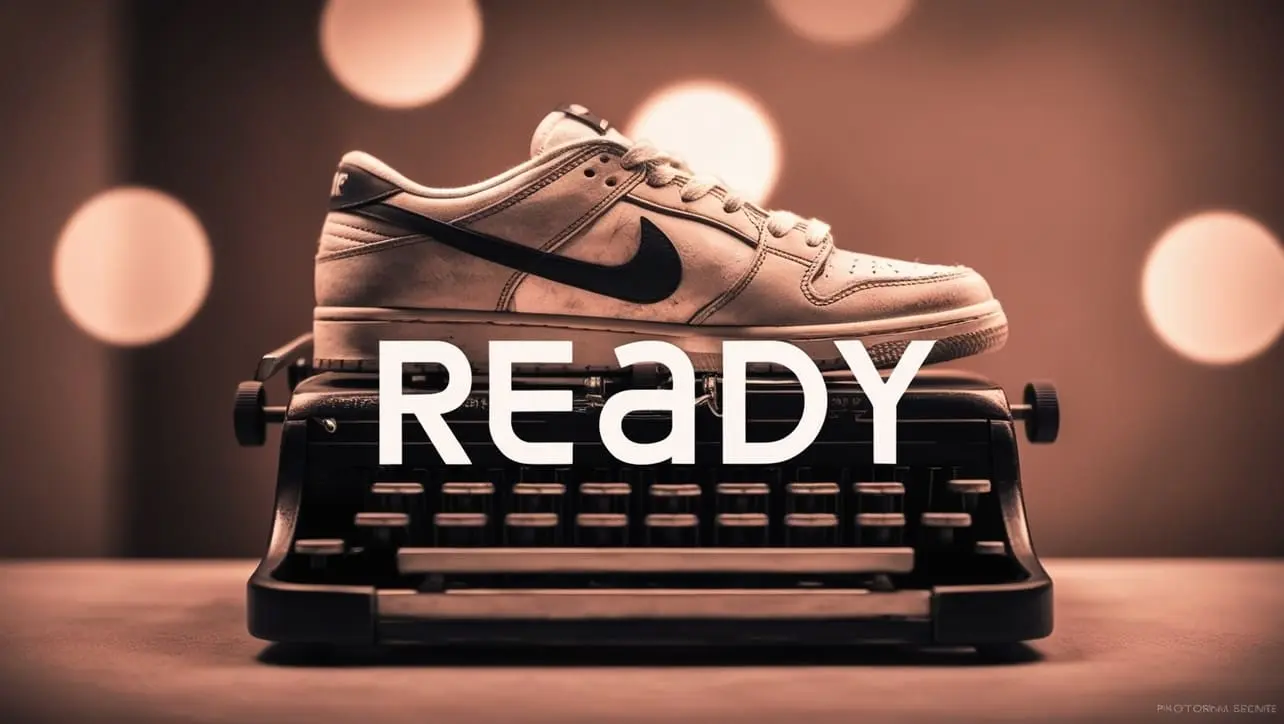
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery jQuery.noConflict() Method
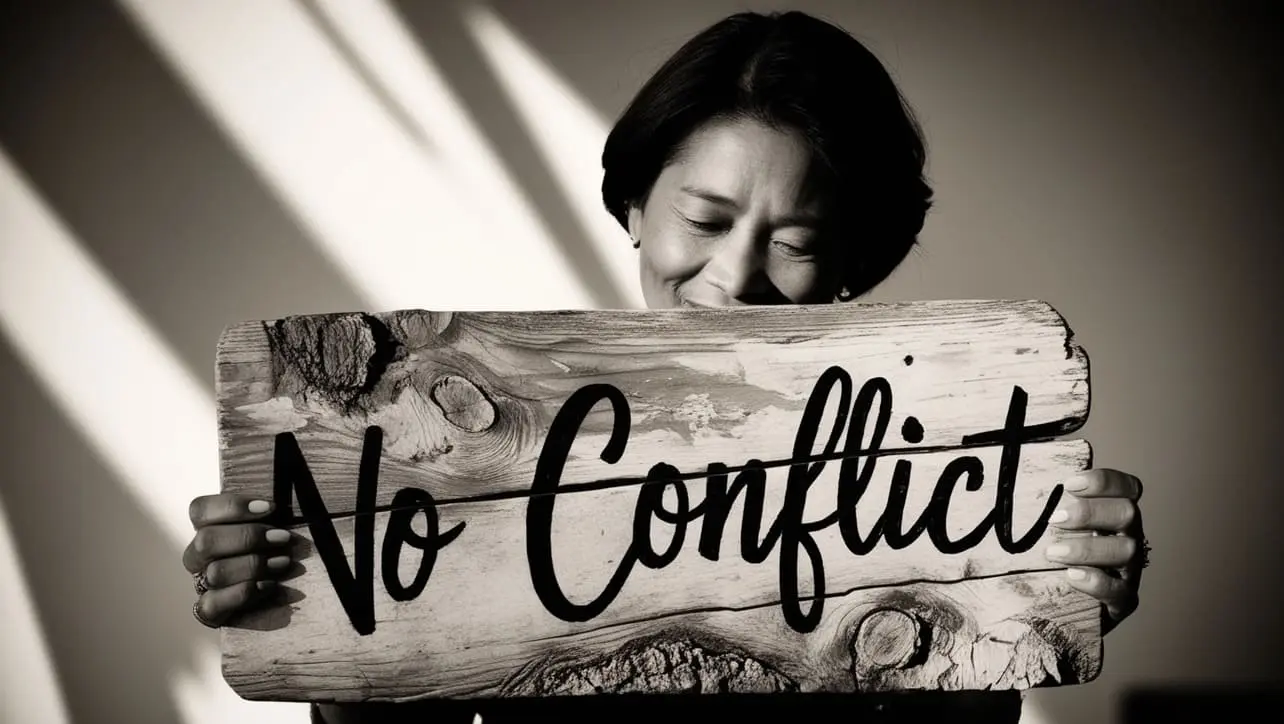
Photo Credit to CodeToFun
Introduction
In the realm of JavaScript libraries, conflicts can arise when multiple libraries use the same variable names or function names. jQuery addresses this issue with its jQuery.noConflict()
method. This method allows you to resolve conflicts between jQuery and other JavaScript libraries that use the $ symbol. Understanding how to use jQuery.noConflict()
is crucial for maintaining compatibility and avoiding conflicts in your web projects.
This guide will explore the jQuery.noConflict()
method in detail, providing clear examples and explanations for effective usage.
Understanding jQuery.noConflict() Method
The jQuery.noConflict()
method is designed to release control of the $ variable back to the original library that defined it, thus preventing conflicts with other libraries that may also use $. This method restores the value of $ to its original state before jQuery was loaded, ensuring compatibility with other libraries that rely on it.
Syntax
The syntax for the jQuery.noConflict()
method is straightforward:
jQuery.noConflict([removeAll]);
Parameters:
- removeAll (Optional): A Boolean value indicating whether to remove all jQuery variables from the global scope. Defaults to
false
.
Example
Basic Usage:
Suppose you have a webpage that uses both jQuery and another library that also uses the $ symbol. To prevent conflicts, you can use
jQuery.noConflict()
as follows:example.jsCopied<script src="other_library.js"></script> <script src="jquery.js"></script> <script> jQuery.noConflict(); // Now you can use jQuery with 'jQuery' instead of '$' jQuery(document).ready(function() { jQuery("button").click(function() { jQuery("p").text("jQuery is working!"); }); }); </script>
In this example,
jQuery.noConflict()
is called to relinquish control of the $ variable, allowing jQuery to be used with jQuery instead.Removing All jQuery Variables:
If you want to completely remove jQuery variables from the global scope, you can pass
true
as a parameter tojQuery.noConflict()
:example.jsCopiedjQuery.noConflict(true);
This will remove all references to jQuery, including jQuery and $, from the global scope.
Conclusion
The jQuery.noConflict()
method is a valuable tool for resolving conflicts between jQuery and other JavaScript libraries that use the $ symbol. By understanding how to use jQuery.noConflict()
effectively, you can ensure compatibility and smooth integration of jQuery into your web projects.
Whether you need to coexist with other libraries or simply maintain clean and organized code, jQuery.noConflict()
provides a reliable solution for managing variable conflicts.
Join our Community:
Author
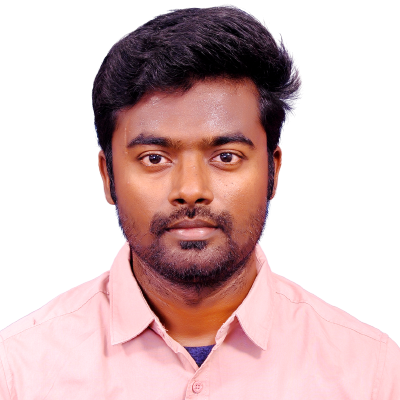
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.noConflict() Method), please comment here. I will help you immediately.