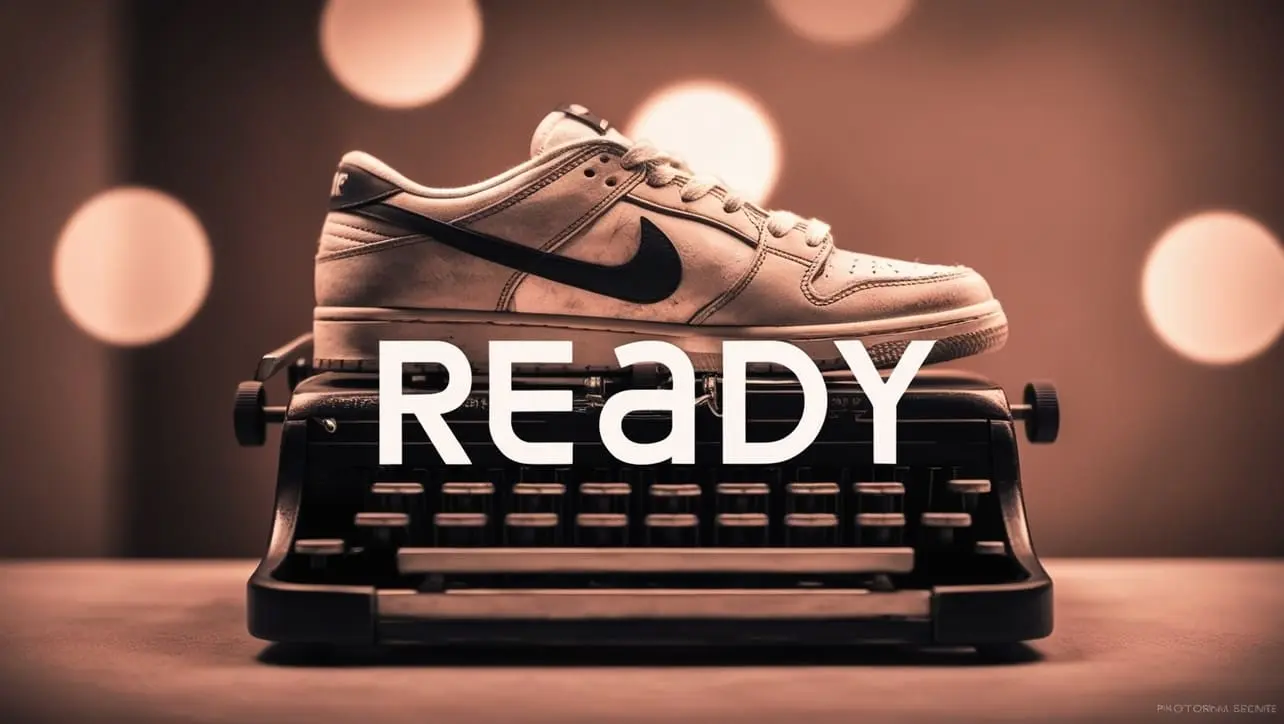
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .uniqueSort() Method
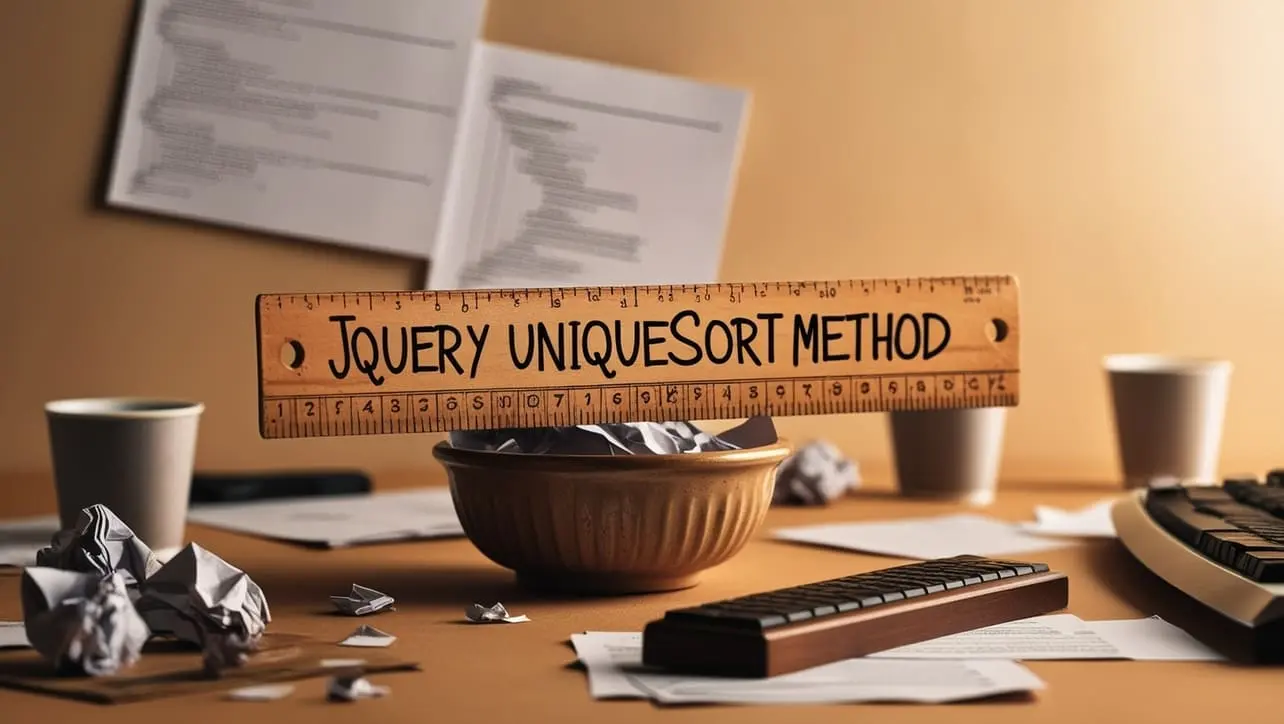
Photo Credit to CodeToFun
Introduction
jQuery offers a myriad of methods to streamline JavaScript programming and simplify DOM manipulation. One such method is .uniqueSort()
, a versatile tool designed to sort and filter arrays of DOM elements efficiently. Understanding how to leverage this method can significantly enhance your ability to manage and manipulate collections of elements in your web applications.
In this guide, we'll delve into the usage of the jQuery .uniqueSort()
method with clear examples to help you harness its full potential.
Understanding .uniqueSort() Method
The .uniqueSort()
method is used to sort and remove duplicate elements from an array. It's particularly handy when dealing with collections of DOM elements retrieved using jQuery selectors, ensuring uniqueness while preserving the order of elements.
Syntax
The syntax for the .uniqueSort()
method is straightforward:
$.uniqueSort(array)
Example
Sorting and Removing Duplicates:
Suppose you have an array of DOM elements and you want to sort them alphabetically while removing duplicates. You can achieve this using the
.uniqueSort()
method as follows:example.jsCopiedvar elements = $("div"); // Assume div elements are selected var sortedUniqueElements = $.uniqueSort(elements.get()); console.log(sortedUniqueElements);
This will log the sorted and unique DOM elements to the console.
Practical Application - Removing Duplicate Classes:
Consider a scenario where you want to remove duplicate classes from a set of elements. You can utilize
.uniqueSort()
along with jQuery's .attr() and .removeClass() methods:example.jsCopiedvar elements = $(".example"); // Assume elements with class "example" are selected elements.each(function() { var classes = $(this).attr("class").split(" "); var uniqueClasses = $.uniqueSort(classes); $(this).removeClass().addClass(uniqueClasses.join(" ")); });
This will remove duplicate classes from each selected element.
Handling Arrays of Mixed Types:
The
.uniqueSort()
method isn't limited to DOM elements; it can handle arrays containing various data types. For example:example.jsCopiedvar mixedArray = [3, 1, 2, "apple", "banana", "apple", true, true, false]; var sortedUniqueArray = $.uniqueSort(mixedArray); console.log(sortedUniqueArray);
This will log the sorted and unique elements of the mixed array to the console.
Performance Considerations:
While
.uniqueSort()
is convenient, be mindful of its performance implications when dealing with large arrays. For extensive data manipulation, consider alternative approaches or optimizations.
Conclusion
The jQuery .uniqueSort()
method provides a powerful solution for sorting arrays and removing duplicates, particularly useful when managing collections of DOM elements. Whether you're sorting elements, removing duplicate classes, or working with arrays of mixed types, this method offers a versatile toolset for efficient data manipulation.
By mastering its usage, you can streamline your JavaScript code and enhance the interactivity of your web applications.
Join our Community:
Author
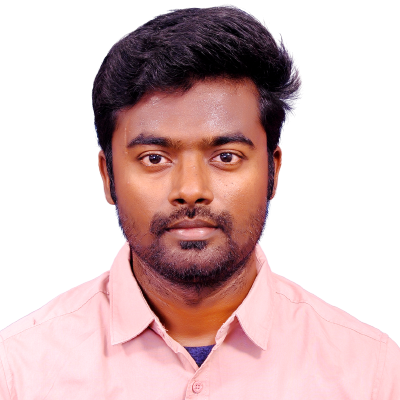
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .uniqueSort() Method), please comment here. I will help you immediately.