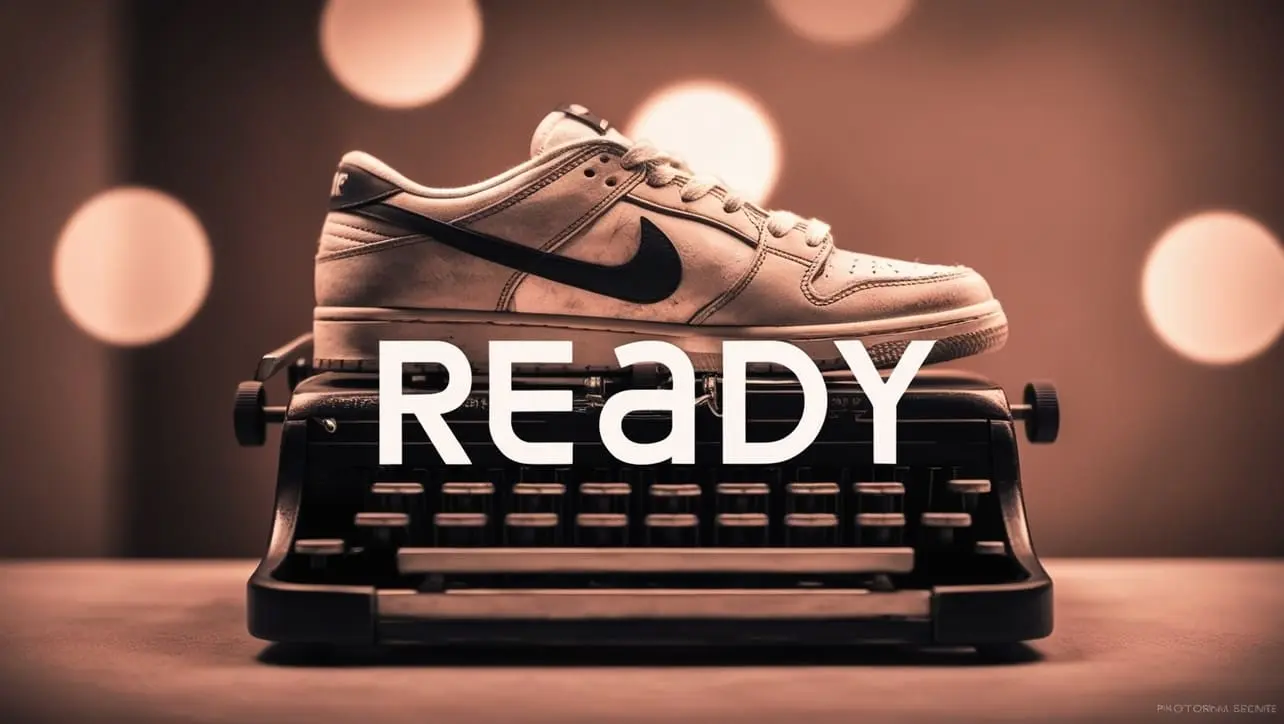
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .slideToggle() Method
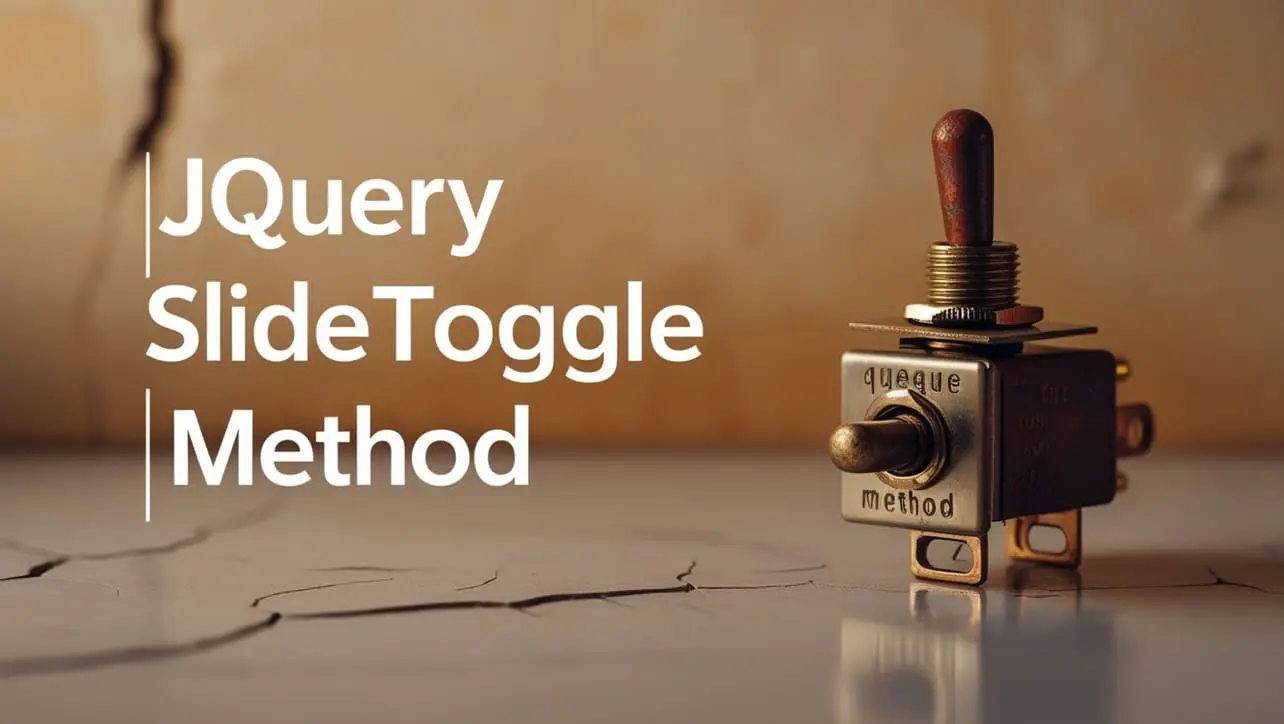
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a plethora of methods to create engaging and interactive web experiences, and one such method is .slideToggle()
. This versatile method allows you to smoothly toggle the visibility of elements with a sliding animation. Understanding and mastering .slideToggle()
can add a touch of elegance to your web projects.
In this guide, we'll explore the usage of the jQuery .slideToggle()
method with detailed examples to help you harness its potential.
🧠 Understanding .slideToggle() Method
The .slideToggle()
method is used to toggle the visibility of elements with a sliding animation. It hides the matched elements if they are visible, and shows them if they are hidden, all while applying a smooth sliding effect.
💡 Syntax
The syntax for the .slideToggle()
method is straightforward:
$(selector).slideToggle(speed, easing, callback);
Parameters:
- speed (optional): Specifies the duration of the animation in milliseconds or a predefined string ("slow", "fast").
- easing (optional): Specifies the easing function for the animation.
- callback (optional): A function to execute once the animation is complete.
📝 Example
Toggling a Div's Visibility:
Let's start with a simple example where clicking a button toggles the visibility of a <div> element with a sliding animation:
index.htmlCopied<button id="toggleButton">Toggle</button> <div id="content" style="display: none;"> Content to toggle </div>
example.jsCopied$("#toggleButton").click(function() { $("#content").slideToggle(); });
Clicking the Toggle button will smoothly slide the content in and out of view.
Specifying Animation Speed:
You can control the speed of the sliding animation by specifying the duration in milliseconds or using predefined strings like
slow
orfast
. Here's an example:example.jsCopied$("#toggleButton").click(function() { $("#content").slideToggle("slow"); });
This will animate the sliding effect slowly.
Adding Easing Effects:
Easing effects can add a touch of sophistication to your animations. Let's use the easeInOutCubic easing effect:
example.jsCopied$("#toggleButton").click(function() { $("#content").slideToggle("slow", "easeInOutCubic"); });
This will apply a smooth easing effect to the sliding animation.
Chaining .slideToggle() with Other Methods:
You can chain
.slideToggle()
with other jQuery methods to create more complex interactions. For example, toggling the visibility of an element and changing its text simultaneously:example.jsCopied$("#toggleButton").click(function() { $("#content").slideToggle().text("Content toggled!"); });
🎉 Conclusion
The jQuery .slideToggle()
method provides a seamless way to add sliding animations to toggle the visibility of elements on your web pages. Whether you're creating collapsible menus, toggling content sections, or implementing interactive elements, .slideToggle()
offers a smooth and elegant solution.
By mastering its usage and exploring its various options, you can enhance the user experience and make your web projects more engaging.
👨💻 Join our Community:
Author
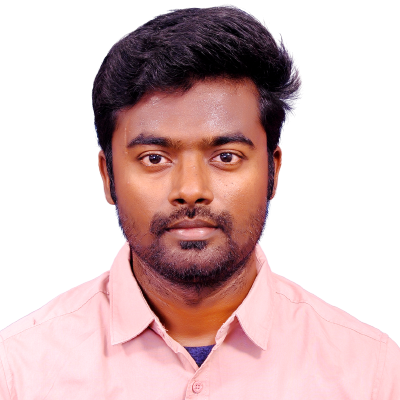
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .slideToggle() Method), please comment here. I will help you immediately.