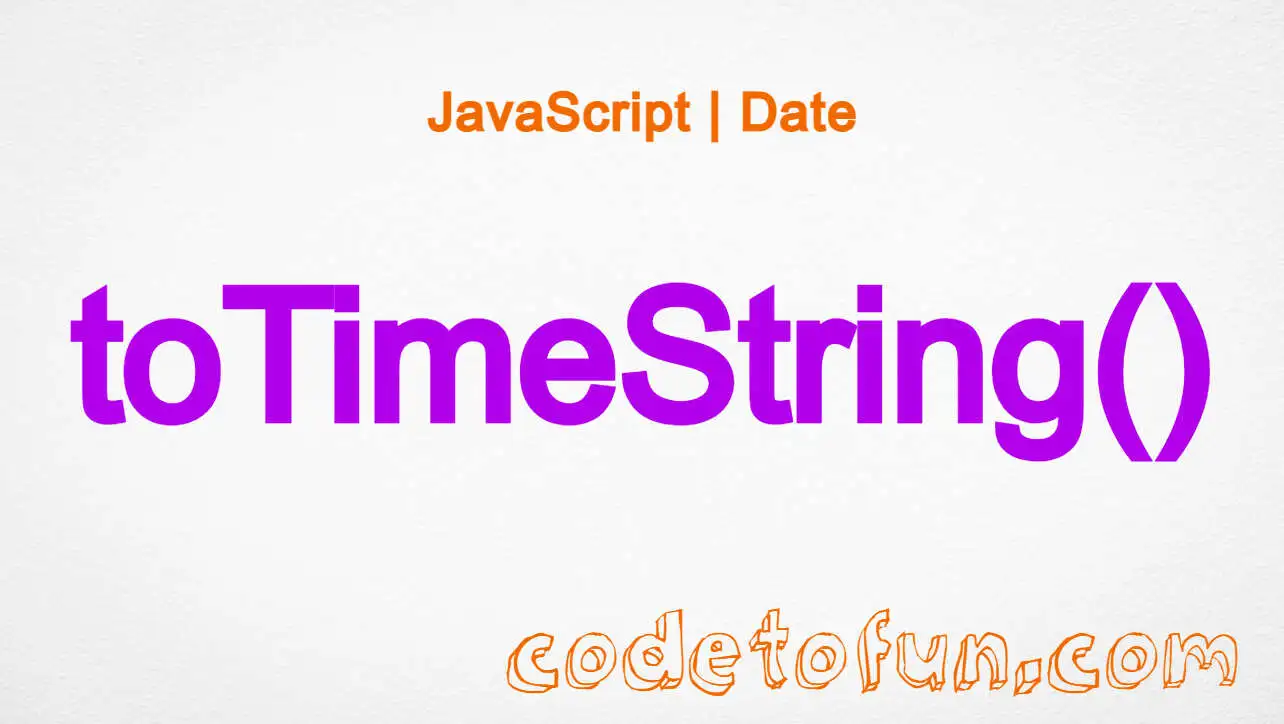
JS Date Methods
JavaScript Date setUTCMonth() Method
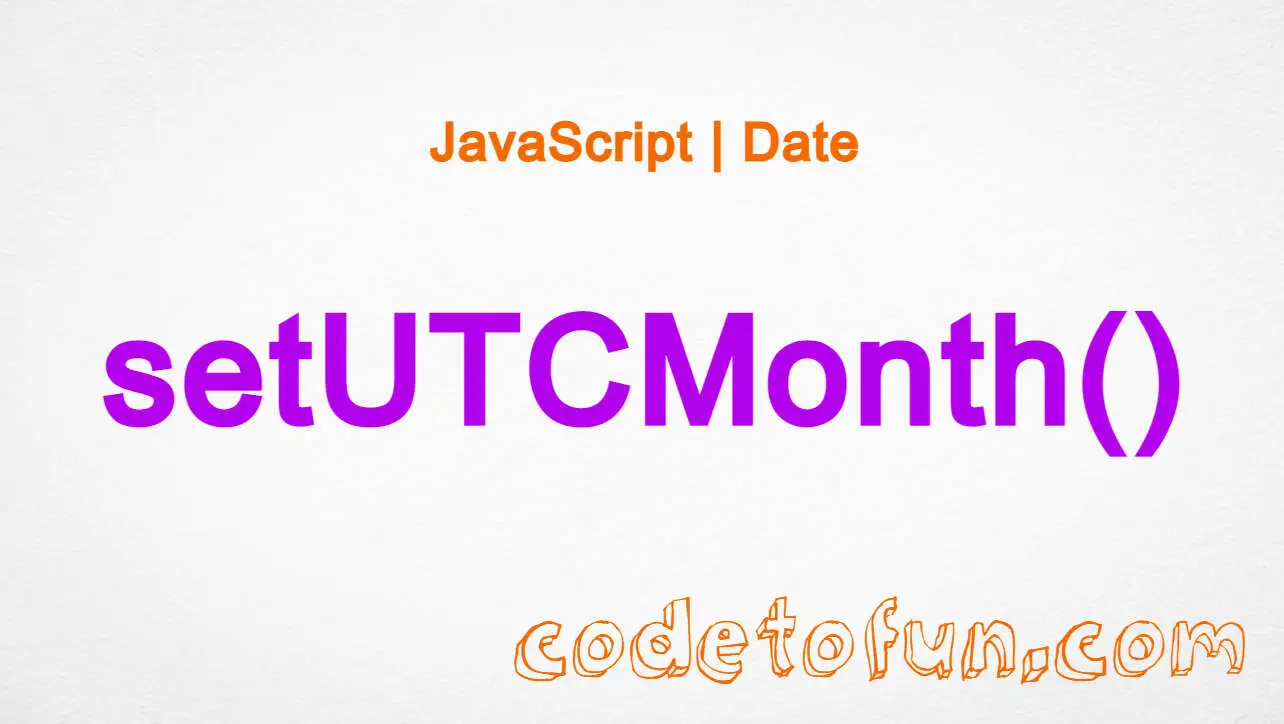
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, handling dates is a common task, and the setUTCMonth()
method provides a powerful tool for manipulating the month component of a Date object in a UTC context.
This guide will walk you through the syntax, usage, best practices, and practical examples of the setUTCMonth()
method.
🧠 Understanding setUTCMonth() Method
The setUTCMonth()
method is used to set the month for a specified Date object in Coordinated Universal Time (UTC). It allows you to modify the month while keeping the other date components unchanged.
💡 Syntax
The syntax for the setUTCMonth()
method is straightforward:
date.setUTCMonth(month[, day]);
- date: The Date object you want to modify.
- month: An integer between 0 (January) and 11 (December) representing the month.
- day (optional): An integer representing the day of the month. If not provided, the day will be set to the first day of the new month.
📝 Example
Let's explore a simple example to illustrate the usage of the setUTCMonth()
method:
// Create a Date object for March 15, 2022, in UTC
const currentDate = new Date(Date.UTC(2022, 2, 15));
// Use setUTCMonth() to change the month to May
currentDate.setUTCMonth(4);
console.log(currentDate.toUTCString());
In this example, we create a Date object for March 15, 2022, and then use setUTCMonth()
to change the month to May (remembering that months are zero-based).
🏆 Best Practices
When working with the setUTCMonth()
method, consider the following best practices:
Input Validation:
Ensure that the input values for the month and day are valid integers within the appropriate ranges.
example.jsCopiedconst newMonth = 7; if (Number.isInteger(newMonth) && newMonth >= 0 && newMonth <= 11) { currentDate.setUTCMonth(newMonth); } else { console.error('Invalid month value.'); }
Immutable Operations:
Since the
setUTCMonth()
method modifies the existing Date object, if immutability is crucial, consider creating a new Date object with the desired values.example.jsCopiedconst newDate = new Date(currentDate); newDate.setUTCMonth(6);
📚 Use Cases
Advancing Months with User Input:
The
setUTCMonth()
method is handy when advancing or moving back a certain number of months based on user input:example.jsCopiedconst monthsToAdvance = 3; currentDate.setUTCMonth(currentDate.getUTCMonth() + monthsToAdvance);
Generating Monthly Reports:
In scenarios where you need to generate monthly reports, the
setUTCMonth()
method facilitates iterating through the months:example.jsCopiedfor (let i = 0; i < 12; i++) { const reportDate = new Date(currentDate); reportDate.setUTCMonth(i); // Generate report for each month }
🎉 Conclusion
The setUTCMonth()
method empowers JavaScript developers to manipulate the month component of Date objects with precision.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setUTCMonth()
method in your JavaScript projects.
👨💻 Join our Community:
Author
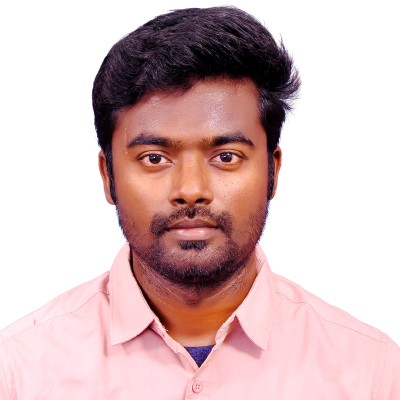
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setUTCMonth() Method), please comment here. I will help you immediately.