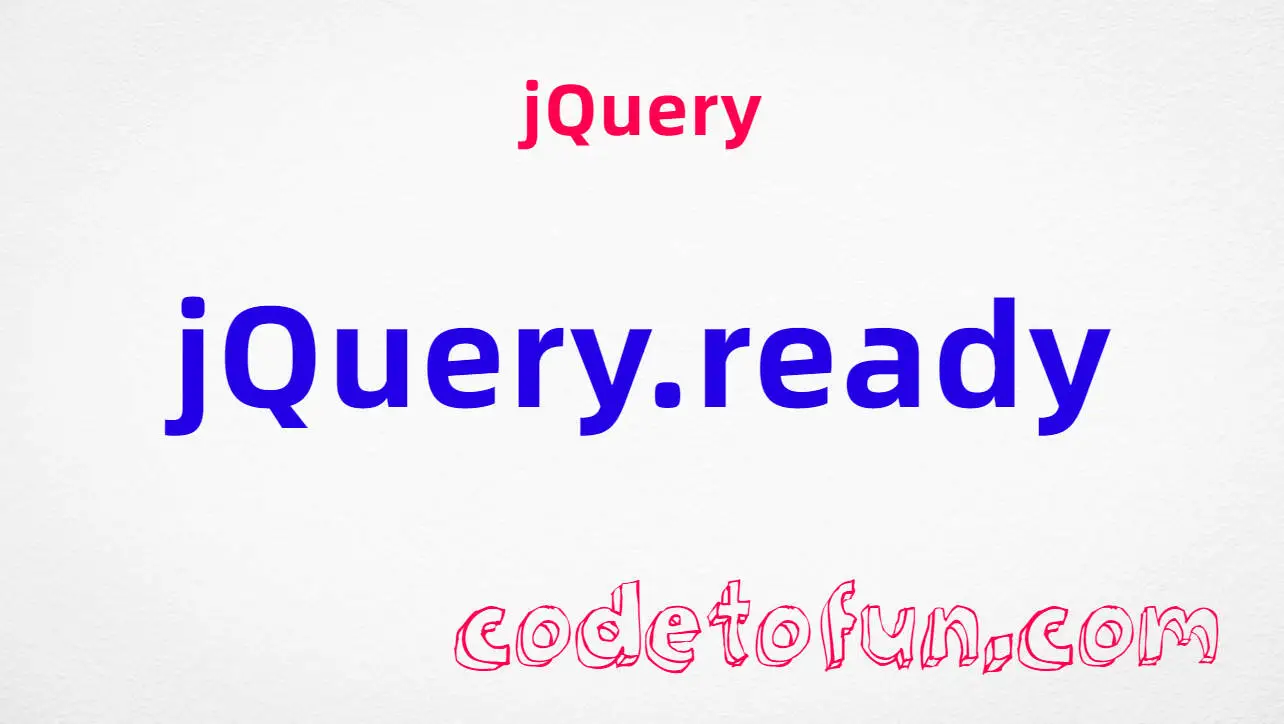
jQuery Basic
jQuery Deferred
- jQuery Deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
jQuery .promise() Method
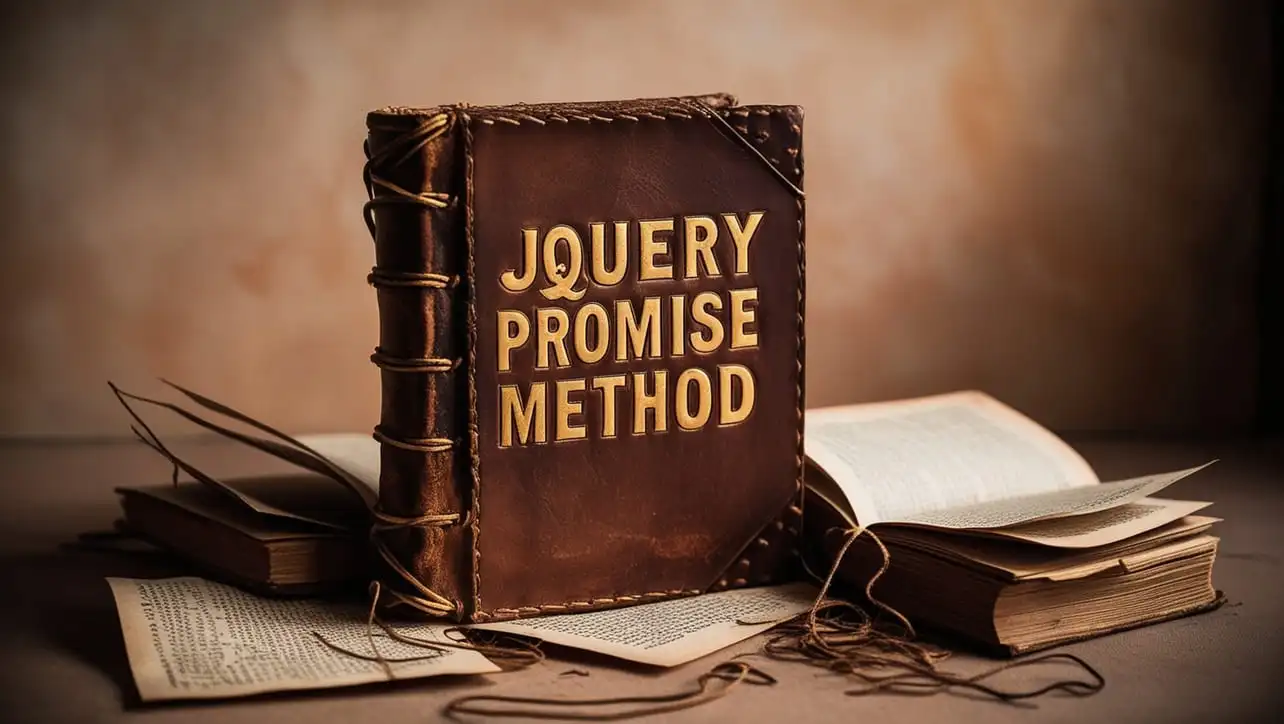
Photo Credit to CodeToFun
🙋 Introduction
In modern web development, asynchronous programming is ubiquitous, enabling dynamic and responsive user experiences. jQuery, a powerful JavaScript library, offers a range of utilities to manage asynchronous operations seamlessly. Among these, the .promise()
method stands out as a versatile tool for handling asynchronous events and synchronizing execution.
This guide will explore the intricacies of the .promise()
method, providing insights into its usage, syntax, and practical applications.
🧠 Understanding .promise() Method
The .promise()
method in jQuery allows developers to interact with the promises generated by animations and asynchronous function calls. It returns a Promise object that represents the state of the selected elements, enabling synchronization and coordination of asynchronous tasks.
💡 Syntax
The syntax for the .promise()
method is straightforward:
.promise( [type ] [, target ] )
- type (Optional): A string indicating the type of promise to return ('fx' for animations or a custom string).
- target (Optional): The target object for the promise (typically the selected element or a custom object).
📝 Example
Let's dive into a simple example to illustrate the usage of the .promise()
method:
$("button").click(function() {
$("div").fadeIn(1000).promise().done(function() {
$(this).addClass("highlight");
});
});
🏆 Best Practices
When working with the .promise()
method, consider the following best practices:
Chaining:
Take advantage of method chaining to streamline code and create expressive sequences of asynchronous operations.
Error Handling:
Incorporate error handling mechanisms, such as .fail() or .catch(), to manage exceptions and unexpected behavior gracefully.
Performance Optimization:
Optimize code execution by minimizing unnecessary promises and ensuring efficient resource utilization.
Promise Composition:
Utilize promise composition techniques, such as Promise.all() or Promise.race(), to coordinate multiple asynchronous tasks effectively.
Documentation:
Document usage patterns and conventions for
.promise()
within your codebase to facilitate collaboration and maintenance.
📚 Use Cases
Animation Sequencing:
Use
.promise()
to chain animations sequentially, ensuring one animation completes before starting the next.Event Handling:
Synchronize event handlers to execute only after specific animations or asynchronous operations are finished.
Custom Effects:
Implement custom effects or functionality that depend on the completion of asynchronous tasks, such as loading data from an external API.
Form Validation:
Validate form inputs asynchronously and handle submission events only after validation promises are resolved.
🎉 Conclusion
The .promise()
method in jQuery empowers developers to harness the full potential of asynchronous programming, enabling seamless coordination and synchronization of tasks in web applications.
By mastering its syntax, exploring practical use cases, and adhering to best practices, you can leverage .promise()
to create dynamic, responsive, and resilient user experiences. Incorporate this powerful tool into your toolkit to elevate the quality and performance of your jQuery-based projects.
👨💻 Join our Community:
Author
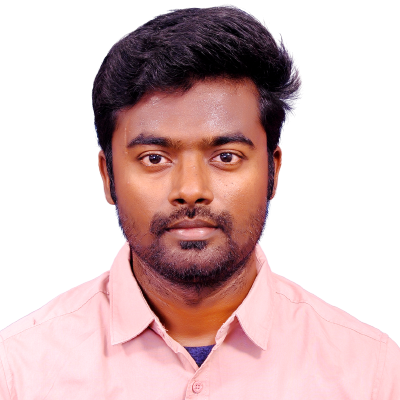
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .promise() Method), please comment here. I will help you immediately.