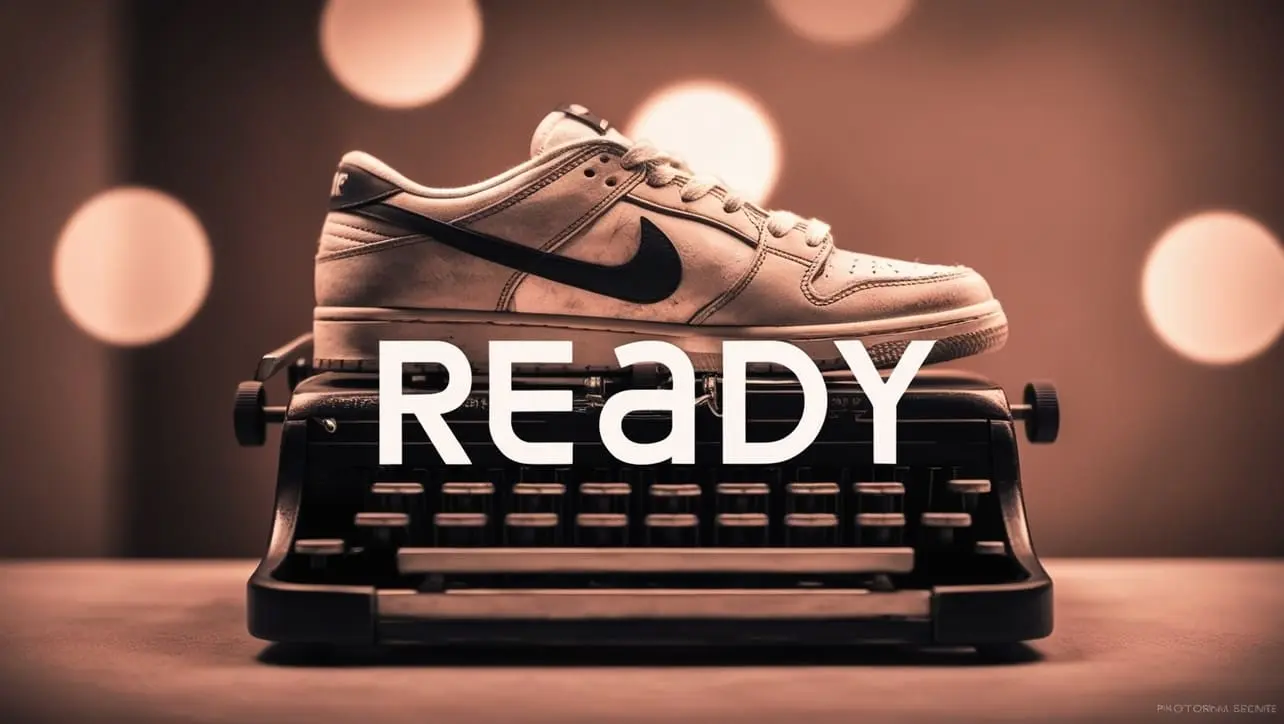
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .mouseenter() Method
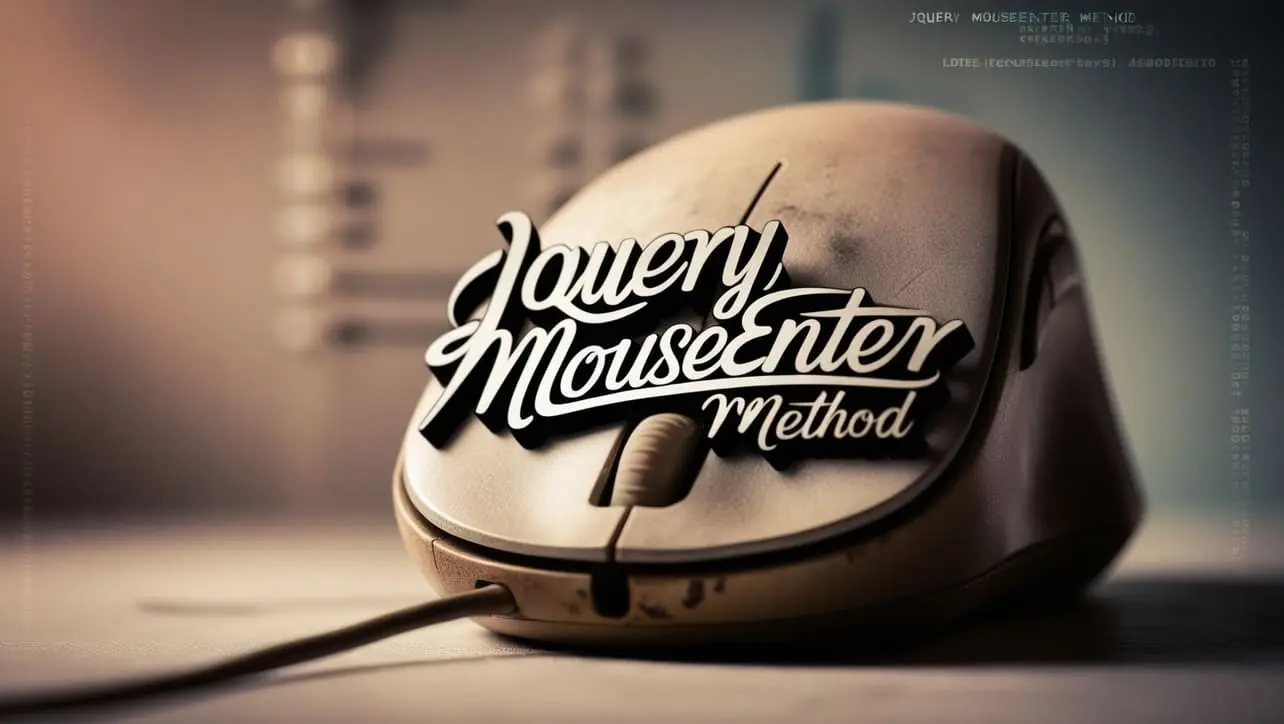
Photo Credit to CodeToFun
Introduction
jQuery offers a plethora of methods to enhance interactivity and user experience on websites. One such method is .mouseenter()
, which allows you to execute a function when the mouse pointer enters an element. This method is particularly useful for creating hover effects and triggering actions based on mouse interactions.
In this guide, we'll delve into the usage of the jQuery .mouseenter()
method with clear examples to illustrate its functionality.
Understanding .mouseenter() Method
The .mouseenter()
method in jQuery is used to bind an event handler function to the "mouseenter" JavaScript event, which is triggered when the mouse pointer enters the specified element.
Syntax
The syntax for the .mouseenter()
method is straightforward:
$(selector).mouseenter(function)
Example
Basic Usage:
Let's start with a basic example where we change the background color of a div when the mouse enters it:
index.htmlCopied<div id="hoverDiv" style="width: 200px; height: 200px; background-color: #f0f0f0;"></div>
example.jsCopied$("#hoverDiv").mouseenter(function() { $(this).css("background-color", "lightblue"); });
In this example, when the mouse enters the #hoverDiv, its background color changes to light blue.
Creating Hover Effects:
You can create more elaborate hover effects by combining
.mouseenter()
with other jQuery methods. For instance, let's change the text color of a paragraph when hovered over:index.htmlCopied<p id="hoverPara">Hover over me!</p>
example.jsCopied$("#hoverPara").mouseenter(function() { $(this).css("color", "red"); }).mouseleave(function() { $(this).css("color", "black"); });
Here, the text color of the paragraph turns red when hovered over and returns to black when the mouse leaves.
Applying Animations:
You can also apply animations using
.mouseenter()
to create more dynamic effects. For example, let's animate the width of a div when hovered over:index.htmlCopied<div id="animateDiv" style="width: 100px; height: 100px; background-color: #ccc;"></div>
example.jsCopied$("#animateDiv").mouseenter(function() { $(this).animate({ width: '200px' }, 300); }).mouseleave(function() { $(this).animate({ width: '100px' }, 300); });
In this example, the width of the #animateDiv expands when the mouse enters and shrinks back when it leaves, creating a smooth animation effect.
Conclusion
The jQuery .mouseenter()
method provides a convenient way to add interactivity to your website by executing functions when the mouse pointer enters specified elements. Whether you're creating simple hover effects, applying animations, or triggering actions based on mouse interactions, this method offers versatility and ease of implementation.
By mastering its usage, you can enhance user experience and engagement on your web pages effortlessly.
Join our Community:
Author
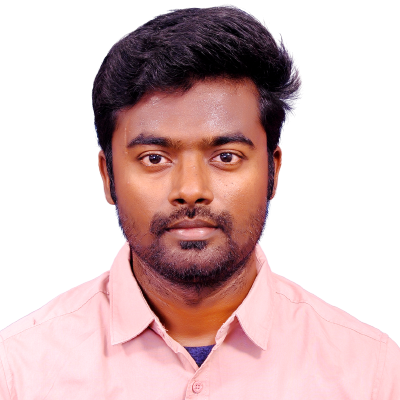
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .mouseenter() Method), please comment here. I will help you immediately.