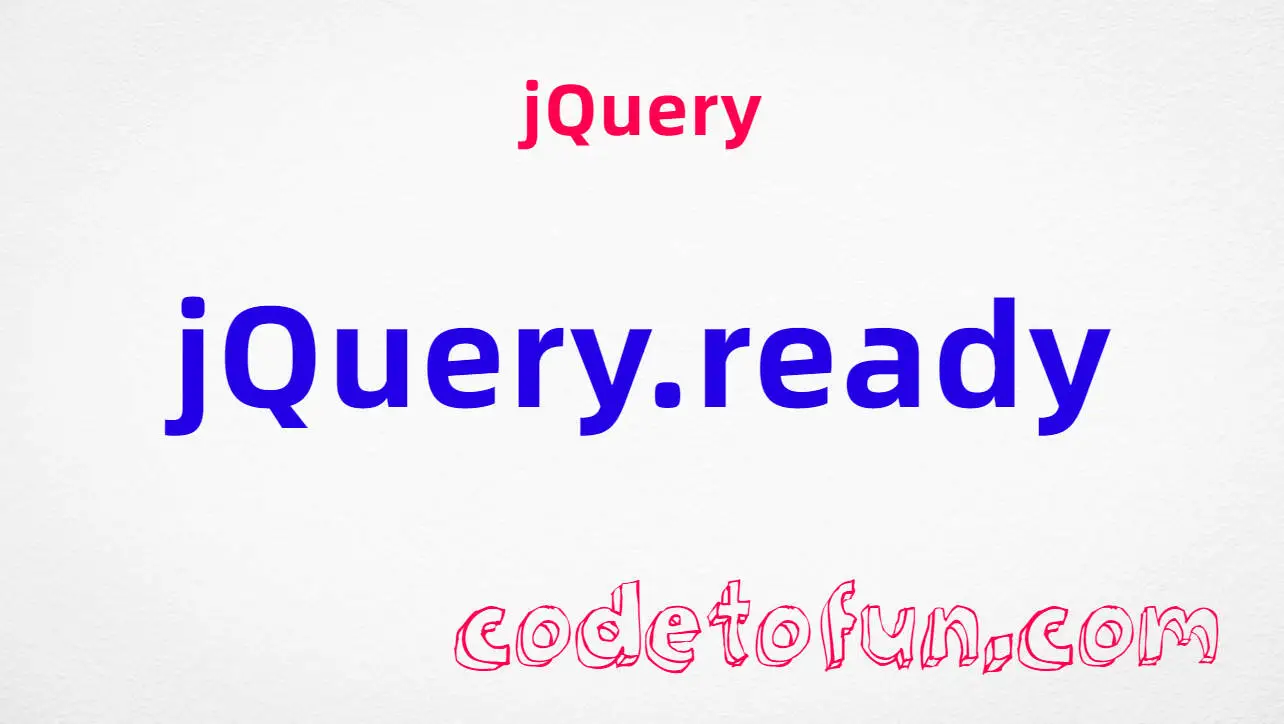
jQuery Basic
jQuery Selectors
- jQuery Selectors
- jQuery *
- jQuery :animated
- jQuery [name|=”value”]
- jQuery [name*=”value”]
- jQuery [name~=”value”]
- jQuery [name$=”value”]
- jQuery [name=”value”]
- jQuery [name!=”value”]
- jQuery [name^=”value”]
- jQuery :button
- jQuery :checkbox
- jQuery :checked
- jQuery Child Selector
- jQuery .class
- jQuery :contains()
- jQuery Descendant Selector
- jQuery :disabled
- jQuery Element
- jQuery :empty
- jQuery :enabled
- jQuery :eq()
- jQuery :even
- jQuery :file
- jQuery :first-child
- jQuery :first-of-type
- jQuery :first
- jQuery :focus
- jQuery :gt()
- jQuery Has Attribute
- jQuery :has()
- jQuery :header
- jQuery :hidden
- jQuery #id
- jQuery :image
- jQuery :input
- jQuery :lang()
- jQuery :last-child
- jQuery :last-of-type
- jQuery :last
- jQuery :lt()
- jQuery [name=”value”][name2=”value2″]
- jQuery (“selector1, selector2, selectorN”)
- jQuery (“prev + next”)
- jQuery (“prev ~ siblings”)
- jQuery :not()
- jQuery :nth-child()
- jQuery :nth-last-child()
- jQuery :nth-last-of-type()
- jQuery :nth-of-type()
- jQuery :odd
- jQuery :only-child
- jQuery :only-of-type
- jQuery :parent
- jQuery :password
- jQuery :radio
- jQuery :reset
- jQuery :root
- jQuery :selected
- jQuery :submit
- jQuery :target
- jQuery :text
- jQuery :visible
jQuery :file Selector
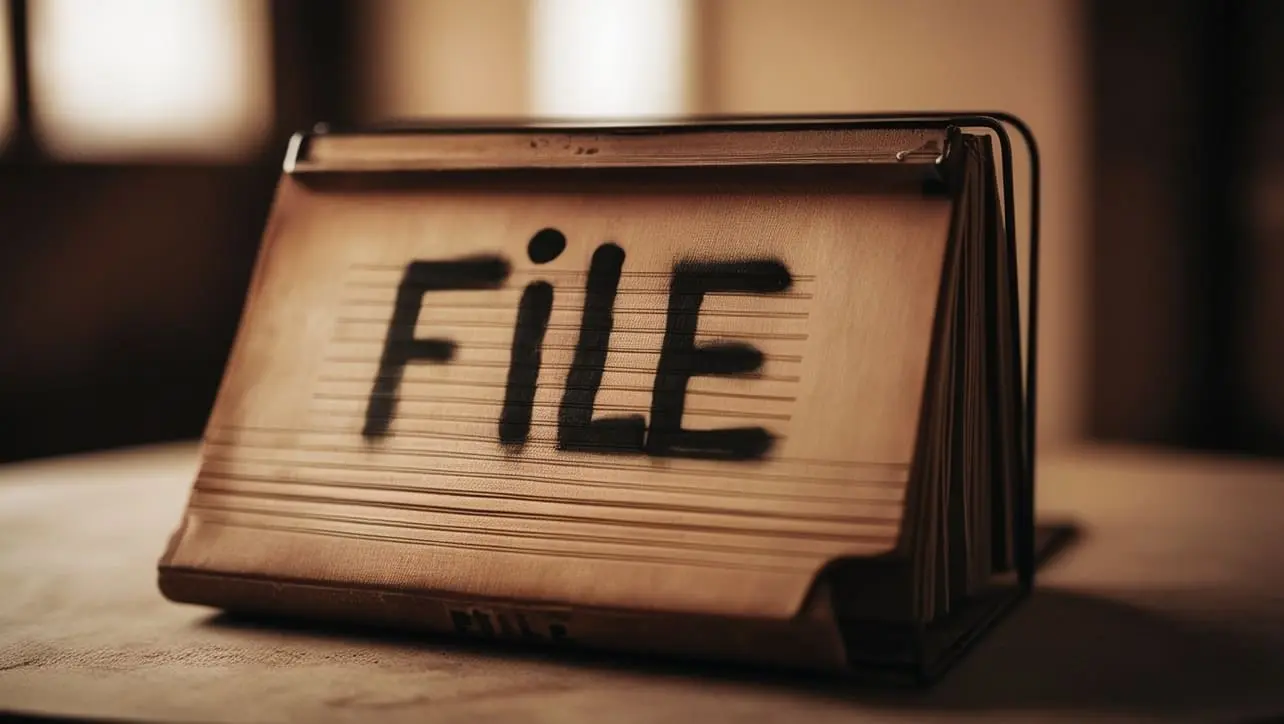
Photo Credit to CodeToFun
🙋 Introduction
In web development, handling file inputs is a common requirement, especially when dealing with forms that require file uploads. jQuery provides a convenient way to target file input elements using the :file
selector. Understanding how to effectively utilize this selector can simplify tasks such as validation, styling, and event handling related to file inputs.
In this guide, we'll explore the usage of the jQuery :file
selector with practical examples to illustrate its versatility.
🧠 Understanding :file Selector
The :file
selector in jQuery is designed specifically to target HTML input elements of type file. It allows developers to select and manipulate these elements easily, enabling various operations such as checking file input values, styling, and event binding.
💡 Syntax
The syntax for the :file
selector is straightforward:
$(":file")
📝 Example
Selecting File Input Elements:
To select file input elements using the
:file
selector, you can use the following syntax:index.htmlCopied<input type="file" id="fileInput">
example.jsCopiedvar fileInput = $(":file");
This will select the file input element with the ID fileInput.
Checking File Input Values:
You can check if a file input has a selected file using the
:file
selector. For example:index.htmlCopied<input type="file" id="fileInput"> <button id="checkButton">Check File</button>
example.jsCopied$("#checkButton").click(function() { if ($("#fileInput").val() !== "") { alert("File selected!"); } else { alert("No file selected."); } });
This will alert "File selected!" if a file is selected in the file input, otherwise it will alert "No file selected."
Styling File Input Elements:
jQuery enables you to apply CSS styles to file input elements dynamically. Here's an example where we style the file input with a custom background color:
index.htmlCopied<input type="file" id="fileInput">
example.jsCopied$(":file").css("background-color", "lightgray");
This will set the background color of the file input to light gray.
Handling Events on File Input Elements:
You can bind events to file input elements using jQuery to perform actions based on user interactions. For instance, let's display the name of the selected file when it's changed:
index.htmlCopied<input type="file" id="fileInput"> <div id="fileName"></div>
example.jsCopied$("#fileInput").change(function() { var fileName = $(this).val().split("\\").pop(); $("#fileName").text("Selected file: " + fileName); });
This will update a <div> element with the ID fileName to display the name of the selected file whenever the file input's value changes.
🎉 Conclusion
The jQuery :file
selector provides a convenient way to interact with file input elements in web development. Whether you need to select file inputs, check their values, style them dynamically, or handle events related to file selection, this selector offers a straightforward solution.
By mastering its usage, you can enhance the functionality and user experience of your web applications that involve file uploads.
👨💻 Join our Community:
Author
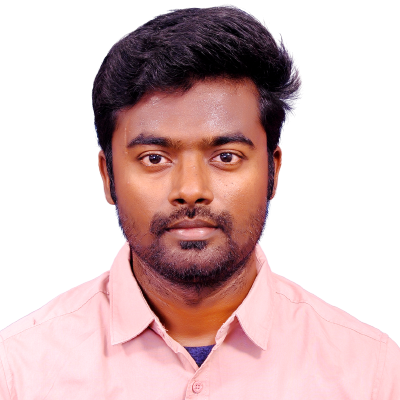
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery :file Selector), please comment here. I will help you immediately.