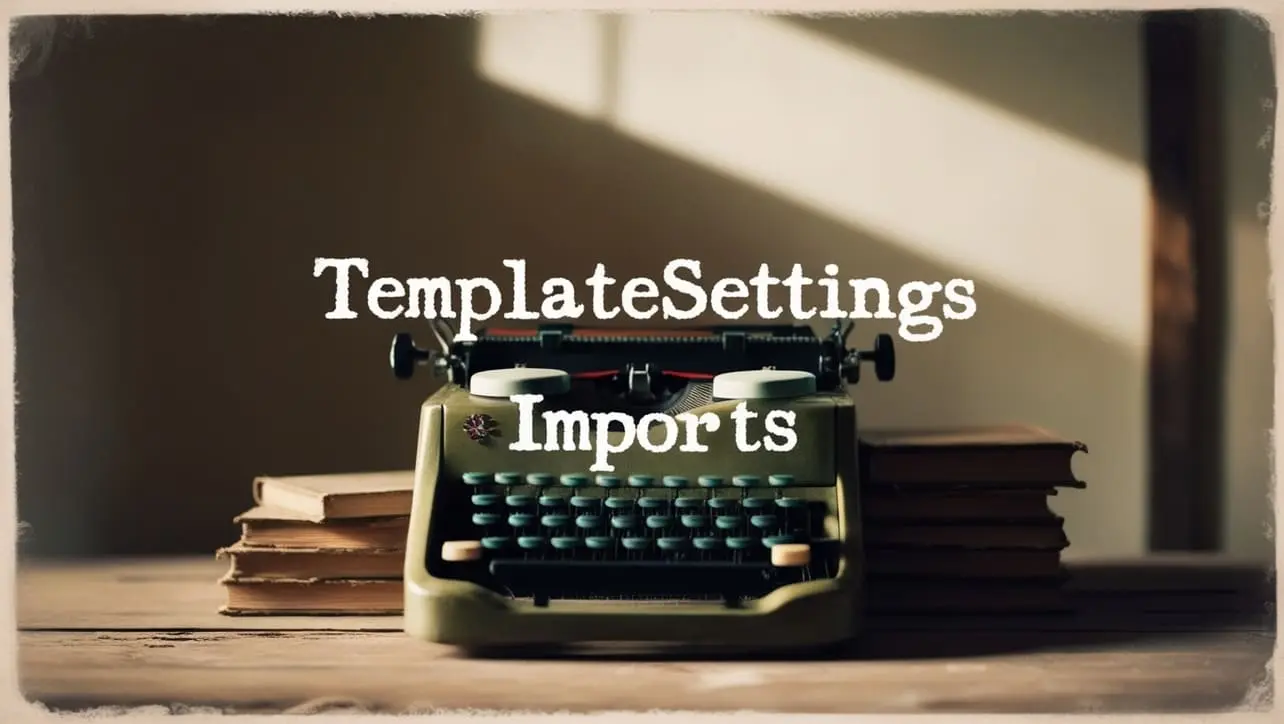
Lodash Home
- Lodash Intro
- Lodash Array
- _.chunk
- _.compact
- _.concat
- _.difference
- _.differenceBy
- _.differenceWith
- _.drop
- _.dropRight
- _.dropRightWhile
- _.dropWhile
- _.fill
- _.findIndex
- _.findLastIndex
- _.flatten
- _.flattenDeep
- _.flattenDepth
- _.fromPairs
- _.head
- _.indexOf
- _.initial
- _.intersection
- _.intersectionBy
- _.intersectionWith
- _.join
- _.last
- _.lastIndexOf
- _.nth
- _.pull
- _.pullAll
- _.pullAllBy
- _.pullAllWith
- _.pullAt
- _.remove
- _.reverse
- _.slice
- _.sortedIndex
- _.sortedIndexBy
- _.sortedIndexOf
- _.sortedLastIndex
- _.sortedLastIndexBy
- _.sortedLastIndexOf
- _.sortedUniq
- _.sortedUniqBy
- _.tail
- _.take
- _.takeRight
- _.takeRightWhile
- _.takeWhile
- _.union
- _.unionBy
- _.unionWith
- _.uniq
- _.uniqBy
- _.uniqWith
- _.unzip
- _.unzipWith
- _.without
- _.xor
- _.xorBy
- _.xorWith
- _.zip
- _.zipObject
- _.zipObjectDeep
- _.zipWith
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.concat() Array Method
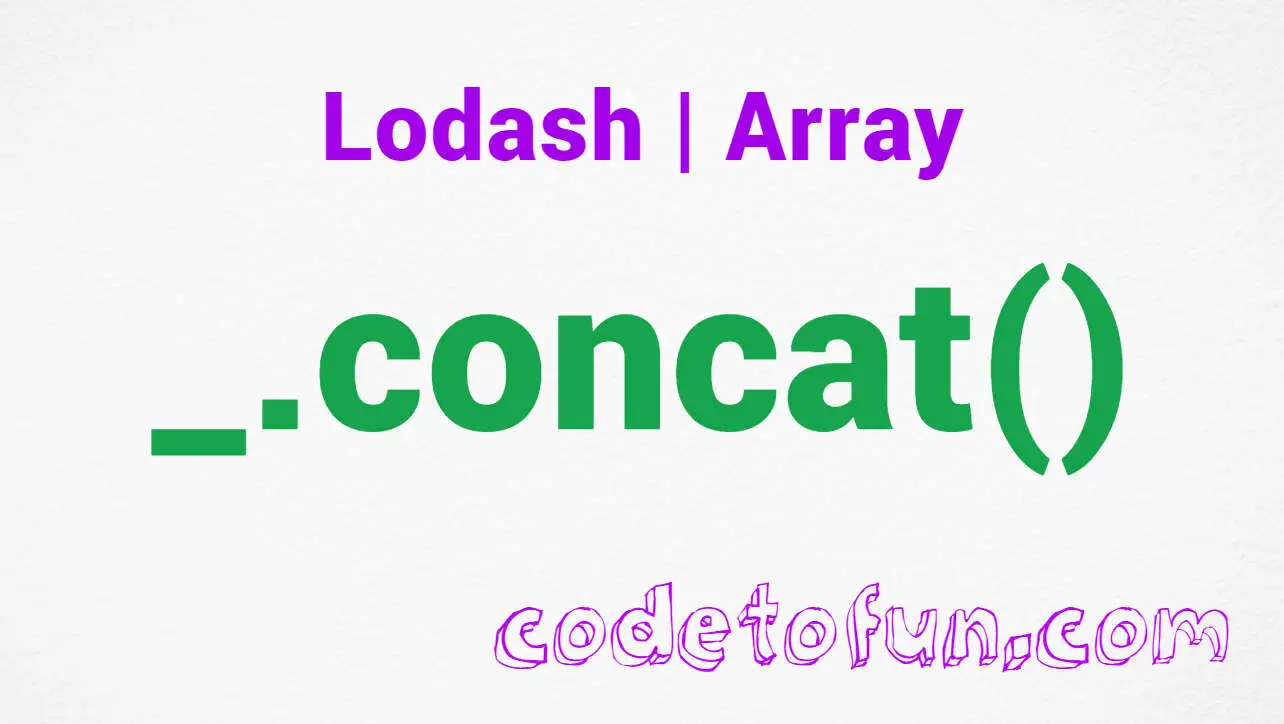
Photo Credit to CodeToFun
🙋 Introduction
Effective array manipulation is a cornerstone of JavaScript development, and Lodash provides a wealth of utility functions to streamline this process. One such versatile tool is the _.concat()
method, which simplifies the task of concatenating arrays.
This method proves invaluable when combining multiple arrays or values into a single array, offering developers a concise and efficient solution.
🧠 Understanding _.concat()
The _.concat()
method in Lodash enables the concatenation of arrays or values into a new array. Whether you need to merge arrays or add individual elements to an existing array, _.concat()
provides a straightforward and effective approach.
💡 Syntax
_.concat(array, [values])
- array: The array to concatenate.
- values: The values or arrays to concatenate.
📝 Example
Let's delve into a practical example to illustrate the utility of _.concat()
:
// Include Lodash library (ensure it's installed via npm)
const _ = require('lodash');
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const concatenatedArray = _.concat(array1, array2);
console.log(concatenatedArray);
// Output: [1, 2, 3, 4, 5, 6]
In this example, array1 and array2 are concatenated into a new array, concatenatedArray.
🏆 Best Practices
Validating Inputs:
Before using
_.concat()
, ensure that the input arrays or values are valid. Handle scenarios where inputs are not arrays to prevent unexpected behavior.validating-inputs.jsCopiedconst validatedArray1 = Array.isArray(array1) ? array1 : []; const validatedArray2 = Array.isArray(array2) ? array2 : []; const concatenatedAndValidatedArray = _.concat(validatedArray1, validatedArray2); console.log(concatenatedAndValidatedArray);
Avoiding Mutations:
_.concat()
creates a new array and does not modify the original arrays. Embrace this immutability to prevent unintended side effects in your code.Concatenating Multiple Arrays:
You can concatenate more than two arrays by providing additional arguments to
_.concat()
.concatenating-multiple-arrays.jsCopiedconst array3 = [7, 8, 9]; const concatenatedMultipleArrays = _.concat(array1, array2, array3); console.log(concatenatedMultipleArrays); // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
📚 Use Cases
Combining Arrays:
The primary use case for
_.concat()
is combining arrays into a single, unified array.combining-arrays.jsCopiedconst userArray = ['John', 'Doe']; const additionalInfo = [25, 'Developer']; const combinedUserInfo = _.concat(userArray, additionalInfo); console.log(combinedUserInfo); // Output: ['John', 'Doe', 25, 'Developer']
Dynamic Array Construction:
Build arrays dynamically by concatenating values based on conditions or user input.
dynamic-array-construction.jsCopiedconst baseArray = [1, 2, 3]; const extraValues = /* ...some dynamically generated values... */; const dynamicArray = _.concat(baseArray, extraValues); console.log(dynamicArray);
Creating Clones:
Use
_.concat()
to create clones of arrays, ensuring the original arrays remain unchanged.creating-clones.jsCopiedconst originalArray = [10, 20, 30]; const arrayClone = _.concat(originalArray); console.log(arrayClone); // Output: [10, 20, 30]
🎉 Conclusion
The _.concat()
method in Lodash is a valuable asset for JavaScript developers seeking an efficient way to concatenate arrays and values. Its simplicity and flexibility make it an excellent choice for a wide range of scenarios, from basic array concatenation to more complex dynamic array construction.
Explore the capabilities of _.concat()
and enhance your array manipulation toolkit with Lodash!
👨💻 Join our Community:
Author
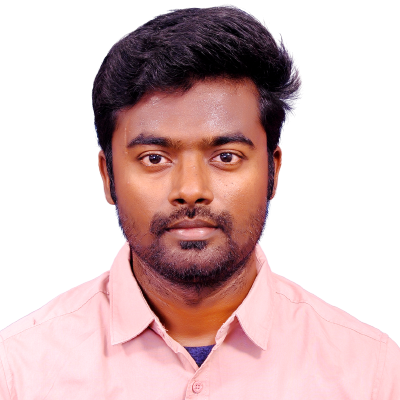
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
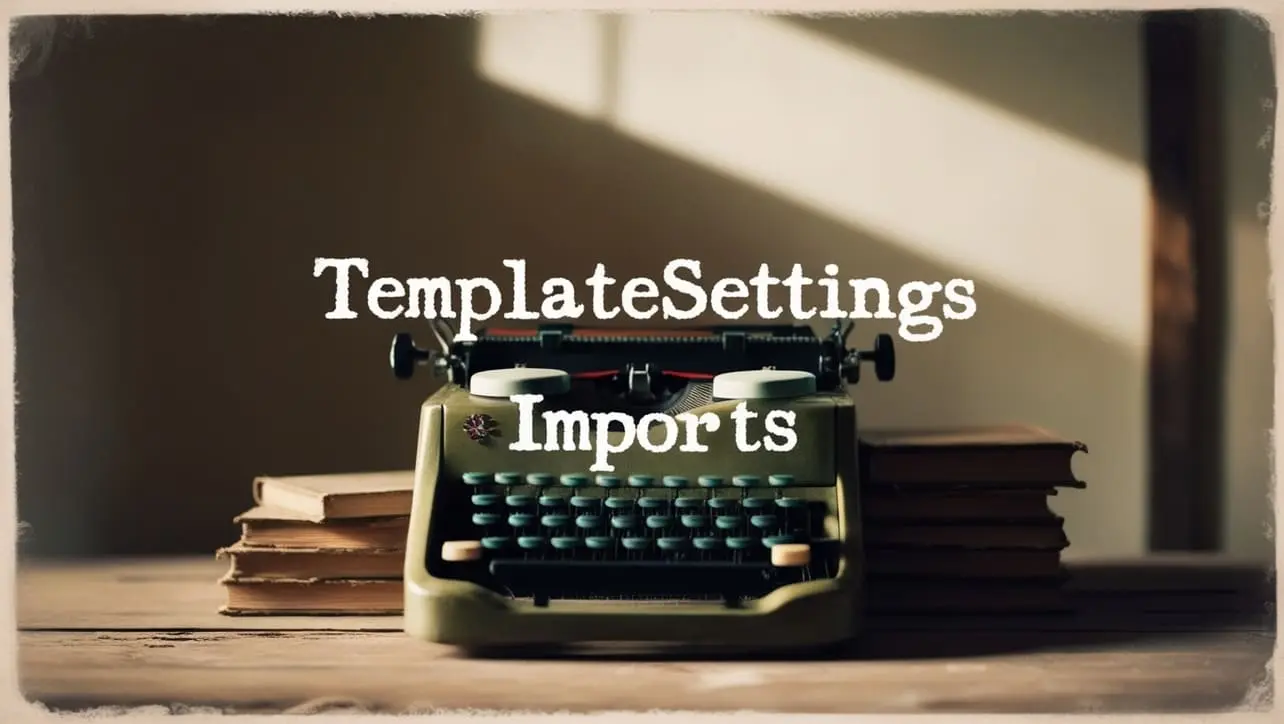
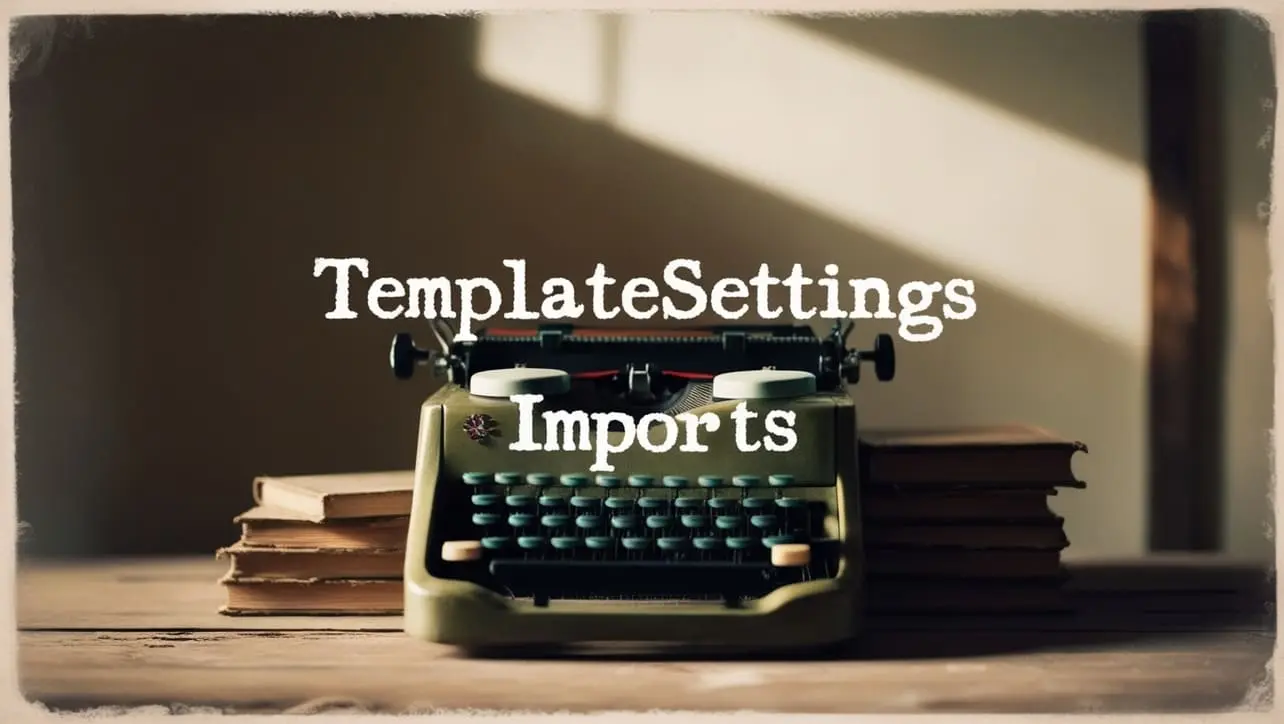
Lodash _.templateSettings.imports Property
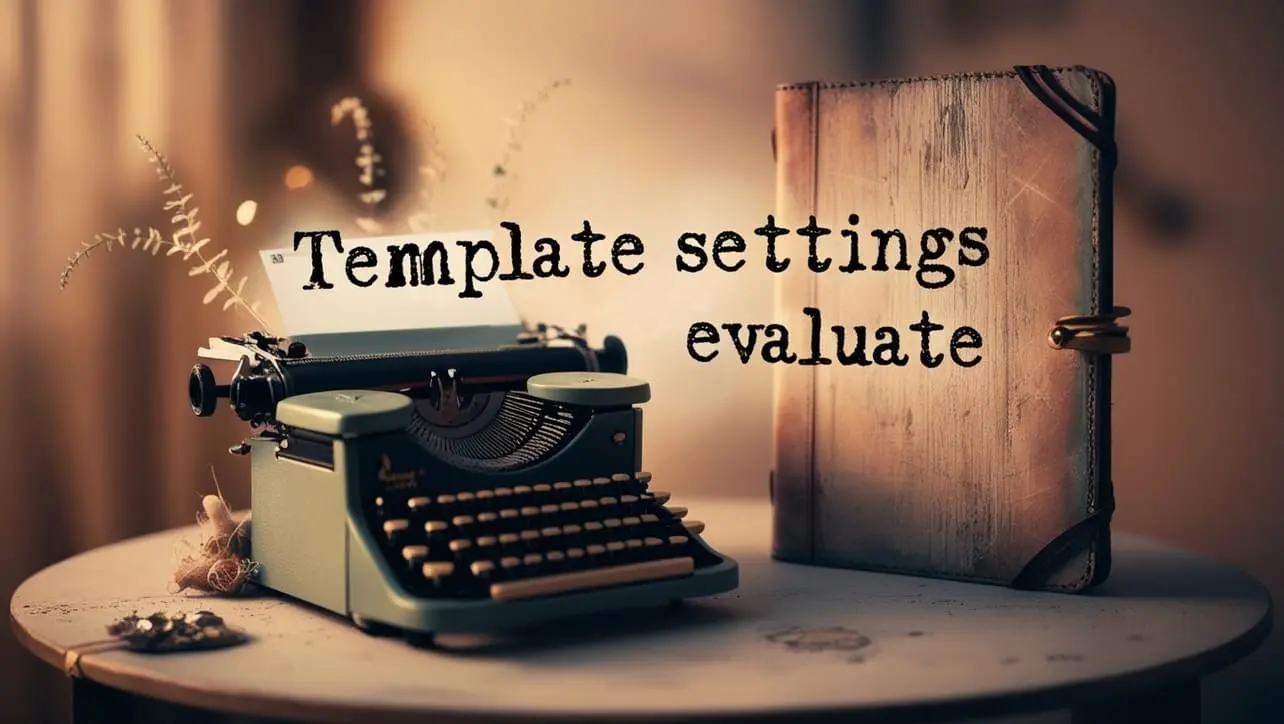
Lodash _.templateSettings.evaluate Property
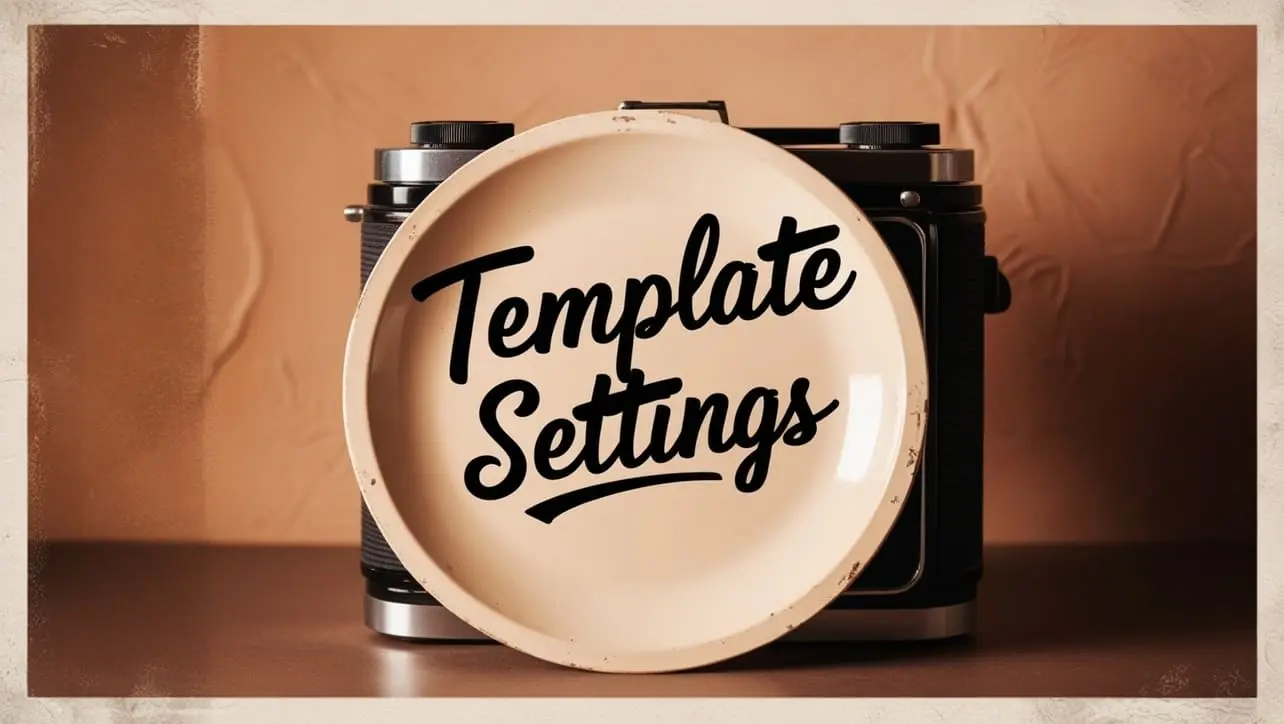
Lodash _.templateSettings Property
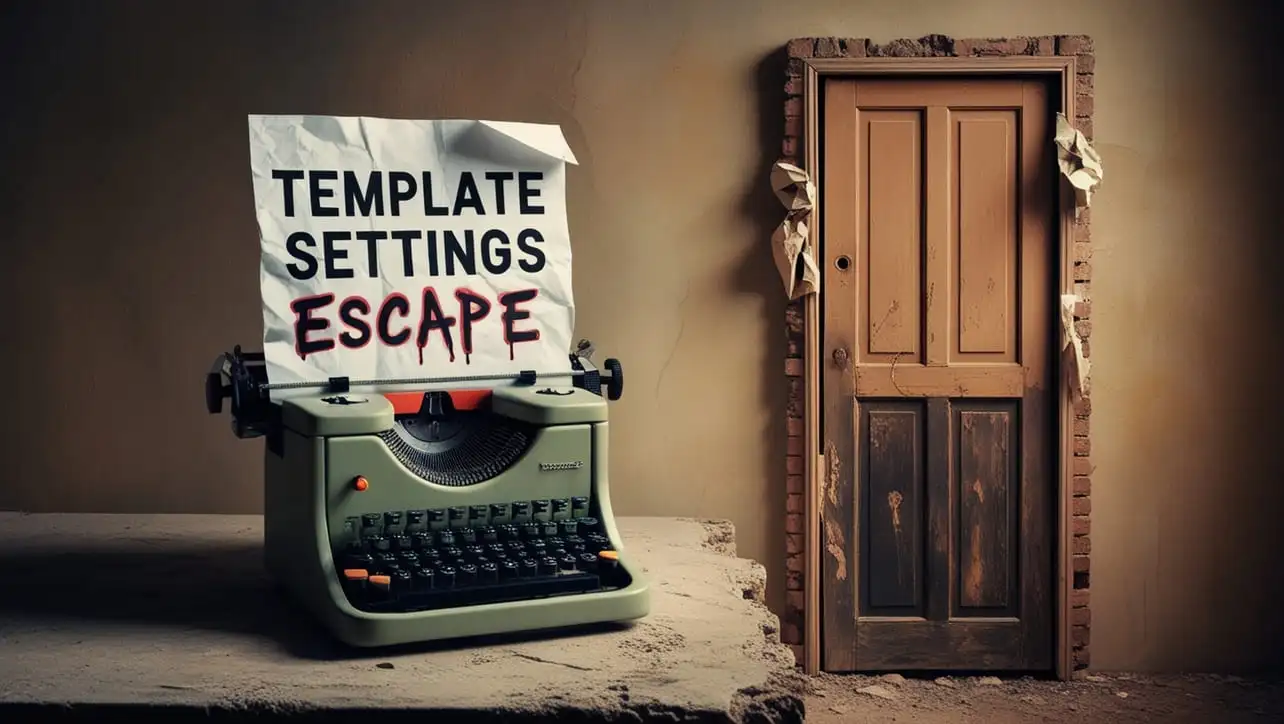
Lodash _.templateSettings.escape Property
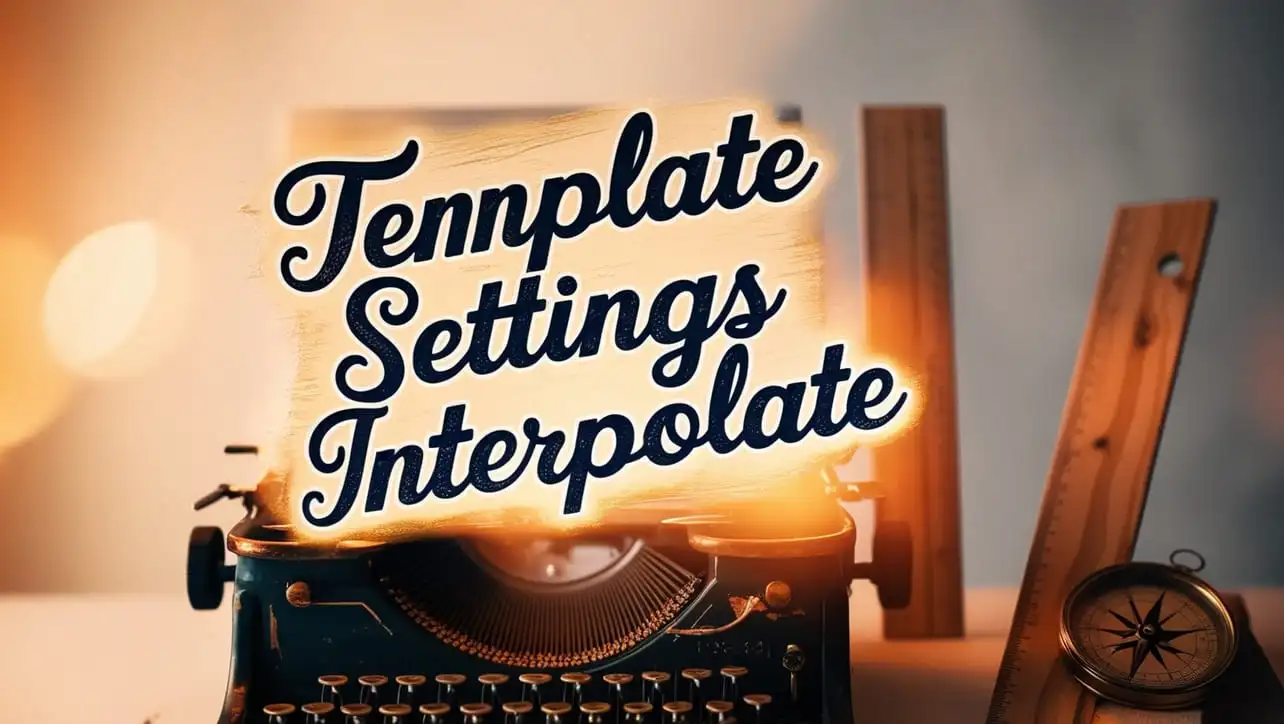
Lodash _.templateSettings.interpolate Property
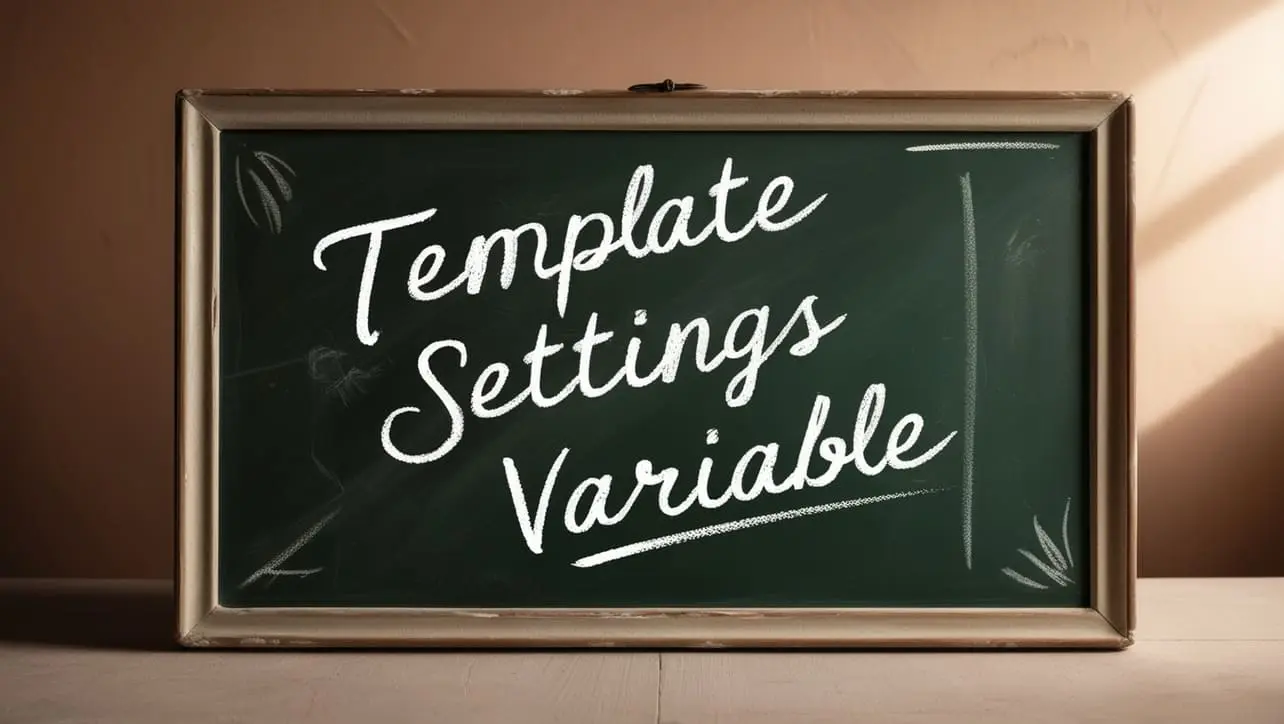
If you have any doubts regarding this article (Lodash _.concat() Array Method), please comment here. I will help you immediately.