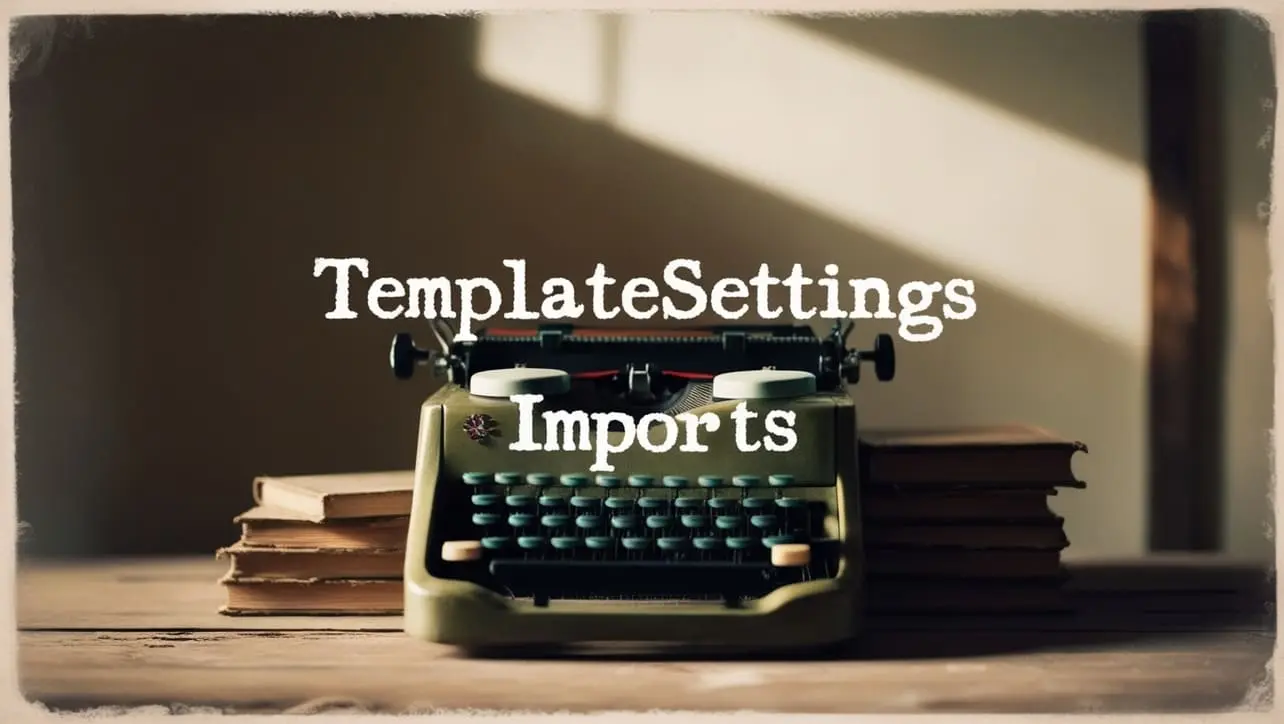
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.drop() Array Method
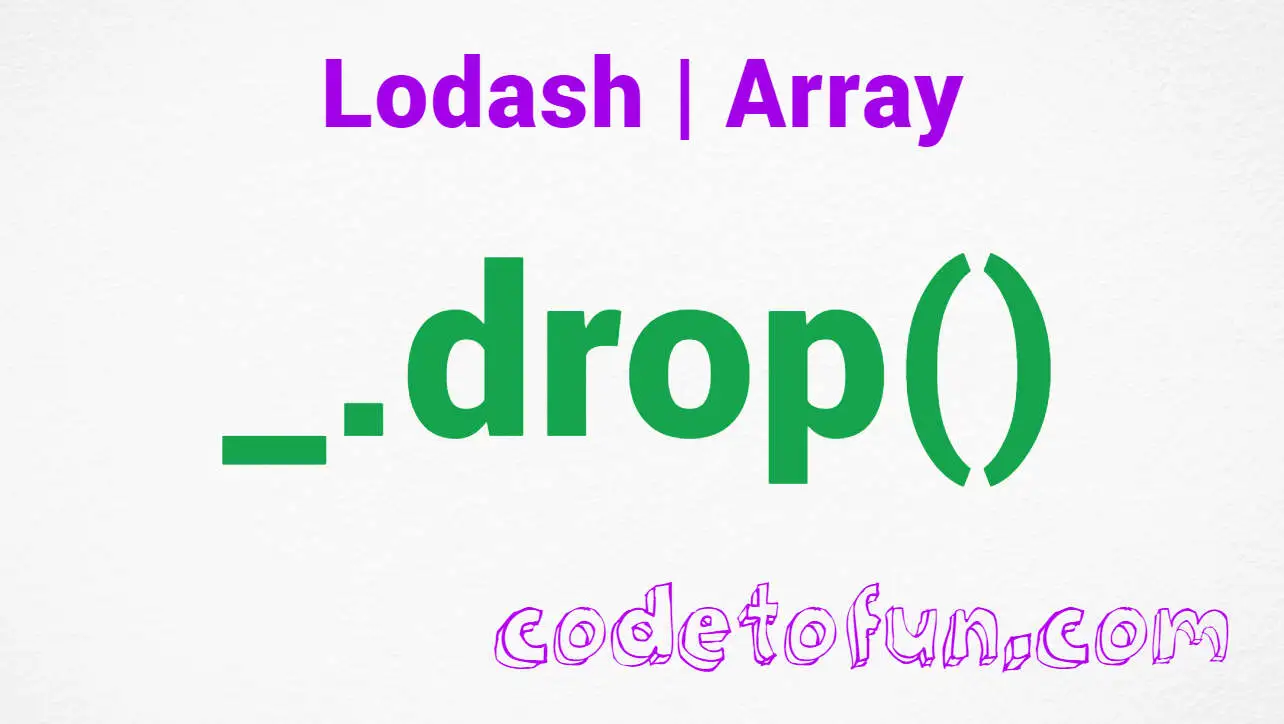
Photo Credit to CodeToFun
🙋 Introduction
Efficient array manipulation is a fundamental aspect of JavaScript programming. The Lodash library provides a range of utility functions, and among them is the _.drop()
method.
This method is designed to simplify the process of excluding elements from the beginning of an array, providing developers with a versatile tool for data manipulation.
🧠 Understanding _.drop()
The _.drop()
method in Lodash allows you to create a new array by excluding a specified number of elements from the beginning of an existing array.
This functionality is particularly useful when dealing with datasets where you want to skip a certain number of initial elements.
💡 Syntax
_.drop(array, [n=1])
- array: The array to process.
- n: The number of elements to drop from the beginning (default is 1).
📝 Example
Let's explore a practical example to illustrate how _.drop()
works:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const originalArray = [1, 2, 3, 4, 5, 6, 7, 8, 9];
const droppedArray = _.drop(originalArray, 3);
console.log(droppedArray);
// Output: [4, 5, 6, 7, 8, 9]
In this example, the originalArray has the first 3 elements dropped, creating a new array with the remaining elements.
🏆 Best Practices
Validate Inputs:
Before using
_.drop()
, ensure that the input array is valid and contains elements. Additionally, validate the number of elements to drop to avoid unexpected behavior.validate-inputs.jsCopiedif (!Array.isArray(originalArray) || originalArray.length === 0) { console.error('Invalid input array'); return; } const elementsToDrop = 3; // Set your desired number of elements to drop if (elementsToDrop < 0) { console.error('Invalid number of elements to drop'); return; } const validatedDroppedArray = _.drop(originalArray, elementsToDrop); console.log(validatedDroppedArray);
Handle Edge Cases:
Consider edge cases, such as dropping more elements than the array contains. Implement appropriate error handling or default behaviors to address these scenarios.
handle-edge-cases.jsCopiedconst elementsToDropMoreThanArrayLength = 15; const droppedArrayEdgeCase = _.drop(originalArray, elementsToDropMoreThanArrayLength); // Returns: [] console.log(droppedArrayEdgeCase);
Use Negative Values:
To drop elements from the end of the array, you can utilize negative values for the n parameter.
use-negative-values.jsCopiedconst elementsToDropFromEnd = -2; const droppedArrayFromEnd = _.drop(originalArray, elementsToDropFromEnd); console.log(droppedArrayFromEnd); // Output: [1, 2, 3, 4, 5, 6, 7]
📚 Use Cases
Pagination:
In scenarios where you're implementing pagination and want to skip a certain number of initial items,
_.drop()
can be a valuable tool.pagination.jsCopiedconst dataForPagination = /* ...fetch data from API or elsewhere... */; const itemsPerPage = 10; const paginatedData = _.drop(dataForPagination, (currentPage - 1) * itemsPerPage); console.log(paginatedData);
Skip Header Rows:
When processing data with header rows in a CSV or similar format,
_.drop()
can help exclude those rows from your data processing logic.skip-header-rows.jsCopiedconst dataWithHeaders = /* ...fetch data from a file or API... */; const numberOfHeaderRows = 1; const dataWithoutHeaders = _.drop(dataWithHeaders, numberOfHeaderRows); console.log(dataWithoutHeaders);
🎉 Conclusion
The _.drop()
method in Lodash is a powerful asset for JavaScript developers working with arrays. Whether you're implementing pagination, processing data, or skipping header rows, this method provides a clean and efficient solution. Incorporate _.drop()
into your projects to enhance array manipulation capabilities and streamline your code.
Explore the full potential of Lodash and elevate your array manipulation skills with _.drop()
!
👨💻 Join our Community:
Author
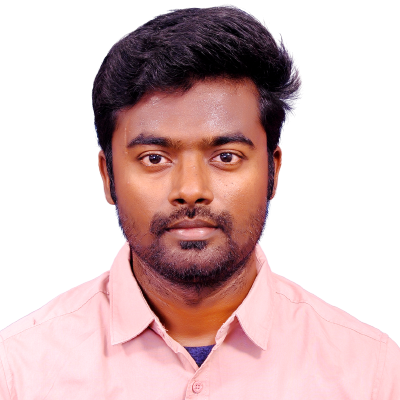
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
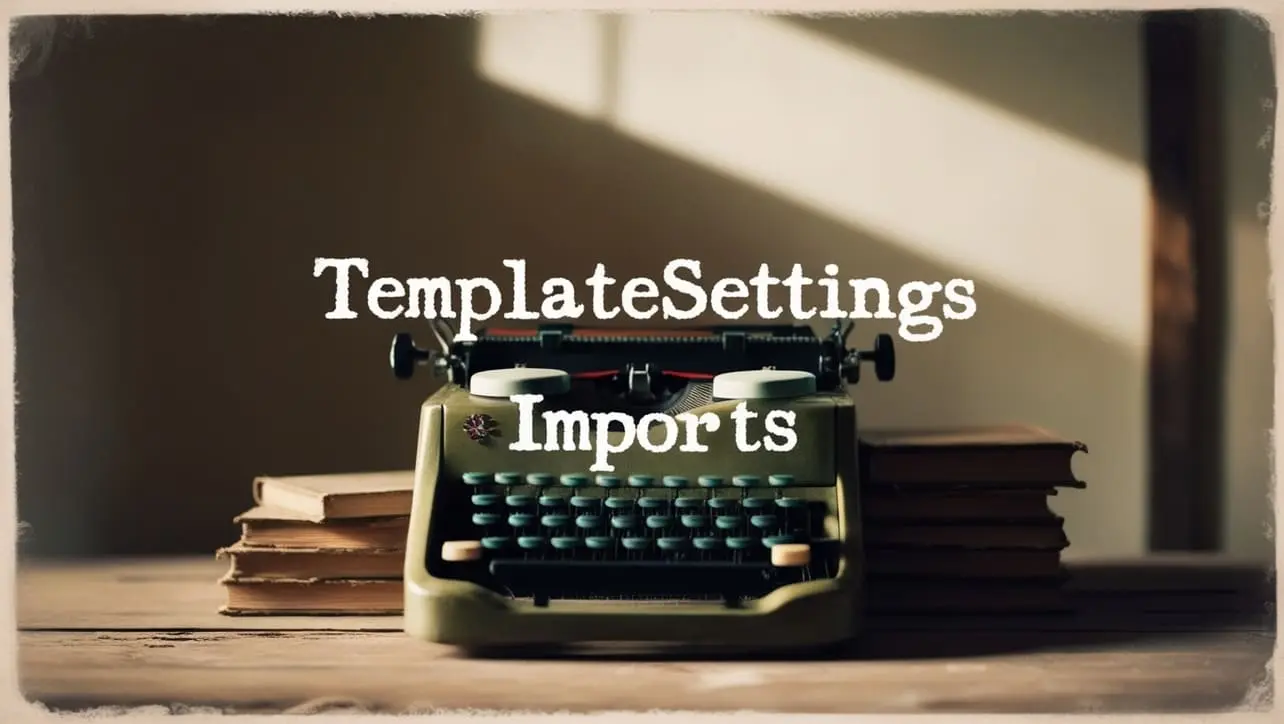
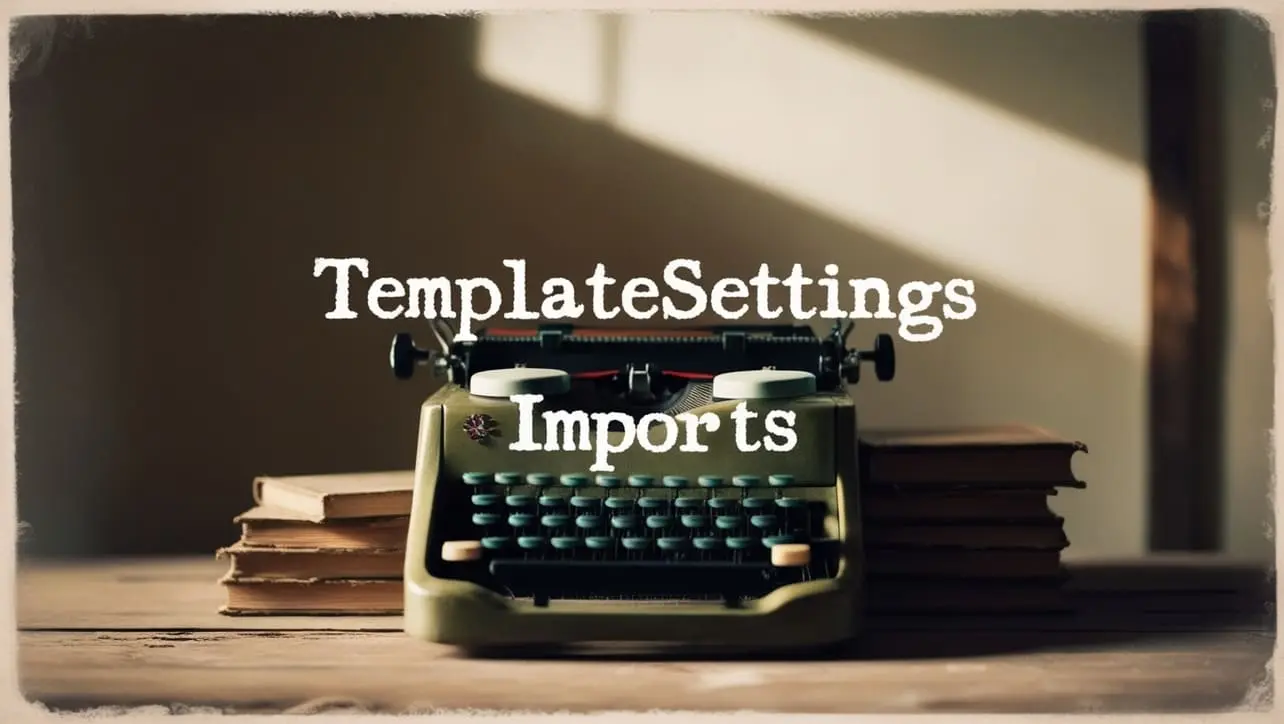
Lodash _.templateSettings.imports Property
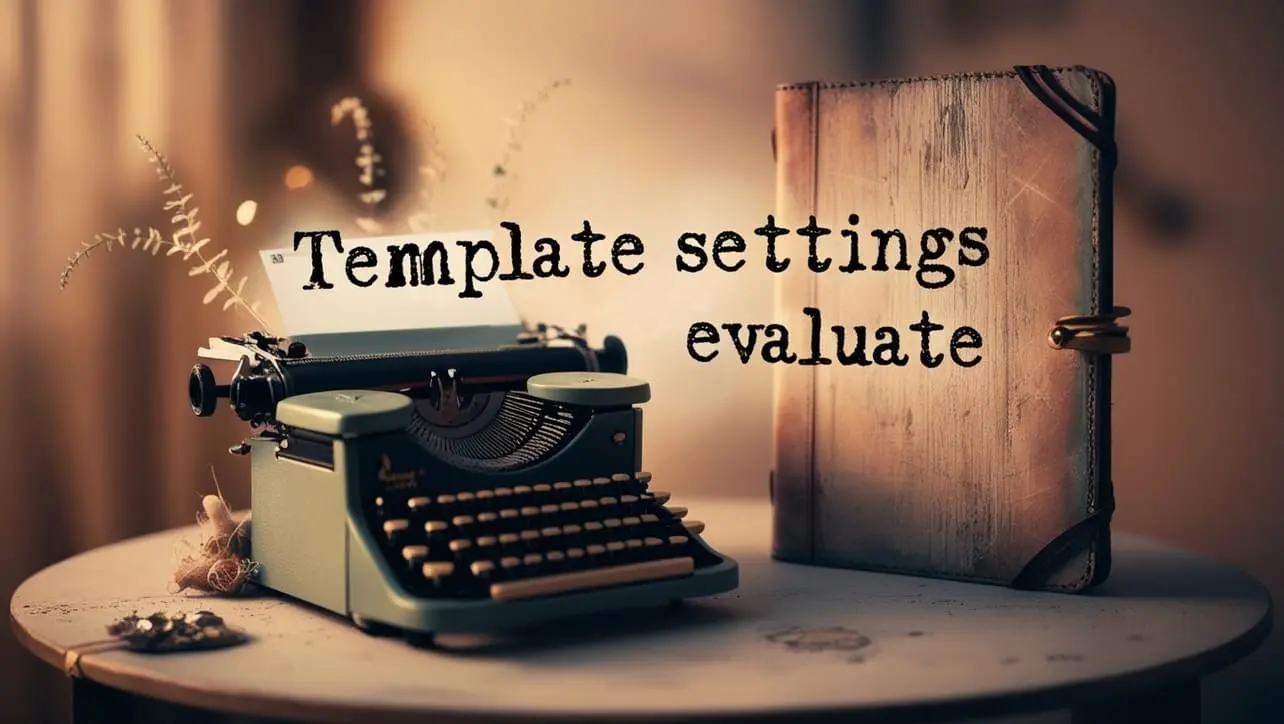
Lodash _.templateSettings.evaluate Property
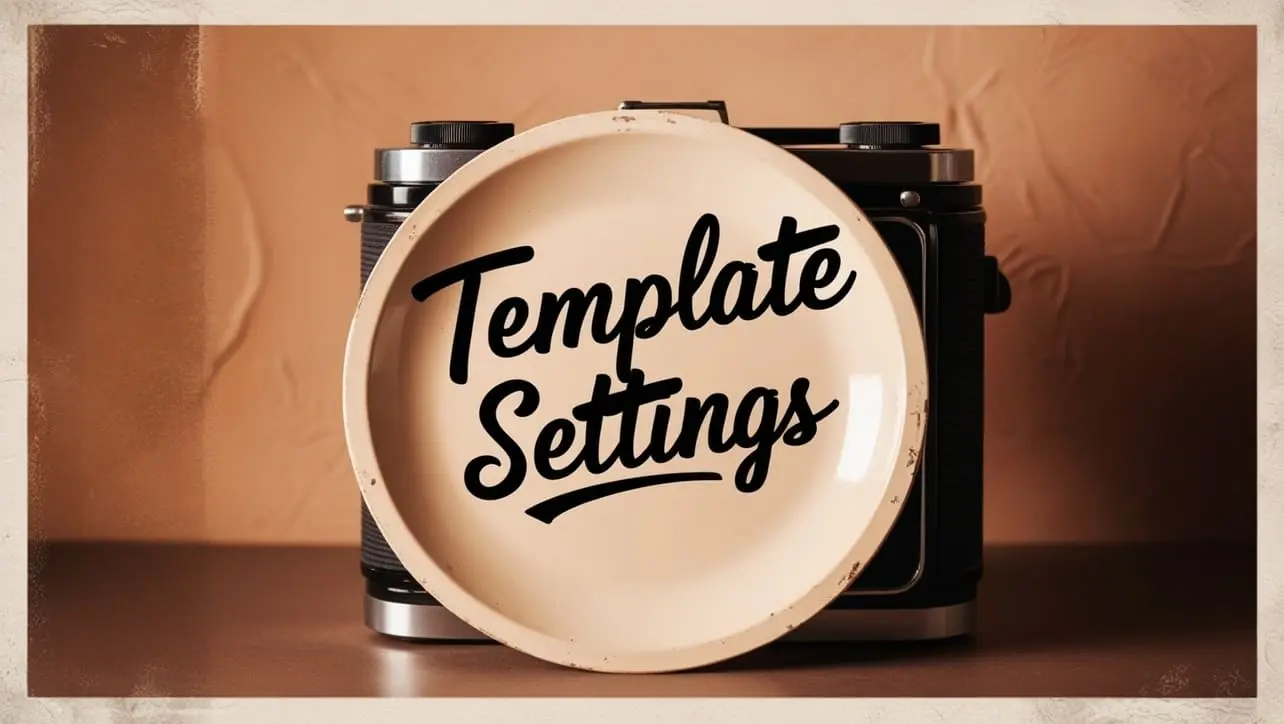
Lodash _.templateSettings Property
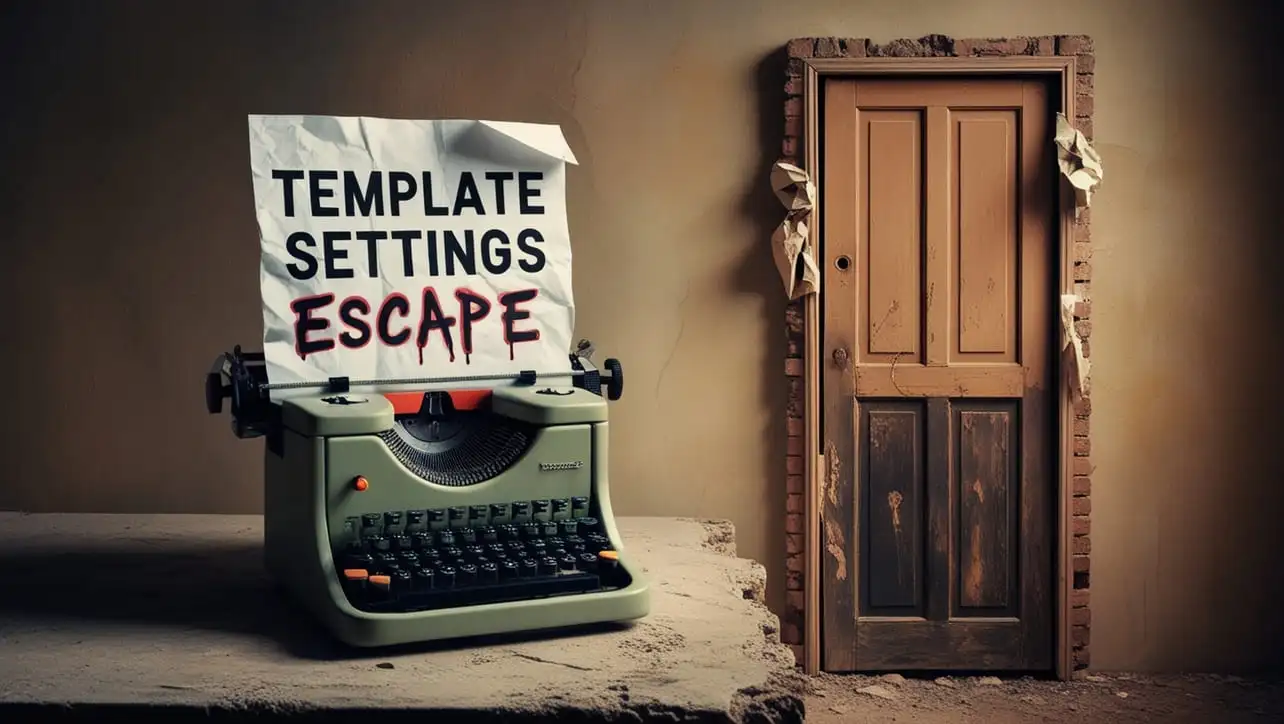
Lodash _.templateSettings.escape Property
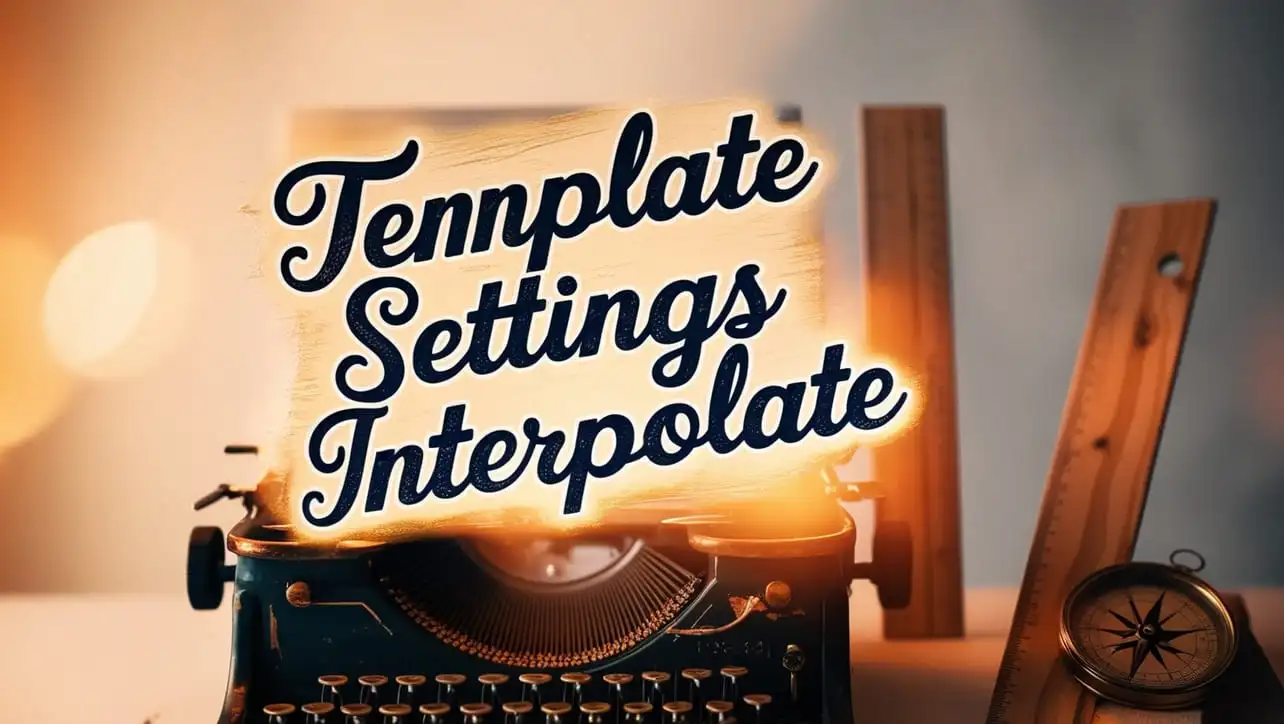
Lodash _.templateSettings.interpolate Property
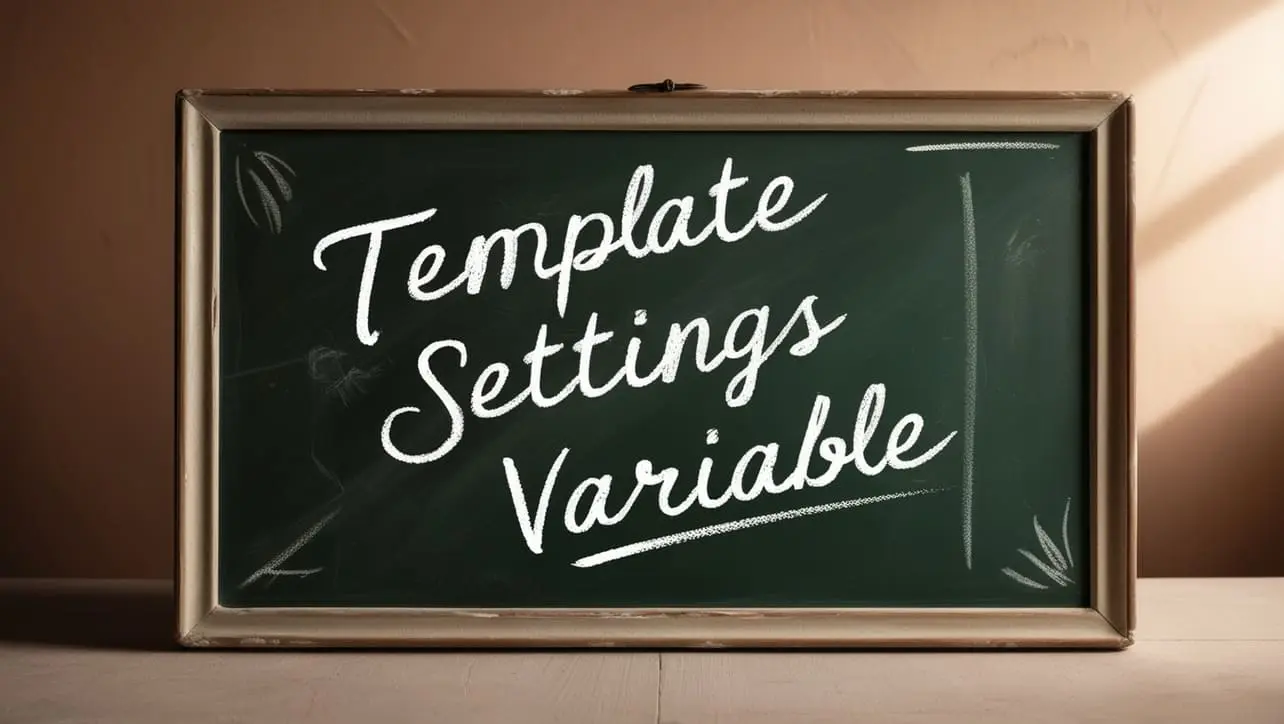
If you have any doubts regarding this article (Lodash _.drop() Array Method), please comment here. I will help you immediately.