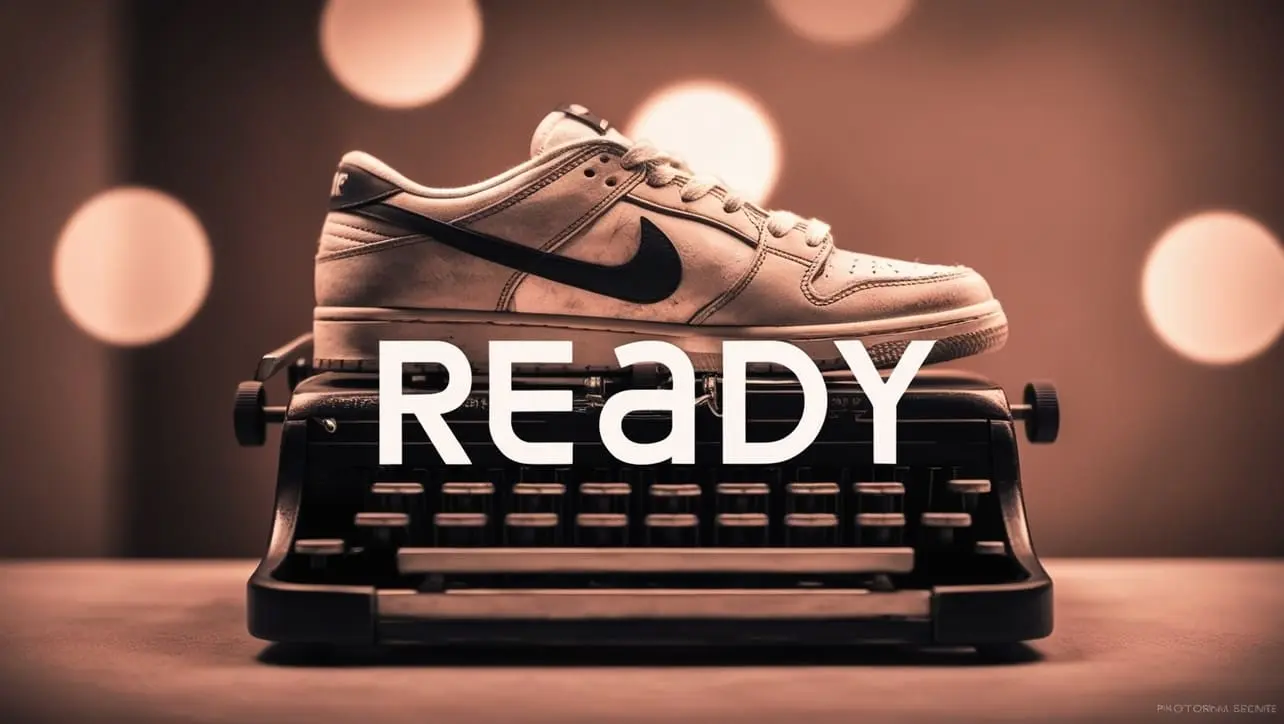
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .mousemove() Method
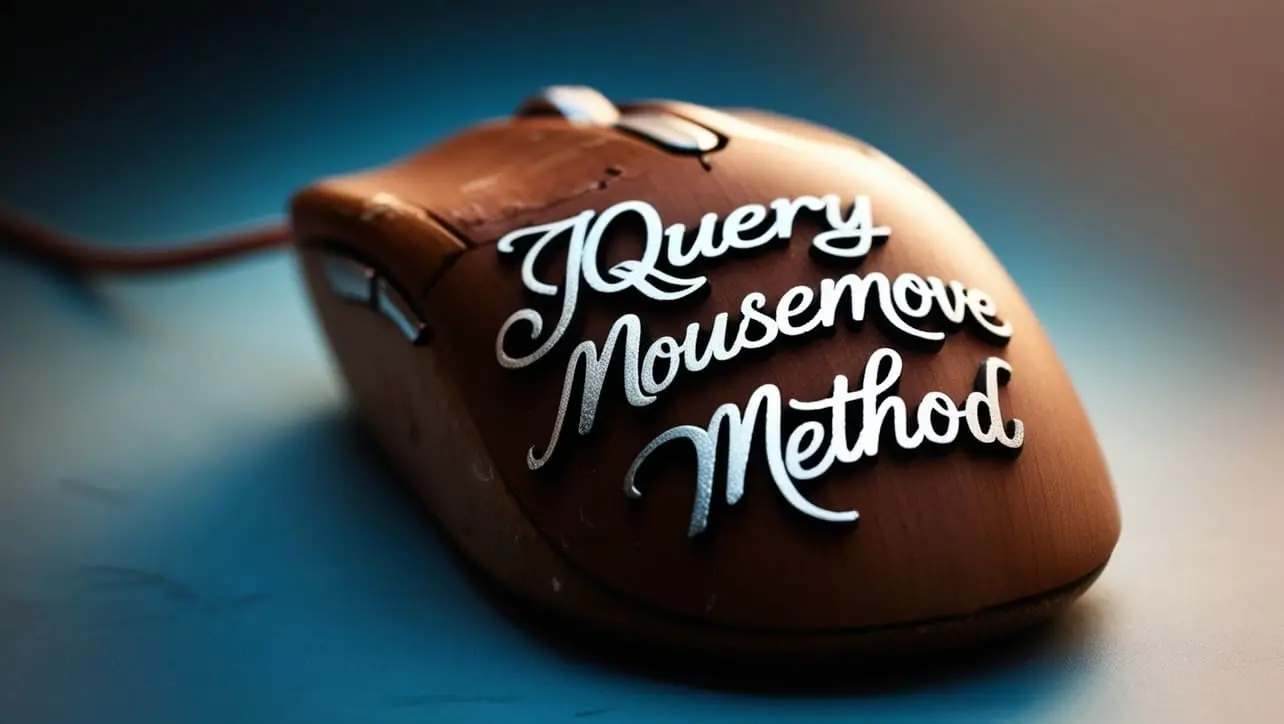
Photo Credit to CodeToFun
🙋 Introduction
jQuery is renowned for its ability to streamline web development tasks, and one of its most versatile methods is .mousemove()
. This method enables you to effortlessly capture mouse movement events, opening up a world of possibilities for creating interactive and dynamic web experiences.
In this comprehensive guide, we'll explore the ins and outs of the jQuery .mousemove()
method, providing clear examples to illustrate its potential.
🧠 Understanding .mousemove() Method
The .mousemove()
method in jQuery allows you to execute a function whenever the mouse pointer is moved over a selected element or the entire document. This makes it incredibly useful for tasks such as tracking user interactions, creating custom animations, or implementing drag-and-drop functionality.
💡 Syntax
The syntax for the .mousemove()
method is straightforward:
$(selector).mousemove(function(event) {
// Code to execute on mouse movement
});
📝 Example
Tracking Mouse Coordinates:
You can use
.mousemove()
to track the position of the mouse pointer on the screen. Here's a simple example:index.htmlCopied<div id="tracker" style="width: 200px; height: 200px; background-color: lightgray;"></div>
example.jsCopied$("#tracker").mousemove(function(event) { var x = event.pageX; var y = event.pageY; console.log("Mouse position - X: " + x + ", Y: " + y); });
This code will log the X and Y coordinates of the mouse pointer whenever it moves over the #tracker element.
Creating Interactive Effects:
You can leverage
.mousemove()
to create interactive effects based on mouse movement. For instance, let's change the background color of an element based on the mouse position:index.htmlCopied<div id="effect" style="width: 200px; height: 200px; background-color: lightblue;"></div>
example.jsCopied$("#effect").mousemove(function(event) { var x = event.pageX; var y = event.pageY; if (x < 100 && y < 100) { $(this).css("background-color", "lightgreen"); } else { $(this).css("background-color", "lightblue"); } });
In this example, the background color of the #effect div changes to light green when the mouse pointer is in the top-left quadrant, and reverts to light blue otherwise.
Implementing Drag-and-Drop Functionality:
You can also use
.mousemove()
in conjunction with other methods to implement drag-and-drop functionality. Here's a basic example:index.htmlCopied<div id="draggable" style="width: 100px; height: 100px; background-color: orange; position: absolute;"></div>
example.jsCopiedvar isDragging = false; $("#draggable").mousedown(function() { isDragging = true; }); $(document).mousemove(function(event) { if (isDragging) { var x = event.pageX; var y = event.pageY; $("#draggable").css({left: x, top: y}); } }); $(document).mouseup(function() { isDragging = false; });
This code allows you to drag the #draggable div around the document using mouse movement.
🎉 Conclusion
The jQuery .mousemove()
method is a powerful tool for capturing and responding to mouse movement events in web development. Whether you're tracking mouse coordinates, creating interactive effects, or implementing drag-and-drop functionality, this method provides a straightforward solution.
By mastering its usage, you can enhance the interactivity and usability of your web applications with ease.
👨💻 Join our Community:
Author
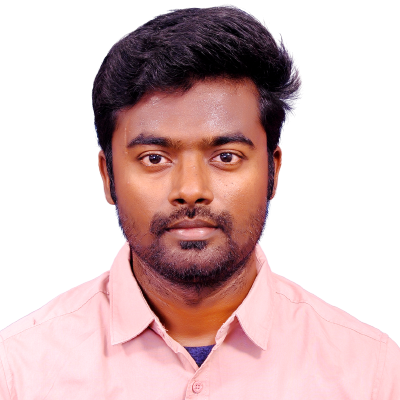
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .mousemove() Method), please comment here. I will help you immediately.