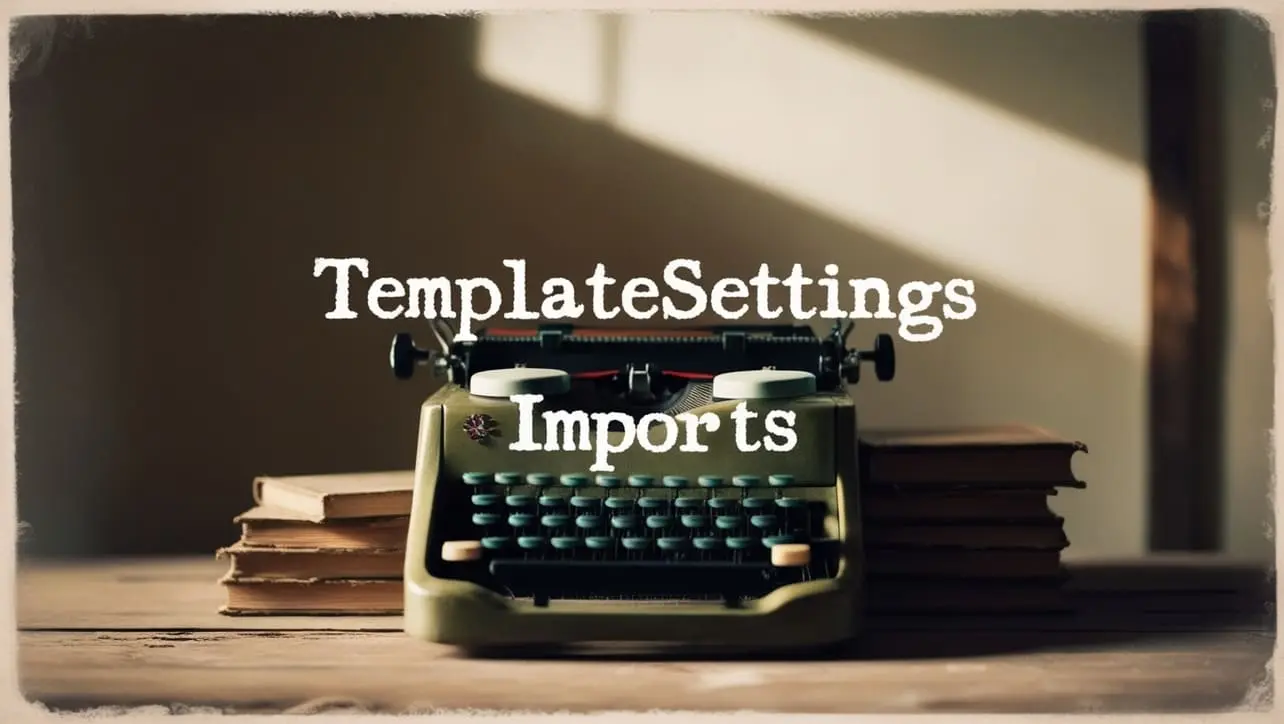
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.nth() Array Method
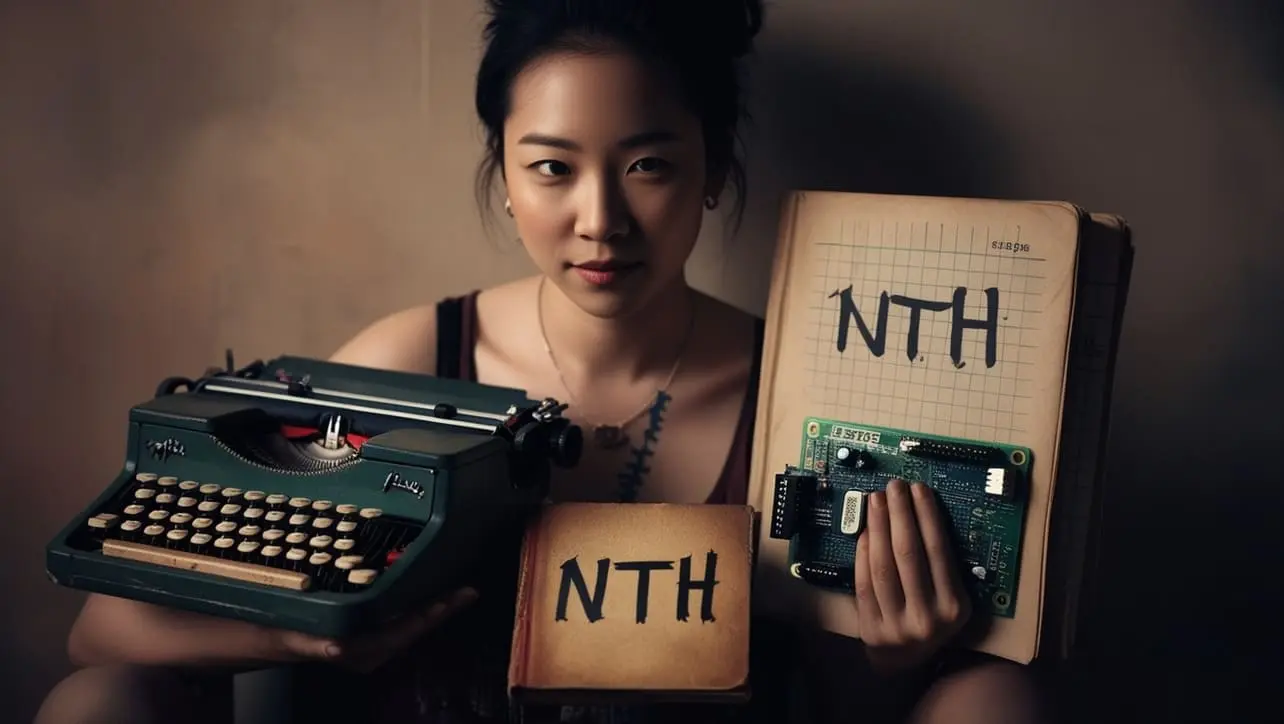
Photo Credit to CodeToFun
🙋 Introduction
When it comes to array manipulation in JavaScript, having efficient methods at your disposal is essential.
Lodash, a popular utility library, offers a wide range of functions, including the versatile _.nth()
method.
This method allows developers to access elements in an array with ease, offering flexibility and convenience.
🧠 Understanding _.nth()
The _.nth()
method in Lodash enables you to retrieve the nth element from an array. This can be particularly handy when you need to access specific elements without resorting to lengthy and error-prone array indexing.
💡 Syntax
_.nth(array, [n=0])
- array: The array from which to retrieve the element.
- n: The index of the element to retrieve (default is 0).
📝 Example
Let's delve into a practical example to illustrate the power of _.nth()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const myArray = [10, 20, 30, 40, 50];
const thirdElement = _.nth(myArray, 2);
console.log(thirdElement);
// Output: 30
In this example, the thirdElement variable holds the value of the third element in the myArray array.
🏆 Best Practices
Validating Inputs:
Before utilizing
_.nth()
, validate that the input array is valid, and if necessary, ensure the index is within bounds.validating-inputs.jsCopiedconst invalidArray = 'Not an array'; const indexToRetrieve = 2; if (!Array.isArray(invalidArray) || indexToRetrieve < 0 || indexToRetrieve >= invalidArray.length) { console.error('Invalid input array or index'); return; } const retrievedElement = _.nth(invalidArray, indexToRetrieve); console.log(retrievedElement);
Default Values:
Consider providing a default value or handling cases where the specified index is out of bounds to prevent unexpected behavior.
default-values.jsCopiedconst outOfBoundsIndex = 10; const defaultValue = 'Default'; const elementWithDefault = _.nth(myArray, outOfBoundsIndex, defaultValue); console.log(elementWithDefault); // Output: 'Default'
Negative Indices:
Lodash
_.nth()
also supports negative indices, providing a convenient way to access elements from the end of the array.negative-indices.jsCopiedconst lastElement = _.nth(myArray, -1); console.log(lastElement); // Output: 50
📚 Use Cases
Simplifying Access:
_.nth()
simplifies the process of accessing specific elements, especially when the index is dynamic or based on certain conditions.simplifying-access.jsCopiedconst dynamicIndex = /* ...calculate dynamically... */; const dynamicElement = _.nth(myArray, dynamicIndex); console.log(dynamicElement);
Handling User Input:
When dealing with user input or external data,
_.nth()
can be used to safely access elements, reducing the risk of errors.handling-user-input.jsCopiedconst userInputIndex = /* ...get index from user input... */; const safeElement = _.nth(myArray, userInputIndex); console.log(safeElement);
🎉 Conclusion
The _.nth()
method in Lodash is a valuable tool for efficiently retrieving elements from arrays. Whether you're working with dynamic indices, user input, or need a concise way to access array elements, _.nth()
provides a clean and convenient solution.
Explore the capabilities of Lodash and enhance your array manipulation tasks with the simplicity and power of _.nth()
!
👨💻 Join our Community:
Author
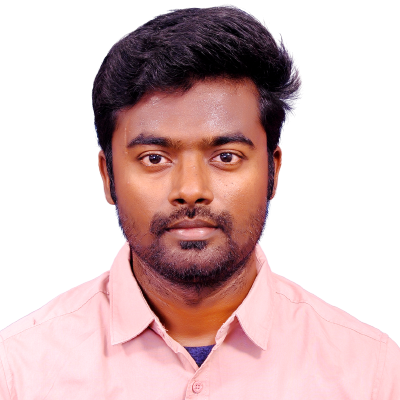
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
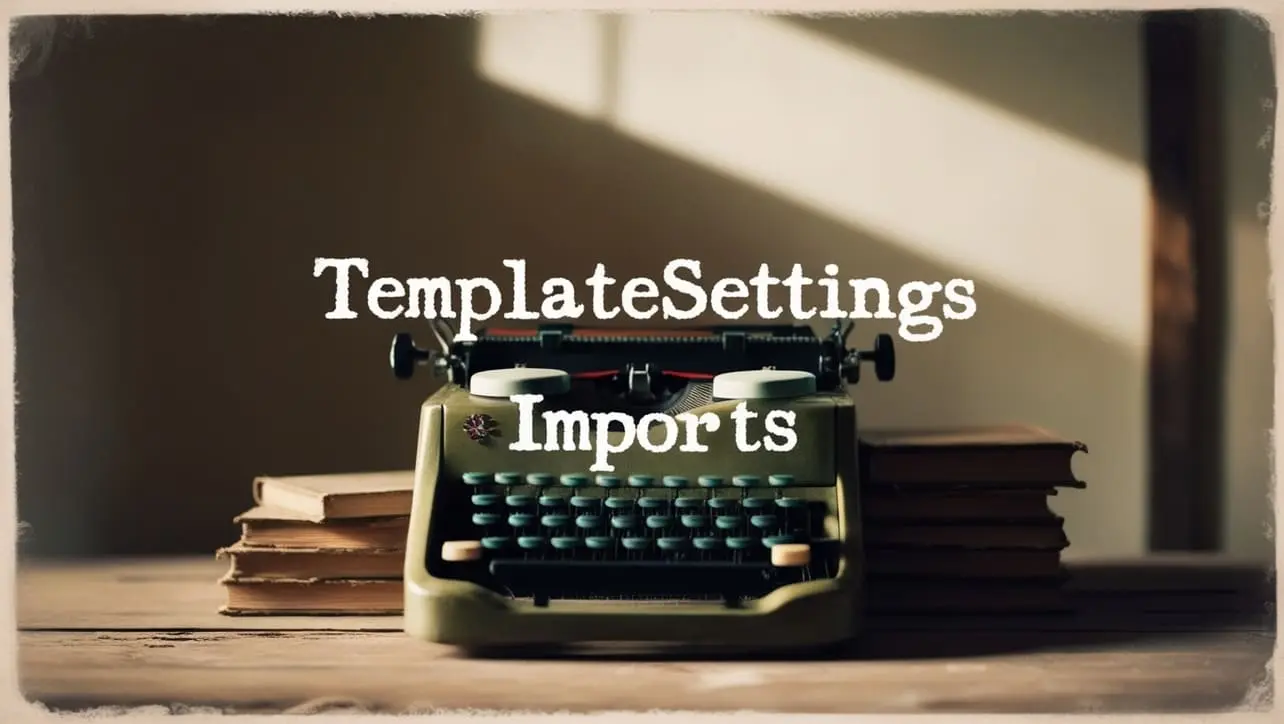
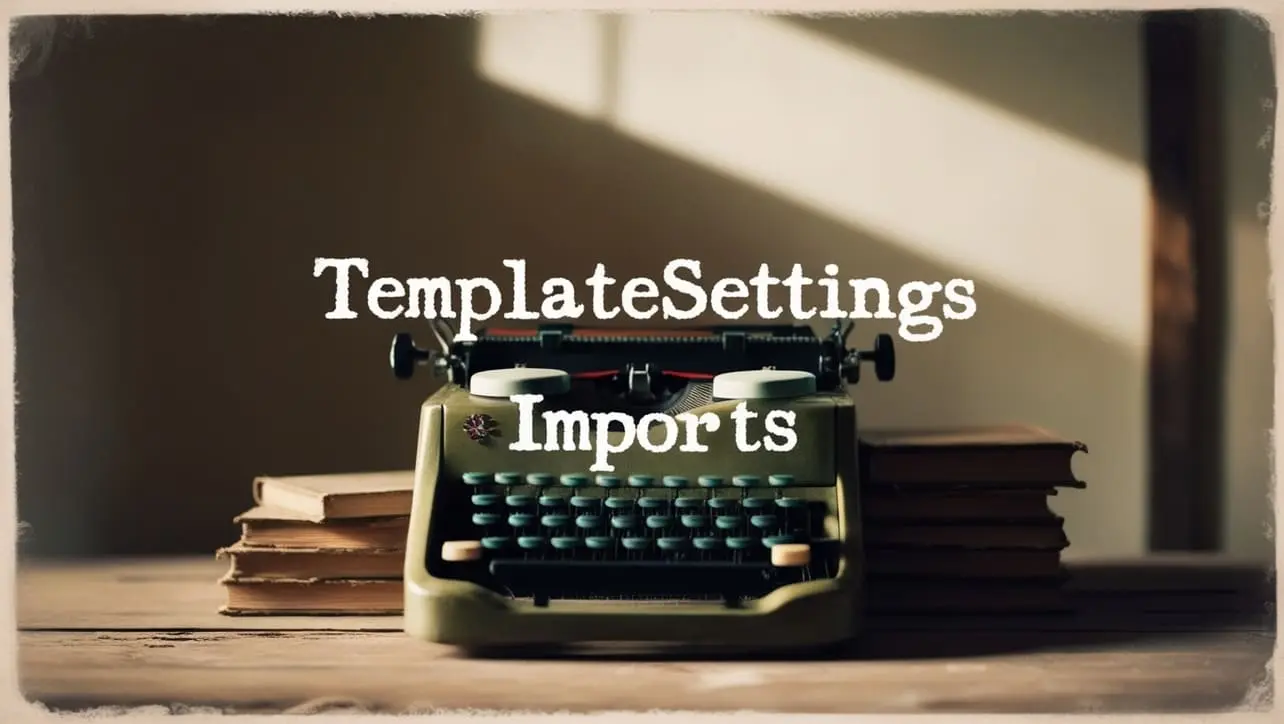
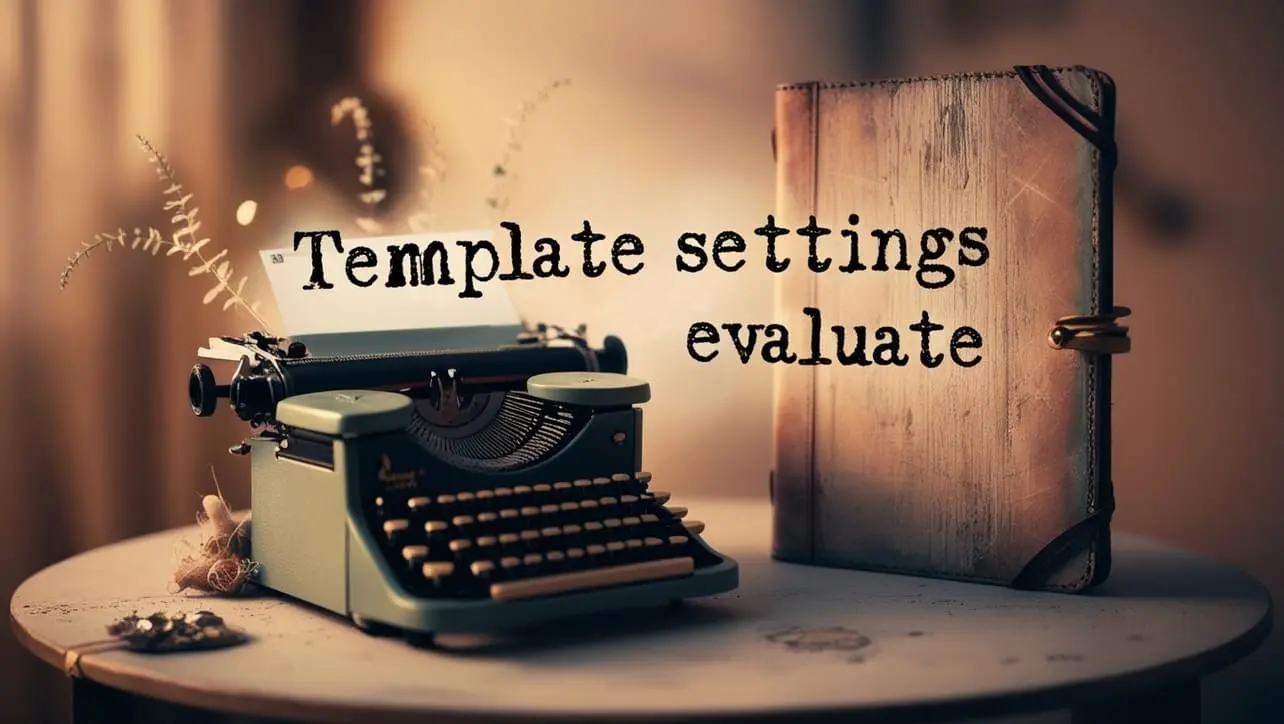
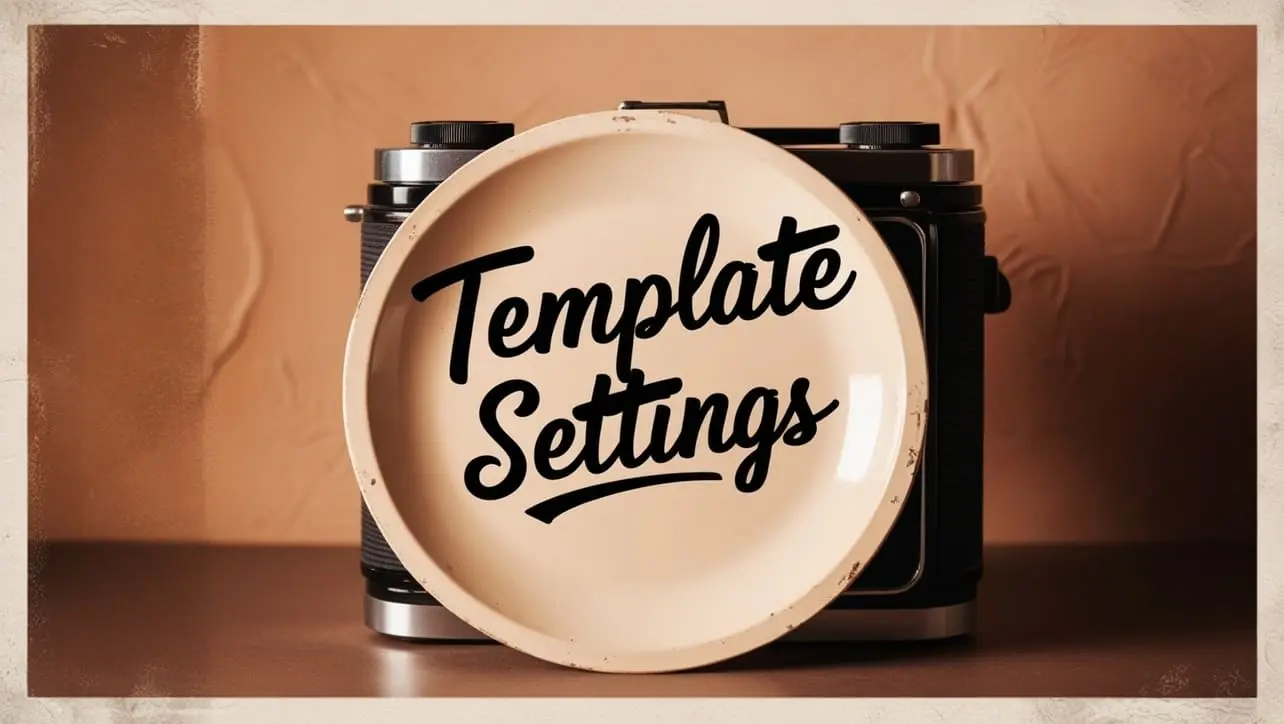
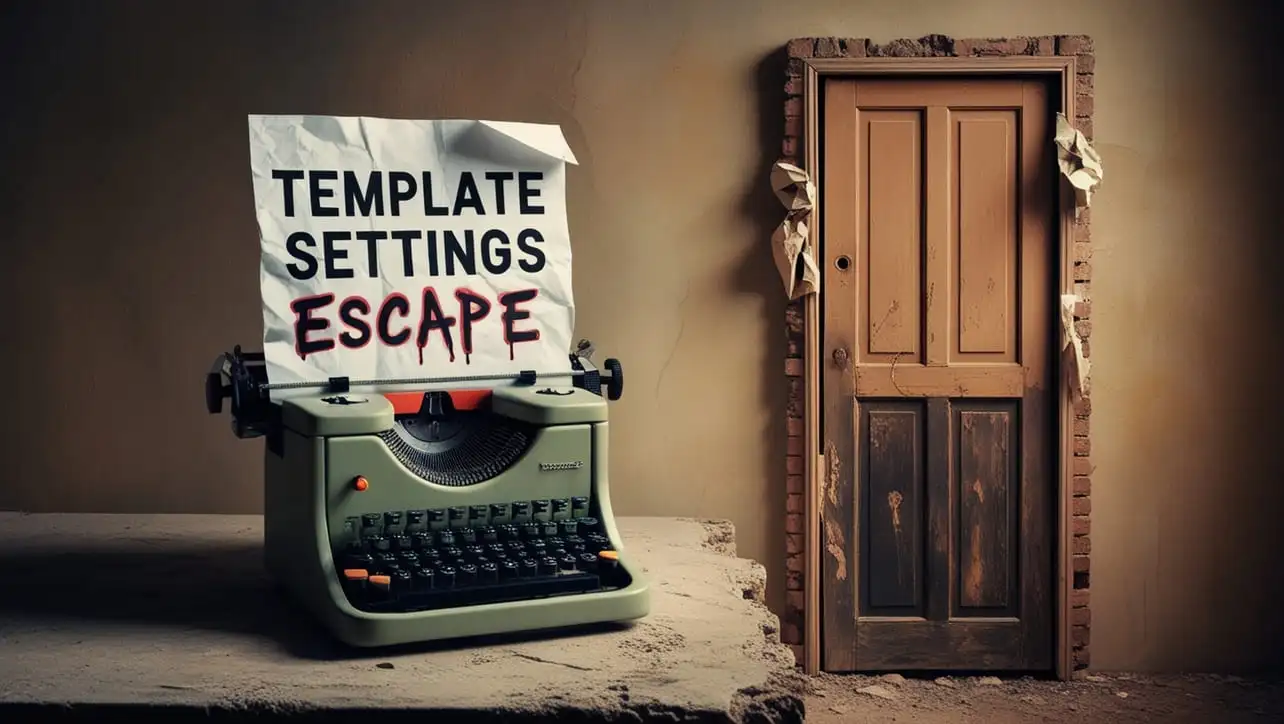
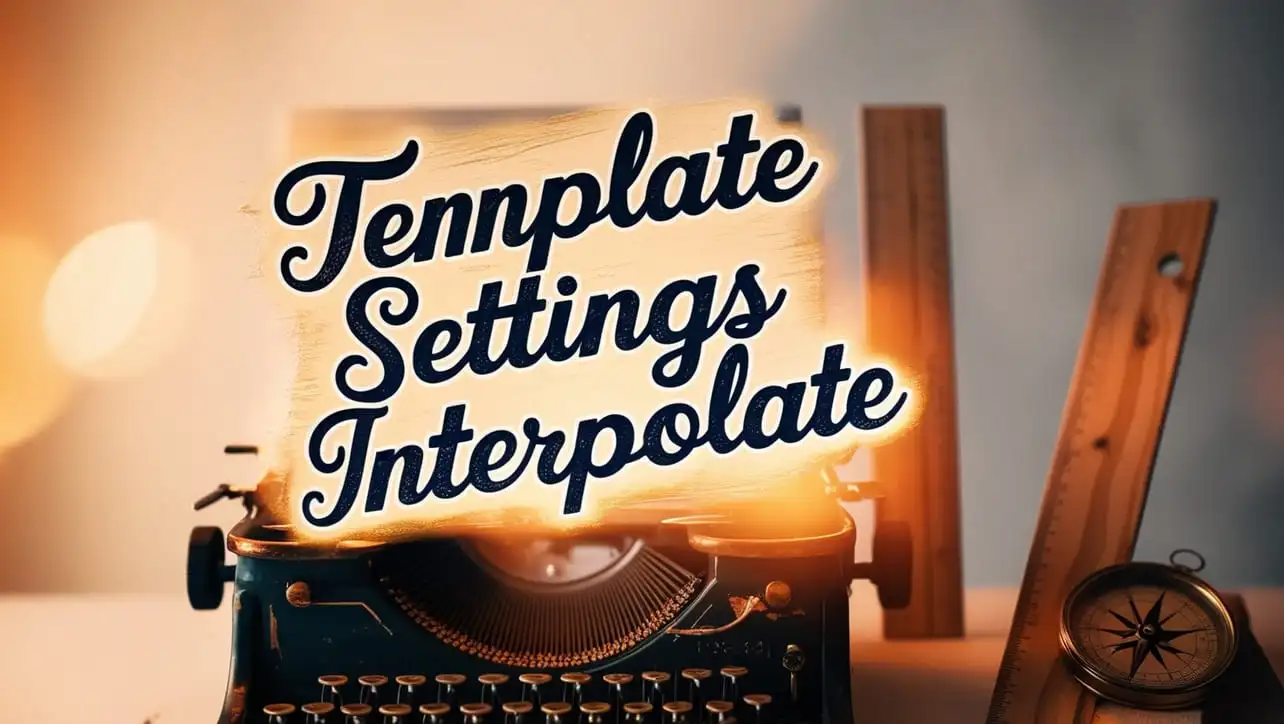
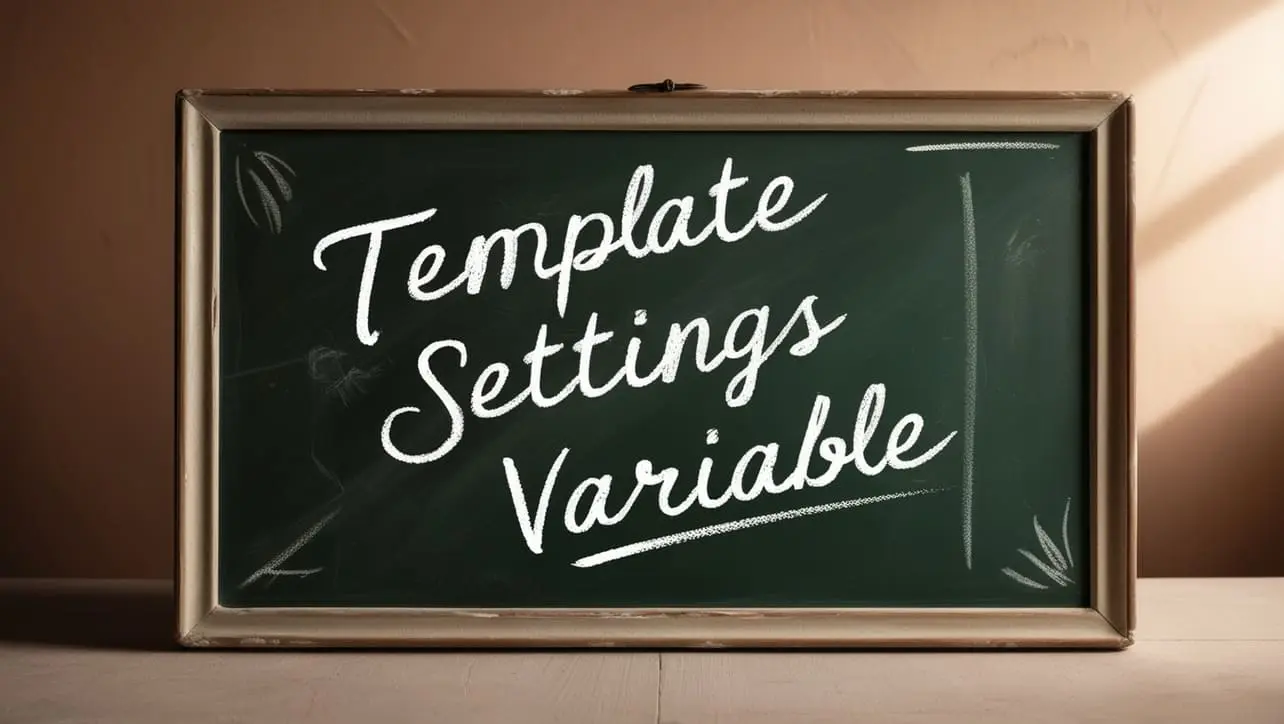
If you have any doubts regarding this article (Lodash _.nth() Array Method), please comment here. I will help you immediately.