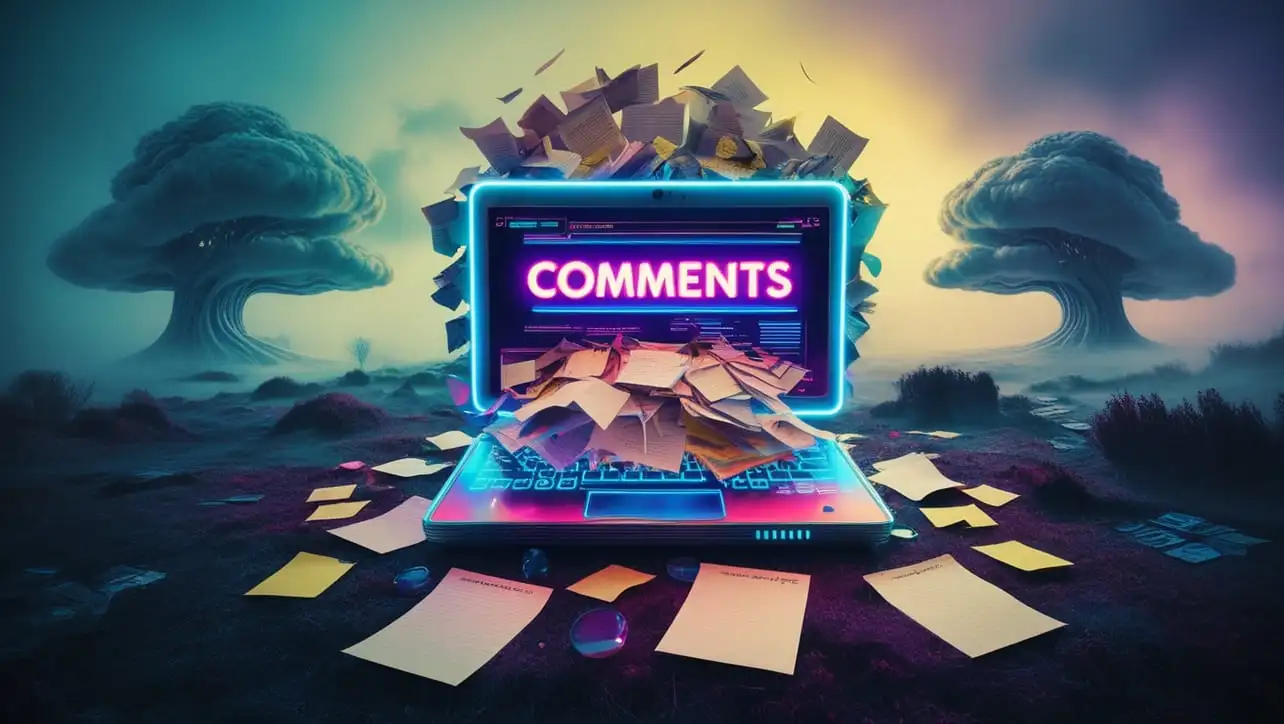
JSON.parse()
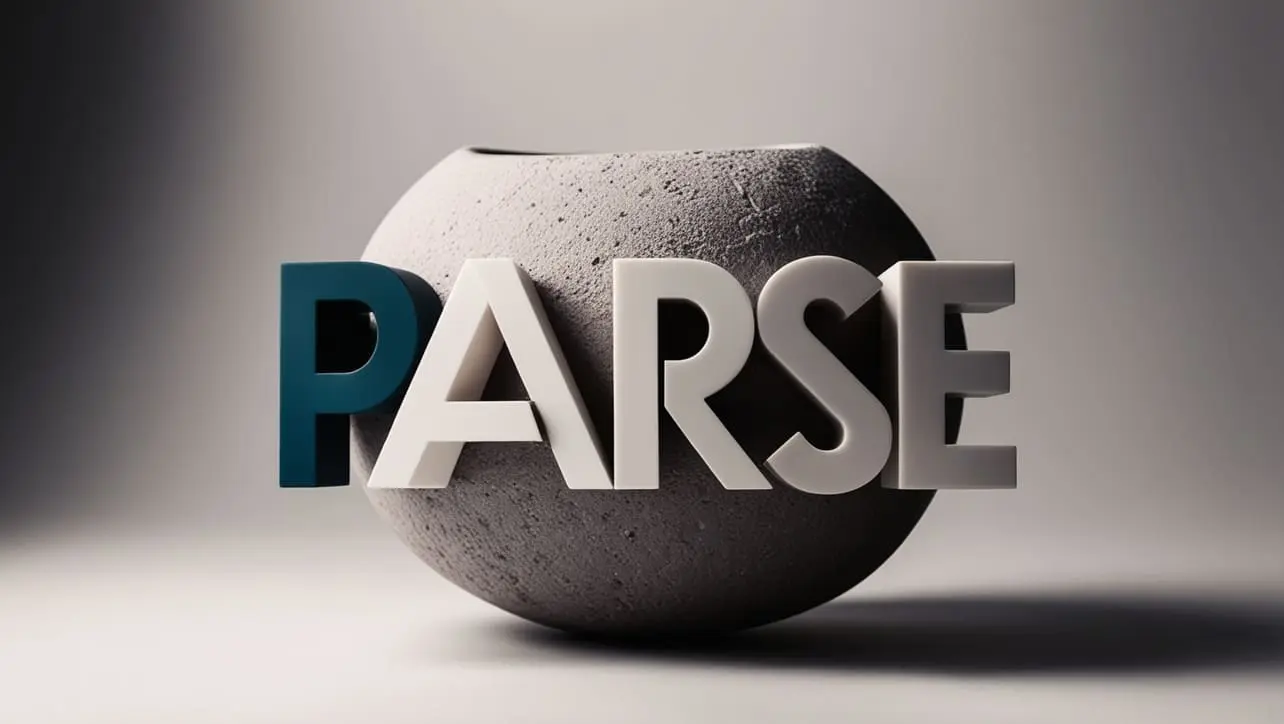
Photo Credit to CodeToFun
🙋 Introduction
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It is a text format that is language-independent but uses conventions familiar to programmers of the C family of languages, including C, C++, Java, JavaScript, Perl, Python, and many others.
🤔 What is JSON?
JSON is a format for structuring data. It is primarily used to transmit data between a server and a web application as text. JSON is built on two structures:
- A collection of name/value pairs: In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
- An ordered list of values: In most languages, this is realized as an array, vector, list, or sequence.
Example of JSON
Here is an example of a simple JSON object:
{
"name": "John Doe",
"age": 30,
"isStudent": false,
"courses": ["Math", "Science", "History"],
"address": {
"street": "123 Main St",
"city": "Anytown",
"zipcode": "12345"
}
}
In this example, the JSON object contains various data types including strings, numbers, booleans, arrays, and other objects.
JSON.parse()
The JSON.parse()
method in JavaScript is used to parse a JSON string and convert it into a JavaScript object. This is particularly useful when you receive JSON data from a web server or an API and need to work with it in your JavaScript code.
💡 Syntax
JSON.parse(text[, reviver])
- text: The string to parse as JSON. It must be a valid JSON string.
- reviver (optional): A function that can transform the results. This function is called for each (key, value) pair and can manipulate the value before it is returned.
Basic Example
Here is a basic example of using JSON.parse()
:
const jsonString = '{"name": "Jane Doe", "age": 25, "isStudent": true}';
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name); // Output: Jane Doe
console.log(jsonObject.age); // Output: 25
console.log(jsonObject.isStudent); // Output: true
In this example, a JSON string is converted into a JavaScript object, and its properties are accessed using dot notation.
Using a Reviver Function
The reviver function allows for custom transformation during the parsing process. Here is an example:
const jsonString = '{"name": "Jane Doe", "birthDate": "1990-01-01T00:00:00Z"}';
const jsonObject = JSON.parse(jsonString, (key, value) => {
if (key === "birthDate") {
return new Date(value);
}
return value;
});
console.log(jsonObject.birthDate instanceof Date); // Output: true
console.log(jsonObject.birthDate); // Output: Mon Jan 01 1990 01:00:00 GMT+0100 (Central European Standard Time)
In this example, the reviver function converts the birthDate string into a JavaScript Date object.
Error Handling
When parsing JSON, errors can occur if the input string is not valid JSON. The JSON.parse()
method throws a SyntaxError exception if the string to parse is not valid JSON. Here is an example of handling errors:
const jsonString = '{"name": "John Doe", "age": 30'; // Missing closing brace
try {
const jsonObject = JSON.parse(jsonString);
} catch (e) {
console.error("Parsing error:", e.message); // Output: Parsing error: Unexpected end of JSON input
}
In this example, an error is caught and handled gracefully, avoiding the script from crashing.
🎉 Conclusion
JSON.parse()
is a powerful method for converting JSON strings into JavaScript objects. Understanding how to use JSON.parse()
, including the optional reviver function and error handling, can greatly enhance your ability to work with JSON data in web development.
JSON remains an essential tool for modern web applications, enabling efficient data interchange between servers and clients.
👨💻 Join our Community:
Author
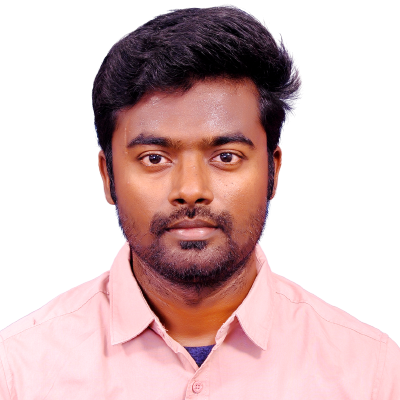
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JSON.parse()), please comment here. I will help you immediately.