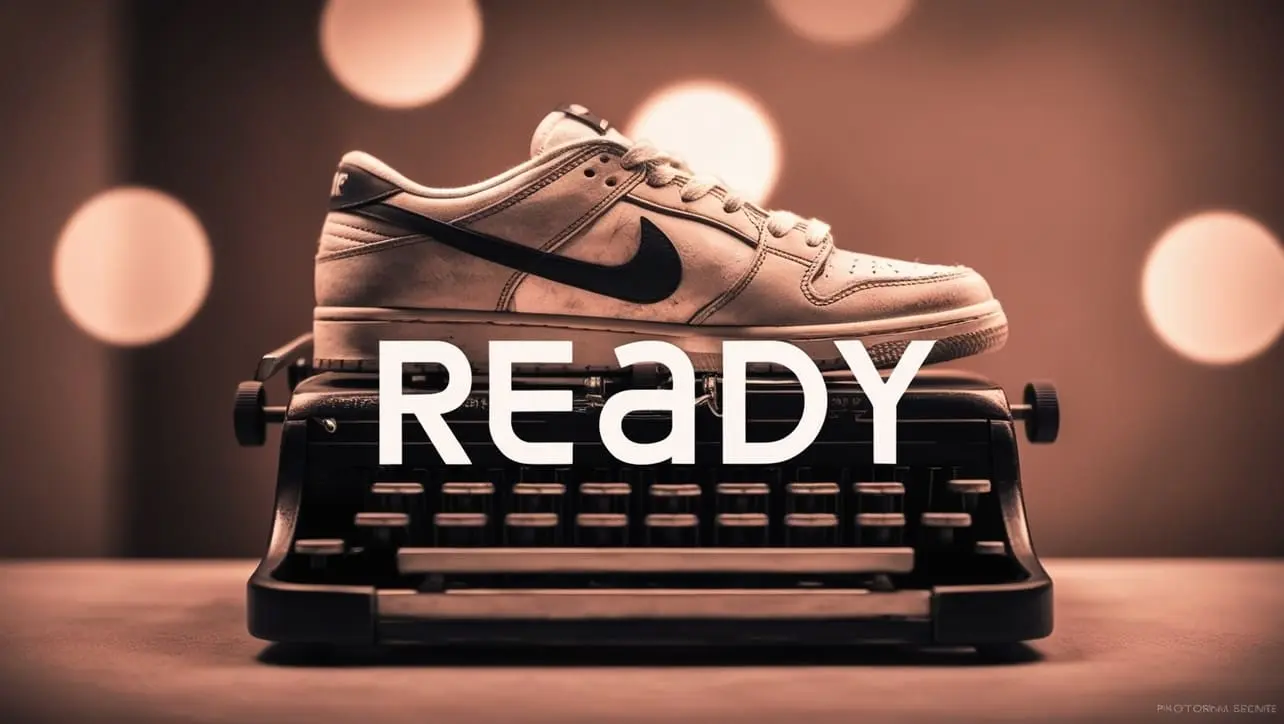
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .submit() Method
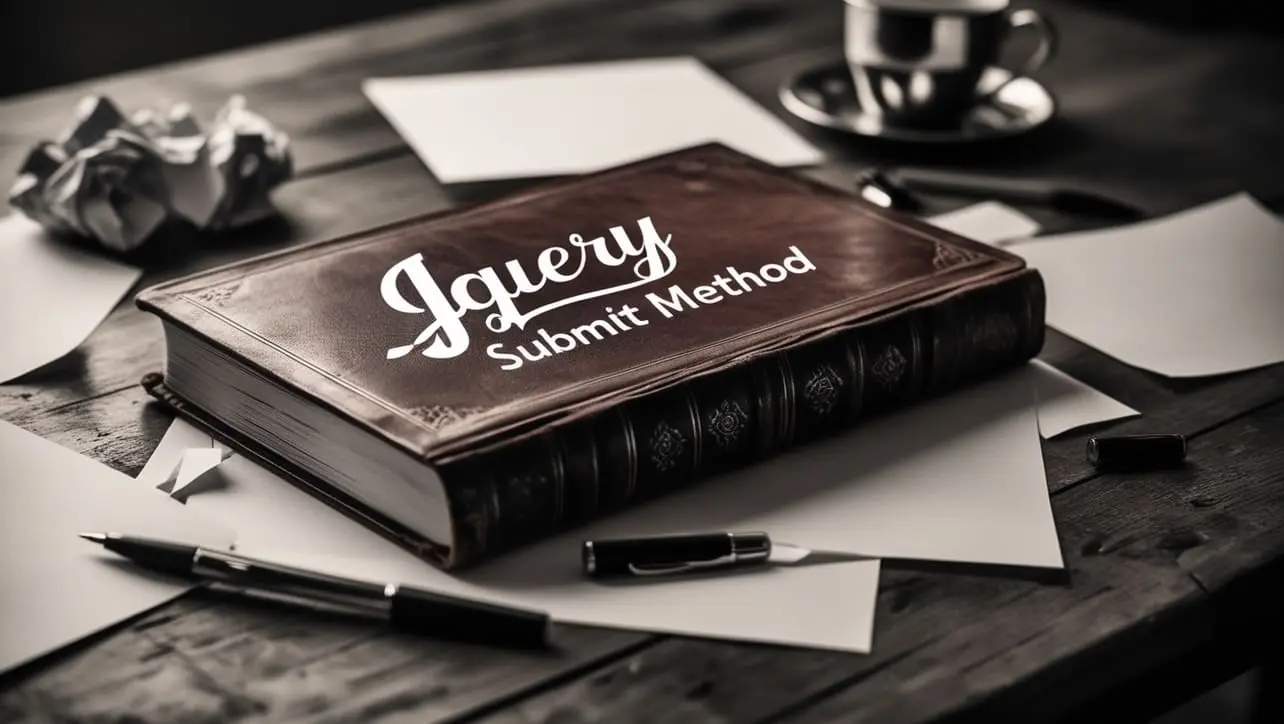
Photo Credit to CodeToFun
Introduction
In web development, form submission is a crucial aspect of user interaction. jQuery simplifies this process with its .submit()
method, providing a convenient way to handle form submissions and perform actions before, during, or after the submission process.
In this guide, we'll explore the jQuery .submit()
method, demonstrating its usage with practical examples to help you streamline your form handling tasks.
Understanding .submit() Method
The .submit()
method in jQuery is used to bind an event handler function to the "submit" JavaScript event, or to trigger that event on a selected form element. This allows you to intercept form submissions, validate input data, perform AJAX requests, or execute any custom logic before the form is submitted to the server.
Syntax
The syntax for the .submit()
method is straightforward:
$("form").submit(function(event) {
// Your code here
});
Example
Intercepting Form Submission:
Suppose you have a form and you want to prevent it from being submitted until certain conditions are met. You can achieve this using the
.submit()
method as follows:index.htmlCopied<form id="myForm"> <input type="text" id="username" placeholder="Username"> <input type="password" id="password" placeholder="Password"> <button type="submit">Submit</button> </form>
example.jsCopied$("#myForm").submit(function(event) { // Prevent the form from submitting event.preventDefault(); // Perform validation var username = $("#username").val(); var password = $("#password").val(); if (username === "" || password === "") { alert("Please fill in all fields."); } else { // Proceed with form submission alert("Form submitted successfully!"); // You can also submit the form programmatically if needed // $(this).unbind('submit').submit(); } });
In this example, the form submission is intercepted, and validation checks are performed. If the required fields are not filled, an alert is shown, and the form submission is prevented. Otherwise, an alert confirms the successful submission.
AJAX Form Submission:
You can also use the
.submit()
method to handle AJAX form submissions without reloading the page. Here's an example using jQuery's AJAX method:example.jsCopied$("#myForm").submit(function(event) { // Prevent the default form submission event.preventDefault(); // Serialize form data var formData = $(this).serialize(); // Perform AJAX request $.ajax({ type: "POST", url: "submit.php", data: formData, success: function(response) { // Handle successful response alert("Form submitted successfully!"); }, error: function(xhr, status, error) { // Handle errors console.error(xhr.responseText); alert("An error occurred. Please try again."); } }); });
This example demonstrates how to submit form data asynchronously using AJAX, allowing you to update parts of the page without reloading the entire document.
Conclusion
The jQuery .submit()
method provides a powerful mechanism for handling form submissions in web applications. Whether you need to intercept form submissions for validation, perform AJAX requests, or execute custom logic, this method offers a convenient solution.
By mastering its usage, you can enhance the interactivity and responsiveness of your web forms, providing a seamless user experience.
Join our Community:
Author
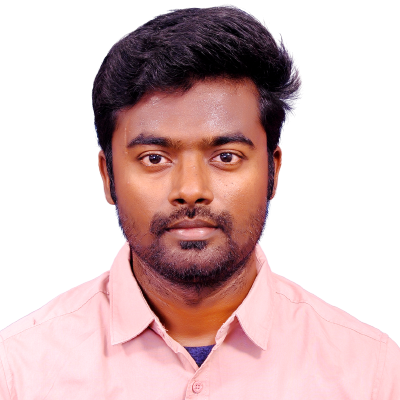
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .submit() Method), please comment here. I will help you immediately.