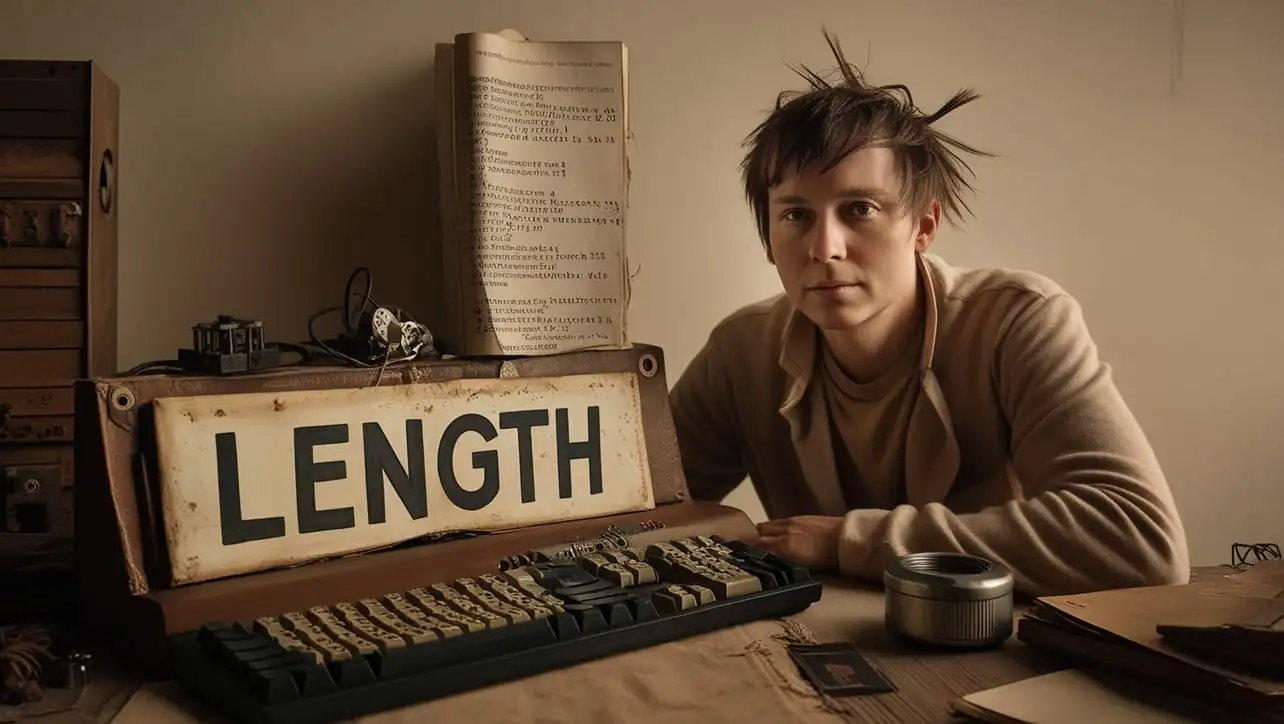
JS String Properties
JavaScript String prototype
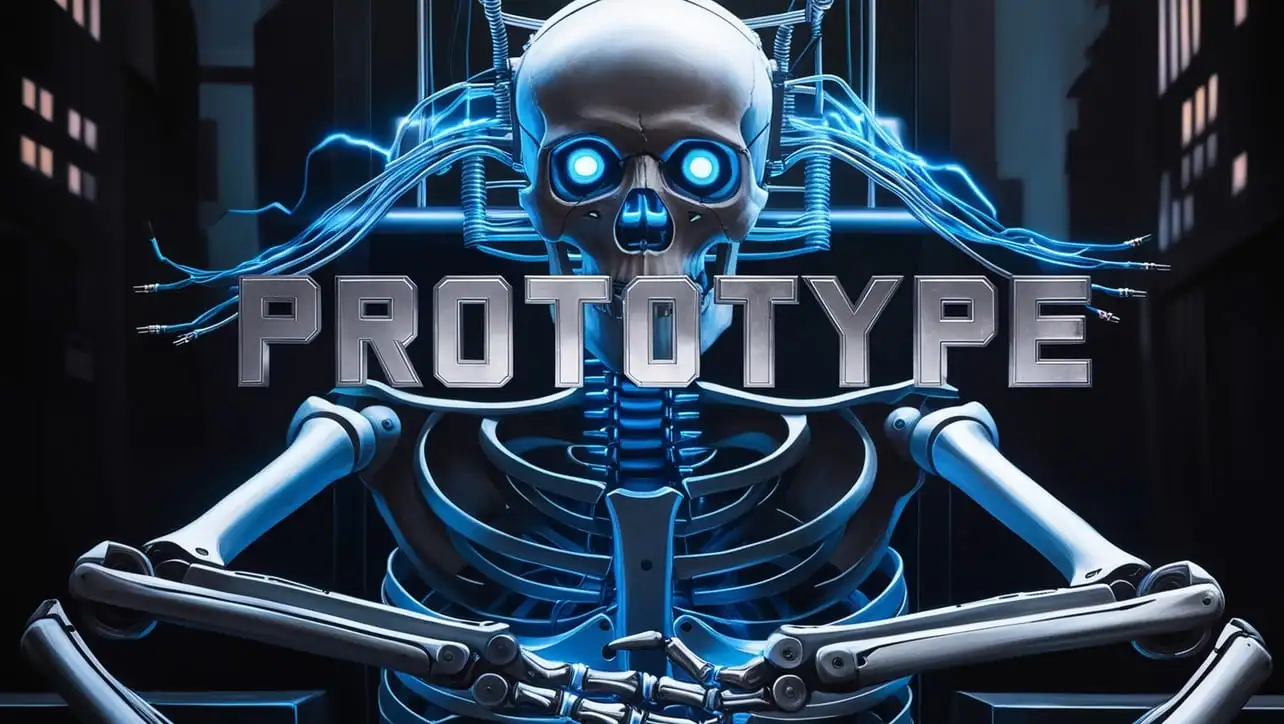
Photo Credit to CodeToFun
Introduction
In JavaScript, strings are immutable sequences of characters, and they come with several built-in methods for manipulation. These methods are stored on the String.prototype
object, allowing all string instances to inherit them. This feature provides a powerful mechanism for performing common string operations.
What Is String Prototype?
The String.prototype
object in JavaScript represents the prototype for the String
constructor. This means every string instance, whether created using string literals or the String
constructor, inherits methods and properties from String.prototype
. These methods allow developers to manipulate string values in various ways, such as searching, modifying, or formatting text.
Common String Prototype Methods
Here are some of the most commonly used methods inherited from String.prototype
:
charAt():
Returns the character at a specified index.
JavaScriptCopiedconst str = "Hello"; console.log(str.charAt(0)); // H
includes():
Checks if a string contains a substring.
JavaScriptCopiedconst sentence = "The quick brown fox"; console.log(sentence.includes("quick")); // true
indexOf():
Returns the index of the first occurrence of a substring.
JavaScriptCopiedconst text = "JavaScript is great!"; console.log(text.indexOf("great")); // 15
slice():
Extracts a section of a string and returns it as a new string.
JavaScriptCopiedconst phrase = "JavaScript"; console.log(phrase.slice(0, 4)); // "Java"
toUpperCase() and toLowerCase():
Converts the string to uppercase or lowercase.
JavaScriptCopiedconst greeting = "Hello World"; console.log(greeting.toUpperCase()); // "HELLO WORLD" console.log(greeting.toLowerCase()); // "hello world"
Extending String Prototype
You can extend String.prototype
by adding your own methods. While this can be useful, it's often discouraged because it can cause conflicts if other libraries define methods with the same names.
String.prototype.reverse = function() {
return this.split('').reverse().join('');
};
console.log("hello".reverse()); // "olleh"
Built-in Methods
Here’s a brief overview of some other useful built-in methods:
replace():
Replaces part of a string with another string.
JavaScriptCopiedconst text = "I like apples"; console.log(text.replace("apples", "oranges")); // "I like oranges"
split():
Splits a string into an array of substrings.
JavaScriptCopiedconst sentence = "JavaScript is awesome"; console.log(sentence.split(" ")); // ["JavaScript", "is", "awesome"]
trim():
Removes whitespace from both ends of a string.
JavaScriptCopiedconst spaced = " Hello "; console.log(spaced.trim()); // "Hello"
Handling Edge Cases
When using string prototype methods, there are some edge cases to be aware of:
Empty Strings:
Methods like
charAt()
orslice()
will return empty strings when the string is empty.JavaScriptCopiedconst empty = ""; console.log(empty.charAt(0)); // ""
Case Sensitivity:
Methods like
includes()
andindexOf()
are case-sensitive.JavaScriptCopiedconst str = "Hello World"; console.log(str.includes("hello")); // false
Best Practices
- Avoid Modifying the Prototype: While it's possible to add methods to
String.prototype
, it's considered bad practice unless necessary, as it can lead to code conflicts and unexpected behavior. - Use Built-in Methods: Always prefer using native JavaScript string methods, as they are optimized for performance and reliability.
- Handling Case Sensitivity: When working with strings where case sensitivity might be an issue, consider converting strings to a uniform case using
toLowerCase()
ortoUpperCase()
.
Example
Here's a simple example showing how to use some of the common String.prototype
methods together:
const sentence = " JavaScript is Fun! ";
// Trim whitespace
const trimmed = sentence.trim();
// Convert to lowercase
const lower = trimmed.toLowerCase();
// Check if it includes the word 'fun'
const hasFun = lower.includes('fun');
console.log(`Is fun in the sentence? ${hasFun}`); // true
Conclusion
JavaScript’s String.prototype
provides a variety of powerful methods for working with strings. Whether you're modifying, searching, or formatting strings, these built-in methods simplify many common operations. However, use caution when extending the prototype to avoid potential conflicts in your code.
👨💻 Join our Community:
Author
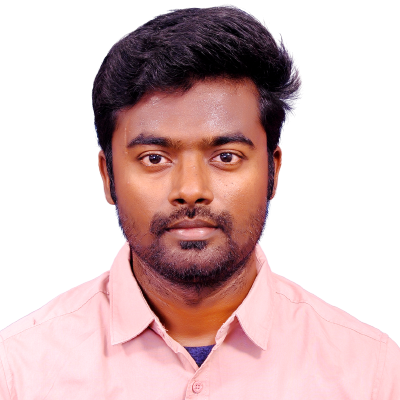
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript String prototype), please comment here. I will help you immediately.