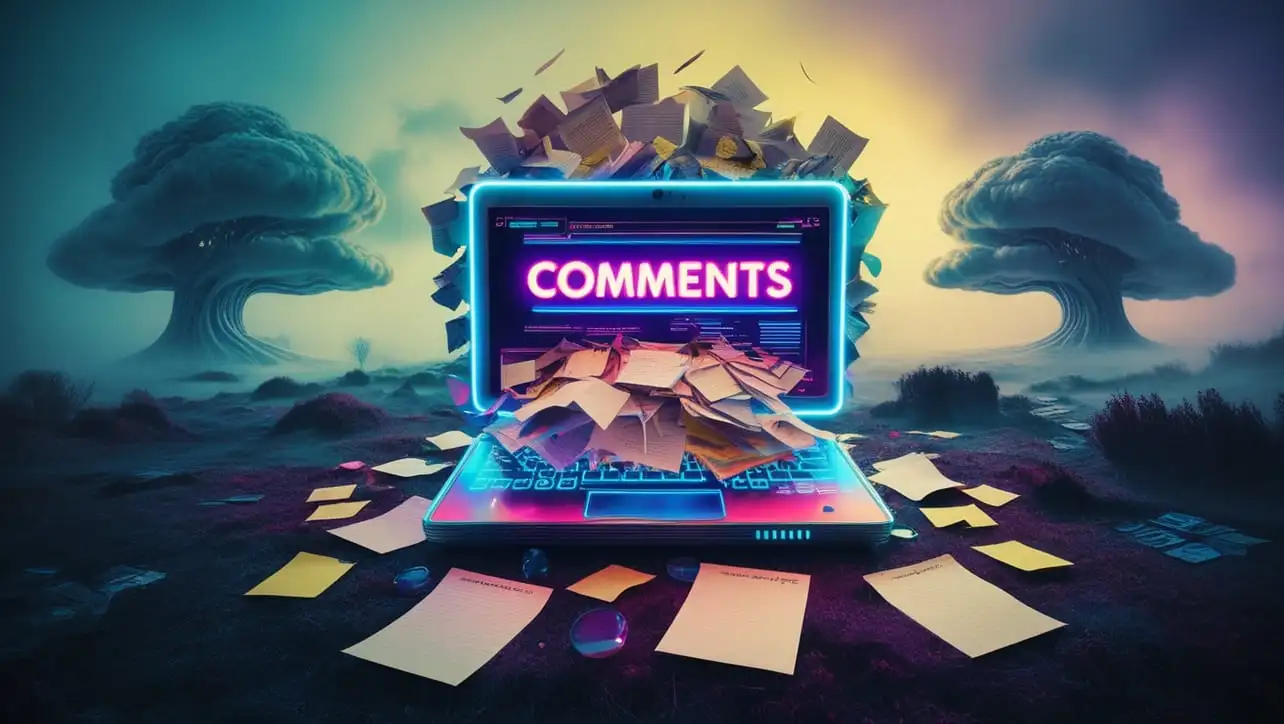
JSON.stringify()
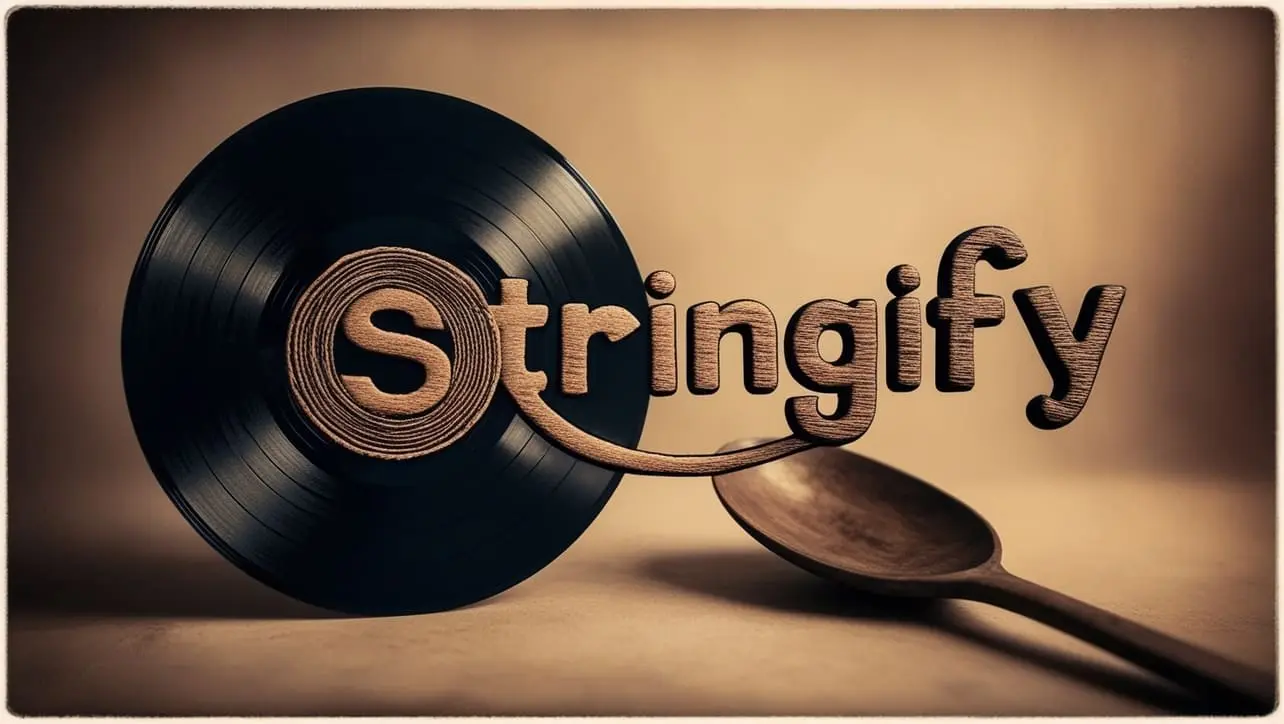
Photo Credit to CodeToFun
π Introduction
JavaScript Object Notation (JSON) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate.
One of the key methods in JavaScript for working with JSON is JSON.stringify()
. This method converts a JavaScript object or value to a JSON string.
π€ What is JSON.stringify()?
The JSON.stringify()
method converts a JavaScript object or value to a JSON string. This is particularly useful for sending data over a network or for storing data in a format that can easily be parsed later.
π‘ Syntax
JSON.stringify(value, replacer, space)
- value: The value to convert to a JSON string.
- replacer (optional): A function that alters the behavior of the stringification process, or an array of strings and numbers that acts as a whitelist for selecting the properties of the object to be included in the JSON string.
- space (optional): A string or number that's used to insert white space into the output JSON string for readability purposes.
Basic Usage
Hereβs a simple example of how to use JSON.stringify()
:
const obj = { name: "John", age: 30, city: "New York" };
const jsonString = JSON.stringify(obj);
console.log(jsonString);
π» Output
In this example, JSON.stringify()
converts the JavaScript object obj into a JSON string.
{"name":"John","age":30,"city":"New York"}
Using the replacer Parameter
The replacer parameter can be a function or an array. As a function, it is called for each property of the object, and can modify or filter the properties that are included in the resulting JSON string.
Example with Function Replacer:
const obj = { name: "John", age: 30, city: "New York" };
function replacer(key, value) {
if (typeof value === "string") {
return undefined;
}
return value;
}
const jsonString = JSON.stringify(obj, replacer);
console.log(jsonString);
π» Output
In this example, the replacer function filters out properties with string values.
{"age":30}
Example with Array Replacer:
const obj = { name: "John", age: 30, city: "New York" };
const jsonString = JSON.stringify(obj, ["name", "city"]);
console.log(jsonString);
π» Output
In this example, only the name
and city
properties are included in the resulting JSON string.
{"name":"John","city":"New York"}
Using the space Parameter
The space parameter is used to add indentation, white space, and line breaks to the output JSON string for readability.
Example with Space Parameter:
const obj = { name: "John", age: 30, city: "New York" };
const jsonString = JSON.stringify(obj, null, 2);
console.log(jsonString);
π» Output
In this example, the JSON string is formatted with an indentation of 2 spaces, making it easier to read.
{ "name": "John", "age": 30, "city": "New York" }
Handling Circular References
When an object contains circular references, JSON.stringify()
will throw an error. Circular references occur when an object references itself, directly or indirectly.
const obj = {};
obj.a = obj;
try {
JSON.stringify(obj);
} catch (error) {
console.log("Error:", error.message);
}
π» Output
To handle circular references, you can use a library like circular-json or write a custom replacer function.
Error: Converting circular structure to JSON
π Conclusion
The JSON.stringify()
method is an essential tool for converting JavaScript objects into JSON strings. Understanding how to use its parameters can help you control the stringification process, ensuring that your data is properly formatted and includes only the desired properties.
Whether you're sending data over a network, storing it, or logging it for debugging, JSON.stringify()
is a versatile and powerful method to have in your JavaScript toolkit.
π¨βπ» Join our Community:
Author
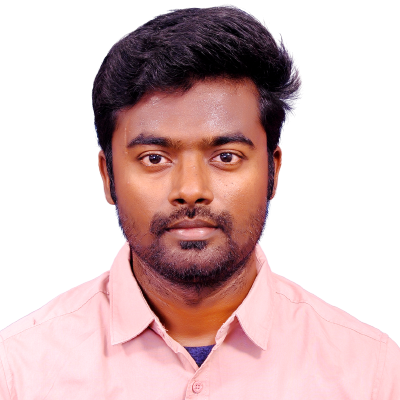
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JSON.stringify()), please comment here. I will help you immediately.