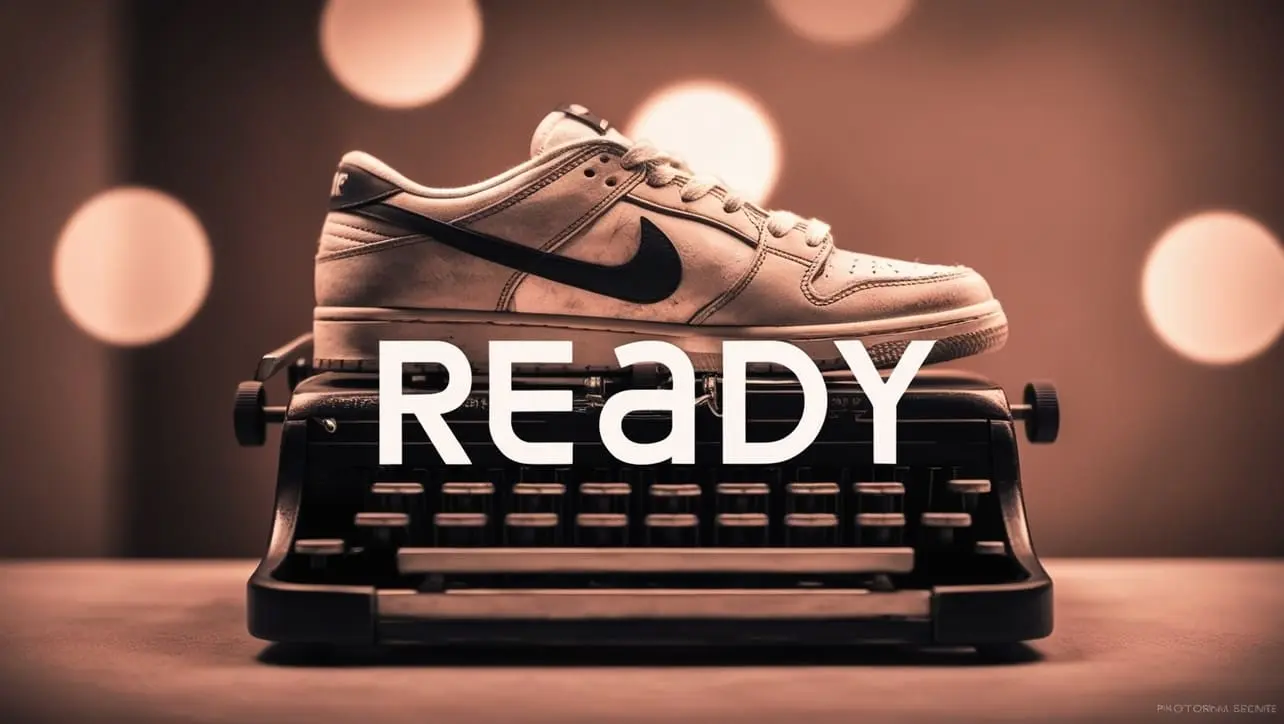
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .width() Method
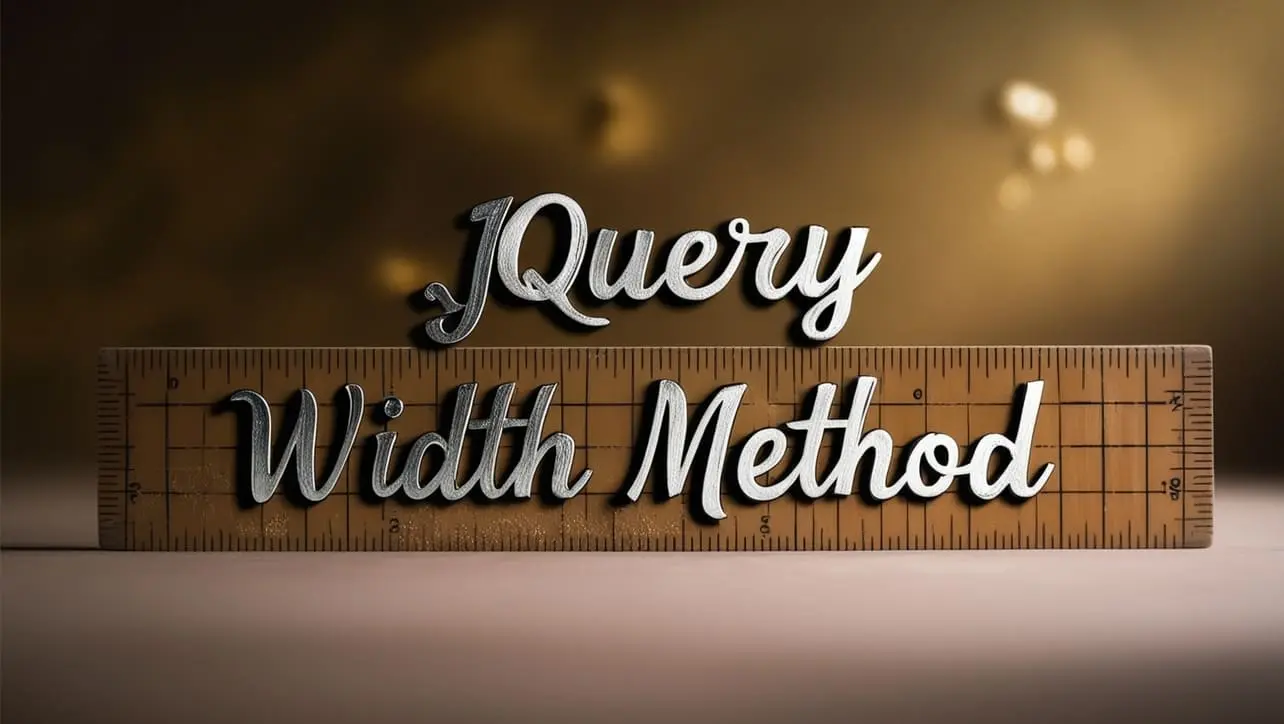
Photo Credit to CodeToFun
Introduction
jQuery offers a myriad of methods to simplify web development, and one such method is .width()
. This method allows you to retrieve the current computed width of the first element in a set of matched elements or set the width of every matched element. Understanding and mastering the .width()
method can significantly enhance your ability to manipulate element widths dynamically.
In this comprehensive guide, we'll explore the usage of the jQuery .width()
method with practical examples to help you harness its full potential.
Understanding .width() Method
The .width()
method in jQuery retrieves the current computed width of the first element in the set of matched elements, including padding but not border or margin. It can also set the width of every matched element to a specified value.
Syntax
The syntax for the .width()
method is straightforward:
$(selector).width()
$(selector).width(value)
Example
Retrieving Width:
To retrieve the width of an element, you can simply call the
.width()
method without passing any parameters. For instance::index.htmlCopied<div id="element" style="width: 200px; padding: 10px; border: 1px solid black;">Sample Element</div>
example.jsCopiedvar width = $("#element").width(); console.log(width); // Output: 200
This will log the computed width of the element (including padding) to the console.
Setting Width:
You can also set the width of an element using the
.width()
method by passing a numeric value representing the desired width in pixels. For example:example.jsCopied$("#element").width(300);
This will set the width of the element to 300 pixels.
Handling Dynamic Width:
The
.width()
method is particularly useful when dealing with dynamically changing widths. Consider the following example where the width of an element changes on window resize:index.htmlCopied<div id="element" style="width: 200px; padding: 10px; border: 1px solid black;">Resizable Element</div>
example.jsCopied$(window).resize(function() { var newWidth = $(window).width(); $("#element").width(newWidth); });
This script will dynamically adjust the width of the element to match the width of the window upon resizing.
Retrieving Inner Width:
If you need to retrieve the inner width of an element (excluding padding), you can use the .innerWidth() method instead of
.width()
.
Conclusion
The jQuery .width()
method is a versatile tool for retrieving and setting element widths with ease. Whether you need to retrieve widths for calculation, set widths dynamically, or handle responsive design, this method provides a straightforward solution.
By mastering its usage, you can manipulate element widths effectively and create visually appealing and responsive web pages effortlessly.
Join our Community:
Author
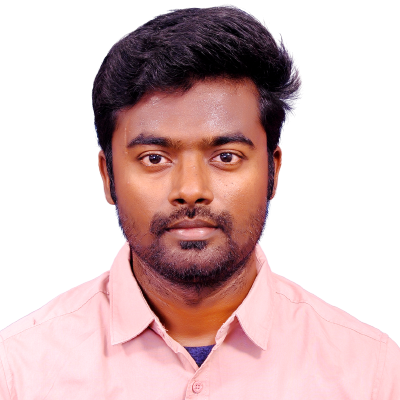
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .width() Method), please comment here. I will help you immediately.