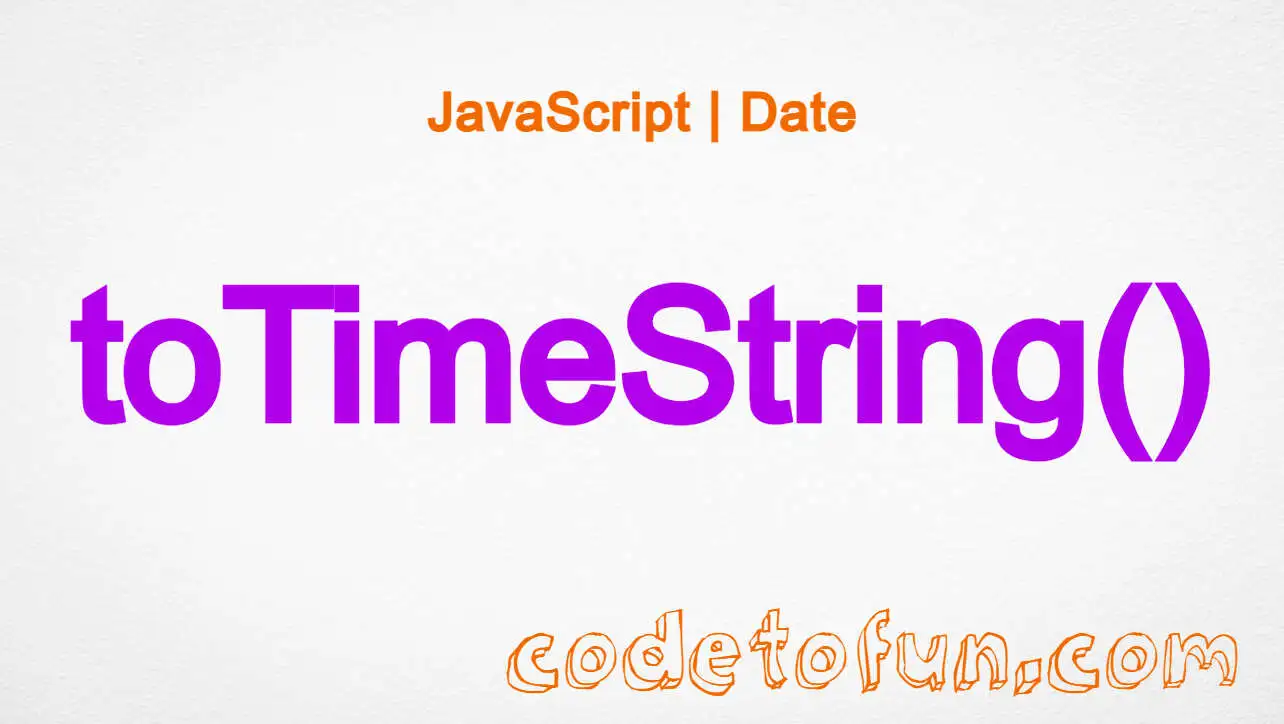
JS Window Methods
JavaScript Window prompt() Method
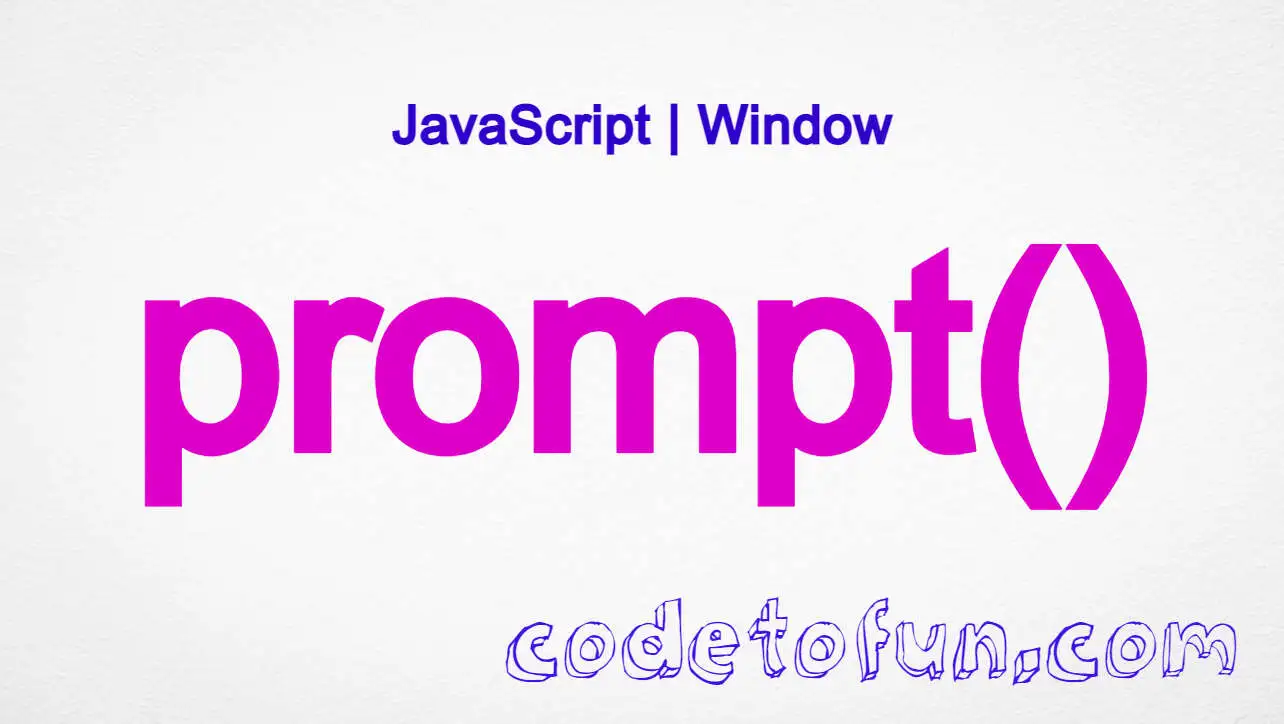
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, user interaction is crucial for creating dynamic and engaging applications. The prompt()
method is a handy feature that allows you to obtain user input through a simple dialog box.
In this guide, we'll explore the prompt()
method, understand its syntax, explore example usage, and discuss best practices and use cases.
🧠 Understanding prompt() Method
The prompt()
method is part of the Window interface in JavaScript and is used to display a dialog box that prompts the user for input. It typically provides a message to the user, an input field, and buttons for "OK" and "Cancel."
💡 Syntax
The syntax for the prompt()
method is straightforward:
window.prompt(message, defaultText);
- message: A string of text that will be displayed as the message in the dialog box.
- defaultText (Optional): A default value that will be pre-filled in the input field.
📝 Example
Let's dive into a simple example to illustrate the usage of the prompt()
method:
// Prompting the user for their name
const userName = prompt('Please enter your name:', 'John Doe');
// Displaying the entered name
alert(`Hello, ${userName}!`);
In this example, the prompt()
method is used to ask the user for their name, and the entered name is then displayed using an alert().
🏆 Best Practices
When working with the prompt()
method, consider the following best practices:
Handle User Cancelation:
Check if the user clicked "Cancel" and handle the scenario accordingly.
example.jsCopiedconst userInput = prompt('Enter something:'); if (userInput === null) { console.log('User canceled the prompt.'); } else { console.log(`User entered: ${userInput}`); }
Sanitize and Validate Input:
Ensure that the user input is sanitized and validated based on your application's requirements.
example.jsCopiedlet age; do { age = prompt('Please enter your age:'); } while (isNaN(age) || age <= 0); console.log(`Valid age entered: ${age}`);
📚 Use Cases
Simple Information Gathering:
The
prompt()
method is commonly used for gathering simple information from users, such as their name or age.example.jsCopiedconst favoriteColor = prompt('What is your favorite color?', 'Blue'); console.log(`Your favorite color is: ${favoriteColor}`);
Numeric Input Validation:
You can use the
prompt()
method to ensure that the user enters a valid numeric value.example.jsCopiedlet userNumber; do { userNumber = prompt('Enter a positive number:'); } while (isNaN(userNumber) || userNumber <= 0); console.log(`Valid positive number entered: ${userNumber}`);
🎉 Conclusion
The prompt()
method is a valuable tool for capturing user input in a straightforward manner.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the prompt()
method in your JavaScript projects.
👨💻 Join our Community:
Author
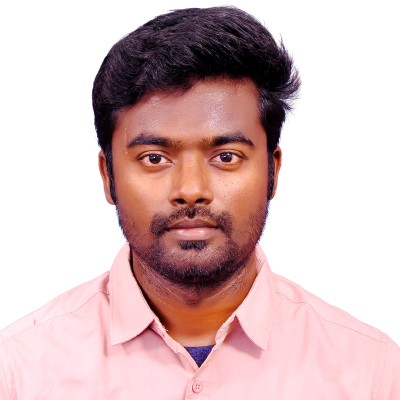
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Window prompt() Method), please comment here. I will help you immediately.