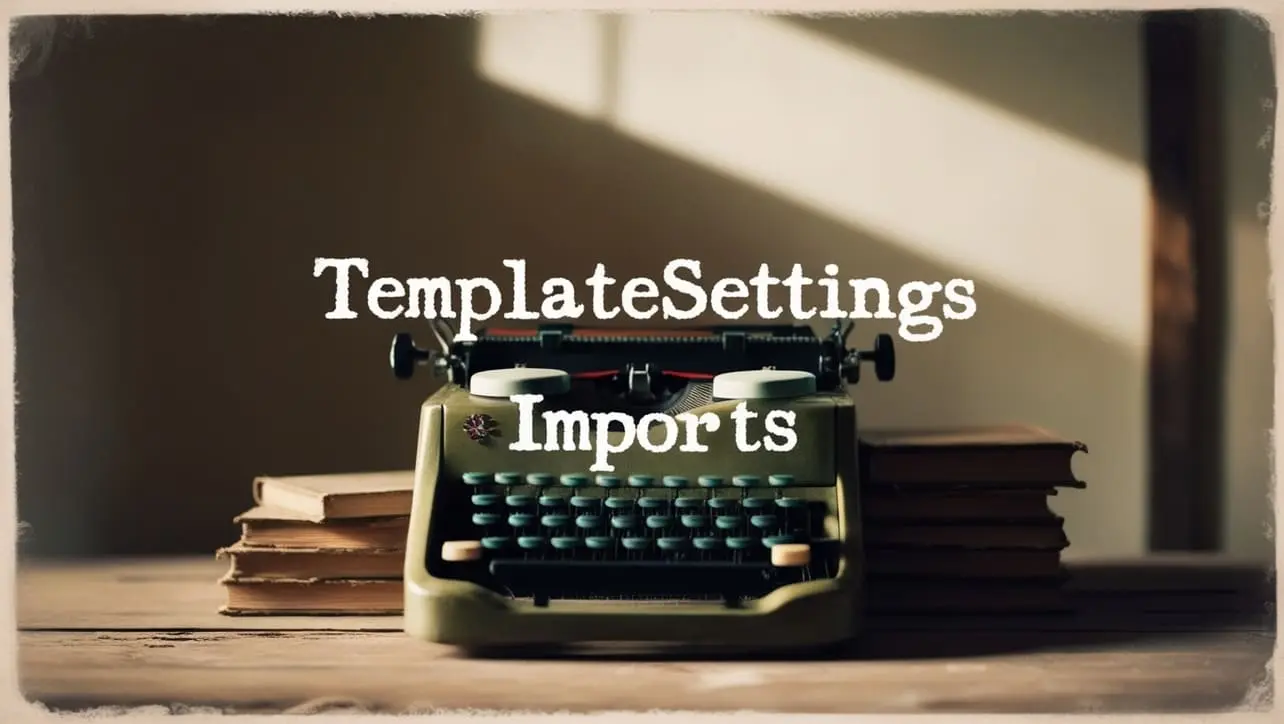
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.flatten() Array Method
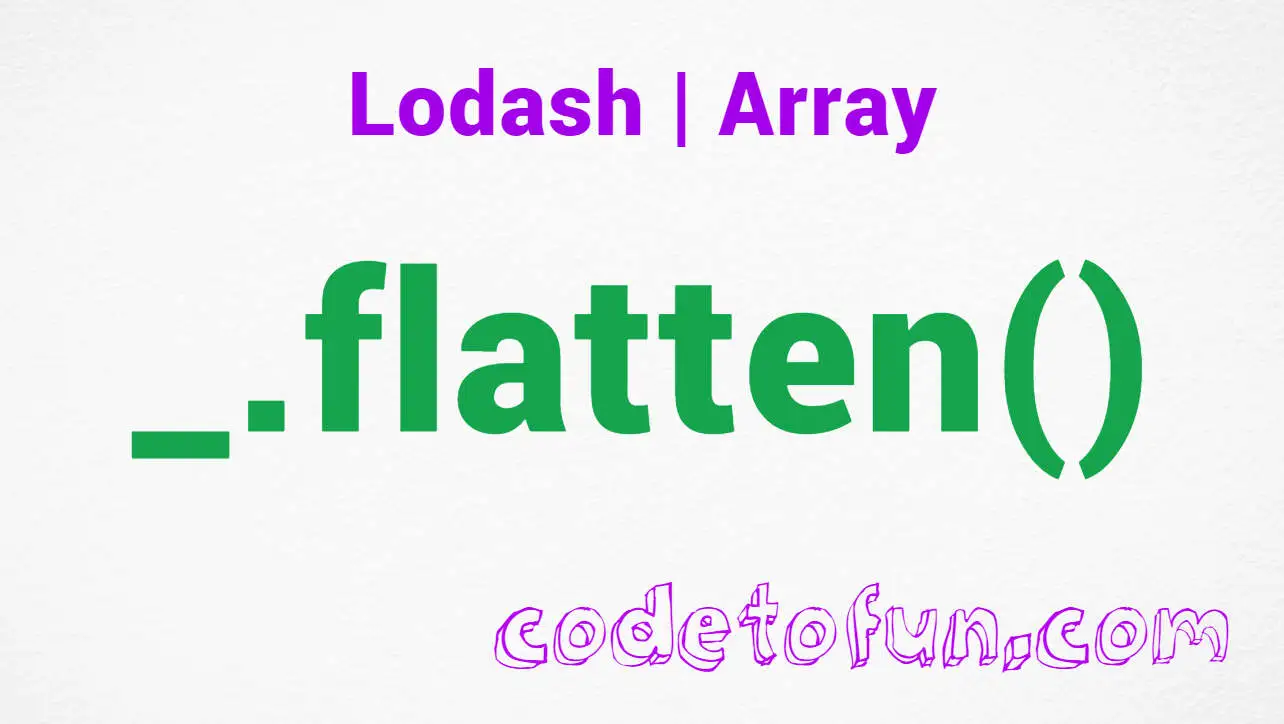
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, dealing with nested arrays is a common challenge. Enter Lodash, a powerful utility library that provides a plethora of functions to simplify array manipulation. Among these functions is the _.flatten()
method, a versatile tool for flattening nested arrays and simplifying data structures.
This method proves invaluable for developers working with complex data that needs to be streamlined for processing or presentation.
🧠 Understanding _.flatten()
The _.flatten()
method in Lodash is designed to flatten nested arrays, reducing multi-dimensional arrays into a single-dimensional array. This can be immensely useful when dealing with arrays containing various levels of nesting, making data more accessible and manageable.
💡 Syntax
_.flatten(array)
- array: The array to flatten.
📝 Example
Let's explore a practical example to understand how _.flatten()
works:
const _ = require('lodash');
const nestedArray = [1, [2, [3, [4]], 5]];
const flattenedArray = _.flatten(nestedArray);
console.log(flattenedArray);
// Output: [1, 2, 3, 4, 5]
In this example, the nestedArray is flattened, resulting in a single-dimensional array.
🏆 Best Practices
Consistent Nesting Levels:
For predictable results, ensure that the arrays you are flattening have consistent nesting levels. This ensures that the flattened array is uniform and meets your expectations.
example.jsCopiedconst inconsistentNestedArray = [1, [2, [3, [4, [5]]]]]; const flattenedInconsistentArray = _.flatten(inconsistentNestedArray); console.log(flattenedInconsistentArray); // Output: [1, 2, 3, [4, [5]]]
Flattening Arrays with Different Types:
_.flatten()
works well with arrays containing elements of different types. However, be aware that it will treat non-array elements as flat values, potentially leading to unexpected results.example.jsCopiedconst mixedTypeArray = [1, 'hello', [2, [3, 'world']], true]; const flattenedMixedTypeArray = _.flatten(mixedTypeArray); console.log(flattenedMixedTypeArray); // Output: [1, 'hello', 2, [3, 'world'], true]
Utilizing _.flattenDeep():
If you need to handle deeply nested arrays with varying levels, consider using _.flattenDeep() for a more comprehensive flattening.
example.jsCopiedconst deeplyNestedArray = [1, [2, [3, [4, [5]]]]]; const deeplyFlattenedArray = _.flattenDeep(deeplyNestedArray); console.log(deeplyFlattenedArray); // Output: [1, 2, 3, 4, 5]
📚 Use Cases
Data Transformation:
_.flatten()
is ideal for transforming nested data structures into a format that is easier to process or visualize. This is particularly useful when dealing with JSON-like structures.example.jsCopiedconst nestedData = { id: 1, details: { name: 'John Doe', addresses: ['123 Main St', '456 Oak Ave'] } }; const flattenedData = _.flatten(Object.values(nestedData)); console.log(flattenedData); // Output: [1, 'John Doe', ['123 Main St', '456 Oak Ave']]
Simplifying List of Lists:
When working with a list of lists,
_.flatten()
simplifies the structure, making it easier to work with and iterate over the elements.example.jsCopiedconst listOfLists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]; const flattenedList = _.flatten(listOfLists); console.log(flattenedList); // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
User Interface Rendering:
In user interfaces, nested structures might be prevalent in component hierarchies. Flattening arrays can simplify the rendering logic, making it more straightforward to display elements.
example.jsCopiedconst componentHierarchy = [Header, [Sidebar, Content, Footer]]; const flattenedComponents = _.flatten(componentHierarchy); // Render flattened components flattenedComponents.forEach(component => render(component));
🎉 Conclusion
The _.flatten()
method in Lodash is a valuable asset for JavaScript developers, offering a straightforward solution for flattening nested arrays. Whether you're working with diverse data structures, transforming data, or simplifying complex lists, _.flatten()
provides a concise and efficient way to handle nested arrays.
Explore the capabilities of _.flatten()
and enhance the simplicity and clarity of your array manipulation tasks in JavaScript!
👨💻 Join our Community:
Author
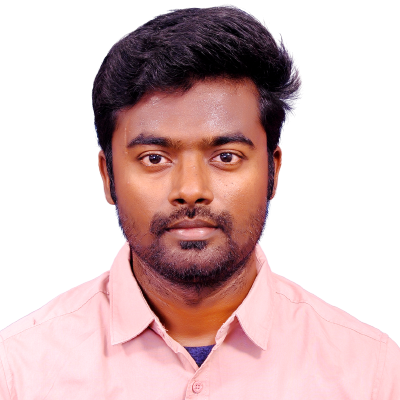
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
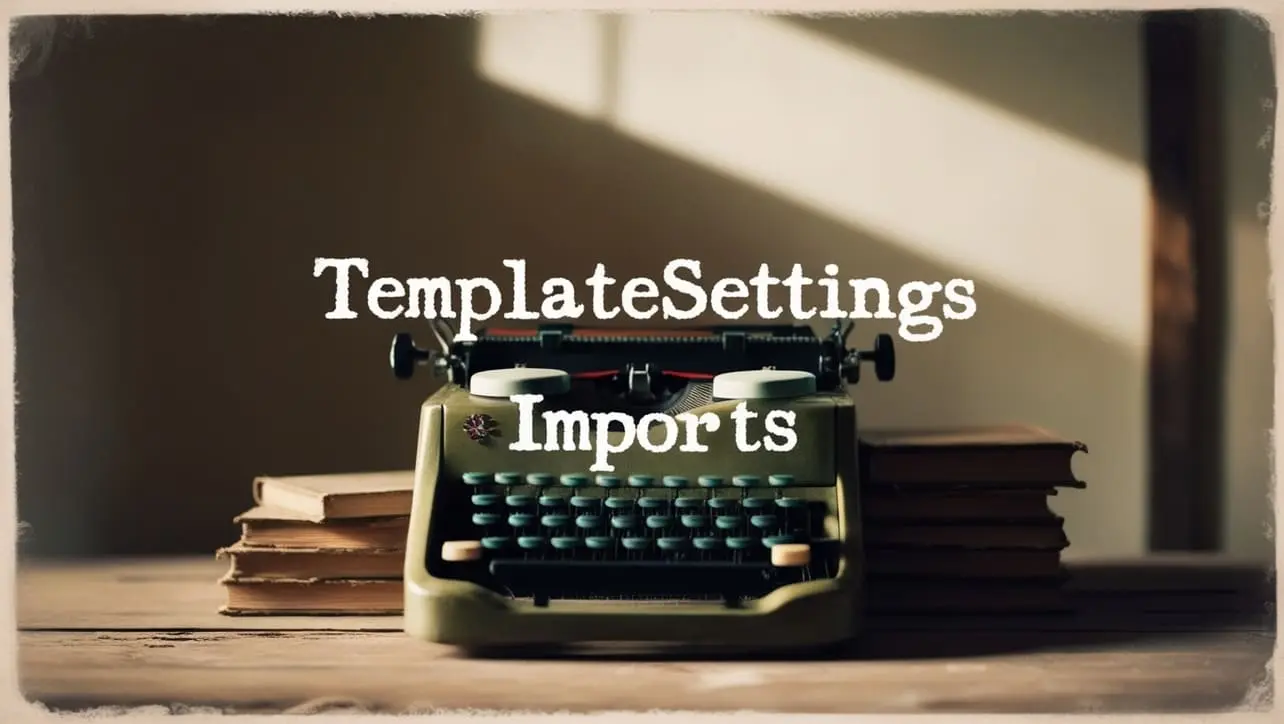
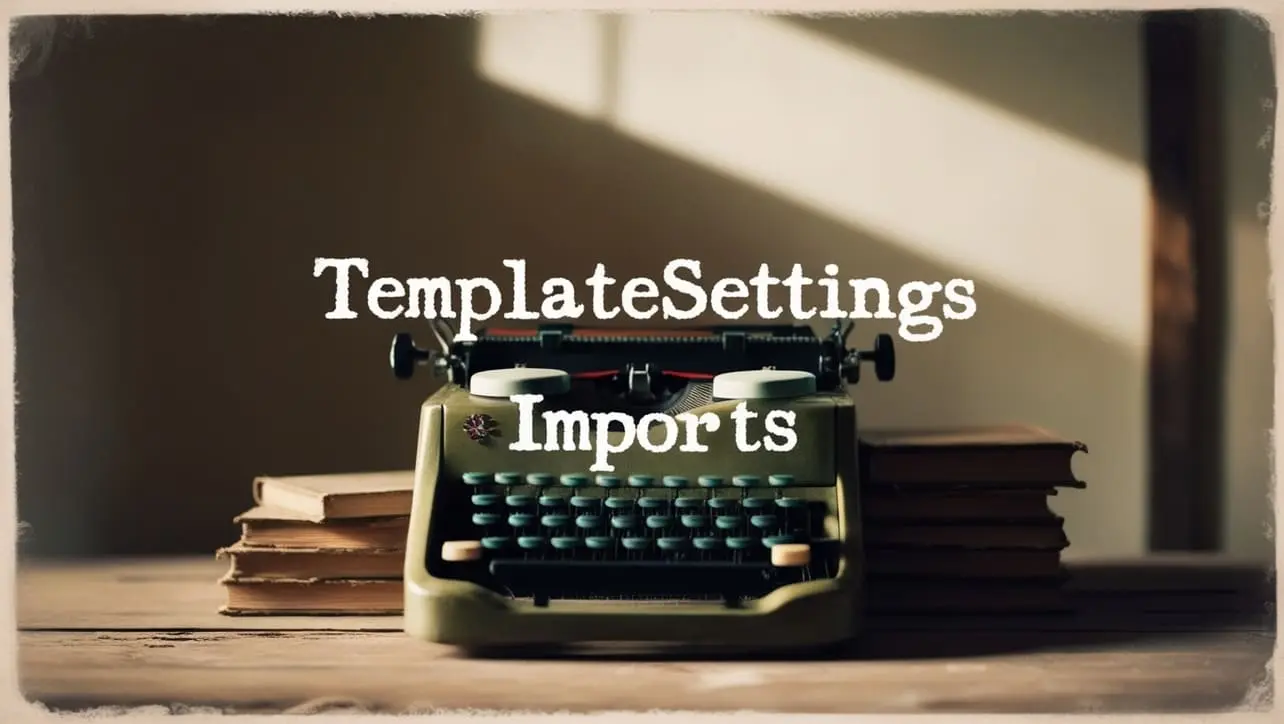
Lodash _.templateSettings.imports Property
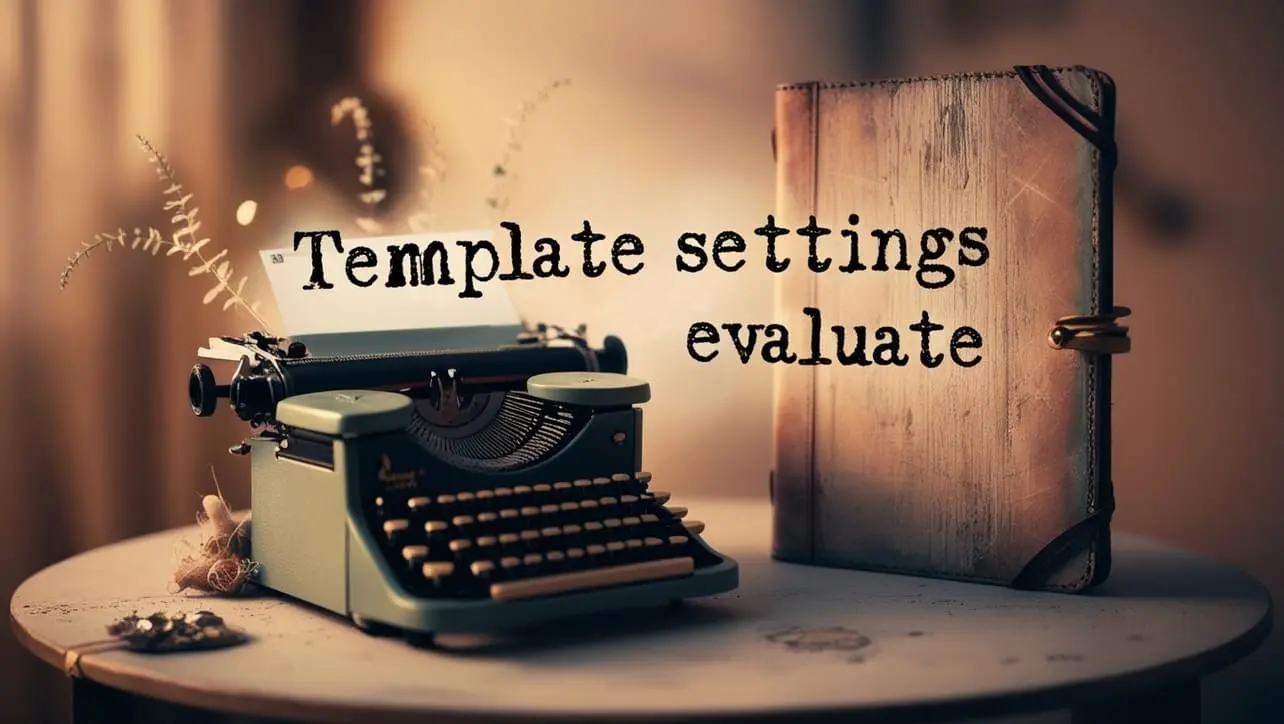
Lodash _.templateSettings.evaluate Property
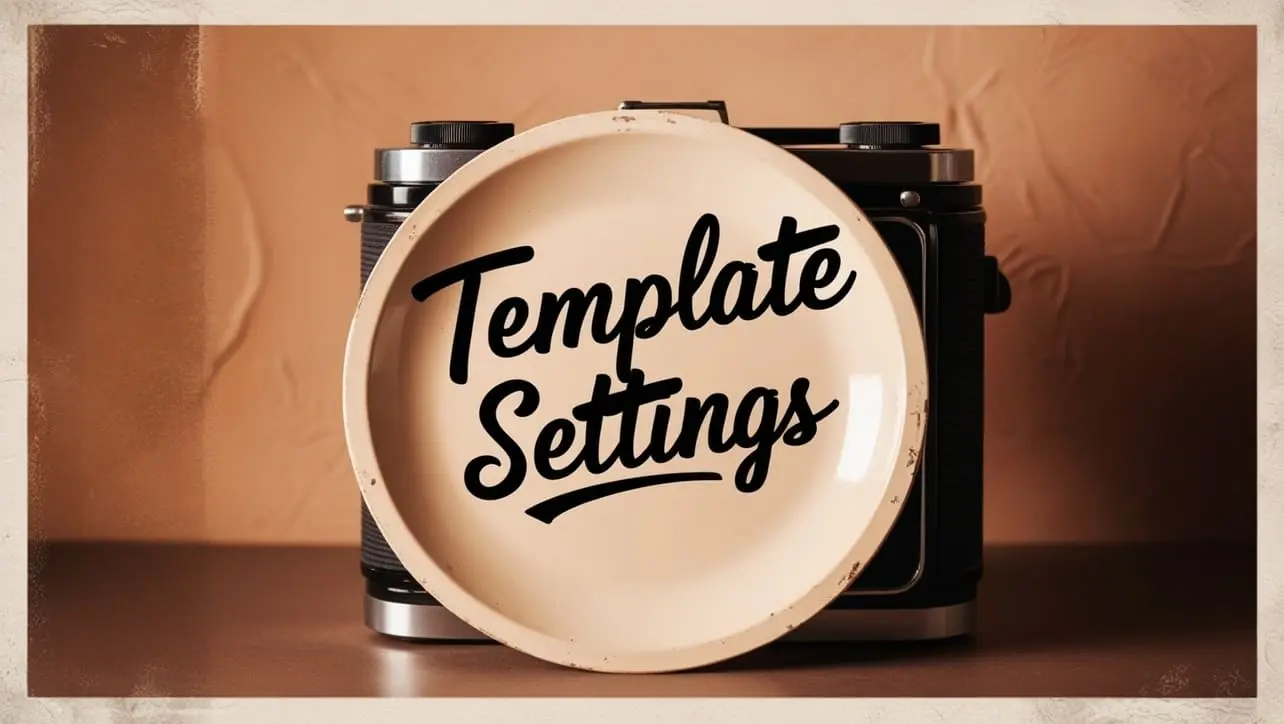
Lodash _.templateSettings Property
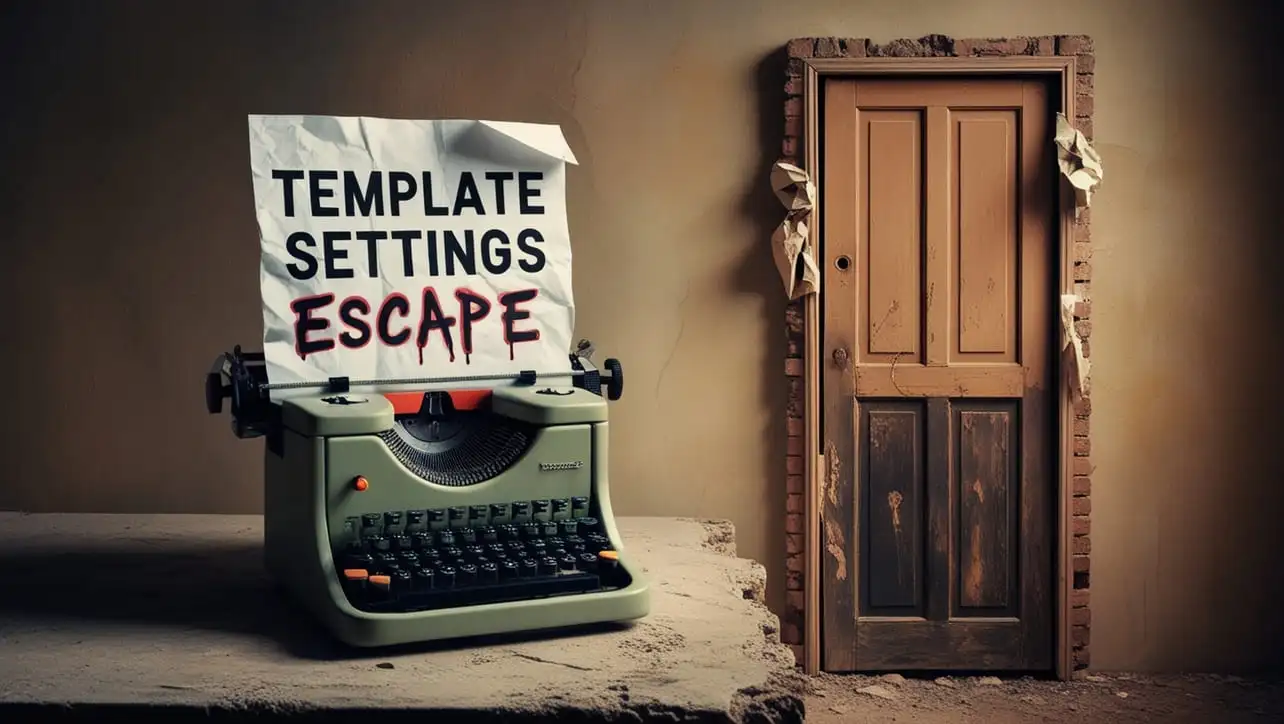
Lodash _.templateSettings.escape Property
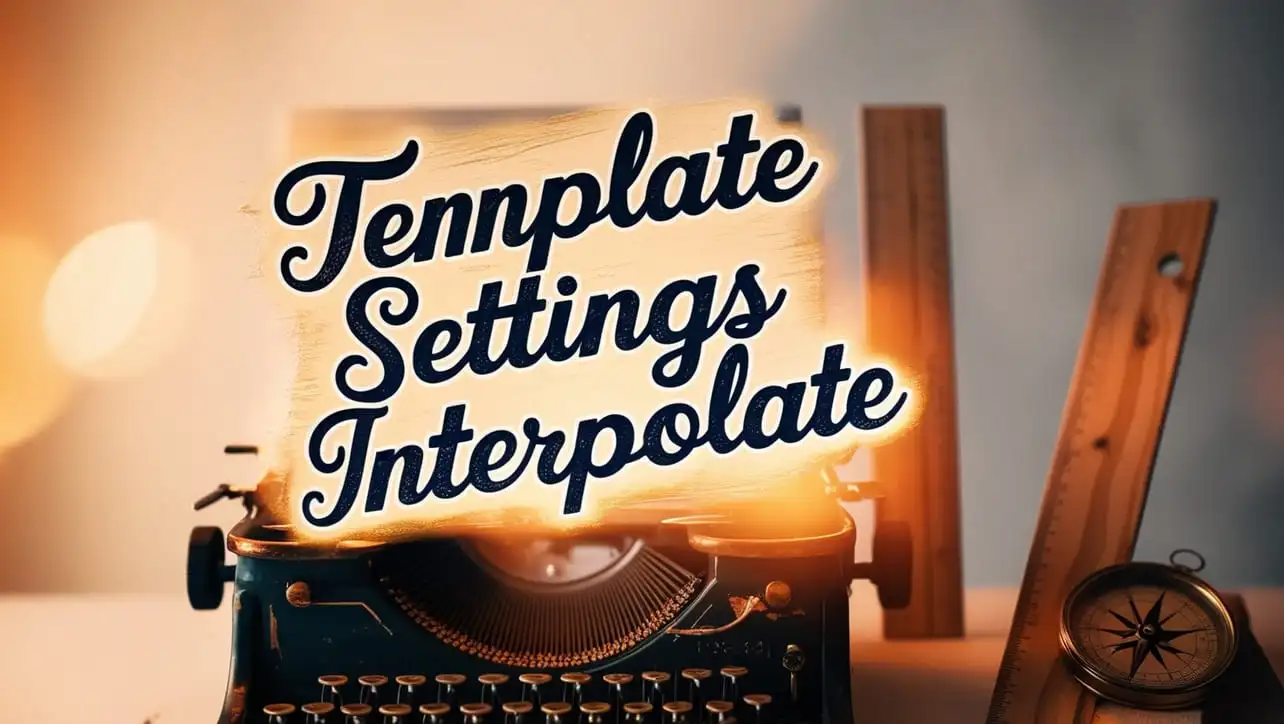
Lodash _.templateSettings.interpolate Property
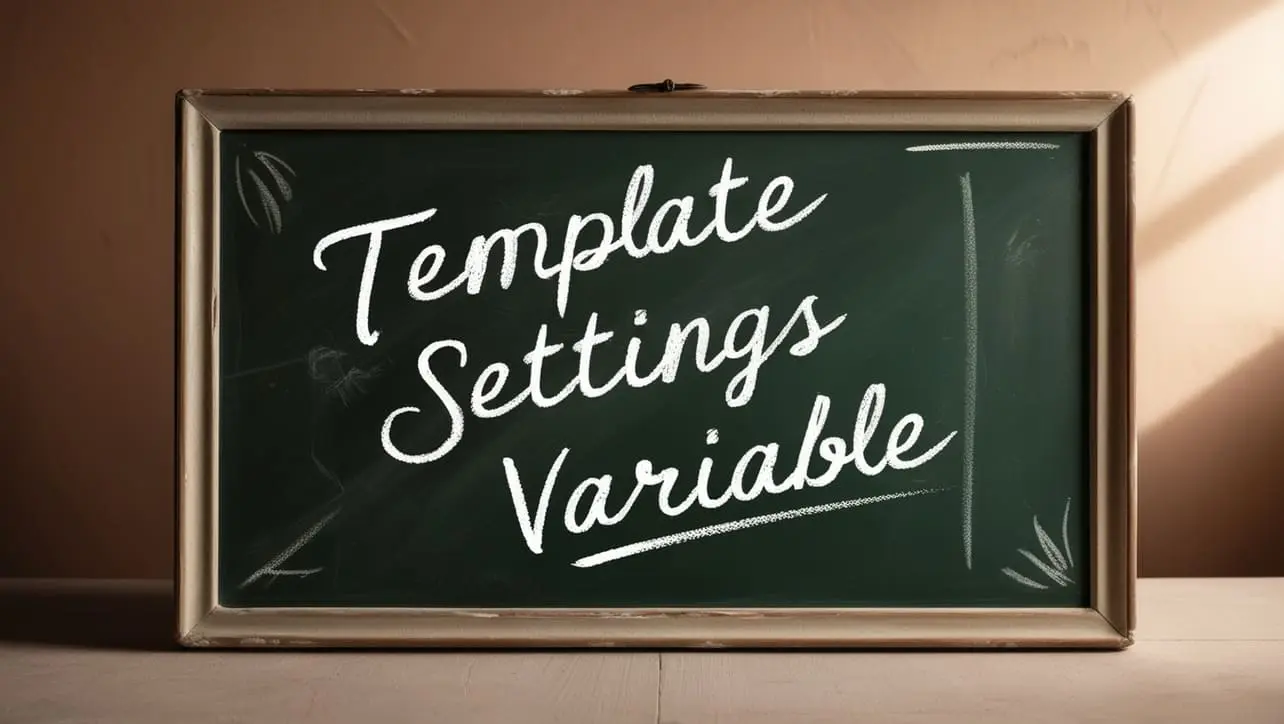
If you have any doubts regarding this article (Lodash _.flatten() Array Method), please comment here. I will help you immediately.