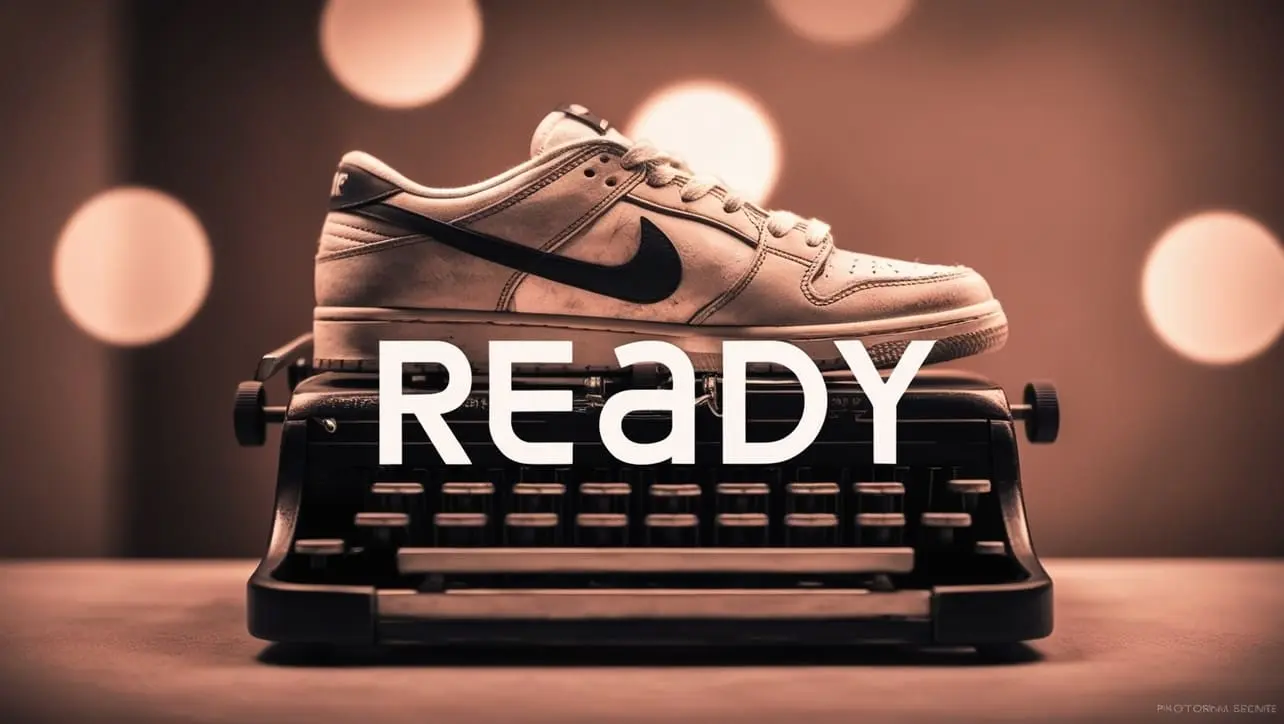
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery scroll Event
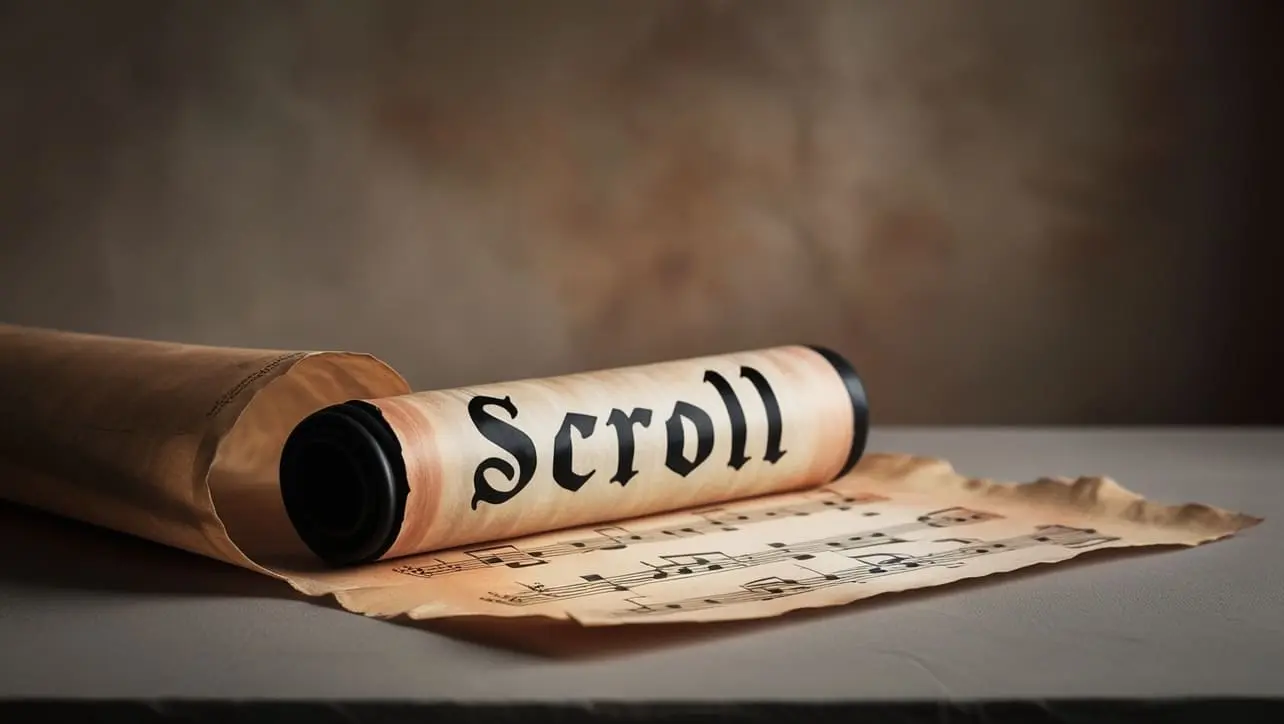
Photo Credit to CodeToFun
🙋 Introduction
Scrolling is a fundamental interaction in web browsing, and jQuery provides powerful tools to handle scroll
events seamlessly. While the .scroll() method is deprecated, the .on() method offers a modern and efficient alternative. Understanding and utilizing the jQuery scroll
event can greatly enhance the interactivity and user experience of your web pages.
In this guide, we'll explore the usage of the jQuery .on() method for scroll
events, accompanied by clear examples to facilitate understanding.
🧠 Understanding scroll Event
The .on() method in jQuery is versatile, allowing you to attach event handlers to elements, including scroll
events. By utilizing the .on() method with the scroll" event type, you can execute code in response to scrolling actions on the webpage.
💡 Syntax
The syntax for the scroll
event is straightforward:
$(selector).on("scroll", [, eventData], handler);
📝 Example
Detecting Scroll Events:
Suppose you want to trigger an alert message when the user scrolls the webpage. You can achieve this using the .on() method as follows:
example.jsCopied$(window).on("scroll", function() { alert("You're scrolling!"); });
This code will display an alert message every time the user scrolls the webpage.
Animating Elements on Scroll:
You can create captivating effects by animating elements as the user scrolls. For instance, let's fade in a div when it comes into view:
index.htmlCopied<div class="fade-in">Fade In On Scroll</div>
index.cssCopied.fade-in { opacity: 0; transition: opacity 0.5s ease; }
example.jsCopied$(window).on("scroll", function() { var scrollPos = $(window).scrollTop(); var windowHeight = $(window).height(); $(".fade-in").each(function() { var offsetTop = $(this).offset().top; if (scrollPos > offsetTop - windowHeight + 200) { $(this).css("opacity", "1"); } }); });
This code will fade in the div with the class fade-in when it comes 200 pixels into view from the bottom of the viewport.
Lazy Loading Images on Scroll:
Implementing lazy loading of images can significantly improve page load performance. Here's how you can load images as the user scrolls:
index.htmlCopied<img data-src="image.jpg" class="lazy-load" alt="Lazy Loaded Image">
example.jsCopied$(window).on("scroll", function() { $(".lazy-load").each(function() { if ($(this).offset().top < $(window).scrollTop() + $(window).height() && !$(this).attr("src")) { $(this).attr("src", $(this).data("src")); } }); });
This code will load images with the class lazy-load as they come into view during scrolling.
🎉 Conclusion
The jQuery .on() method provides a flexible and efficient approach to handle scroll
events in web development. Whether you need to execute code, create animations, or implement performance optimizations based on scrolling actions, this method offers a robust solution.
By mastering its usage, you can enhance the interactivity and user experience of your web pages effectively.
👨💻 Join our Community:
Author
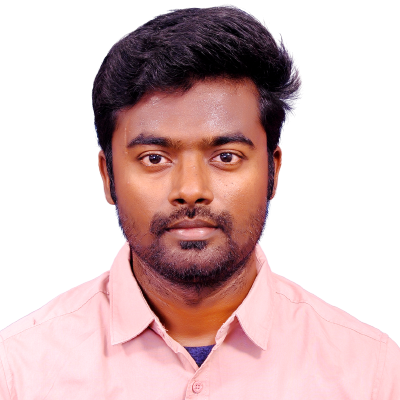
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery scroll Event), please comment here. I will help you immediately.