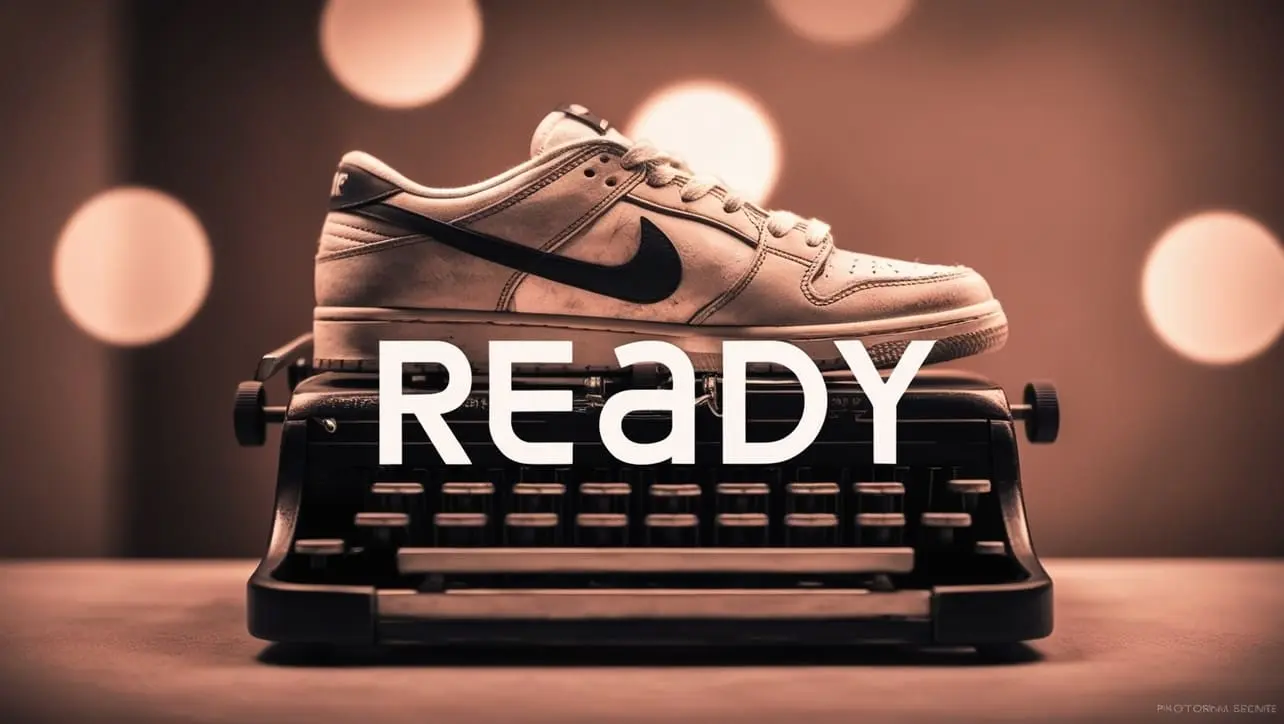
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery jQuery.noop() Method
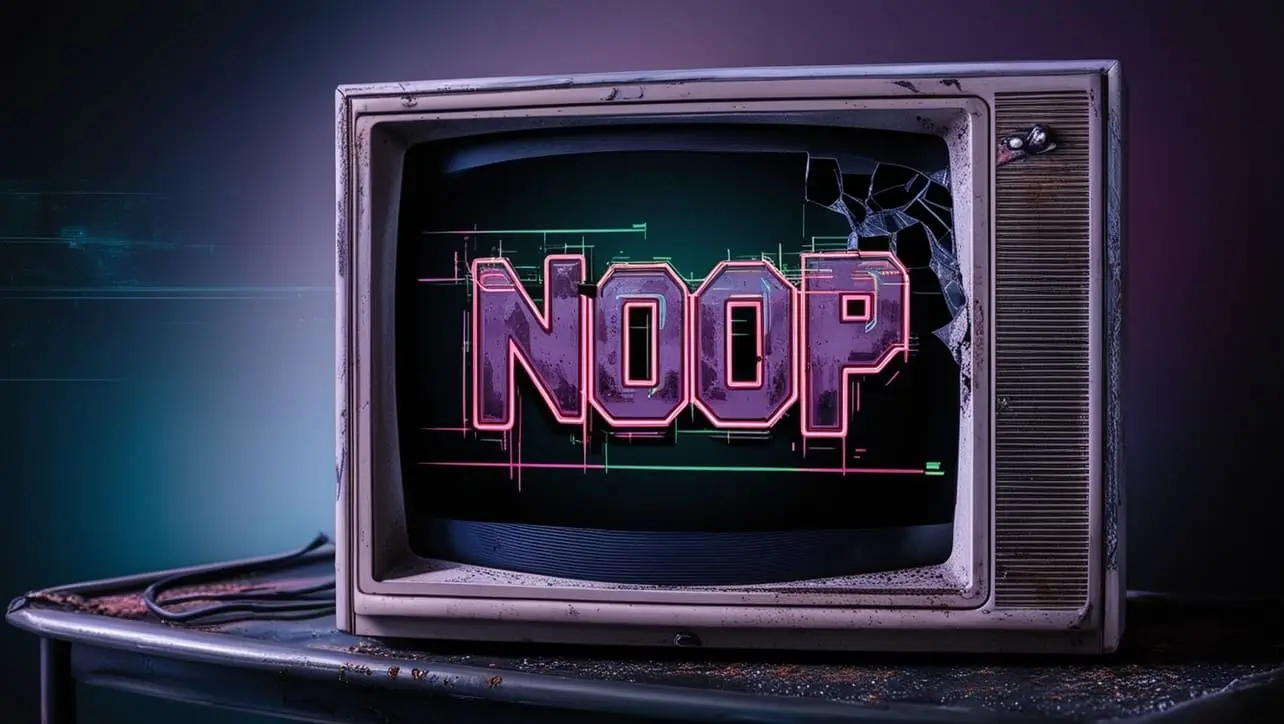
Photo Credit to CodeToFun
Introduction
jQuery is renowned for its simplicity and efficiency in handling JavaScript tasks. Among its arsenal of methods is jQuery.noop()
, a handy utility function that simplifies event handling and callback functions.
In this guide, we'll explore the purpose and usage of the jQuery.noop()
method with clear examples to illustrate its practical applications.
Understanding jQuery.noop() Method
The jQuery.noop()
method is a simple yet powerful utility provided by jQuery. It returns a function that does nothing. While this may seem trivial at first glance, it serves a crucial purpose in event handling and callback functions, especially when you need to provide a placeholder or default value for a function.
Syntax
The syntax for the jQuery.noop()
method is straightforward:
jQuery.noop()
Example
Using jQuery.noop() as a Placeholder:
Consider a scenario where you're defining event handlers but haven't yet implemented the functionality. You can use
jQuery.noop()
as a placeholder to indicate that the function will be defined later. For instance:example.jsCopied$("#myButton").click(jQuery.noop);
In this example, clicking #myButton will trigger no action until you define the functionality.
Default Callback Function in jQuery Methods:
Some jQuery methods accept callback functions as parameters. In cases where you don't want to provide a callback, but the method requires one, you can use
jQuery.noop()
as a default placeholder. For example:example.jsCopied$.ajax({ url: "example.com/data", success: jQuery.noop, error: function(xhr, status, error) { console.error("Error:", error); } });
Here, if the success callback isn't required,
jQuery.noop()
serves as a placeholder.Conditional Usage with jQuery.noop():
You can also conditionally use
jQuery.noop()
based on certain conditions. For instance:example.jsCopiedvar callback = someCondition ? actualFunction : jQuery.noop; callback();
In this case, callback will execute actualFunction if someCondition is true, otherwise, it will execute
jQuery.noop()
.
Conclusion
The jQuery.noop()
method may seem simple, but its utility in event handling and callback functions cannot be understated. Whether you need a placeholder for future functionality, a default callback in jQuery methods, or conditional execution, jQuery.noop()
provides a clean and concise solution.
By incorporating this method into your jQuery toolkit, you can streamline your code and improve its readability and maintainability.
Join our Community:
Author
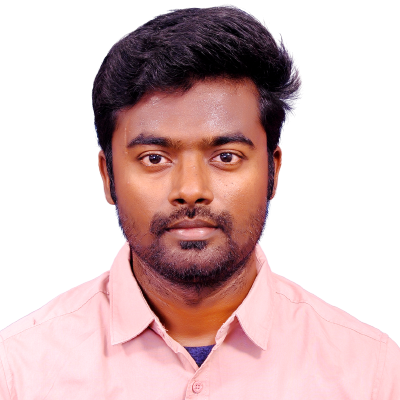
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.noop() Method), please comment here. I will help you immediately.