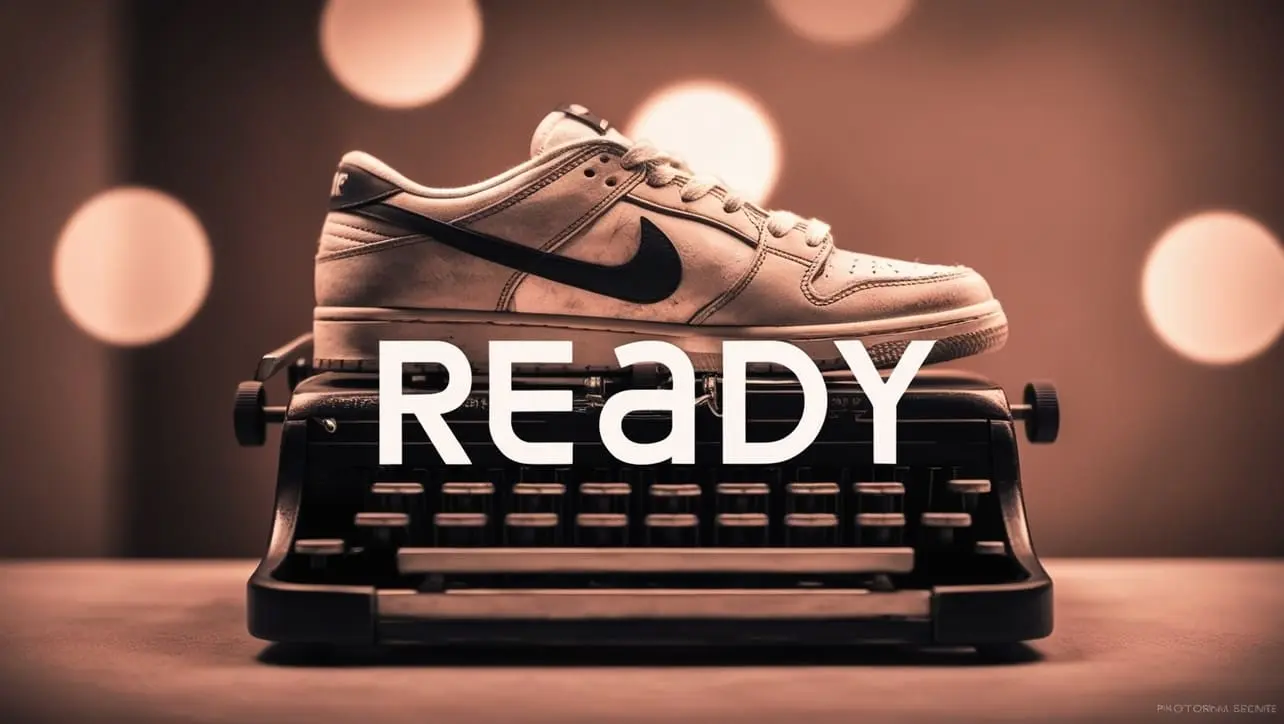
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .serialize() Method
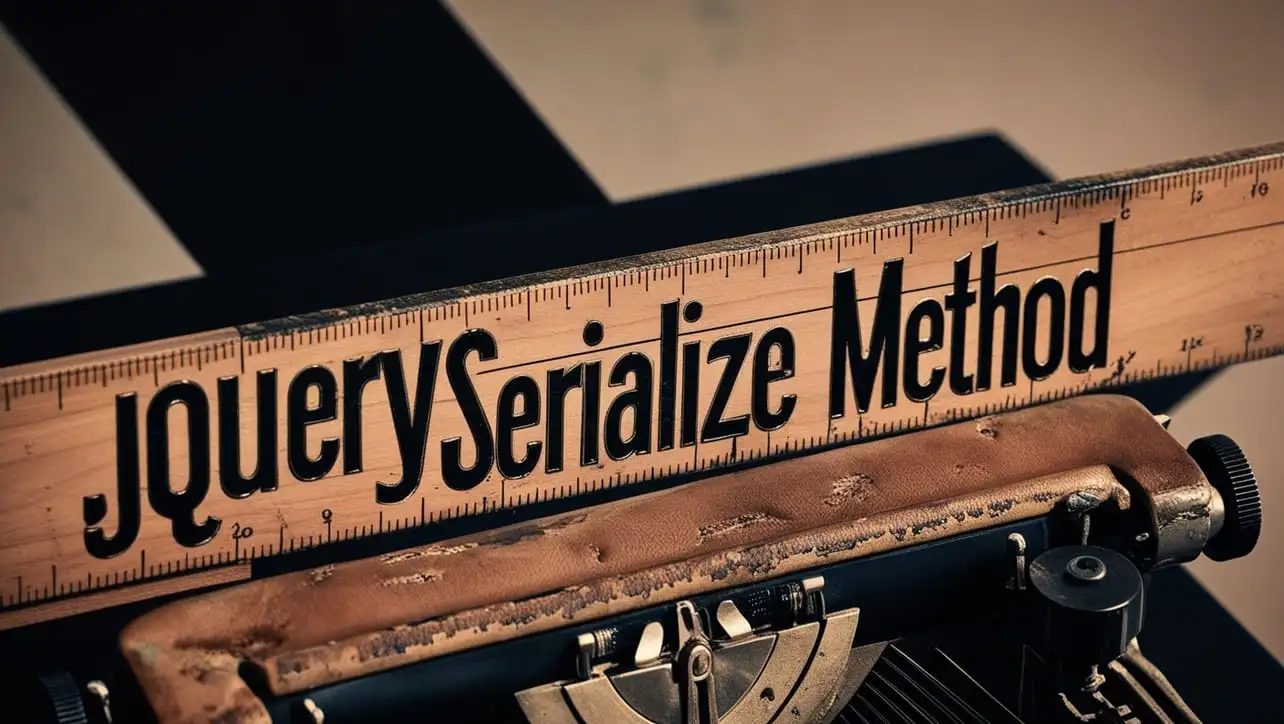
Photo Credit to CodeToFun
🙋 Introduction
In web development, forms play a crucial role in collecting user data. jQuery simplifies form handling with its powerful methods, one of which is .serialize()
. This method allows you to serialize form data into a query string format easily. Understanding how to utilize .serialize()
effectively can streamline data submission and processing.
In this guide, we'll explore the jQuery .serialize()
method with clear examples to illustrate its usage and benefits.
🧠 Understanding .serialize() Method
The .serialize()
method in jQuery converts form data into a format that can be easily transmitted via AJAX requests or submitted as part of a URL query string. It gathers the form elements and their values and formats them in a key-value pair structure.
💡 Syntax
The syntax for the .serialize()
method is straightforward:
$( "form" ).serialize()
📝 Example
Serializing Form Data:
Consider a simple HTML form with input fields for username and email:
index.htmlCopied<form id="myForm"> <input type="text" name="username" value="John"> <input type="email" name="email" value="john@example.com"> </form>
You can serialize this form data using the
.serialize()
method as follows:example.jsCopiedvar formData = $( "#myForm" ).serialize(); console.log(formData);
This will output: username=John&email=john%40example.com
AJAX Submission with Serialized Data:
You can use the serialized form data to submit an AJAX request to a server:
example.jsCopied$.ajax({ url: "submit.php", type: "post", data: $( "#myForm" ).serialize(), success: function(response) { console.log("Form submitted successfully"); }, error: function(xhr, status, error) { console.error("Error:", error); } });
This sends the serialized form data to submit.php for processing.
Combining with .submit() for Form Submission:
You can also use
.serialize()
in conjunction with the .submit() method to handle form submission:example.jsCopied$( "#myForm" ).submit(function(event) { event.preventDefault(); // Prevent default form submission var formData = $(this).serialize(); console.log("Form data:", formData); // Additional processing or AJAX submission can be done here });
Excluding Specific Form Elements:
You can exclude specific form elements from serialization by using the :not() selector. For example, to exclude inputs with the class exclude, you can modify the selector as follows:
example.jsCopied$( "form :input:not(.exclude)" ).serialize()
🎉 Conclusion
The jQuery .serialize()
method simplifies the process of collecting and formatting form data for submission or manipulation. Whether you're sending data via AJAX requests, handling form submissions, or performing custom data processing, .serialize()
offers a convenient solution.
By mastering its usage, you can enhance the efficiency and functionality of your web applications.
👨💻 Join our Community:
Author
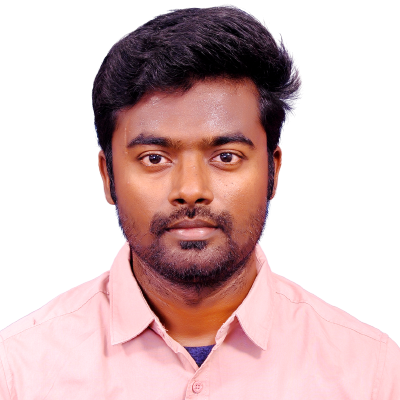
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .serialize() Method), please comment here. I will help you immediately.