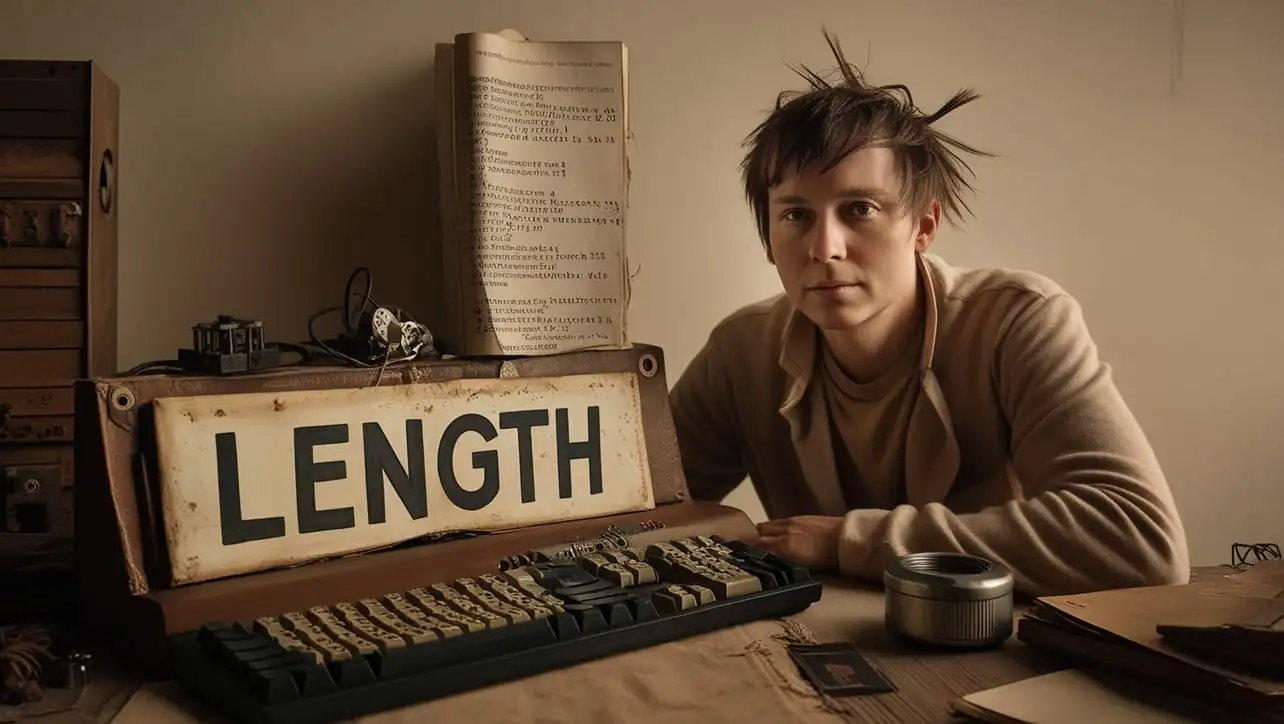
JS Basic
JS Interview Programs
- JS Interview Programs
- JS Abundant Number
- JS Amicable Number
- JS Armstrong Number
- JS Average of N Numbers
- JS Automorphic Number
- JS Biggest of three numbers
- JS Binary to Decimal
- JS Common Divisors
- JS Composite Number
- JS Condense a Number
- JS Cube Number
- JS Decimal to Binary
- JS Decimal to Octal
- JS Disarium Number
- JS Even Number
- JS Evil Number
- JS Factorial of a Number
- JS Fibonacci Series
- JS GCD
- JS Happy Number
- JS Harshad Number
- JS LCM
- JS Leap Year
- JS Magic Number
- JS Matrix Addition
- JS Matrix Division
- JS Matrix Multiplication
- JS Matrix Subtraction
- JS Matrix Transpose
- JS Maximum Value of an Array
- JS Minimum Value of an Array
- JS Multiplication Table
- JS Natural Number
- JS Number Combination
- JS Odd Number
- JS Palindrome Number
- JS Pascalβs Triangle
- JS Perfect Number
- JS Perfect Square
- JS Power of 2
- JS Power of 3
- JS Pronic Number
- JS Prime Factor
- JS Prime Number
- JS Smith Number
- JS Strong Number
- JS Sum of Array
- JS Sum of Digits
- JS Swap Two Numbers
- JS Triangular Number
JavaScript Program to Check Power of 3
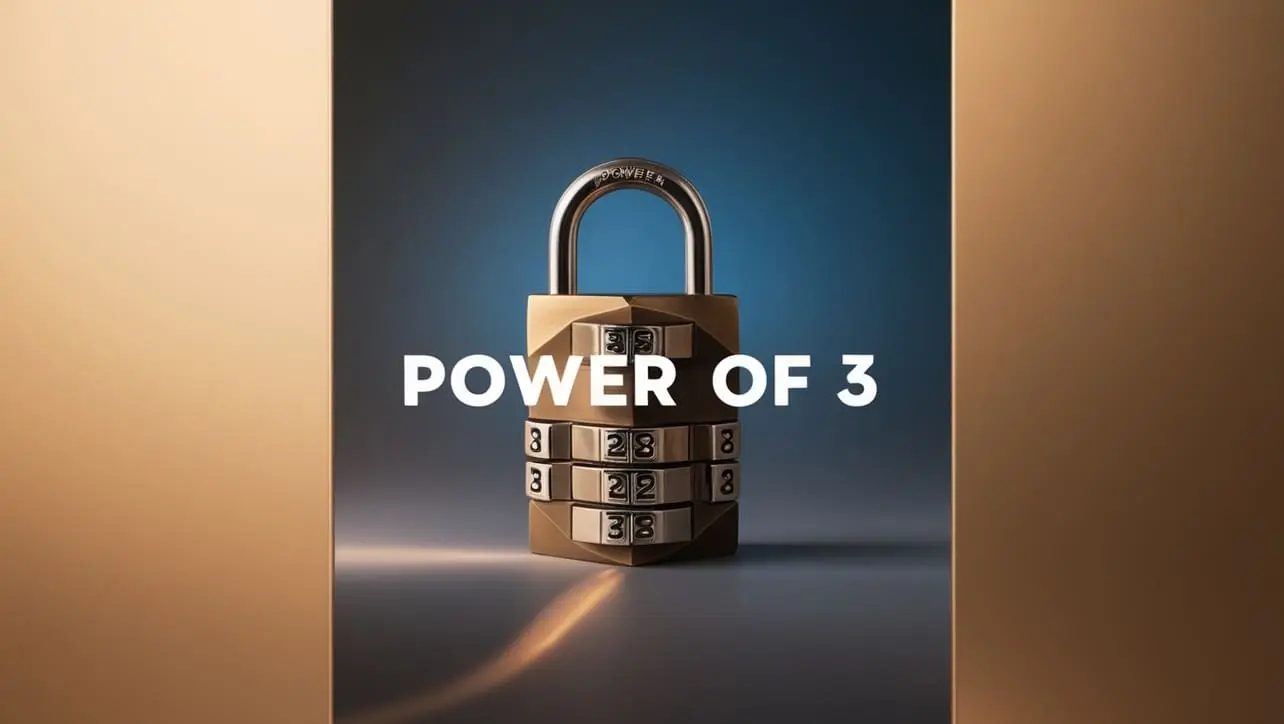
Photo Credit to CodeToFun
π Introduction
In the realm of programming, one often encounters the need to check if a given number is a power of another.
In this context, we'll explore a JavaScript program designed specifically to check if a number is a power of 3.
The logic behind this program involves repeatedly dividing the number by 3 until it becomes 1, indicating that it is a power of 3.
π Example
Let's take a look at the JavaScript code that accomplishes this functionality.
// Function to check if a number is a power of 3
function isPowerOf3(n) {
// Keep dividing the number by 3 until it is greater than 1
while (n % 3 === 0 && n > 1) {
n /= 3;
}
// If the final value is 1, the original number is a power of 3
return n === 1;
}
// Driver program
// Replace this value with your desired number
const number = 27;
// Check if the number is a power of 3
if (isPowerOf3(number)) {
console.log(`${number} is a power of 3`);
} else {
console.log(`${number} is not a power of 3`);
}
π» Testing the Program
To test the program with different numbers, simply replace the value of number in the code.
27 is a power of 3
Run the script to see if the given number is a power of 3.
π§ How the Program Works
- The program defines a function isPowerOf3 that takes a number as input and checks if it is a power of 3.
- Inside the function, it repeatedly divides the number by 3 until it becomes greater than 1.
- If the final value is 1, the original number is a power of 3, and the function returns true; otherwise, it returns false.
- The driver program tests a specific number and logs whether it is a power of 3 or not.
π Between the Given Range
Let's take a look at the javascript code that checks for powers of 3 in the given range.
// Function to check if a number is a power of 3
function isPowerOf3(num) {
while (num > 1) {
if (num % 3 !== 0) {
return false;
}
num /= 3;
}
return num === 1;
}
// Check and display the power of 3 for numbers in the range 1 to 20
let powersOf3 = [];
for (let i = 1; i <= 20; i++) {
if (isPowerOf3(i)) {
powersOf3.push(i);
}
}
// Output format: Power of 3 in the range 1 to 20: num1, num2, ...
console.log(`Power of 3 in the range 1 to 20: \n${powersOf3.join(' ')}`);
π» Testing the Program
Power of 3 in the range 1 to 20: 1 3 9
The program is designed to check powers of 3 in the range 1 to 20. To test the program, simply run it as is.
π§ How the Program Works
This version of the program uses a while loop to repeatedly divide the number by 3 until it is no longer divisible evenly. If the final result is 1, then the number is a power of 3.
π§ Understanding the Concept of Power of 3
Before diving into the code, let's understand the concept of a power of 3.
A number is considered a power of 3 if it can be expressed as 3^n, where n is an integer.
For example, 1, 3, 9, 27, and so on are powers of 3.
π’ Optimizing the Program
While the provided program is straightforward, there are alternative approaches to check if a number is a power of 3. Consider exploring other mathematical techniques for optimization.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
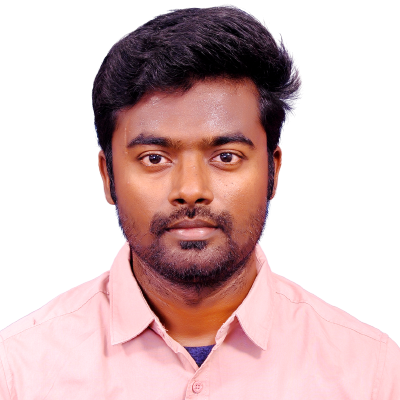
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Program to Check Power of 3), please comment here. I will help you immediately.