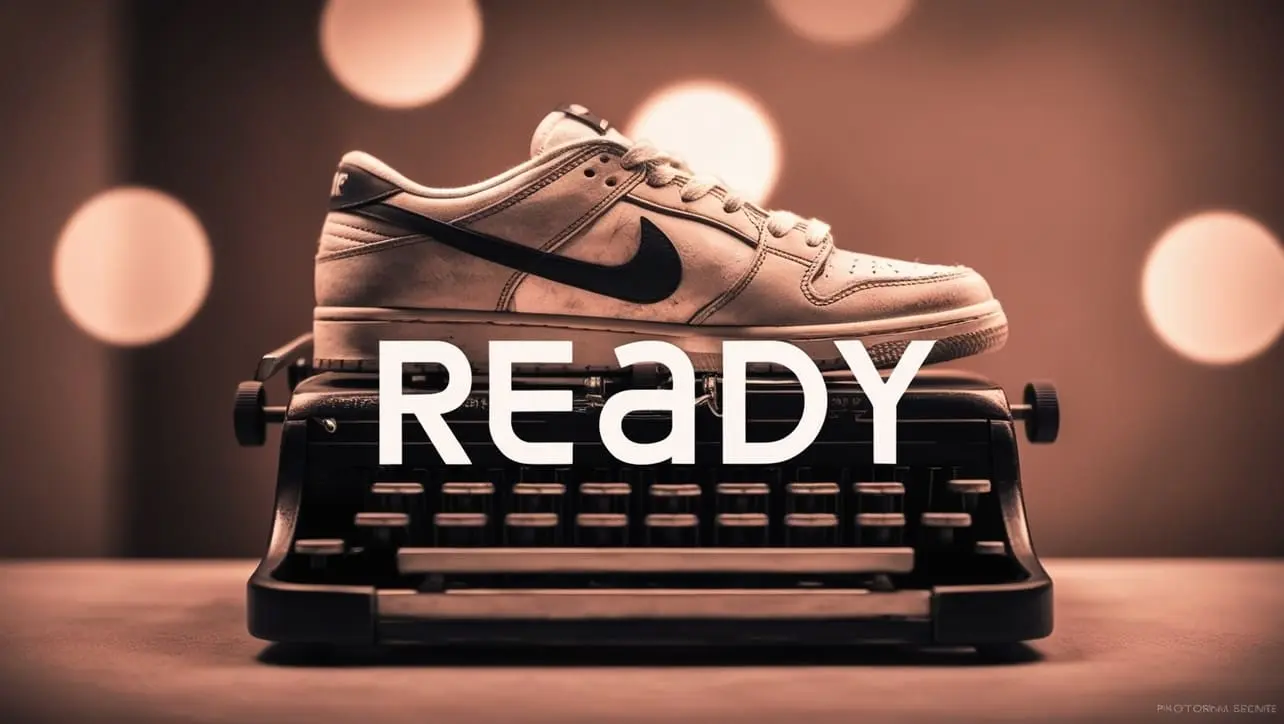
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- *
- :animated
- [name|=”value”]
- [name*=”value”]
- [name~=”value”]
- [name$=”value”]
- [name=”value”]
- [name!=”value”]
- [name^=”value”]
- :button
- :checkbox
- :checked
- Child Selector
- .class
- :contains()
- Descendant Selector
- :disabled
- Element
- :empty
- :enabled
- :eq()
- :even
- :file
- :first-child
- :first-of-type
- :first
- :focus
- :gt()
- Has Attribute
- :has()
- :header
- :hidden
- #id
- :image
- :input
- :lang()
- :last-child
- :last-of-type
- :last
- :lt()
- [name=”value”][name2=”value2″]
- (“selector1, selector2, selectorN”)
- (“prev + next”)
- (“prev ~ siblings”)
- :not()
- :nth-child()
- :nth-last-child()
- :nth-last-of-type()
- :nth-of-type()
- :odd
- :only-child
- :only-of-type
- :parent
- :password
- :radio
- :reset
- :root
- :selected
- :submit
- :target
- :text
- :visible
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery :first Selector
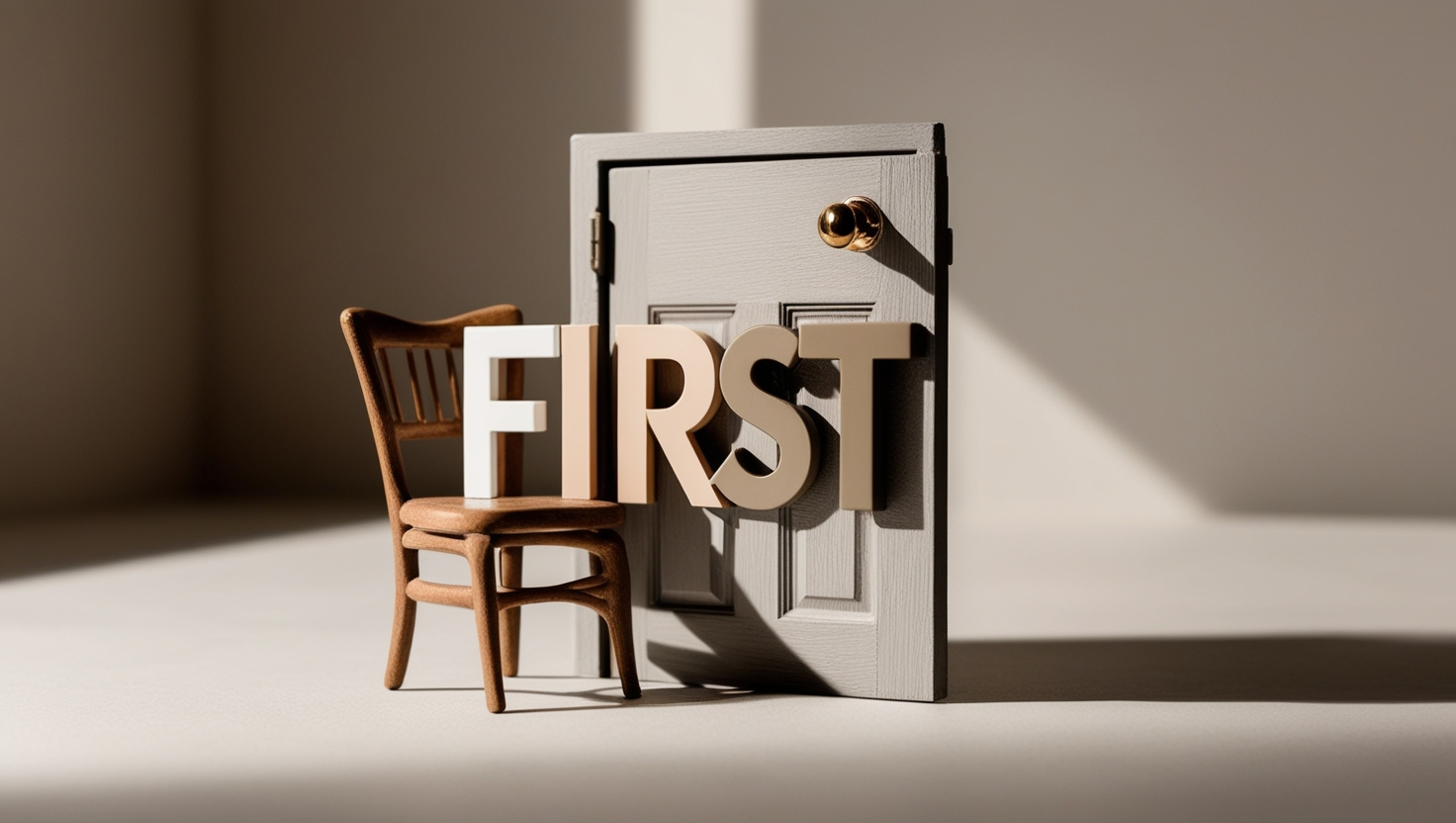
Photo Credit to CodeToFun
🙋 Introduction
In jQuery, selectors are powerful tools that allow developers to target specific elements in the DOM (Document Object Model) efficiently. One such selector is :first
, which enables you to select the first element within a set of matched elements. Understanding how to utilize the :first
selector can streamline your jQuery code and enhance your ability to manipulate web page elements.
This guide will explore the functionality of the jQuery :first
selector with illustrative examples to aid comprehension.
🧠 Understanding :first Selector
The :first
selector in jQuery targets the first element within a set of matched elements. It is particularly useful when you want to manipulate or apply actions specifically to the first element in a selection.
💡 Syntax
The syntax for the :first
selector is straightforward:
$(":first")
📝 Example
Selecting the First Element:
Suppose you have a list of paragraphs and you want to select the first one. You can use the
:first
selector as follows:index.htmlCopied<p>First paragraph</p> <p>Second paragraph</p> <p>Third paragraph</p>
example.jsCopied$("p:first").css("font-weight", "bold");
This will make the text of the first paragraph bold.
Applying Different Styles to the First Element:
You can apply different CSS styles to the first element using the
:first
selector. For example:index.htmlCopied<div class="box">Box 1</div> <div class="box">Box 2</div> <div class="box">Box 3</div>
example.jsCopied$(".box:first").css("background-color", "lightblue");
This will set the background color of the first .box element to light blue.
Handling Events on the First Element:
You can also bind events to the first element using the
:first
selector. Here's an example where we alert a message when the first button is clicked:index.htmlCopied<button>Button 1</button> <button>Button 2</button> <button>Button 3</button>
example.jsCopied$("button:first").click(function() { alert("First button clicked!"); });
Manipulating Content of the First Element:
You can manipulate the content of the first element using jQuery methods. For instance, changing the text of the first paragraph:
example.jsCopied$("p:first").text("New text for the first paragraph");
🎉 Conclusion
The jQuery :first
selector provides a convenient way to target and manipulate the first element within a set of matched elements. Whether you need to style, handle events, or manipulate content specifically for the first element, this selector offers a straightforward solution.
By incorporating the :first
selector into your jQuery toolkit, you can enhance the interactivity and dynamism of your web pages efficiently.
👨💻 Join our Community:
Author
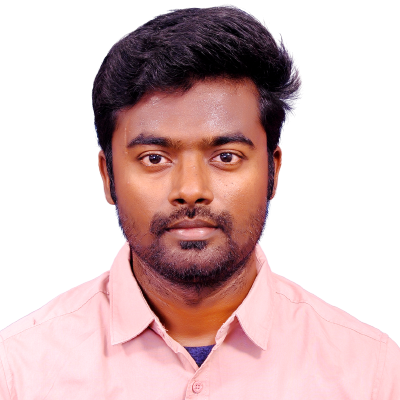
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery :first Selector), please comment here. I will help you immediately.