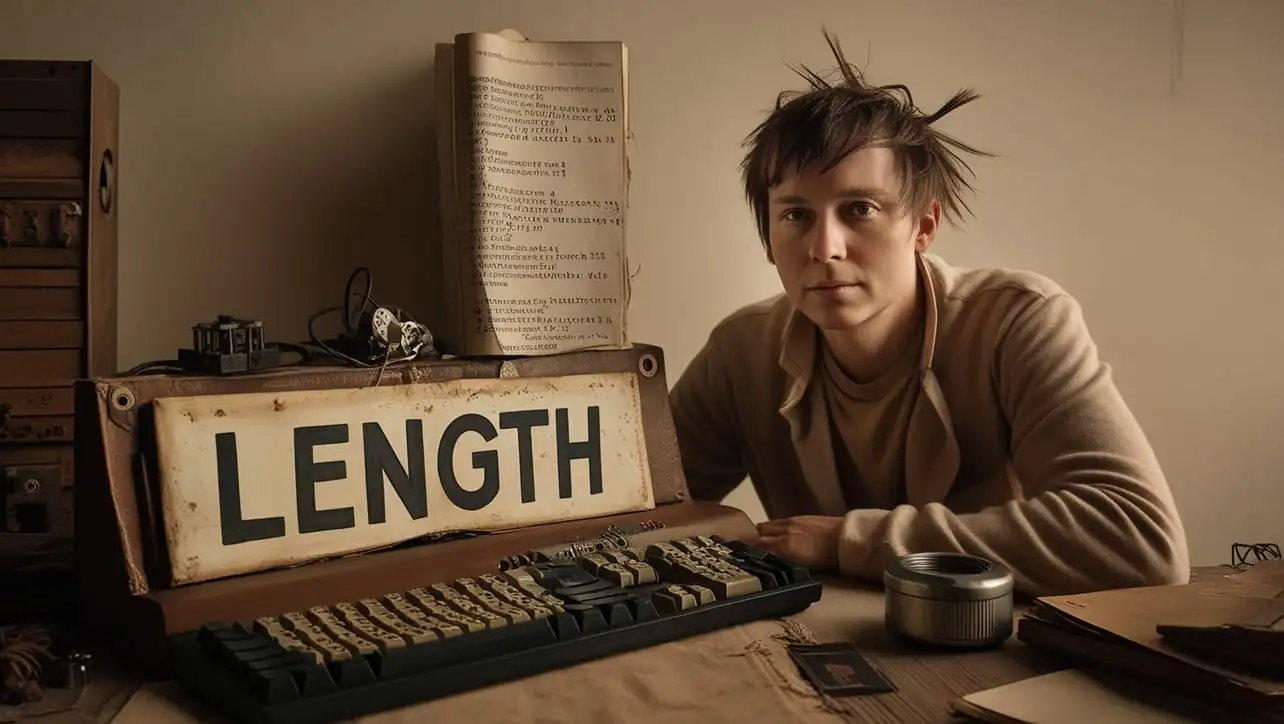
JavaScript String constructor
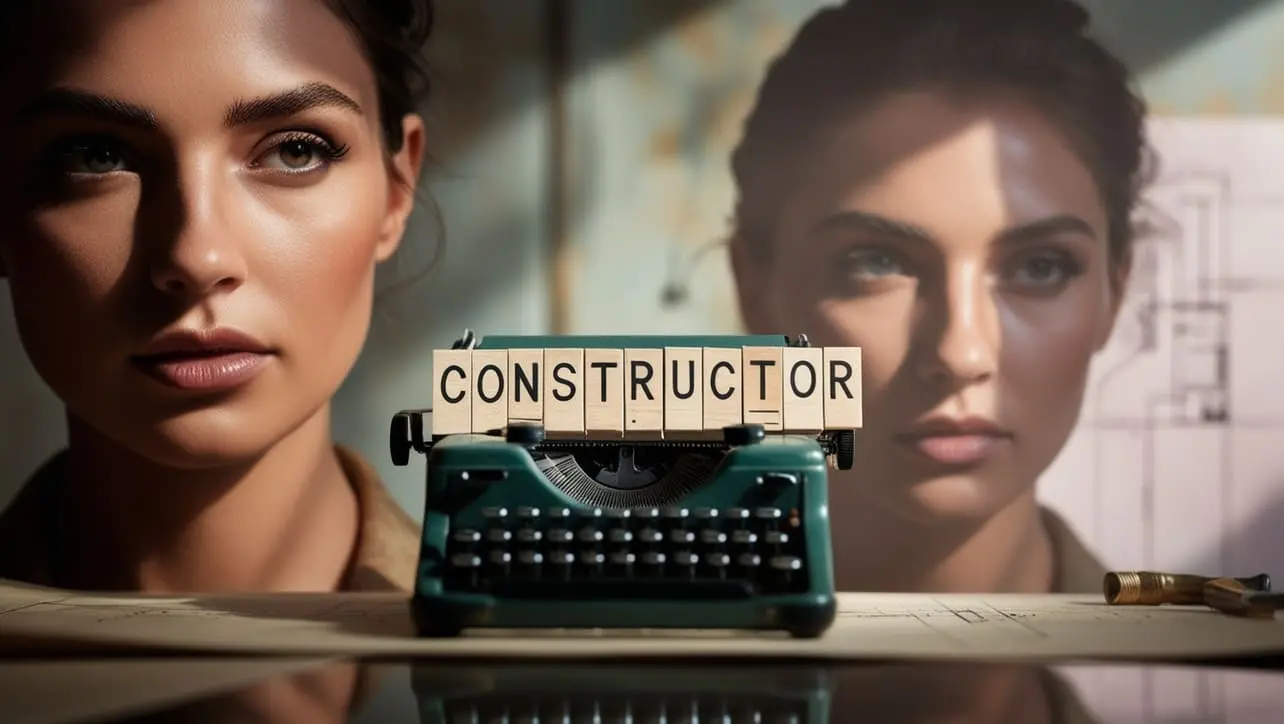
Photo Credit to CodeToFun
Introduction
The JavaScript String
constructor is a built-in function used to create and manipulate strings. It can be used to create new string objects and convert different data types into strings.
Understanding the String
constructor and how it differs from string literals is key for managing string values effectively in JavaScript.
What is the String Constructor?
The String
constructor in JavaScript is a global object used to create string objects. Unlike string literals, which are primitive data types, strings created using the String
constructor are objects and have access to additional properties and methods.
String Constructor vs. String Literals
String literals are created by enclosing text within single or double quotes:
let strLiteral = "Hello, World!";
Using the String
constructor creates a string object:
let strObject = new String("Hello, World!");
While both represent text, strObject
is an instance of the String
object, whereas strLiteral
is a primitive string.
Creating String Objects
To create a new string object, you can use the String
constructor with the new
keyword:
let myString = new String("Hello");
console.log(myString); // String {"Hello"}
Or, without new
, which returns a string primitive:
let myString = String(42);
console.log(typeof myString); // "string"
Converting Data Types to Strings
The String
constructor can convert various data types into strings:
let num = 123;
let strNum = String(num); // Converts number to string "123"
let bool = true;
let strBool = String(bool); // Converts boolean to string "true"
This is useful when you need to perform string operations on non-string data types.
Using String Properties and Methods
Strings created with the String
constructor inherit properties and methods from the String
prototype. Some commonly used methods include:
length:
Returns the length of the string.
JavaScriptCopiedlet str = new String("JavaScript"); console.log(str.length); // 10
toUpperCase():
Converts the string to uppercase.
JavaScriptCopiedconsole.log(str.toUpperCase()); // "JAVASCRIPT"
charAt():
Returns the character at the specified index.
JavaScriptCopiedconsole.log(str.charAt(4)); // "S"
Common Pitfalls
Object vs. Primitive:
When using the
String
constructor withnew
, you create a string object instead of a primitive string. This can lead to unexpected behavior when comparing strings.JavaScriptCopiedlet str1 = new String("test"); let str2 = "test"; console.log(str1 === str2); // false
Performance:
Creating string objects with the
String
constructor is less efficient than using string literals. Use string literals when you don’t need an object.Type Coercion:
When using
String
withoutnew
, it can cause implicit type conversion that may lead to bugs if not carefully managed.
Example
Here’s an example illustrating the creation and use of the String
constructor:
// Create a string object
let greeting = new String("Hello, World!");
// Access length property
console.log("Length:", greeting.length);
// Use toLowerCase method
console.log("Lowercase:", greeting.toLowerCase());
// Compare with primitive string
let primitiveGreeting = "Hello, World!";
console.log(greeting == primitiveGreeting); // true
console.log(greeting === primitiveGreeting); // false
Conclusion
The JavaScript String
constructor is a versatile tool for working with string data. While string literals are often sufficient for most cases, the String
constructor provides additional flexibility when working with strings as objects. Understanding the differences between string objects and string primitives can help you write more efficient and accurate code.
Join our Community:
Author
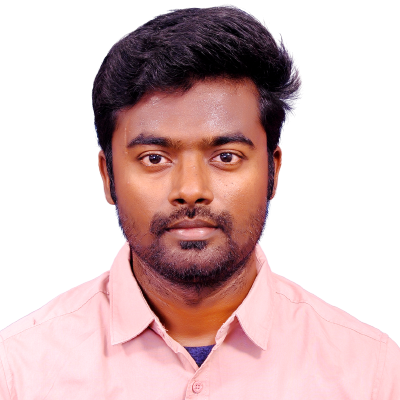
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript String constructor), please comment here. I will help you immediately.