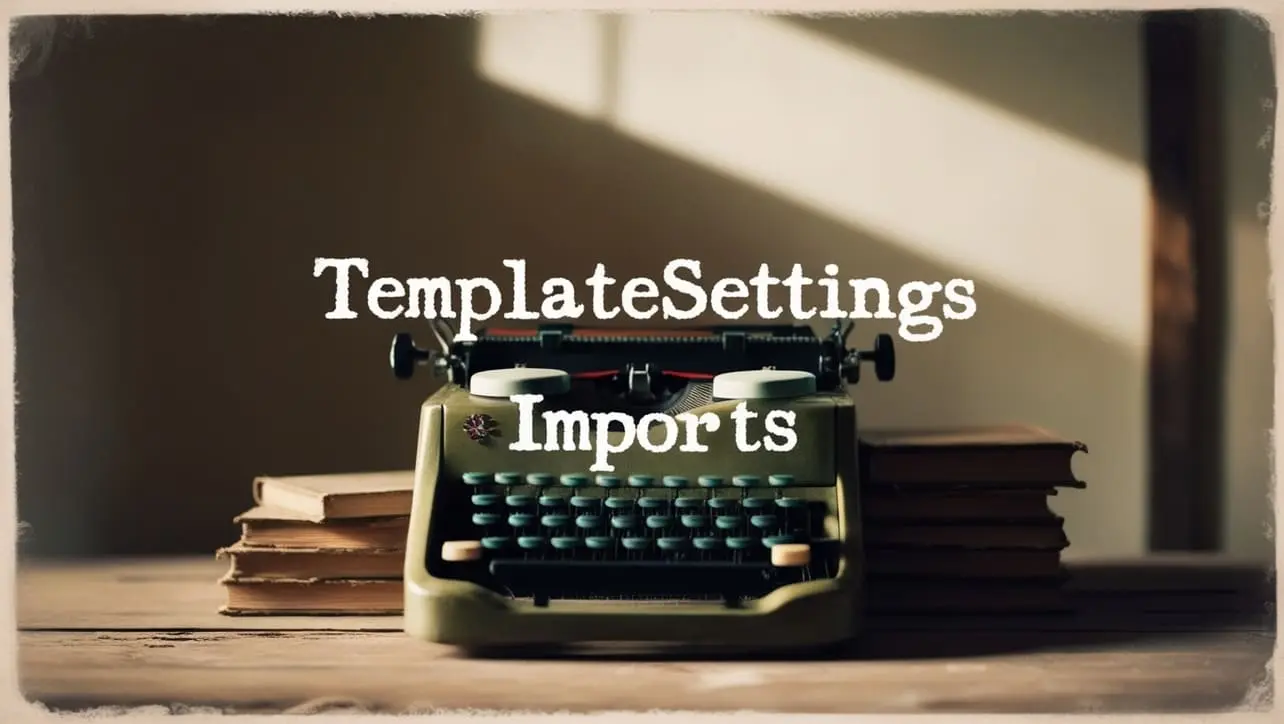
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.fromPairs() Array Method
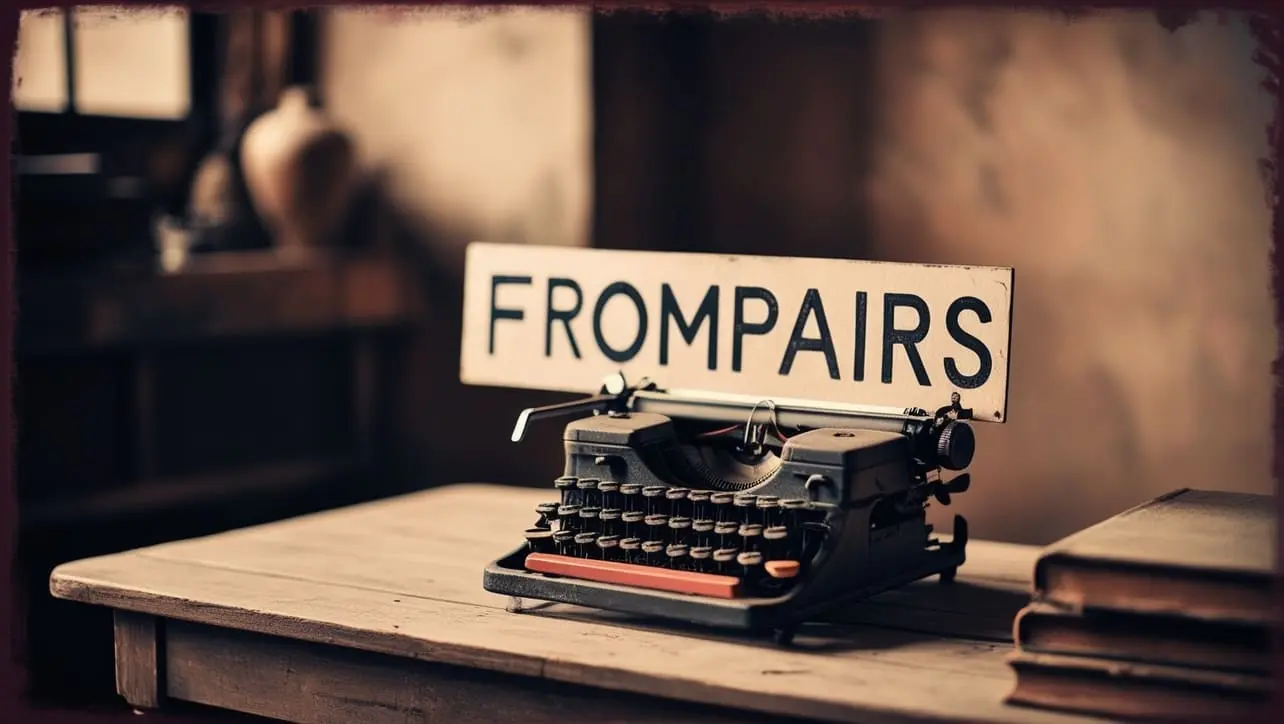
Photo Credit to CodeToFun
🙋 Introduction
When it comes to transforming data structures in JavaScript, Lodash provides an arsenal of utility functions.
The _.fromPairs()
method is a powerful tool that simplifies the process of converting an array of key-value pairs into an object. This function is invaluable for tasks like data manipulation and configuration settings.
🧠 Understanding _.fromPairs()
The _.fromPairs()
method in Lodash takes an array of key-value pairs and creates an object from them. This is particularly useful when dealing with data received in a flattened format, like from a form or an API response.
💡 Syntax
_.fromPairs(pairs)
- pairs: The array of key-value pairs to convert into an object.
📝 Example
Let's delve into a practical example to illustrate the application of _.fromPairs()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const keyValuePairs = [['a', 1], ['b', 2], ['c', 3]];
const resultObject = _.fromPairs(keyValuePairs);
console.log(resultObject);
// Output: { a: 1, b: 2, c: 3 }
In this example, the keyValuePairs array is transformed into an object using _.fromPairs()
.
🏆 Best Practices
Validating Input:
Before using
_.fromPairs()
, ensure that the input array contains valid key-value pairs. Validate the structure and types to avoid unexpected results.validating-input.jsCopiedconst invalidPairs = [['a', 1], ['b']]; if (!invalidPairs.every(pair => Array.isArray(pair) && pair.length === 2)) { console.error('Invalid key-value pairs'); return; } const validatedObject = _.fromPairs(invalidPairs); console.log(validatedObject); // Output: { a: 1 }
Handle Duplicates:
Be cautious of potential duplicate keys in the input array. In JavaScript objects, keys must be unique, and using
_.fromPairs()
with duplicate keys may lead to unexpected behavior.handle-duplicates.jsCopiedconst duplicateKeys = [['name', 'John'], ['age', 30], ['name', 'Doe']]; // Handle duplicates (choose an appropriate strategy) const resultObjectWithDuplicates = _.fromPairs(duplicateKeys); console.log(resultObjectWithDuplicates);
Utilize _.toPairs() for Reversal:
To reverse the process and convert an object into an array of key-value pairs, you can use _.toPairs().
reversal.jsCopiedconst reversedPairs = _.toPairs(resultObject); console.log(reversedPairs);
📚 Use Cases
Data Transformation:
When dealing with data in the form of arrays, such as data obtained from API responses or form submissions,
_.fromPairs()
can be a handy tool for transforming it into a more structured object.data-transformation.jsCopiedconst formDataArray = [['username', 'john_doe'], ['email', 'john@example.com'], ['age', 25]]; const formDataObject = _.fromPairs(formDataArray); console.log(formDataObject);
Object Construction:
In scenarios where dynamic object creation is required, such as building configuration objects or options,
_.fromPairs()
offers a concise way to create objects from arrays.object-construction.jsCopiedconst configurationArray = [['theme', 'dark'], ['fontSize', 16], ['showLabels', true]]; const configurationObject = _.fromPairs(configurationArray); console.log(configurationObject);
🎉 Conclusion
The _.fromPairs()
method in Lodash simplifies the process of converting arrays of key-value pairs into JavaScript objects. Its ease of use and versatility make it a valuable addition to the toolkit of any JavaScript developer working with data transformations. Incorporate _.fromPairs()
into your projects to streamline the conversion of array data into structured objects.
Explore the world of Lodash and unlock the potential of array-to-object transformations with _.fromPairs()
!
👨💻 Join our Community:
Author
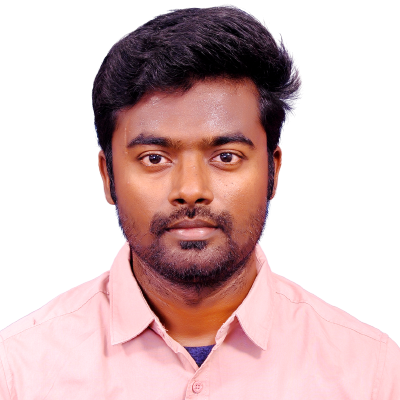
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
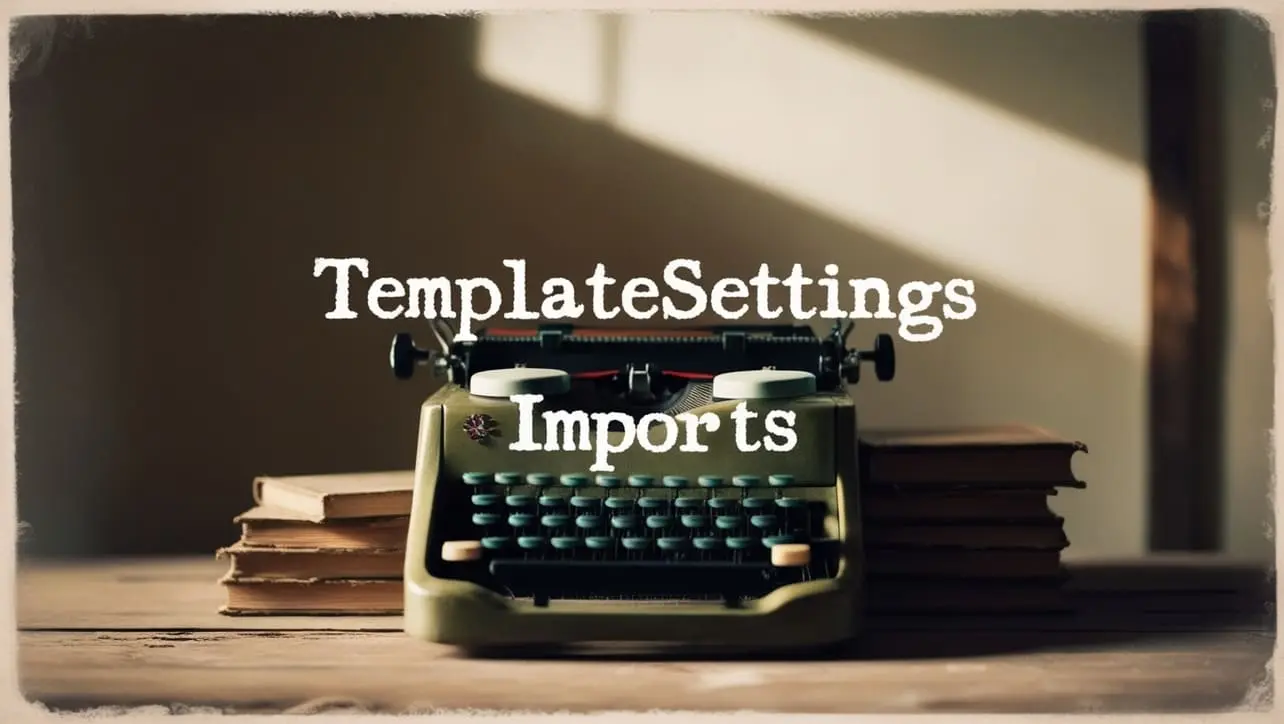
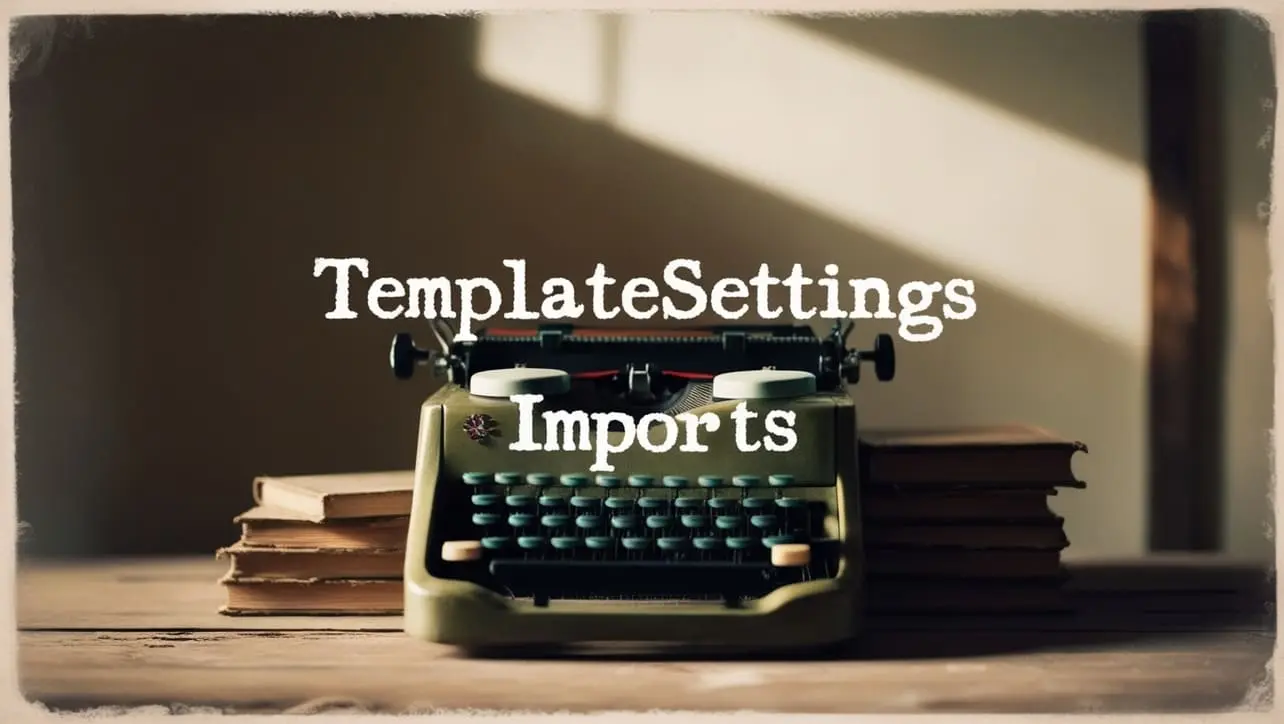
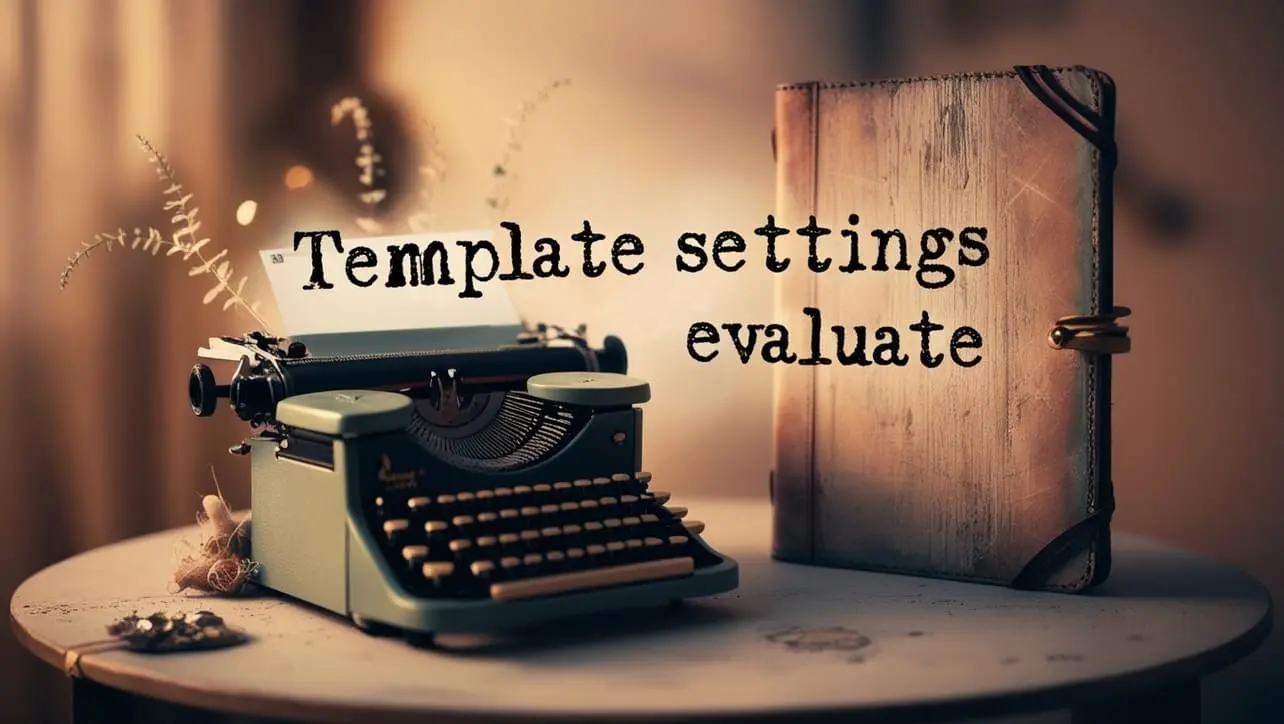
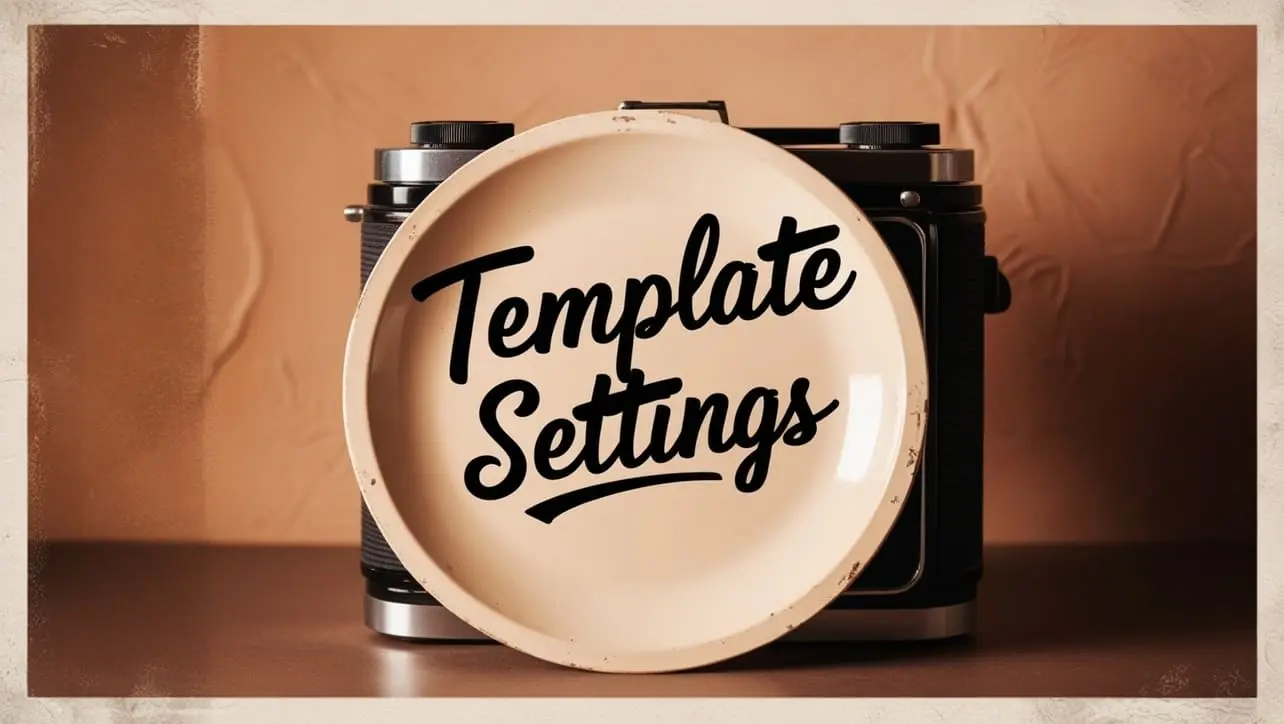
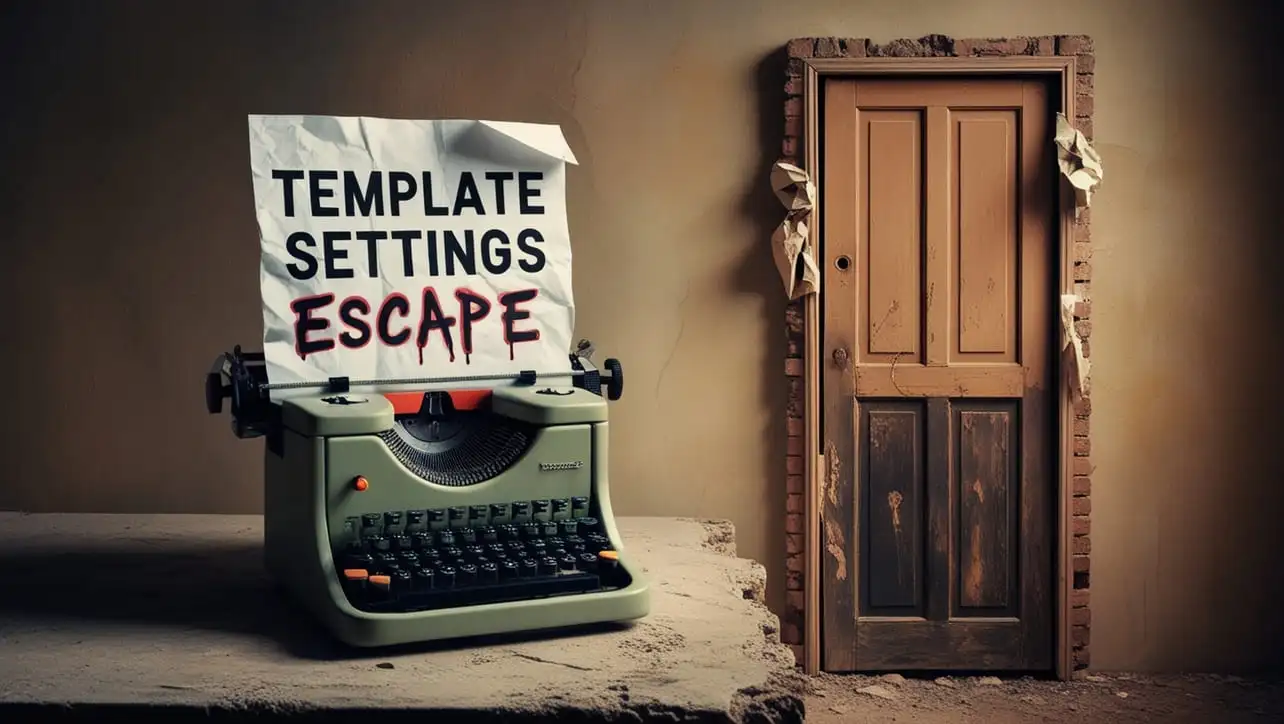
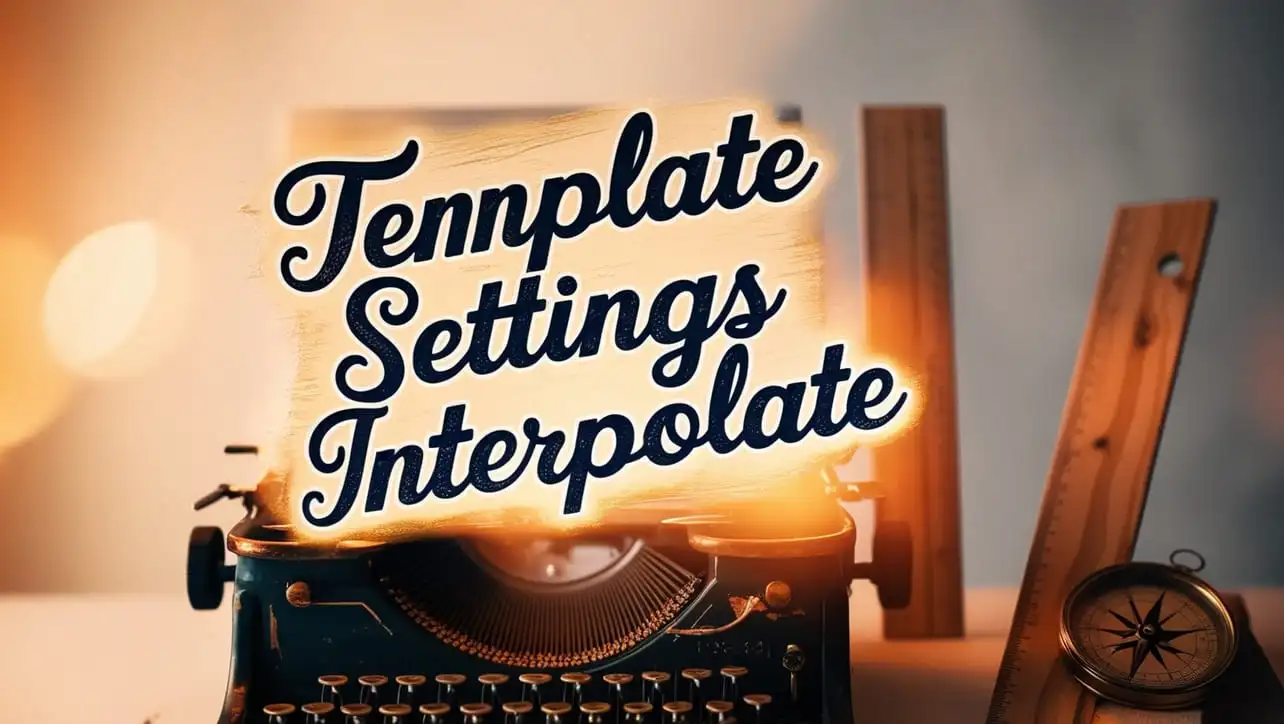
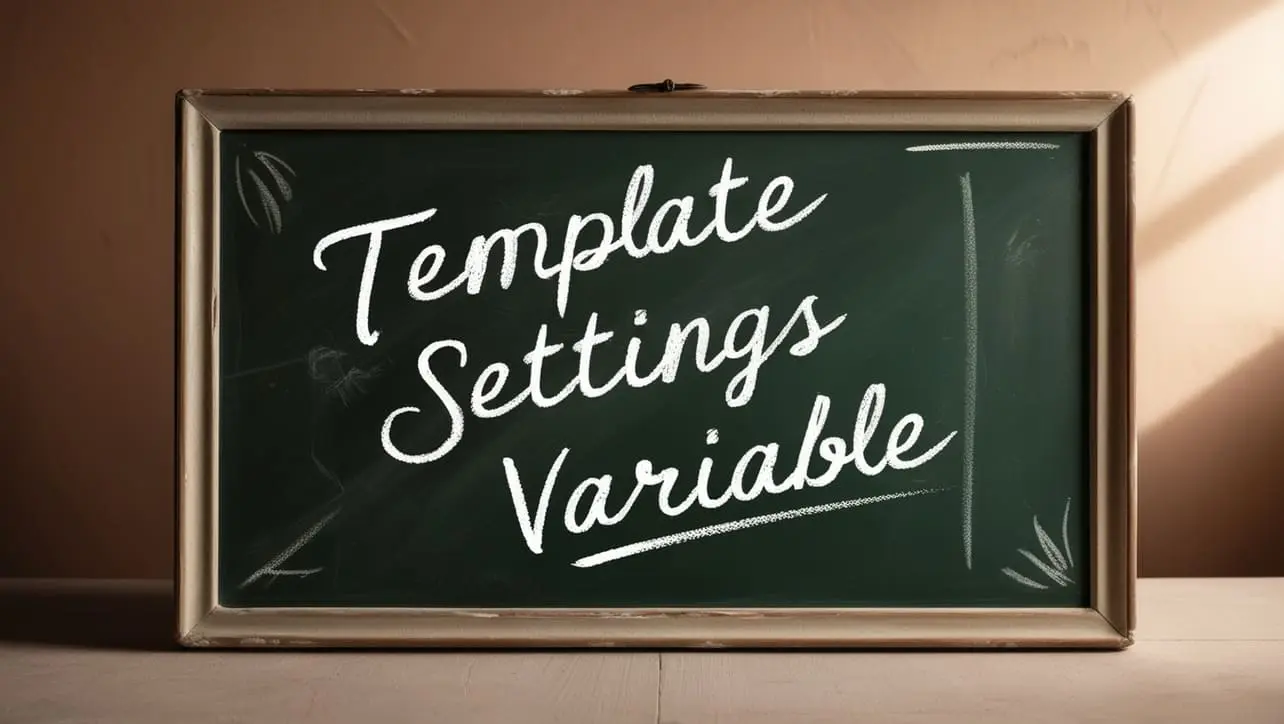
If you have any doubts regarding this article (Lodash _.fromPairs() Array Method), please comment here. I will help you immediately.