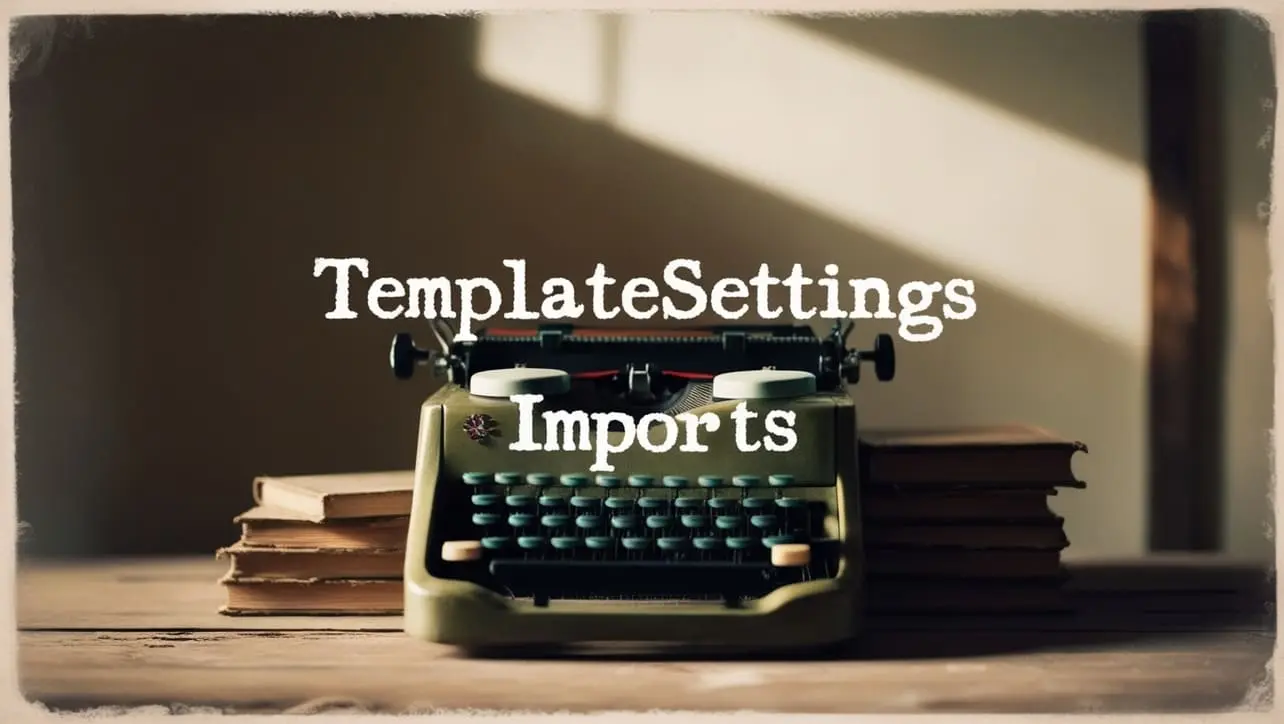
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.flattenDepth() Array Method
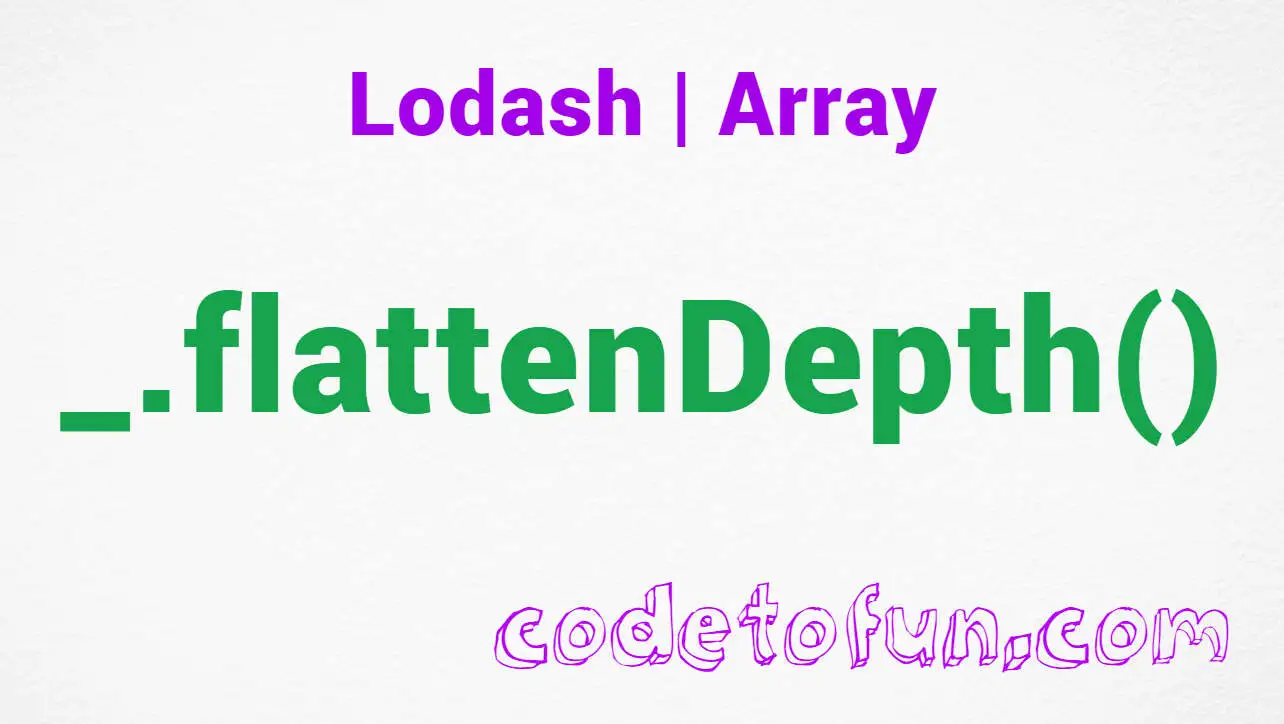
Photo Credit to CodeToFun
🙋 Introduction
Working with nested arrays in JavaScript can sometimes be challenging, especially when you need a flattened representation.
The Lodash library comes to the rescue with its powerful utility functions, and one such tool is the _.flattenDepth()
method. This method allows you to flatten an array to a specified depth, making array manipulation more convenient and efficient.
🧠 Understanding _.flattenDepth()
The _.flattenDepth()
method in Lodash is designed to flatten an array up to a specified depth. This is particularly useful when you have nested arrays, and you want to simplify the structure while retaining control over the depth of flattening.
💡 Syntax
_.flattenDepth(array, [depth=1])
- array: The array to flatten.
- depth: The depth to which the array should be flattened (default is 1).
📝 Example
Let's dive into a practical example to illustrate the functionality of _.flattenDepth()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const nestedArray = [1, [2, [3, [4]], 5]];
const flattenedArray = _.flattenDepth(nestedArray, 2);
console.log(flattenedArray);
// Output: [1, 2, 3, [4], 5]
In this example, the nestedArray is flattened to a depth of 2, resulting in a simplified array structure.
🏆 Best Practices
Understand Your Data Structure:
Before using
_.flattenDepth()
, have a clear understanding of your data structure. Knowing the depth of nesting will help you choose an appropriate value for the depth parameter.data-structure.jsCopiedconst deeplyNestedArray = [1, [2, [3, [4, [5]]]]]; const flattenedDeepArray = _.flattenDepth(deeplyNestedArray, 3); console.log(flattenedDeepArray);
Choose the Right Depth:
Experiment with different depth values to achieve the desired flattened structure. Choosing the right depth is crucial for obtaining the desired result.
Choose-the-right-depth.jsCopiedconst customDepthArray = [1, [2, [3, [4, [5]]]]]; const customDepth = 2; const customDepthResult = _.flattenDepth(customDepthArray, customDepth); console.log(customDepthResult);
📚 Use Cases
Simplifying Nested Structures:
When dealing with complex nested structures,
_.flattenDepth()
can simplify the array, making it easier to work with.simplifying-nested-structures.jsCopiedconst complexNestedArray = [1, [2, [3, [4, [5]]]]]; const simplifiedArray = _.flattenDepth(complexNestedArray, 2); console.log(simplifiedArray);
Handling Variable Depth:
In scenarios where the depth of nesting varies, using
_.flattenDepth()
allows you to control the flattening process and handle different data structures.handling-variable-depth.jsCopiedconst variableDepthArray = [1, [2, [3, [4, [5]]]]]; const variableDepth = 3; const variableDepthResult = _.flattenDepth(variableDepthArray, variableDepth); console.log(variableDepthResult);
🎉 Conclusion
The _.flattenDepth()
method in Lodash is a valuable asset for any JavaScript developer dealing with nested arrays. Whether you need to simplify complex structures or handle variable nesting depths, this method provides a flexible and efficient solution.
Incorporate _.flattenDepth()
into your array manipulation toolkit and experience the power of simplified and controlled flattening. Explore the capabilities of Lodash and streamline your JavaScript development!
👨💻 Join our Community:
Author
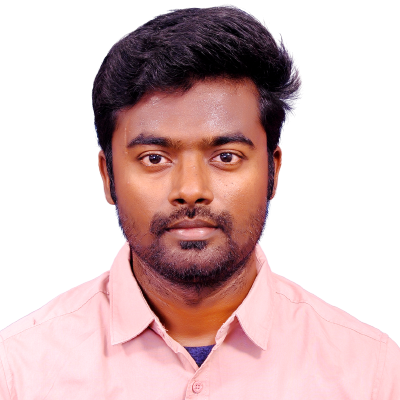
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
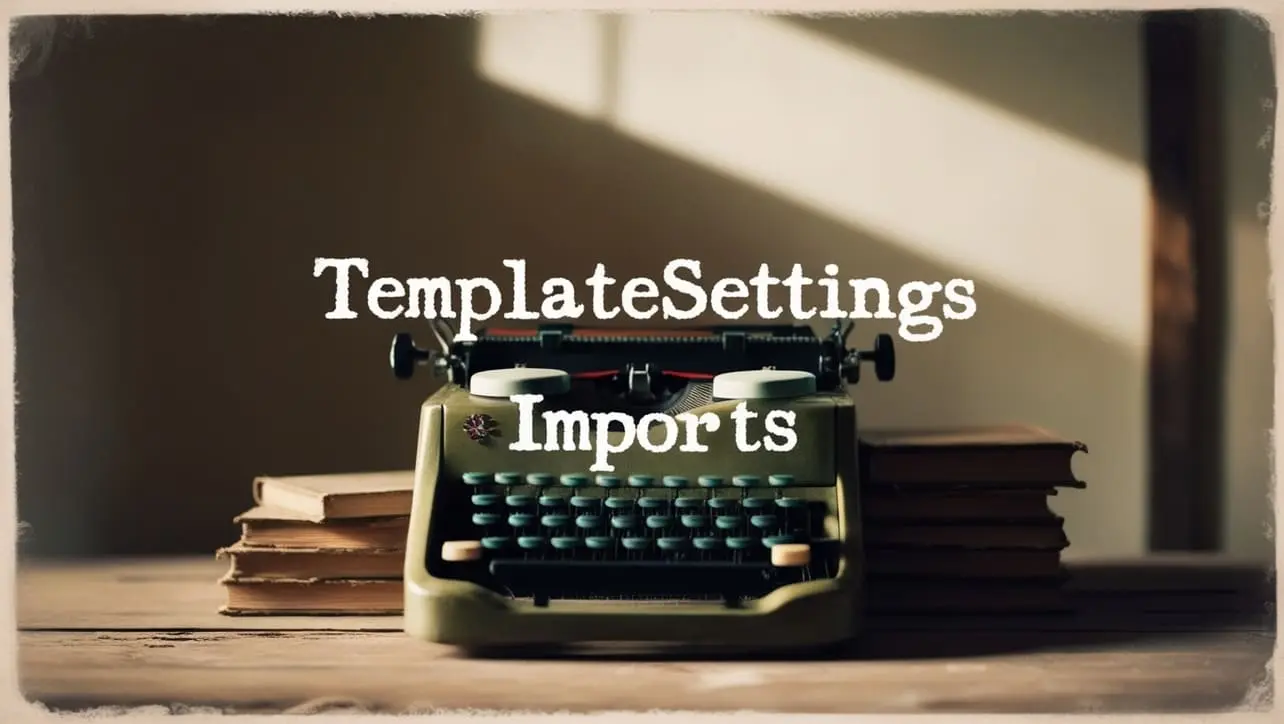
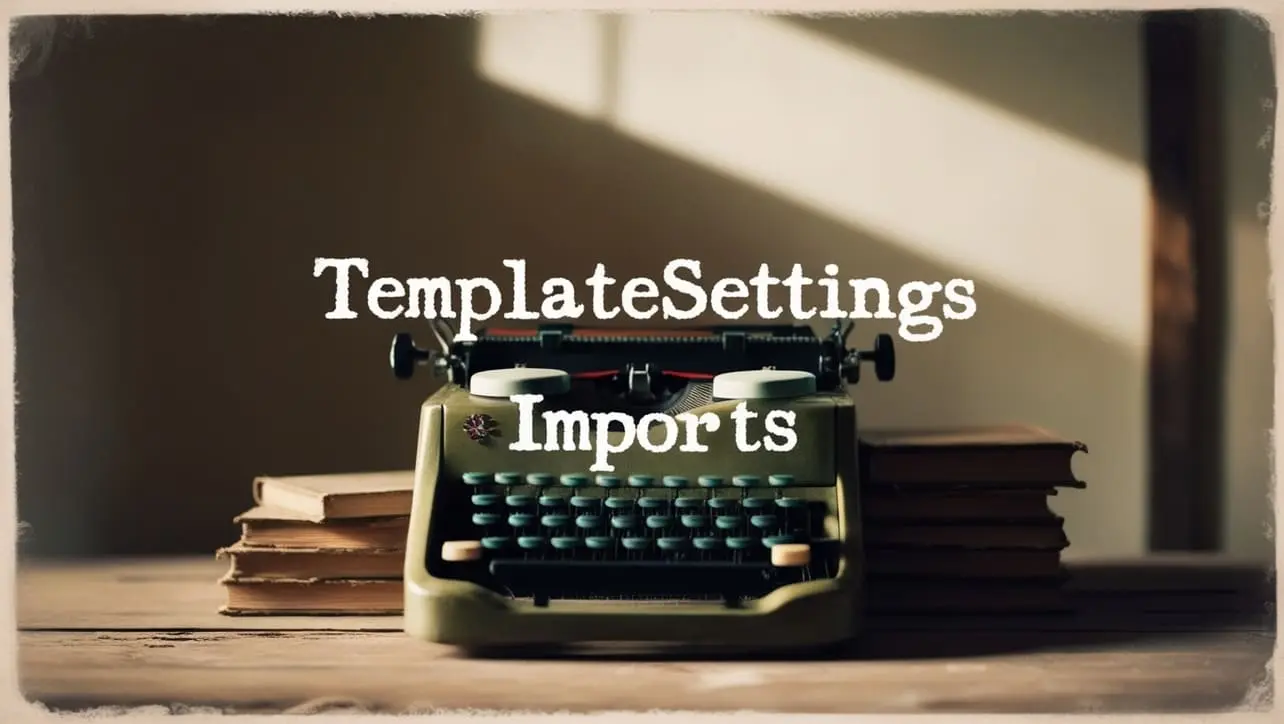
Lodash _.templateSettings.imports Property
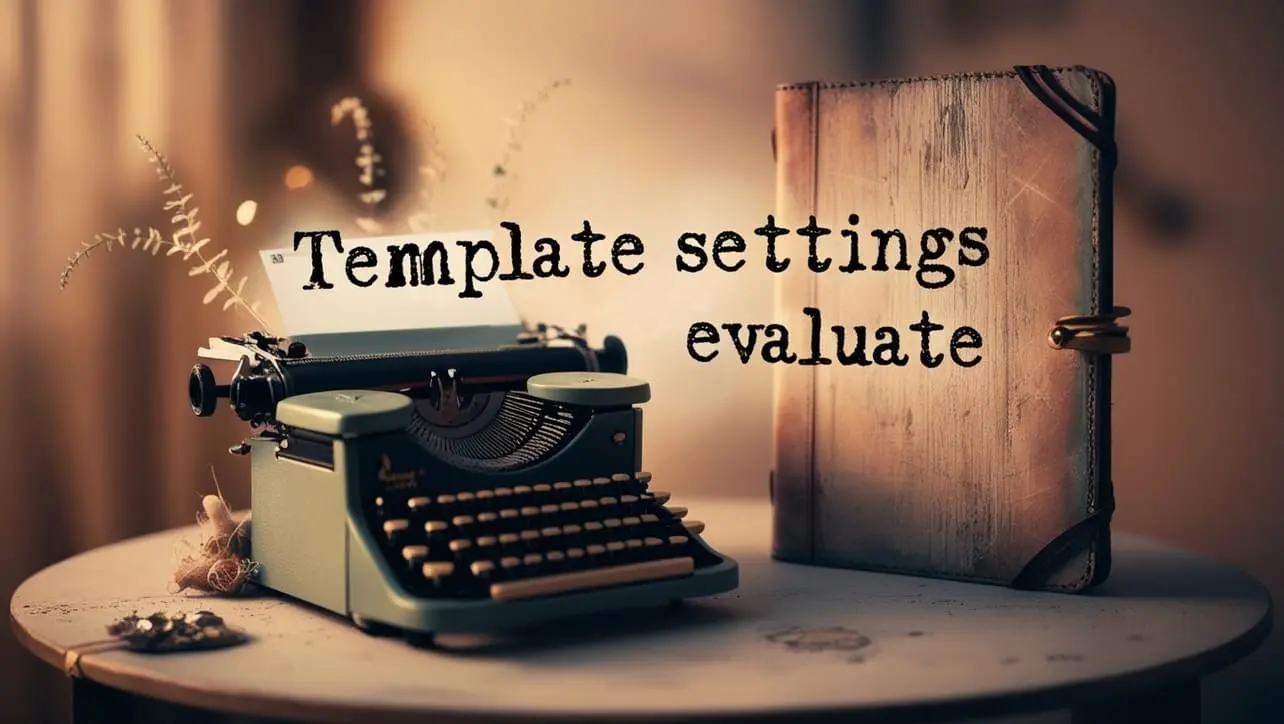
Lodash _.templateSettings.evaluate Property
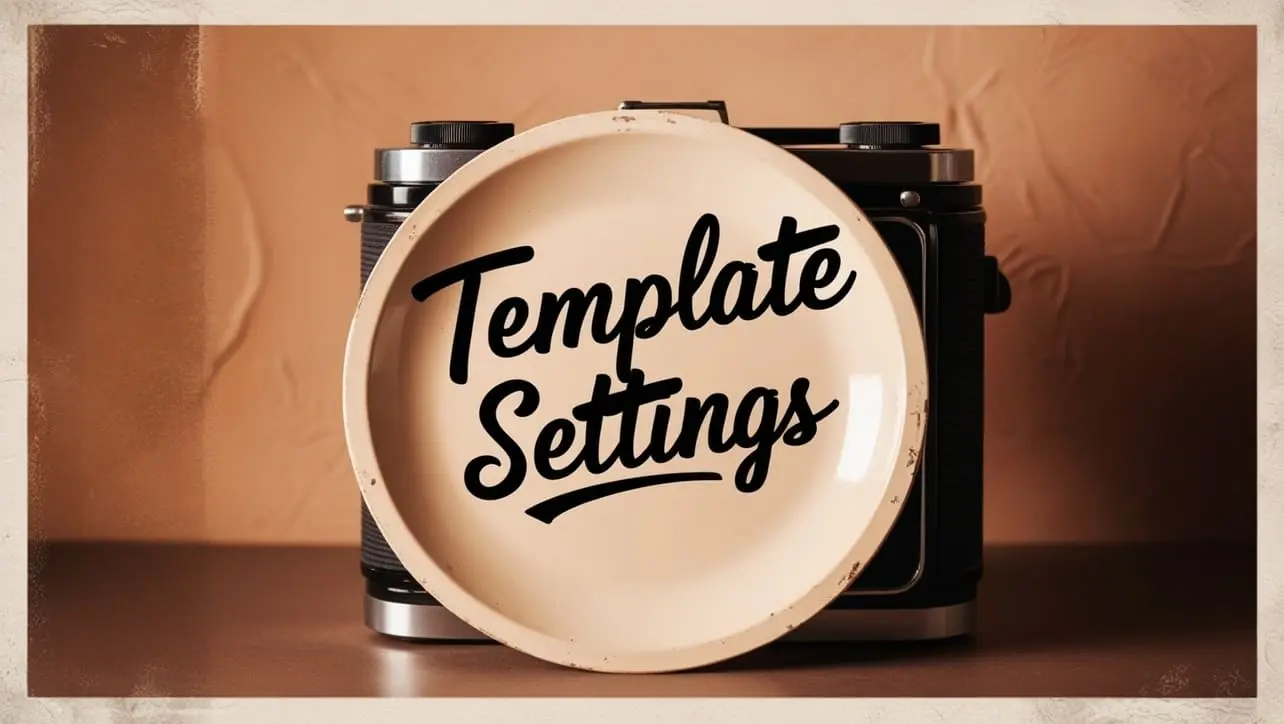
Lodash _.templateSettings Property
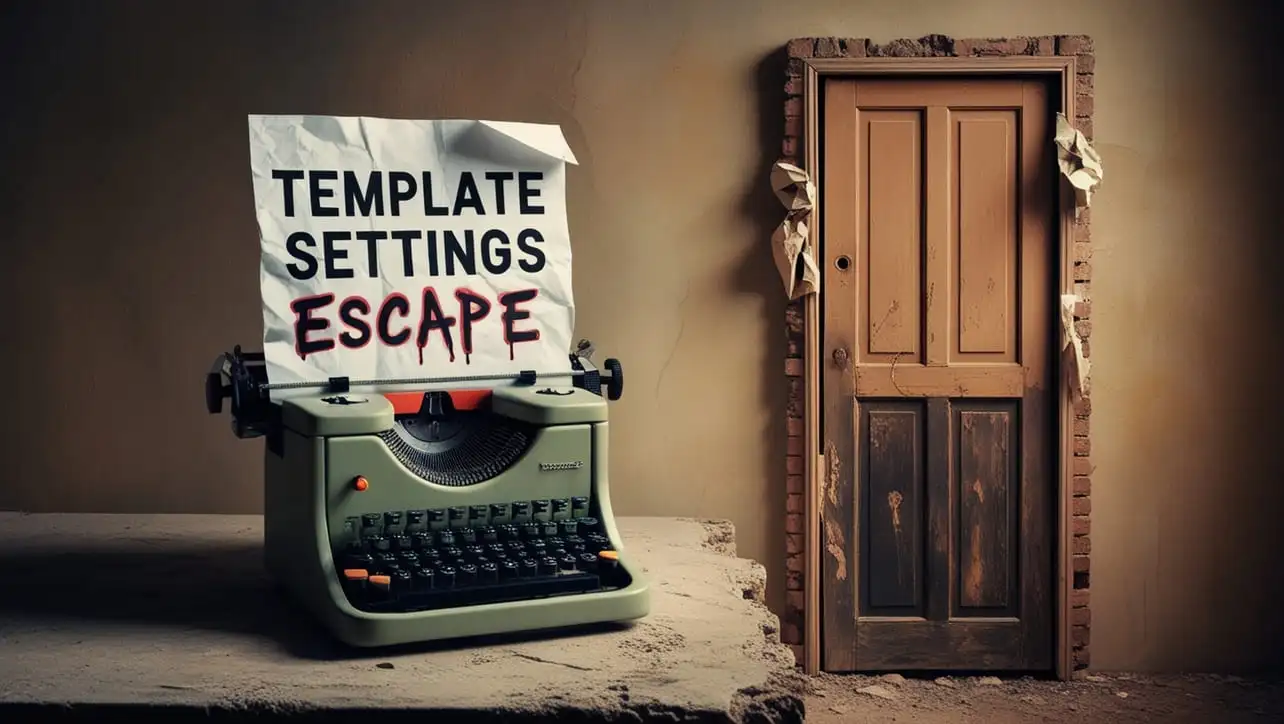
Lodash _.templateSettings.escape Property
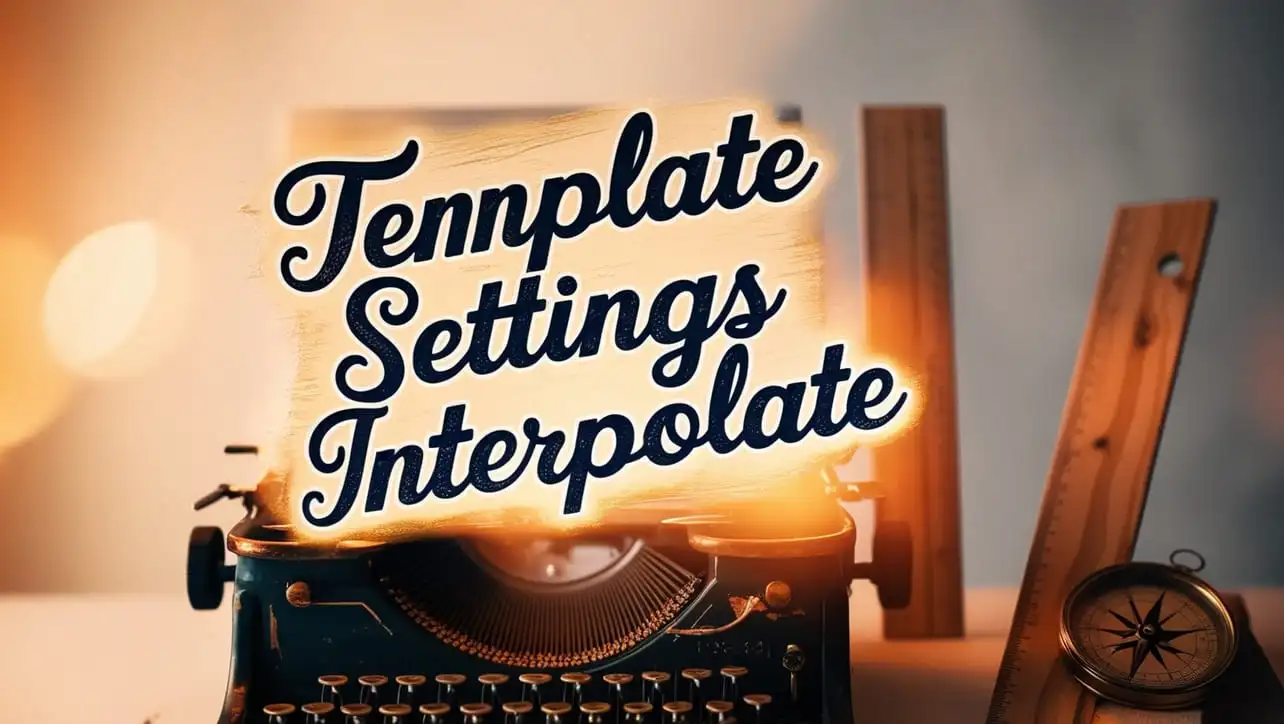
Lodash _.templateSettings.interpolate Property
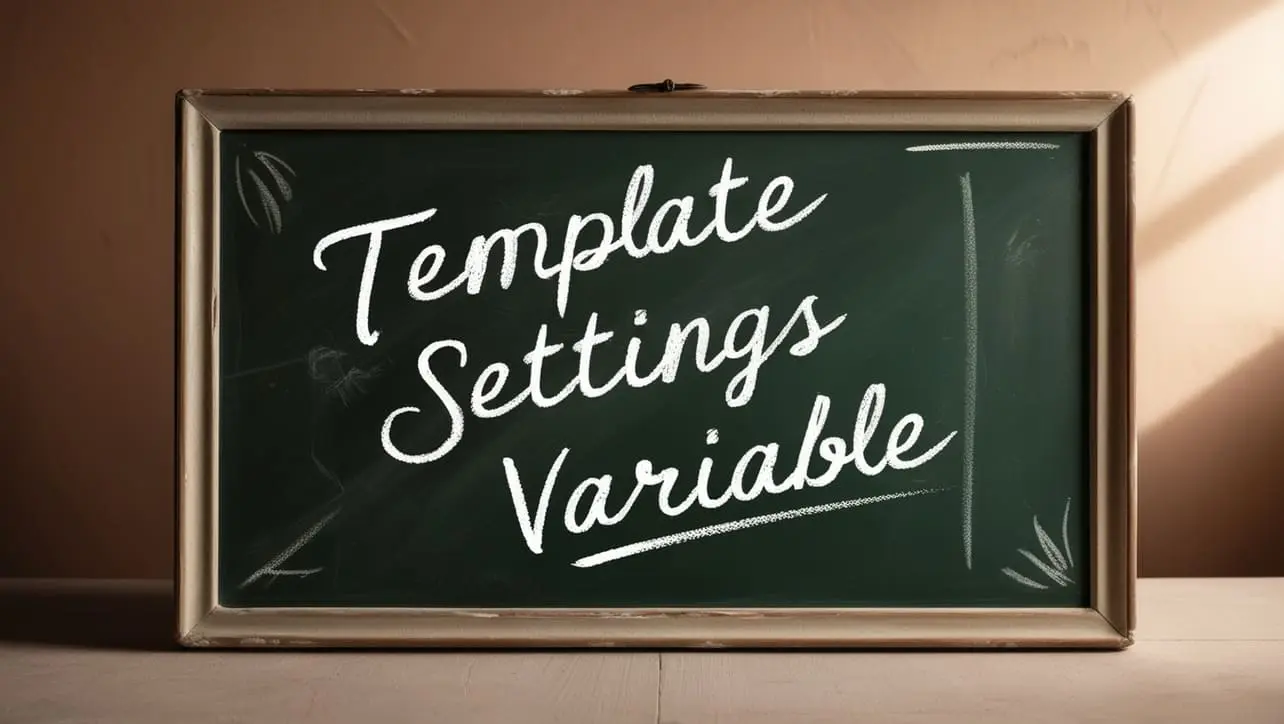
If you have any doubts regarding this article (Lodash _.flattenDepth() Array Method), please comment here. I will help you immediately.