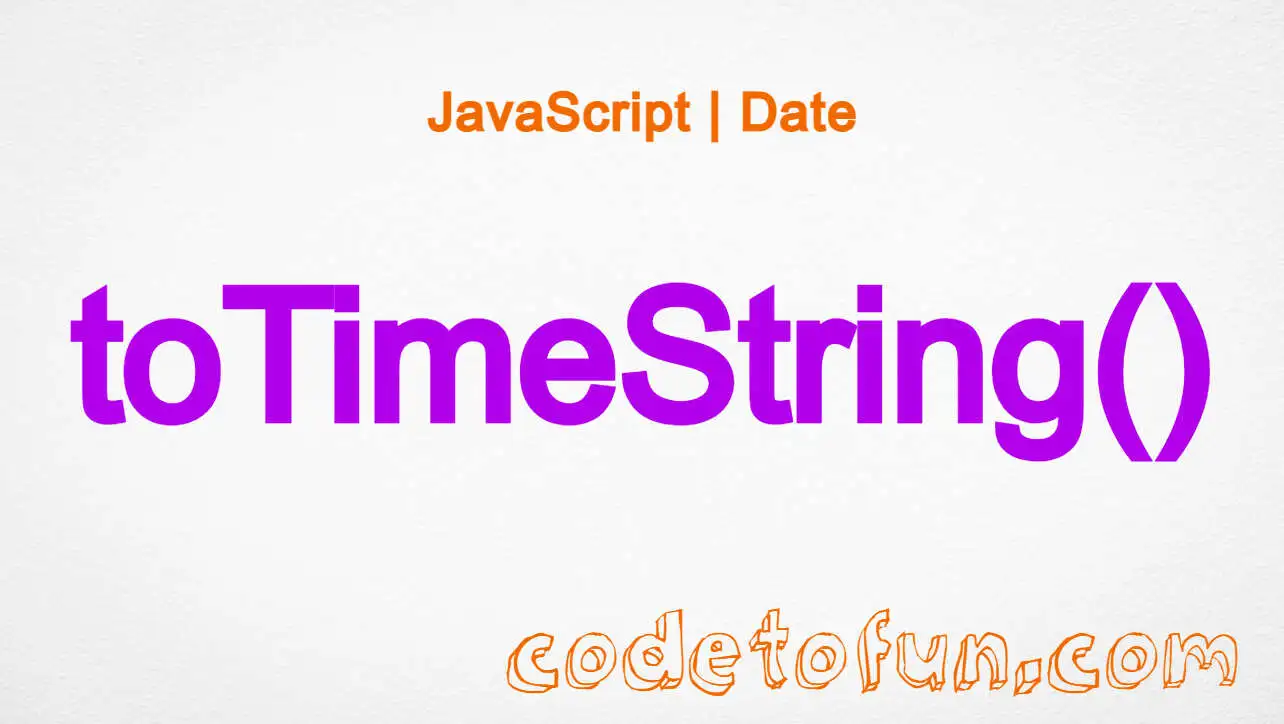
JS Date Methods
JavaScript Date setTime() Method
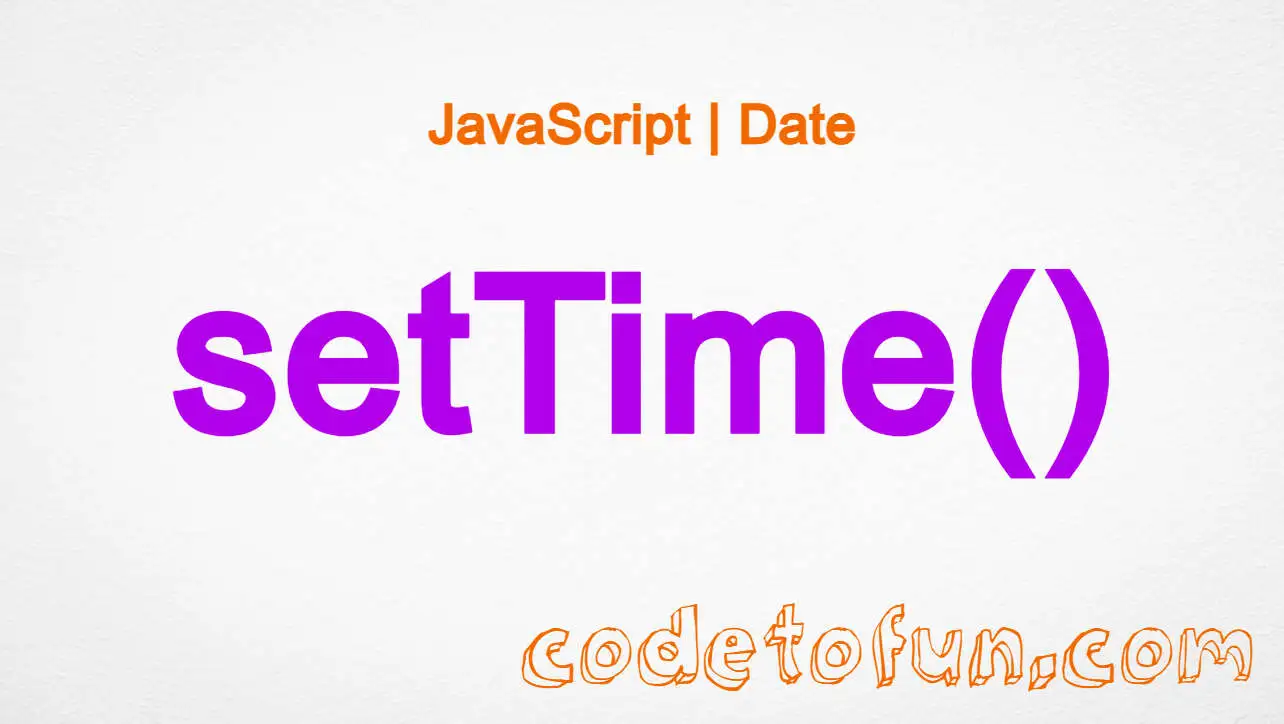
Photo Credit to CodeToFun
🙋 Introduction
Working with dates in JavaScript is a common requirement for many applications, and the setTime()
method provides a powerful way to manipulate the time value of a Date object.
In this comprehensive guide, we'll explore the syntax, usage, best practices, and practical examples of the setTime()
method to empower your date handling capabilities.
🧠 Understanding setTime() Method
The setTime()
method is part of the Date object in JavaScript and allows you to set the time value of a Date object to a specified number of milliseconds since January 1, 1970, 00:00:00 UTC (the Unix Epoch). This method is particularly useful for adjusting existing Date objects or creating new ones with a specific time.
💡 Syntax
The syntax for the setTime()
method is straightforward:
date.setTime(milliseconds);
- date: The Date object you want to modify.
- milliseconds: The numeric value representing the number of milliseconds since the Unix Epoch.
📝 Example
Let's dive into a practical example to illustrate the usage of the setTime()
method:
// Create a Date object
const myDate = new Date();
// Set the time to 24 hours later
const millisecondsInADay = 24 * 60 * 60 * 1000; // 1 day in milliseconds
const nextDay = new Date(myDate.getTime() + millisecondsInADay);
console.log(nextDay);
In this example, we use setTime()
to adjust the time value of the myDate object to be 24 hours later.
🏆 Best Practices
When working with the setTime()
method, consider the following best practices:
Immutable Approach:
Prefer creating new Date objects instead of modifying existing ones to follow an immutable approach.
example.jsCopiedconst originalDate = new Date(); const modifiedDate = new Date(originalDate.getTime() + 1000); // 1 second later
Error Handling:
Ensure that the input value for milliseconds is valid to avoid unexpected behavior.
example.jsCopiedconst invalidMilliseconds = 'invalid'; if (!isNaN(invalidMilliseconds)) { myDate.setTime(invalidMilliseconds); } else { console.error('Invalid milliseconds value.'); }
📚 Use Cases
Creating Future Dates:
The
setTime()
method is commonly used to create Date objects representing future dates:example.jsCopiedconst futureDate = new Date(); futureDate.setTime(futureDate.getTime() + (7 * 24 * 60 * 60 * 1000)); // 7 days later
Resetting Time to Midnight:
You can use
setTime()
to reset the time portion of a Date object to midnight:example.jsCopiedconst currentDate = new Date(); currentDate.setHours(0, 0, 0, 0); // Reset time to midnight
🎉 Conclusion
The setTime()
method empowers your JavaScript applications with precise control over date and time values. Whether adjusting existing Date objects or creating new ones, understanding and utilizing the setTime()
method can greatly enhance your date handling capabilities.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the setTime()
method in your JavaScript projects.
👨💻 Join our Community:
Author
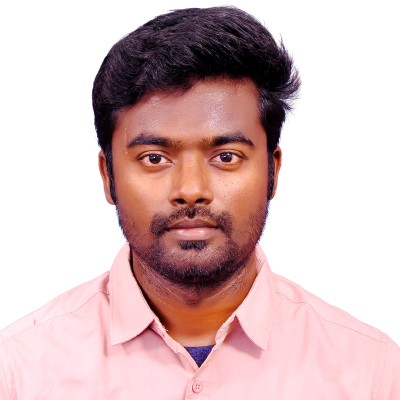
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Date setTime() Method), please comment here. I will help you immediately.