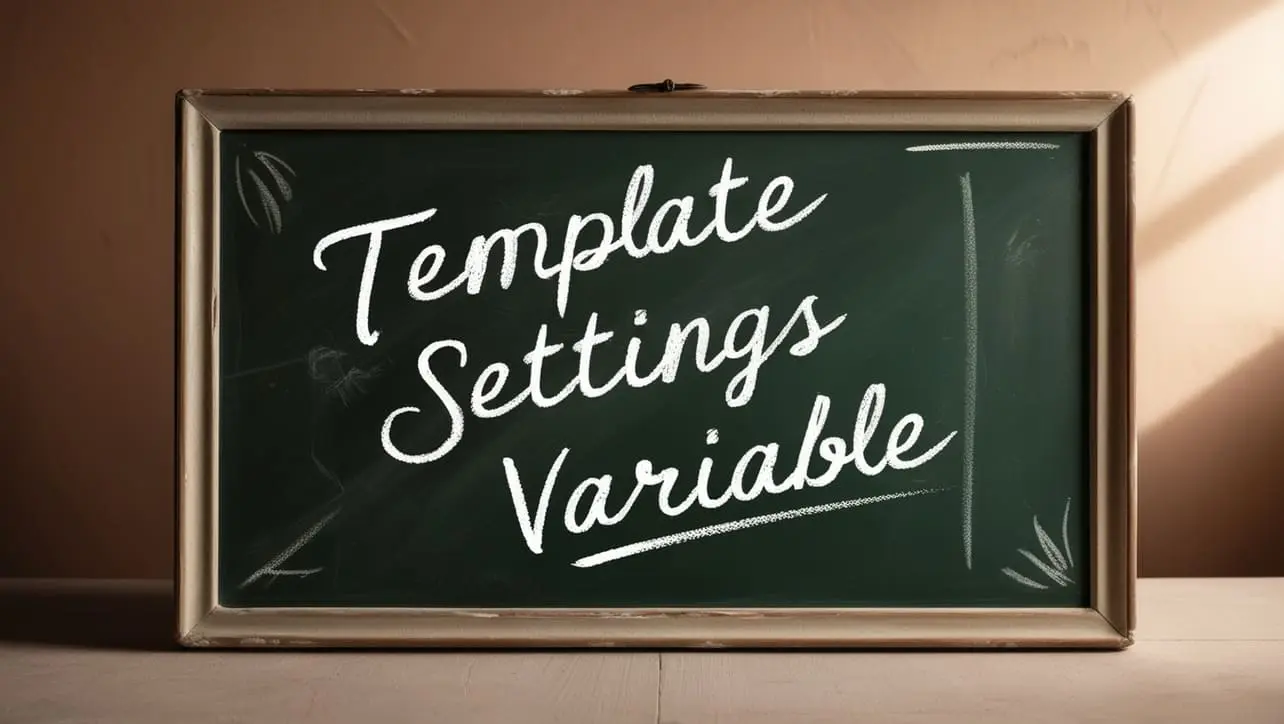
If you have any doubts regarding this article (Lodash _.pullAll() Array Method), please comment here. I will help you immediately.