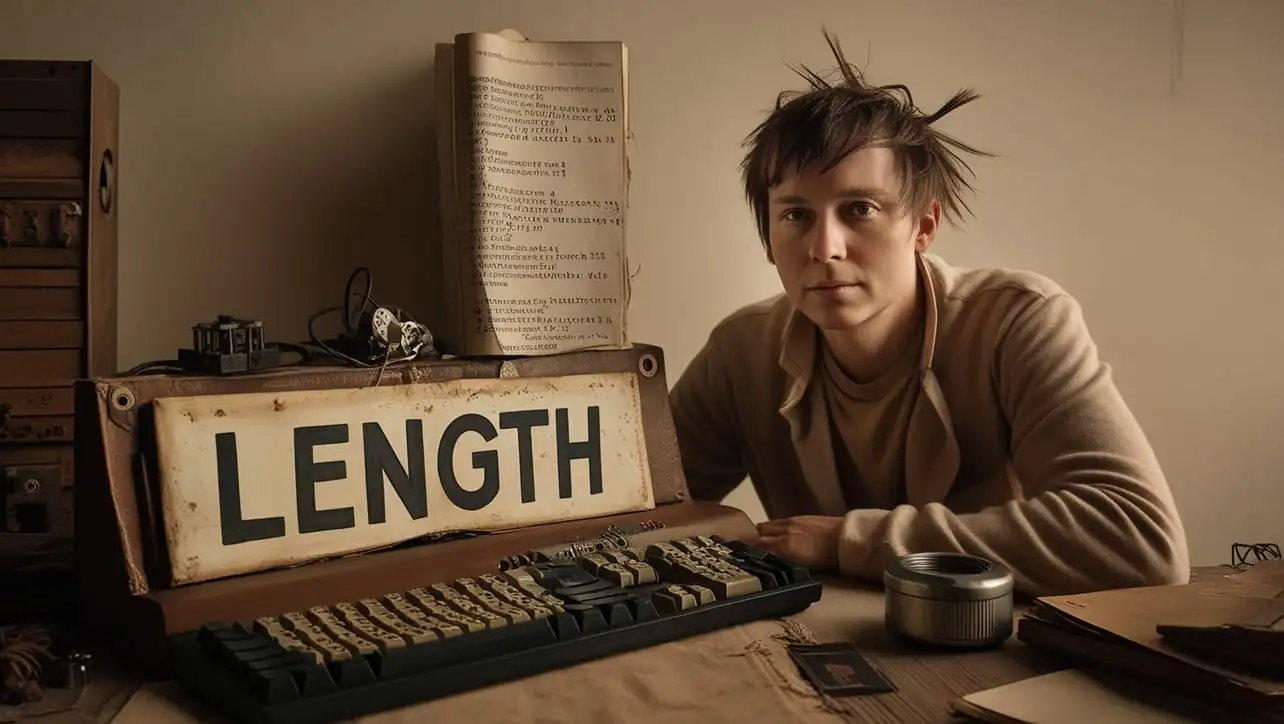
JS Date Methods
JavaScript Date toUTCString() Method
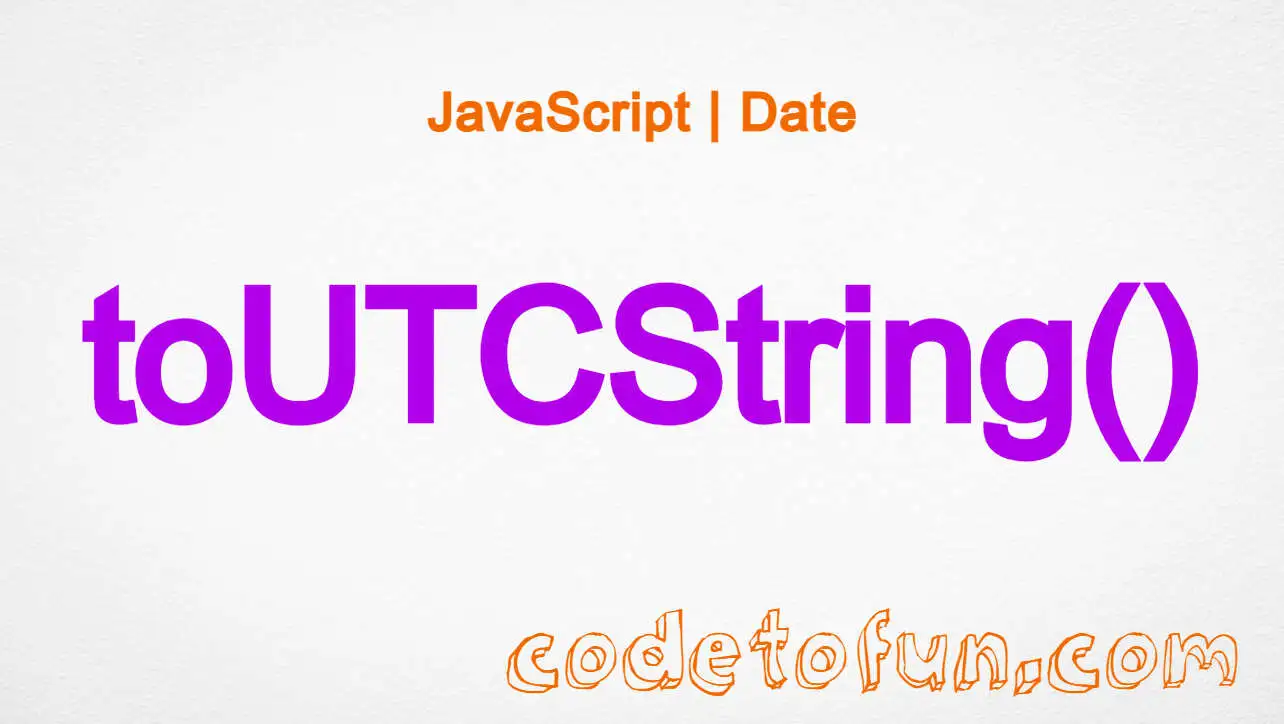
Photo Credit to CodeToFun
🙋 Introduction
Working with dates is a common task in programming, and JavaScript provides a robust Date object to handle various date-related operations. The toUTCString()
method is a valuable addition to the Date object, offering a convenient way to obtain a string representation of a date in Coordinated Universal Time (UTC).
In this guide, we'll delve into the toUTCString()
method, exploring its syntax, usage, best practices, and practical scenarios.
🧠 Understanding toUTCString() Method
The toUTCString()
method is part of the JavaScript Date object and is used to obtain a string representation of a date in UTC. This method returns a human-readable string that conforms to the RFC 1123 date-time format.
💡 Syntax
The syntax for the toUTCString()
method is straightforward:
date.toUTCString();
- date: The Date object for which you want to obtain the UTC string representation.
📝 Example
Let's dive into a simple example to illustrate the usage of the toUTCString()
method:
// Creating a Date object for the current date and time
const currentDate = new Date();
// Using toUTCString() to obtain the UTC string representation
const utcString = currentDate.toUTCString();
console.log(utcString);
In this example, the toUTCString()
method is called on a Date object to obtain the UTC string representation of the current date and time.
🏆 Best Practices
When working with the toUTCString()
method, consider the following best practices:
Timezone Awareness:
Be aware that the
toUTCString()
method always returns the date and time in Coordinated Universal Time (UTC). If timezone information is crucial, consider using other methods like toLocaleString().example.jsCopiedconst localString = currentDate.toLocaleString(); console.log(localString);
Formatting Options:
If you require more control over the date and time format, explore other methods like Intl.DateTimeFormat or external libraries like moment.js for advanced formatting options.
example.jsCopiedconst options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric', hour: 'numeric', minute: 'numeric', second: 'numeric', timeZoneName: 'short' }; const formattedDate = new Intl.DateTimeFormat('en-US', options).format(currentDate); console.log(formattedDate);
📚 Use Cases
Logging UTC Timestamps:
The
toUTCString()
method is particularly useful when logging timestamps in a standardized format, especially in scenarios where UTC is the preferred timezone:example.jsCopiedconst eventTimestamp = new Date(); console.log(`Event occurred at: ${eventTimestamp.toUTCString()}`);
Sending UTC Dates in API Requests:
When working with APIs, especially those that expect dates in UTC format, the
toUTCString()
method simplifies the process of formatting dates for API requests:example.jsCopiedconst apiRequestData = { timestamp: currentDate.toUTCString(), // Other request data... }; sendApiRequest(apiRequestData);
🎉 Conclusion
The toUTCString()
method provides a straightforward way to obtain a string representation of a date in Coordinated Universal Time.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the toUTCString()
method in your JavaScript projects.
👨💻 Join our Community:
Author
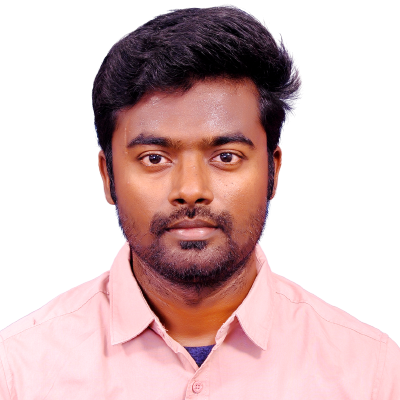
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date toUTCString() Method), please comment here. I will help you immediately.