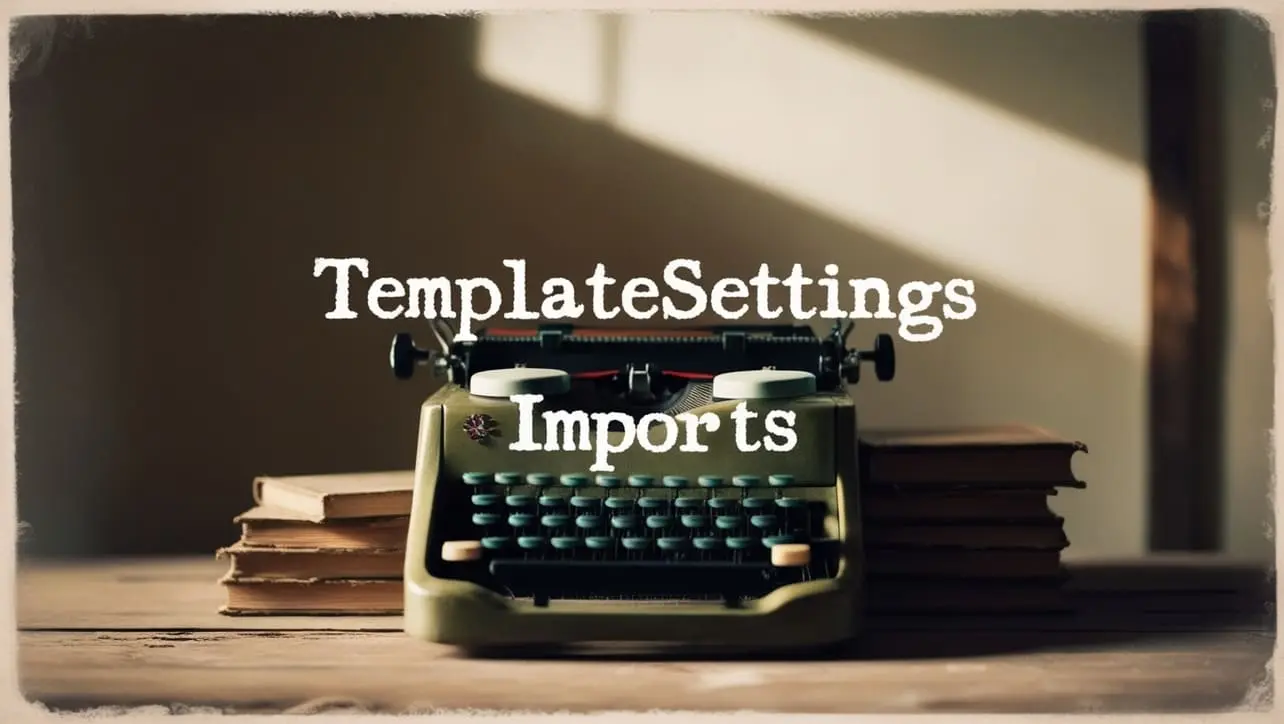
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.findLastIndex() Array Method
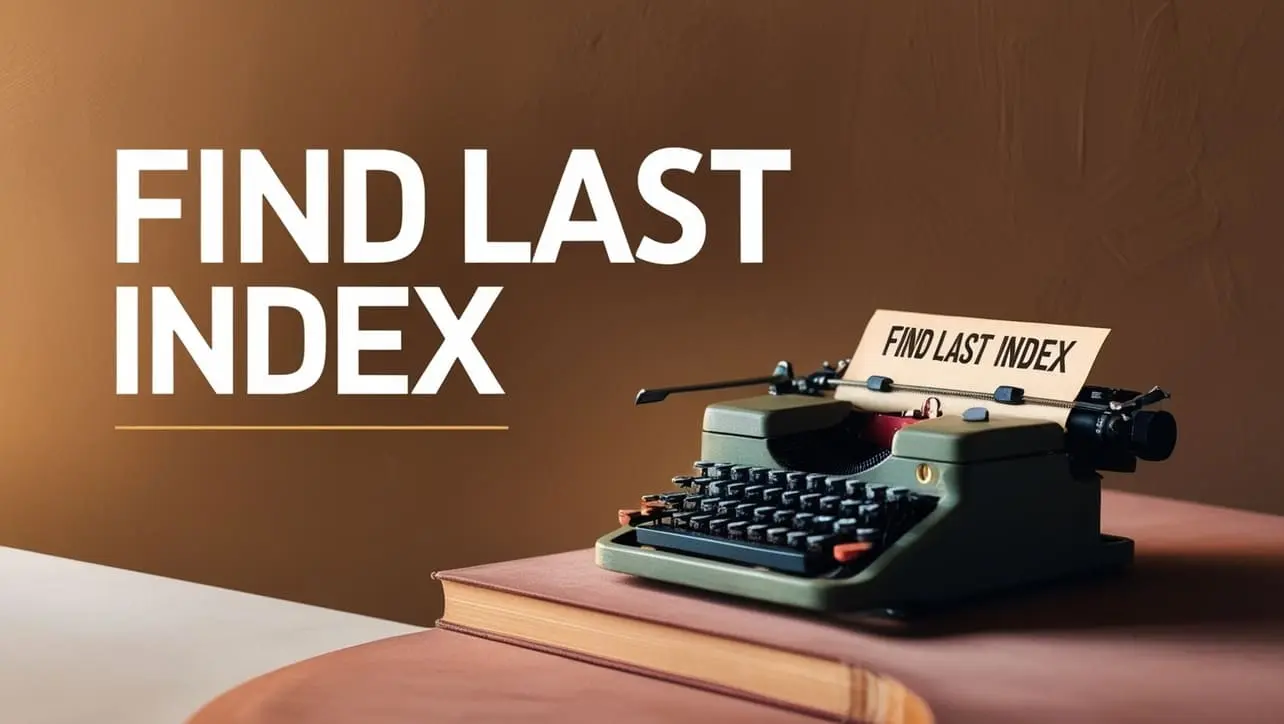
Photo Credit to CodeToFun
🙋 Introduction
When it comes to working with arrays in JavaScript, efficient search operations are crucial. Lodash, a widely-used utility library, provides a robust solution with the _.findLastIndex()
method.
This method empowers developers to locate the last occurrence of an element in an array, offering flexibility and ease of use.
🧠 Understanding _.findLastIndex()
The _.findLastIndex()
method in Lodash enables you to find the index of the last element in an array that satisfies a given condition. This is particularly useful when dealing with datasets where the order of occurrence matters.
💡 Syntax
_.findLastIndex(array, [predicate=_.identity], [fromIndex=array.length-1])
- array: The array to search.
- predicate: The function invoked per iteration.
- fromIndex: The index to start searching from (default is array.length-1).
📝 Example
Let's explore a practical example to illustrate the functionality of _.findLastIndex()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const numbers = [1, 2, 3, 4, 2, 5, 6, 2, 7];
const lastIndex = _.findLastIndex(numbers, (num) => num === 2);
console.log(lastIndex);
// Output: 7
In this example, _.findLastIndex()
is used to find the last index where the value is equal to 2 in the numbers array.
🏆 Best Practices
Define a Clear Predicate Function:
When using
_.findLastIndex()
, ensure that your predicate function is well-defined and accurately captures the condition you are searching for.clear-predicate-function.jsCopiedconst users = [ { id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Alice' } ]; const lastAliceIndex = _.findLastIndex(users, (user) => user.name === 'Alice'); console.log(lastAliceIndex); // Output: 2
Handle Non-Matching Scenarios:
If there's a possibility that the predicate won't match any elements in the array, consider adding logic to handle such scenarios.
non-matching-scenarios.jsCopiedconst nonMatchingIndex = _.findLastIndex(numbers, (num) => num === 8); if (nonMatchingIndex === -1) { console.log('Element not found in the array.'); }
Leverage fromIndex for Optimization:
If you have knowledge of the array structure, consider using the fromIndex parameter to optimize your search.
optimization,jsCopiedconst optimizedIndex = _.findLastIndex(numbers, (num) => num === 2, 5); console.log(optimizedIndex); // Output: 4
📚 Use Cases
Managing Historical Data:
In scenarios where you are dealing with historical data,
_.findLastIndex()
can help identify the last occurrence of a specific event or state.managing-historical-data.jsCopiedconst historicalData = /* ...fetch historical data... */; const lastEventIndex = _.findLastIndex(historicalData, (event) => event.type === 'userLogin'); console.log(lastEventIndex);
Reversing Iteration:
When iterating backward through an array, finding the last index of a certain condition becomes effortless with
_.findLastIndex()
.reversing-iteration.jsCopiedconst reversedNumbers = numbers.slice().reverse(); const lastIndexInReversed = _.findLastIndex(reversedNumbers, (num) => num === 2); console.log(lastIndexInReversed); // Output: 2
🎉 Conclusion
The _.findLastIndex()
method in Lodash is a valuable asset for developers seeking an efficient way to locate the last occurrence of an element in an array. Its flexibility, combined with thoughtful predicate functions, opens up a realm of possibilities for array manipulation and search operations.
Embrace the power of Lodash and enhance your array-handling capabilities with _.findLastIndex()
!
👨💻 Join our Community:
Author
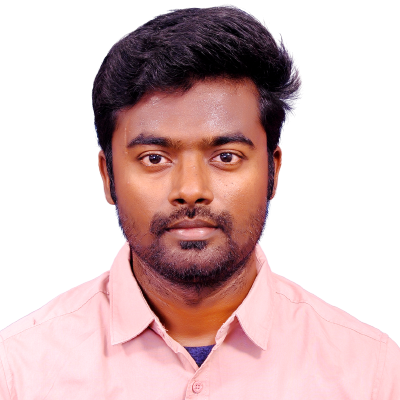
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
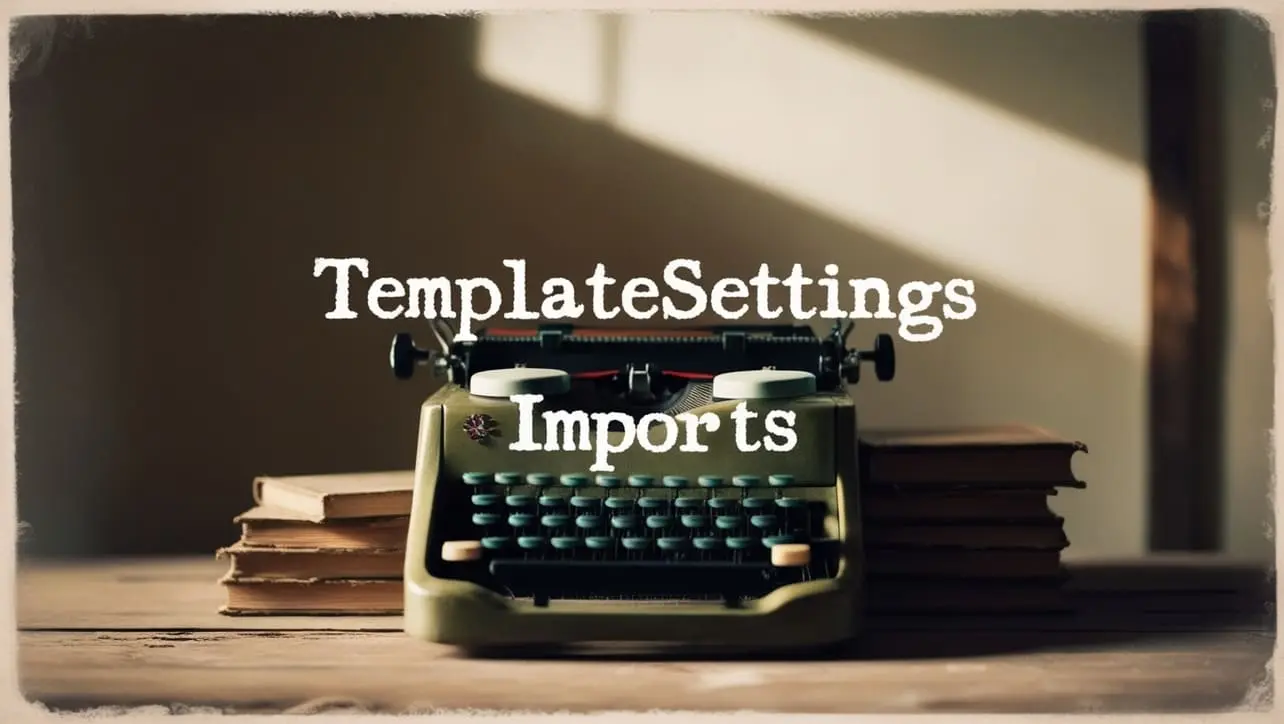
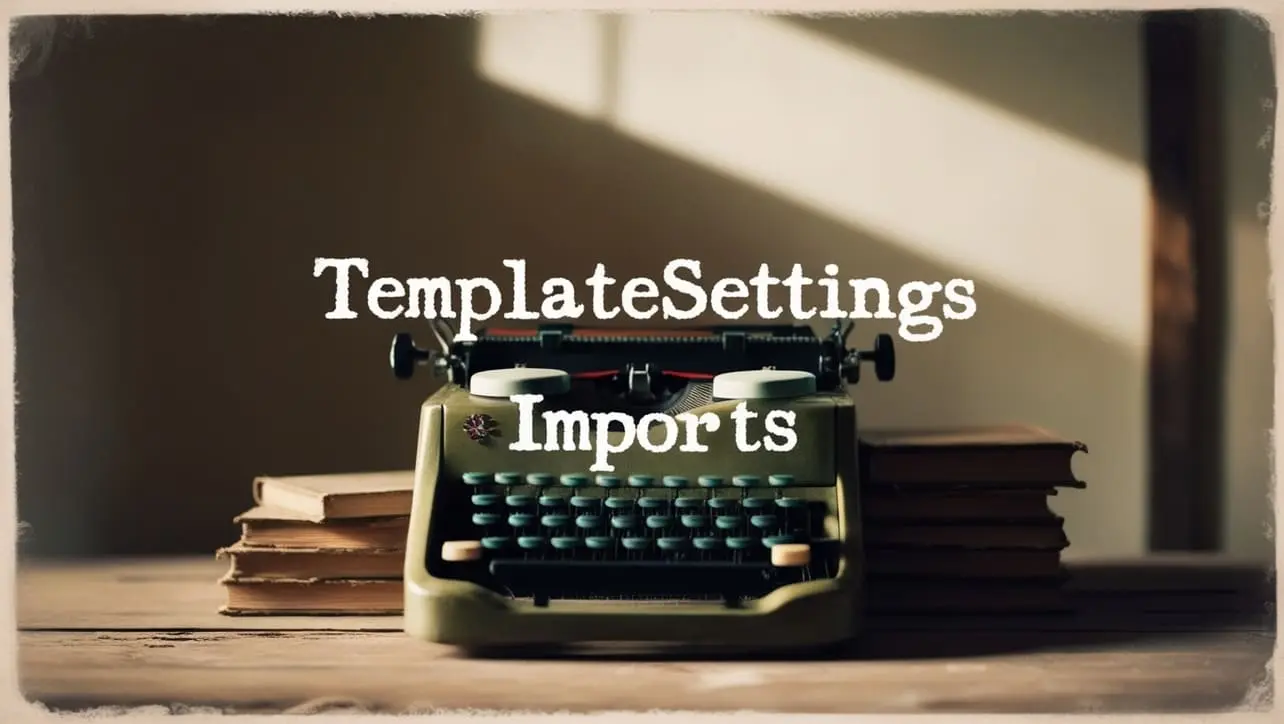
Lodash _.templateSettings.imports Property
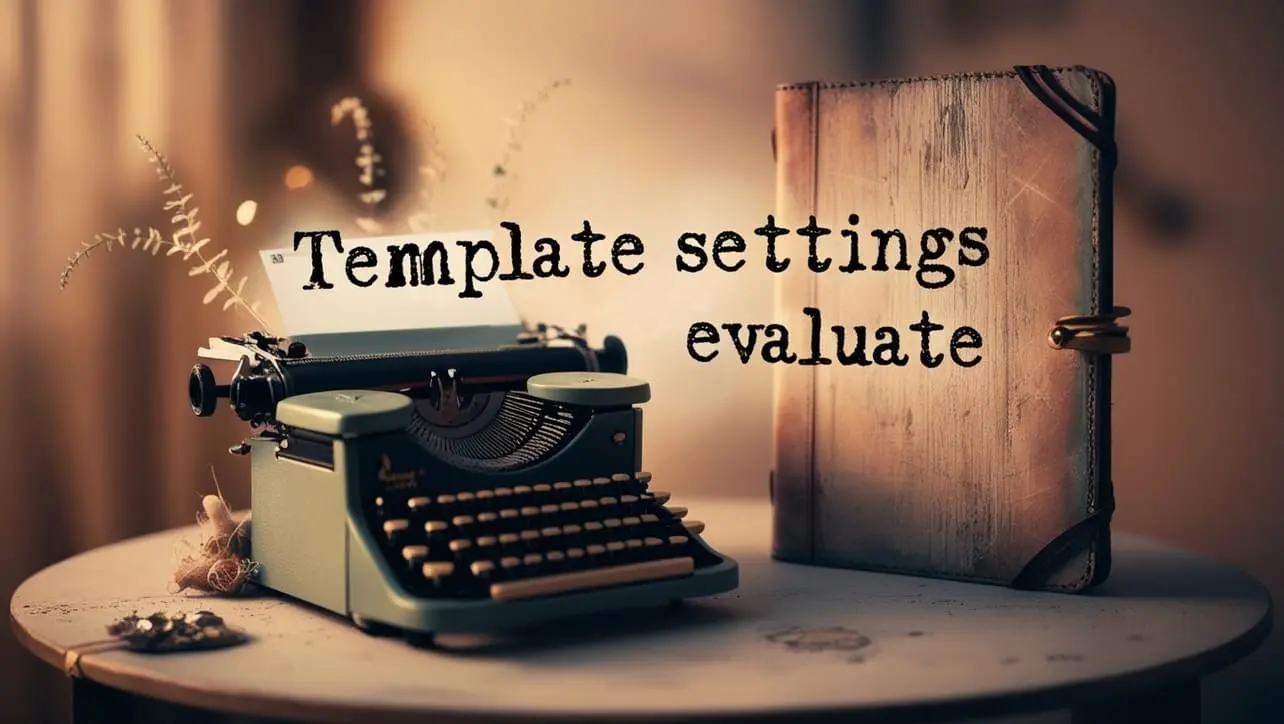
Lodash _.templateSettings.evaluate Property
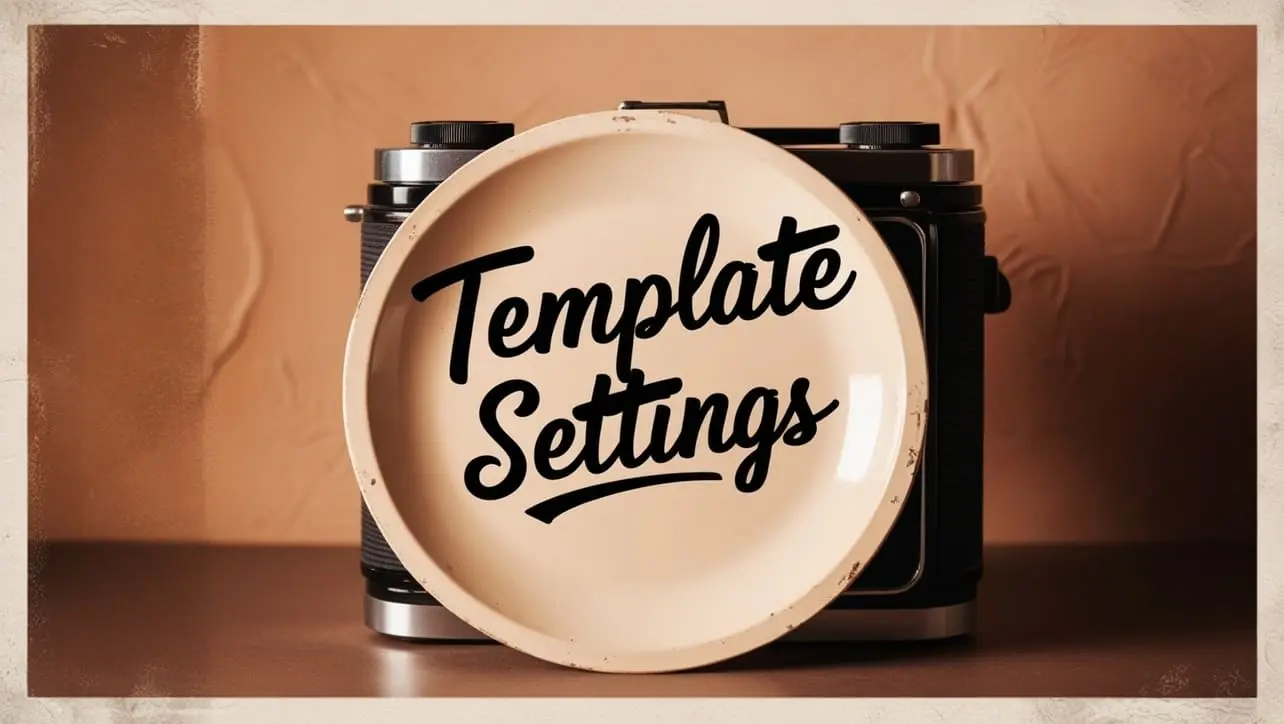
Lodash _.templateSettings Property
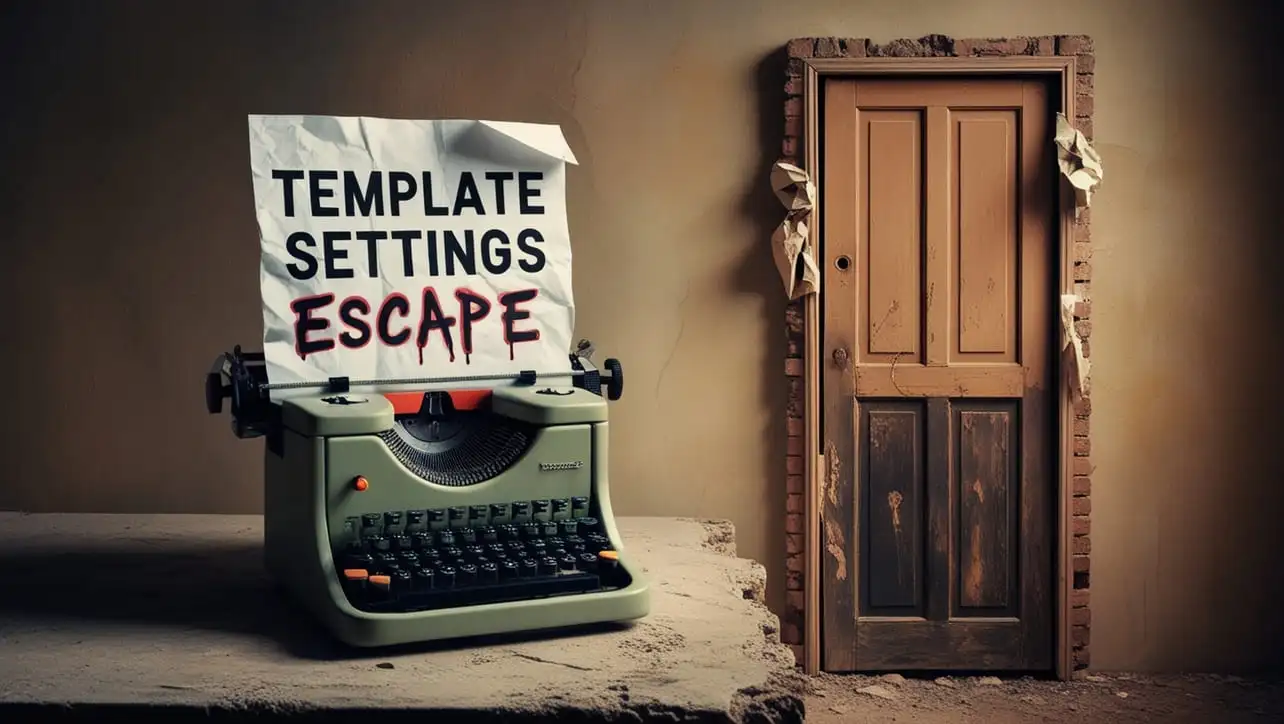
Lodash _.templateSettings.escape Property
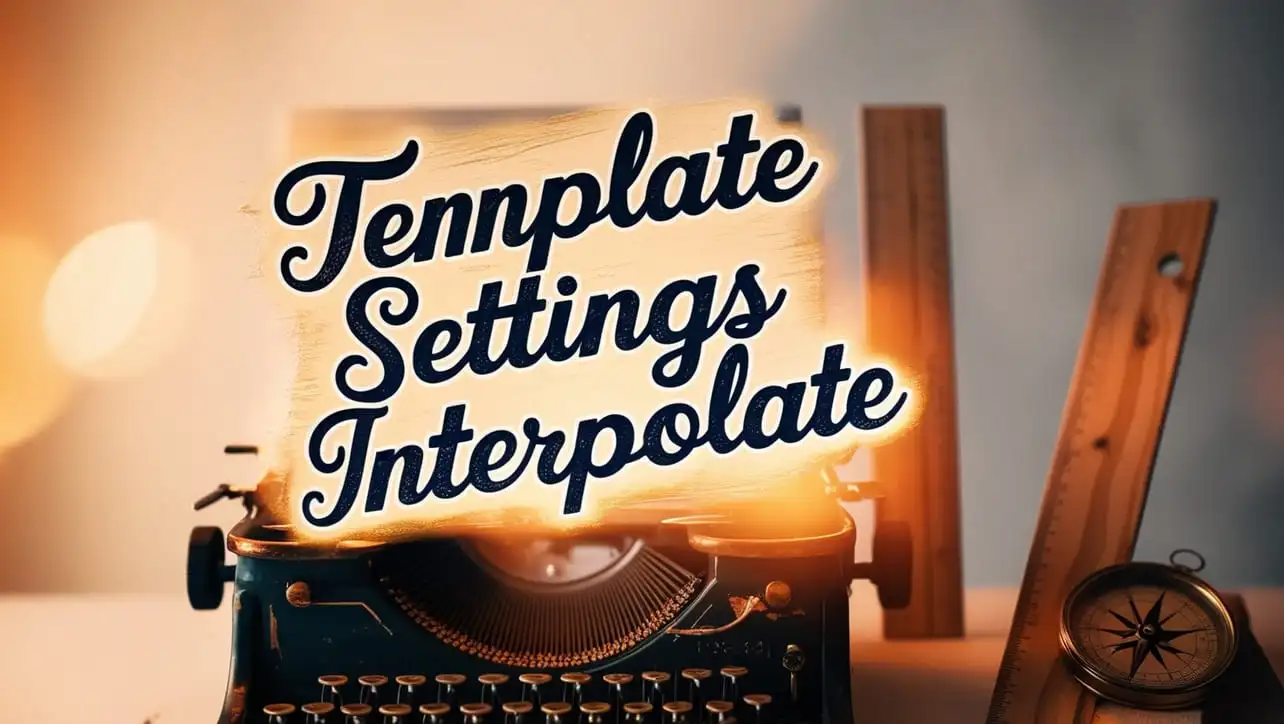
Lodash _.templateSettings.interpolate Property
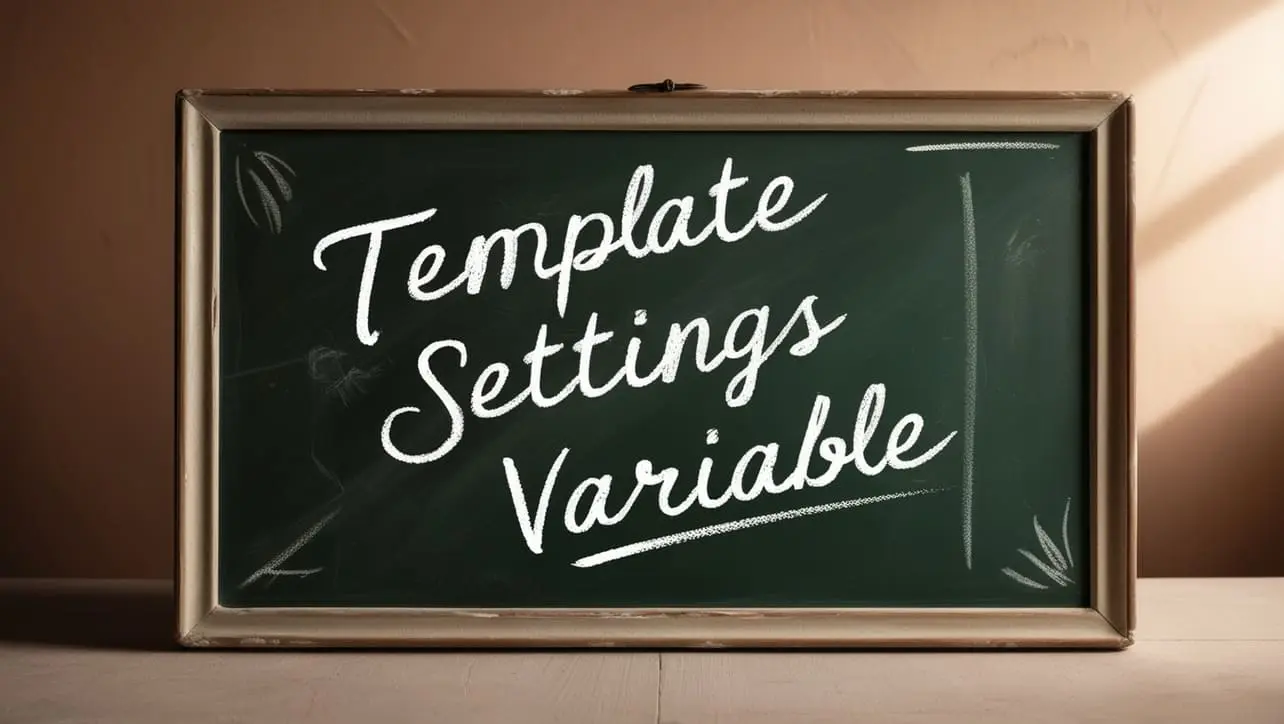
If you have any doubts regarding this article (Lodash _.findLastIndex() Array Method), please comment here. I will help you immediately.