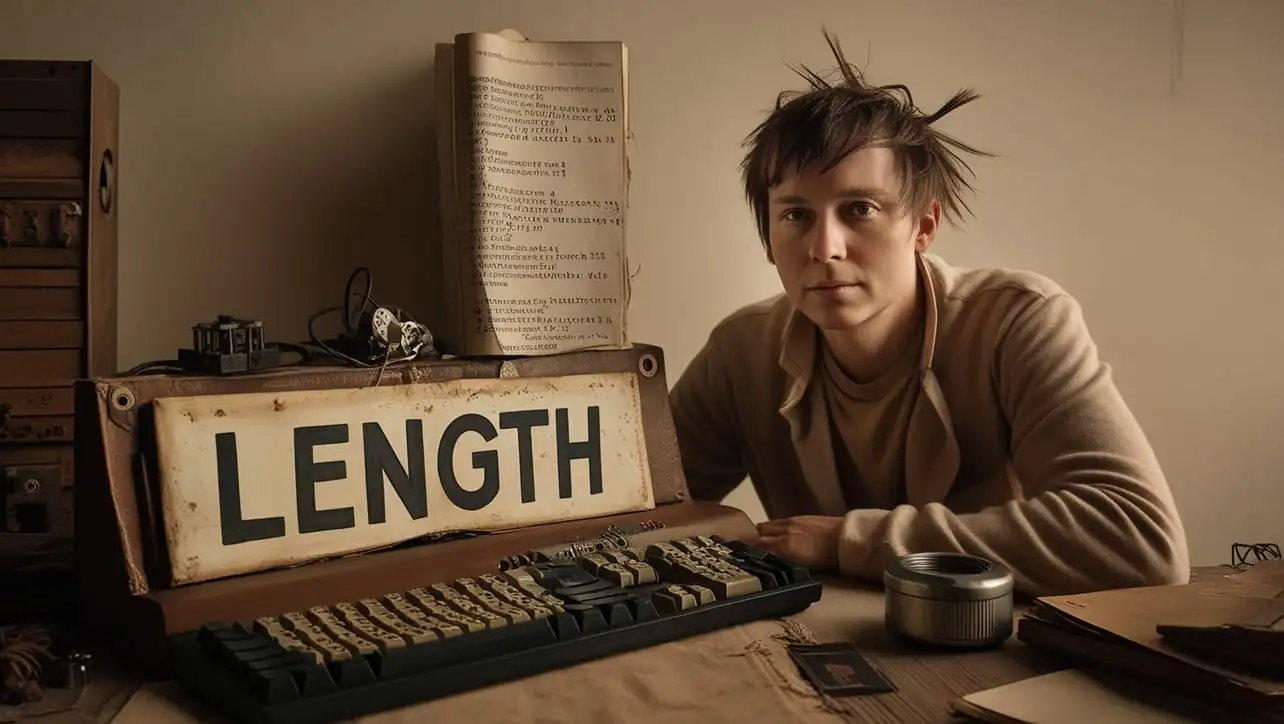
JS Basic
JS Interview Programs
- JS Interview Programs
- JS Abundant Number
- JS Amicable Number
- JS Armstrong Number
- JS Average of N Numbers
- JS Automorphic Number
- JS Biggest of three numbers
- JS Binary to Decimal
- JS Common Divisors
- JS Composite Number
- JS Condense a Number
- JS Cube Number
- JS Decimal to Binary
- JS Decimal to Octal
- JS Disarium Number
- JS Even Number
- JS Evil Number
- JS Factorial of a Number
- JS Fibonacci Series
- JS GCD
- JS Happy Number
- JS Harshad Number
- JS LCM
- JS Leap Year
- JS Magic Number
- JS Matrix Addition
- JS Matrix Division
- JS Matrix Multiplication
- JS Matrix Subtraction
- JS Matrix Transpose
- JS Maximum Value of an Array
- JS Minimum Value of an Array
- JS Multiplication Table
- JS Natural Number
- JS Number Combination
- JS Odd Number
- JS Palindrome Number
- JS Pascalβs Triangle
- JS Perfect Number
- JS Perfect Square
- JS Power of 2
- JS Power of 3
- JS Pronic Number
- JS Prime Factor
- JS Prime Number
- JS Smith Number
- JS Strong Number
- JS Sum of Array
- JS Sum of Digits
- JS Swap Two Numbers
- JS Triangular Number
JavaScript Program to Check Armstrong Number
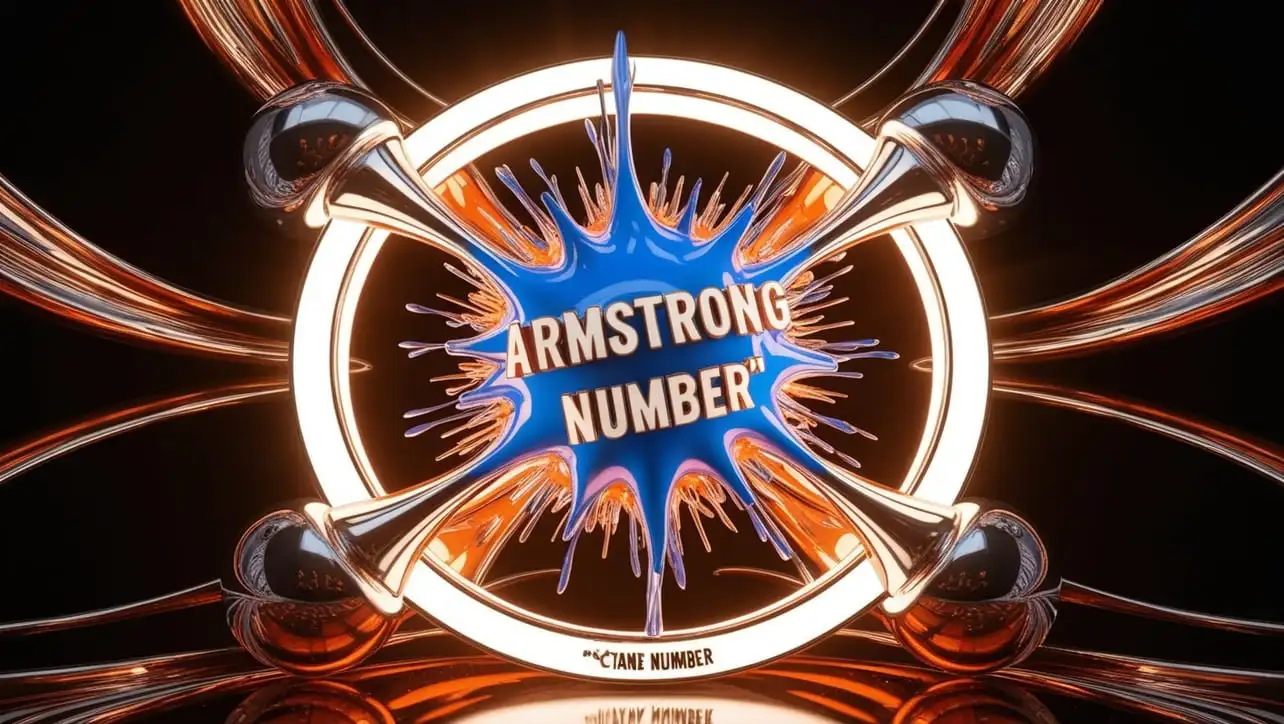
Photo Credit to CodeToFun
π Introduction
In the realm of programming, solving mathematical problems is a common task. One such interesting problem is checking whether a given number is an Armstrong number.
Armstrong numbers, also known as narcissistic numbers or pluperfect digital invariants, are numbers that are the sum of their own digits each raised to the power of the number of digits.
In this tutorial, we will explore a JavaScript program designed to check whether a given number is an Armstrong number. The program involves calculating the sum of the digits raised to the power of the number of digits and comparing it with the original number.
π Example
Let's delve into the JavaScript code that accomplishes this task.
<!DOCTYPE html>
<html>
<body>
<script>
// Function to check if a number is an Armstrong number
function isArmstrong(number) {
let originalNumber = number;
let n = 0;
let result = 0;
// Count the number of digits
while (originalNumber !== 0) {
originalNumber = Math.floor(originalNumber / 10);
n++;
}
// Reset originalNumber to the actual number
originalNumber = number;
// Calculate the sum of nth power of individual digits
while (originalNumber !== 0) {
const remainder = originalNumber % 10;
result += Math.pow(remainder, n);
originalNumber = Math.floor(originalNumber / 10);
}
// Check if the result is equal to the original number
return result === number;
}
// Driver program
// Replace this value with the number you want to check
const number = 153;
// Call the function to check if the number is Armstrong
if (isArmstrong(number)) {
console.log(`${number} is an Armstrong number.`);
} else {
console.log(`${number} is not an Armstrong number.`);
}
</script>
</body>
</html>
π» Testing the Program
To test the program with different numbers, modify the value of number in the code.
153 is an Armstrong number.
π§ How the Program Works
- The program defines a function isArmstrong that takes a number as input and checks whether it is an Armstrong number.
- Replace the value of number in the main program with the desired number you want to check.
- The program calls the isArmstrong function and prints the result using console.log.
π Between the Given Range
Let's explore the JavaScript code that checks for Armstrong numbers in the specified range.
// Function to check if a number is an Armstrong number
function isArmstrongNumber(number) {
const numString = number.toString();
const numDigits = numString.length;
let sum = 0;
for (let i = 0; i < numDigits; i++) {
sum += Math.pow(parseInt(numString[i]), numDigits);
}
return sum === number;
}
// Check Armstrong numbers in the range of 1 to 50
for (let i = 1; i <= 50; i++) {
if (isArmstrongNumber(i)) {
console.log(`${i} is an Armstrong number.`);
}
}
π» Testing the Program
Armstrong numbers in the range from 1 to 50: 1, 2, 3, 4, 5, 6, 7, 8, 9,
The provided code checks for Armstrong numbers in the range of 1 to 50. Run the script to see the Armstrong numbers within this range.
π§ How the Program Works
- The program defines a function isArmstrongNumber that checks if a given number is an Armstrong number.
- Inside the function, it converts the number to a string to determine the number of digits.
- It then iterates through each digit, raises it to the power of the number of digits, and accumulates the sum.
- The main section checks and prints Armstrong numbers in the range of 1 to 50.
π Between the Given Range
Let's take a look at the javascript code that checks for strong numbers in the range of 1 to 200.
// Function to calculate the factorial of a number
function factorial(num) {
if (num === 0 || num === 1) {
return 1;
} else {
return num * factorial(num - 1);
}
}
// Function to check if a number is a strong number
function isStrongNumber(num) {
let originalNum = num;
let sum = 0;
while (num > 0) {
let digit = num % 10;
sum += factorial(digit);
num = Math.floor(num / 10);
}
return sum === originalNum;
}
// Finding strong numbers in the range 1 to 200
function findStrongNumbersInRange() {
let strongNumbers = [];
for (let i = 1; i <= 200; i++) {
if (isStrongNumber(i)) {
strongNumbers.push(i);
}
}
return strongNumbers;
}
// Driver program
let strongNumbersInRange = findStrongNumbersInRange();
console.log("Strong Numbers in the Range 1 to 200:");
console.log(strongNumbersInRange.join(" "));
π» Testing the Program
Strong Numbers in the Range 1 to 200: 1 2 145
Run the program to see the strong numbers in the range of 1 to 200.
π§ How the Program Works
- The program defines a function factorial to calculate the factorial of a number.
- It defines a function isStrongNumber to check if a given number is a strong number.
- The findStrongNumbersInRange function iterates through numbers from 1 to 200 and identifies strong numbers.
- The driver program logs the strong numbers in the specified range.
π§ Understanding the Concept of Armstrong Number
Before delving into the code, let's understand the concept behind Armstrong numbers. An Armstrong number is a number that is the sum of its own digits each raised to the power of the number of digits.
For example, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 = 153.
π’ Optimizing the Program
While the provided program is straightforward, consider exploring and implementing alternative approaches or optimizations for checking Armstrong numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
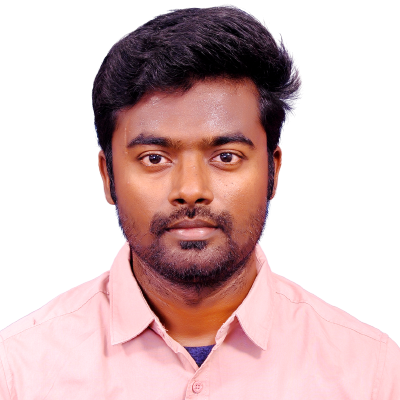
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Program to Check Armstrong Number) please comment here. I will help you immediately.