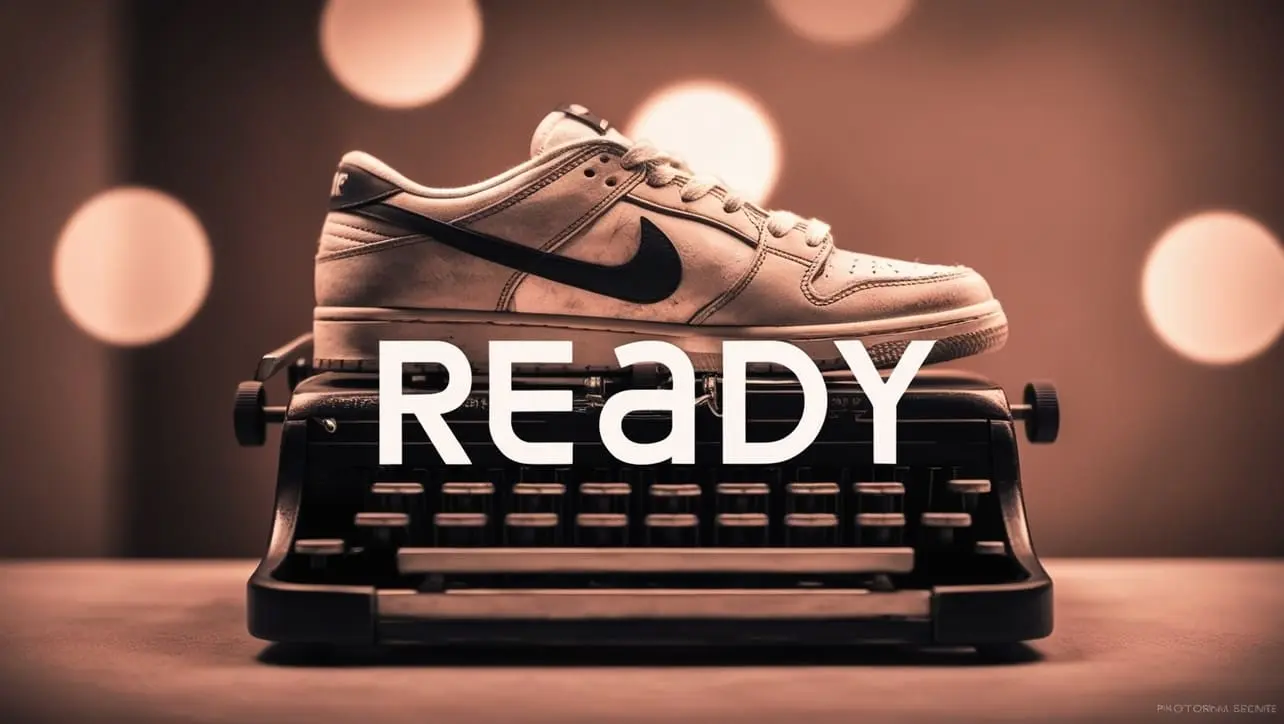
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .toArray() Method
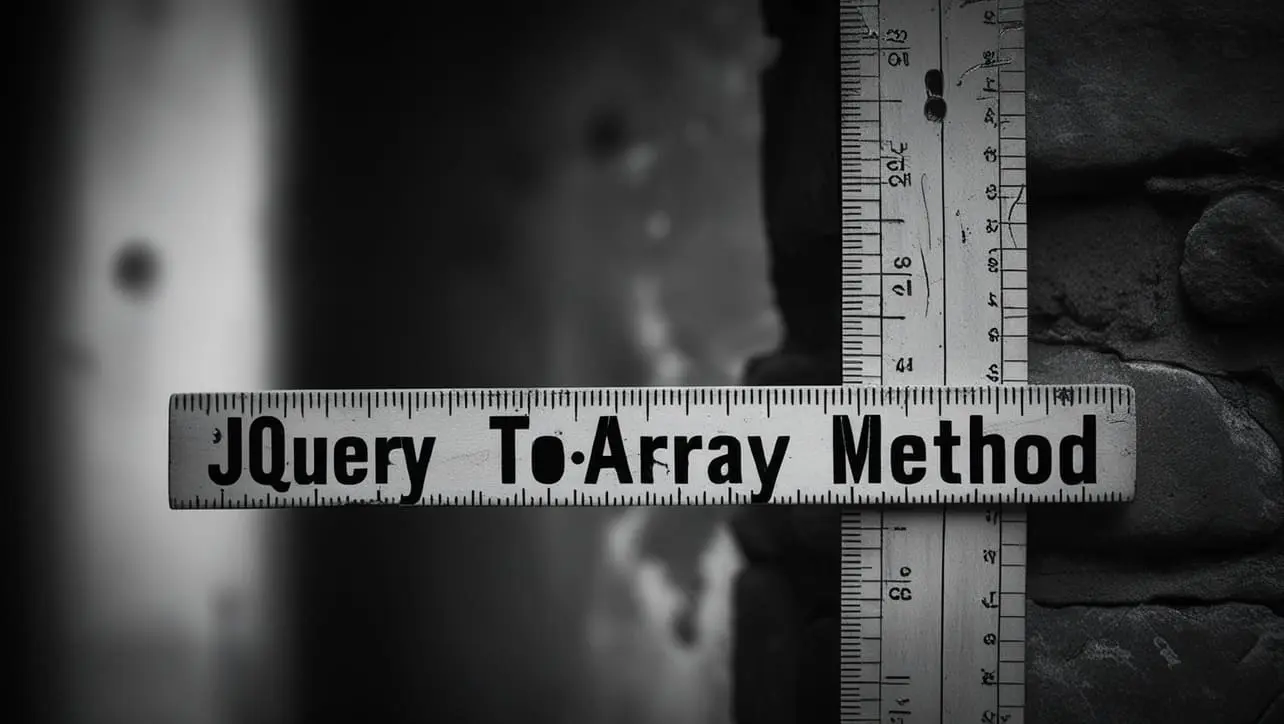
Photo Credit to CodeToFun
Introduction
In jQuery, the .toArray()
method is a handy utility that converts a jQuery object into an array. This method provides flexibility and convenience in handling sets of DOM elements returned by jQuery selectors. Understanding how to use .toArray()
effectively can streamline your code and make manipulation of DOM elements more intuitive.
This guide aims to elucidate the functionality of the .toArray()
method with clear examples for practical implementation.
Understanding .toArray() Method
The .toArray()
method in jQuery converts the selected elements into a native JavaScript array. This allows you to utilize array methods and properties to manipulate the elements more efficiently.
Syntax
The syntax for the .toArray()
method is straightforward:
$(selector).toArray()
Example
Converting Selected Elements to Array:
Suppose you have a jQuery selector that targets a set of HTML elements, such as paragraphs (<p>). You can use the
.toArray()
method to convert these elements into a JavaScript array:example.jsCopiedvar paragraphsArray = $("p").toArray();
Now, paragraphsArray contains all the <p> elements as individual items in an array, facilitating easier manipulation.
Accessing and Manipulating Array Elements:
Once the elements are converted into an array, you can access and manipulate them using standard array methods. For instance, let's change the text content of the second paragraph:
example.jsCopiedparagraphsArray[1].textContent = "New text for the second paragraph";
This modifies the text content of the second paragraph element in the array.
Iterating Through Array Elements:
You can iterate through the array of elements using loops like forEach() or traditional for loops. Here's an example using forEach() to log the text content of each paragraph:
example.jsCopiedparagraphsArray.forEach(function(paragraph) { console.log(paragraph.textContent); });
This will log the text content of each paragraph element to the console.
Combining with Other Array Methods:
Since the result of
.toArray()
is a standard JavaScript array, you can combine it with other array methods like map(), filter(), or reduce() to perform complex operations on DOM elements more efficiently.
Conclusion
The jQuery .toArray()
method provides a convenient way to convert jQuery objects into native JavaScript arrays, facilitating easier manipulation and interaction with DOM elements. By understanding its usage and leveraging the power of JavaScript arrays, you can streamline your code and enhance the efficiency of your web development projects.
Whether you need to access, manipulate, or iterate through sets of DOM elements, .toArray()
offers a versatile solution for various scenarios.
Join our Community:
Author
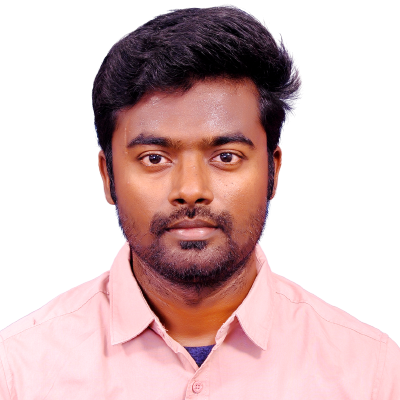
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .toArray() Method), please comment here. I will help you immediately.