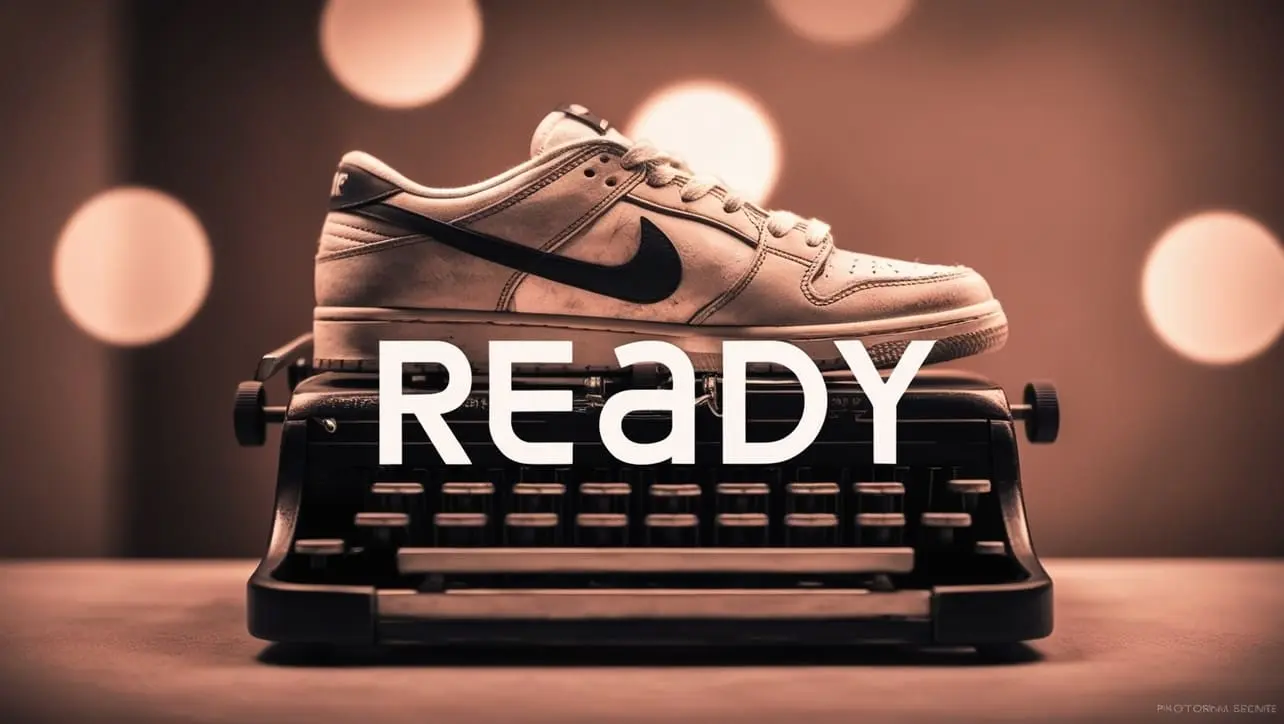
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- *
- :animated
- [name|=”value”]
- [name*=”value”]
- [name~=”value”]
- [name$=”value”]
- [name=”value”]
- [name!=”value”]
- [name^=”value”]
- :button
- :checkbox
- :checked
- Child Selector
- .class
- :contains()
- Descendant Selector
- :disabled
- Element
- :empty
- :enabled
- :eq()
- :even
- :file
- :first-child
- :first-of-type
- :first
- :focus
- :gt()
- Has Attribute
- :has()
- :header
- :hidden
- #id
- :image
- :input
- :lang()
- :last-child
- :last-of-type
- :last
- :lt()
- [name=”value”][name2=”value2″]
- (“selector1, selector2, selectorN”)
- (“prev + next”)
- (“prev ~ siblings”)
- :not()
- :nth-child()
- :nth-last-child()
- :nth-last-of-type()
- :nth-of-type()
- :odd
- :only-child
- :only-of-type
- :parent
- :password
- :radio
- :reset
- :root
- :selected
- :submit
- :target
- :text
- :visible
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery :last Selector
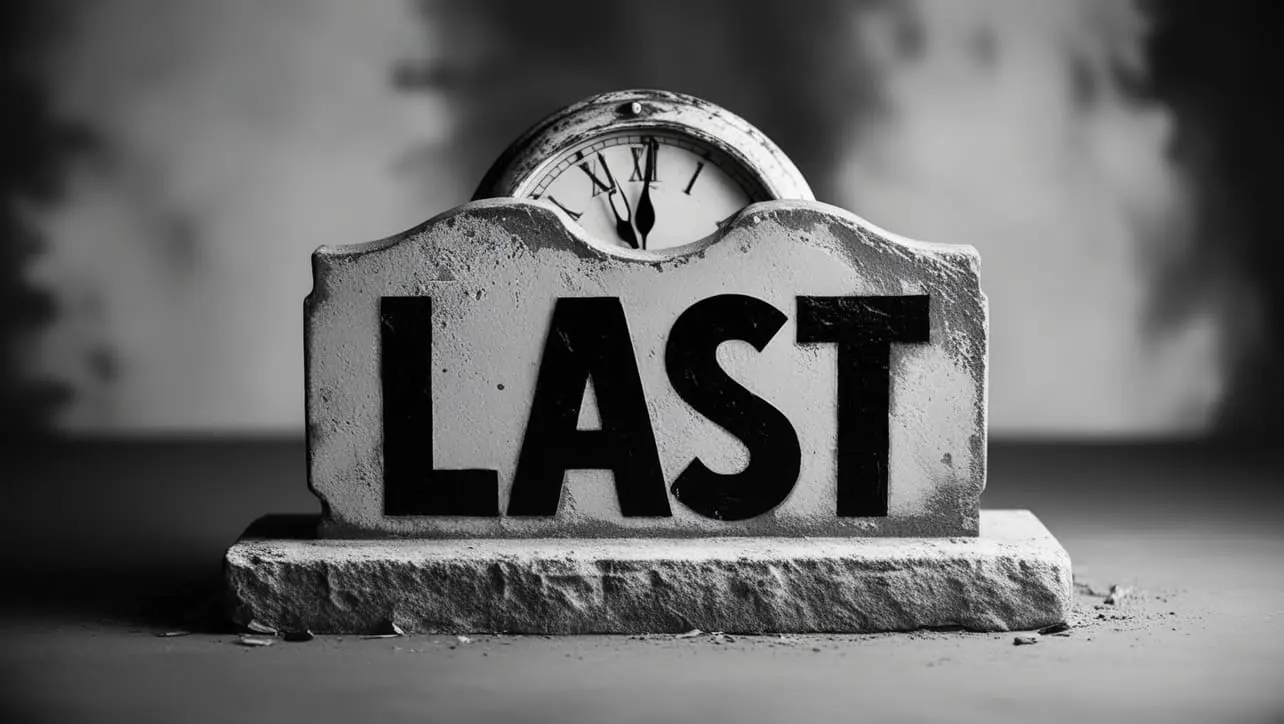
Photo Credit to CodeToFun
🙋 Introduction
In jQuery, selectors play a crucial role in targeting specific elements within a web page for manipulation. One such selector is :last
, which allows you to select the last element of a matched set. Understanding how to use the :last
selector effectively can streamline your code and enhance the functionality of your web applications.
In this guide, we'll explore the usage of the jQuery :last
selector with practical examples to help you grasp its potential.
🧠 Understanding :last Selector
The :last
selector in jQuery is used to select the last matched element within a set of elements. It is particularly useful when you want to target and manipulate the last element of a group, such as the last item in a list or the final element in a container.
💡 Syntax
The syntax for the :last
selector is straightforward:
$("selector:last")
📝 Example
Selecting the Last Element:
Consider a scenario where you have a list of items, and you want to select and manipulate the last item using jQuery. Here's how you can achieve this with the
:last
selector:index.htmlCopied<ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul>
example.jsCopied$("li:last").css("font-weight", "bold");
This will make the text of the last <li> element bold.
Applying Styles to the Last Element:
You can also apply CSS styles dynamically to the last element selected using the
:last
selector. For instance, let's change the background color of the last paragraph in a container:index.htmlCopied<div id="container"> <p>Paragraph 1</p> <p>Paragraph 2</p> <p>Paragraph 3</p> </div>
example.jsCopied$("#container p:last").css("background-color", "lightblue");
This will set the background color of the last <p> element within the #container div to light blue.
Targeting the Last Child Element:
You can also use the
:last
selector to target the last child element of a parent element. For example, let's select the last child <span> element within a <div>:index.htmlCopied<div id="parent"> <span>Span 1</span> <span>Span 2</span> <span>Span 3</span> </div>
example.jsCopied$("#parent span:last").addClass("highlight");
This will add a CSS class named highlight to the last <span> element within the #parent div.
🎉 Conclusion
The jQuery :last
selector provides a convenient way to target and manipulate the final element within a selection, whether it's the last item in a list, the last child element within a container, or any other relevant scenario.
By mastering its usage and combining it with other selectors and methods, you can enhance the interactivity and functionality of your web applications effortlessly.
👨💻 Join our Community:
Author
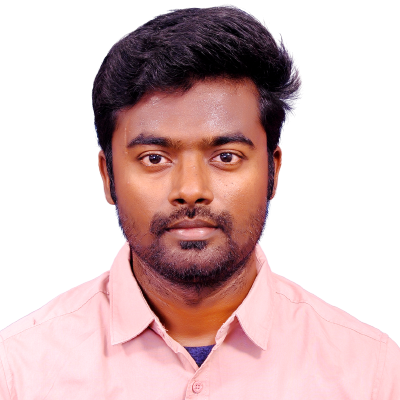
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery :last Selector), please comment here. I will help you immediately.