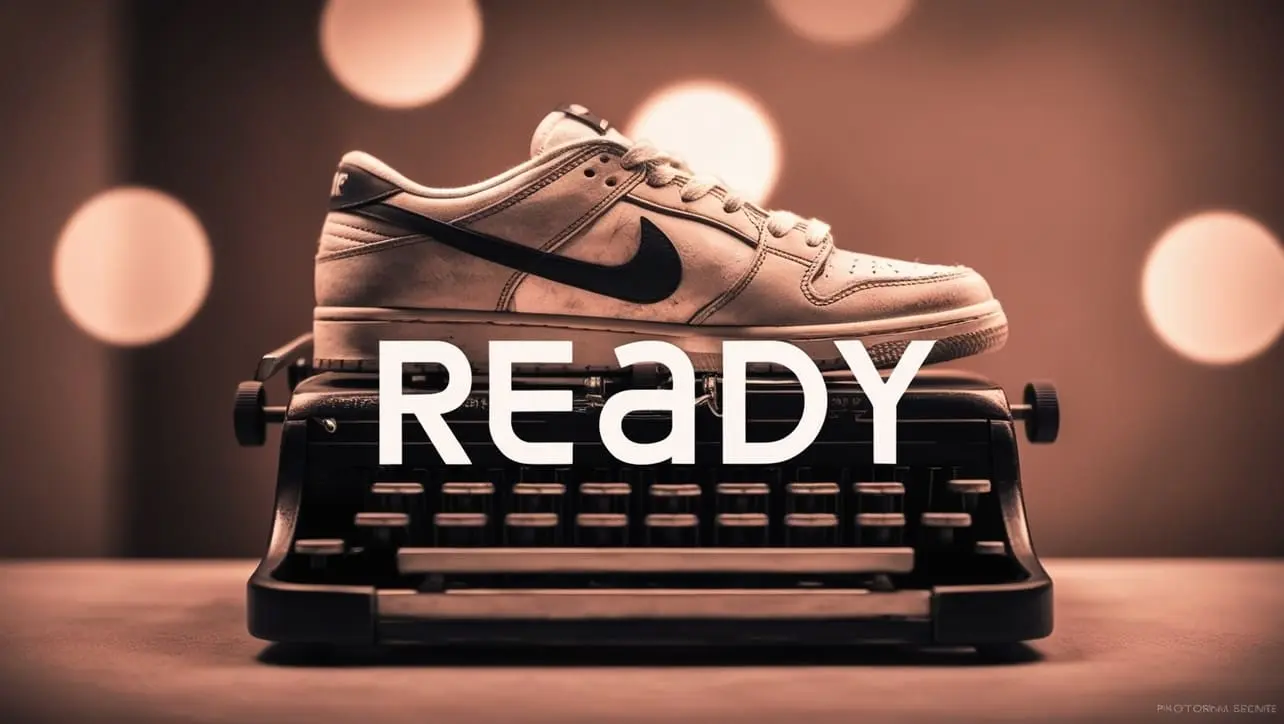
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery jQuery.trim() Method
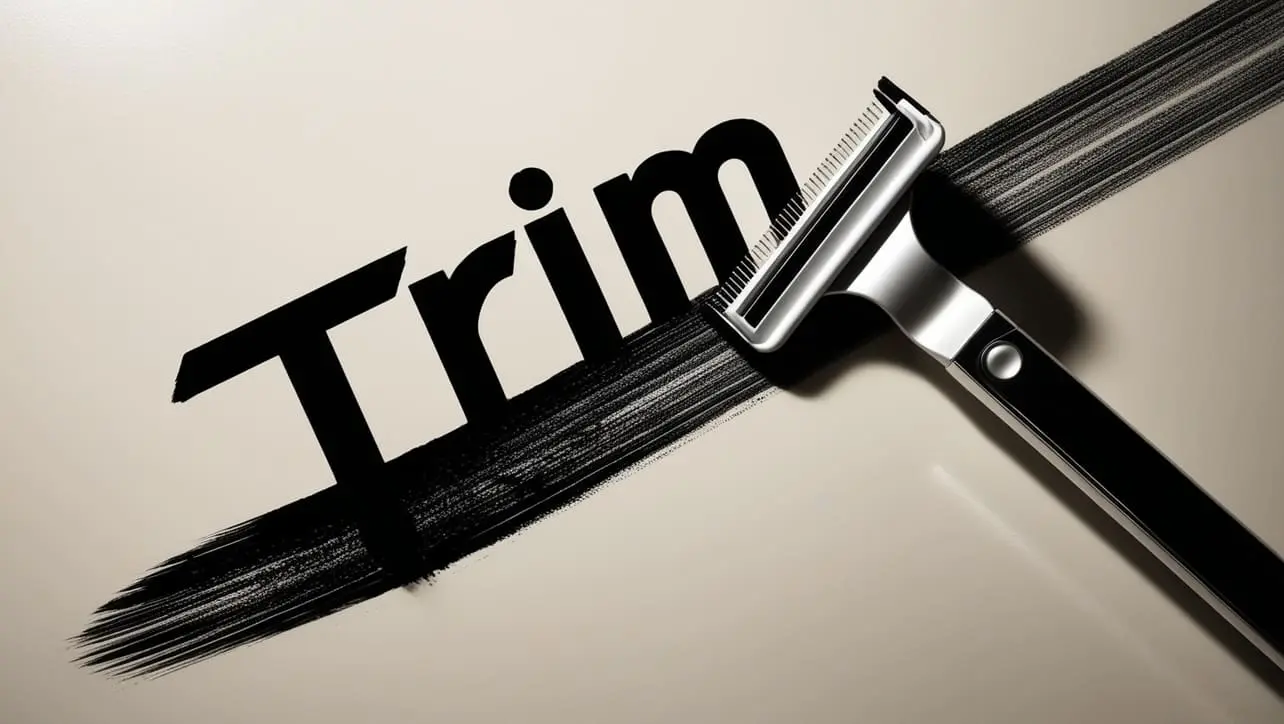
Photo Credit to CodeToFun
🙋 Introduction
In web development, managing and manipulating strings is a common task. jQuery simplifies this process with its trim()
method, which removes leading and trailing white spaces from a string. Understanding how to use this method effectively can improve the readability and efficiency of your code.
In this guide, we'll explore the jQuery trim()
method with clear examples to help you grasp its utility.
🧠 Understanding jQuery.trim() Method
The trim()
method in jQuery is used to remove white spaces (spaces, tabs, and newlines) from both ends of a string. It is particularly handy when dealing with user inputs or string manipulations where leading and trailing white spaces may cause issues.
💡 Syntax
The syntax for the jQuery.trim()
method is straightforward:
$.trim(str)
or
jQuery.trim(str)
📝 Example
Basic Usage:
Let's start with a simple example where we trim a string:
example.jsCopiedvar str = " Hello, world! "; var trimmedStr = $.trim(str); console.log(trimmedStr); // Output: "Hello, world!"
This removes the leading and trailing white spaces from the string str.
Trim User Input:
Often, user inputs may contain unintended white spaces. Here's how you can use
trim()
to clean up user inputs:index.htmlCopied<input type="text" id="userInput" placeholder="Enter your name"> <button id="submitBtn">Submit</button>
example.jsCopied$("#submitBtn").click(function() { var userInput = $("#userInput").val(); var trimmedInput = $.trim(userInput); console.log(trimmedInput); });
This will log the trimmed user input to the console when the submit button is clicked.
Checking for Empty Input:
You can also utilize
trim()
to check if a string is empty after removing white spaces:example.jsCopiedvar userInput = " "; if($.trim(userInput) === "") { console.log("Input is empty!"); } else { console.log("Input is not empty!"); }
This will log "Input is empty!" since the trimmed userInput results in an empty string.
Compatibility with Vanilla JavaScript:
Although jQuery provides its
trim()
method, you can achieve the same functionality using native JavaScript'strim()
method introduced in ECMAScript 5:example.jsCopiedvar str = " Hello, world! "; var trimmedStr = str.trim(); console.log(trimmedStr); // Output: "Hello, world!"
🎉 Conclusion
The jQuery trim()
method is a useful tool for removing leading and trailing white spaces from strings. Whether you're cleaning up user inputs, checking for empty strings, or simply manipulating strings, this method offers a straightforward solution.
By incorporating trim()
into your code, you can ensure cleaner and more efficient string handling in your web applications.
👨💻 Join our Community:
Author
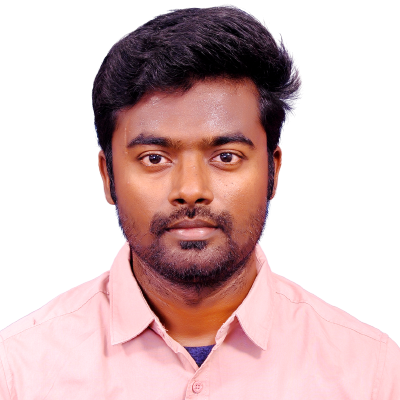
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.trim() Method), please comment here. I will help you immediately.