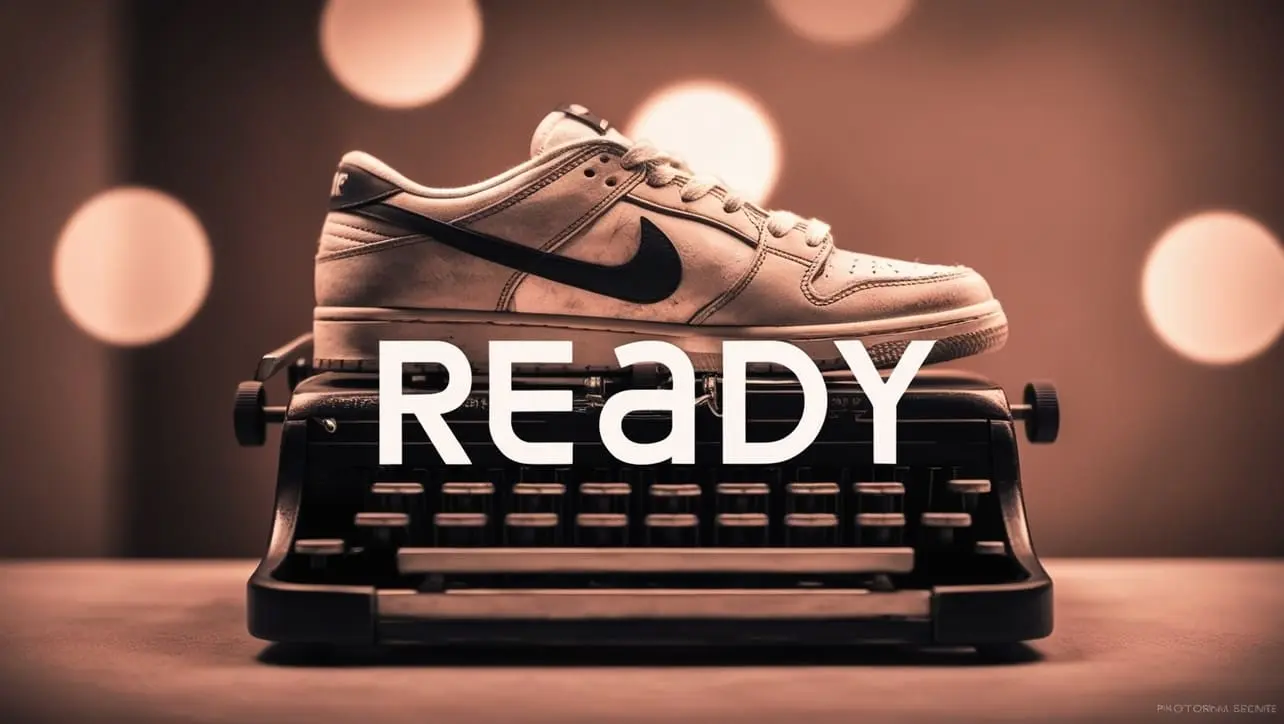
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- *
- :animated
- [name|=”value”]
- [name*=”value”]
- [name~=”value”]
- [name$=”value”]
- [name=”value”]
- [name!=”value”]
- [name^=”value”]
- :button
- :checkbox
- :checked
- Child Selector
- .class
- :contains()
- Descendant Selector
- :disabled
- Element
- :empty
- :enabled
- :eq()
- :even
- :file
- :first-child
- :first-of-type
- :first
- :focus
- :gt()
- Has Attribute
- :has()
- :header
- :hidden
- #id
- :image
- :input
- :lang()
- :last-child
- :last-of-type
- :last
- :lt()
- [name=”value”][name2=”value2″]
- (“selector1, selector2, selectorN”)
- (“prev + next”)
- (“prev ~ siblings”)
- :not()
- :nth-child()
- :nth-last-child()
- :nth-last-of-type()
- :nth-of-type()
- :odd
- :only-child
- :only-of-type
- :parent
- :password
- :radio
- :reset
- :root
- :selected
- :submit
- :target
- :text
- :visible
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery :reset Selector
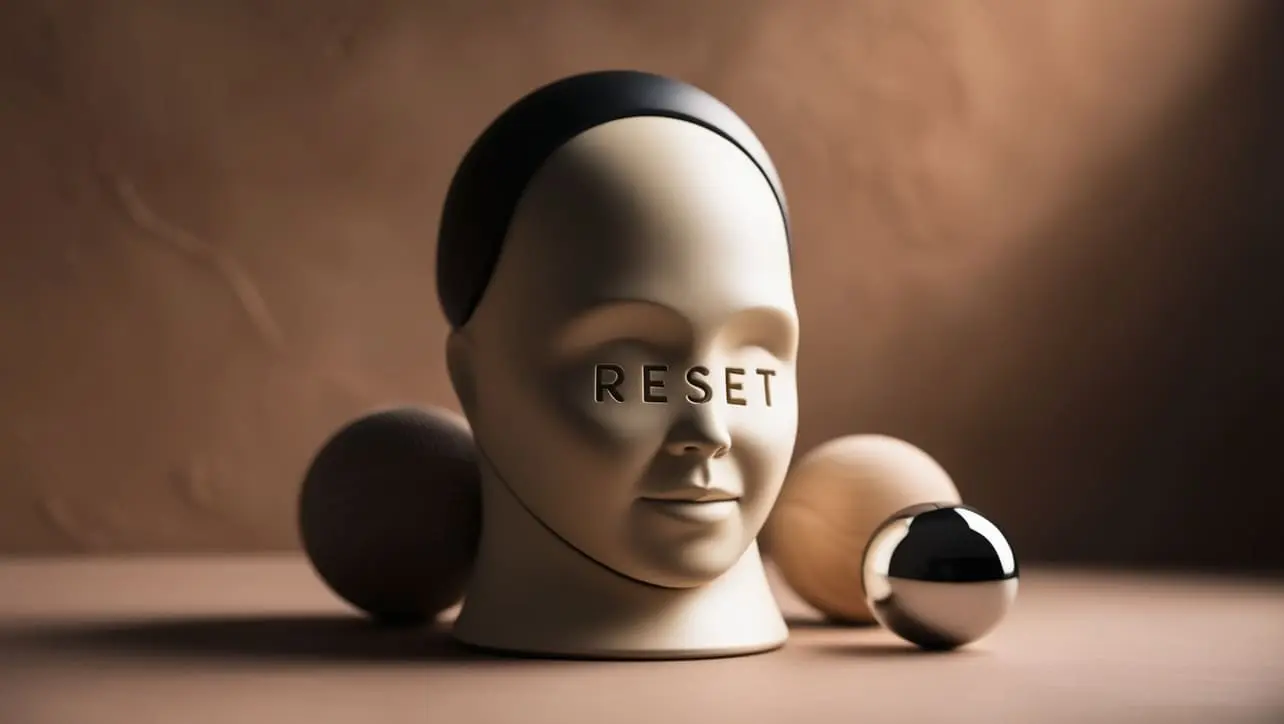
Photo Credit to CodeToFun
🙋 Introduction
jQuery offers a plethora of selectors to target specific elements in HTML documents, facilitating dynamic manipulation and event handling. Among these selectors, the :reset
selector stands out as a valuable tool for targeting form reset buttons. Understanding and leveraging the capabilities of the :reset
selector can significantly enhance your ability to create intuitive and interactive web forms.
In this comprehensive guide, we'll explore the functionality of the jQuery :reset
selector with practical examples to illuminate its usage.
🧠 Understanding :reset Selector
The :reset
selector in jQuery is designed to target HTML input elements with the type attribute set to "reset". These elements typically represent form reset buttons, allowing users to revert form fields to their initial state. By selecting these buttons dynamically, you can perform various actions or apply event handlers to enhance the user experience.
💡 Syntax
The syntax for the :reset
selector is straightforward:
$(":reset")
📝 Example
Selecting Reset Buttons:
Suppose you have a form with a reset button, and you want to select it using jQuery. You can achieve this effortlessly using the
:reset
selector:index.htmlCopied<form id="myForm"> <input type="text" name="username" value="John"> <input type="reset" value="Reset Form"> </form>
example.jsCopied$("#myForm :reset").click(function() { alert("Form reset button clicked!"); });
This code attaches a click event handler to the reset button within the form with the ID myForm, triggering an alert when clicked.
Customizing Reset Button Behavior:
You can customize the behavior of the reset button by executing specific actions when it is clicked. For example, let's clear the input fields of a form upon reset button click:
index.htmlCopied<form id="myForm"> <input type="text" name="username" value="John"> <input type="reset" value="Reset Form"> </form>
example.jsCopied$("#myForm :reset").click(function() { $("#myForm input[type='text']").val(""); });
This script ensures that all text input fields within the form are cleared when the reset button is clicked.
Enhancing User Feedback:
You can provide visual feedback to users when the reset button is clicked to reinforce their action. For instance, let's change the background color of the form upon reset:
index.htmlCopied<form id="myForm"> <input type="text" name="username" value="John"> <input type="reset" value="Reset Form"> </form>
example.jsCopied$("#myForm :reset").click(function() { $("#myForm").css("background-color", "lightgray"); });
This script changes the background color of the form to light gray when the reset button is clicked.
🎉 Conclusion
The jQuery :reset
selector provides a powerful means to target and manipulate form reset buttons, enabling you to create more responsive and user-friendly web forms. Whether you need to customize button behavior, enhance user feedback, or execute specific actions upon reset, this selector offers versatile solutions.
By mastering its usage, you can elevate the interactivity and usability of your web forms, enriching the overall user experience.
👨💻 Join our Community:
Author
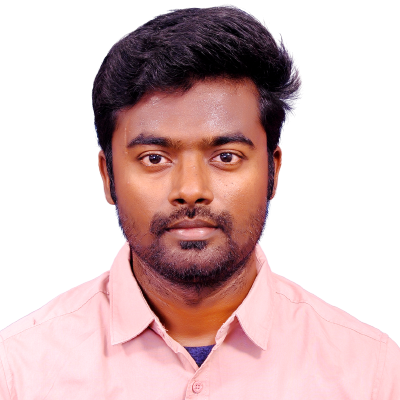
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery :reset Selector), please comment here. I will help you immediately.