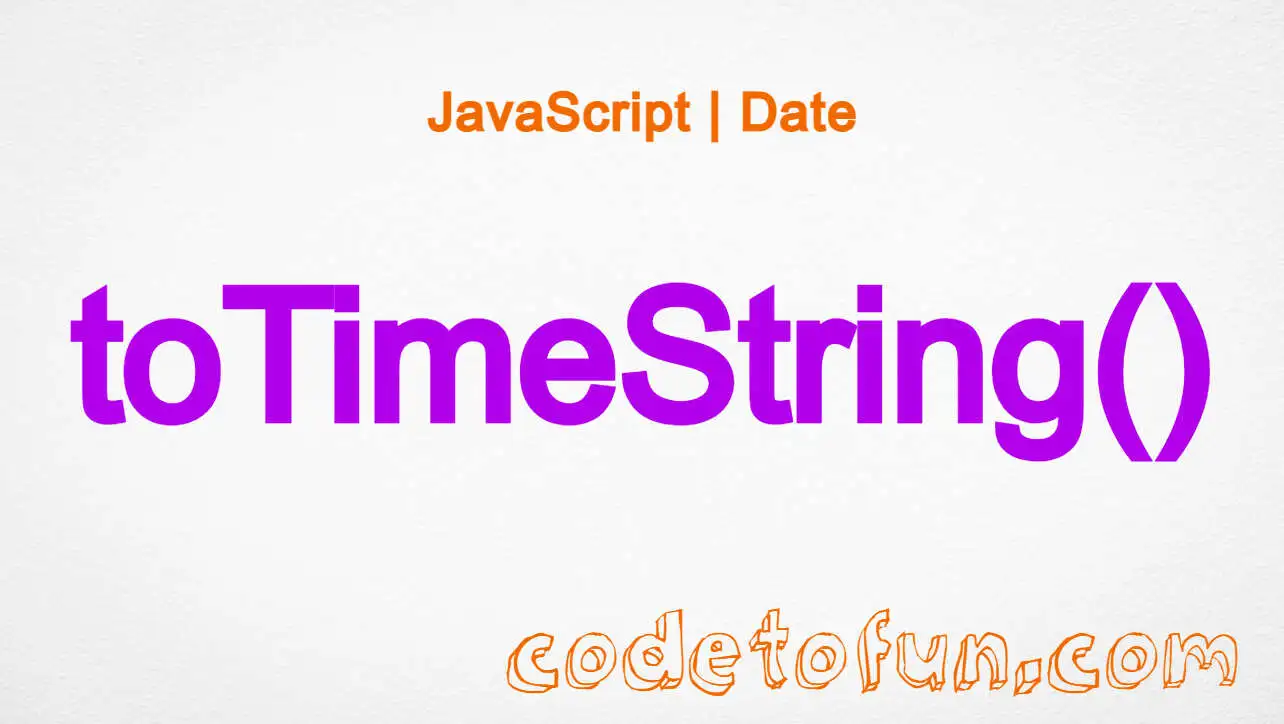
JS Date Methods
JavaScript Date setUTCMilliseconds() Method
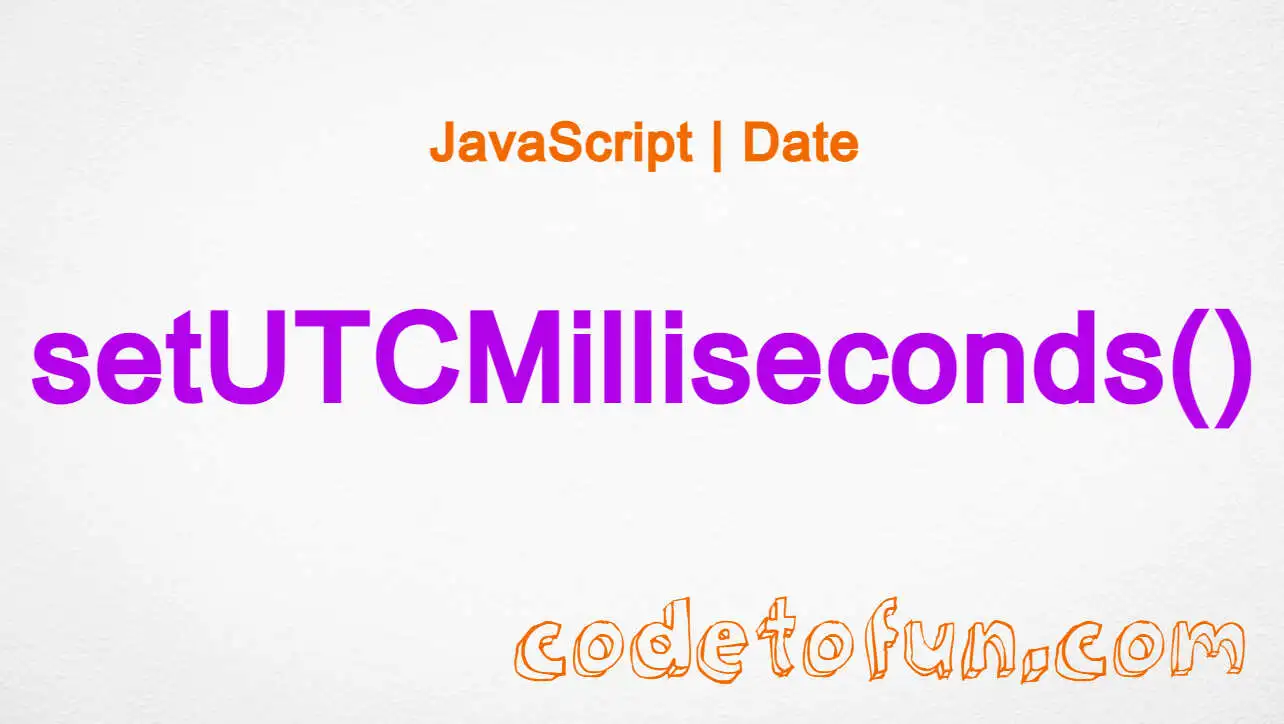
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, working with dates is a common task, and the setUTCMilliseconds()
method provides a powerful way to manipulate the milliseconds component of a Date object.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the setUTCMilliseconds()
method.
🧠 Understanding setUTCMilliseconds() Method
The setUTCMilliseconds()
method is used to set the milliseconds component of a Date object in Coordinated Universal Time (UTC). It allows for precise adjustments to the time portion, providing a granular level of control over date manipulation.
💡 Syntax
The syntax for the setUTCMilliseconds()
method is straightforward:
dateObj.setUTCMilliseconds(millisecondsValue);
- dateObj: The Date object to be modified.
- millisecondsValue: An integer representing the milliseconds to set. Should be in the range 0 to 999.
📝 Example
Let's dive into a practical example to illustrate the usage of the setUTCMilliseconds()
method:
// Create a Date object
const currentDate = new Date();
// Set milliseconds to 500
currentDate.setUTCMilliseconds(500);
console.log(currentDate.toISOString());
In this example, we create a new Date object and use setUTCMilliseconds()
to set the milliseconds to 500. The resulting date is then logged in ISO format.
🏆 Best Practices
When working with the setUTCMilliseconds()
method, consider the following best practices:
Validation:
Ensure that the provided milliseconds value is within the valid range (0 to 999) to prevent unexpected behavior.
example.jsCopiedconst newMillisecondsValue = 800; if (newMillisecondsValue >= 0 && newMillisecondsValue <= 999) { currentDate.setUTCMilliseconds(newMillisecondsValue); } else { console.error('Invalid milliseconds value.'); }
Immutable Approach:
If you need to keep the original date object unchanged, consider creating a new Date object with the modified milliseconds value.
example.jsCopiedconst modifiedDate = new Date(currentDate); modifiedDate.setUTCMilliseconds(600);
📚 Use Cases
Precise Timing in Animations:
The
setUTCMilliseconds()
method can be beneficial in scenarios where precise timing is crucial, such as controlling animation frames:example.jsCopiedfunction animateWithMilliseconds(timestamp) { const animationStartDate = new Date(2022, 0, 1); // January 1, 2022 animationStartDate.setUTCMilliseconds(timestamp % 1000); // Rest of the animation logic }
Synchronization with External Data:
When working with external data that includes milliseconds information, you can use
setUTCMilliseconds()
for synchronization:example.jsCopiedconst externalData = { timestamp: 1646071285400, // Example timestamp with milliseconds }; const syncedDate = new Date(externalData.timestamp);
🎉 Conclusion
The setUTCMilliseconds()
method empowers developers to fine-tune the time component of Date objects in UTC.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setUTCMilliseconds()
method in your JavaScript projects.
👨💻 Join our Community:
Author
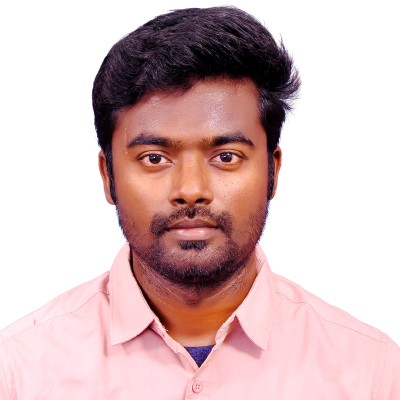
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setUTCMilliseconds() Method), please comment here. I will help you immediately.